
ASP.NET 2.0 Everyday Apps For Dummies (2006)
.pdf
Chapter 3: Designing Secure ASP.NET Applications |
67 |
E-mail is inherently insecure, and the password sent to the user is not encrypted.
As a result, you should carefully evaluate your application’s security requirements before you use this control.
In its simplest form, the PasswordRecovery control looks like this:
<asp:PasswordRecovery id=”PasswordRecovery1” runat=”Server” >
<MailDefinition
From=”name@domain.com”
Subject=”Subject Line” BodyFileName=”BodyFile.txt” />
</asp:PasswordRecovery>
If you prefer, you can code the mail-definition settings directly into the <PasswordRecovery> element, like this:
<asp:PasswordRecovery id=”PasswordRecovery1” runat=”Server” MailDefinition-From=”name@domain.com”
MailDefinition-Subject=”Subject Line” MailDefinition-BodyFileName=”BodyFile.txt” />
</asp:PasswordRecovery>
As you can see, the <MailDefinition> child element is required to provide the information necessary to send an e-mail message with the user’s name and password. As with the CreateUserWizard control, the body of the e-mail message is supplied by a text file that can include the variables <%UserName%> and <%Password%>, which are replaced by the user’s name and password when the mail is sent.
The attributes listed in Table 3-3 let you customize the appearance of the PasswordRecovery control. Here’s an example that changes the text labels displayed by the control:
<asp:PasswordRecovery id=”PasswordRecovery1” runat=”Server” UserNameTitleText=
“Forgot Your Password Again, Eh?<br /><br />” UserNameInstructionText=
“Enter your user name.<br /><br />” UserNameLabelText=”User name:” QuestionTitleText=
“Forgot Your Password Again, Eh?<br /><br />” QuestionInstructionText=
“Answer the secret question.<br /><br />” QuestionLabelText=”<br />Secret question:” AnswerLabelText=”<br />Your answer:” SuccessText=”Your new password has been e-mailed to
you.”
/>
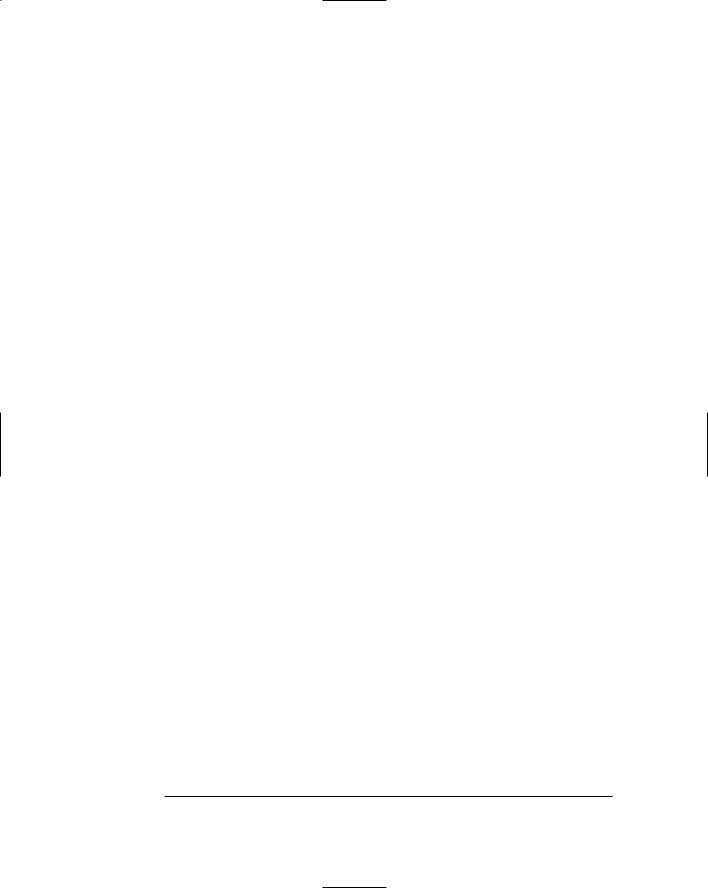
68 |
Part II: Building Secure Applications |
|
|
||
|
|
||||
|
|
|
|
|
|
|
|
Table 3-3 |
Attributes for the PasswordRecovery control |
||
|
|
Attribute |
|
|
Explanation |
|
|
id |
|
|
The ID associated with the |
|
|
|
|
|
PasswordRecovery control. |
|
|
runat |
|
|
Runat=Server is required for all ASP.NET |
|
|
|
|
|
server controls. |
|
|
|
|
|
|
|
|
AnswerLabelText |
The text that’s displayed in the label for the |
||
|
|
|
|
|
Answer field. |
|
|
|
|
|
|
|
|
GeneralFailureText |
The text that’s displayed if the password |
||
|
|
|
|
|
