
- •Table of Contents
- •Introduction
- •Saving Time with This Book
- •Foolish Assumptions
- •Part I: Making the Desktop Work for You
- •Part II: Getting the Most from Your File System
- •Part III: Good Housekeeping with Linux
- •Part IV: Tweaking the Kernel on Your Linux System
- •Part V: Securing Your Workspace
- •Part VI: Networking Like a Professional
- •Part VII: Monitoring Your System
- •Part VIII: Serving Up the Internet and More
- •Part X: Programming Tricks
- •Part XI: The Scary (Or Fun!) Stuff
- •Icons Used in This Book
- •Discovering Your Protocols
- •Managing Snapshots with the camera: Protocol
- •Remote File Management with fish:
- •Getting Help with help:, info:, and man:
- •Other KDE Protocols
- •Using GNOME VFS Modules
- •Stacking VFS Modules
- •Working with Packages: rpm and rpms
- •Putting VFS to Work at the Command Line
- •Burning CDs with a VFS
- •Skinning Your Desktop with VFS
- •Classifying Data with MIME
- •Creating KDE File Associations
- •Creating New MIME Types with GNOME
- •Making Basic Prompt Transformations
- •Adding Dynamically Updated Data to Your Prompt
- •Colorizing Your Prompt
- •Seeing a Red Alert When You Have Superuser Privileges
- •Saving Your Work
- •Completing Names Automatically
- •Using the Escape Key to Your Advantage
- •Customizing Completion for Maximum Speed
- •Using cd and ls to Navigate through bash
- •Setting Your CDPATH Variables to Find Directories Fast
- •Streamlining Archive Searches
- •Turning the Output of a Command into a Variable with $( )
- •Using $UID and $EUID in Shell Scripts
- •Customizing Variables for Rapid Transit
- •Finding the Right Shell Script
- •Choosing your victims
- •Timing is everything
- •Cleaning up made easy
- •Changing prototype scripts
- •Customizing Your Autostart File
- •Navigating the History List
- •Scrolling
- •Summoning a command by number
- •Searching through history
- •Customizing the History List
- •Adjusting key default settings
- •Filtering the history list
- •Executing Commands Quickly with History Variables
- •Viewing Your Aliases
- •Using Aliases for Complex Commands
- •Automating Tedious Tasks with Functions
- •Filtering file searches by file type
- •Automatic downloading
- •Monitoring Your System in a Snap
- •Un-tarring the Easy Way
- •What Is Samba?
- •Getting Up and Running with Samba
- •Checking whether Samba is installed
- •Enabling Samba
- •Adjusting the workgroup name and creating user accounts
- •Giving a Windows machine access to your home directory
- •Sharing Linux files and directories with other computers
- •Hooking Everyone Up to the Printer
- •Sharing Linux printers with SWAT
- •Using a Windows printer from Linux
- •Plugging In to Remote Data with Linux Programs Quickly
- •Finding Files with locate
- •Finding Files with find
- •Qualifying Your Search with the find Command
- •Doing updated filename searches
- •Adding time-based qualifications
- •Filtering by file size
- •Perusing commonly used qualifications
- •Acting on What You Find
- •Displaying specific info with -printf
- •Checking disk usage by user
- •Executing commands with find
- •Building Complex Commands with xargs
- •Creating Archives with File Roller
- •Inspecting and Extracting Archives with File Roller
- •Adding Functionality to tar with Complex Commands
- •Building archives from the command line
- •Archiving complex search results
- •Backing up an installed package
- •Uprooting Entire Directory Trees with scp
- •Splitting Big Files into Manageable Chunks
- •Building Software from Downloaded tarballs
- •Compiling a tarball: The basic steps
- •Downloading and compiling SuperKaramba
- •Versatile Downloading with wget
- •Mirroring sites with wget
- •Verifying your bookmarks with wget
- •Downloading files with wget
- •Downloading and unpacking in one quick step
- •Downloading and Uploading with curl
- •Setting Up ADIOS
- •Downloading ADIOS
- •Burning ADIOS to CD
- •Installing ADIOS
- •Finding Your Way around UML
- •Connecting to the Internet from an ADIOS VM
- •Using a GUI with UML
- •Installing Software into UML
- •Merging Changes to Your Prototype
- •Querying RPM Packages for Content
- •Digesting Information
- •Creating a Package Index
- •Querying for Prerequisites
