
- •Table of Contents
- •Introduction
- •Saving Time with This Book
- •Foolish Assumptions
- •Part I: Making the Desktop Work for You
- •Part II: Getting the Most from Your File System
- •Part III: Good Housekeeping with Linux
- •Part IV: Tweaking the Kernel on Your Linux System
- •Part V: Securing Your Workspace
- •Part VI: Networking Like a Professional
- •Part VII: Monitoring Your System
- •Part VIII: Serving Up the Internet and More
- •Part X: Programming Tricks
- •Part XI: The Scary (Or Fun!) Stuff
- •Icons Used in This Book
- •Discovering Your Protocols
- •Managing Snapshots with the camera: Protocol
- •Remote File Management with fish:
- •Getting Help with help:, info:, and man:
- •Other KDE Protocols
- •Using GNOME VFS Modules
- •Stacking VFS Modules
- •Working with Packages: rpm and rpms
- •Putting VFS to Work at the Command Line
- •Burning CDs with a VFS
- •Skinning Your Desktop with VFS
- •Classifying Data with MIME
- •Creating KDE File Associations
- •Creating New MIME Types with GNOME
- •Making Basic Prompt Transformations
- •Adding Dynamically Updated Data to Your Prompt
- •Colorizing Your Prompt
- •Seeing a Red Alert When You Have Superuser Privileges
- •Saving Your Work
- •Completing Names Automatically
- •Using the Escape Key to Your Advantage
- •Customizing Completion for Maximum Speed
- •Using cd and ls to Navigate through bash
- •Setting Your CDPATH Variables to Find Directories Fast
- •Streamlining Archive Searches
- •Turning the Output of a Command into a Variable with $( )
- •Using $UID and $EUID in Shell Scripts
- •Customizing Variables for Rapid Transit
- •Finding the Right Shell Script
- •Choosing your victims
- •Timing is everything
- •Cleaning up made easy
- •Changing prototype scripts
- •Customizing Your Autostart File
- •Navigating the History List
- •Scrolling
- •Summoning a command by number
- •Searching through history
- •Customizing the History List
- •Adjusting key default settings
- •Filtering the history list
- •Executing Commands Quickly with History Variables
- •Viewing Your Aliases
- •Using Aliases for Complex Commands
- •Automating Tedious Tasks with Functions
- •Filtering file searches by file type
- •Automatic downloading
- •Monitoring Your System in a Snap
- •Un-tarring the Easy Way
- •What Is Samba?
- •Getting Up and Running with Samba
- •Checking whether Samba is installed
- •Enabling Samba
- •Adjusting the workgroup name and creating user accounts
- •Giving a Windows machine access to your home directory
- •Sharing Linux files and directories with other computers
- •Hooking Everyone Up to the Printer
- •Sharing Linux printers with SWAT
- •Using a Windows printer from Linux
- •Plugging In to Remote Data with Linux Programs Quickly
- •Finding Files with locate
- •Finding Files with find
- •Qualifying Your Search with the find Command
- •Doing updated filename searches
- •Adding time-based qualifications
- •Filtering by file size
- •Perusing commonly used qualifications
- •Acting on What You Find
- •Displaying specific info with -printf
- •Checking disk usage by user
- •Executing commands with find
- •Building Complex Commands with xargs
- •Creating Archives with File Roller
- •Inspecting and Extracting Archives with File Roller
- •Adding Functionality to tar with Complex Commands
- •Building archives from the command line
- •Archiving complex search results
- •Backing up an installed package
- •Uprooting Entire Directory Trees with scp
- •Splitting Big Files into Manageable Chunks
- •Building Software from Downloaded tarballs
- •Compiling a tarball: The basic steps
- •Downloading and compiling SuperKaramba
- •Versatile Downloading with wget
- •Mirroring sites with wget
- •Verifying your bookmarks with wget
- •Downloading files with wget
- •Downloading and unpacking in one quick step
- •Downloading and Uploading with curl
- •Setting Up ADIOS
- •Downloading ADIOS
- •Burning ADIOS to CD
- •Installing ADIOS
- •Finding Your Way around UML
- •Connecting to the Internet from an ADIOS VM
- •Using a GUI with UML
- •Installing Software into UML
- •Merging Changes to Your Prototype
- •Querying RPM Packages for Content
- •Digesting Information
- •Creating a Package Index
- •Querying for Prerequisites
