
ASP.NET 2.0 Everyday Apps For Dummies (2006)
.pdf
Chapter 3: Designing Secure ASP.NET Applications |
57 |
Passport authentication: Uses Microsoft’s Passport service to authenticate users. When you use Passport authentication, a user must have a valid Passport account to access the application. Of the three authentication modes, this one is the least used.
The rest of this chapter (and the rest of this book) assumes you will use forms-based authentication when you need to restrict access to your Web applications.
Configuring forms-based authentication
To configure an ASP.NET application to use forms-based authentication, add an <authentication> element to the <system.web> section of the application’s root web.config file. In its simplest form, this element looks like this:
<system.web>
<authentication mode=”Forms” /> </system.web>
That’s all you need do to enable the authentication of forms, if you’re okay with using the default settings. If you want, you can customize the settings by adding a <forms> subelement beneath the <authentication> element. Here’s an example:
<system.web>
<authentication mode=”Forms” > <forms loginUrl=”Login.aspx” />
</authentication>
</system.web>
Here, forms-based authentication is configured so that the login page is named Signin.aspx rather than the default Login.aspx.
You can also add a <Credentials> element that lists the names and passwords of the users. However, it’s considered bad form (groan) to list the passwords directly in the web.config file because of security risks. So a separate database is usually used instead.
Configuring authorization
Authorization — that is, the process of determining which users are allowed to access what resources in a Web application — is another capability you configure via the web.config file. The key to configuring authorization is realizing two vital aspects of how it works:
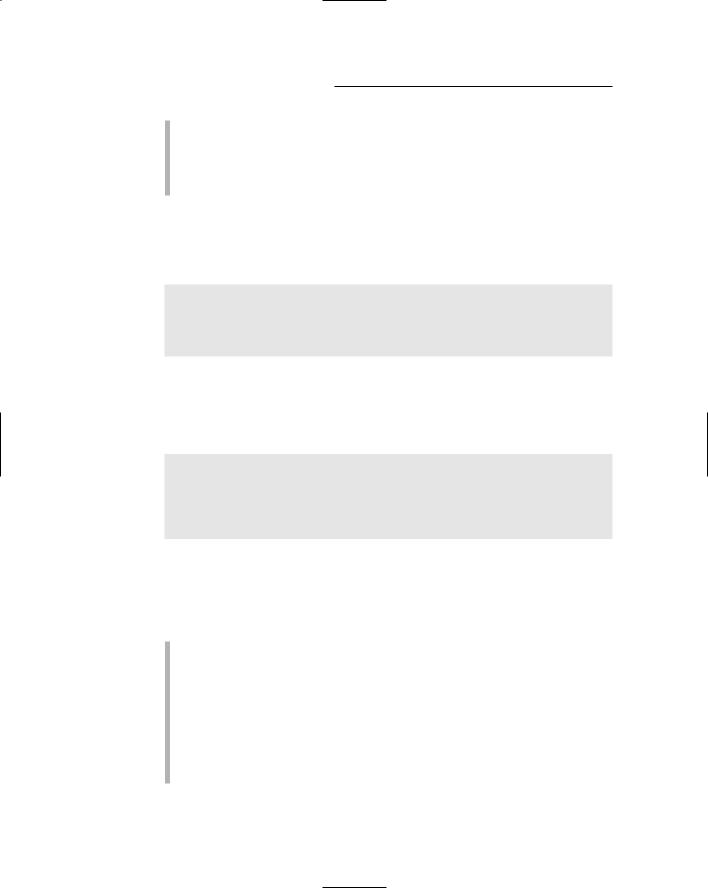
58 |
Part II: Building Secure Applications |
Each folder within a Web application can have its own web.config file.
The authorization settings in a given web.config file apply only to the files in the folder where a web.config file appears, plus any subfolders. (Note, however, that a subfolder can have its own web.config file, which overrides the settings it gets from the parent folder’s web.config file.)
To configure access restrictions for the files in a folder, add a web.config file to the folder, then add an <authorization> element to the web.config file’s <system.web> element. Then, add one or more <allow> or <deny> elements. Here’s an example:
<system.web>
<authorization>
<deny users=”?” /> </authorization>
</system.web>
In this example, the ? wildcard prohibits anonymous users. As a result, only authenticated users will be allowed to access the pages in the folder where this web.config file appears.
You can also allow or deny access to specific users. Here’s an example:
<system.web>
<authorization>
<allow users=”Tom, Dick, Harry” />
<deny users=”*” /> </authorization>
</system.web>
Here, the <allow> element grants access to users named Tom, Dick, and Harry, and the <deny> element uses a wildcard to deny access to all other users.
Here are a few additional points regarding these elements:
The ? wildcard refers only to users who haven’t logged in. The * wildcard refers to all users, whether they’ve logged in or not.
