
- •Table of Contents
- •Preface
- •What is ASP.NET?
- •Installing the Required Software
- •Installing the Web Server
- •Installing Internet Information Services (IIS)
- •Installing Cassini
- •Installing the .NET Framework and the SDK
- •Installing the .NET Framework
- •Installing the SDK
- •Configuring the Web Server
- •Configuring IIS
- •Configuring Cassini
- •Where do I Put my Files?
- •Using localhost
- •Virtual Directories
- •Using Cassini
- •Installing SQL Server 2005 Express Edition
- •Installing SQL Server Management Studio Express
- •Installing Visual Web Developer 2005
- •Writing your First ASP.NET Page
- •Getting Help
- •Summary
- •ASP.NET Basics
- •ASP.NET Page Structure
- •Directives
- •Code Declaration Blocks
- •Comments in VB and C# Code
- •Code Render Blocks
- •ASP.NET Server Controls
- •Server-side Comments
- •Literal Text and HTML Tags
- •View State
- •Working with Directives
- •ASP.NET Languages
- •Visual Basic
- •Summary
- •VB and C# Programming Basics
- •Programming Basics
- •Control Events and Subroutines
- •Page Events
- •Variables and Variable Declaration
- •Arrays
- •Functions
- •Operators
- •Breaking Long Lines of Code
- •Conditional Logic
- •Loops
- •Object Oriented Programming Concepts
- •Objects and Classes
- •Properties
- •Methods
- •Classes
- •Constructors
- •Scope
- •Events
- •Understanding Inheritance
- •Objects In .NET
- •Namespaces
- •Using Code-behind Files
- •Summary
- •Constructing ASP.NET Web Pages
- •Web Forms
- •HTML Server Controls
- •Using the HTML Server Controls
- •Web Server Controls
- •Standard Web Server Controls
- •Label
- •Literal
- •TextBox
- •HiddenField
- •Button
- •ImageButton
- •LinkButton
- •HyperLink
- •CheckBox
- •RadioButton
- •Image
- •ImageMap
- •PlaceHolder
- •Panel
- •List Controls
- •DropDownList
- •ListBox
- •RadioButtonList
- •CheckBoxList
- •BulletedList
- •Advanced Controls
- •Calendar
- •AdRotator
- •TreeView
- •SiteMapPath
- •Menu
- •MultiView
- •Wizard
- •FileUpload
- •Web User Controls
- •Creating a Web User Control
- •Using the Web User Control
- •Master Pages
- •Using Cascading Style Sheets (CSS)
- •Types of Styles and Style Sheets
- •Style Properties
- •The CssClass Property
- •Summary
- •Building Web Applications
- •Introducing the Dorknozzle Project
- •Using Visual Web Developer
- •Meeting the Features
- •The Solution Explorer
- •The Web Forms Designer
- •The Code Editor
- •IntelliSense
- •The Toolbox
- •The Properties Window
- •Executing your Project
- •Using Visual Web Developer’s Built-in Web Server
- •Using IIS
- •Using IIS with Visual Web Developer
- •Core Web Application Features
- •Web.config
- •Global.asax
- •Using Application State
- •Working with User Sessions
- •Using the Cache Object
- •Using Cookies
- •Starting the Dorknozzle Project
- •Preparing the Sitemap
- •Using Themes, Skins, and Styles
- •Creating a New Theme Folder
- •Creating a New Style Sheet
- •Styling Web Server Controls
- •Adding a Skin
- •Applying the Theme
- •Building the Master Page
- •Using the Master Page
- •Extending Dorknozzle
- •Debugging and Error Handling
- •Debugging with Visual Web Developer
- •Other Kinds of Errors
- •Custom Errors
- •Handling Exceptions Locally
- •Summary
- •Using the Validation Controls
- •Enforcing Validation on the Server
- •Using Validation Controls
- •RequiredFieldValidator
- •CompareValidator
- •RangeValidator
- •ValidationSummary
- •RegularExpressionValidator
- •Some Useful Regular Expressions
- •CustomValidator
- •Validation Groups
- •Updating Dorknozzle
- •Summary
- •What is a Database?