can’t be recovered. |
|
|
QuestionFailureText |
The text that’s displayed if the user provides |
||
|
|
|
|
|
the wrong answer for the secret question. |
|
|
|
|
|
|
|
|
QuestionInstructionText |
The text that’s displayed in the label that |
||
|
|
|
|
|
instructs the user to answer the secret |
|
|
|
|
|
question. |
|
|
QuestionLabelText |
The text that’s displayed in the label that |
||
|
|
|
|
|
identifies the secret question. |
|
|
|
|
|
|
|
|
QuestionTitleText |
The text that’s displayed in the title area |
||
|
|
|
|
|
when the secret question is asked. |
|
|
|
|
|
|
|
|
SubmitButtonImageUrl |
The URL of an image used on the Submit |
||
|
|
|
|
|
button. |
|
|
SubmitButtonText |
The text displayed on the Submit button. |
||
|
|
|
|
|
|
|
|
SubmitButtonType |
The button type for the Submit button. You |
||
|
|
|
|
|
can specify Button, Link, or Image. |
|
|
|
|
|
|
|
|
SuccessPageUrl |
The URL of the page to be displayed when the |
||
|
|
|
|
|
password has been successfully recovered. |
|
|
|
|
|
|
|
|
SuccessText |
|
|
The text to display when the password has |
|
|
|
|
|
been successfully recovered. Note that this |
|
|
|
|
|
text is not displayed if the |
|
|
|
|
|
SuccessPageUrl is provided. |
|
|
|
|
|
|
|
|
TextLayout |
|
|
Specifies the position of the labels relative to |
|
|
|
|
|
the user name and password text boxes. If |
you specify TextOnLeft, the labels appear to the left of the text boxes. If you specify TextOnTop, the labels appear above the text boxes.
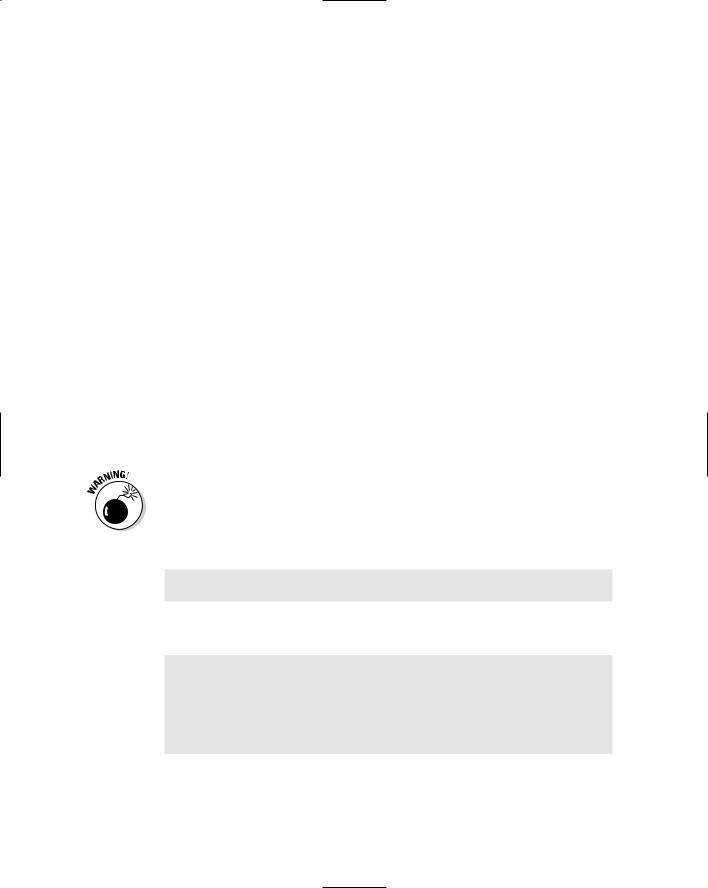
|
|
Chapter 3: Designing Secure ASP.NET Applications |
69 |
||
|
|
||||
|
|
|
|
|
|
|
Attribute |
Explanation |
|
|
|
|
UserNameFailureText |
The text that’s displayed if the user provides |
|
||
|
|
|
an incorrect user name. |
|
|
|
|
|
|
|
|
|
UserNameInstructionText |
The text that’s displayed in the instruction |
|
||
|
|
|
area when the user name is requested. |
|
|
|
UserNameLabelText |
The text that’s displayed in the label that |
|
||
|
|
|
identifies the User Name field. |
|
|
|
|
|
|
|
|
|
UserNameTitleText |
The text that’s displayed in the title area |
|
||
|
|
|
when the user name is requested. |
|
|
|
|
|
|
|
|
Using the ChangePassword control
The ChangePassword control lets a user change his or her password. The ChangePassword control can be configured to accept the user name of the account whose password you want to change. If the control isn’t configured to accept the user name, then the actual user must be logged in to change the password.