If you list more than one user name in an <allow> or <deny> element, separate the names with commas.
The order in which you list the <allow> and <deny> elements is important. As soon as ASP.NET finds an <allow> or <deny> rule that applies to the current user, the rule is applied and any remaining elements are ignored. Thus, you should list rules that list specific users first, followed by rules that use the ? wildcard, followed by rules that use the * wildcard.
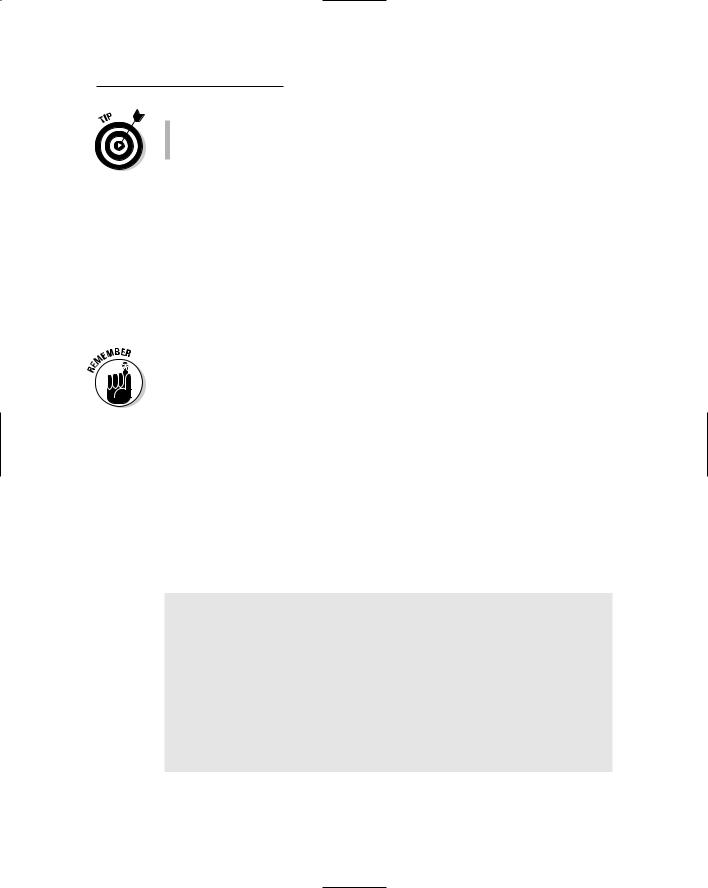
Chapter 3: Designing Secure ASP.NET Applications |
59 |
If you don’t want to mess with coding the authorization elements yourself, you can use the new browser-based configuration tool instead. From Visual Studio, choose Web Site ASP.NET Configuration to access this tool.
Understanding membership providers
Prior to ASP.NET 2.0, you had to develop custom programming to store and retrieve user data from a database. Now, ASP.NET 2.0 uses a provider model that provides a standardized interface to the objects that maintain the useraccount information. In addition, ASP.NET 2.0 comes with a standard membership provider, an application that stores basic user-account information in a SQL Server database. The Login controls are designed to work with this membership provider.
If the standard provider isn’t adequate to your needs, you can write your own membership provider to use instead. As long as your custom provider conforms to the ASP.NET membership provider interface, the Login controls can work with it.
To create a custom provider, you simply create a class that inherits the abstract class System.Web.Security.MembershipProvider. This class requires that you implement about two dozen properties and methods. (For example, you must implement a CreateUser method that creates a user account and a ValidateUser method that validates a user based on a name and password. For openers.) For more information about creating your own membership provider, refer to the Help.
After you’ve created a custom membership provider, you can use settings in the web.config file to indicate that you want to use it instead of the standard membership provider. Here’s an example:
<system.web>
<membership defaultProvider=”MyMemberProvider” > <providers>
<add name=”MyMemberProvider” type=”MyNameSpace.MyMemberProvider” connectionStringName=”MyProviderConn” enablePasswordRetrieval=”false” enablePasswordReset=”true” requiresQuestionAndAnswer=”true”
/>
</providers>
</membership>
</system.web>
Here, a custom membership provider named MyMemberProvider is created using the class MyNameSpace.MyMemberProvider. This provider is designated as the default provider, used throughout the application unless you specify otherwise.