- •Dissecting an RPM Package
- •Using RPM at the Command Line
- •Removing RPMs
- •Flagging Down RPM
- •Getting Graphic with RPM
- •Using Rpmdrake to install from media
- •Installing from your Konqueror browser
- •Verifying Your System
- •Reading the Tamper-Proof Seal
- •Setting Up Synaptic and apt in a Snap
- •Keeping Up-to-Date with apt and Synaptic: The Basics
- •Handy Hints about Synaptic
- •Changing repositories
- •Viewing package details
- •Installing new packages with Synaptic
- •Importing the Keys to the Repository
- •Letting Task Scheduler Work for You
- •Scheduling a new task
- •Editing a task
- •Adding environment variables
- •Reining In Resources with Disk Quotas
- •Installing the quota RPM package
- •Enabling file system quotas
- •Getting your files together
- •Setting quotas
- •Reviewing your quotas
- •Using System Accounting to Keep Track of Users
- •Setting up system accounting
- •Looking up user login hours
- •Checking out command and program usage
- •Running Down the Runlevels
- •Runlevel basics
- •Customizing runlevels in Fedora
- •Customizing runlevels in SuSE
- •Customizing runlevels in Mandrake
- •Customizing runlevels at the command line
- •Switching to a new runlevel
- •Disabling Unused Services
- •Removing Unneeded Services
- •Learning about modules
- •Installing a module with insmod
- •Taking care of dependencies automatically with modprobe and depmod
- •Loading a module for a slightly different kernel with insmod and modprobe
- •Removing modules with rmmod
- •Step 1: Making an Emergency Plan, or Boot Disk
- •Step 2: Finding the Source Code
- •Step 4: Customizing the Kernel
- •Step 5: Building the Kernel
- •Understanding the Principles of SELinux
- •Everything is an object
- •Identifying subjects in SELinux
- •Understanding the security context
- •Disabling or Disarming SELinux
- •Playing the Right Role
- •Exploring the Process-Related Entries in /proc
- •Surveying Your System from /proc
- •Popping the Cork: Speeding Up WINE with /proc
- •Reading and Understanding File Permissions
- •Controlling Permissions at the Command Line
- •Changing File Permissions from a Desktop
- •Encryption Made Easy with kgpg and the KDE Desktop
- •Creating keys with kgpg
- •Sharing your key with the world
- •Importing a public key from a public-key server
- •Encrypting and decrypting documents with drag-and-drop ease
- •Encrypting Documents with gpg at the Command Line
- •Sharing a secret file
- •Creating a key pair and receiving encrypted documents
- •Encrypting documents on your home system
- •Encrypting E-Mail for Added Security
- •Encrypting with Ximian Evolution
- •Setting up Mozilla e-mail for encryption
- •Sending and receiving encrypted messages with Mozilla mail
- •Using Cross-Platform Authentication with Linux and Windows
- •Prepping for cross-platform authentication
- •Setting up cross-platform authentication
- •Using PAM and Kerberos to Serve Up Authentication
- •Establishing synchronized system times
- •Testing your domain name server (DNS)
- •Setting up a Key Distribution Center
- •Setting up automatic ticket management with Kerberos and PAM
- •Adding users to the Key Distribution Center
- •Building Good Rules with PAM
- •Phase
- •Control level
- •Module pathname
- •Arguments
- •Dissecting a Configuration File
- •Skipping a Password with PAM
- •Feeling the Power
- •Gaining Superuser Privileges
- •Pretending to Be Other Users
- •Limiting Privileges with sudo
- •Installing sudo
- •Adding Up the Aliases
- •Adding Aliases to the sudo Configuration File
- •Defining the Alias
- •Creating a User_Alias
- •Creating a Runas_Alias
- •Simplifying group managment with a Host_Alias
- •Mounting and unmounting CDs without the superuser password
- •Managing access to dangerous commands with command aliases
- •Using SSH for Top-Speed Connections
- •Setting Up Public-Key Authentication to Secure SSH
- •Generating the key pair
- •Distributing your public key
- •Passing on your passphrase
- •Logging In with SSH and Key Authentication
- •Starting from the command line
- •Getting graphic
- •Creating Shortcuts to Your Favorite SSH Locations
- •Copying Files with scp
- •Secure (And Fast) Port Forwarding with SSH