- •Dissecting an RPM Package
- •Using RPM at the Command Line
- •Removing RPMs
- •Flagging Down RPM
- •Getting Graphic with RPM
- •Using Rpmdrake to install from media
- •Installing from your Konqueror browser
- •Verifying Your System
- •Reading the Tamper-Proof Seal
- •Setting Up Synaptic and apt in a Snap
- •Keeping Up-to-Date with apt and Synaptic: The Basics
- •Handy Hints about Synaptic
- •Changing repositories
- •Viewing package details
- •Installing new packages with Synaptic
- •Importing the Keys to the Repository
- •Letting Task Scheduler Work for You
- •Scheduling a new task
- •Editing a task
- •Adding environment variables
- •Reining In Resources with Disk Quotas
- •Installing the quota RPM package
- •Enabling file system quotas
- •Getting your files together
- •Setting quotas
- •Reviewing your quotas
- •Using System Accounting to Keep Track of Users
- •Setting up system accounting
- •Looking up user login hours
- •Checking out command and program usage
- •Running Down the Runlevels
- •Runlevel basics
- •Customizing runlevels in Fedora
- •Customizing runlevels in SuSE
- •Customizing runlevels in Mandrake
- •Customizing runlevels at the command line
- •Switching to a new runlevel
- •Disabling Unused Services
- •Removing Unneeded Services
- •Learning about modules
- •Installing a module with insmod
- •Taking care of dependencies automatically with modprobe and depmod
- •Loading a module for a slightly different kernel with insmod and modprobe
- •Removing modules with rmmod
- •Step 1: Making an Emergency Plan, or Boot Disk
- •Step 2: Finding the Source Code
- •Step 4: Customizing the Kernel
- •Step 5: Building the Kernel
- •Understanding the Principles of SELinux
- •Everything is an object
- •Identifying subjects in SELinux
- •Understanding the security context
- •Disabling or Disarming SELinux
- •Playing the Right Role
- •Exploring the Process-Related Entries in /proc
- •Surveying Your System from /proc
- •Popping the Cork: Speeding Up WINE with /proc
- •Reading and Understanding File Permissions
- •Controlling Permissions at the Command Line
- •Changing File Permissions from a Desktop
- •Encryption Made Easy with kgpg and the KDE Desktop
- •Creating keys with kgpg
- •Sharing your key with the world
- •Importing a public key from a public-key server
- •Encrypting and decrypting documents with drag-and-drop ease
- •Encrypting Documents with gpg at the Command Line
- •Sharing a secret file
- •Creating a key pair and receiving encrypted documents
- •Encrypting documents on your home system
- •Encrypting E-Mail for Added Security
- •Encrypting with Ximian Evolution
- •Setting up Mozilla e-mail for encryption
- •Sending and receiving encrypted messages with Mozilla mail
- •Using Cross-Platform Authentication with Linux and Windows
- •Prepping for cross-platform authentication
- •Setting up cross-platform authentication
- •Using PAM and Kerberos to Serve Up Authentication
- •Establishing synchronized system times
- •Testing your domain name server (DNS)
- •Setting up a Key Distribution Center
- •Setting up automatic ticket management with Kerberos and PAM
- •Adding users to the Key Distribution Center
- •Building Good Rules with PAM
- •Phase
- •Control level
- •Module pathname
- •Arguments
- •Dissecting a Configuration File
- •Skipping a Password with PAM
- •Feeling the Power
- •Gaining Superuser Privileges
- •Pretending to Be Other Users
- •Limiting Privileges with sudo
- •Installing sudo
- •Adding Up the Aliases
- •Adding Aliases to the sudo Configuration File
- •Defining the Alias
- •Creating a User_Alias
- •Creating a Runas_Alias
- •Simplifying group managment with a Host_Alias
- •Mounting and unmounting CDs without the superuser password
- •Managing access to dangerous commands with command aliases
- •Using SSH for Top-Speed Connections
- •Setting Up Public-Key Authentication to Secure SSH
- •Generating the key pair
- •Distributing your public key
- •Passing on your passphrase
- •Logging In with SSH and Key Authentication
- •Starting from the command line
- •Getting graphic
- •Creating Shortcuts to Your Favorite SSH Locations
- •Copying Files with scp
- •Secure (And Fast) Port Forwarding with SSH
