
- •Credits
- •About the Authors
- •About the Reviewers
- •www.PacktPub.com
- •Table of Contents
- •Preface
- •Introduction
- •Installing Groovy on Windows
- •Installing Groovy on Linux and OS X
- •Executing Groovy code from the command line
- •Using Groovy as a command-line text file editor
- •Running Groovy with invokedynamic support
- •Building Groovy from source
- •Managing multiple Groovy installations on Linux
- •Using groovysh to try out Groovy commands
- •Starting groovyConsole to execute Groovy snippets
- •Configuring Groovy in Eclipse
- •Configuring Groovy in IntelliJ IDEA
- •Introduction
- •Using Java classes from Groovy
- •Embedding Groovy into Java
- •Compiling Groovy code
- •Generating documentation for Groovy code
- •Introduction
- •Searching strings with regular expressions
- •Writing less verbose Java Beans with Groovy Beans
- •Inheriting constructors in Groovy classes
- •Defining code as data in Groovy
- •Defining data structures as code in Groovy
- •Implementing multiple inheritance in Groovy
- •Defining type-checking rules for dynamic code
- •Adding automatic logging to Groovy classes
- •Introduction
- •Reading from a file
- •Reading a text file line by line
- •Processing every word in a text file
- •Writing to a file
- •Replacing tabs with spaces in a text file
- •Deleting a file or directory
- •Walking through a directory recursively
- •Searching for files
- •Changing file attributes on Windows
- •Reading data from a ZIP file
- •Reading an Excel file
- •Extracting data from a PDF
- •Introduction
- •Reading XML using XmlSlurper
- •Reading XML using XmlParser
- •Reading XML content with namespaces
- •Searching in XML with GPath
- •Searching in XML with XPath
- •Constructing XML content
- •Modifying XML content
- •Sorting XML nodes
- •Serializing Groovy Beans to XML
- •Introduction
- •Parsing JSON messages with JsonSlurper
- •Constructing JSON messages with JsonBuilder
- •Modifying JSON messages
- •Validating JSON messages
- •Converting JSON message to XML
- •Converting JSON message to Groovy Bean
- •Using JSON to configure your scripts
- •Introduction
- •Creating a database table
- •Connecting to an SQL database
- •Modifying data in an SQL database
- •Calling a stored procedure
- •Reading BLOB/CLOB from a database
- •Building a simple ORM framework
- •Using Groovy to access Redis
- •Using Groovy to access MongoDB
- •Using Groovy to access Apache Cassandra
- •Introduction
- •Downloading content from the Internet
- •Executing an HTTP GET request
- •Executing an HTTP POST request
- •Constructing and modifying complex URLs
- •Issuing a REST request and parsing a response
- •Issuing a SOAP request and parsing a response
- •Consuming RSS and Atom feeds
- •Using basic authentication for web service security
- •Using OAuth for web service security
- •Introduction
- •Querying methods and properties
- •Dynamically extending classes with new methods
- •Overriding methods dynamically
- •Adding performance logging to methods
- •Adding transparent imports to a script
- •DSL for executing commands over SSH
- •DSL for generating reports from logfiles
- •Introduction
- •Processing collections concurrently
- •Downloading files concurrently
- •Splitting a large task into smaller parallel jobs
- •Running tasks in parallel and asynchronously
- •Using actors to build message-based concurrency
- •Using STM to atomically update fields
- •Using dataflow variables for lazy evaluation
- •Index
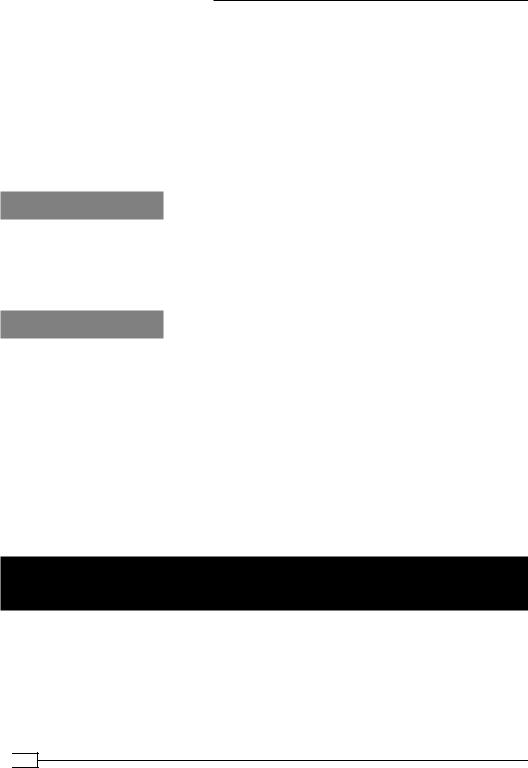
Working with Web Services in Groovy
fragment = 'some_anchor'
path = 'some_folder/some_page.html' query = [param1: 2, param2: 'x']
}
4.With the information passed to URIBuilder, we can create URI, URL, or String instances at any point:
uri.toURI()
uri.toURL()
uri.toString()
How it works...