- •Finding Your Firewall
- •Setting up a simple firewall in Mandrake Linux
- •Setting up a simple firewall in Fedora Linux
- •Setting up a simple firewall in SuSE Linux
- •Editing the Rules with Webmin
- •Starting a Webmin session
- •Reading the rules with Webmin
- •Changing the rules
- •Editing existing rules
- •Adding a new rule with Webmin
- •Sharing Desktops with VNC
- •Inviting Your Friends to Use Your Desktop
- •Serving Up a New Desktop with VNC Server
- •Using tsclient to View Remote Desktops from Linux
- •Using tsclient with a VNC server
- •Using tsclient with an RDP server
- •Creating New VNC Desktops on Demand
- •Switching display managers in SuSE Linux
- •Switching display managers in Mandrake Linux
- •Connecting gdm and VNC
- •Exploring Your Network with lsof
- •Running lsof
- •Interpreting the lsof output
- •Reading file types
- •Discovering Network Connections
- •Other Timesaving lsof Tricks
- •Packet Sniffing with the Ethereal Network Analyzer
- •Starting Ethereal
- •Capturing packets
- •Applying filters to screen packets
- •Peeking in packets
- •Color-coding packets coming from your network
- •Getting Up and Running with Nessus
- •Installing programs Nessus needs to run
- •Installing Nessus
- •Adding a user to Nessus
- •Generating a certificate
- •Starting the daemon and the interface
- •Reading the grim results
- •Keeping Your Plug-ins Up-to-Date
- •Chatting in the Fedora Chat Room
- •Looking for Answers in the SuSE Chat Room
- •Processing Processes with procps
- •Using ps to filter process status information
- •Viewing ps output the way you want to see it
- •Making parent-child relationships stand out in a ps listing
- •Climbing the family tree with pstree
- •Finding processes with pgrep
- •Killing Processes with pkill
- •Killing Processes with killall
- •Closing Windows with xkill
- •Managing Users and Groups with the Fedora/Mandrake User Manager
- •Adding new users
- •Modifying user accounts
- •Adding groups
- •Filtering users and groups
- •Managing Users and Groups with the SuSE User Administrator
- •Adding new users
- •Modifying user accounts
- •Adding groups
- •Filtering users and groups
- •Adding and deleting log files from the viewer
- •Setting up alerts and warnings
- •Viewing your log files from SuSE
- •Monitoring your log files from SuSE
- •Customizing Your Log Files
- •Keeping an Eye on Resources with KDE System Guard
- •Finding and killing runaway processes
- •Prioritizing processes to smooth a network bottleneck
- •Watching your system load
- •Creating a new worksheet
- •Creating system resource logs
- •Displaying network resources
- •Using Synaptic to download and install Apache
- •Installing Apache from disc
- •Starting the Apache Service
- •Building a Quick Web Page with OpenOffice.org
- •Taking Your Site Public with Dynamic DNS
- •Understanding how dynamic DNS works
- •Setting up dynamic DNS
- •Updating your IP address
- •Installing the Fedora HTTP Configuration tool
- •Putting the HTTP Configuration tool to work
- •Watching Your Web Server Traffic with apachetop
- •Installing apachetop
- •Running and exiting apachetop
- •Navigating apachetop
- •Switching among the log files (or watching several at once)
- •Changing the display time of apachetop statistics
- •Accessing MySQL Control Center features
- •Viewing, managing, and repairing a database with the Databases controls
- •Putting the Server Administration controls to work
- •Adding a new user
- •Watching Your MySQL Traffic with mtop
- •Gathering all the packages that mtop needs
- •Installing mtop
- •Monitoring traffic
- •Building a MySQL Server
- •Installing the necessary packages
- •Starting the MySQL server
- •Replicating MySQL Data
- •Configuring replication: The three topologies
- •Setting up replication for a single slave and master
- •Choosing a Method to Back Up MySQL Data
- •Backing Up and Restoring with mysqldump
- •mysqldump backup options
- •Backing up multiple databases
- •Compressing the archive
- •Restoring a mysqldump archive
- •Making a mysqlhotcopy of Your Database
- •Archiving a Replication Slave
- •Taking Care of Business with MySQL Administrator
- •Installing MySQL Administrator
- •Starting MySQL Administrator
