
- •Table of Contents
- •Preface
- •What is ASP.NET?
- •Installing the Required Software
- •Installing the Web Server
- •Installing Internet Information Services (IIS)
- •Installing Cassini
- •Installing the .NET Framework and the SDK
- •Installing the .NET Framework
- •Installing the SDK
- •Configuring the Web Server
- •Configuring IIS
- •Configuring Cassini
- •Where do I Put my Files?
- •Using localhost
- •Virtual Directories
- •Using Cassini
- •Installing SQL Server 2005 Express Edition
- •Installing SQL Server Management Studio Express
- •Installing Visual Web Developer 2005
- •Writing your First ASP.NET Page
- •Getting Help
- •Summary
- •ASP.NET Basics
- •ASP.NET Page Structure
- •Directives
- •Code Declaration Blocks
- •Comments in VB and C# Code
- •Code Render Blocks
- •ASP.NET Server Controls
- •Server-side Comments
- •Literal Text and HTML Tags
- •View State
- •Working with Directives
- •ASP.NET Languages
- •Visual Basic
- •Summary
- •VB and C# Programming Basics
- •Programming Basics
- •Control Events and Subroutines
- •Page Events
- •Variables and Variable Declaration
- •Arrays
- •Functions
- •Operators
- •Breaking Long Lines of Code
- •Conditional Logic
- •Loops
- •Object Oriented Programming Concepts
- •Objects and Classes
- •Properties
- •Methods
- •Classes
- •Constructors
- •Scope
- •Events
- •Understanding Inheritance
- •Objects In .NET
- •Namespaces
- •Using Code-behind Files
- •Summary
- •Constructing ASP.NET Web Pages
- •Web Forms
- •HTML Server Controls
- •Using the HTML Server Controls
- •Web Server Controls
- •Standard Web Server Controls
- •Label
- •Literal
- •TextBox
- •HiddenField
- •Button
- •ImageButton
- •LinkButton
- •HyperLink
- •CheckBox
- •RadioButton
- •Image
- •ImageMap
- •PlaceHolder
- •Panel
- •List Controls
- •DropDownList
- •ListBox
- •RadioButtonList
- •CheckBoxList
- •BulletedList
- •Advanced Controls
- •Calendar
- •AdRotator
- •TreeView
- •SiteMapPath
- •Menu
- •MultiView
- •Wizard
- •FileUpload
- •Web User Controls
- •Creating a Web User Control
- •Using the Web User Control
- •Master Pages
- •Using Cascading Style Sheets (CSS)
- •Types of Styles and Style Sheets
- •Style Properties
- •The CssClass Property
- •Summary
- •Building Web Applications
- •Introducing the Dorknozzle Project
- •Using Visual Web Developer
- •Meeting the Features
- •The Solution Explorer
- •The Web Forms Designer
- •The Code Editor
- •IntelliSense
- •The Toolbox
- •The Properties Window
- •Executing your Project
- •Using Visual Web Developer’s Built-in Web Server
- •Using IIS
- •Using IIS with Visual Web Developer
- •Core Web Application Features
- •Web.config
- •Global.asax
- •Using Application State
- •Working with User Sessions
- •Using the Cache Object
- •Using Cookies
- •Starting the Dorknozzle Project
- •Preparing the Sitemap
- •Using Themes, Skins, and Styles
- •Creating a New Theme Folder
- •Creating a New Style Sheet
- •Styling Web Server Controls
- •Adding a Skin
- •Applying the Theme
- •Building the Master Page
- •Using the Master Page
- •Extending Dorknozzle
- •Debugging and Error Handling
- •Debugging with Visual Web Developer
- •Other Kinds of Errors
- •Custom Errors
- •Handling Exceptions Locally
- •Summary
- •Using the Validation Controls
- •Enforcing Validation on the Server
- •Using Validation Controls
- •RequiredFieldValidator
- •CompareValidator
- •RangeValidator
- •ValidationSummary
- •RegularExpressionValidator
- •Some Useful Regular Expressions
- •CustomValidator
- •Validation Groups
- •Updating Dorknozzle
- •Summary
- •What is a Database?