The ChangePassword control can also be configured to e-mail the new password to the user. Note that because e-mail is inherently insecure, you should carefully evaluate your application’s security requirements before you use this feature.
In its simplest form, the ChangePassword control looks like this:
<asp:ChangePassword id=”ChangePassword1”
runat=”Server” />
If you want to e-mail the changed password to the user, you should add a <MailDefinition> child element, like this:
<asp:ChangePassword id=”ChangePassword1” runat=”Server” >
<MailDefinition
From=”name@domain.com”
Subject=”Subject Line” BodyFileName=”BodyFile.txt” />
</asp:ChangePassword>
Here, the body of the e-mail message is supplied by a text file that can include the variables <%UserName%> and <%Password%>. When the message is sent, these variables are replaced by the user’s name and password. (You can see an example of how this works in Chapter 4.)
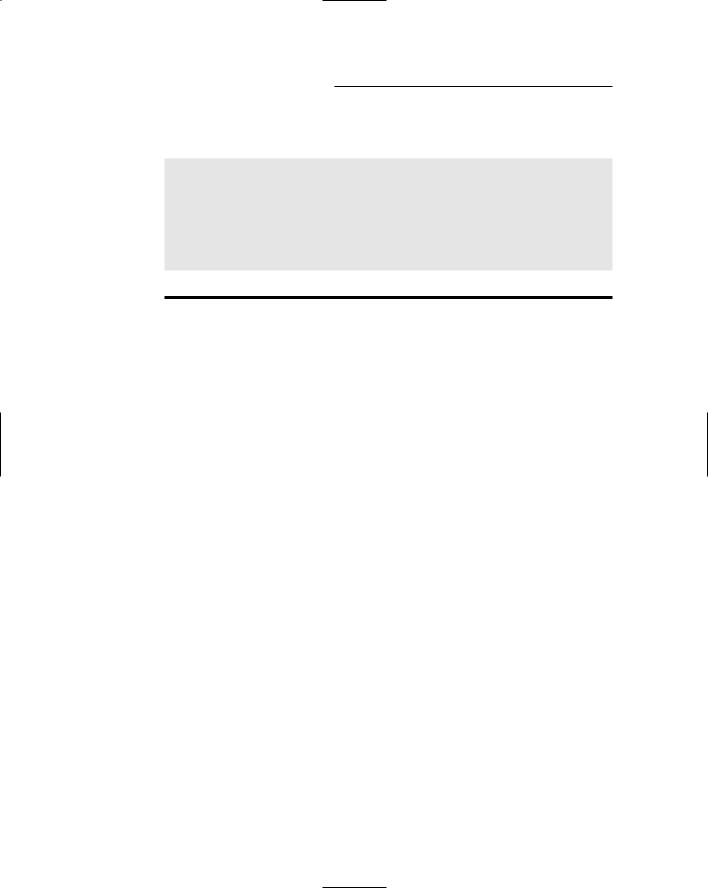
70 |
Part II: Building Secure Applications |
You can customize the appearance and behavior of the ChangePassword using the attributes listed in Table 3-4. Here’s an example:
<asp:ChangePassword id=”ChangePassword1” runat=”Server” ChangePasswordTitleText=
“Change Your Password<br /><br />” PasswordLabelText=”Enter your current password:”
NewPasswordLabelText=”Enter the new password:” ConfirmNewPasswordLabelText=”Confirm the new
password:”
/>
Table 3-4 |
Attributes for the ChangePassword control |
|
Attribute |
|
Explanation |
id |
|
The ID associated with the |
|
|
ChangePassword control. |
|
|
|
runat |
|
Runat=”Server” is required for all |
|
|
ASP.NET server controls. |
|
|
|
CancelButtonImageUrl |
The URL of an image used on the |
|
|
|
Cancel button. |
CancelButtonText |
The text displayed on the Cancel |
|
|
|
button. |
|
|
|
CancelButtonType |
The button type for the Cancel button. |
|
|
|
You can specify Button, Link, or |
|
|
Image. |
CancelDestinationPageUrl |
The URL of the page that’s displayed if |
