
ASP.NET 2.0 Everyday Apps For Dummies (2006)
.pdf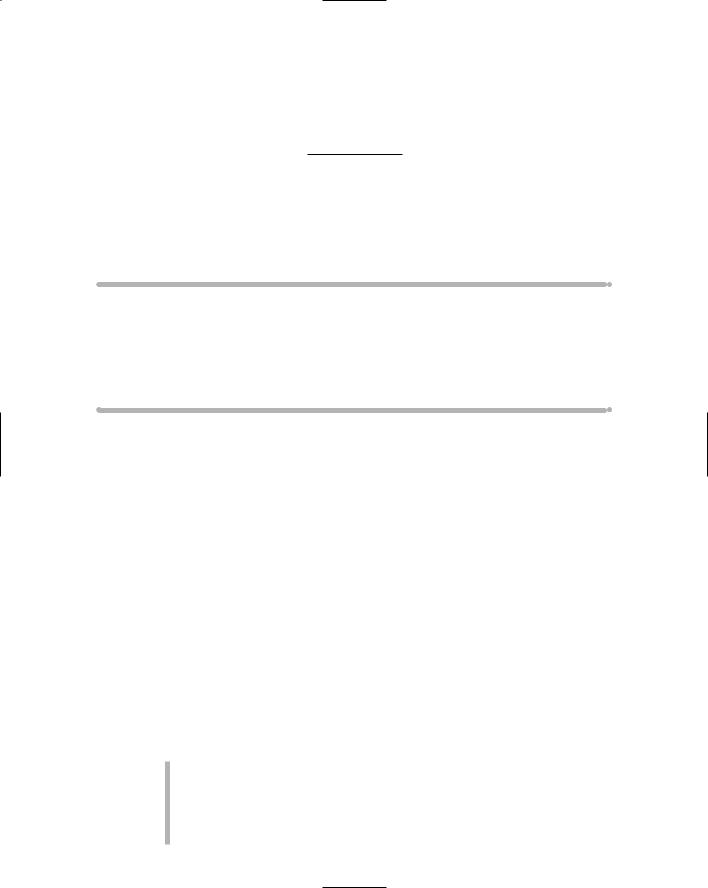
Chapter 5
Building a Product Catalog
Application
In This Chapter
Designing the Product Catalog application
Creating the database for the Product Catalog application
Looking at the new SqlDataSource, GridView, and DetailsView controls
Building the Product Catalog application’s Web pages
Product catalogs used to be phone-book-size tomes; now they’re applications — among the most common found on the Web. That’s
because most companies want to show off their products. Small companies sometimes create Web sites that have just a few products. Other companies, such as Amazon.com, have online catalogs with hundreds of thousands of products. Still, the idea is the same: online product catalogs exist because they drive sales.
Many Web sites not only let users browse the product catalog, but also let users purchase products directly from the Web site. You’ll see an example of an online purchasing application in the next chapter. The application presented in this chapter focuses on presenting product information that’s stored in a database in an interesting and useful way.
The Application’s User Interface
Figure 5-1 shows the user-interface flow for the Product Catalog application that’s built in this chapter. This application’s user interface has just three pages:
default.aspx displays a list of products for a category selected by the user.
product.aspx displays details about a specific product selected by the user.
cart.aspx is displayed when the user chooses to purchase a product.