- •Choosing an SSL Certificate
- •Creating a Certificate Signing Request
- •Creating a Signing Authority with openssl
- •Creating a certificate authority
- •Signing a CSR
- •Exploring Your Certificate Collection with Mozilla
- •Introducing hotway
- •Getting Started with hotway
- •Setting Up Evolution to Read HTTPMail Accounts with hotway
- •Ringing the Bells and Blowing the Whistles: Your Evolution Summary Page
- •Installing SpamAssassin
- •Installing from the distribution media
- •Installing from RPM downloads
- •Starting the service
- •Fine-Tuning SpamAssassin to Separate the Ham from the Spam
- •Customizing settings
- •Saving your settings
- •Adding a New Filter to Evolution
- •Serving Up a Big Bowl of the RulesDuJour
- •Registering Your Address
- •Taming a Sendmail Server
- •Tweaking Your Configuration Files with Webmin
- •Serving up mail for multiple domains
- •Relaying e-mail
- •Using aliases to simplify mail handling
- •Deciding What to Archive
- •Choosing Archive Media
- •Tape drives
- •Removable and external disk drives
- •Removable media
- •Optical media (CDs and DVDs)
- •Online storage
- •Choosing an Archive Scheme
- •Full backups
- •Differential backups
- •Incremental backups
- •Incremental versus differential backups
- •Choosing an Archive Program
- •Estimating Your Media Needs
- •Creating Data Archives with tar
- •Backing up files and directories
- •Backing up account information and passwords
- •Targeting bite-sized backups for speedier restores
- •Rolling whole file systems into a tarball
- •Starting an Incremental Backup Cycle
- •Restoring from Backup with tar
- •Backing Up to CD (Or DVD) with cdbackup
- •Creating the backup
- •Restoring from a CD or DVD backup
- •Restoring from a disc containing multiple archives
- •Combining the Power of tar with ssh for Quick Remote Backups
- •Testing the ssh connection to the remote host
- •Creating a tar archive over the ssh connection
- •Backing up to tape drives on remote machines
- •Backing Up to a Remote Computer with rdist and ssh
- •Testing the ssh connection to the remote host
- •Creating the distfile
- •Backing up
- •Getting Started with CVS
- •Checking whether CVS is installed
- •Discovering what to use CVS for
- •Creating a CVS Repository
- •Populating Your Repository with Files
- •Simplifying CVS with cervisia
- •Installing cervisia
- •Putting files in your sandbox
- •Adding more files to your repository
- •Committing your changes
- •Browsing your log files
- •Marking milestones with tags
- •Branching off with cervisia
- •Using the libcurl Library (C Programming)
- •Uploading a File with a Simple Program Using libcurl
- •Line 7: Defining functions and data types
- •Line 14: Calling the initialization function
- •Lines 18– 21: Defining the transfer
- •Line 23: Starting the transfer
- •Line 26: Finishing the upload
- •Installing the Ming Library
- •Building a Simple Flash Movie with Ming
- •Examining the program
- •Compiling the program
- •Running the program
- •Building Interactive Movies with Ming
- •Examining the program
- •Compiling the program
- •Running the program
- •Doing the curl E-shuffle with PHP
- •Combining PHP with curl and XML: An overview
- •Checking out the XML file
- •Downloading and displaying the XML file with a PHP script (and curl)
- •Sending E-Mail from PHP When Problems Occur
- •Debugging Perl Code with DDD
- •Installing and starting DDD
- •Examining the main window
- •Reviewing and stepping through source code
- •Making Stop Signs: Using Breakpoints to Watch Code
- •Setting a breakpoint
- •Modifying a breakpoint
- •Opening the data window
- •Adding a variable to the data window
- •Changing the display to a table
- •Using the Backtrace feature
- •Using the Help menu
- •Making Fedora Distribution CDs
- •Downloading the ISO images
- •Verifying the checksums
- •Burning an ISO File to Disc at the Command Line
- •Finding the identity of your drive
- •Running a test burn
- •Burning the distribution discs
- •Burning CDs without Making an ISO First
- •Finding setuid quickly and easily with kfind
- •Finding setuid and setgid programs at the command line
- •Deciding to Turn Off setuid or setgid
- •Changing the setuid or setgid Bit
- •Who Belongs in Jail?