|
|
|
the user clicks the Cancel button. |
|
|
|
ChangePasswordButtonImageUrl |
The URL of an image used on the |
|
|
|
Change Password button. |
|
|
|
ChangePasswordButtonText |
The text displayed on the Change |
|
|
|
Password button. |
ChangePasswordButtonType |
The button type for the Change |
|
|
|
Password button. You can specify |
|
|
Button, Link, or Image. |
|
|
|
ChangePasswordFailureText |
The text that’s displayed if the pass- |
|
|
|
word can’t be changed. |
ChangePasswordTitleText |
The text that’s displayed as the title for |
|
|
|
the Change Password control. |
|
|
|
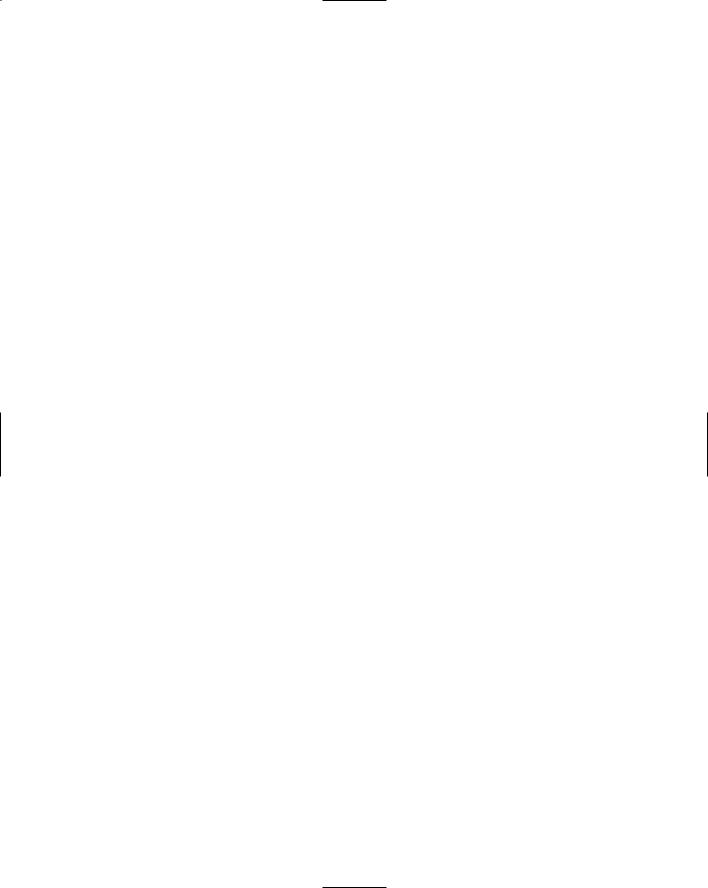
|
|
Chapter 3: Designing Secure ASP.NET Applications |
71 |
||
|
|
||||
|
|
|
|
|
|
|
Attribute |
Explanation |
|
|
|
|
ConfirmNewPasswordLabelText |
The text that’s displayed in the label |
|
||
|
|
|
that identifies the Confirm |
|
|
|
|
|
Password field. |
|
|
|
ContinueButtonImageUrl |
The URL of an image used on the |
|
||
|
|
|
Continue button. |
|
|
|
|
|
|
|
|
|
ContinueButtonText |
The text displayed on the Continue |
|
||
|
|
|
button. |
|
|
|
|
|
|
|
|
|
ContinueButtonType |
The button type for the Continue |
|
||
|
|
|
button. You can specify Button, |
|
|
|
|
|
Link, or Image. |
|
|
|
|
|
|
|
|
|
ContinueDestinationPageUrl |
The URL of the page that’s displayed if |
|
||
|
|
|
the user clicks the Continue button. |
|
|
|
|
|
|
|
|
|
CreateUserText |
The text displayed as a link to the |
|
||
|
|
|
application’s Create User page. |
|
|
|
CreateUserUrl |
The URL of the application’s Create |
|
||
|
|
|
User page. |
|
|
|
|
|
|
|
|
|
DisplayUserName |
A Boolean that indicates whether the |
|
||
|
|
|
user will be asked to enter a user |
|
|
|
|
|
name. If True, the |
|
|
|
|
|
ChangePassword control can be |
|
|
|
|
|
used to change the password of an |
|
|
|
|
|
account other than the one to which |
|
|
|
|
|
the user is currently logged in. |
|
|
|
|
|
|
|
|
|
InstructionText |
