
- •Table of Contents
- •Preface
- •What is ASP.NET?
- •Installing the Required Software
- •Installing the Web Server
- •Installing Internet Information Services (IIS)
- •Installing Cassini
- •Installing the .NET Framework and the SDK
- •Installing the .NET Framework
- •Installing the SDK
- •Configuring the Web Server
- •Configuring IIS
- •Configuring Cassini
- •Where do I Put my Files?
- •Using localhost
- •Virtual Directories
- •Using Cassini
- •Installing SQL Server 2005 Express Edition
- •Installing SQL Server Management Studio Express
- •Installing Visual Web Developer 2005
- •Writing your First ASP.NET Page
- •Getting Help
- •Summary
- •ASP.NET Basics
- •ASP.NET Page Structure
- •Directives
- •Code Declaration Blocks
- •Comments in VB and C# Code
- •Code Render Blocks
- •ASP.NET Server Controls
- •Server-side Comments
- •Literal Text and HTML Tags
- •View State
- •Working with Directives
- •ASP.NET Languages
- •Visual Basic
- •Summary
- •VB and C# Programming Basics
- •Programming Basics
- •Control Events and Subroutines
- •Page Events
- •Variables and Variable Declaration
- •Arrays
- •Functions
- •Operators
- •Breaking Long Lines of Code
- •Conditional Logic
- •Loops
- •Object Oriented Programming Concepts
- •Objects and Classes
- •Properties
- •Methods
- •Classes
- •Constructors
- •Scope
- •Events
- •Understanding Inheritance
- •Objects In .NET
- •Namespaces
- •Using Code-behind Files
- •Summary
- •Constructing ASP.NET Web Pages
- •Web Forms
- •HTML Server Controls
- •Using the HTML Server Controls
- •Web Server Controls
- •Standard Web Server Controls
- •Label
- •Literal
- •TextBox
- •HiddenField
- •Button
- •ImageButton
- •LinkButton
- •HyperLink
- •CheckBox
- •RadioButton
- •Image
- •ImageMap
- •PlaceHolder
- •Panel
- •List Controls
- •DropDownList
- •ListBox
- •RadioButtonList
- •CheckBoxList
- •BulletedList
- •Advanced Controls
- •Calendar
- •AdRotator
- •TreeView
- •SiteMapPath
- •Menu
- •MultiView
- •Wizard
- •FileUpload
- •Web User Controls
- •Creating a Web User Control
- •Using the Web User Control
- •Master Pages
- •Using Cascading Style Sheets (CSS)
- •Types of Styles and Style Sheets
- •Style Properties
- •The CssClass Property
- •Summary
- •Building Web Applications
- •Introducing the Dorknozzle Project
- •Using Visual Web Developer
- •Meeting the Features
- •The Solution Explorer
- •The Web Forms Designer
- •The Code Editor
- •IntelliSense
- •The Toolbox
- •The Properties Window
- •Executing your Project
- •Using Visual Web Developer’s Built-in Web Server
- •Using IIS
- •Using IIS with Visual Web Developer
- •Core Web Application Features
- •Web.config
- •Global.asax
- •Using Application State
- •Working with User Sessions
- •Using the Cache Object
- •Using Cookies
- •Starting the Dorknozzle Project
- •Preparing the Sitemap
- •Using Themes, Skins, and Styles
- •Creating a New Theme Folder
- •Creating a New Style Sheet
- •Styling Web Server Controls
- •Adding a Skin
- •Applying the Theme
- •Building the Master Page
- •Using the Master Page
- •Extending Dorknozzle
- •Debugging and Error Handling
- •Debugging with Visual Web Developer
- •Other Kinds of Errors
- •Custom Errors
- •Handling Exceptions Locally
- •Summary
- •Using the Validation Controls
- •Enforcing Validation on the Server
- •Using Validation Controls
- •RequiredFieldValidator
- •CompareValidator
- •RangeValidator
- •ValidationSummary
- •RegularExpressionValidator
- •Some Useful Regular Expressions
- •CustomValidator
- •Validation Groups
- •Updating Dorknozzle
- •Summary
- •What is a Database?