- •Using UML to Jail Programs
- •Using lsof to Find Out Which Files Are Open
- •Debugging Your Environment with strace
- •Investigating Programs with ltrace
- •Handy strace and ltrace Options
- •Recording Program Errors with valgrind
- •Hardening Your Hat with Bastille
- •Downloading and installing Bastille and its dependencies
- •Welcome to the Bastille
- •Addressing file permission issues
- •Clamping down on SUID privileges
- •Moving on to account security
- •Making the boot process more secure
- •Securing connection broker
- •Limiting compiler access
- •Limiting access to hackers
- •Logging extra information
- •Keeping the daemons in check
- •Securing sendmail
- •Closing the gaps in Apache
- •Keeping temporary files safe
- •Building a better firewall
- •Port scanning with Bastille
- •Turning LIDS On and Off
- •Testing LIDS before Applying It to Your System
- •Controlling File Access with LIDS
- •Hiding Processes with LIDS
- •Running Down the Privilege List
- •Getting Graphical at the Command Line
- •Getting graphical in GNOME
- •Getting graphical with KDE
- •Staying desktop neutral
- •Index
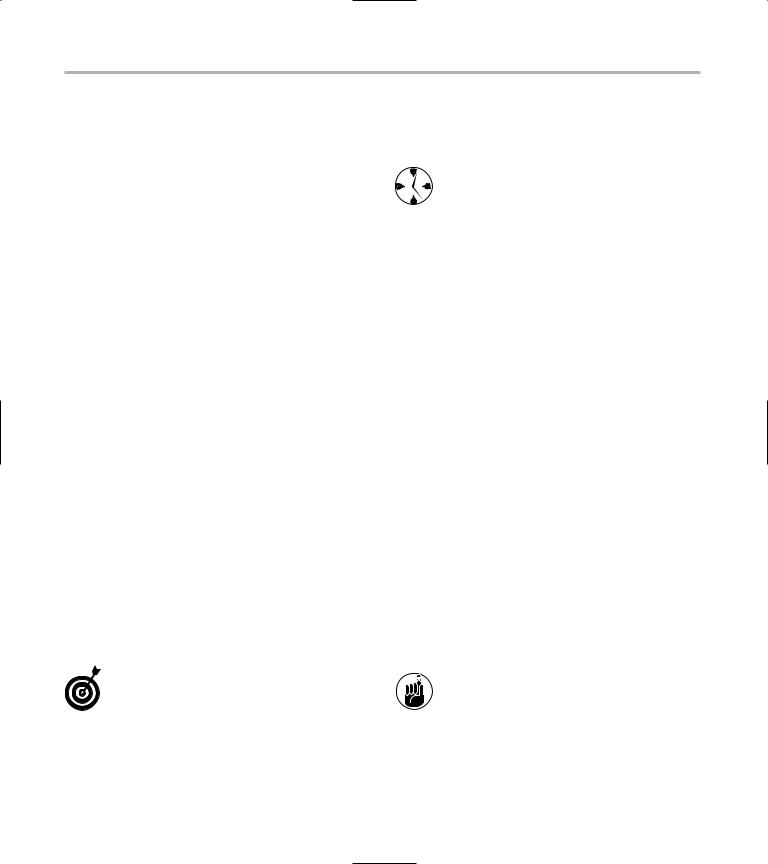
404 Technique 53: Using Open-Source APIs to Save Time
Using the libcurl Library (C Programming)
curl is a command line tool that can upload and download files from HTTP and FTP servers. Actually, that’s a gross oversimplification; curl knows how to deal with HTTP, FTP, secure HTTP and FTP servers, gopher servers, telnet servers, local files, and many more protocols.