- •Creating your First Database
- •Creating a New Database Using Visual Web Developer
- •Creating Database Tables
- •Data Types
- •Column Properties
- •Primary Keys
- •Creating the Employees Table
- •Creating the Remaining Tables
- •Executing SQL Scripts
- •Populating the Data Tables
- •Relational Database Design Concepts
- •Foreign Keys
- •Using Database Diagrams
- •Diagrams and Table Relationships
- •One-to-one Relationships
- •One-to-many Relationships
- •Many-to-many Relationships
- •Summary
- •Speaking SQL
- •Reading Data from a Single Table
- •Using the SELECT Statement
- •Selecting Certain Fields
- •Selecting Unique Data with DISTINCT
- •Row Filtering with WHERE
- •Selecting Ranges of Values with BETWEEN
- •Matching Patterns with LIKE
- •Using the IN Operator
- •Sorting Results Using ORDER BY
- •Limiting the Number of Results with TOP
- •Reading Data from Multiple Tables
- •Subqueries
- •Table Joins
- •Expressions and Operators
- •Transact-SQL Functions
- •Arithmetic Functions
- •String Functions
- •Date and Time Functions
- •Working with Groups of Values
- •The COUNT Function
- •Grouping Records Using GROUP BY
- •Filtering Groups Using HAVING
- •The SUM, AVG, MIN, and MAX Functions
- •Updating Existing Data
- •The INSERT Statement
- •The UPDATE Statement
- •The DELETE Statement
- •Stored Procedures
- •Summary
- •Introducing ADO.NET
- •Importing the SqlClient Namespace
- •Defining the Database Connection
- •Preparing the Command
- •Executing the Command
- •Setting up Database Authentication
- •Reading the Data
- •Using Parameters with Queries
- •Bulletproofing Data Access Code
- •Using the Repeater Control
- •More Data Binding
- •Inserting Records
- •Updating Records
- •Deleting Records
- •Using Stored Procedures
- •Summary
- •DataList Basics
- •Handling DataList Events
- •Editing DataList Items and Using Templates
- •DataList and Visual Web Developer
- •Styling the DataList
- •Summary
- •Using the GridView Control
- •Customizing the GridView Columns
- •Styling the GridView with Templates, Skins, and CSS
- •Selecting Grid Records
- •Using the DetailsView Control
- •Styling the DetailsView
- •GridView and DetailsView Events
- •Entering Edit Mode
- •Using Templates
- •Updating DetailsView Records
- •Summary
- •Advanced Data Access
- •Using Data Source Controls
- •Binding the GridView to a SqlDataSource
- •Binding the DetailsView to a SqlDataSource
- •Displaying Lists in DetailsView
- •More on SqlDataSource
- •Working with Data Sets and Data Tables
- •What is a Data Set Made From?
- •Binding DataSets to Controls
- •Implementing Paging
- •Storing Data Sets in View State
- •Implementing Sorting
- •Filtering Data
- •Updating a Database from a Modified DataSet
- •Summary
- •Security and User Authentication
- •Basic Security Guidelines
- •Securing ASP.NET 2.0 Applications
- •Working with Forms Authentication
- •Authenticating Users
- •Working with Hard-coded User Accounts
- •Configuring Forms Authentication
- •Configuring Forms Authorization
- •Storing Users in Web.config
- •Hashing Passwords
- •Logging Users Out
- •ASP.NET 2.0 Memberships and Roles
- •Creating the Membership Data Structures
- •Using your Database to Store Membership Data
- •Using the ASP.NET Web Site Configuration Tool
- •Creating Users and Roles
- •Changing Password Strength Requirements
- •Securing your Web Application
- •Using the ASP.NET Login Controls
- •Authenticating Users
- •Customizing User Display
- •Summary
- •Working with Files and Email
- •Writing and Reading Text Files
- •Setting Up Security
- •Writing Content to a Text File
- •Reading Content from a Text File
- •Accessing Directories and Directory Information
- •Working with Directory and File Paths
- •Uploading Files
- •Sending Email with ASP.NET
- •Configuring the SMTP Server
- •Sending a Test Email
- •Creating the Company Newsletter Page
- •Summary
- •The WebControl Class
- •Properties
