
- •Contents
- •Preface to the Second Edition
- •Introduction
- •Rails Is Agile
- •Finding Your Way Around
- •Acknowledgments
- •Getting Started
- •The Architecture of Rails Applications
- •Models, Views, and Controllers
- •Active Record: Rails Model Support
- •Action Pack: The View and Controller
- •Installing Rails
- •Your Shopping List
- •Installing on Windows
- •Installing on Mac OS X
- •Installing on Linux
- •Development Environments
- •Rails and Databases
- •Rails and ISPs
- •Creating a New Application
- •Hello, Rails!
- •Linking Pages Together
- •What We Just Did
- •Building an Application
- •The Depot Application
- •Incremental Development
- •What Depot Does
- •Task A: Product Maintenance
- •Iteration A1: Get Something Running
- •Iteration A2: Add a Missing Column
- •Iteration A3: Validate!
- •Iteration A4: Prettier Listings
- •Task B: Catalog Display
- •Iteration B1: Create the Catalog Listing
- •Iteration B4: Linking to the Cart
- •Task C: Cart Creation
- •Sessions
- •Iteration C1: Creating a Cart
- •Iteration C2: A Smarter Cart
- •Iteration C3: Handling Errors
- •Iteration C4: Finishing the Cart
- •Task D: Add a Dash of AJAX
- •Iteration D1: Moving the Cart
- •Iteration D3: Highlighting Changes
- •Iteration D4: Hide an Empty Cart
- •Iteration D5: Degrading If Javascript Is Disabled
- •What We Just Did
- •Task E: Check Out!
- •Iteration E1: Capturing an Order
- •Task F: Administration
- •Iteration F1: Adding Users
- •Iteration F2: Logging In
- •Iteration F3: Limiting Access
- •Iteration F4: A Sidebar, More Administration
- •Task G: One Last Wafer-Thin Change
- •Generating the XML Feed
- •Finishing Up
- •Task T: Testing
- •Tests Baked Right In
- •Unit Testing of Models
- •Functional Testing of Controllers
- •Integration Testing of Applications
- •Performance Testing
- •Using Mock Objects
- •The Rails Framework
- •Rails in Depth
- •Directory Structure
- •Naming Conventions
- •Logging in Rails
- •Debugging Hints
- •Active Support
- •Generally Available Extensions
- •Enumerations and Arrays
- •String Extensions
- •Extensions to Numbers
- •Time and Date Extensions
- •An Extension to Ruby Symbols
- •with_options
- •Unicode Support
- •Migrations
- •Creating and Running Migrations
- •Anatomy of a Migration
- •Managing Tables
- •Data Migrations
- •Advanced Migrations
- •When Migrations Go Bad
- •Schema Manipulation Outside Migrations
- •Managing Migrations
- •Tables and Classes
- •Columns and Attributes
- •Primary Keys and IDs
- •Connecting to the Database
- •Aggregation and Structured Data
- •Miscellany
- •Creating Foreign Keys
- •Specifying Relationships in Models
- •belongs_to and has_xxx Declarations
- •Joining to Multiple Tables
- •Acts As
- •When Things Get Saved
- •Preloading Child Rows
- •Counters
- •Validation
- •Callbacks
- •Advanced Attributes
- •Transactions
- •Action Controller: Routing and URLs
- •The Basics
- •Routing Requests
- •Action Controller and Rails
- •Action Methods
- •Cookies and Sessions
- •Caching, Part One
- •The Problem with GET Requests
- •Action View
- •Templates
- •Using Helpers
- •How Forms Work
- •Forms That Wrap Model Objects
- •Custom Form Builders
- •Working with Nonmodel Fields
- •Uploading Files to Rails Applications
- •Layouts and Components
- •Caching, Part Two
- •Adding New Templating Systems
- •Prototype
- •Script.aculo.us
- •RJS Templates
- •Conclusion
- •Action Mailer
- •Web Services on Rails
- •Dispatching Modes
- •Using Alternate Dispatching
- •Method Invocation Interception
- •Testing Web Services
- •Protocol Clients
- •Secure and Deploy Your Application
- •Securing Your Rails Application
- •SQL Injection
- •Creating Records Directly from Form Parameters
- •Avoid Session Fixation Attacks
- •File Uploads
- •Use SSL to Transmit Sensitive Information
- •Knowing That It Works
- •Deployment and Production
- •Starting Early
- •How a Production Server Works
- •Repeatable Deployments with Capistrano
- •Setting Up a Deployment Environment
- •Checking Up on a Deployed Application
- •Production Application Chores
- •Moving On to Launch and Beyond
- •Appendices
- •Introduction to Ruby
- •Classes
- •Source Code
- •Resources
- •Index
- •Symbols

Chapter 2
The Architecture of Rails Applications
One of the interesting features of Rails is that it imposes some fairly serious constraints on how you structure your web applications. Surprisingly, these constraints make it easier to create applications—a lot easier. Let’s see why.