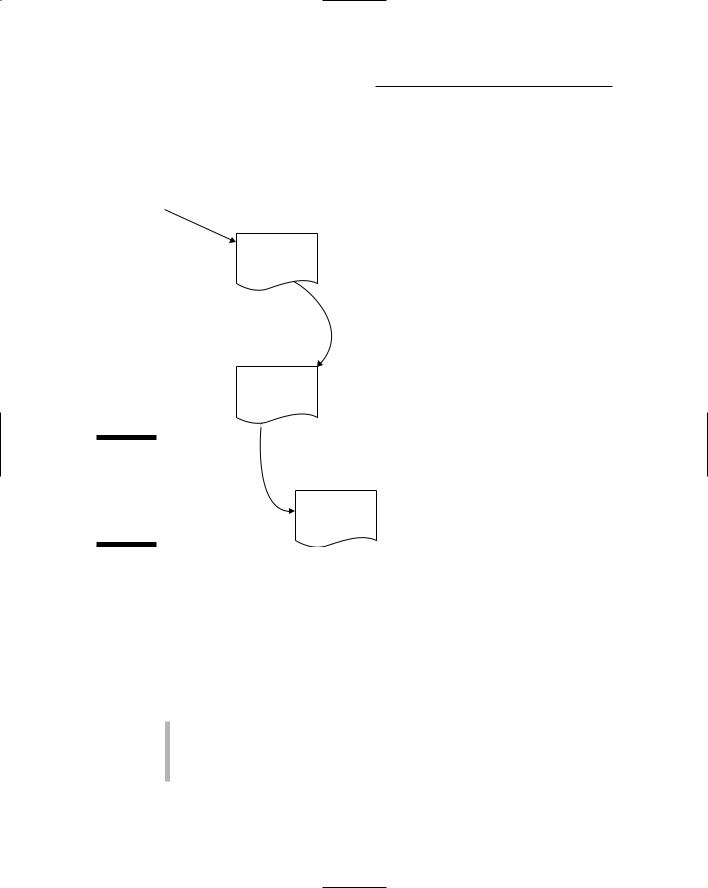
108 Part III: Building E-Commerce Applications
For this application, the cart.aspx page simply displays a message indicating that the shopping cart isn’t implemented. (You’ll find an implementation of the Shopping Cart page in the next chapter.)
User requests page
|
Default.aspx |
|
Product List |
|
page |
|
View |
|
detail |
|
Product.aspx |
|
Product |
|
Detail |
|
page |
Figure 5-1: |
|
The user |
Cart.aspx |
interface for |
|
the Product |
Cart page |
Catalog |
|
application. |
|
The Product List page
Figure 5-2 shows the Product List page (default.aspx) displayed in a browser window. This page displays a list of products for a category selected by the user. This is the default start page for the application.
The following paragraphs describe the more interesting aspects of the Product List page:
The page uses a Master Page named default.master. The Master Page simply displays the banner image that appears at the top of the page.
The application’s database includes a separate table for featured products that includes a sale price and space for a promotional message.
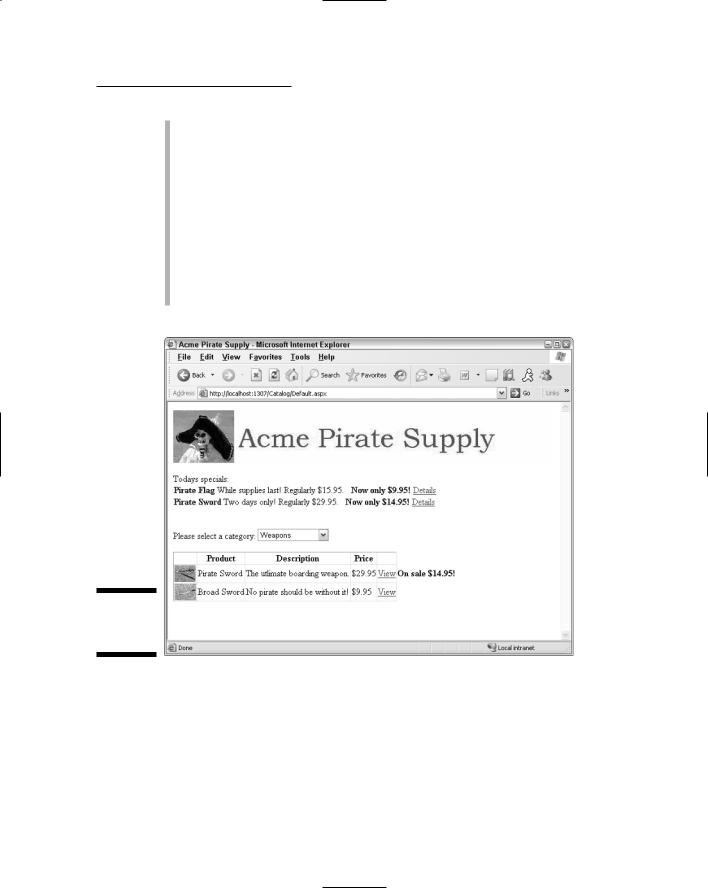
Chapter 5: Building a Product Catalog Application 109
Any products in this table are listed at the top of the Product List page. Then the user can click the Details link to go to the Product page for the featured product.