Internally the URIBuilder class stores all URI components such as, scheme (http:// and https://), user information (username:password), host, port, path, and fragment separately. This allows creating new URLs incrementally and
changing existing URLs without a need to write custom string parsing/concatenating logic.
See also
ff http://groovy.codehaus.org/modules/http-builder/doc/uribuilder. html
ff http://groovy.codehaus.org/modules/http-builder/apidocs/ groovyx/net/http/URIBuilder.html
Other implementations of URI builder functionality can be found in JAX-WS API and Apache's HttpClient available in the following link:
ff http://hc.apache.org/httpcomponents-client-ga/httpclient/ apidocs/org/apache/http/client/utils/URIBuilder.html
ff http://docs.oracle.com/javaee/6/api/javax/ws/rs/core/ UriBuilder.html
Issuing a REST request and parsing a response
A proper introduction to REST is out of scope for this recipe. Countless materials describing the REST architectural style are available both online and offline. Wikipedia's entry on Representational State Transfer at http://en.wikipedia.org/wiki/ Representational_State_Transfer provides a compact description of REST's main concepts, its limits, and the guiding principles to design RESTful applications. A more tongue-in-cheek introduction to REST can be found on Ryan Tomayko's website at
http://tomayko.com/writings/rest-to-my-wife.
272
www.it-ebooks.info
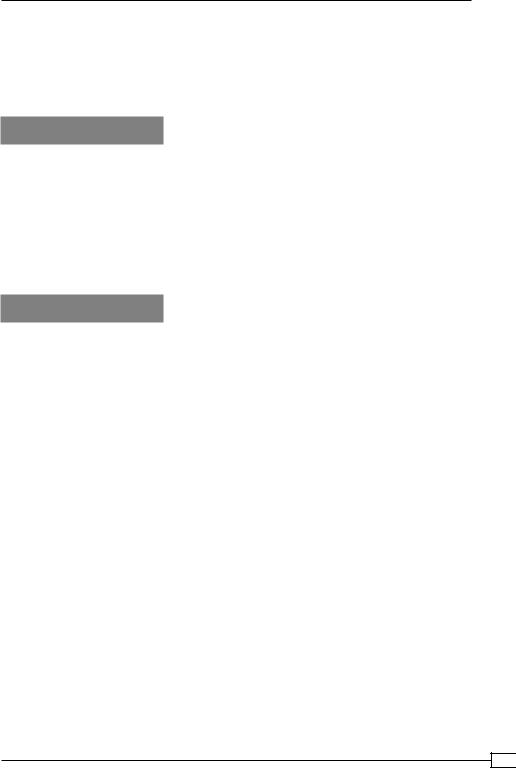
Chapter 8
There are several ways to execute a REST request using Groovy. In this recipe, we are going to show how to execute a GET operation against a RESTful resource using RESTClient from
the HTTPBuilder project (http://groovy.codehaus.org/modules/http-builder/ doc/index.html). HTTPBuilder is a wrapper for Apache HttpClient, with some Groovy syntactical sugar thrown on top.
Getting ready
For this recipe, we will use the RESTful API exposed by the Bing Map Service (http://www.bing.com/maps/). The API allows performing tasks such as, creating a static map with pushpins, geocoding an address, retrieving imagery metadata, or creating a route.
In order to access the service, an authentication key must be obtained through a simple registration process (see https://www.bingmapsportal.com). The key has to be passed with each REST request.
How to do it...
The following steps will use the Location API, through which we will find latitude and longitude coordinates for a specific location:
1.The first step is to create a class. The class has a method, which accepts the information to query the map service with:
@Grab( group='org.codehaus.groovy.modules.http-builder', module='http-builder',
version='0.6'
)
import static groovyx.net.http.ContentType.JSON import static groovyx.net.http.ContentType.XML import groovyx.net.http.RESTClient
class LocationFinder {
static final KEY = '...'