- •Creating your First Database
- •Creating a New Database Using Visual Web Developer
- •Creating Database Tables
- •Data Types
- •Column Properties
- •Primary Keys
- •Creating the Employees Table
- •Creating the Remaining Tables
- •Executing SQL Scripts
- •Populating the Data Tables
- •Relational Database Design Concepts
- •Foreign Keys
- •Using Database Diagrams
- •Diagrams and Table Relationships
- •One-to-one Relationships
- •One-to-many Relationships
- •Many-to-many Relationships
- •Summary
- •Speaking SQL
- •Reading Data from a Single Table
- •Using the SELECT Statement
- •Selecting Certain Fields
- •Selecting Unique Data with DISTINCT
- •Row Filtering with WHERE
- •Selecting Ranges of Values with BETWEEN
- •Matching Patterns with LIKE
- •Using the IN Operator
- •Sorting Results Using ORDER BY
- •Limiting the Number of Results with TOP
- •Reading Data from Multiple Tables
- •Subqueries
- •Table Joins
- •Expressions and Operators
- •Transact-SQL Functions
- •Arithmetic Functions
- •String Functions
- •Date and Time Functions
- •Working with Groups of Values
- •The COUNT Function
- •Grouping Records Using GROUP BY
- •Filtering Groups Using HAVING
- •The SUM, AVG, MIN, and MAX Functions
- •Updating Existing Data
- •The INSERT Statement
- •The UPDATE Statement
- •The DELETE Statement
- •Stored Procedures
- •Summary
- •Introducing ADO.NET
- •Importing the SqlClient Namespace
- •Defining the Database Connection
- •Preparing the Command
- •Executing the Command
- •Setting up Database Authentication
- •Reading the Data
- •Using Parameters with Queries
- •Bulletproofing Data Access Code
- •Using the Repeater Control
- •More Data Binding
- •Inserting Records
- •Updating Records
- •Deleting Records
- •Using Stored Procedures
- •Summary
- •DataList Basics
- •Handling DataList Events
- •Editing DataList Items and Using Templates
- •DataList and Visual Web Developer
- •Styling the DataList
- •Summary
- •Using the GridView Control
- •Customizing the GridView Columns
- •Styling the GridView with Templates, Skins, and CSS
- •Selecting Grid Records
- •Using the DetailsView Control
- •Styling the DetailsView
- •GridView and DetailsView Events
- •Entering Edit Mode
- •Using Templates
- •Updating DetailsView Records
- •Summary
- •Advanced Data Access
- •Using Data Source Controls
- •Binding the GridView to a SqlDataSource
- •Binding the DetailsView to a SqlDataSource
- •Displaying Lists in DetailsView
- •More on SqlDataSource
- •Working with Data Sets and Data Tables
- •What is a Data Set Made From?
- •Binding DataSets to Controls
- •Implementing Paging
- •Storing Data Sets in View State
- •Implementing Sorting
- •Filtering Data
- •Updating a Database from a Modified DataSet
- •Summary
- •Security and User Authentication
- •Basic Security Guidelines
- •Securing ASP.NET 2.0 Applications
- •Working with Forms Authentication
- •Authenticating Users
- •Working with Hard-coded User Accounts
- •Configuring Forms Authentication
- •Configuring Forms Authorization
- •Storing Users in Web.config
- •Hashing Passwords
- •Logging Users Out
- •ASP.NET 2.0 Memberships and Roles
- •Creating the Membership Data Structures
- •Using your Database to Store Membership Data
- •Using the ASP.NET Web Site Configuration Tool
- •Creating Users and Roles
- •Changing Password Strength Requirements
- •Securing your Web Application
- •Using the ASP.NET Login Controls
- •Authenticating Users
- •Customizing User Display
- •Summary
- •Working with Files and Email
- •Writing and Reading Text Files
- •Setting Up Security
- •Writing Content to a Text File
- •Reading Content from a Text File
- •Accessing Directories and Directory Information
- •Working with Directory and File Paths
- •Uploading Files
- •Sending Email with ASP.NET
- •Configuring the SMTP Server
- •Sending a Test Email
- •Creating the Company Newsletter Page
- •Summary
- •The WebControl Class
- •Properties
- •Methods
- •Standard Web Controls
- •AdRotator
- •Properties
- •Events
- •BulletedList
- •Properties
- •Events
- •Button
- •Properties
- •Events
- •Calendar
- •Properties
- •Events
- •CheckBox
- •Properties
- •Events
- •CheckBoxList
- •Properties
- •Events
- •DropDownList
- •Properties
- •Events
- •FileUpload
- •Properties
- •Methods
- •HiddenField
- •Properties
- •HyperLink
- •Properties
- •Image
- •Properties
- •ImageButton
- •Properties
- •Events
- •ImageMap
- •Properties
- •Events
- •Label