A drop-down list is used to select a product category. When the user selects a category from this list, the page is updated to list the products for the selected category. Note that the category values aren’t hardcoded into the program. Instead, they’re retrieved from the application’s database.
The product list shows the products for the selected category. The user can click the View link to go to the Product page for a particular product. Note that if the product is currently on sale, the sale price is shown in the Product List.
Figure 5-2:
The Product
List page.
The Product Detail page
Figure 5-3 shows the Product Detail page (product.aspx) displayed in a browser window. As you can see, this page displays the details for a particular product. The user can reach this page by clicking the View or Details link for a product on the Product List page.
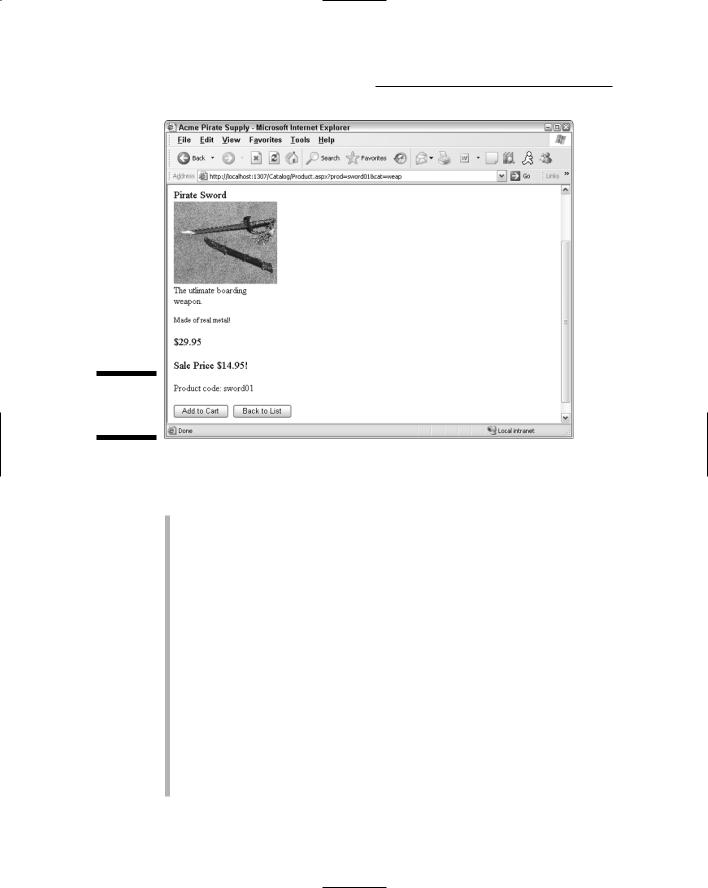
110 Part III: Building E-Commerce Applications
Figure 5-3:
The Product
Detail page.
The following paragraphs describe some of the more interesting details about this page:
Like the Product List page, this page also uses the default.Master Page as its Master Page. However, I scrolled down a bit to show the entire contents of the page. As a result, the banner that appears at the top of the page isn’t visible in the figure.