Downloading a file with curl is easy. For example, to download a file named news.html from the example.com Web site (and save it to a file named mylocalfile), execute the following command:
$curl -o mylocalfile http://example.com/ news.html
curl is very similar to the more familiar wget, except that wget can only download data; curl can move data in both directions.
You can harness the power of curl in your own C programs by making calls to the libcurl API. The libcurl library comes in two flavors that you can mix and match. The easy API defines only six functions (and you can write useful, network-enabled programs using only four of those functions). The multi interface is more complex. When you call a function in the easy API, your program pauses until the function completes. The multi interface, on the other hand, performs transfers in the background, returning control to your program as soon as you initiate a transfer. In other words, the easy API is synchronous, and the multi interface is asynchronous.
You can find the curl (and libcurl) Web site at curl.haxx.se. You’ll find complete (and easy-to-follow) documentation about both the easy and the multi interface.
The Fedora, SuSE, and Mandrake distributions all include the curl command line program in an RPM package named curl. To write libcurl-enabled programs, install the curl package and the curl-devel package.
The multi API is built with functions defined by the easy API. That means that you can mix functions from both interfaces.
Everything that you know about the easy interface is applicable when you build a more sophisticated program based on the multi interface.
Uploading a File with a Simple Program Using libcurl
Every libcurl program starts with a call to an initialization function (typically curl_easy_init()) and ends with a call to a cleanup function (typically curl_easy_cleanup()). In between these calls, you use the curl_easy_setopt() and curl_easy_perform() functions to create and execute transfer jobs. Listing 53-1 shows a simple program (named curlupload) that uses libcurl’s easy API to upload a file.
When you run curlupload (we show you how to compile it in a moment), include two command line parameters:
The name of the file you want to upload
The upload destination, specified in the form of a URL