The text that’s displayed in the instruc- |
|
||
|
|
|
tion area of the ChangePassword |
|
|
|
|
|
control. |
|
|
|
NewPasswordLabelText |
The text that’s displayed in the label that |
|
||
|
|
|
identifies the New Password field. |
|
|
|
|
|
|
|
|
|
NewPasswordRegularExpression |
A regular expression used to validate |
|
||
|
|
|
the new password. |
|
|
|
|
|
|
|
|
|
PasswordHintText |
The text that’s displayed to inform the |
|
||
|
|
|
user of any password requirements, |
|
|
|
|
|
such as minimum length or required |
|
|
|
|
|
use of special characters. |
|
|
|
|
|
|
|
|
|
PasswordLabelText |
The text that’s displayed by the label |
|
||
|
|
|
that identifies the Current Password |
|
|
|
|
|
field. |
|
|
|
|
|
|
|
|
(continued)
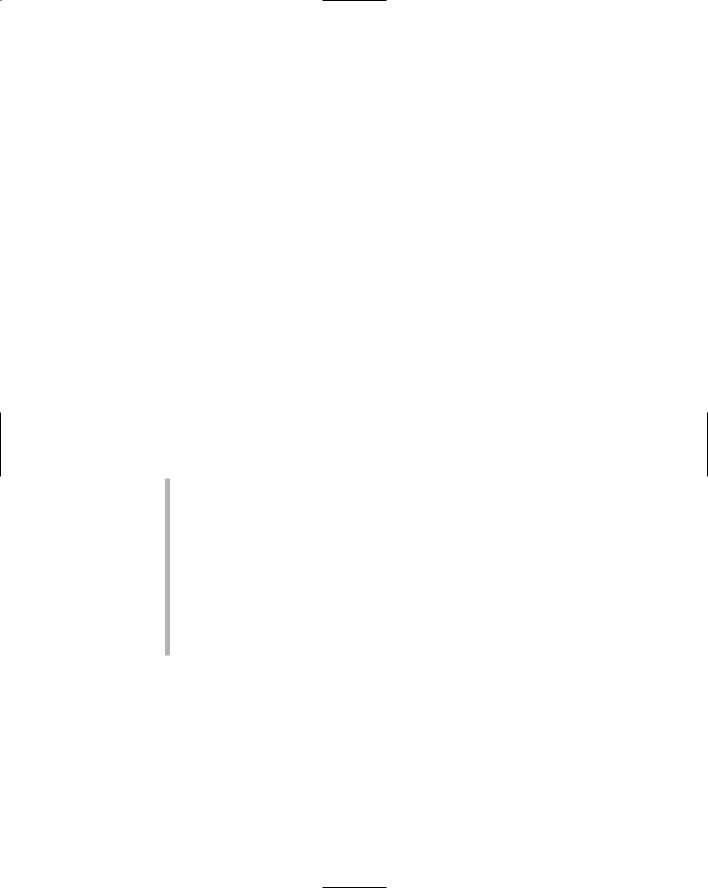
72 |
Part II: Building Secure Applications |
|
|
|
|||
|
|
|
|
|
|
Table 3-4 (continued) |
|
|
|
Attribute |
Explanation |
|
|
PasswordRecoveryText |
The text displayed as a link to the appli- |
|
|
|
cation’s Password Recovery page. |
|
|
PasswordRecoveryUrl |
The URL of the application’s Password |
|
|
|
Recovery page. |
|
|
|
|
|
|
SuccessPageUrl |
The URL of the page to be displayed |
|
|
|
when the password has been suc- |
|
|
|
cessfully changed. |
|
|
SuccessText |
The text to display when the password |
|
|
|
has been successfully changed. Note |
|
|
|
that this text is not displayed if the |
|
|
|
SuccessPageUrl is provided. |
|
|
UserNameLabelText |
The text that’s displayed in the label |
|
|
|
that identifies the User Name field. |
|
|
|
|
Here are a couple of additional details you need to know about the
ChangePassword control:
By default, the ChangePassword control requires that the user be logged in already. However, that changes if you specify
DisplayUserName=”True”. Then, the ChangePassword control displays a user name text box. The ChangePassword control then lets the user change the password for any user, provided the user enters a valid user name and password.