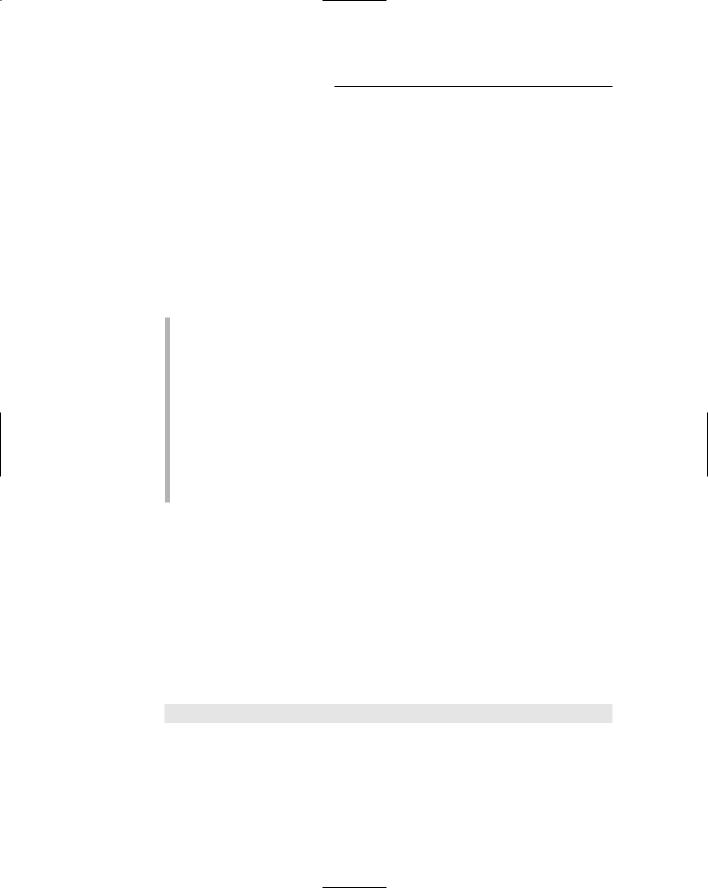
60 |
Part II: Building Secure Applications |
Fortunately, the standard membership provider is suitable for most applications. So you don’t have to create your own membership provider unless your application has unusual requirements.
Using ASP.NET Login Controls
One of the most useful new features in ASP.NET 2.0 is a suite of seven controls designed to simplify applications that authenticate users. In Visual Studio 2005, these controls are located in the toolbox under the Login tab. The seven controls are as follows:
Login: Lets the user log in by entering a user name and password.
CreateUserWizard: Lets the user create a new user account.
PasswordRecovery: Lets the user retrieve a forgotten password.
ChangePassword: Lets the user change his or her password.
LoginView: Displays the contents of a template based on the user’s login status.
LoginStatus: If the user is logged in, displays a link that logs the user out. If the user isn’t logged in, displays a link that leads to the application’s login page.
LoginName: Displays the user’s login name if the user is logged in.
The following sections describe the basics of using each of these controls.
Using the Login control
The Login control provides a convenient way to let users log in to an application. You should use the Login control on a page named Login.aspx. That way, the Login control will be displayed automatically whenever a user tries to access a page that requires the user to be logged in.
In its simplest form, the Login control looks like this:
<asp:Login id=”Login1” runat=”Server” />
The Login control displays text boxes that let the user enter a user name and password. When those are filled in, the Login control uses the membership provider to look up the user name and password in the membership database. If the user name and password are valid, the user is logged in and the page that was originally requested is displayed. If not, an error message is displayed and the user is not logged in.
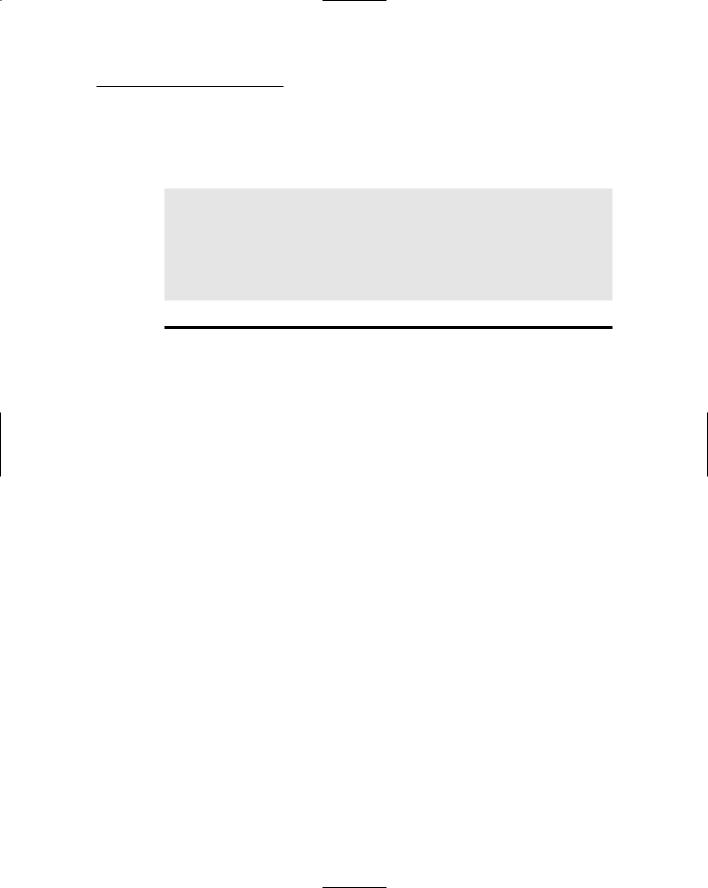
Chapter 3: Designing Secure ASP.NET Applications |
61 |
You can customize the Login control by using any of the optional attributes listed in Table 3-1. For example, here’s a Login control that uses custom titles and displays links to a new user-registration page and a passwordrecovery page:
<asp:Login id=”Login1” runat=”Server”
TitleText=”Please enter your user name and password:” UserNameLabelText=”Your user name:” PasswordLabelText=”Your password:”
CreateUserText=”Register as a new user”
CreateUserUrl=”~/Login/Register.aspx”
PasswordRecoveryText=”Forgot your password?”