- •Methods
- •Standard Web Controls
- •AdRotator
- •Properties
- •Events
- •BulletedList
- •Properties
- •Events
- •Button
- •Properties
- •Events
- •Calendar
- •Properties
- •Events
- •CheckBox
- •Properties
- •Events
- •CheckBoxList
- •Properties
- •Events
- •DropDownList
- •Properties
- •Events
- •FileUpload
- •Properties
- •Methods
- •HiddenField
- •Properties
- •HyperLink
- •Properties
- •Image
- •Properties
- •ImageButton
- •Properties
- •Events
- •ImageMap
- •Properties
- •Events
- •Label
- •Properties
- •LinkButton
- •Properties
- •Events
- •ListBox
- •Properties
- •Events
- •Literal
- •Properties
- •MultiView
- •Properties
- •Methods
- •Events
- •Panel
- •Properties
- •PlaceHolder
- •Properties
- •RadioButton
- •Properties
- •Events
- •RadioButtonList
- •Properties
- •Events
- •TextBox
- •Properties
- •Events
- •Properties
- •Validation Controls
- •CompareValidator
- •Properties
- •Methods
- •CustomValidator
- •Methods
- •Events
- •RangeValidator
- •Properties
- •Methods
- •RegularExpressionValidator
- •Properties
- •Methods
- •RequiredFieldValidator
- •Properties
- •Methods
- •ValidationSummary
- •Properties
- •Navigation Web Controls
- •SiteMapPath
- •Properties
- •Methods
- •Events
- •Menu
- •Properties
- •Methods
- •Events
- •TreeView
- •Properties
- •Methods
- •Events
- •HTML Server Controls
- •HtmlAnchor Control
- •Properties
- •Events
- •HtmlButton Control
- •Properties
- •Events
- •HtmlForm Control
- •Properties
- •HtmlGeneric Control
- •Properties
- •HtmlImage Control
- •Properties
- •HtmlInputButton Control
- •Properties
- •Events
- •HtmlInputCheckBox Control
- •Properties
- •Events
- •HtmlInputFile Control
- •Properties
- •HtmlInputHidden Control
- •Properties
- •HtmlInputImage Control
- •Properties
- •Events
- •HtmlInputRadioButton Control
- •Properties
- •Events
- •HtmlInputText Control
- •Properties
- •Events
- •HtmlSelect Control
- •Properties
- •Events
- •HtmlTable Control
- •Properties
- •HtmlTableCell Control
- •Properties
- •HtmlTableRow Control
- •Properties
- •HtmlTextArea Control
- •Properties
- •Events
- •Index

Chapter 11: Managing Content Using Grid View and Details View
the table data cells. The DataList makes things a bit easier by generating a singlecolumn table for us, but even so, the built-in functionality isn’t overwhelming.
Here, we’ll learn about two more controls that offer much more functionality and make it very easy for you to create a table: GridView and DetailsView. These controls, which form part of ASP.NET’s set of data controls, give developers much more power and flexibility over presentation, as you’ll see in the next few sections. The processes by which information within a table is presented, formatted, and edited have been completely revamped with the GridView and
DetailsView controls.
GridView vs DataGrid
The GridView control supersedes the DataGrid control, which was the star of all data-displaying controls in ASP.NET 1.0 and 1.1. The DataGrid is still present in ASP.NET 2.0 for backwards-compatibility purposes, but for any new development, the GridView is recommended. The DetailsView control is new in ASP.NET 2.0 and doesn’t have a corresponding control in earlier versions.
Using the GridView Control
The GridView control solves a problem that has plagued developers for years: data presentation. The GridView control generates simple HTML tables, so information within a GridView is presented to the end user in a familiar, cleanly formatted, tabular structure. Similar to the Repeater control, the GridView can also automatically display all the content contained in an SqlDataReader on a page, based on styles we set within its templates. However, unlike the Repeater control, the GridView offers several more advanced features, such as sorting or paging (i.e. splitting a large result set into pages), and makes it easy to modify the data in the underlying data source.
