
- •Credits
- •About the Authors
- •About the Reviewers
- •www.PacktPub.com
- •Table of Contents
- •Preface
- •Introduction
- •Installing Groovy on Windows
- •Installing Groovy on Linux and OS X
- •Executing Groovy code from the command line
- •Using Groovy as a command-line text file editor
- •Running Groovy with invokedynamic support
- •Building Groovy from source
- •Managing multiple Groovy installations on Linux
- •Using groovysh to try out Groovy commands
- •Starting groovyConsole to execute Groovy snippets
- •Configuring Groovy in Eclipse
- •Configuring Groovy in IntelliJ IDEA
- •Introduction
- •Using Java classes from Groovy
- •Embedding Groovy into Java
- •Compiling Groovy code
- •Generating documentation for Groovy code
- •Introduction
- •Searching strings with regular expressions
- •Writing less verbose Java Beans with Groovy Beans
- •Inheriting constructors in Groovy classes
- •Defining code as data in Groovy
- •Defining data structures as code in Groovy
- •Implementing multiple inheritance in Groovy
- •Defining type-checking rules for dynamic code
- •Adding automatic logging to Groovy classes
- •Introduction
- •Reading from a file
- •Reading a text file line by line
- •Processing every word in a text file
- •Writing to a file
- •Replacing tabs with spaces in a text file
- •Deleting a file or directory
- •Walking through a directory recursively
- •Searching for files
- •Changing file attributes on Windows
- •Reading data from a ZIP file
- •Reading an Excel file
- •Extracting data from a PDF
- •Introduction
- •Reading XML using XmlSlurper
- •Reading XML using XmlParser
- •Reading XML content with namespaces
- •Searching in XML with GPath
- •Searching in XML with XPath
- •Constructing XML content
- •Modifying XML content
- •Sorting XML nodes
- •Serializing Groovy Beans to XML
- •Introduction
- •Parsing JSON messages with JsonSlurper
- •Constructing JSON messages with JsonBuilder
- •Modifying JSON messages
- •Validating JSON messages
- •Converting JSON message to XML
- •Converting JSON message to Groovy Bean
- •Using JSON to configure your scripts
- •Introduction
- •Creating a database table
- •Connecting to an SQL database
- •Modifying data in an SQL database
- •Calling a stored procedure
- •Reading BLOB/CLOB from a database
- •Building a simple ORM framework
- •Using Groovy to access Redis
- •Using Groovy to access MongoDB
- •Using Groovy to access Apache Cassandra
- •Introduction
- •Downloading content from the Internet
- •Executing an HTTP GET request
- •Executing an HTTP POST request
- •Constructing and modifying complex URLs
- •Issuing a REST request and parsing a response
- •Issuing a SOAP request and parsing a response
- •Consuming RSS and Atom feeds
- •Using basic authentication for web service security
- •Using OAuth for web service security
- •Introduction
- •Querying methods and properties
- •Dynamically extending classes with new methods
- •Overriding methods dynamically
- •Adding performance logging to methods
- •Adding transparent imports to a script
- •DSL for executing commands over SSH
- •DSL for generating reports from logfiles
- •Introduction
- •Processing collections concurrently
- •Downloading files concurrently
- •Splitting a large task into smaller parallel jobs
- •Running tasks in parallel and asynchronously
- •Using actors to build message-based concurrency
- •Using STM to atomically update fields
- •Using dataflow variables for lazy evaluation
- •Index

6
Working with JSON in Groovy
In this chapter, we will cover:
ff
ff
ff
ff
ff
ff
ff
Parsing JSON messages with JsonSlurper Constructing JSON messages with JsonBuilder Modifying JSON messages
Validating JSON messages Converting JSON message to XML
Converting JSON message to Groovy Bean
Using JSON to configure your scripts
Introduction
JSON (JavaScript Object Notation) (http://www.json.org/) is a data interchange format, similar in scope to XML or CSV. It uses a very lightweight serialization form and works very well for exchanging data between a web client (a browser) and a remote server, but it is not limited to only that.
JSON is also a subset of the object literal notation of JavaScript. Being a subset of JavaScript implies that a JSON payload can be processed by the language natively. This is the main reason why JSON has become such a popular format for transferring serialized data between a remote API (often a RESTful API) and a JavaScript client.
www.it-ebooks.info
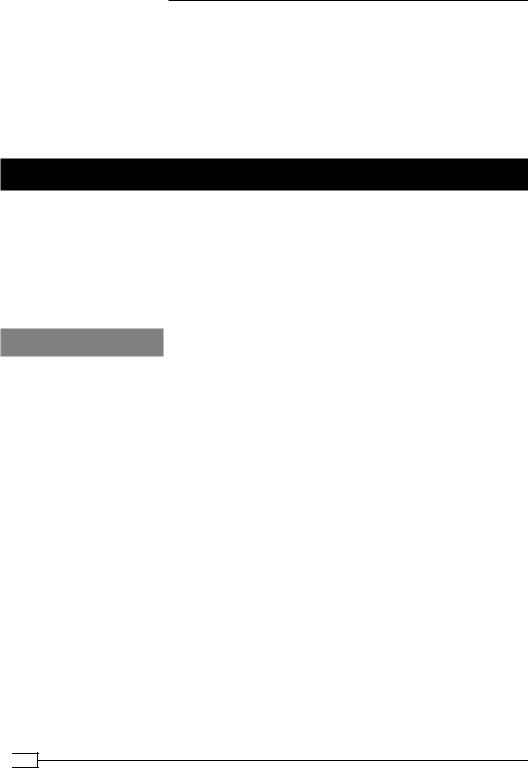
Working with JSON in Groovy
But JSON is also very lightweight—especially if we compare it to the very chatty XML, the dethroned king of serialization—making it a suitable candidate for a general purpose data processing format (if you can live with some of its limitations such as the lack of namespace or poor complex data types support).