The ChangePassword control has two views. The initial view — the Change Password view — includes the text boxes that let the user enter a new password. The Success view is displayed only if the password is successfully changed. It displays a confirmation message. Note that if you specify the SuccessPageUrl attribute, Success view is never displayed. Instead, the page at the specified URL is displayed.
Using the LoginView control
The LoginView control is a templated control that displays the contents of one of its templates, depending on the login status of the user. This enables you to customize the content of your Web site for different types of users. For example, the User Authentication application presented later in this chapter uses a LoginView control to display a link to the administration page that’s visible only to members of the Admin role.
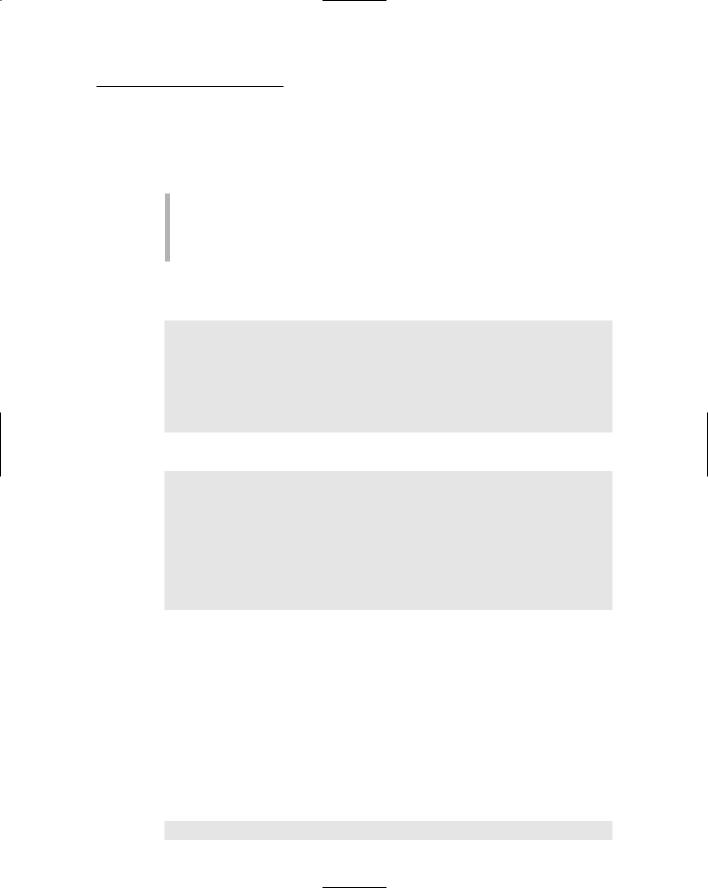
Chapter 3: Designing Secure ASP.NET Applications |
73 |
Unlike the other login controls presented so far in this chapter, the LoginView control doesn’t rely much on the use of attributes to customize its appearance or behavior. Instead, you customize the LoginView control by using three types of templates, each of which is coded as a child element:
Anonymous template: Displayed if the user isn’t logged in.
LoggedIn template: Displayed if the user is logged in.
RoleGroup template: Displayed if the user is logged in and is a member of a particular role group.
The first two template types are simply specified as child elements of the LoginView control. Consider this example:
<asp:LoginView runat=”Server” id=”LoginView1”> <AnonymousTemplate>
This template is displayed for anonymous users. </AnonymousTemplate>
<LoggedInTemplate>
This template is displayed for logged in users. </LoggedInTemplate>
</asp:LoginView>
The role group templates are a little more complicated. They’re coded like this:
<asp:LoginView runat=”Server” id=”LoginView1”> <RoleGroups>
<asp:RoleGroup Roles=”Admin”> <ContentTemplate>
This template is displayed for
administrators.
</ContentTemplate>
</asp:RoleGroup>
</RoleGroups>
</asp:LoginView>
Note that the <RoleGroups> element can contain more than one <RoleGroup> element. In addition, <RoleGroup> elements can be used along with Anonymous and LoggedIn templates.