To sum up, GridView controls provide the following functionality:
database table-like presentation
table headers and footers
paging
sorting
428

Using the GridView Control
style modification through templates
customizable columns for advanced editing
You’ll learn about some of these features in this chapter, though we’ll leave the more advanced ones (sorting, paging, and editing) for the next chapter.
As with any other ASP.NET server control, GridView controls are added to the page using a specific element:
<asp:GridView id="myGridView" runat="server" />
Once we add the GridView to the page, we can bind an SqlDataReader to it as follows:
Visual Basic
myGridView.DataSource = myDataReader myGridView.DataBind()
C#
myGridView.DataSource = myDataReader; myGridView.DataBind();
So far, the GridView doesn’t seem to function very differently from the Repeater control, right? Think again! The Repeater control didn’t work unless we specified content within the required <ItemTemplate> and </ItemTemplate> tags. The GridView takes the structure of the database table automatically, and presents the data to the user in a cleanly formatted HTML table.
Let’s take a look at GridView in action as we develop the Dorknozzle intranet’s address book page. Start by opening the Dorknozzle project, if it’s not already open, and creating a new web form named AddressBook.aspx, based on the Dorknozzle.master master page. Also, make sure the new web form uses a codebehind file.
Now, open AddressBook.aspx, and complete its code as shown in the following code snippet.
File: AddressBook.aspx (excerpt)
<%@ Page Language="VB" MasterPageFile="~/DorkNozzle.master" AutoEventWireup="True" CodeFile="AddressBook.aspx.vb" Inherits="AddressBook" title="Dorknozzle Address Book" %>
<asp:Content ID="Content1" ContentPlaceHolderID="ContentPlaceHolder1" Runat="Server">
429

Chapter 11: Managing Content Using Grid View and Details View
<h1>Address Book</h1>
<asp:GridView id="grid" runat="server" />
</asp:Content>
By switching to Design View you can see how your grid is represented in the designer, as Figure 11.1 indicates.
Figure 11.1. Viewing AddressBook.aspx in Design View
Now, double-click on an empty portion of the page to have the form’s Page_Load event handler generated for you in the code-behind file. In Page_Load, we’ll call a method named BindGrid, which will, in turn, create a database connection and a database command object, execute that command, and bind the resulting data reader to the grid, as shown below:
Visual Basic |
File: AddressBook.aspx.vb (excerpt) |
Imports System.Data.SqlClient
430

Using the GridView Control
Partial Class AddressBook
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, _ ByVal e As System.EventArgs) Handles Me.Load
If Not IsPostBack Then BindGrid()
End If
End Sub
Private Sub BindGrid()
'Define data objects Dim conn As SqlConnection Dim comm As SqlCommand
Dim reader As SqlDataReader
'Read the connection string from Web.config Dim connectionString As String = _
ConfigurationManager.ConnectionStrings( _ "Dorknozzle").ConnectionString
'Initialize connection
conn = New SqlConnection(connectionString) ' Create command
comm = New SqlCommand( _
"SELECT EmployeeID, Name, City, State, MobilePhone " & _ "FROM Employees", conn)
' Enclose database code in Try-Catch-Finally Try
'Open the connection conn.Open()
'Execute the command
reader = comm.ExecuteReader()
'Fill the grid with data grid.DataSource = reader grid.DataBind()