Because this product is listed as one of the featured products, its sale price is shown.
The buttons at the bottom of the page let the user add the current product to the shopping cart or return to the Product List page.
Notice that the URL that appears in the browser’s address bar includes two query string fields: prod=sword01 and cat=weap. The first field indicates which product the user selected in the Product List page. The Product Detail page uses this field to retrieve the correct product from the database. The second field saves the category selected by the user on the Product List page (the Product Detail page doesn’t use this field). However, if the user clicks the Back to List button to return to the Product List page, the cat field is passed back to the Product List page. Then the Product List page uses it to select the category that the user had previously selected.
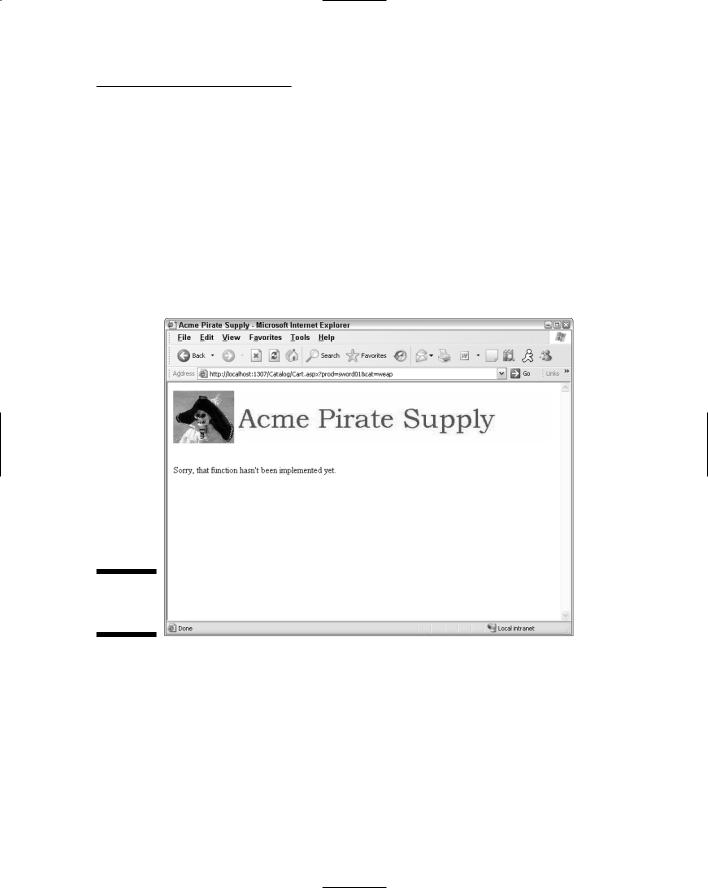
Chapter 5: Building a Product Catalog Application 111
The Cart page
This application provides a dummy Cart page, as shown in Figure 5-4. As you can see, this page simply indicates that the shopping cart function hasn’t yet been implemented. For an implementation of the shopping-cart page, refer to the next chapter.
Notice that the URL in this figure includes a query string that indicates which product the user wants to add to the cart. The dummy Cart page used in this application doesn’t do anything with this query string — but the actual Shopping Cart application (presented in Chapter 6) has plenty of use for it.
Figure 5-4:
The Cart
page.
Designing the Product
Catalog Application
The Product Catalog Application is designed to be simple enough to present in this book, yet complicated enough to realistically address some of the design considerations that go into this type of application. There are several important decisions that need to be made for any application of this sort.

112 Part III: Building E-Commerce Applications
For example, how will the database be designed to store the product information? In particular, how will the database represent products and categories, and how will the products featured on sale be represented? In addition, the database design must address how images of the product will be accessed. For more details on the database design for this application, refer to the section “Designing the Product Database” later in this chapter.