- •Creating your First Database
- •Creating a New Database Using Visual Web Developer
- •Creating Database Tables
- •Data Types
- •Column Properties
- •Primary Keys
- •Creating the Employees Table
- •Creating the Remaining Tables
- •Executing SQL Scripts
- •Populating the Data Tables
- •Relational Database Design Concepts
- •Foreign Keys
- •Using Database Diagrams
- •Diagrams and Table Relationships
- •One-to-one Relationships
- •One-to-many Relationships
- •Many-to-many Relationships
- •Summary
- •Speaking SQL
- •Reading Data from a Single Table
- •Using the SELECT Statement
- •Selecting Certain Fields
- •Selecting Unique Data with DISTINCT
- •Row Filtering with WHERE
- •Selecting Ranges of Values with BETWEEN
- •Matching Patterns with LIKE
- •Using the IN Operator
- •Sorting Results Using ORDER BY
- •Limiting the Number of Results with TOP
- •Reading Data from Multiple Tables
- •Subqueries
- •Table Joins
- •Expressions and Operators
- •Transact-SQL Functions
- •Arithmetic Functions
- •String Functions
- •Date and Time Functions
- •Working with Groups of Values
- •The COUNT Function
- •Grouping Records Using GROUP BY
- •Filtering Groups Using HAVING
- •The SUM, AVG, MIN, and MAX Functions
- •Updating Existing Data
- •The INSERT Statement
- •The UPDATE Statement
- •The DELETE Statement
- •Stored Procedures
- •Summary
- •Introducing ADO.NET
- •Importing the SqlClient Namespace
- •Defining the Database Connection
- •Preparing the Command
- •Executing the Command
- •Setting up Database Authentication
- •Reading the Data
- •Using Parameters with Queries
- •Bulletproofing Data Access Code
- •Using the Repeater Control
- •More Data Binding
- •Inserting Records
- •Updating Records
- •Deleting Records
- •Using Stored Procedures
- •Summary
- •DataList Basics
- •Handling DataList Events
- •Editing DataList Items and Using Templates
- •DataList and Visual Web Developer
- •Styling the DataList
- •Summary
- •Using the GridView Control
- •Customizing the GridView Columns
- •Styling the GridView with Templates, Skins, and CSS
- •Selecting Grid Records
- •Using the DetailsView Control
- •Styling the DetailsView
- •GridView and DetailsView Events
- •Entering Edit Mode
- •Using Templates
- •Updating DetailsView Records
- •Summary
- •Advanced Data Access
- •Using Data Source Controls
- •Binding the GridView to a SqlDataSource
- •Binding the DetailsView to a SqlDataSource
- •Displaying Lists in DetailsView
- •More on SqlDataSource
- •Working with Data Sets and Data Tables
- •What is a Data Set Made From?
- •Binding DataSets to Controls
- •Implementing Paging
- •Storing Data Sets in View State
- •Implementing Sorting
- •Filtering Data
- •Updating a Database from a Modified DataSet
- •Summary
- •Security and User Authentication
- •Basic Security Guidelines
- •Securing ASP.NET 2.0 Applications
- •Working with Forms Authentication
- •Authenticating Users
- •Working with Hard-coded User Accounts
- •Configuring Forms Authentication
- •Configuring Forms Authorization
- •Storing Users in Web.config
- •Hashing Passwords
- •Logging Users Out
- •ASP.NET 2.0 Memberships and Roles
- •Creating the Membership Data Structures
- •Using your Database to Store Membership Data
- •Using the ASP.NET Web Site Configuration Tool
- •Creating Users and Roles
- •Changing Password Strength Requirements
- •Securing your Web Application
- •Using the ASP.NET Login Controls
- •Authenticating Users
- •Customizing User Display
- •Summary
- •Working with Files and Email
- •Writing and Reading Text Files
- •Setting Up Security
- •Writing Content to a Text File
- •Reading Content from a Text File
- •Accessing Directories and Directory Information
- •Working with Directory and File Paths
- •Uploading Files
- •Sending Email with ASP.NET
- •Configuring the SMTP Server
- •Sending a Test Email
- •Creating the Company Newsletter Page
- •Summary
- •The WebControl Class
- •Properties
- •Methods
- •Standard Web Controls
- •AdRotator
- •Properties
- •Events
- •BulletedList
- •Properties
- •Events
- •Button
- •Properties
- •Events
- •Calendar
- •Properties
- •Events
- •CheckBox
- •Properties
- •Events
- •CheckBoxList
- •Properties
- •Events
- •DropDownList
- •Properties
- •Events
- •FileUpload
- •Properties
- •Methods
- •HiddenField
- •Properties
- •HyperLink
- •Properties
- •Image
- •Properties
- •ImageButton
- •Properties
- •Events
- •ImageMap
