
- •Credits
- •About the Authors
- •About the Reviewers
- •www.PacktPub.com
- •Table of Contents
- •Preface
- •Introduction
- •Installing Groovy on Windows
- •Installing Groovy on Linux and OS X
- •Executing Groovy code from the command line
- •Using Groovy as a command-line text file editor
- •Running Groovy with invokedynamic support
- •Building Groovy from source
- •Managing multiple Groovy installations on Linux
- •Using groovysh to try out Groovy commands
- •Starting groovyConsole to execute Groovy snippets
- •Configuring Groovy in Eclipse
- •Configuring Groovy in IntelliJ IDEA
- •Introduction
- •Using Java classes from Groovy
- •Embedding Groovy into Java
- •Compiling Groovy code
- •Generating documentation for Groovy code
- •Introduction
- •Searching strings with regular expressions
- •Writing less verbose Java Beans with Groovy Beans
- •Inheriting constructors in Groovy classes
- •Defining code as data in Groovy
- •Defining data structures as code in Groovy
- •Implementing multiple inheritance in Groovy
- •Defining type-checking rules for dynamic code
- •Adding automatic logging to Groovy classes
- •Introduction
- •Reading from a file
- •Reading a text file line by line
- •Processing every word in a text file
- •Writing to a file
- •Replacing tabs with spaces in a text file
- •Deleting a file or directory
- •Walking through a directory recursively
- •Searching for files
- •Changing file attributes on Windows
- •Reading data from a ZIP file
- •Reading an Excel file
- •Extracting data from a PDF
- •Introduction
- •Reading XML using XmlSlurper
- •Reading XML using XmlParser
- •Reading XML content with namespaces
- •Searching in XML with GPath
- •Searching in XML with XPath
- •Constructing XML content
- •Modifying XML content
- •Sorting XML nodes
- •Serializing Groovy Beans to XML
- •Introduction
- •Parsing JSON messages with JsonSlurper
- •Constructing JSON messages with JsonBuilder
- •Modifying JSON messages
- •Validating JSON messages
- •Converting JSON message to XML
- •Converting JSON message to Groovy Bean
- •Using JSON to configure your scripts
- •Introduction
- •Creating a database table
- •Connecting to an SQL database
- •Modifying data in an SQL database
- •Calling a stored procedure
- •Reading BLOB/CLOB from a database
- •Building a simple ORM framework
- •Using Groovy to access Redis
- •Using Groovy to access MongoDB
- •Using Groovy to access Apache Cassandra
- •Introduction
- •Downloading content from the Internet
- •Executing an HTTP GET request
- •Executing an HTTP POST request
- •Constructing and modifying complex URLs
- •Issuing a REST request and parsing a response
- •Issuing a SOAP request and parsing a response
- •Consuming RSS and Atom feeds
- •Using basic authentication for web service security