Another important aspect of the design is how the application keeps track of the state information, such as which product the user is viewing. For example, when the user chooses to see more detail for a specific product, how will the application pass the selected product from the Product List page to the Product Detail page so the Product Detail page knows which product to display? And how will the application remember which product category was being viewed, so the same category can be redisplayed when the user returns to the Product List page?
Although there are several alternatives for storing this type of state information in ASP.NET, this application saves the product and category information in query strings appended to the end of the URLs used to request the application’s pages. Two query-string fields are used:
prod: Passes the ID of the product to be displayed by the Product Detail page.
cat: Passes the ID of the category that’s selected on the Product List page.
For example, suppose the user selects the Weapons category and clicks the View link for the first sword. Then the URL used to display the Product.aspx page will look like this:
~\Product.aspx?prod=sword01&cat=weap
Here, the ID of the product to be displayed is sword01 and the ID of the selected category is weap.
If the user clicks the Back to List button, the application returns to the
Default.aspx page via the following URL:
~\Default.aspx?cat=weap
That way, the Default.aspx page will know to set the category drop-down list to Weapons.
If, on the other hand, the user clicks the Add to Cart button to order the product, this URL will be used to display the Cart.aspx page:
~\Product.aspx?prod=sword01&cat=weap
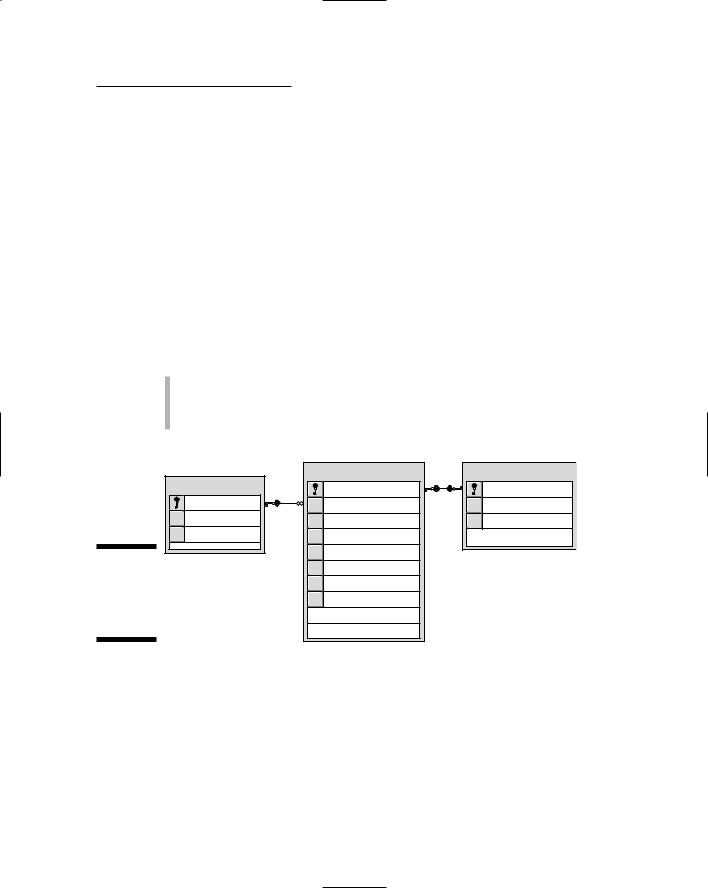
Chapter 5: Building a Product Catalog Application 113
Thus, the product and category ID values are passed from the Product.aspx page to the Cart.aspx page. (For more information about what the actual Cart page does with these values, refer to Chapter 6.)
Note that when the application is first started, the URL used to display
the Default.aspx page doesn’t include any query strings. As a result, the Default.aspx page is designed so that if it isn’t passed a cat query-string field, it defaults to the first category.