PasswordRecoveryUrl=”~/Login/Recover.aspx” />
Table 3-1 |
Attributes for the Login control |
Attribute |
Explanation |
id |
The ID associated with the Login control. |
|
|
runat |
Runat=”Server” is required for all ASP.NET |
|
server controls. |
|
|
CreateUserIconUrl |
The URL of an image used as a link to a page that |
|
registers a new user. |
|
|
CreateUserText |
Text that’s displayed as a link to a page that registers |
|
a new user. |
|
|
CreateUserUrl |
The URL of a page that registers a new user. |
|
|
DestinationPageUrl |
Sets the URL of the page that’s displayed when the |
|
user successfully logs in. If this attribute is omitted, |
|
the page that was originally requested is displayed. |
|
|
DisplayRememberMe |
A Boolean that indicates whether the Login control |
|
should display a check box that lets the user choose |
|
to leave a cookie so the user can automatically be |
|
logged in in the future. |
|
|
FailureText |
The text message that’s displayed if the user name or |
|
password is incorrect. The default reads: Your |
|
login attempt has failed. Please |
|
try again. |
|
|
InstructionText |
A text value displayed immediately beneath the title |
|
text, intended to provide login instructions for the |
|
user. The default is an empty string. |
|
|
LoginButtonText |
The text that’s displayed on the Login button. |
|
|
(continued)
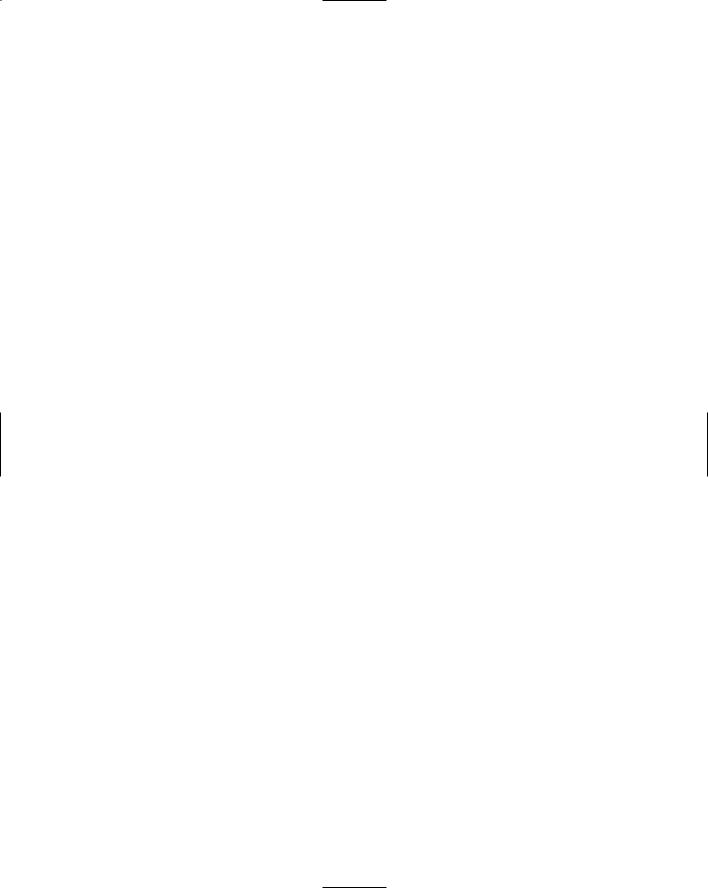
62 |
Part II: Building Secure Applications |
|
|
|
|
|
|||
|
|
|
|
|
|
|
Table 3-1 (continued) |
|
|
|
|
Attribute |
Explanation |
|
|
|
LoginButtonType |
The button type for the Login button. You can |
|
|
|
|
|
specify Button, Link, or Image. (If you |
|
|
|
|
specify Image, then you should also specify |
|
|
|
|
the LoginButtonImageUrl attribute.) |
|
|
|
|
|
|
|
Orientation |
Specifies the layout of the login controls. You |
|
|
|
|
|
can specify Horizontal or Vertical. |
|
|
|
|
The default is Vertical. |
|
|
|
|
|
|
|
PasswordLabelText |