- •Using OAuth for web service security
- •Introduction
- •Querying methods and properties
- •Dynamically extending classes with new methods
- •Overriding methods dynamically
- •Adding performance logging to methods
- •Adding transparent imports to a script
- •DSL for executing commands over SSH
- •DSL for generating reports from logfiles
- •Introduction
- •Processing collections concurrently
- •Downloading files concurrently
- •Splitting a large task into smaller parallel jobs
- •Running tasks in parallel and asynchronously
- •Using actors to build message-based concurrency
- •Using STM to atomically update fields
- •Using dataflow variables for lazy evaluation
- •Index
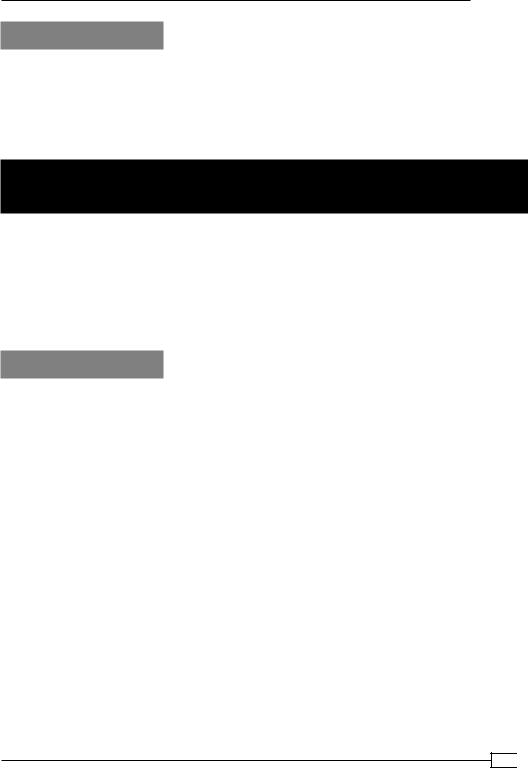
Chapter 6
See also
ff Constructing JSON messages with JsonBuilder
ff The Reading XML using XmlSlurper recipe in Chapter 5, Working with XML in Groovy
ff http://www.json.org/
ff http://groovy.codehaus.org/gapi/groovy/json/JsonSlurper.html
Constructing JSON messages with JsonBuilder
This recipe provides an overview of another class introduced in Groovy 1.8, which helps to construct JSON messages, the JsonBuilder.
This class works like any other builder class in Groovy (see the Defining data structures as code in Groovy recipe from Chapter 3, Using Groovy Language Features). A data structure based on Lists and Maps is defined, and JSON is split out when the string representation is requested.
How to do it...
The following steps will show some examples of using JsonBuilder.
1.Let's start right away with a simple script that builds the representation of a fictional customer:
import groovy.json.JsonBuilder
def builder = new JsonBuilder() builder.customer {
name 'John' lastName 'Appleseed' address {
streetName 'Gordon street' city 'Philadelphia' houseNumber 20
}
}
println builder.toPrettyString()
201
www.it-ebooks.info
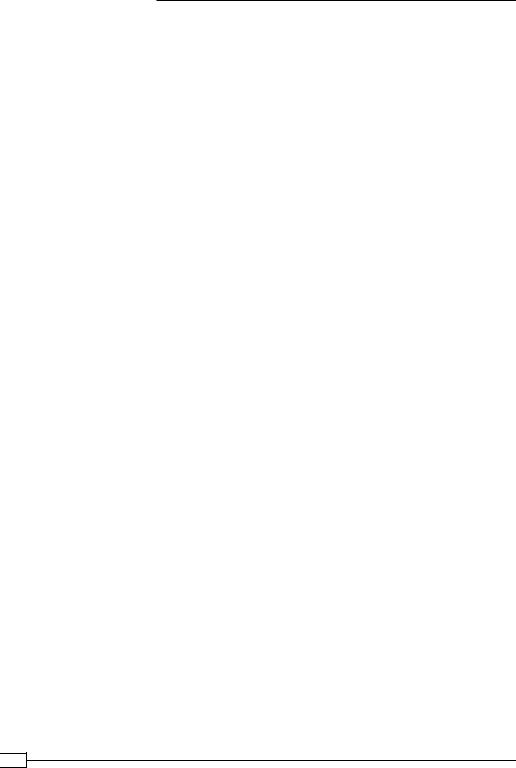
Working with JSON in Groovy
2.The output of the previous script yields:
{
"customer": { "name": "John",
"lastName": "Appleseed", "address": {
"streetName": "Gordon street", "city": "Philadelphia", "houseNumber": 20
}
}
}
3.Here is a slightly more complex example of JsonBuilder usage, used to build the definition of a chart widget:
def chart = [ items: [
type: 'chart', height: 200, width: 300, axes: [
{
type 'Time' fields ([ 'x' ]) position 'left' title 'Time'
},
{
type 'Numeric' fields ( [ 'y' ] ) position 'bottom' title 'Profit in EUR'
}
]
]
]
def builder = new JsonBuilder(chart) println builder.toPrettyString()
The output of the previous statement is:
{
"items": [
{
202
www.it-ebooks.info
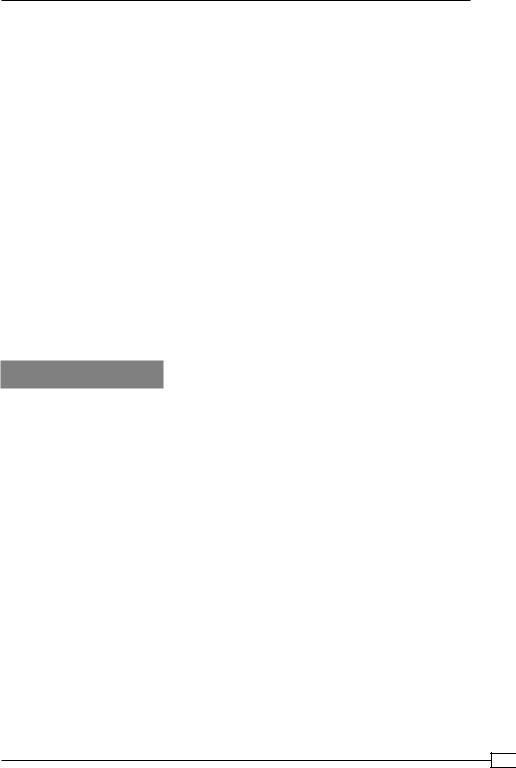
Chapter 6
"type": "chart", "height": 200, "width": 300, "axes": [
{
