
- •Credits
- •About the Authors
- •About the Reviewers
- •www.PacktPub.com
- •Table of Contents
- •Preface
- •Introduction
- •Installing Groovy on Windows
- •Installing Groovy on Linux and OS X
- •Executing Groovy code from the command line
- •Using Groovy as a command-line text file editor
- •Running Groovy with invokedynamic support
- •Building Groovy from source
- •Managing multiple Groovy installations on Linux
- •Using groovysh to try out Groovy commands
- •Starting groovyConsole to execute Groovy snippets
- •Configuring Groovy in Eclipse
- •Configuring Groovy in IntelliJ IDEA
- •Introduction
- •Using Java classes from Groovy
- •Embedding Groovy into Java
- •Compiling Groovy code
- •Generating documentation for Groovy code
- •Introduction
- •Searching strings with regular expressions
- •Writing less verbose Java Beans with Groovy Beans
- •Inheriting constructors in Groovy classes
- •Defining code as data in Groovy
- •Defining data structures as code in Groovy
- •Implementing multiple inheritance in Groovy
- •Defining type-checking rules for dynamic code
- •Adding automatic logging to Groovy classes
- •Introduction
- •Reading from a file
- •Reading a text file line by line
- •Processing every word in a text file
- •Writing to a file
- •Replacing tabs with spaces in a text file
- •Deleting a file or directory
- •Walking through a directory recursively
- •Searching for files
- •Changing file attributes on Windows
- •Reading data from a ZIP file
- •Reading an Excel file
- •Extracting data from a PDF
- •Introduction
- •Reading XML using XmlSlurper
- •Reading XML using XmlParser
- •Reading XML content with namespaces
- •Searching in XML with GPath
- •Searching in XML with XPath
- •Constructing XML content
- •Modifying XML content
- •Sorting XML nodes
- •Serializing Groovy Beans to XML
- •Introduction
- •Parsing JSON messages with JsonSlurper
- •Constructing JSON messages with JsonBuilder
- •Modifying JSON messages
- •Validating JSON messages
- •Converting JSON message to XML
- •Converting JSON message to Groovy Bean
- •Using JSON to configure your scripts
- •Introduction
- •Creating a database table
- •Connecting to an SQL database
- •Modifying data in an SQL database
- •Calling a stored procedure
- •Reading BLOB/CLOB from a database
- •Building a simple ORM framework
- •Using Groovy to access Redis
- •Using Groovy to access MongoDB
- •Using Groovy to access Apache Cassandra
- •Introduction
- •Downloading content from the Internet
- •Executing an HTTP GET request
- •Executing an HTTP POST request
- •Constructing and modifying complex URLs
- •Issuing a REST request and parsing a response
- •Issuing a SOAP request and parsing a response
- •Consuming RSS and Atom feeds
- •Using basic authentication for web service security
- •Using OAuth for web service security
- •Introduction
- •Querying methods and properties
- •Dynamically extending classes with new methods
- •Overriding methods dynamically
- •Adding performance logging to methods
- •Adding transparent imports to a script
- •DSL for executing commands over SSH
- •DSL for generating reports from logfiles
- •Introduction
- •Processing collections concurrently
- •Downloading files concurrently
- •Splitting a large task into smaller parallel jobs
- •Running tasks in parallel and asynchronously
- •Using actors to build message-based concurrency
- •Using STM to atomically update fields
- •Using dataflow variables for lazy evaluation
- •Index
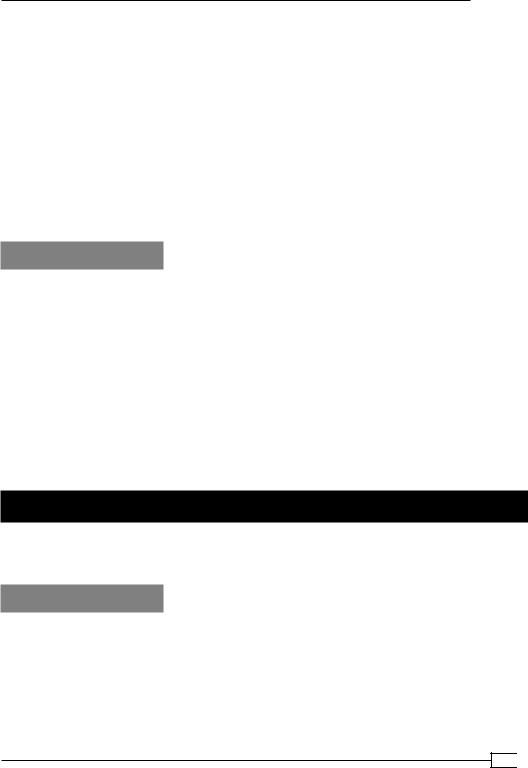
Chapter 4
The previous example is very condensed, but it's not as obvious and easy to read compared to the initially described methods.