Designing the Product Database
The Product Catalog application requires a database to store the information about the products to be displayed. Figure 5-5 shows a diagram of the database. As you can see, it consists of three tables:
Categories
Products
FeaturedProducts
Categories |
Products |
FeaturedProducts |
productid |
productid |
|
catid |
catid |
featuretext |
name |
name |
saleprice |
[desc] |
shorttext |
|
Figure 5-5: |
longtext |
|
price |
|
|
The Product |
thumbnail |
|
Catalog |
|
|
image |
|
|
application’s |
|
|
|
|
|
database. |
|
|
The following sections describe the details of each of these tables.
The Categories table
The Categories table contains one row for each category of product represented in the database. Table 5-1 lists the columns defined for this table.
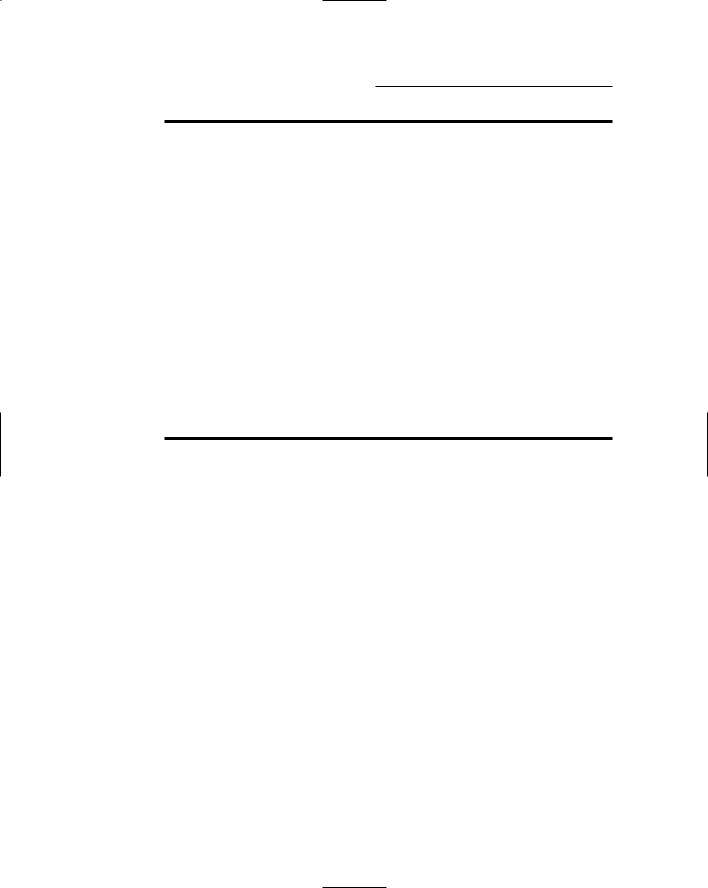
114 Part III: Building E-Commerce Applications
Table 5-1 |
|
The Categories Table |
Column name |
Type |
Description |
catid |
VARCHAR(10) |
An alphanumeric code (up to 10 characters) |
|
|
that uniquely identifies each category. This is |
|
|
the primary key for the Categories table. |
|
|
|
name |
VARCHAR(50) |
A text field that provides the name of the |
|
|
category. |
|
|
|
desc |
VARCHAR(MAX) |
A text field that provides a description of the |
|
|
category. |
The Products table
The Products table contains one row for each product represented in the database. Table 5-2 lists the columns used by the Products table.