"type": "Time", "fields": [ "x" ], "position": "left", "title": "Time"
},
{
"type": "Numeric", "fields": [ "y" ], "position": "bottom", "title": "Profit in EUR"
}
]
}
]
}
How it works...
JsonBuilder can produce JSON-formatted output from Maps, Lists, and Java Beans (see the Converting JSON message to Groovy Bean recipe). The output is stored in memory, and it can be written to a stream or processed further. If we want to directly stream (instead of storing
it in memory) the data as it's created, we can use StreamingJsonBuilder instead of the
JsonBuilder.
In the example at step 1, we create an instance of the JsonBuilder class and we simply define the key/value pairs that contain the data structure we wish to create. The approach is very similar to any other builder, such as MarkupBuilder (see the Constructing XML content recipe in Chapter 5, Working with XML in Groovy).
In the second example, the builder is directly created by passing a variable containing a Map. The Map's keys are Strings as defined by the JSON standard, but the assigned values can be one of the following types:
ff One of the simple data type (for example, Boolean and String) permitted by JSON ff Another Map
ff Collection of the above
ff A closure or a collection of closures
203
www.it-ebooks.info

Working with JSON in Groovy
To demonstrate the flexibility of JsonBuilder in our example, we used different types, including closures.
See also
ff Parsing JSON messages with JsonSlurper
ff The Constructing XML content recipe in Chapter 5, Working with XML in Groovy
ff http://groovy.codehaus.org/gapi/groovy/json/JsonBuilder.html
Modifying JSON messages
After we've got acquainted with a way to read existing JSON messages (see the Parsing JSON messages with JsonSlurper recipe) and create our own (see Constructing JSON messages with JsonBuilder recipe), we need to have the ability to modify the messages that flow through our system.
This recipe will show how straightforward it is to alter the content of a JSON document in Groovy.
How to do it...
Let's use the same JSON data located in the ui.json file that we used in the Parsing JSON messages with JsonSlurper recipe.
1.First of all we need to load and parse the JSON file: import groovy.json.*
def reader = new FileReader('ui.json') def ui = new JsonSlurper().parse(reader)
Since the data is actually just a nested structure, which consists of Maps, Lists and primitive data types, we can use the same API we would use for collections to navigate and change JSON data.
2.Consider the following snippet of code, which changes and removes some bits of the original JSON message:
ui.items[0].type = 'panel' ui.items[0].title = 'Main Window' ui.items[0].remove('axes') ui.items[0].remove('series')
204
www.it-ebooks.info