This chapter's recipes introduce the reader to Groovy native support for reading and producing JSON. We also look at some more advanced recipes such as conversion from and to XML and Groovy Beans.
Parsing JSON messages with JsonSlurper
The first thing you probably want to do with the JSON data is to parse it. JSON support was introduced in Groovy 1.8. The class for consuming JSON is named JsonSlurper and it
behaves similarly to the XmlSlurper, presented in the Reading XML using XmlSlurper recipe in Chapter 5, Working with XML in Groovy.
This recipe introduces the reader to JsonSlurper and shows how to parse a JSON file and how to navigate the data structure created by JsonSlurper.
Getting ready
Let's assume we have a ui.json file which stores a definition of the UI layout for some
JavaScript framework:
{
"items":[
{
"type":"chart",
"height":270,
"width":319,
"animate":true,
"insetPadding":20,
"axes":[
{
"type":"Time", "fields":[ "x" ], "position":"left", "title":"Time"
},
{
"type":"Numeric", "fields":[ "y" ], "position":"bottom", "title":"Profit in EUR"
198
www.it-ebooks.info
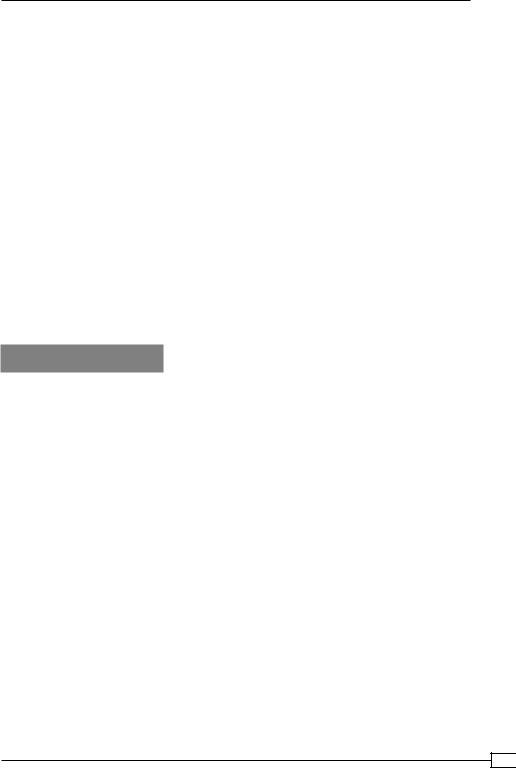
Chapter 6
}
],
"series":[
{
"type":"bar",
"label":{
"display":"insideEnd",
"field":"y",
"color":"#333", "text-anchor":"middle"
},
"axis":"bottom",
"xField":"x", "yField":[ "y" ]
}
]
}
]
}
How to do it...
The JSON data can be easily parsed with the help of the groovy.json.JsonSlurper class.
1.The groovy.json package is not included in the list of packages imported by default. For this reason, you need to explicitly import JsonSlurper:
import groovy.json.JsonSlurper
def reader = new FileReader('ui.json') def ui = new JsonSlurper().parse(reader)
The parse method of JsonSlurper accepts a File or a Reader object. It is also possible to pass a String to JsonSlurper by invoking the parseText method.
2.After the JSON payload is successfully parsed, you can easily navigate through the resulting object using the familiar GPath-like expressions and closures:
ui.items.each { println it.type }
println ui.items[0]
.axes
.find { it.fields.contains('y')
}.title
199
www.it-ebooks.info

Working with JSON in Groovy
3.The complete output of the script looks like:
chart
Profit in EUR
How it works...
The first line in step 2 iterates through all the elements in the items node, which is a top-level collection in our JSON object and it prints the value of the type attribute of each of those objects. Since we have only one object in that collection it only prints chart in return.
The second line uses a slightly more complex expression. The expression's goal is simply to find the title used by the y axis.
The expression selects the first element of the items collection and subsequently gets the axes collection. It then searches the axes collection for the first element of the nested fields collection having the y value. Finally, it returns the title of the found element.
Unlike XmlSlurper, which uses an intermediate set of classes to present the XML tree, JsonSlurper transforms a JSON message to a nested combination of Maps and Lists.
For example, to show the actual classes used behind the scenes, we can fire up the following code:
println ui.getClass() println ui.items.getClass()
It will print out the following class names:
class java.util.HashMap class java.util.ArrayList
Knowing that the structure created by JsonSlurper is backed by Groovy's Collections, you can easily leverage all the Collection extension methods (such as each, find, and
collect) available in Groovy to build expressions that search and modify your JSON objects.
There's more...
JsonSlurper is rather well-suited for most parsing needs. On the other hand, there are plenty of libraries available in the Java/Groovy ecosystem, which offer similar functionality:
ff Google GSON: http://code.google.com/p/google-gson/ ff JSONLib: http://json-lib.sourceforge.net/
ff Jackson: http://jackson.codehaus.org/
ff JSON.simple: http://code.google.com/p/json-simple/
200
www.it-ebooks.info