2.1Models, Views, and Controllers
Back in 1979, Trygve Reenskaug came up with a new architecture for developing interactive applications. In his design, applications were broken into three types of components: models, views, and controllers.
The model is responsible for maintaining the state of the application. Sometimes this state is transient, lasting for just a couple of interactions with the user. Sometimes the state is permanent and will be stored outside the application, often in a database.
A model is more than just data; it enforces all the business rules that apply to that data. For example, if a discount shouldn’t be applied to orders of less than $20, the model will enforce the constraint. This makes sense; by putting the implementation of these business rules in the model, we make sure that nothing else in the application can make our data invalid. The model acts as both a gatekeeper and a data store.
The view is responsible for generating a user interface, normally based on data in the model. For example, an online store will have a list of products to be displayed on a catalog screen. This list will be accessible via the model, but it will be a view that accesses the list from the model and formats it for the end user. Although the view may present the user with various ways of inputting data, the view itself never handles incoming data. The view’s work is done once the data is displayed. There may well be many views that access the same model data, often for different purposes. In the online store, there’ll
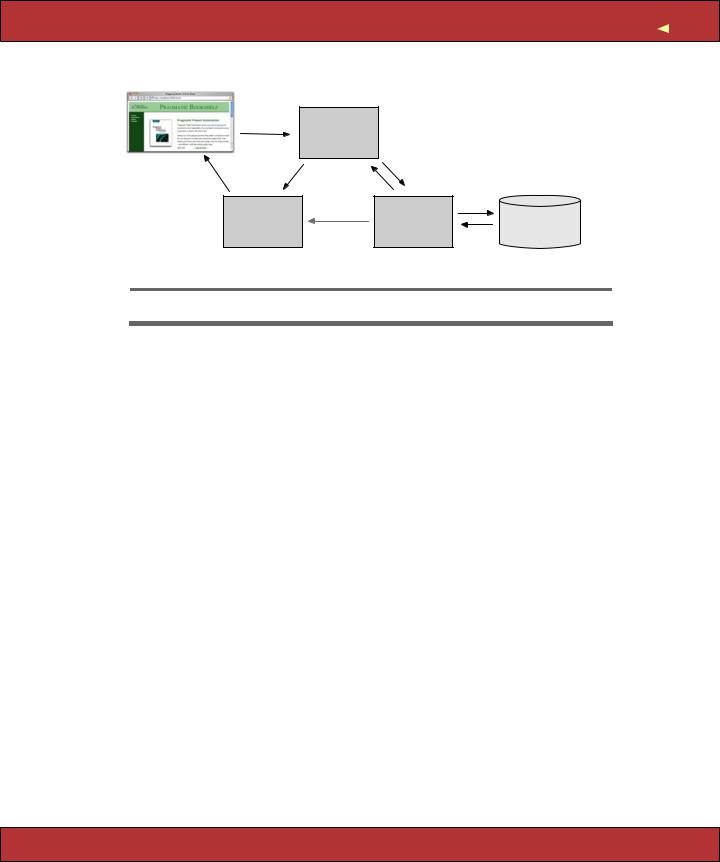
MODELS, VIEWS, AND CONTROLLERS |
23 |
|
|
! Browser sends request |
|
|
|
" Controller interacts with model |
|
|
! |
# Controller invokes view |
|
|
$ View renders next browser screen |
||
|
Controller |
||
$ |
# |
" |
|
|
View |
Model |
Database |
Figure 2.1: The Model-View-Controller Architecture
be a view that displays product information on a catalog page and another set of views used by administrators to add and edit products.
