
- •Table of Contents
- •Preface
- •What is ASP.NET?
- •Installing the Required Software
- •Installing the Web Server
- •Installing Internet Information Services (IIS)
- •Installing Cassini
- •Installing the .NET Framework and the SDK
- •Installing the .NET Framework
- •Installing the SDK
- •Configuring the Web Server
- •Configuring IIS
- •Configuring Cassini
- •Where do I Put my Files?
- •Using localhost
- •Virtual Directories
- •Using Cassini
- •Installing SQL Server 2005 Express Edition
- •Installing SQL Server Management Studio Express
- •Installing Visual Web Developer 2005
- •Writing your First ASP.NET Page
- •Getting Help
- •Summary
- •ASP.NET Basics
- •ASP.NET Page Structure
- •Directives
- •Code Declaration Blocks
- •Comments in VB and C# Code
- •Code Render Blocks
- •ASP.NET Server Controls
- •Server-side Comments
- •Literal Text and HTML Tags
- •View State
- •Working with Directives
- •ASP.NET Languages
- •Visual Basic
- •Summary
- •VB and C# Programming Basics
- •Programming Basics
- •Control Events and Subroutines
- •Page Events
- •Variables and Variable Declaration
- •Arrays
- •Functions
- •Operators
- •Breaking Long Lines of Code
- •Conditional Logic
- •Loops
- •Object Oriented Programming Concepts
- •Objects and Classes
- •Properties
- •Methods
- •Classes
- •Constructors
- •Scope
- •Events
- •Understanding Inheritance
- •Objects In .NET
- •Namespaces
- •Using Code-behind Files
- •Summary
- •Constructing ASP.NET Web Pages
- •Web Forms
- •HTML Server Controls
- •Using the HTML Server Controls
- •Web Server Controls
- •Standard Web Server Controls
- •Label
- •Literal
- •TextBox
- •HiddenField
- •Button
- •ImageButton
- •LinkButton
- •HyperLink
- •CheckBox
- •RadioButton
- •Image
- •ImageMap
- •PlaceHolder
- •Panel
- •List Controls
- •DropDownList
- •ListBox
- •RadioButtonList
- •CheckBoxList
- •BulletedList
- •Advanced Controls
- •Calendar
- •AdRotator
- •TreeView
- •SiteMapPath
- •Menu
- •MultiView
- •Wizard
- •FileUpload
- •Web User Controls
- •Creating a Web User Control
- •Using the Web User Control
- •Master Pages
- •Using Cascading Style Sheets (CSS)
- •Types of Styles and Style Sheets
- •Style Properties
- •The CssClass Property
- •Summary
- •Building Web Applications
- •Introducing the Dorknozzle Project
- •Using Visual Web Developer
- •Meeting the Features
- •The Solution Explorer
- •The Web Forms Designer
- •The Code Editor
- •IntelliSense
- •The Toolbox
- •The Properties Window
- •Executing your Project
- •Using Visual Web Developer’s Built-in Web Server
- •Using IIS
- •Using IIS with Visual Web Developer
- •Core Web Application Features
- •Web.config
- •Global.asax
- •Using Application State
- •Working with User Sessions
- •Using the Cache Object
- •Using Cookies
- •Starting the Dorknozzle Project
- •Preparing the Sitemap
- •Using Themes, Skins, and Styles
- •Creating a New Theme Folder
- •Creating a New Style Sheet
- •Styling Web Server Controls
- •Adding a Skin
- •Applying the Theme
- •Building the Master Page
- •Using the Master Page
- •Extending Dorknozzle
- •Debugging and Error Handling
- •Debugging with Visual Web Developer
- •Other Kinds of Errors
- •Custom Errors
- •Handling Exceptions Locally
- •Summary
- •Using the Validation Controls
- •Enforcing Validation on the Server
- •Using Validation Controls
- •RequiredFieldValidator
- •CompareValidator
- •RangeValidator
- •ValidationSummary
- •RegularExpressionValidator
- •Some Useful Regular Expressions
- •CustomValidator
- •Validation Groups
- •Updating Dorknozzle
- •Summary
- •What is a Database?