- •Properties
- •LinkButton
- •Properties
- •Events
- •ListBox
- •Properties
- •Events
- •Literal
- •Properties
- •MultiView
- •Properties
- •Methods
- •Events
- •Panel
- •Properties
- •PlaceHolder
- •Properties
- •RadioButton
- •Properties
- •Events
- •RadioButtonList
- •Properties
- •Events
- •TextBox
- •Properties
- •Events
- •Properties
- •Validation Controls
- •CompareValidator
- •Properties
- •Methods
- •CustomValidator
- •Methods
- •Events
- •RangeValidator
- •Properties
- •Methods
- •RegularExpressionValidator
- •Properties
- •Methods
- •RequiredFieldValidator
- •Properties
- •Methods
- •ValidationSummary
- •Properties
- •Navigation Web Controls
- •SiteMapPath
- •Properties
- •Methods
- •Events
- •Menu
- •Properties
- •Methods
- •Events
- •TreeView
- •Properties
- •Methods
- •Events
- •HTML Server Controls
- •HtmlAnchor Control
- •Properties
- •Events
- •HtmlButton Control
- •Properties
- •Events
- •HtmlForm Control
- •Properties
- •HtmlGeneric Control
- •Properties
- •HtmlImage Control
- •Properties
- •HtmlInputButton Control
- •Properties
- •Events
- •HtmlInputCheckBox Control
- •Properties
- •Events
- •HtmlInputFile Control
- •Properties
- •HtmlInputHidden Control
- •Properties
- •HtmlInputImage Control
- •Properties
- •Events
- •HtmlInputRadioButton Control
- •Properties
- •Events
- •HtmlInputText Control
- •Properties
- •Events
- •HtmlSelect Control
- •Properties
- •Events
- •HtmlTable Control
- •Properties
- •HtmlTableCell Control
- •Properties
- •HtmlTableRow Control
- •Properties
- •HtmlTextArea Control
- •Properties
- •Events
- •Index

Chapter 11: Managing Content Using Grid View and Details View
File: SkinFile.skin (excerpt)
<asp:DetailsView runat="server" CssClass="GridMain" CellPadding="4" GridLines="None">
<RowStyle CssClass="GridRow" /> <HeaderStyle CssClass="GridHeader" />
</asp:DetailsView>
Here, we’ve defined a similar style to the GridView control, which will ensure that our page has a consistent appearance. Save your work, open AddressBook.aspx in the browser, and select an employee. You should see something similar to Figure 11.11.
We’re really making progress now. There’s only one problem—our employee records don’t include any addresses, and at this moment there’s no way to add any! Let’s take care of this.
GridView and DetailsView Events
In order to use the GridView and DetailsView controls effectively, we need to know how to handle their events. In this section, we’ll learn about the events raised by these controls, with an emphasis on the events that relate to editing and updating data, as our next goal will be to allow users to edit the employee details in the DetailsView.
Earlier, you learned how to respond to the user’s clicking of the Select button by handling the GridView’s SelectedIndexChanged event. Soon you’ll implement editing functionality, which you’ll achieve by adding an Edit button to the
DetailsView control. The editing features of the GridView and the DetailsView are very similar, so you can apply the same principles, and even almost the same code, to both of them.
Both the GridView and DetailsView controls support Edit command buttons, which will place Edit buttons in the control when the page loads. Once one of the Edit buttons has been clicked, the row (in case of GridView) or the entire form (in case of DetailsView) will become editable, and instead of an Edit button, users will see Update and Cancel buttons.
These features are pretty amazing, because you can achieve this “edit mode” without writing any HTML at all: the columns know how to render their editable modes by themselves. If you don’t like their default edit mode appearances, you can customize them using templates.
452

GridView and DetailsView Events
Before writing any code, let’s see what this edit mode looks like. Figure 11.12 shows a GridView control with one of its rows in edit mode.
Figure 11.12. GridView in edit mode
Figure 11.13 shows a DetailsView control in edit mode.
Figure 11.13. DetailsView in edit mode
453
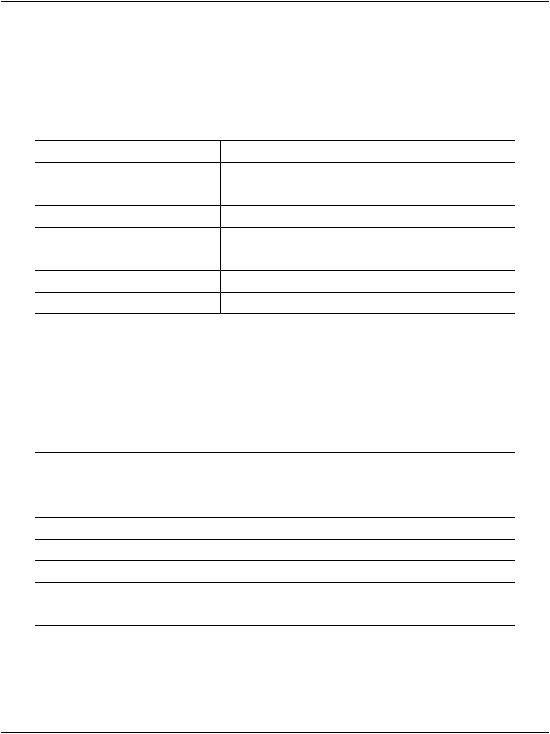
Chapter 11: Managing Content Using Grid View and Details View
When command buttons such as Edit are clicked, they raise events that we can handle on the server side. The GridView supports more kinds of command buttons, each of which triggers a certain event that we can handle. The action types and the events they trigger are listed in Table 11.1.