Table 5-2 |
|
The Products Table |
Column name |
Type |
Description |
productid |
VARCHAR(10) |
An alphanumeric code (up to 10 characters) |
|
|
that uniquely identifies each product. This is |
|
|
the primary key for the Products table. |
|
|
|
catid |
VARCHAR(10) |
A code that identifies the product’s category. |
|
|
A foreign-key constraint ensures that only |
|
|
values present in the Categories table |
|
|
can be used for this column. |
|
|
|
name |
VARCHAR(50) |
A text field that provides the name of the |
|
|
product. |
shorttext |
VARCHAR(MAX) |
A text field that provides a short description |
|
|
of the product. |
|
|
|
longtext |
VARCHAR(MAX) |
A text field that provides a longer description |
|
|
of the product. |
|
|
|
price |
MONEY |
The price for a single unit of the product. |
|
|
|
thumbnail |
VARCHAR(40) The name of the thumbnail image file. |
|
|
|
|
image |
VARCHAR(40) The name of the main image file. |
|
|
|
|
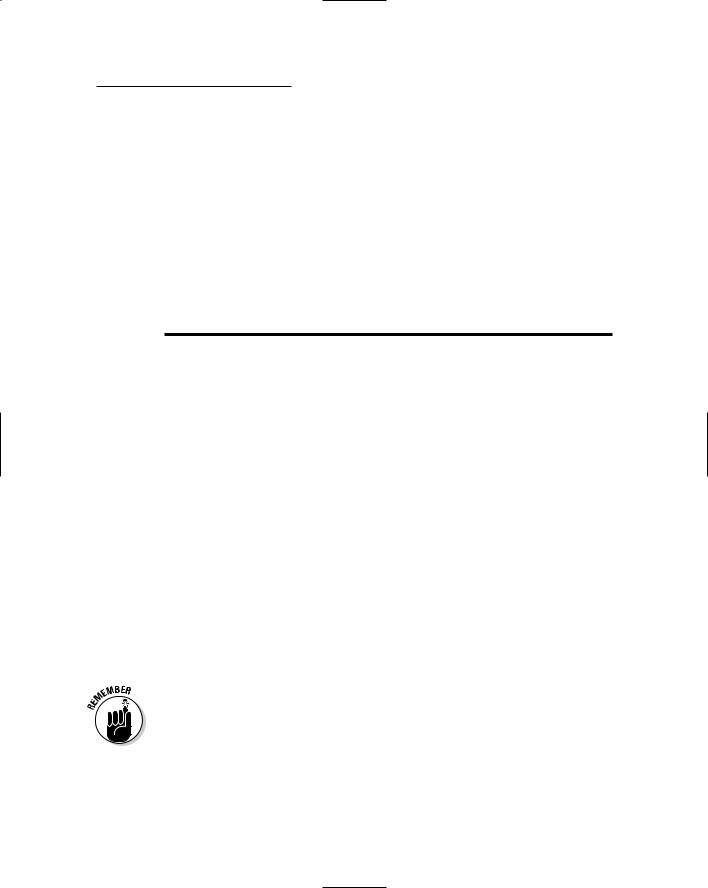
Chapter 5: Building a Product Catalog Application 115
Note that the thumbnail and image fields contain just the filename of the image files, not the complete path to the images. The application adds
~\Images\ to the filenames to locate the files. (For example, sword01.jpg will become ~\Images\sword01.jpg.)
The FeaturedProducts table
The FeaturedProducts table contains one row for each product that’s currently on sale or being featured. Note that each row in this table corresponds to a row in the Products table. Table 5-3 lists the columns used by the FeaturedProducts table.
Table 5-3 |
The FeaturedProducts Table |
|
Column name |
Type |
Description |
productid |
VARCHAR(10) |
An alphanumeric code (up to 10 characters) |
|
|
that uniquely identifies the product fea- |
|
|
tured. This is the primary key for the |
|
|
FeaturedProducts table, and a |
|
|
foreign-key constraint ensures that the |
|
|
value must match a value present in the |
|
|
Products table. |
featuretext |
VARCHAR(MAX) |
Promotional text that describes the item |
|
|
being featured. |
|
|
|
saleprice |
MONEY |
The sale price for the item. |
|
|
|
Note that each row in the FeaturedProducts table corresponds to a row in the Products table, and the relationship is one-to-one. Each row in the Products table can have only one corresponding row in the FeaturedProducts table. However, not every row in the Products table has a corresponding row in the FeaturedProducts table, which is (by definition) only for those products currently being featured.