The text that’s displayed in the label that |
|
|
|
|
|
identifies the Password field. |
|
|
|
|
|
|
|
PasswordRecoveryIconUrl |
The URL of an image used as a link to a page |
|
|
|
|
|
that recovers a lost password. |
|
|
PasswordRecoveryText |
Text that’s displayed as a link to a page that |
|
|
|
|
|
recovers a lost password. |
|
|
|
|
|
|
|
PasswordRecoveryUrl |
The URL of a page that recovers a lost |
|
|
|
|
|
password. |
|
|
|
|
|
|
|
RememberMeText |
The text displayed for the Remember Me |
|
|
|
|
|
check box. |
|
|
TextLayout |
Specifies the position of the labels relative to |
|
|
|
|
|
the user name and password text boxes. If |
|
|
|
|
you specify TextOnLeft, the labels |
|
|
|
|
appear to the left of the text boxes. If you |
|
|
|
|
specify TextOnTop, the labels appear |
|
|
|
|
above the text boxes. |
|
|
|
|
|
|
|
TitleText |
The text that’s displayed at the top of the |
|
|
|
|
|
Login control. |
|
|
UserNameLabelText |
The text that’s displayed in the label that |
|
|
|
|
|
identifies the User Name field. |
|
|
|
|
|
Using the CreateUserWizard control
The CreateUserWizard control automates the task of entering the information for a new user and creating a record for the user in the membership database. It displays text boxes that let the user enter a user name, a password, an e-mail address, a security question, and the answer to the security question. Note that there are actually two text boxes for the password; the user must enter the same password in both text boxes.
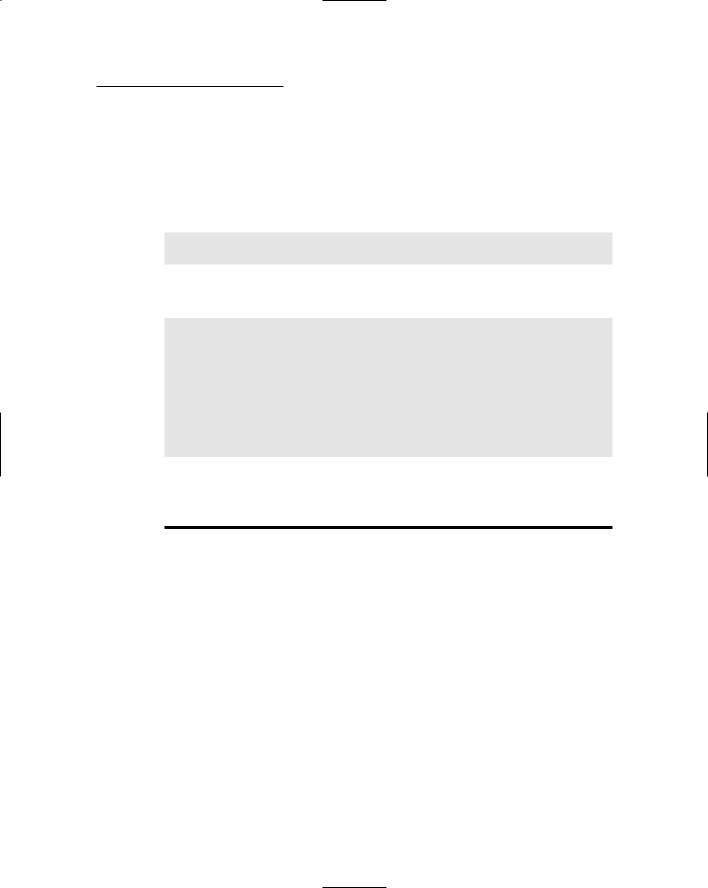
Chapter 3: Designing Secure ASP.NET Applications |
63 |
When the user clicks the New User button, the CreateUserWizard control attempts to create a new user account, basing it on the information entered by the user. If the account is successfully created, the user is logged in to the new account. If the account can’t be created (for example, because an account with the same user name already exists), an error message is displayed.