- •Properties
- •Events
- •Label
- •Properties
- •LinkButton
- •Properties
- •Events
- •ListBox
- •Properties
- •Events
- •Literal
- •Properties
- •MultiView
- •Properties
- •Methods
- •Events
- •Panel
- •Properties
- •PlaceHolder
- •Properties
- •RadioButton
- •Properties
- •Events
- •RadioButtonList
- •Properties
- •Events
- •TextBox
- •Properties
- •Events
- •Properties
- •Validation Controls
- •CompareValidator
- •Properties
- •Methods
- •CustomValidator
- •Methods
- •Events
- •RangeValidator
- •Properties
- •Methods
- •RegularExpressionValidator
- •Properties
- •Methods
- •RequiredFieldValidator
- •Properties
- •Methods
- •ValidationSummary
- •Properties
- •Navigation Web Controls
- •SiteMapPath
- •Properties
- •Methods
- •Events
- •Menu
- •Properties
- •Methods
- •Events
- •TreeView
- •Properties
- •Methods
- •Events
- •HTML Server Controls
- •HtmlAnchor Control
- •Properties
- •Events
- •HtmlButton Control
- •Properties
- •Events
- •HtmlForm Control
- •Properties
- •HtmlGeneric Control
- •Properties
- •HtmlImage Control
- •Properties
- •HtmlInputButton Control
- •Properties
- •Events
- •HtmlInputCheckBox Control
- •Properties
- •Events
- •HtmlInputFile Control
- •Properties
- •HtmlInputHidden Control
- •Properties
- •HtmlInputImage Control
- •Properties
- •Events
- •HtmlInputRadioButton Control
- •Properties
- •Events
- •HtmlInputText Control
- •Properties
- •Events
- •HtmlSelect Control
- •Properties
- •Events
- •HtmlTable Control
- •Properties
- •HtmlTableCell Control
- •Properties
- •HtmlTableRow Control
- •Properties
- •HtmlTextArea Control
- •Properties
- •Events
- •Index

Updating a Database from a Modified DataSet
The DataView class has a property named RowFilter that allows you to specify an expression similar to that of an SQL statement’s WHERE clause. For instance, the following filter selects all departments whose names start with “a”:
Visual Basic
dataTable.DefaultView.RowFilter = "Department LIKE 'a%'"
C#
dataTable.DefaultView.RowFilter = "Department LIKE 'a%'";
Updating a Database from a Modified
DataSet
So far, we’ve used the DataSet exclusively for retrieving and binding database data to controls such as the GridView. The reverse operation—updating data within a database from a DataSet—is also possible using the Update method of the SqlDataAdapter.
The SqlDataAdapter has the following four properties, which represent the main database commands:
SelectCommand
InsertCommand
UpdateCommand
DeleteCommand
The SelectCommand contains the command that’s executed when we call Fill. The other properties are quite similar, except that you must call the Update method instead.
If we want to insert, update, or remove records in a database, we simply make modifications to the data in the DataSet or DataTable, then call the Update method of the SqlDataAdapter. This will automatically execute the SQL queries specified in the InsertCommand, UpdateCommand, and DeleteCommand properties as appropriate.
The excellent news is that ADO.NET also provides an object named
SqlCommandBuilder, which creates the UPDATE, DELETE, and INSERT code for us.
521

Chapter 12: Advanced Data Access
Basically, we just need to populate the DataSet or DataTable objects (usually by performing a SELECT query), then use SqlDataAdapter and SqlCommandBuilder to do the rest of the work for us.
In the example below, we’ll see a modified version of BindGrid that adds a new department, called New Department, to the database. The new lines are highlighted (note that I’ve simplified BindGrid by removing the code that stores and retrieves the DataSet from view state, as well as the code that sorts the results):
Visual Basic
Private Sub BindGrid() ' Define data objects
Dim conn As SqlConnection Dim dataSet As New DataSet
Dim adapter As SqlDataAdapter
Dim dataRow As DataRow
Dim commandBuilder As SqlCommandBuilder
'Read the connection string from Web.config Dim connectionString As String = _
ConfigurationManager.ConnectionStrings( _ "Dorknozzle").ConnectionString
'Initialize connection
conn = New SqlConnection(connectionString) ' Create adapter
adapter = New SqlDataAdapter( _
"SELECT DepartmentID, Department FROM Departments", _ conn)
'Fill the DataSet adapter.Fill(dataSet, "Departments")
'Make changes to the table
dataRow = dataSet.Tables("Departments").NewRow() dataRow("Department") = "New Department" dataSet.Tables("Departments").Rows.Add(dataRow) ' Submit the changes