- •Finding Your Firewall
- •Setting up a simple firewall in Mandrake Linux
- •Setting up a simple firewall in Fedora Linux
- •Setting up a simple firewall in SuSE Linux
- •Editing the Rules with Webmin
- •Starting a Webmin session
- •Reading the rules with Webmin
- •Changing the rules
- •Editing existing rules
- •Adding a new rule with Webmin
- •Sharing Desktops with VNC
- •Inviting Your Friends to Use Your Desktop
- •Serving Up a New Desktop with VNC Server
- •Using tsclient to View Remote Desktops from Linux
- •Using tsclient with a VNC server
- •Using tsclient with an RDP server
- •Creating New VNC Desktops on Demand
- •Switching display managers in SuSE Linux
- •Switching display managers in Mandrake Linux
- •Connecting gdm and VNC
- •Exploring Your Network with lsof
- •Running lsof
- •Interpreting the lsof output
- •Reading file types
- •Discovering Network Connections
- •Other Timesaving lsof Tricks
- •Packet Sniffing with the Ethereal Network Analyzer
- •Starting Ethereal
- •Capturing packets
- •Applying filters to screen packets
- •Peeking in packets
- •Color-coding packets coming from your network
- •Getting Up and Running with Nessus
- •Installing programs Nessus needs to run
- •Installing Nessus
- •Adding a user to Nessus
- •Generating a certificate
- •Starting the daemon and the interface
- •Reading the grim results
- •Keeping Your Plug-ins Up-to-Date
- •Chatting in the Fedora Chat Room
- •Looking for Answers in the SuSE Chat Room
- •Processing Processes with procps
- •Using ps to filter process status information
- •Viewing ps output the way you want to see it
- •Making parent-child relationships stand out in a ps listing
- •Climbing the family tree with pstree
- •Finding processes with pgrep
- •Killing Processes with pkill
- •Killing Processes with killall
- •Closing Windows with xkill
- •Managing Users and Groups with the Fedora/Mandrake User Manager
- •Adding new users
- •Modifying user accounts
- •Adding groups
- •Filtering users and groups
- •Managing Users and Groups with the SuSE User Administrator
- •Adding new users
- •Modifying user accounts
- •Adding groups
- •Filtering users and groups
- •Adding and deleting log files from the viewer
- •Setting up alerts and warnings
- •Viewing your log files from SuSE
- •Monitoring your log files from SuSE
- •Customizing Your Log Files
- •Keeping an Eye on Resources with KDE System Guard
- •Finding and killing runaway processes
- •Prioritizing processes to smooth a network bottleneck
- •Watching your system load
- •Creating a new worksheet
- •Creating system resource logs
- •Displaying network resources
- •Using Synaptic to download and install Apache
- •Installing Apache from disc
- •Starting the Apache Service
- •Building a Quick Web Page with OpenOffice.org
- •Taking Your Site Public with Dynamic DNS
- •Understanding how dynamic DNS works
- •Setting up dynamic DNS
- •Updating your IP address
- •Installing the Fedora HTTP Configuration tool
- •Putting the HTTP Configuration tool to work
- •Watching Your Web Server Traffic with apachetop
- •Installing apachetop
- •Running and exiting apachetop
- •Navigating apachetop
- •Switching among the log files (or watching several at once)
- •Changing the display time of apachetop statistics
- •Accessing MySQL Control Center features
- •Viewing, managing, and repairing a database with the Databases controls
- •Putting the Server Administration controls to work
- •Adding a new user
- •Watching Your MySQL Traffic with mtop
- •Gathering all the packages that mtop needs
- •Installing mtop
- •Monitoring traffic
- •Building a MySQL Server
- •Installing the necessary packages
- •Starting the MySQL server
- •Replicating MySQL Data
- •Configuring replication: The three topologies
- •Setting up replication for a single slave and master
- •Choosing a Method to Back Up MySQL Data
- •Backing Up and Restoring with mysqldump
- •mysqldump backup options
- •Backing up multiple databases
- •Compressing the archive
- •Restoring a mysqldump archive
- •Making a mysqlhotcopy of Your Database
- •Archiving a Replication Slave
- •Taking Care of Business with MySQL Administrator
- •Installing MySQL Administrator
- •Starting MySQL Administrator
