
- •Introduction
- •Saving Time with This Book
- •Conventions Used in This Book
- •Part II: Working with the Pre-Processor
- •Part III: Types
- •Part IV: Classes
- •Part V: Arrays and Templates
- •Part VI: Input and Output
- •Part VII: Using the Built-in Functionality
- •Part VIII: Utilities
- •Part IX: Debugging C++ Applications
- •Part X: The Scary (or Fun!) Stuff
- •Icons Used in This Book
- •Creating and Implementing an Encapsulated Class
- •Creating a Mailing-List Application
- •Testing the Mailing-List Application
- •Customizing a Class with Polymorphism
- •Testing the Virtual Function Code
- •Why Do the Destructors Work?
- •Delayed Construction
- •The cDate Class
- •Testing the cDate Class
- •Creating the Header File
- •Testing the Header File
- •The Assert Problem
- •Fixing the Assert Problem
- •Using the const Construct
- •Identifying the Errors
- •Fixing the Errors
- •Fixing What Went Wrong with the Macro
- •Using Macros Appropriately
- •Using the sizeof Function
- •Evaluating the Results
- •Using sizeof with Pointers
- •Implementing the Range Class
- •Testing the Range Class
- •Creating the Matrix Class
- •Matrix Operations
- •Multiplying a Matrix by a Scalar Value
- •Multiplying a Matrix by Scalar Values, Take 2
- •Testing the Matrix Class
- •Implementing the Enumeration Class
- •Testing the Enumeration Class
- •Implementing Structures
- •Interpreting the Output
- •Defining Constants
- •Testing the Constant Application
- •Using the const Keyword
- •Illustrating Scope
- •Interpreting the Output
- •Using Casts
- •Addressing the Compiler Problems
- •Testing the Changes
- •Implementing Member-Function Pointers
- •Updating Your Code with Member-Function Pointers
- •Testing the Member Pointer Code
- •Customizing Functions We Wrote Ourselves
- •Testing the Default Code
- •Fixing the Problem
- •Testing the Complete Class
- •Implementing Virtual Inheritance
- •Correcting the Code
- •Rules for Creating Overloaded Operators
- •Using Conversion Operators
- •Using Overloaded Operators
- •Testing the MyString Class
- •Rules for Implementing new and delete Handlers
- •Overloading new and delete Handlers
- •Testing the Memory Allocation Tracker
- •Implementing Properties
- •Testing the Property Class
- •Implementing Data Validation with Classes
- •Testing Your SSN Validator Class
- •Creating the Date Class
- •Testing the Date Class
- •Some Final Thoughts on the Date Class
- •Creating a Factory Class
- •Testing the Factory
- •Enhancing the Manager Class
- •Implementing Mix-In Classes
- •Testing the Template Classes
- •Implementing Function Templates
- •Creating Method Templates
- •Using the Vector Class
- •Creating the String Array Class
- •Working with Vector Algorithms
- •Creating an Array of Heterogeneous Objects
- •Creating the Column Class
- •Creating the Row Class
- •Creating the Spreadsheet Class
- •Testing Your Spreadsheet
- •Working with Streams
- •Testing the File-Reading Code
- •Creating the Test File
- •Reading Delimited Files
- •Testing the Code
- •Creating the XML Writer
- •Testing the XML Writer
- •Creating the Configuration-File Class
- •Setting Up Your Test File
- •Building the Language Files
- •Creating an Input Text File
- •Reading the International File
- •Testing the String Reader
- •Creating a Translator Class
- •Testing the Translator Class
- •Creating a Virtual File Class
- •Testing the Virtual File Class
- •Using the auto_ptr Class
- •Creating a Memory Safe Buffer Class
- •Throwing and Logging Exceptions
- •Dealing with Unhandled Exceptions
- •Re-throwing Exceptions
- •Creating the Wildcard Matching Class
- •Testing the Wildcard Matching Class
- •Creating the URL Codec Class
- •Testing the URL Codec Class
- •Testing the Rot13 Algorithm
- •Testing the XOR Algorithm
- •Implementing the transform Function to Convert Strings
- •Testing the String Conversions
- •Implementing the Serialization Interface
- •Creating the Buffer Class
- •Testing the Buffer Class
- •Creating the Multiple-Search-Path Class
- •Testing the Multiple-Search-Path Class
- •Testing the Flow Trace System
- •The assert Macro
- •Logging
- •Testing the Logger Class
- •Design by Contract
- •Adding Logging to the Application
- •Making Functions Inline
- •Avoiding Temporary Objects
- •Passing Objects by Reference
- •Choosing Initialization Instead of Assignment
- •Learning How Code Operates
- •Testing the Properties Class
- •Creating the Locking Mechanism
- •Testing the Locking Mechanism
- •Testing the File-Guardian Class
- •Implementing the Complex Class
- •Creating the Conversion Code
- •Testing the Conversion Code
- •A Sample Program
- •Componentizing
- •Restructuring
- •Specialization
- •Index

Testing the cDate Class |
35 |
LISTING 5-2: NON-INLINE METHODS
bool cDate::IsValidDate( int day, int month, long year )
{
// Is the month valid?
if ( month < 0 || month > 11 ) return false;
// Is the year valid?
if ( year < 0 || year > 9999 ) return false;
//Is the number of days valid for this month/year? if ( day < 0 || day > MonthDays(month, year) )
return false;
//Must be ok
return true;
} |
|
bool cDate::IsLeapYear( long year ) |
|
{ |
|
int year_end = year % 100; |
// year in century |
if (((year%4) == 0) && ((year_end !=0) || ((year%400) == 0))) return true;
return false;
}
Putting this code into a single object and sharing that code among various projects that might need this functionality offers some obvious advantages:
If the code needs to be changed, for example, to account for some bug in the leap year calculation, this change can all be done in one place.