commandBuilder = New SqlCommandBuilder(adapter) adapter.Update(dataSet.Tables("Departments"))
' Bind the grid to the DataSet departmentsGrid.DataSource = _
dataSet.Tables("Departments").DefaultView departmentsGrid.DataBind()
End Sub
C#
private void BindGrid()
{
// Define data objects
522
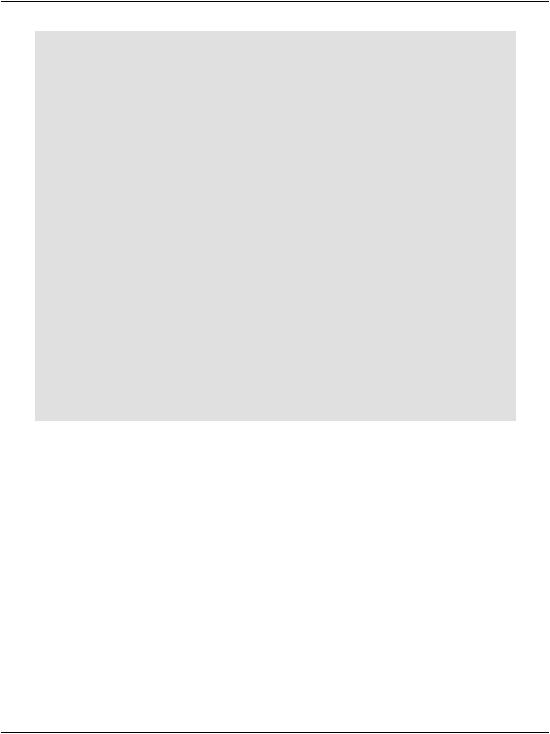
Updating a Database from a Modified DataSet
SqlConnection conn;
DataSet dataSet = new DataSet();
SqlDataAdapter adapter;
DataRow dataRow ;
SqlCommandBuilder commandBuilder;
//Read the connection string from Web.config string connectionString =
ConfigurationManager.ConnectionStrings[
"Dorknozzle"].ConnectionString;
//Initialize connection
conn = new SqlConnection(connectionString); // Create adapter
adapter = new SqlDataAdapter(
"SELECT DepartmentID, Department FROM Departments", conn);
//Fill the DataSet adapter.Fill(dataSet, "Departments");
//Make changes to the table
dataRow = dataSet.Tables["Departments"].NewRow(); dataRow["Department"] = "New Department"; dataSet.Tables["Departments"].Rows.Add(dataRow); // Submit the changes
commandBuilder = new SqlCommandBuilder(adapter); adapter.Update(dataSet.Tables["Departments"]); departmentsGrid.DataSource = dataSet.Tables["Departments"]; departmentsGrid.DataBind();
}
If you run this code a few times, you’ll have lots of “New Department” departments added to the database, as shown in Figure 12.27.
As you can see, adding a new record is a trivial task. The work that’s required to submit the changes to the database requires us to write just two rows of code. The rest of the new code creates the new row that was inserted.
We create an SqlCommandBuilder object, passing in our SqlDataAdapter. The SqlCommandBuilder class is responsible for detecting modifications to the DataSet and deciding what needs to be inserted, updated, or deleted to apply those changes to the database. Having done this, SqlCommandBuilder generates the necessary SQL queries and stores them in the SqlDataAdapter for the Update method to use. It should be no surprise, then, that our next action is to call the Update method of the SqlDataAdapter object, passing in the DataTable that needs updating.
523

Chapter 12: Advanced Data Access
Figure 12.27. Adding many new departments
Deleting all of these new departments is also an easy task. The following code browses the Departments DataTable and deletes all departments with the name
New Department:
Visual Basic
' Define data objects
Dim conn As SqlConnection Dim dataSet As New DataSet
Dim adapter As SqlDataAdapter
Dim commandBuilder As SqlCommandBuilder
'Read the connection string from Web.config Dim connectionString As String = _
ConfigurationManager.ConnectionStrings( _ "Dorknozzle").ConnectionString
'Initialize connection
conn = New SqlConnection(connectionString) ' Create adapter
adapter = New SqlDataAdapter( _
"SELECT DepartmentID, Department FROM Departments", _ conn)
' Fill the DataSet
524

Updating a Database from a Modified DataSet
adapter.Fill(dataSet, "Departments")
' Make changes to the table
For Each dataRow As DataRow In dataSet.Tables("Departments").Rows If dataRow("Department") = "New Department" Then
dataRow.Delete() End If
Next
' Submit the changes
commandBuilder = New SqlCommandBuilder(adapter) adapter.Update(dataSet.Tables("Departments"))
' Bind the grid to the DataSet departmentsGrid.DataSource = _
dataSet.Tables("Departments").DefaultView departmentsGrid.DataBind()
Note that in the C# version the conversion to string needs to be performed explicitly:
C#
//Define data objects SqlConnection conn;
DataSet dataSet = new DataSet(); SqlDataAdapter adapter; SqlCommandBuilder commandBuilder;
//Read the connection string from Web.config string connectionString =
ConfigurationManager.ConnectionStrings[
"Dorknozzle"].ConnectionString;
//Initialize connection
conn = new SqlConnection(connectionString); // Create adapter
adapter = new SqlDataAdapter(
"SELECT DepartmentID, Department FROM Departments", conn);
//Fill the DataSet adapter.Fill(dataSet, "Departments");
//Make changes to the table
foreach (DataRow dataRow in dataSet.Tables["Departments"].Rows)
{
if(dataRow["Department"].ToString() == "New Department")
{
dataRow.Delete();
}
}
// Submit the changes
commandBuilder = new SqlCommandBuilder(adapter); adapter.Update(dataSet.Tables["Departments"]);
525