Using the LoginName control
The LoginName control is straightforward: It simply displays the user’s name, assuming the user is logged in. If the user is not logged in, the LoginName control displays nothing.
In its simplest form, the LoginName control looks like this:
<asp:LoginName runat=”server” id=”LoginName1” />
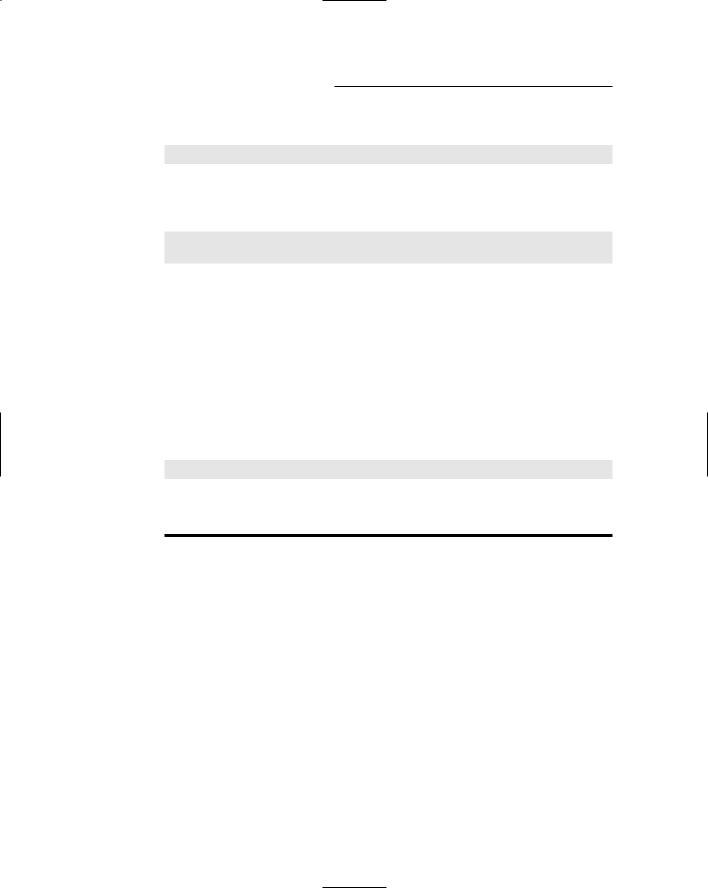
74 |
Part II: Building Secure Applications |
You might be tempted to precede the LoginName control with text, like this:
Hello, <asp:LoginName runat=”server” id=”LoginName1” />
Unfortunately, this technique won’t work right if the user isn’t logged in — the text literal (Hello,) will be displayed but the name won’t be. Instead, you can specify a format string, like this:
<asp:LoginName runat=”server” ID=”LoginName1”
FormatString=”Hello, {0}” />
That way, Hello, will be added as a prefix to the name if the user is logged in. If the user isn’t logged in, nothing is displayed.
Using the LoginStatus control
The LoginStatus control displays a link that lets the user log in to — or log out of — a Web site. If the user is already logged in, the link lets the user log out. If the user isn’t logged in, the link lets the user log in.
The simple form of the LoginStatus control looks like this:
<asp:LoginStatus runat=”server” id=”LoginStatus1” />
You can customize the control by using the attributes listed in Table 3-5.