For example, to upload a file named photo.jpg to an FTP server, use this command:
$curlupload ./photo.jpg ftp://ftp. example.com/pub/upload/
When you use this example in your own code, be sure to add some error checking. Check the command line arguments and be sure the files are valid files. See the libcurl documentation to find out how errors are reported to your program.
The following sections take a closer look at some lines in the curlupload program.
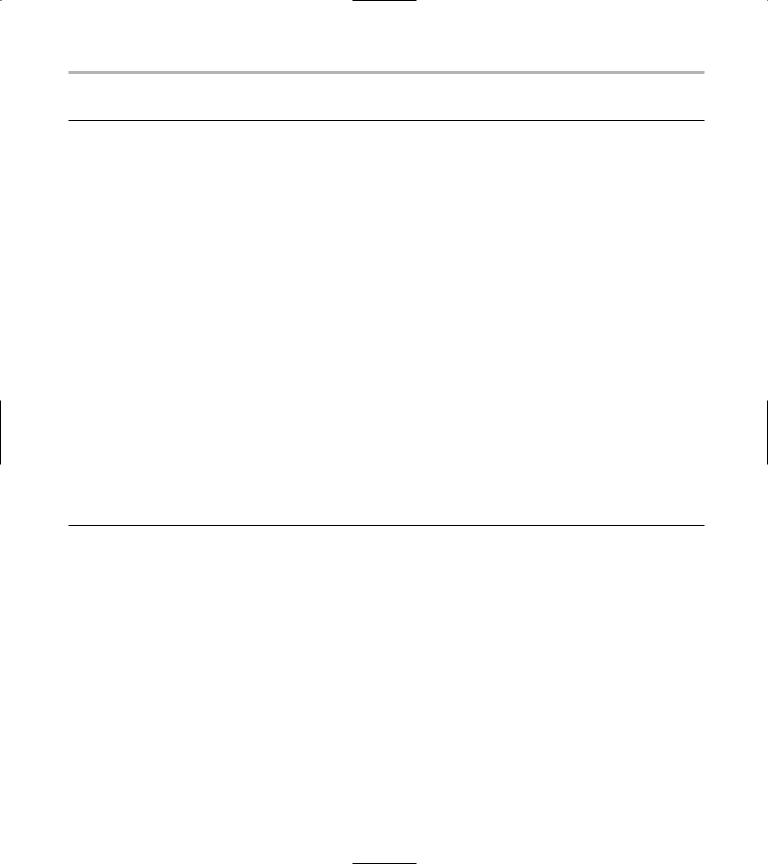
Uploading a File with a Simple Program Using libcurl |
405 |
LISTING 53-1: CURLUPLOAD.C
1 |
/* File: |
curlupload.c |
2 |
** |
usage: curlupload <filename> <URL> |
3 |
*/ |
|
4 |
|
|
5 |
#include |
<stdlib.h> |
6 |
#include |
<string.h> |
7 |
#include |
<curl/curl.h> |
8 |
|
|
9 |
int main( int argc, char * argv[] ) |
|
10 |
{ |
|
11 |
CURL |
* curl; |
12 |
FILE |
* srcFile = fopen( argv[1], “rb” ); |
13 |
|
|
14if(( curl = curl_easy_init()) != NULL )
15{
16char errorMsg[CURL_ERROR_SIZE];
17
18curl_easy_setopt( curl, CURLOPT_ERRORBUFFER, errorMsg );
19curl_easy_setopt( curl, CURLOPT_UPLOAD, TRUE );
20curl_easy_setopt( curl, CURLOPT_URL, argv[2] );
21curl_easy_setopt( curl, CURLOPT_READDATA, srcFile );
22
23if( curl_easy_perform( curl ) != 0 )
24fprintf( stderr, “%s\n”, errorMsg );
26curl_easy_cleanup( curl );
27 |
} |
28 |
|
29return( EXIT_SUCCESS );
30}
Line 7: Defining functions and data types
The libcurl C functions and data types are defined in the curl/curl.h header file, so libcurl-enabled programs should #include that file (see line 7).
Line 14: Calling the initialization function
Line 14 shows the call to the libcurl initialization function, curl_easy_init(). curl_easy_init(). This call returns a handle.
A handle is a small piece of data that a library uses to keep track of the state of something. In the case of libcurl, the handle holds the state of a transfer job. In libcurl programs, a handle takes the form of a CURL pointer (see line 11).
So what does a CURL pointer point to? Who knows? That’s the whole point of a handle — the API’s author puts anything that he needs into the structure behind the handle, but the author of a client application (that’s you) can’t see the data. The API creates the handle, and the client application just gives it back to the API whenever it’s needed.