Since v1.7.1, Groovy has also introduced a very powerful traverse method in the java. io.File class. In its simplest form, it does the same as eachFileRecurse:
currentDir.traverse { File file -> println file.name
}
The traverse method has an overloaded version that also accepts a Map of additional parameters, which allows you to control various aspects of directory traversing behavior.
More advanced traverse options are described in the Searching for files recipe.
See also
The following recipes contain some additional information on the topics discussed in this recipe:
ff
ff
Searching for files
The Defining code as data in Groovy recipe in Chapter 3, Using Groovy language features
Also, it's worth examining the Groovydoc for the File, FileType, and Closure classes:
ff http://groovy.codehaus.org/groovy-jdk/java/io/File.html
ff http://groovy.codehaus.org/api/groovy/io/FileType.html
ff http://groovy.codehaus.org/api/groovy/lang/Closure.html
Searching for files
In this recipe, we will show how easy it is to filter out files and folders during a search operation on the file system.
Getting ready
Let's assume that we are searching for files in a current working directory. For that, we need to define a variable of the java.io.File type that points to the . path:
def currentDir = new File('.')
153
www.it-ebooks.info
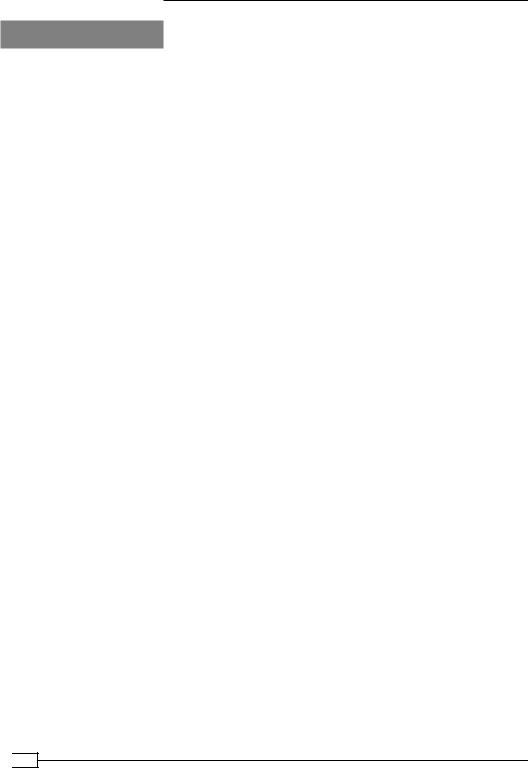
Working with Files in Groovy
How to do it...
As we already mentioned in the Walking through a directory recursively recipe, Groovy adds a powerful traverse method for helping us with our searching task. Let's explore the options that are given by that method:
1.The simplest way to search for specific files is to apply some filtering logic within a closure that we pass to the traverse method:
currentDir.traverse { File file ->
if (file.name.endsWith('.groovy')) { println file.name
}
}
2.The traverse method has another overloaded version that accepts a Map of the parameters. One of those parameters is nameFilter, which should be of the java.util.regex.Pattern type. So, our initial code snippet can be written in the following way:
currentDir.traverse(nameFilter: ~/.*\.groovy/) { File file ->
println file.name
}
3.The traverse method has even more tricks up its sleeve. By specifying the type of the entries (you need to import the groovy.io.FileType class beforehand), we can search for the pattern of the names we want to exclude (excludeNameFilter):
import static groovy.io.FileType.*
...
currentDir.traverse( type: FILES,
nameFilter: ~/.*\.groovy/, excludeNameFilter: ~/^C.*$/) { File file ->
println file.name
}
4.A closure that is passed as a final input argument can also be passed through a visit parameter in the map:
currentDir.traverse( |
|
|
type: |
FILES, |
|
nameFilter: |
{ it.matches(/.*\.groovy/) |
}, |
excludeNameFilter: |
{ it.matches(/^C.*$/) |
}, |
visit: |
{ println it.name |
} |
) |
|
|
154
www.it-ebooks.info
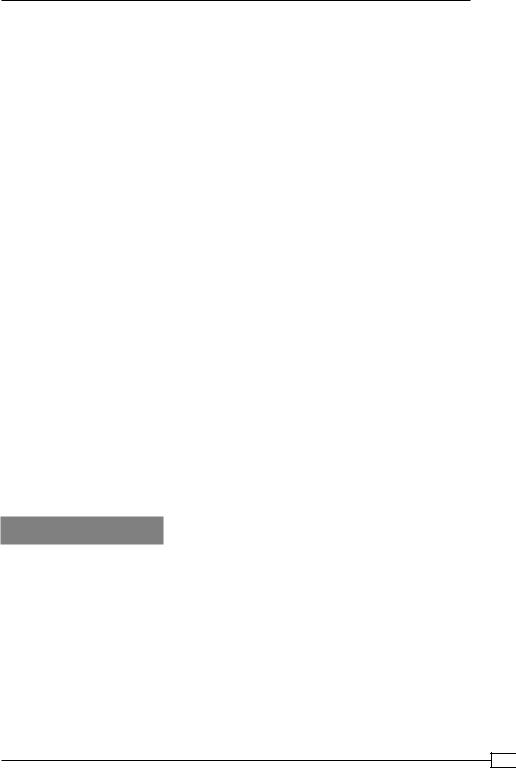
Chapter 4
5.You can also filter on file attributes, other than name, by using the filter/ excludeFilter closure parameters, which are given on java.io.File objects:
def today = new Date()
currentDir.traverse( filter: {
|
|
it.lastModified() < (today-5).time && |
|
|
it.name.endsWith('.groovy') |
|
}, |
|
excludeFilter: { |
it.isDirectory() }, |
|
visit: |
{ |
println it.name } |
)
6.You can prune some directory tree branches by using the preDir (or postDir) closure parameters:
import static groovy.io.FileVisitResult.*
...