Controllers orchestrate the application. Controllers receive events from the outside world (normally user input), interact with the model, and display an appropriate view to the user.
This triumvirate—the model, view, and controller—together form an architecture known as MVC. Figure 2.1 shows MVC in abstract terms.
MVC was originally intended for conventional GUI applications, where developers found the separation of concerns led to far less coupling, which in turn made the code easier to write and maintain. Each concept or action was expressed in just one well-known place. Using MVC was like constructing a skyscraper with the girders already in place—it was a lot easier to hang the rest of the pieces with a structure already there.
In the software world, we often ignore good ideas from the past as we rush headlong to meet the future. When developers first started producing web applications, they went back to writing monolithic programs that intermixed presentation, database access, business logic, and event handling in one big ball of code. But ideas from the past slowly crept back in, and folks started experimenting with architectures for web applications that mirrored the 20- year-old ideas in MVC. The results were frameworks such as WebObjects, Struts, and JavaServer Faces. All are based (with varying degrees of fidelity) on the ideas of MVC.
Report erratum
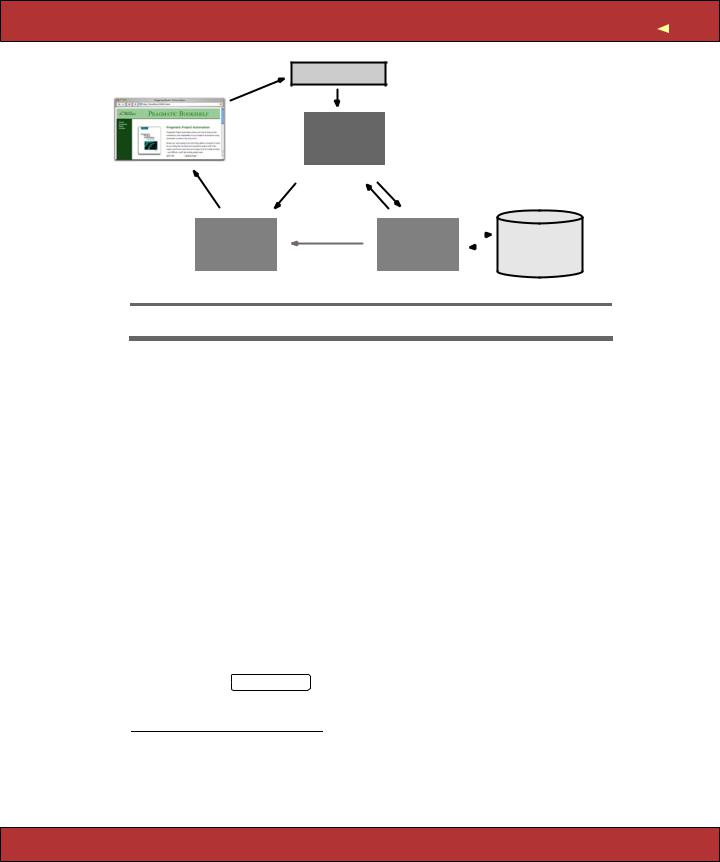
MODELS, VIEWS, AND CONTROLLERS |
24 |
! |
Routing |
! http://my.url/store/add_to_cart/123 |
|
"" Routing finds Store controller
|
|
|
|
|
|
|
|
|
|
|
# Controller interacts with model |
|||||||
|
|
|
|
|
|
|
|
|
|
|
||||||||
|
|
|
|
|
|
|
|
|
|
|
$ Controller invokes view |
|||||||
|
|
|
|
|
|
|
|
Store |
|
|
||||||||
|
|
|
|
|
|
|
|
|
|
%&View renders next browser screen |
||||||||
|
|
|
|
|
|
|
|
Controller |
|
|
|
|
|
|
|
|
|
|
|
|
% |
$ |
|
|
|
# |
|
|
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Display |
|
|
|
|
|
|
|
|
Active |
|
|
|
|
|
|
Database |
|
|
|
|
|
|
|
|
|
|
|
|
|
||||||
|
|
Cart |
|
|
|
|
|
|
|
|
Record |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
|
View |
|
|
|
|
|
|
|
|
Model |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Figure 2.2: Rails and MVC
Ruby on Rails is an MVC framework, too. Rails enforces a structure for your application—you develop models, views, and controllers as separate chunks of functionality and it knits them all together as your program executes. One of the joys of Rails is that this knitting process is based on the use of intelligent defaults so that you typically don’t need to write any external configuration metadata to make it all work. This is an example of the Rails philosophy of favoring convention over configuration.