Table 11.1. GridView action types and the events they trigger
Action |
Events triggered when clicked |
Select |
SelectedIndexChanging, SelectIndexChanged |
Edit |
RowEditing |
Update |
RowUpdating, RowUpdated |
Cancel |
RowCancelingEdit |
Delete |
RowDeleting, RowDeleted |
(sorting buttons) |
RowSorting, RowSorted |
(custom action) |
RowCommand |
The DetailsView control, on the other hand, has buttons and events that refer to items, rather than rows, which makes sense when you realize that DetailsView is used to display the items in one record, while GridView displays a few items from many records. The DetailsView action types, and the events they generate, are listed in Table 11.2.
Table 11.2. DetailsView action types and the events they trigger
Action |
Events triggered when clicked |
(paging controls) |
PageIndexChanging, PageIndexChanged |
Delete |
ItemDeleting, ItemDeleted |
Insert |
ItemInserting, ItemInserted |
Edit |
ModeChanging, ModeChanged |
Update |
ItemUpdating, ItemUpdated |
Delete |
RowDeleting, RowDeleted |
(custom action) |
ItemCommand |
Notice that, except for the RowCommand (for the GridView) and the ItemCommand (for the DetailsView) events, we have events that are named in the present tense (i.e. those that end in “ing,” such as SelectedIndexChanging and ItemUpdating),
454
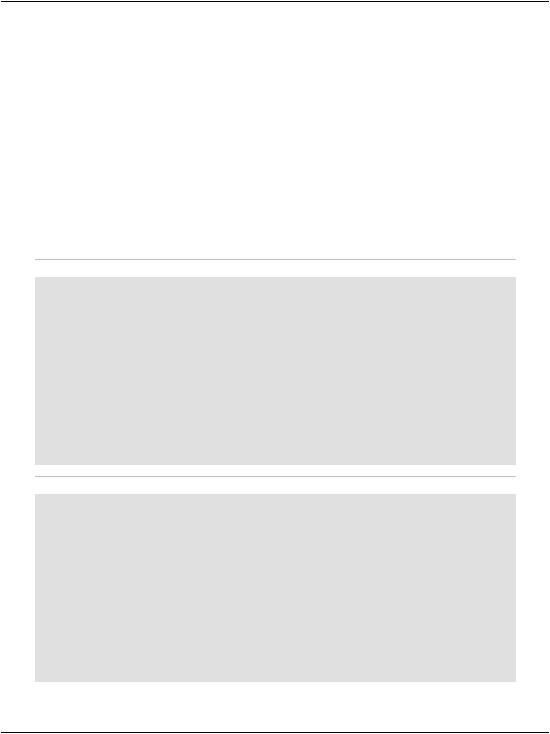
GridView and DetailsView Events
and events that are named in the past tense (i.e. those that end in “ed,” such as SelectIndexChanged and ItemUpdated). The events that end in “ing” are fired just before their past tense counterparts, and should be handled only if you want to implement some logic to determine whether the action in question should be performed.
The “ed” events, on the other hand, should perform the actual task of the button. We saw such an event handler when we handled the SelectIndexChanged event of our GridView control. In this handler, we queried the database to get the details of the selected employee, then displayed the result in a DetailsView control.
If we wanted to disallow the selection of a particular employee (say, the employee with the ID 1), we could do so by setting e.Cancel to False in the SelectIndexChanging event handler, as shown below:
Visual Basic
Protected Sub grid_SelectedIndexChanging(ByVal sender As Object, _ ByVal e As GridViewSelectEventArgs) _
Handles grid.SelectedIndexChanging
' Obtain the index of the selected row
Dim selectedRowIndex As Integer = grid.SelectedIndex ' Read the employee ID
Dim employeeId As Integer = _ grid.DataKeys(selectedRowIndex).Value
' Cancel the selection if Employee #1 was selected If employeeId = 1 Then
e.Cancel = False End If
End Sub
C#
protected void grid_SelectedIndexChanging(object sender, GridViewSelectEventArgs e)
{
//Obtain the index of the selected row int selectedRowIndex = grid.SelectedIndex;
//Read the employee ID
int employeeId = (int)grid.DataKeys[selectedRowIndex].Value; // Cancel the selection if Employee #1 was selected
if (employeeId == 1)
{
e.Cancel = false;
}
}
455