
- •Introduction
- •Saving Time with This Book
- •Conventions Used in This Book
- •Part II: Working with the Pre-Processor
- •Part III: Types
- •Part IV: Classes
- •Part V: Arrays and Templates
- •Part VI: Input and Output
- •Part VII: Using the Built-in Functionality
- •Part VIII: Utilities
- •Part IX: Debugging C++ Applications
- •Part X: The Scary (or Fun!) Stuff
- •Icons Used in This Book
- •Creating and Implementing an Encapsulated Class
- •Creating a Mailing-List Application
- •Testing the Mailing-List Application
- •Customizing a Class with Polymorphism
- •Testing the Virtual Function Code
- •Why Do the Destructors Work?
- •Delayed Construction
- •The cDate Class
- •Testing the cDate Class
- •Creating the Header File
- •Testing the Header File
- •The Assert Problem
- •Fixing the Assert Problem
- •Using the const Construct
- •Identifying the Errors
- •Fixing the Errors
- •Fixing What Went Wrong with the Macro
- •Using Macros Appropriately
- •Using the sizeof Function
- •Evaluating the Results
- •Using sizeof with Pointers
- •Implementing the Range Class
- •Testing the Range Class
- •Creating the Matrix Class
- •Matrix Operations
- •Multiplying a Matrix by a Scalar Value
- •Multiplying a Matrix by Scalar Values, Take 2
- •Testing the Matrix Class
- •Implementing the Enumeration Class
- •Testing the Enumeration Class
- •Implementing Structures
- •Interpreting the Output
- •Defining Constants
- •Testing the Constant Application
- •Using the const Keyword
- •Illustrating Scope
- •Interpreting the Output
- •Using Casts
- •Addressing the Compiler Problems
- •Testing the Changes
- •Implementing Member-Function Pointers
- •Updating Your Code with Member-Function Pointers
- •Testing the Member Pointer Code
- •Customizing Functions We Wrote Ourselves
- •Testing the Default Code
- •Fixing the Problem
- •Testing the Complete Class
- •Implementing Virtual Inheritance
- •Correcting the Code
- •Rules for Creating Overloaded Operators
- •Using Conversion Operators
- •Using Overloaded Operators
- •Testing the MyString Class
- •Rules for Implementing new and delete Handlers
- •Overloading new and delete Handlers
- •Testing the Memory Allocation Tracker
- •Implementing Properties
- •Testing the Property Class
- •Implementing Data Validation with Classes
- •Testing Your SSN Validator Class
- •Creating the Date Class
- •Testing the Date Class
- •Some Final Thoughts on the Date Class
- •Creating a Factory Class
- •Testing the Factory
- •Enhancing the Manager Class
- •Implementing Mix-In Classes
- •Testing the Template Classes
- •Implementing Function Templates
- •Creating Method Templates
- •Using the Vector Class
- •Creating the String Array Class
- •Working with Vector Algorithms
- •Creating an Array of Heterogeneous Objects
- •Creating the Column Class
- •Creating the Row Class
- •Creating the Spreadsheet Class
- •Testing Your Spreadsheet
- •Working with Streams
- •Testing the File-Reading Code
- •Creating the Test File
- •Reading Delimited Files
- •Testing the Code
- •Creating the XML Writer
- •Testing the XML Writer
- •Creating the Configuration-File Class
- •Setting Up Your Test File
- •Building the Language Files
- •Creating an Input Text File
- •Reading the International File
- •Testing the String Reader
- •Creating a Translator Class
- •Testing the Translator Class
- •Creating a Virtual File Class
- •Testing the Virtual File Class
- •Using the auto_ptr Class
- •Creating a Memory Safe Buffer Class
- •Throwing and Logging Exceptions
- •Dealing with Unhandled Exceptions
- •Re-throwing Exceptions
- •Creating the Wildcard Matching Class
- •Testing the Wildcard Matching Class
- •Creating the URL Codec Class
- •Testing the URL Codec Class
- •Testing the Rot13 Algorithm
- •Testing the XOR Algorithm
- •Implementing the transform Function to Convert Strings
- •Testing the String Conversions
- •Implementing the Serialization Interface
- •Creating the Buffer Class