In its simplest form, the CreateUserWizard control looks like this:
<asp:CreateUserWizard id=”CreateUserWizard1”
runat=”Server” />
You can customize the CreateUserWizard control with the attributes listed in Table 3-2. Here’s an example:
<asp:CreateUserWizard id=”CreateUserWizard1” runat=”Server”
HeaderText=”New User Registration” InstructionText=”Please enter your user information:” UserNameLabelText=”Your user name:”
PasswordLabelText=”Your password:”
ConfirmPasswordLabelText=”Confirm password:”
EmailLabelText=”Your e-mail address:”
QuestionLabelText=”Your secret question:”
AnswerLabelText=”The answer:” />
In this example, attributes are used to set the text values displayed by the labels that identify the data-entry text boxes.
Table 3-2 |
Attributes for the CreateUserWizard control |
|
Attribute |
|
Explanation |
id |
|
The ID associated with the |
|
|
CreateUserWizard control. |
runat |
|
Runat=”Server” is required for all |
|
|
ASP.NET server controls. |
AnswerLabelText |
The text that’s displayed in the label for the |
|
|
|
Answer field. |
CancelButtonImageUrl |
The URL of an image used on the Cancel |
|
|
|
button. |
CancelButtonText |
The text displayed on the Cancel button. |
|
|
|
|
CancelButtonType |
The button type for the Cancel button. You can |
|
|
|
specify Button, Link, or Image. |
|
|
|
CancelDestinationPageUrl |
The URL of the page that’s displayed if the |
|
|
|
user clicks the Cancel button. |
|
|
|
(continued)
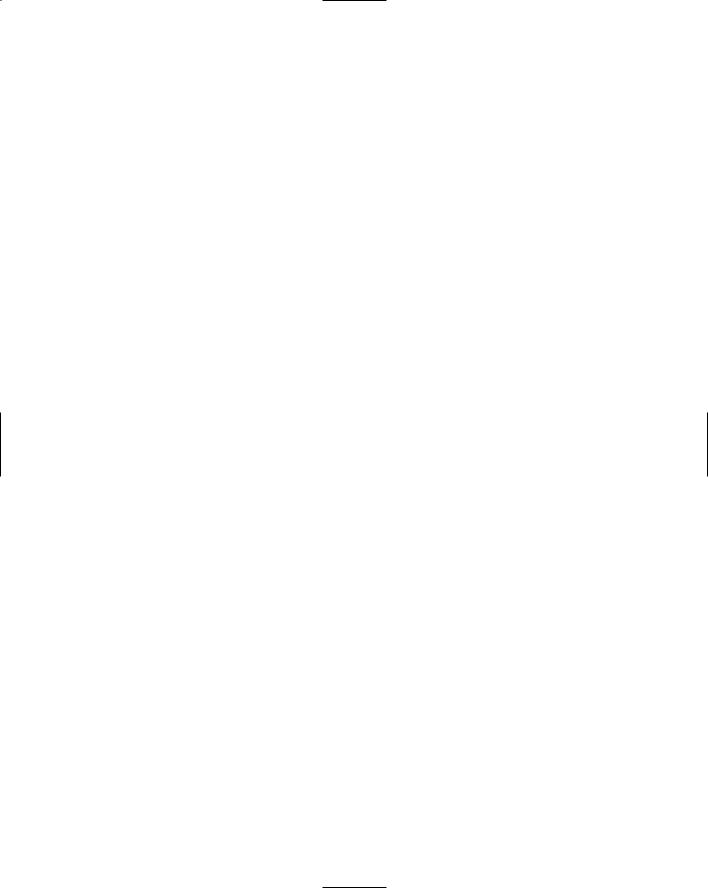
64 |
Part II: Building Secure Applications |
|
|
|
|
|
|||
|
|
|
|
|
|
|
Table 3-2 (continued) |
|
|
|
|
Attribute |
Explanation |
|
|
|
CompleteStepText |
The text displayed when the user suc- |
|
|
|
|
|
cessfully creates an account. (Note that |
|
|
|
|
the Complete step isn’t shown unless |
|
|
|
|
LoginCreatedUser is set to False.) |
|
|
|
|
|
|
|
ContinueButtonImageUrl |
The URL of an image used on the Continue |
|
|
|
|
|
button on the Success page. |
|
|
|
|
|
|
|
ContinueButtonText |
The text displayed on the Continue button |
|
|
|
|
|
on the Success page. |
|
|
ContinueButtonType |
The button type for the Continue button on |
|
|
|
|
|
the Success page. You can specify |
|
|
|
|
Button, Link, or Image. |
|
|
|
|
|
|
|
ContinueDestinationPageUrl |
The URL of the page that’s displayed if |
|
|
|
|
|
the user clicks the Continue button on the |
|
|
|
|
Success page. |
|
|
|
|
|
|
|
ConfirmPasswordLabelText |
The text that’s displayed in the label that |