In a Rails application, incoming requests are first sent to a router, which works out where in the application the request should be sent and how the request itself should be parsed. Ultimately, this phase identifies a particular method (called an action in Rails parlance) somewhere in the controller code. The action might look at data in the request itself, it might interact with the model, and it might cause other actions to be invoked. Eventually the action prepares information for the view, which renders something to the user.
Figure 2.2, shows how Rails handles an incoming request. In this example, the application has previously displayed a product catalog page and the user has just clicked the Add To Cart button next to one of the products. This button links to http://my.url/store/add_to_cart/123, where add_to_cart is an action in our application and 123 is our internal id for the selected product.1
1. We cover the format of Rails URLs later in the book. However, it’s worth pointing out here that having URLs perform actions such as add to cart can be dangerous. See Section 21.6, The Problem with GET Requests, on page 462 for more details.
Report erratum
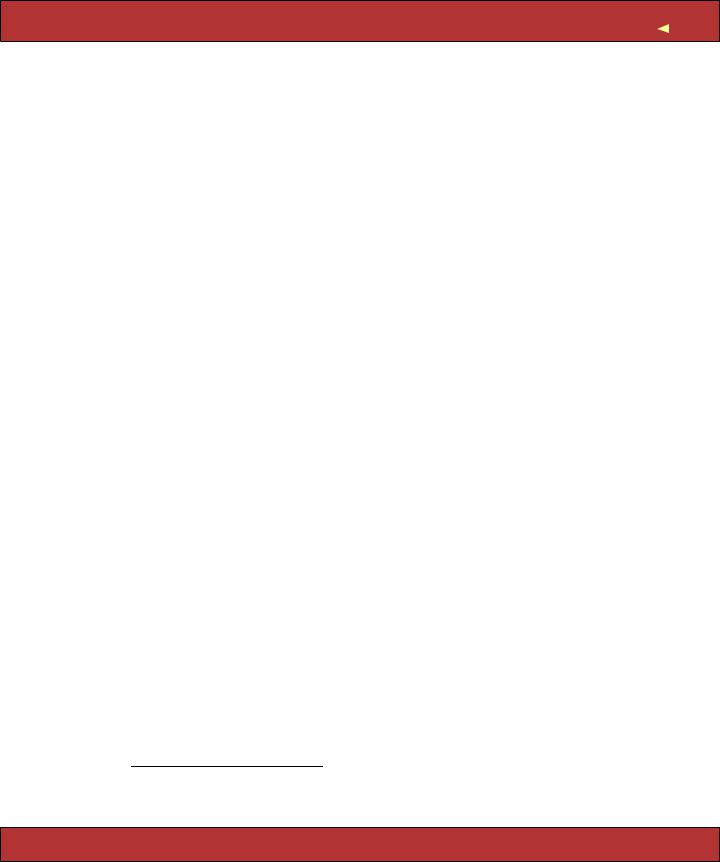
ACTIVE RECORD: RAILS MODEL SUPPOR T |
25 |
The routing component receives the incoming request and immediately picks it apart. In this simple case, it takes the first part of the path, store, as the name of the controller and the second part, add_to_cart, as the name of an action. The last part of the path, 123, is by convention extracted into an internal parameter called id. As a result of all this analysis, the router knows it has to invoke the add_to_cart method in the controller class StoreController (we’ll talk about naming conventions on page 240).
The add_to_cart method handles user requests. In this case it finds the current user’s shopping cart (which is an object managed by the model). It also asks the model to find the information for product 123. It then tells the shopping cart to add that product to itself. (See how the model is being used to keep track of all the business data; the controller tells it what to do, and the model knows how to do it.)
Now that the cart includes the new product, we can show it to the user. The controller arranges things so that the view has access to the cart object from the model, and it invokes the view code. In Rails, this invocation is often implicit; again conventions help link a particular view with a given action.