- •Testing the Buffer Class
- •Creating the Multiple-Search-Path Class
- •Testing the Multiple-Search-Path Class
- •Testing the Flow Trace System
- •The assert Macro
- •Logging
- •Testing the Logger Class
- •Design by Contract
- •Adding Logging to the Application
- •Making Functions Inline
- •Avoiding Temporary Objects
- •Passing Objects by Reference
- •Choosing Initialization Instead of Assignment
- •Learning How Code Operates
- •Testing the Properties Class
- •Creating the Locking Mechanism
- •Testing the Locking Mechanism
- •Testing the File-Guardian Class
- •Implementing the Complex Class
- •Creating the Conversion Code
- •Testing the Conversion Code
- •A Sample Program
- •Componentizing
- •Restructuring
- •Specialization
- •Index
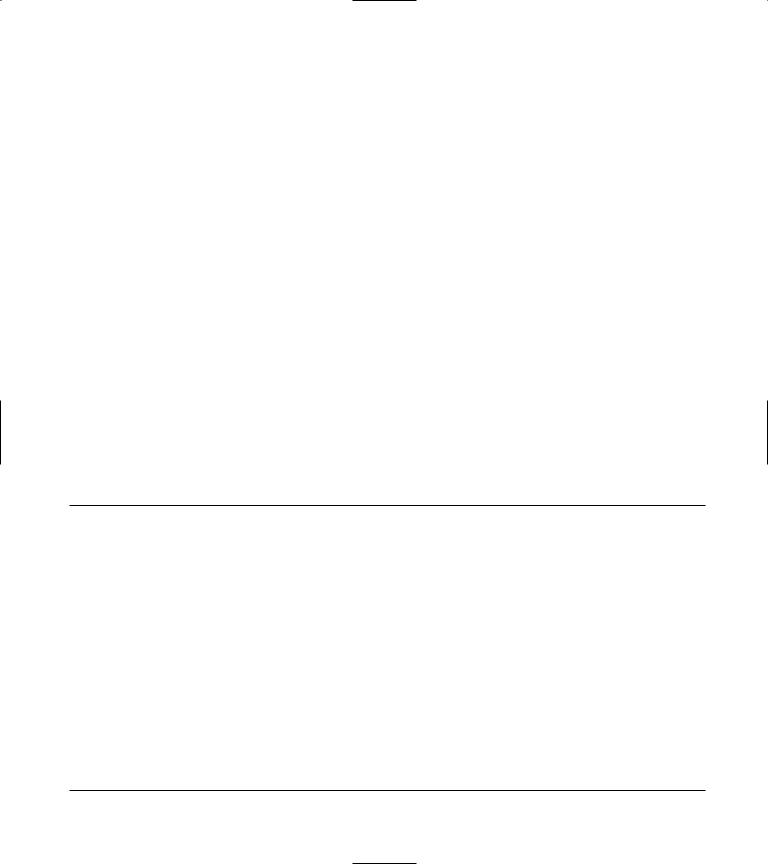
|
|
Testing the String Reader |
277 |
{ |
|
|
|
// First, see if we have this id. |
|
|
|
vector< StringIndex >::const_iterator iter; |
|
|
|
for (iter = _indices.begin(); iter != _indices.end(); ++iter ) |
|
||
if ( (*iter).getID() == id ) |
|
|
|
return _loadString( (*iter) ); |
|
|
|
return string(“”); |
|
|
|
} |
|
|
|
}; |
|
|
|
|
|
|
|
3. Save the source code as a file in your editor |
1. |
In the code editor of your choice, reopen |
|
and close the editor application. |
|
the source file to hold the code for your test |
program.
Testing the String Reader
After you create a class, you should create a test driver that not only ensures that your code is correct, but also shows people how to use your code.
The following steps show you how to create a test driver that illustrates how to read a language file, and shows how the class is intended to be used:
In this example, I named the test program ch46a.cpp.
2. Type the code from Listing 46-3 into your file.
Better yet, copy the code from the source file on this book’s companion Web site.
LISTING 46-3: THE STRINGREADER TEST DRIVER
int main(int argc, char **argv)
{
if ( argc < 3 )
{
printf(“Usage: |
ch6_1 string-file-name id1 [id2, ..]\n”); |
|
printf(“Where: |
string-file-name |
is the name of the compressed string file to use\n”); |
printf(“ |
id1..etc are the |
ids to display\n”); |
return -1; |
|
|
}
StringReader sReader(argv[1]);
for ( int i=2; i<argc; ++i )
{
int id = atoi(argv[i]);
string s = sReader.getString( id ); printf(“String %d: [%s]\n”, id, s.c_str() );
}
return 0;
}

278 Technique 46: Creating an Internationalization Class
3. Save the source-code file in the code editor.
4. Compile and run the source file with your favorite compiler on your favorite operating system.
If you have done everything right, running the application with the arguments shown should produce the following result on your console window:
$ ./a.exe my.eng 2 3 5 9 String 2: [Goodbye] String 3: [Why me?] String 5: [French] String 9: []
You will also see the diagnostics, which are not shown, for the application. If you do not wish to
view the diagnostics, comment out the dump method call shown at line 6 in Listing 46-2.
By looking at the output above, we can see that when we read data back in from the language file, using the indices we have defined in the program, we get back the same strings that we put there in the original language text file. This shows that the program is working properly and that we are getting the language data directly from the file — not from strings embedded in the application.

47 Hashing Out
Translations
Technique
Save Time By
Understanding hash tables
Creating a translation application with hash tables
Creating a translationtext file
Testing your application
The hash table is one of the most valuable constructs in the Standard Template Library. Hash tables are data structures that contain two types of values, usually key-value pairs. A hash table is
used anytime you want to either replace one value with another, or map a given key to a given value. (As you may imagine, it’s a commonly used encryption tool.)