static final URL = 'http://dev.virtualearth.net' static final BASE_PATH = '/REST/v1/Locations/'
def printCityCoordinates(countryCode, city) {
def mapClient = new RESTClient(URL) def path = "${countryCode}/${city}" def response = mapClient.get(
273
www.it-ebooks.info
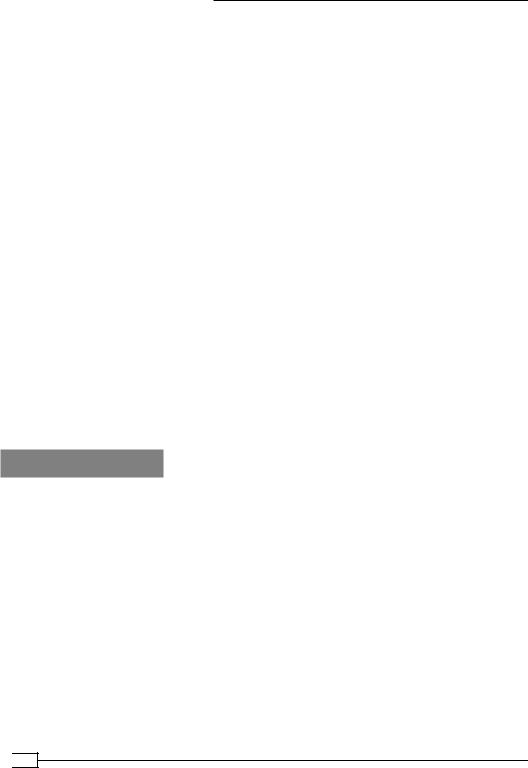
Working with Web Services in Groovy
path: "${BASE_PATH}${path}", query: [key: KEY]
)
assert response.status == 200
assert response.contentType == JSON.toString()
println response. data. resourceSets. resources. point. coordinates
}
}
2.We can use the class in the following way:
LocationFinder map = new LocationFinder() map.printCityCoordinates('fr', 'paris') map.printCityCoordinates('uk', 'london')
3.The code will output the coordinates for Paris and London:
[[[48.856929779052734, 2.3412001132965088]]]
[[[51.506320953369141, -0.12714000046253204]]]
How it works...
The RESTClient class greatly simplifies dealing with REST resources. It leverages the automatic content type parsing and encoding, which essentially parses the response for us and converts it into the proper type. The get method accepts a Map of arguments, including the actual path to the resource, the request content type, query, and headers. For a full list of arguments, refer to the Javadoc at http://groovy.codehaus.org/modules/httpbuilder/apidocs/groovyx/net/http/HTTPBuilder.RequestConfigDelegate. html#setPropertiesFromMap(java.util.Map).
In the previous example, the response is automatically parsed and transformed into a nested data structure, which makes dealing with the response values a very simple affair.
274
www.it-ebooks.info
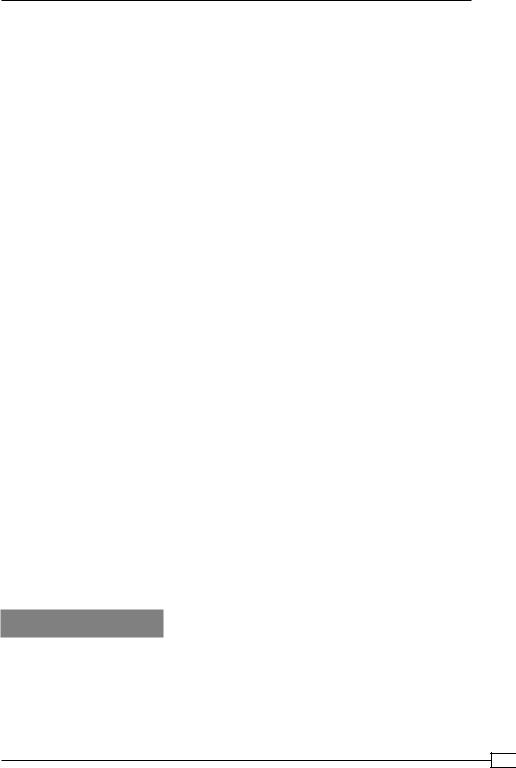
Chapter 8
By default, all request methods return an HttpResponseDecorator instance, which provides convenient access to the response headers and the parsed response body. The response body is parsed based on the content type. HTTPBuilder's default response handlers function in the same way: the response data is parsed (or buffered in the case of a binary or text response) and returned from the request method. The response either carries no data, or it is expected to be parsable and transformed into some object, which is always accessible through the getData() method.
The actual data returned by the service looks like the following code snippet:
{
"authenticationResultCode": "ValidCredentials", "brandLogoUri": "...",
"copyright": "...", "resourceSets": [
{
"estimatedTotal": 1, "resources": [
{
"name": "Paris, Paris, France", "point": {
"type": "Point", "coordinates": [
48.856929779053,
2.3412001132965
]
},
...
}
]
}
],
"statusCode": 200, "statusDescription": "OK", "traceId": "..."
}
See also
ff http://groovy.codehaus.org/modules/http-builder/doc/rest.html
ff http://msdn.microsoft.com/en-us/library/ff701713.aspx ff https://www.bingmapsportal.com
275
www.it-ebooks.info