More importantly, if changes are made to implement a newer, faster way to calculate the leap year or the day of the week, or even to add functionality, none of those changes affect the calling programs in the least. They will still work with the interface as it stands now.
Testing the cDate Class
After you create a class, it is important to create a test driver — doing so not only ensures that your code is correct, but also shows people how to use your code.
1. In the code editor of your choice, reopen the source file to hold the code for your test program.
In this example, I named the test program ch1_5.cpp.
2. Type the code from Listing 5-3 into your file.
Better yet, copy the code from the source file on this book’s companion Web site.
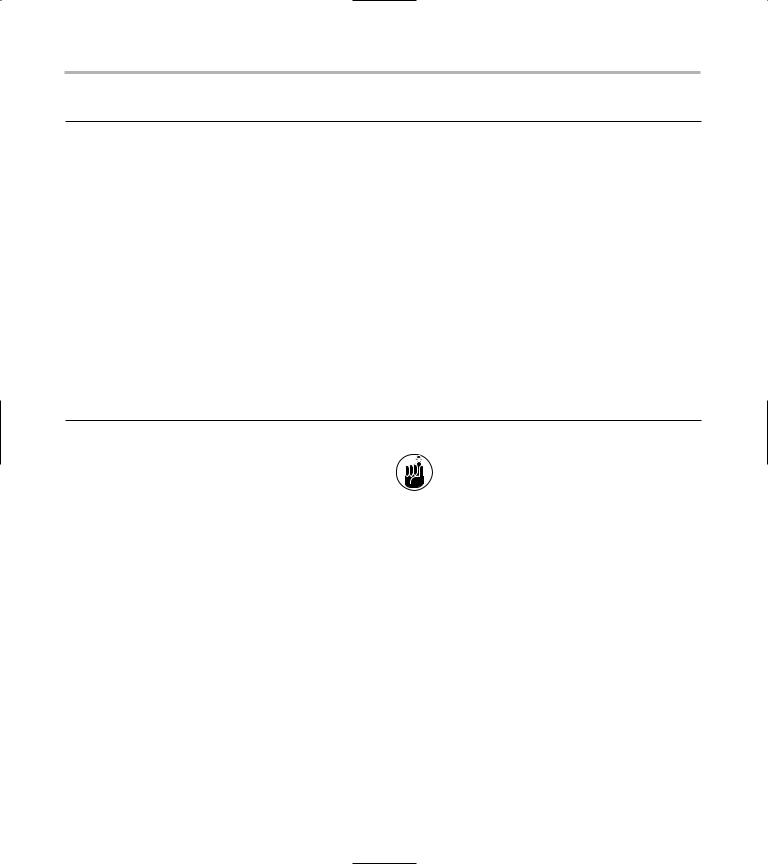
36 Technique 5: Separating Rules and Data from Code
LISTING 5-3: THE CDATE CLASS TEST PROGRAM
#include <iostream> using namespace std;
int main(int argc, char **argv)
{
//Do some testing. First, a valid date cDate d1(31, 11, 2004);
//Now, an invalid one.
cDate d2(31, 12, 2004);
//Finally, let’s just create a blank one. cDate d3;
//Print them out
cout << “D1: “ << “Month: “ << d1.Month() << “ Day: “ << d1.Day() << “ Year: “ << d1.Year() << endl;
cout << “D2: “ << “Month: “ << d2.Month() << “ Day: “ << d2.Day() << “ Year: “ << d2.Year() << endl;
cout << “D3: “ << “Month: “ << d3.Month() << “ Day: “ << d3.Day() << “ Year: “ << d3.Year() << endl;
return 0;
}
3. Save the source code as a file in your code editor and close the editor application.
4. Compile the source code with your favorite compiler on your favorite operating system.
5. Run the program on your favorite operating system console.
If you have done everything properly, you should see the following output from the program on the console window:
$ ./a.exe
D1: Month: 11 Day: 31 Year: 2004 D2: Month: 2011708128 Day: -1 Year:
2011671585
D3: Month: 8 Day: 7 Year: 2004
Note that the numbers shown in the output may be different on your computer, because they are somewhat random. You should simply expect to see very invalid values.
This, then, is the advantage to working with objectoriented programming in C++: You can make changes “behind the scenes” without interfering with the work of others. You make it possible for people to get access to data and algorithms without having to struggle with how they’re stored or implemented. Finally, you can fix or extend the implementations
of your algorithms without requiring your users to change all their applications that use those algorithms.

Part II
Working with the Pre-Processor