'Close the reader reader.Close()
Finally
' Close the connection
conn.Close()
End Try
End Sub
End Class
431
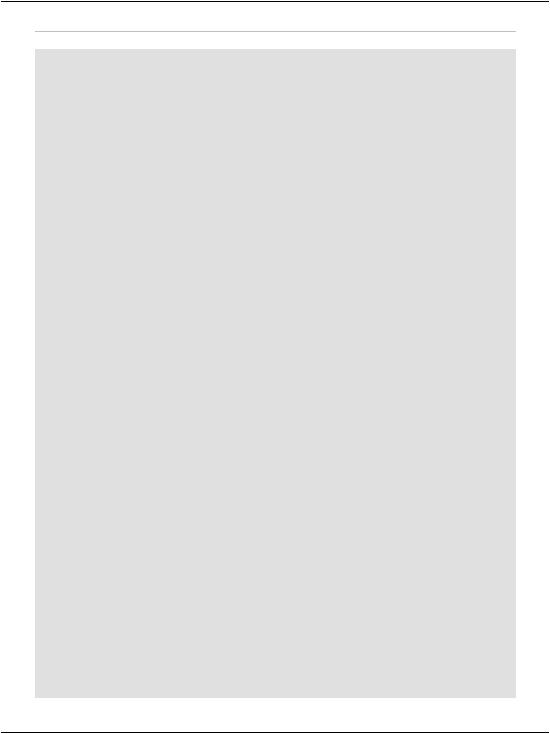
Chapter 11: Managing Content Using Grid View and Details View
C# |
File: AddressBook.aspx.cs (excerpt) |
|
|
using System; |
|
using System.Data; |
|
using System.Configuration; |
|
using System.Collections; |
|
using System.Web; |
|
using System.Web.Security; |
|
using System.Web.UI; |
|
using System.Web.UI.WebControls; |
|
using System.Web.UI.WebControls.WebParts; |
|
using System.Web.UI.HtmlControls; |
|
using System.Data.SqlClient; |
|
public partial class AddressBook : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGrid();
}
}
private void BindGrid()
{
//Define data objects SqlConnection conn; SqlCommand comm; SqlDataReader reader;
//Read the connection string from Web.config string connectionString =
ConfigurationManager.ConnectionStrings[
"Dorknozzle"].ConnectionString;
//Initialize connection
conn = new SqlConnection(connectionString); // Create command
comm = new SqlCommand(
"SELECT EmployeeID, Name, City, State, MobilePhone " + "FROM Employees", conn);
// Enclose database code in Try-Catch-Finally try
{
//Open the connection conn.Open();
//Execute the command
reader = comm.ExecuteReader(); // Fill the grid with data
432

Using the GridView Control
grid.DataSource = reader; grid.DataBind();
// Close the reader reader.Close();
}
finally
{
// Close the connection conn.Close();
}
}
}
What’s going on here? If you disregard the fact that you’re binding the
SqlDataReader to a GridView instead of a Repeater or DataList, the code is almost identical to that which we saw in the previous chapter.
Now save your work and open the page in the browser. Figure 11.2 shows how the GridView presents all of the data within the Employees table in a cleanly formatted structure.
Figure 11.2. Displaying the address book in GridView
433

Chapter 11: Managing Content Using Grid View and Details View
Well, okay, perhaps it doesn’t look all that clean right now! However, the display will change as we get some practice using the GridView’s powerful yet intuitive formatting capabilities. You’ll notice that the GridView closely resembles the structure of the query’s result as you might see it in SQL Server Management Studio. All the names of the columns in the database table show as headers within the table, and all the rows from the database table are repeated down the page.
If you look at the generated page source (right-click the page in browser and choose View Source or similar), you’ll see that the GridView indeed generated a table for you:
<table cellspacing="0" rules="all" border="1" id="ctl00_ContentPlaceHolder1_grid" style="border-collapse:collapse;">
<tr>
<th scope="col">EmployeeID</th> <th scope="col">Name</th>
<th scope="col">City</th> <th scope="col">State</th>
<th scope="col">MobilePhone</th> </tr>
<tr>
<td>1</td>
<td>Zak Ruvalcaba</td> <td>San Diego</td> <td>Ca</td> <td>555-555-5551</td>
</tr>
<tr>
<td>2</td>
<td>Jessica Ruvalcaba</td> <td>San Diego</td> <td>Ca</td> <td>555-555-5552</td>
</tr>
</table>
Formatted for Readability
The HTML generated by ASP.NET won’t look exactly as it does above. You’ll find that ASP.NET will output long, difficult-to-read lines of td elements, each of which appears directly after the previous one. We’ve simply made it a little easier to read; the two HTML tables are otherwise identical.
434