|
|
|
|
|
identifies the Password |
|
|
|
|
Confirmation field. |
|
|
CreateUserButtonImageUrl |
The URL of an image used on the Create |
|
|
|
|
|
User button. |
|
|
|
|
|
|
|
CreateUserButtonText |
The text displayed on the Create User |
|
|
|
|
|
button. |
|
|
|
|
|
|
|
CreateUserButtonType |
The button type for the Create User button. |
|
|
|
|
|
You can specify Button, Link, or Image. |
|
|
DisableCreatedUser |
A Boolean that indicates whether the new |
|
|
|
|
|
user should be allowed to log in. The default |
|
|
|
|
is False. You can set this to True if you |
|
|
|
|
require an administrator to approve a new |
|
|
|
|
account before allowing the user to log in. |
|
|
|
|
|
|
|
DisplayCancelButton |
A Boolean that indicates whether to display |
|
|
|
|
|
the Cancel button. The default is False. |
|
|
|
|
|
|
|
EmailLabelText |
The text that’s displayed in the label for |
|
|
|
|
|
the Email field. |
|
|
HeaderText |
The text that’s displayed in the header |
|
|
|
|
|
area of the control. |
|
|
|
|
|
|
|
InstructionText |
The text that’s displayed in the instruction |
|
|
|
|
|
area, immediately below the header text. |
|
|
|
|
|
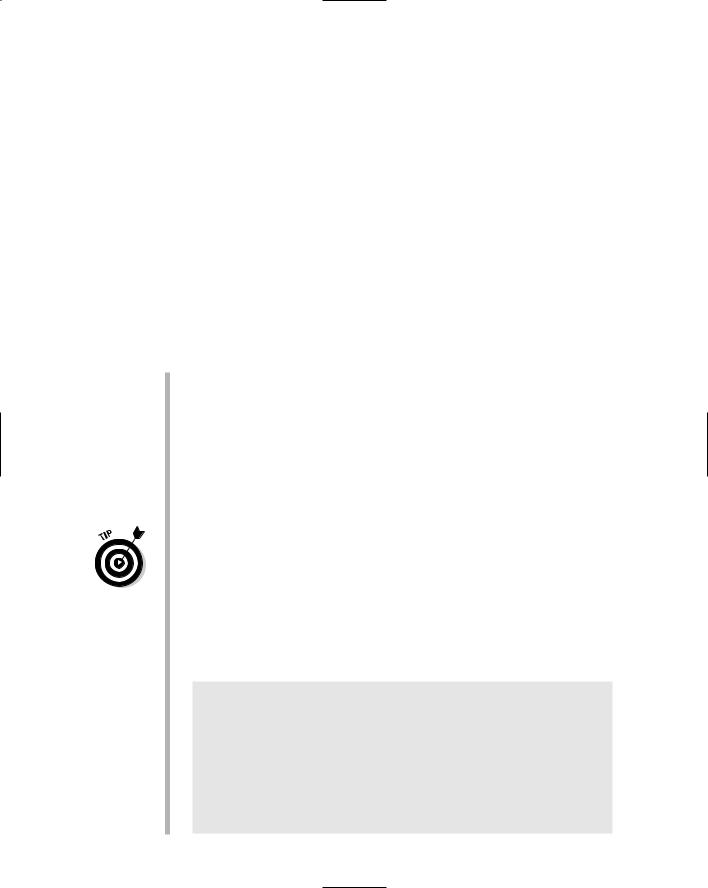
|
|
Chapter 3: Designing Secure ASP.NET Applications |
65 |
||
|
|
||||
|
|
|
|
|
|
|
Attribute |
Explanation |
|
|
|
|
LoginCreatedUser |
A Boolean that indicates whether the new user should |
|
||
|
|
|
automatically be logged in. The default is True. |
|
|
|
|
|
|
|
|
|
PasswordLabelText |
The text that’s displayed in the label for the |
|
||
|
|
|
Password field. |
|
|
|
|
|
|
|
|
|
QuestionLabelText |
The text that’s displayed in the label for the |
|
||
|
|
|
Security Question field. |
|
|
|
UserNameLabelText |
The text that’s displayed in the label for the User |
|
||
|
|
|
Name field. |
|
|
|
|
|
|
|
|
Here are a few other things you should know about the CreateUserWizard control:
You can apply an AutoFormat to the CreateUserWizard control. Or you can use style attributes (not listed in Table 3-2) to customize the appearance of the wizard. For more information, refer to the Help.
By default, the user is logged in the moment an account is created. You can prevent this by specifying LoginCreatedUser=”False”.
You can disable a new user account by specifying DisableCreatedUser=”True”. This capability is especially handy when you want to require an administrator’s approval before you allow new users to access the site.