- •Creating your First Database
- •Creating a New Database Using Visual Web Developer
- •Creating Database Tables
- •Data Types
- •Column Properties
- •Primary Keys
- •Creating the Employees Table
- •Creating the Remaining Tables
- •Executing SQL Scripts
- •Populating the Data Tables
- •Relational Database Design Concepts
- •Foreign Keys
- •Using Database Diagrams
- •Diagrams and Table Relationships
- •One-to-one Relationships
- •One-to-many Relationships
- •Many-to-many Relationships
- •Summary
- •Speaking SQL
- •Reading Data from a Single Table
- •Using the SELECT Statement
- •Selecting Certain Fields
- •Selecting Unique Data with DISTINCT
- •Row Filtering with WHERE
- •Selecting Ranges of Values with BETWEEN
- •Matching Patterns with LIKE
- •Using the IN Operator
- •Sorting Results Using ORDER BY
- •Limiting the Number of Results with TOP
- •Reading Data from Multiple Tables
- •Subqueries
- •Table Joins
- •Expressions and Operators
- •Transact-SQL Functions
- •Arithmetic Functions
- •String Functions
- •Date and Time Functions
- •Working with Groups of Values
- •The COUNT Function
- •Grouping Records Using GROUP BY
- •Filtering Groups Using HAVING
- •The SUM, AVG, MIN, and MAX Functions
- •Updating Existing Data
- •The INSERT Statement
- •The UPDATE Statement
- •The DELETE Statement
- •Stored Procedures
- •Summary
- •Introducing ADO.NET
- •Importing the SqlClient Namespace
- •Defining the Database Connection
- •Preparing the Command
- •Executing the Command
- •Setting up Database Authentication
- •Reading the Data
- •Using Parameters with Queries
- •Bulletproofing Data Access Code
- •Using the Repeater Control
- •More Data Binding
- •Inserting Records
- •Updating Records
- •Deleting Records
- •Using Stored Procedures
- •Summary
- •DataList Basics
- •Handling DataList Events
- •Editing DataList Items and Using Templates
- •DataList and Visual Web Developer
- •Styling the DataList
- •Summary
- •Using the GridView Control
- •Customizing the GridView Columns
- •Styling the GridView with Templates, Skins, and CSS
- •Selecting Grid Records
- •Using the DetailsView Control
- •Styling the DetailsView
- •GridView and DetailsView Events
- •Entering Edit Mode
- •Using Templates
- •Updating DetailsView Records
- •Summary
- •Advanced Data Access
- •Using Data Source Controls
- •Binding the GridView to a SqlDataSource
- •Binding the DetailsView to a SqlDataSource
- •Displaying Lists in DetailsView
- •More on SqlDataSource
- •Working with Data Sets and Data Tables
- •What is a Data Set Made From?
- •Binding DataSets to Controls
- •Implementing Paging
- •Storing Data Sets in View State
- •Implementing Sorting
- •Filtering Data
- •Updating a Database from a Modified DataSet
- •Summary
- •Security and User Authentication
- •Basic Security Guidelines
- •Securing ASP.NET 2.0 Applications
- •Working with Forms Authentication
- •Authenticating Users
- •Working with Hard-coded User Accounts
- •Configuring Forms Authentication
- •Configuring Forms Authorization
- •Storing Users in Web.config
- •Hashing Passwords
- •Logging Users Out
- •ASP.NET 2.0 Memberships and Roles
- •Creating the Membership Data Structures
- •Using your Database to Store Membership Data
- •Using the ASP.NET Web Site Configuration Tool
- •Creating Users and Roles
- •Changing Password Strength Requirements
- •Securing your Web Application
- •Using the ASP.NET Login Controls
- •Authenticating Users
- •Customizing User Display
- •Summary
- •Working with Files and Email
- •Writing and Reading Text Files
- •Setting Up Security
- •Writing Content to a Text File
- •Reading Content from a Text File
- •Accessing Directories and Directory Information
- •Working with Directory and File Paths
- •Uploading Files
- •Sending Email with ASP.NET
- •Configuring the SMTP Server
- •Sending a Test Email
- •Creating the Company Newsletter Page
- •Summary
- •The WebControl Class
- •Properties
- •Methods
- •Standard Web Controls
- •AdRotator
- •Properties
- •Events
- •BulletedList
- •Properties
- •Events
- •Button
- •Properties
- •Events
- •Calendar
- •Properties
- •Events
- •CheckBox
- •Properties
- •Events
- •CheckBoxList
- •Properties
- •Events
- •DropDownList
- •Properties
- •Events
- •FileUpload
- •Properties
- •Methods
- •HiddenField
- •Properties
- •HyperLink
- •Properties
- •Image
- •Properties
- •ImageButton
- •Properties
- •Events
- •ImageMap
- •Properties
- •Events
- •Label
- •Properties
- •LinkButton
- •Properties
- •Events
- •ListBox
- •Properties
- •Events
- •Literal
- •Properties
- •MultiView