currentDir.traverse( |
|
|
preDir: |
{ |
|
|
if (it.name == '.svn') { |
|
|
return SKIP_SUBTREE |
|
|
} |
|
|
}, |
|
nameFilter: |
{ it.matches(/.*\.groovy/) |
}, |
excludeNameFilter: |
{ it.matches(/^C.*$/) |
}, |
visit: |
{ println it.name |
} |
) |
|
|
How it works...
As you can guess, the first code snippet will walk through all the subdirectories and print all Groovy (*.groovy) source file names that are found.
In the Searching strings with regular expressions recipe in Chapter 3, Using Groovy Language Features, we mentioned the ~// operator, which constructs a Pattern object. That's what we use in the second example to match the *.groovy files and print their name again.
In the third step, we add a bit more logic to the code, since we only go through normal files by specifying FileType.FILES in the type parameter. Other possible values are FileType. ANY and FileType.DIRECTORIES. The search also excludes all files starting with C with the help of a regular expression passed to the excludeNameFilter parameter.
155
www.it-ebooks.info
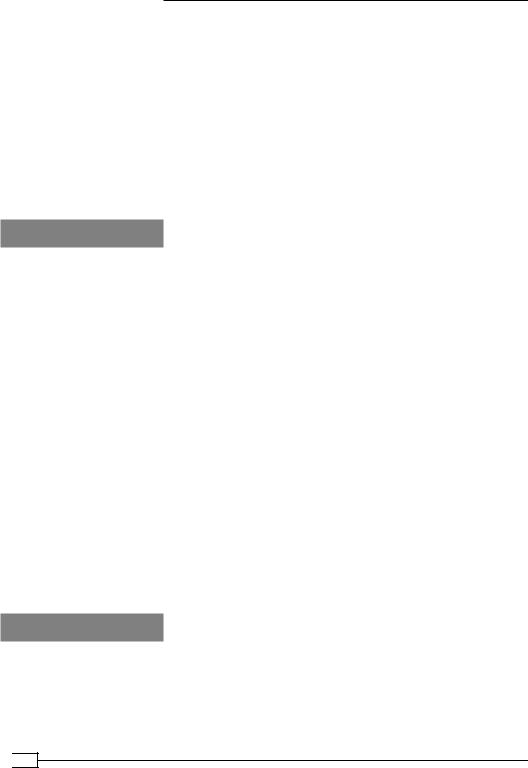
Working with Files in Groovy
The fourth example is identical to the third one, except that all filtering logic is passed through named parameters in a map.
The fifth code snippet prints the names of the files having the *.groovy extension, which are older than 5 days and which are not directories.
The last code example behaves in the same way as the third snippet, but it also excludes the .svn directory and all subdirectories from searching. The closure passed to preDir
returns an instance of the groovy.io.FileVisitResult enumeration, which controls the traversing behavior. Other possible enumeration values are CONTINUE, SKIP_SIBLINGS, and TERMINATE. In general, preDir and postDir can execute any code, and it's not mandatory to return FileVisitResult.
There's more...
There are other useful options available for the traverse method:
ff maxDepth: It says how deep should we go in our search.
ff sort: It is a closure that sets the sorting order in which files and directories will be processed.
ff visitRoot: It indicates that the visit closure should also be called for the target search directory.
ff preRoot: It indicates that the preDir closure should also be called for the target search directory.
ff postRoot: It indicates that postDir closure should also be called for the target search directory.
If you just want to search in the current directory without visiting its subdirectories, you can use the eachFileMatch method instead:
currentDir.eachFileMatch(~/.*\.groovy/) { File file -> println file.name
}
There is also an eachDirMatch method, in case you only need to walk through the matching first-level directories.
See also
An initial introduction to walking through directory trees was given in the Walking through a directory recursively recipe.
156
www.it-ebooks.info