That’s all there is to an MVC web application. By following a set of conventions and partitioning your functionality appropriately, you’ll discover that your code becomes easier to work with and your application becomes easier to extend and maintain. Seems like a good trade.
If MVC is simply a question of partitioning your code a particular way, you might be wondering why you need a framework such as Ruby on Rails. The answer is straightforward: Rails handles all of the low-level housekeeping for you—all those messy details that take so long to handle by yourself—and lets you concentrate on your application’s core functionality. Let’s see how....
2.2Active Record: Rails Model Support
In general, we’ll want our web applications to keep their information in a relational database. Order-entry systems will store orders, line items, and customer details in database tables. Even applications that normally use unstructured text, such as weblogs and news sites, often use databases as their backend data store.
Although it might not be immediately apparent from the SQL2 you use to access them, relational databases are actually designed around mathematical set theory. Although this is good from a conceptual point of view, it makes it difficult to combine relational databases with object-oriented programming
2. SQL, referred to by some as Structured Query Language, is the language used to query and update relational databases.
Report erratum
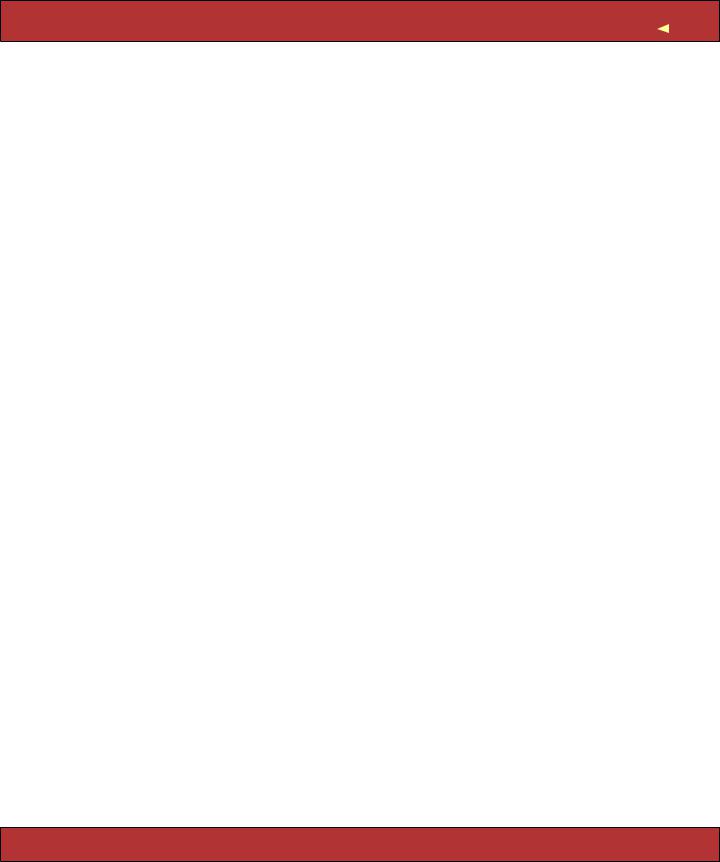
ACTIVE RECORD: RAILS MODEL SUPPOR T |
26 |
languages. Objects are all about data and operations, and databases are all about sets of values. Operations that are easy to express in relational terms are sometimes difficult to code in an OO system. The reverse is also true.
Over time, folks have worked out ways of reconciling the relational and OO views of their corporate data. Let’s look at two different approaches. One organizes your program around the database; the other organizes the database around your program.
Database-centric Programming
The first folks who coded against relational databases programmed in procedural languages such as C and COBOL. These folks typically embedded SQL directly into their code, either as strings or by using a preprocessor that converted SQL in their source into lower-level calls to the database engine.
The integration meant that it became natural to intertwine the database logic with the overall application logic. A developer who wanted to scan through orders and update the sales tax in each order might write something exceedingly ugly, such as
EXEC SQL BEGIN DECLARE SECTION; int id;
float amount;
EXEC SQL END DECLARE SECTION;
EXEC SQL DECLARE c1 AS CURSOR FOR select id, amount from orders;
while (1) { float tax;
EXEC SQL WHENEVER NOT FOUND DO break; EXEC SQL FETCH c1 INTO :id, :amount; tax = calc_sales_tax(amount)
EXEC SQL UPDATE orders set tax = :tax where id = :id;
}
EXEC SQL CLOSE c1; EXEC SQL COMMIT WORK;
Scary stuff, eh? Don’t worry. We won’t be doing any of this, even though this style of programming is common in scripting languages such as Perl and PHP. It’s also available in Ruby. For example, we could use Ruby’s DBI library to produce similar-looking code. (This example, like the previous one, has no error checking.)