- •Properties
- •Methods
- •Events
- •Panel
- •Properties
- •PlaceHolder
- •Properties
- •RadioButton
- •Properties
- •Events
- •RadioButtonList
- •Properties
- •Events
- •TextBox
- •Properties
- •Events
- •Properties
- •Validation Controls
- •CompareValidator
- •Properties
- •Methods
- •CustomValidator
- •Methods
- •Events
- •RangeValidator
- •Properties
- •Methods
- •RegularExpressionValidator
- •Properties
- •Methods
- •RequiredFieldValidator
- •Properties
- •Methods
- •ValidationSummary
- •Properties
- •Navigation Web Controls
- •SiteMapPath
- •Properties
- •Methods
- •Events
- •Menu
- •Properties
- •Methods
- •Events
- •TreeView
- •Properties
- •Methods
- •Events
- •HTML Server Controls
- •HtmlAnchor Control
- •Properties
- •Events
- •HtmlButton Control
- •Properties
- •Events
- •HtmlForm Control
- •Properties
- •HtmlGeneric Control
- •Properties
- •HtmlImage Control
- •Properties
- •HtmlInputButton Control
- •Properties
- •Events
- •HtmlInputCheckBox Control
- •Properties
- •Events
- •HtmlInputFile Control
- •Properties
- •HtmlInputHidden Control
- •Properties
- •HtmlInputImage Control
- •Properties
- •Events
- •HtmlInputRadioButton Control
- •Properties
- •Events
- •HtmlInputText Control
- •Properties
- •Events
- •HtmlSelect Control
- •Properties
- •Events
- •HtmlTable Control
- •Properties
- •HtmlTableCell Control
- •Properties
- •HtmlTableRow Control
- •Properties
- •HtmlTextArea Control
- •Properties
- •Events
- •Index

Selecting Ranges of Values with BETWEEN
(2 row(s) affected)
But wait! How do I know the name of the department with the ID of 6? Well, you could use a similar query to find out. Try this:
SELECT Department
FROM Departments
WHERE DepartmentID = 6
Executing this query reveals that the department with the ID of 6 is Engineering.
Department
--------------------------------------------------
Engineering
(1 row(s) affected)
Selecting Ranges of Values with BETWEEN
There may be times when you’ll want to search within a database table for rows that fall within a certain range of values. For instance, if you wanted to retrieve from the Departments table all departments that have IDs between 2 and 5, you could use the BETWEEN keyword like so:
SELECT DepartmentID, Department
FROM Departments
WHERE DepartmentID BETWEEN 2 AND 5
As we requested, all departments whose IDs are between 2 and 5 are returned. Note that the range is inclusive, so departments with IDs of 2 and 5 will also be retrieved.
Note that any conditions that use BETWEEN could be easily rewritten by combining two “greater than or equal” and “less than or equal” conditions:
SELECT DepartmentID, Department
FROM Departments
WHERE DepartmentID >= 2 AND DepartmentID <= 5
We could also use the NOT keyword before the BETWEEN keyword to specify all items that fall outside the range, as follows:
303

Chapter 8: Speaking SQL
SELECT DepartmentID, Department
FROM Departments
WHERE DepartmentID NOT BETWEEN 2 AND 5
In this example, all rows whose DepartmentIDs are less than 2 or greater than 5 are returned.
Matching Patterns with LIKE
As we’ve just seen, the WHERE clause allows us to filter results based on criteria that we specify. The example we discussed earlier filtered rows by comparing two numbers, but SQL also knows how to handle strings. For example, if we wanted to search the company’s Employees table for all employees named Zak Ruvalcaba, we'd use the following SQL statement:
SELECT EmployeeID, Username
FROM Employees
WHERE Name = 'Zak Ruvalcaba'
However, we won’t see many such queries in reality. In real-world scenarios, most record matching is done by matching the primary key of the table to some specific value. When an arbitrary string such as a name is used (as in the example above), it’s likely that we’re searching for data based on partially complete information.
A more realistic example is one in which we want to find all employees with the surname Ruvalcaba. The LIKE keyword allows us to perform pattern matching with the help of wildcard characters. The wildcard characters supported by SQL Server are the percentage symbol (%), which matches any sequence of zero or more characters, and the underscore symbol (_), which matches exactly one character.
If we wanted to find all names within our Employees table with the surname of Ruvalcaba, we could modify the SQL query using a wildcard, as follows:
SELECT EmployeeID, Name
FROM Employees
WHERE Name LIKE '%Ruvalcaba'
With this query, all records in which the Name column ends with Ruvalcaba are returned, as shown below.