One of the most useful things that you can do with a hash table is to store and look up existing values and replace them with new ones. For example, in a translation application, it would be nice to be able to build a dictionary of words and their resulting translations. The ability to retrieve information based on a key-value pair can save a lot of time in an application. For example, a search and replace feature could use this functionality. Alternatively, you could use this functionality to implement a command parser, with a mapping of strings to integer values. Using hash tables can save you a lot of time in translating one type of input to another in your applications. In this technique, we will look at that exact problem and how to solve it in your application code.
Creating a Translator Class
We will use the hash table function to create a class that “translates” text by replacing certain keywords in the text with other words. Imagine that we want to replace all occurrences of the word computer with the word pc, for example. This type of conversion is commonly referred to as a filter, because it takes input and filters it to a new output format. In this section we will create the Translator class, which will store the text we wish to replace, along with the text we wish to insert in the place of the original.
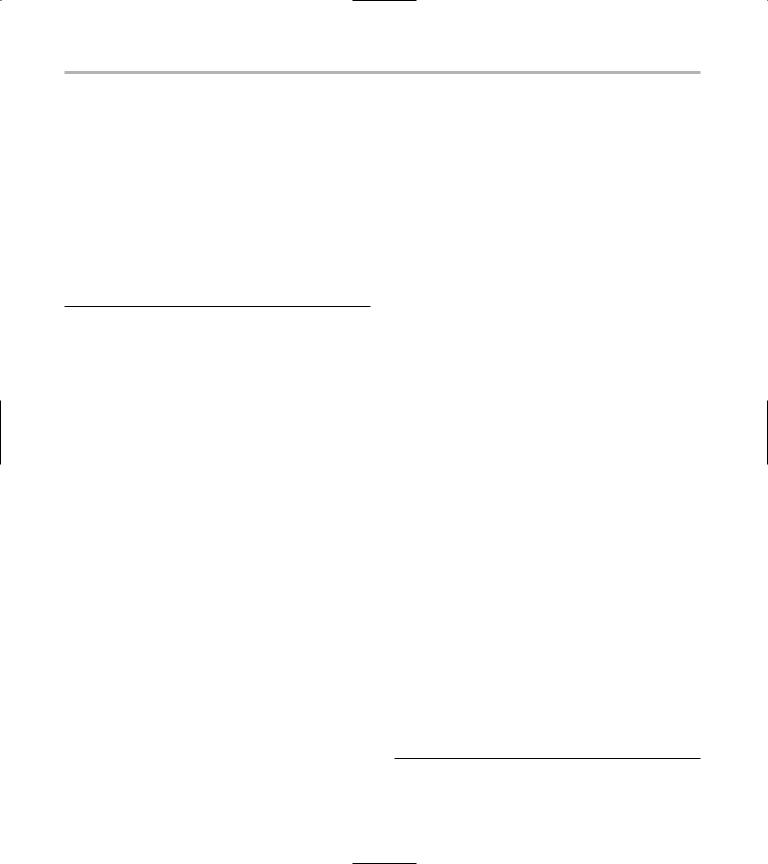
280 Technique 47: Hashing Out Translations
1. In the code editor of your choice, create a new file to hold the code for the source file of the technique.
In this example, the file is named ch47, although you can use whatever you choose.
2. Type the following code from Listing 47-1 into your file.
Better yet, copy the code from the source file on this book’s companion Web site.
LISTING 47-1: THE TRANSLATOR CLASS
#include <stdio.h> #include <string> #include <map> #include <fstream> #include <iostream>
using namespace std;
class Translator |
|
|
|
|
{ |
|
|
|
|
private: |
|
|
|
|
string |
_fileName; |
|
|
2 |
map<string,string> |
_dictionary; |
|
||
protected: |
|
|
|
|
void Clear() |
|
|
|
|
{ |
|
|
|
|
_dictionary.erase( |
|
|
|
|
_dictionary.begin(), _dictionary.end() ); |
||||
} |
|
|
|
1 |
bool Load( const char *fileName ) |
|
|||
{ |
|
|
|
ifstream in(fileName); if ( in.fail() )
return false;
// Just read in pairs of values.
while ( !in.eof() )
{
string word;
string replacement; in >> word;
if ( word.length() )
{
in >> replacement; _dictionary[word] = replace-
ment;
}
}
return true;
}
public:
Translator( void )
{
}
Translator( const char *fileName )
{
Clear();
setFileName( fileName );
}
Translator( const Translator& aCopy )
{
Clear();
setFileName( aCopy.getFileName() );
}
Translator operator=( const Translator& aCopy )
{
Clear();
setFileName( aCopy.getFileName() );
} |
|
|
void setFileName( const string& |
|
|
sFileName ) |
|
|
{ |
|
|
_fileName = sFileName; |
|
|
Load(_fileName.c_str()); |
|
|
} |
|
|
string getFileName( void ) const |
|
|
{ |
|
|
return _fileName; |
|
|
} |
|
3 |
string replace( string in ) |
|
|
{ |
|
map<string,string>::iterator iter;
iter = _dictionary.find( in );
if ( iter != _dictionary.end() )
{
return iter->second;
}
return in;
}
};