Table 3-5 |
Attributes for the LoginStatus control |
Attribute |
Explanation |
id |
The ID associated with the LoginStatus control. |
|
|
runat |
Runat=”Server” is required for all ASP.NET server |
|
controls. |
|
|
LoginImageUrl |
The URL of an image used for the Login link. |
|
|
LoginText |
The text displayed by the Login link. |
|
|
LogoutAction |
Specifies what happens when the user logs out. You |
|
can specify Redirect to redirect the user to the |
|
page specified in the LogoutPageUrl attribute, |
|
RedirectToLoginPage to redirect the user to |
|
the application’s login page, or Refresh to refresh the |
|
current page. |
|
|
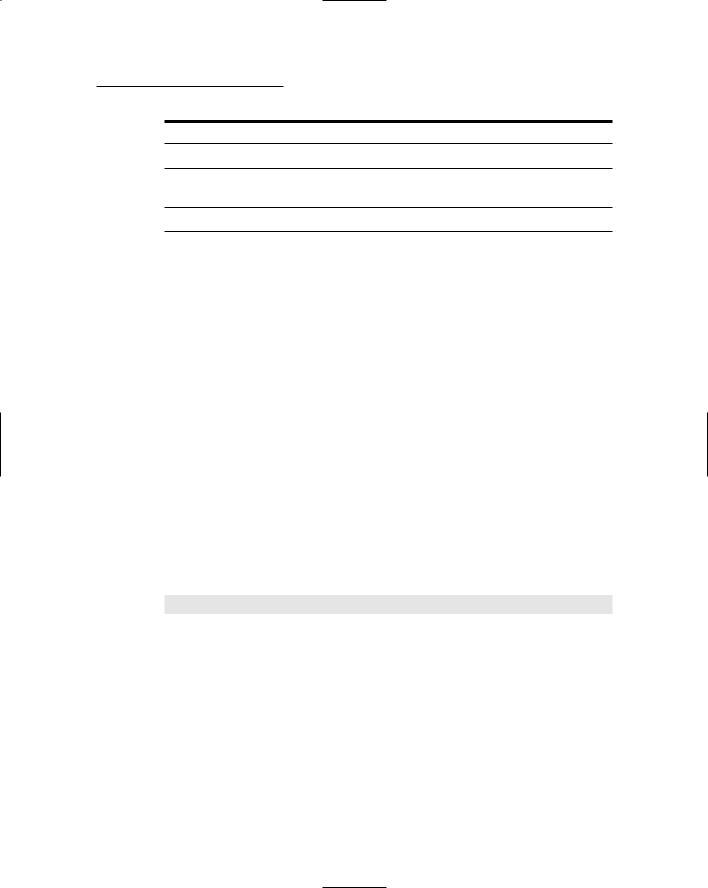
Chapter 3: Designing Secure ASP.NET Applications |
75 |
Attribute |
Explanation |
LogoutImageUrl The URL of an image used for the Logout link.
LogoutPageUrl The URL of the page to redirect to when the user logs out if the LogoutAction attribute specifies Redirect.
LogoutText |
The text displayed by the Logout link. |
Protecting Against Other Threats
Although the main security technique for ASP.NET applications is user authentication and authorization, not all security threats are related to unauthorized users accessing Web pages. The following sections describe some of the more common types of threats besides unauthorized access — and offer pointers to protect your ASP.NET applications against those threats.
Avoid malicious scripts
Cross-site scripting (also known as XSS) is a hacking technique in which a malicious user enters a short snippet of JavaScript into a text box, hoping that the application will save the JavaScript in the database and redisplay it later. Then, when the script gets displayed, the browser will execute the script.
For example, suppose your application asks the user to enter his or her name into a text box — and instead of entering a legitimate name, the user enters the following:
<script>alert(“Gotcha!”);</script>
Then this string gets saved in the user’s Name column in the application’s database. Later on, when the application retrieves the name to display it on a Web page, the browser sees the <script> element and executes the script. In this case, the script simply displays an alert dialog box with the message Gotcha! But the script could easily be up to more malicious business — for example, stealing values from cookies stored on the user’s computer.
Fortunately, ASP.NET includes built-in protection against this type of script attack. By default, every input value is checked; if the value is potentially dangerous input, the server refuses to accept it. ASP.NET throws an exception and displays an unattractive error page, as shown in Figure 3-1.

76 |
Part II: Building Secure Applications |
Figure 3-1:
The error page displayed when a user enters potentially dangerous input.
You can manually disable this process that checks for dangerous input — page by page — if you add ValidateRequest=”False” to the Page directive for each page. Or you can disable the check for an entire site by adding the following code to the web.config file:
<system.web>
<pages validateRequest=”False” /> </system.web>
If you do this, however, you must be careful to manually validate any input to make sure it doesn’t contain suspicious content. The easiest way to do that is to call the HtmlEncode method of the Server class before you save any text-input data to a database. Here’s an example:
string Name = Server.HtmlEncode(txtName.Text);
This method replaces any HTML special characters (such as < and >) with codes such as < and >, which a browser will display but not execute.
By default, bound controls (that is, controls that automatically display data derived from a database) automatically encode data before they display it. As a result, XSS protection is automatic for data displayed by the GridView and other bound controls. Sure, you can disable this protection (by specifying HtmlEncode=”False” for any bound fields you don’t want encoded) — but I wouldn’t recommend it. Doing so leaves your application vulnerable to script attacks.