- •Choosing an SSL Certificate
- •Creating a Certificate Signing Request
- •Creating a Signing Authority with openssl
- •Creating a certificate authority
- •Signing a CSR
- •Exploring Your Certificate Collection with Mozilla
- •Introducing hotway
- •Getting Started with hotway
- •Setting Up Evolution to Read HTTPMail Accounts with hotway
- •Ringing the Bells and Blowing the Whistles: Your Evolution Summary Page
- •Installing SpamAssassin
- •Installing from the distribution media
- •Installing from RPM downloads
- •Starting the service
- •Fine-Tuning SpamAssassin to Separate the Ham from the Spam
- •Customizing settings
- •Saving your settings
- •Adding a New Filter to Evolution
- •Serving Up a Big Bowl of the RulesDuJour
- •Registering Your Address
- •Taming a Sendmail Server
- •Tweaking Your Configuration Files with Webmin
- •Serving up mail for multiple domains
- •Relaying e-mail
- •Using aliases to simplify mail handling
- •Deciding What to Archive
- •Choosing Archive Media
- •Tape drives
- •Removable and external disk drives
- •Removable media
- •Optical media (CDs and DVDs)
- •Online storage
- •Choosing an Archive Scheme
- •Full backups
- •Differential backups
- •Incremental backups
- •Incremental versus differential backups
- •Choosing an Archive Program
- •Estimating Your Media Needs
- •Creating Data Archives with tar
- •Backing up files and directories
- •Backing up account information and passwords
- •Targeting bite-sized backups for speedier restores
- •Rolling whole file systems into a tarball
- •Starting an Incremental Backup Cycle
- •Restoring from Backup with tar
- •Backing Up to CD (Or DVD) with cdbackup
- •Creating the backup
- •Restoring from a CD or DVD backup
- •Restoring from a disc containing multiple archives
- •Combining the Power of tar with ssh for Quick Remote Backups
- •Testing the ssh connection to the remote host
- •Creating a tar archive over the ssh connection
- •Backing up to tape drives on remote machines
- •Backing Up to a Remote Computer with rdist and ssh
- •Testing the ssh connection to the remote host
- •Creating the distfile
- •Backing up
- •Getting Started with CVS
- •Checking whether CVS is installed
- •Discovering what to use CVS for
- •Creating a CVS Repository
- •Populating Your Repository with Files
- •Simplifying CVS with cervisia
- •Installing cervisia
- •Putting files in your sandbox
- •Adding more files to your repository
- •Committing your changes
- •Browsing your log files
- •Marking milestones with tags
- •Branching off with cervisia
- •Using the libcurl Library (C Programming)
- •Uploading a File with a Simple Program Using libcurl
- •Line 7: Defining functions and data types
- •Line 14: Calling the initialization function
- •Lines 18– 21: Defining the transfer
- •Line 23: Starting the transfer
- •Line 26: Finishing the upload
- •Installing the Ming Library
- •Building a Simple Flash Movie with Ming
- •Examining the program
- •Compiling the program
- •Running the program
- •Building Interactive Movies with Ming
- •Examining the program
- •Compiling the program
- •Running the program
- •Doing the curl E-shuffle with PHP
- •Combining PHP with curl and XML: An overview
- •Checking out the XML file
- •Downloading and displaying the XML file with a PHP script (and curl)
- •Sending E-Mail from PHP When Problems Occur
- •Debugging Perl Code with DDD
- •Installing and starting DDD
- •Examining the main window
- •Reviewing and stepping through source code
- •Making Stop Signs: Using Breakpoints to Watch Code
- •Setting a breakpoint
- •Modifying a breakpoint
- •Opening the data window
- •Adding a variable to the data window
- •Changing the display to a table
- •Using the Backtrace feature
- •Using the Help menu
- •Making Fedora Distribution CDs
- •Downloading the ISO images
- •Verifying the checksums
- •Burning an ISO File to Disc at the Command Line
- •Finding the identity of your drive
- •Running a test burn
- •Burning the distribution discs
- •Burning CDs without Making an ISO First
- •Finding setuid quickly and easily with kfind
- •Finding setuid and setgid programs at the command line
- •Deciding to Turn Off setuid or setgid
- •Changing the setuid or setgid Bit
- •Who Belongs in Jail?