The CreateUserWizard control inherits the new Wizard control. As a result (you guessed it), many of the CreateUserWizard control’s basic features are derived from the Wizard control. For more information about the Wizard control, refer to Chapter 6.
By default, the wizard has two steps, named CreateUserWizardStep and CompleteWizardStep. You can add additional steps if you want. You can also add a sidebar that displays a link to each of the wizard’s steps. Each wizard step is represented by a template in a <WizardSteps> child element. For example, when you create a CreateUserWizard control in Visual Studio, the default steps are defined with code similar to this:
<asp:CreateUserWizard runat=”server” ID=”CreateUserWizard1” > <WizardSteps>
<asp:CreateUserWizardStep runat=”server” ID=”CreateUserWizardStep1”>
</asp:CreateUserWizardStep> <asp:CompleteWizardStep runat=”server”
ID=”CompleteWizardStep1”>
</asp:CompleteWizardStep>
</WizardSteps>
</asp:CreateUserWizard>
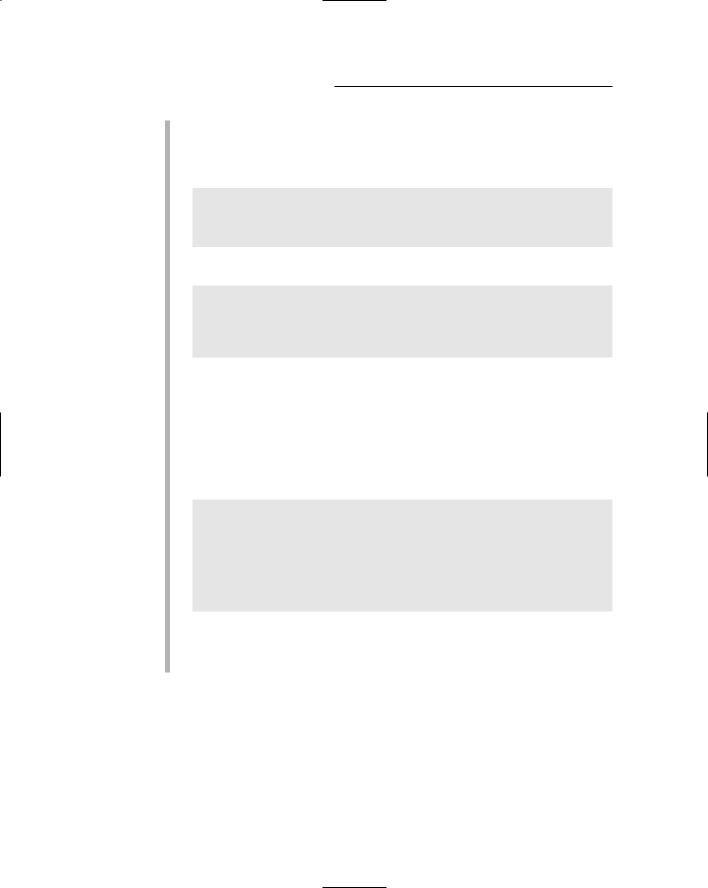
66 |
Part II: Building Secure Applications |
For more information about defining your own wizard steps, see the Help.
The CreateUserWizard control can send a confirmation e-mail to the new user. For that to work, you can add a <MailDefinition> child element, like this:
<MailDefinition
From=”name@domain.com”
Subject=”Subject Line”
BodyFileName=”BodyFile.txt” />
If you prefer, you can use MailDefinition attributes directly in the <CreateUserWizard> element, like this:
<asp:CreateUserWizard runat=”server” ID=”CreateUserWizard1” > MailDefinition-From=”name@domain.com” MailDefintion-Subject=”Subject Line” MailDefinition-BodyFileName=”BodyFile.txt” >
Either method is an acceptable way to specify mail-definition settings.
The body of the e-mail message will be taken from the file indicated by the BodyFileName attribute. Note that this text file can include the special variables <%UserName%> and <%Password%> to include the user’s account name and password in the message.
Besides the <MailDefinition> child element, you must also provide a <MailSettings> element in the application’s web.config file. Here’s a simple example:
<system.net>
<mailSettings>
<smtp>
<network host=”smtp.somewhere.com”
from=”Admin@MyDomain.com” /> </smtp>
</mailSettings>
</system.net>
Here the network host is smtp.somewhere.com and the from address for the e-mail is Admin@MyDomain.com. Naturally, you’ll need to change these settings to reflect the host name of the SMTP server that delivers the mail, as well as the from address you want to use.
Using the PasswordRecovery control
The PasswordRecovery control lets a user retrieve a forgotten password. The user must correctly answer the secret question on file for the user. Then the password is reset to a random value, and the new password is e-mailed to the e-mail address on file with the user.