EmployeeID |
Name |
----------- |
-------------------------------------------------- |
1 |
Zak Ruvalcaba |
304
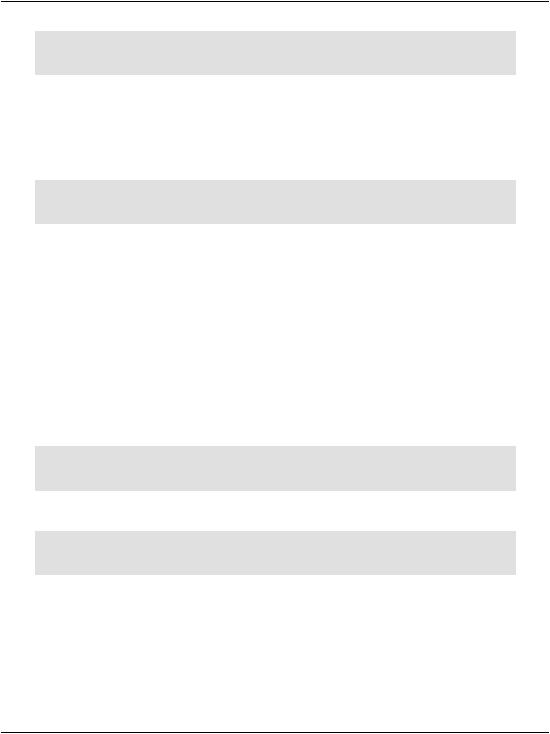
Using the IN Operator
2 |
Jessica Ruvalcaba |
(2 row(s) affected)
As we knew that the last name was Ruvalcaba, we only needed to place a wildcard immediately before the last name. But what would happen if we didn’t know how to spell the entire last name? That name is pretty difficult to spell! You could solve the problem by modifying your SQL statement to use two wildcards as follows:
SELECT EmployeeID, Name
FROM Employees
WHERE Name LIKE '%Ruv%'
In this case, the wildcard is placed before and after the string Ruv. Although this statement would return the same values we saw in the results table above, it would also return any employees whose names (first or last) contain the sequence Ruv. As SQL is case-insensitive, this would include the names Sarah Ruvin, Jonny Noruvitch, Truvor MacDonald, and so on.
Using the IN Operator
We use the IN operator in SELECT queries primarily to specify a list of values that we want to match in our WHERE clause. Let’s say we want to find all employees who live in California, Indiana, and Maryland. You could write the following SQL statement to accomplish this task:
SELECT Name, State
FROM Employees
WHERE State = 'CA' OR State = 'IN' OR State = 'MD'
A better way to write this statement uses the IN operator as follows:
SELECT Name, State
FROM Employees
WHERE State IN ('CA', 'IN', 'MD')
If you execute this query, you’ll get the expected results. Since our database only contains employees living in CA, only those records will be displayed.
Name |
State |
-------------------------------- |
-------------------------------- |
Zak Ruvalcaba |
Ca |
Jessica Ruvalcaba |
Ca |
305

Chapter 8: Speaking SQL
Ted Lindsey |
Ca |
|
Shane Weebe |
Ca |
|
David |
Levinson |
Ca |
Geoff |
Kim |
Ca |
(6 row(s) affected)
Sorting Results Using ORDER BY
Unless you specify some sorting criteria, SQL Server can’t guarantee to return the results in a particular order. We’ll most likely receive the results sorted by the primary key, because it’s easier for SQL Server to present the results in this way than any other, but this ordering isn’t guaranteed. This explains why, in some of the examples we’ve completed so far, the order of the results you see on your machine may differ from what you see in this book. The ORDER BY clause provides you with a quick way to sort the results of your query in either ascending or descending order. For instance, to retrieve the names of your employees in alphabetical order, you would need to execute this command:
SELECT EmployeeID, Name
FROM Employees
ORDER BY Name
Looks simple, doesn’t it?
EmployeeID Name
----------- --------------------------------------------------
5David Levinson
6Geoff Kim
2 |
Jessica Ruvalcaba |
|
4 |
Shane Weebe |
|
3 |
Ted |
Lindsey |
1 |
Zak |
Ruvalcaba |
(6 row(s) affected)
Note that the default ordering here is ascending (i.e. running from A to Z). You could add the DESC designation (for descending) to the end of the statement, to order the results backwards:
SELECT EmployeeID, Name
FROM Employees
ORDER BY Name DESC
306