- •Using UML to Jail Programs
- •Using lsof to Find Out Which Files Are Open
- •Debugging Your Environment with strace
- •Investigating Programs with ltrace
- •Handy strace and ltrace Options
- •Recording Program Errors with valgrind
- •Hardening Your Hat with Bastille
- •Downloading and installing Bastille and its dependencies
- •Welcome to the Bastille
- •Addressing file permission issues
- •Clamping down on SUID privileges
- •Moving on to account security
- •Making the boot process more secure
- •Securing connection broker
- •Limiting compiler access
- •Limiting access to hackers
- •Logging extra information
- •Keeping the daemons in check
- •Securing sendmail
- •Closing the gaps in Apache
- •Keeping temporary files safe
- •Building a better firewall
- •Port scanning with Bastille
- •Turning LIDS On and Off
- •Testing LIDS before Applying It to Your System
- •Controlling File Access with LIDS
- •Hiding Processes with LIDS
- •Running Down the Privilege List
- •Getting Graphical at the Command Line
- •Getting graphical in GNOME
- •Getting graphical with KDE
- •Staying desktop neutral
- •Index

Doing the curl E-shuffle with PHP 415
Doing the curl E-shuffle with PHP
If you read Technique 53, you already know quite a bit about curl. curl is a command line tool that makes it quick and easy to upload and download data from other computers. curl can interact with
Web or FTP servers, secure servers, and even directory servers. The curl project developers have created an easy-to-use library, named libcurl, that you can use to build your own curl-enabled programs. libcurl programs can be written in a number of languages. In the previous technique, you find out how to use libcurl from a C program; in this technique, we show you how to use libcurl from a PHP script.
Combining PHP with curl and XML: An overview
PHP scripts usually run within a Web server (not within a Web browser). It might seem a bit strange for a Web server to connect to another server to retrieve information, but that’s actually a very useful thing to do. You may want to relay information (like news headlines) from a remote server to the Web browser. Or, you may want to translate information
LISTING 54-1: WEATHER REPORT FOR MICROSOFT
you obtain from another server into a more friendly and useful format. Using libcurl from a PHP script makes it easy to retrieve remote information.
Thousands of Web sites publish information in XML format (eXtensible Markup Language). We mention earlier that XML data is somewhat self-identifying; each piece of data is surrounded by a pair of tags that describe the data contained within. For example, suppose you see the following text inside an XML stream:
<Forecast>Sunny</Forecast>
You could reasonably assume that Sunny is a forecast (probably part of a weather forecast). XML was designed to make it easier for programs to make sense of a piece of data. PHP knows how to parse through an XML data stream and pick out the pieces that you’re interested in.
In this section, we show you how to use PHP, curl, and XML to display weather conditions on your Web site.
Checking out the XML file
The Web site www.ejse.com publishes XML weather conditions for your zip code. A typical weather report from EJSE might look like Listing 54-1.