Method definition
֒→ page 632
def update_sales_tax
update = @db.prepare("update orders set tax=? where id=?") @db.select_all("select id, amount from orders") do |id, amount|
tax = calc_sales_tax(amount) update.execute(tax, id)
end end
Report erratum
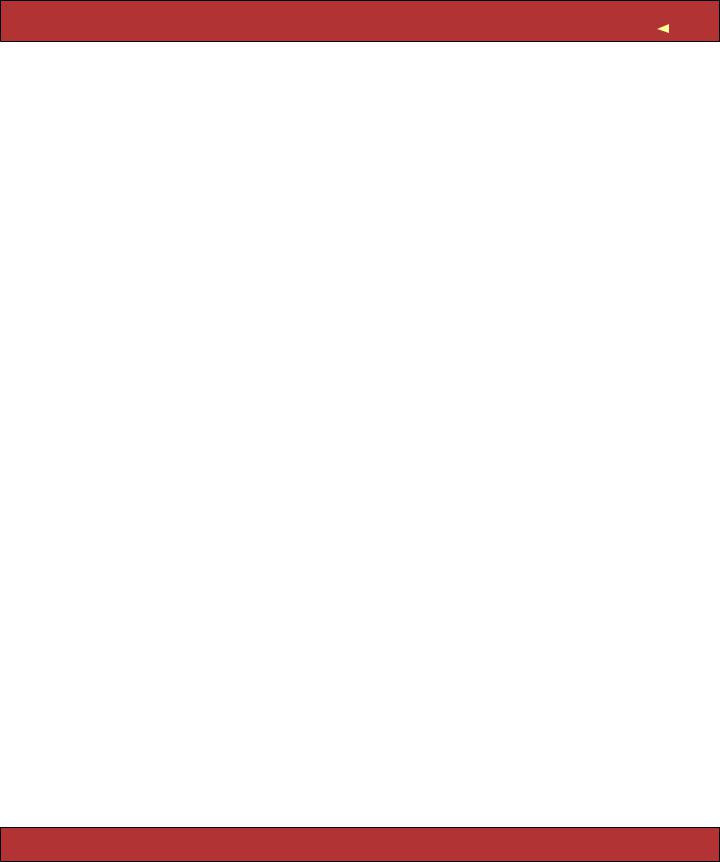
ACTIVE RECORD: RAILS MODEL SUPPOR T |
27 |
This approach is concise and straightforward and indeed is widely used. It seems like an ideal solution for small applications. However, there is a problem. Intermixing business logic and database access like this can make it hard to maintain and extend the applications in the future. And you still need to know SQL just to get started on your application.
Say, for example, our enlightened state government passes a new law that says we have to record the date and time that sales tax was calculated. That’s not a problem, we think. We just have to get the current time in our loop, add a column to the SQL update statement, and pass the time to the execute call.
But what happens if we set the sales tax column in many different places in the application? Now we’ll need to go through and find all these places, updating each. We have duplicated code, and (if we miss a place where the column is set) we have a source of errors.
In regular programming, object orientation has taught us that encapsulation solves these types of problems. We’d wrap everything to do with orders in a class; we’d have a single place to update when the regulations change.
Folks have extended these ideas to database programming. The basic premise is trivially simple. We wrap access to the database behind a layer of classes. The rest of our application uses these classes and their objects—it never interacts with the database directly. This way we’ve encapsulated all the schemaspecific stuff into a single layer and decoupled our application code from the low-level details of database access. In the case of our sales tax change, we’d simply change the class that wrapped the orders table to update the time stamp whenever the sales tax was changed.
In practice this concept is harder to implement than it might appear. Real-life database tables are interconnected (an order might have multiple line items, for example), and we’d like to mirror this in our objects: the order object should contain a collection of line item objects. But we then start getting into issues of object navigation, performance, and data consistency. When faced with these complexities, the industry did what it always does: it invented a three-letter acronym: ORM, which stands for object-relational mapping. Rails uses ORM.