Lines 18– 21: Defining the transfer
To create a transfer job, use curl_easy_setopt() to define the characteristics of the transfer. Each time you call curl_easy_setopt(), you pass three parameters:
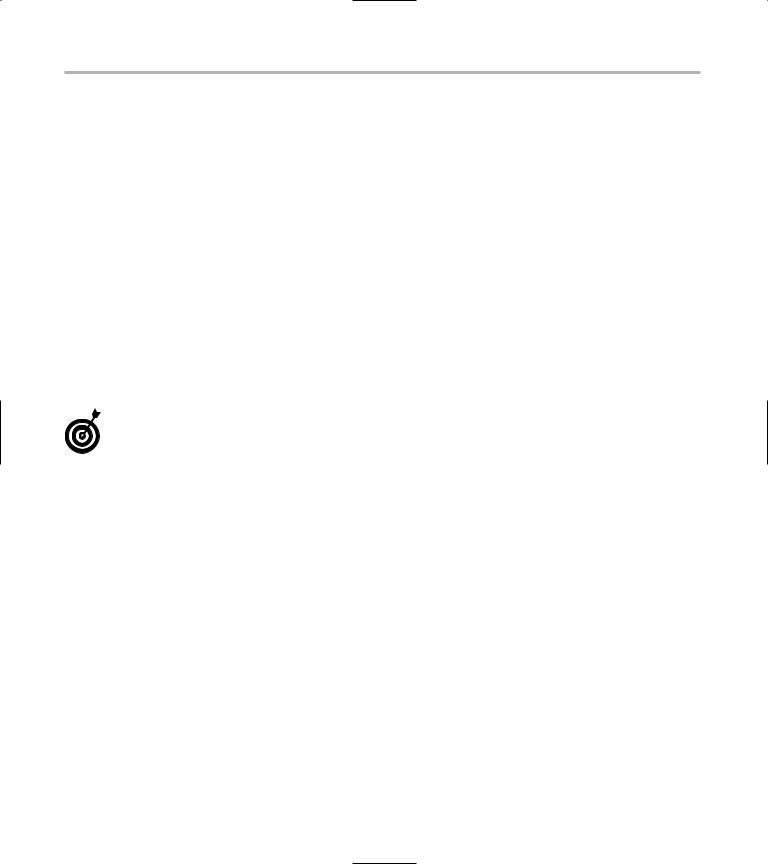
406 Technique 53: Using Open-Source APIs to Save Time
The CURL handle returned by curl_easy_init()
The symbolic name of the option you want to change
The new value for the option
To upload a file, you must set four transfer options, as shown on Lines 18–21.
Line 18: Routing libcurl error messages
Line 18 sets the CURLOPT_ERRORBUFFER option. This option tells libcurl where to store any error messages in case something whacks out. When you set the CURLOPT_ERRORBUFFER option, the third argument specifies the buffer where libcurl will store the error messages. The documentation tells you that the error message buffer should be at least CURL_ERROR_SIZE bytes long (see line 16).
Speaking of documentation, when you install the curl RPM package, the documentation for libcurl is installed as well. See info curl_easy_setopt (or man curl_easy_ setopt if you prefer) for a complete list of transfer options and values.
Line 19: Telling libcurl you want to upload a file
At line 19, you define the CURLOPT_UPLOAD option to let libcurl know that you want to upload a file (by default, libcurl assumes that you want to download data).
You may have noticed that the data type for the third argument to curl_easy_setopt() varies. In some cases, the third argument is a char *; in others, it’s a numeric value; and a few options expect a Boolean value. Unfortunately, you have to infer the proper data type from the online documentation.
Line 20: Telling libcurl where to send data
specified in the form of a URL, and curlupload simply passes along the second command line argument (argv[2]). CURLOPT_URL is arguably the most important and most powerful transfer option.
libcurl supports full URL syntax, so you can include a host name, user name, password, directory, and file name when you run curlupload. Here are a few examples:
ftp://example.com
A simple URL specifies a protocol (ftp) and a host name (example.com). libcurl can upload data by using http or ftp. You can’t upload to a URL this simple because you have to specify a target filename (but you can download from a simple URL).
ftp://freddie@example.com
If you don’t specify a user name, libcurl assumes that you want to log in to an FTP server as user anonymous. If you want to connect using a different user name, just insert the user name, followed by @ in front of the host name.
ftp://freddie:password@example.com
You can also include a password in a URL by inserting a colon (:) and the password following the user name.
ftp://example.com/pub/
A URL that ends with a / specifies a directory name. You can’t upload to a URL that ends with /, but you can download from such a URL.
ftp://example.com/pub/drinks.txt
Because this URL does not end in a /, libcurl assumes that drinks.txt is the name of the destination file.
Line 21: Telling libcurl where the data resides
To tell libcurl where you want the data sent, set |
The last option (line 21) tells libcurl where to find |
|
the data that you want to upload. You can give upload |
||
the CURLOPT_URL option (line 20). The destination is |
||
data to libcurl two ways: |
||
|