<WeatherInfo>
<Location>Redmond, WA</Location> <IconIndex>26</IconIndex> <Temprature>47°F</Temprature> <FeelsLike>44°F</FeelsLike> <Forecast>Cloudy</Forecast> <Visibility>Unlimited </Visibility> <Pressure>29.43 inches and rising</Pressure> <DewPoint>40°F</DewPoint>
<UVIndex>0 Minimal</UVIndex> <Humidity>77%</Humidity>
<Wind>From the Northwest at 6 mph</Wind> <ReportedAt>Renton, WA</ReportedAt> <LastUpdated>
Thursday, February 26, 2004, at 9:53 AM Pacific Standard Time (Thursday, 12:53 PM EST). </LastUpdated>
</WeatherInfo>
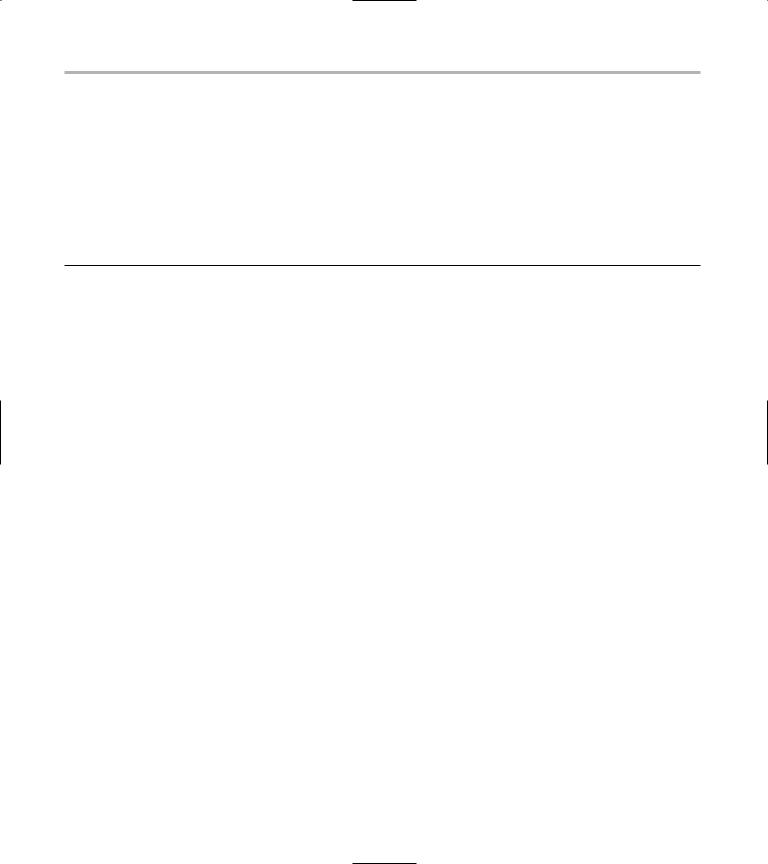
416 Technique 54: Timesaving PHP Tricks
(That’s not a typo; the XML stream misspells temperature.)
Notice that each piece of data is surrounded by a pair of tags that describes the data within. For example, the forecast (Cloudy) is surrounded by the tags
<Forecast> and </Forecast> — that’s the essence of XML.
Downloading and displaying the XML file with a PHP script (and curl)
Listing 54-2 shows a reasonably short PHP script that downloads the current weather conditions for a given zip code (currently hard-coded to 98052) and parses through the XML.
LISTING 54-2: FINDING WEATHER CONDITIONS WITH PHP
1 <?php
2
3 $weather = array();
4
5$xmlData = loadWeather();
6 parseWeather( $weather, $xmlData );
7 displayWeather( $weather );
8
9function loadWeather()
10{
11$url = “http://www.ejse.com/WeatherService/Service.asmx/GetWeatherInfo?zipCode=98052”;
12$curl = curl_init();
13
14curl_setopt( $curl, CURLOPT_URL, $url );
15curl_setopt( $curl, CURLOPT_RETURNTRANSFER, 1 );
17$xmlData = curl_exec( $curl );
19curl_close( $curl );
21return( $xmlData );
22}
24function parseWeather( $weather, $data )
25{
26$parser = xml_parser_create();
28xml_set_element_handler( $parser, “startTag”, “endTag” );
29xml_set_character_data_handler( $parser, “dataHandler” );