Object-Relational Mapping
ORM libraries map database tables to classes. If a database has a table called orders, our program will have a class named Order. Rows in this table correspond to objects of the class—a particular order is represented as an object of class Order. Within that object, attributes are used to get and set the individual columns. Our Order object has methods to get and set the amount, the sales tax, and so on.
Report erratum
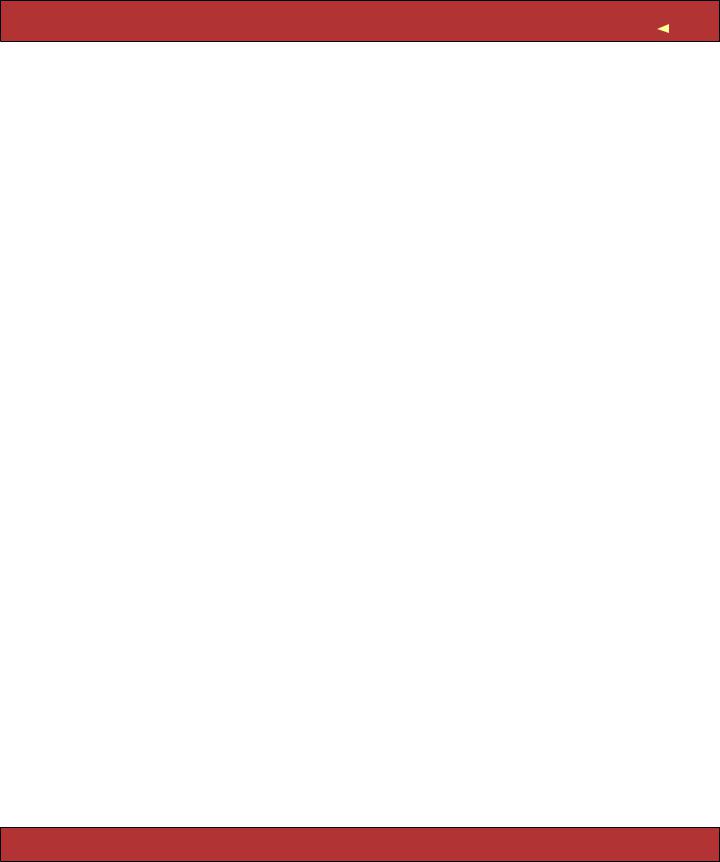
ACTIVE RECORD: RAILS MODEL SUPPOR T |
28 |
In addition, the Rails classes that wrap our database tables provide a set of class-level methods that perform table-level operations. For example, we might need to find the order with a particular id. This is implemented as a class method that returns the corresponding Order object. In Ruby code, this might look like
order = Order.find(1)
puts "Order #{order.customer_id}, amount=#{order.amount}"
class method
֒→ page 634
puts
֒→ page 632
Sometimes these class-level methods return collections of objects.
Order.find(:all, :conditions => "name='dave'" ).each do |order| puts order.amount
end
Finally, the objects corresponding to individual rows in a table have methods that operate on that row. Probably the most widely used is save, the operation that saves the row to the database.
Order.find(:all, :conditions => "name='dave'" ).each do |order| order.discount = 0.5
order.save end
So an ORM layer maps tables to classes, rows to objects, and columns to attributes of those objects. Class methods are used to perform table-level operations, and instance methods perform operations on the individual rows.
In a typical ORM library, you supply configuration data to specify the mappings between entities in the database and entities in the program. Programmers using these ORM tools often find themselves creating and maintaining a boatload of XML configuration files.
iterating
֒→ page 639
Active Record
Active Record is the ORM layer supplied with Rails. It closely follows the standard ORM model: tables map to classes, rows to objects, and columns to object attributes. It differs from most other ORM libraries in the way it is configured. By relying on convention and starting with sensible defaults, Active Record minimizes the amount of configuration that developers perform. To illustrate this, here’s a program that uses Active Record to wrap our orders table.
require 'active_record'
class Order < ActiveRecord::Base end
order = Order.find(1) order.discount = 0.5 order.save
Report erratum