I could just as easily combined these tables by including the saleprice and featuretext columns in the Products table. I chose to implement
FeaturedProducts as a separate table to simplify the query that retrieves the list of featured products.
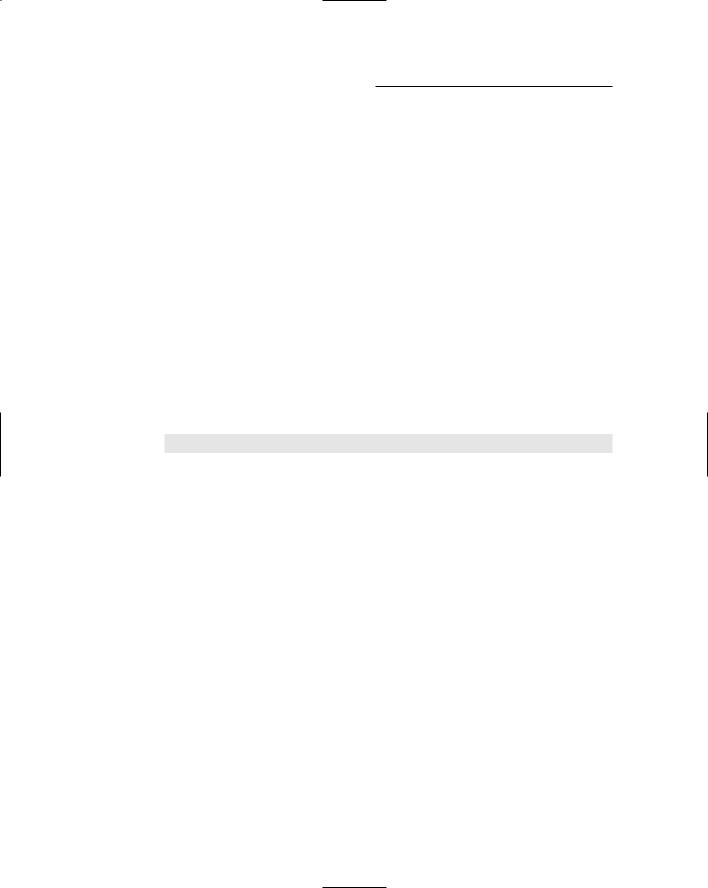
116 Part III: Building E-Commerce Applications
However, most design decisions involve trade-offs, and this one is no exception. Although using a separate table for the featured products list simplifies the query that retrieves the featured products, it complicates the queries that retrieve product rows. That’s because whenever you retrieve a product row, you must also check to see if that product is on sale. Otherwise the user won’t know the actual price of the product. As a result, the query that retrieves products for the Default.aspx and Product.aspx pages must use an outer join to retrieve data from both tables.
For more information about the queries used to access this database, see the section “Querying the database,” later in this chapter.
Creating the database
You can create the Products database manually from within Visual Studio by using the Database Explorer. Alternatively, you can run the CreateProducts. sql script that’s shown in Listing 5-1. To run this script, open a commandprompt window and change to the directory that contains the script. Then enter this command:
sqlcmd -S localhost\SQLExpress -i CreateProducts.sql
Note that this command assumes you’re running SQL Server Express on your own computer. If you’re using SQL Server on a different server, you’ll need to change localhost\SQLExpress to the correct name. If you’re not sure what name to use, ask your database administrator.
Listing 5-1: The CreateProducts.sql script
USE master |
1 |
GO |
|
IF EXISTS(SELECT * FROM sysdatabases |
2 |
WHERE name=’Products’) |
|
DROP DATABASE Products |
|
GO |
|
CREATE DATABASE Products |
3 |
ON (NAME=Product, |
|
FILENAME = ‘C:\APPS\Products.mdf’, |
|
SIZE=10 ) |
|
GO |
|
|
|