31xml_parse( $parser, $data );
33xml_parser_free( $parser );
34}
36function startTag( $parser, $tagName, $tagAttributes )
37{
38global $tag;
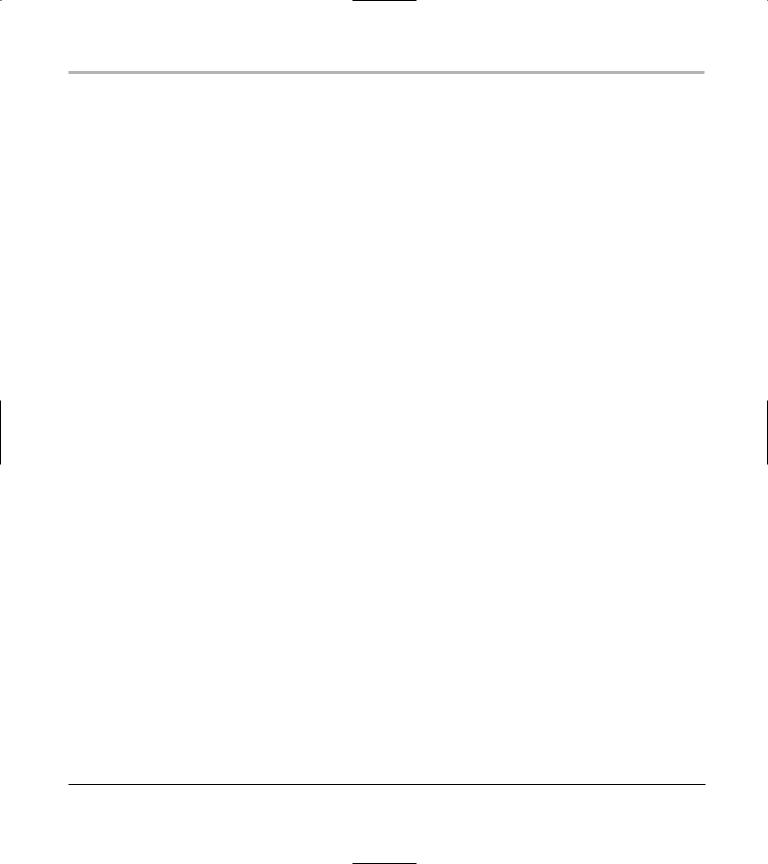
Doing the curl E-shuffle with PHP 417
40$tag = $tagName;
41}
42
43function endTag( $parser, $tagName )
44{
45global $tag;
46
47$tag = “”;
48}
49
50function dataHandler( $parser, $data )
51{
52global $weather, $tag;
53
54$weather[ $tag ] = $data;
55}
56
57function displayWeather( $weather )
58{
59echo( “<html>\n” );
60 |
echo( |
“<head>\n” ); |
|
|
|
61 |
echo( |
“ <title>” ); |
|
|
|
62 |
echo( |
“Current Conditions At “ . $weather[“LOCATION”] . “</title>\n” ); |
|||
63 |
echo( |
“</head>\n” ); |
|
|
|
64 |
|
|
|
|
|
65 |
echo( |
“<body>\n” ); |
|
|
|
66 |
echo( |
“<h4>\n” ); |
|
|
|
67 |
echo( |
“Current Weather for: “ . $weather[“LOCATION”] . “<br>” ); |
|||
68 |
echo( |
“</h4>\n” ); |
|
|
|
69 |
|
|
|
|
|
70 |
echo( |
‘<TABLE CELLPADDING=”2” CELLSPACING=”0” BORDER=1>’ ); |
|
||
71 |
echo( |
“<TR><TD>Forecast</TD> <TD>” |
. $weather[“FORECAST”] |
. “</TD></TR>” |
|
|
); |
|
|
|
|
72 |
echo( |
“<TR><TD>Temperature</TD> |
<TD>” . $weather[“TEMPRATURE”] |
. “</TD></TR>” |
|
|
); |
|
|
|
|
73 |
echo( |
“<TR><TD>Wind</TD> |
<TD>” . $weather[“WIND”] |
. “</TD></TR>” |
|
|
); |
|
|
|
|
74 |
echo( |
“<TR><TD>Wind Chill</TD> |
<TD>” . $weather[“FEELSLIKE”] |
. “</TD></TR>” |
|
|
); |
|
|
|
|
75 |
echo( |
“<TR><TD>Dewpoint</TD> |
<TD>” . $weather[“DEWPOINT”] |
. “</TD></TR>” |
|
|
); |
|
|
|
|
76 |
echo( |
“<TR><TD>Pressure</TD> |
<TD>” . $weather[“PRESSURE”] |
. “</TD></TR>” |
|
|
); |
|
|
|
|
77 |
echo( |
“<TR><TD>UV Index</TD> |
<TD>” . $weather[“UVINDEX”] |
. “</TD></TR>” |
|
|
); |
|
|
|
|
78 |
echo( |
“</TABLE>” ); |
|
|
|
79 |
echo( |
“</body>\n” ); |
|
|
|
80echo( “</html>\n” );
81}
82
83 ?>
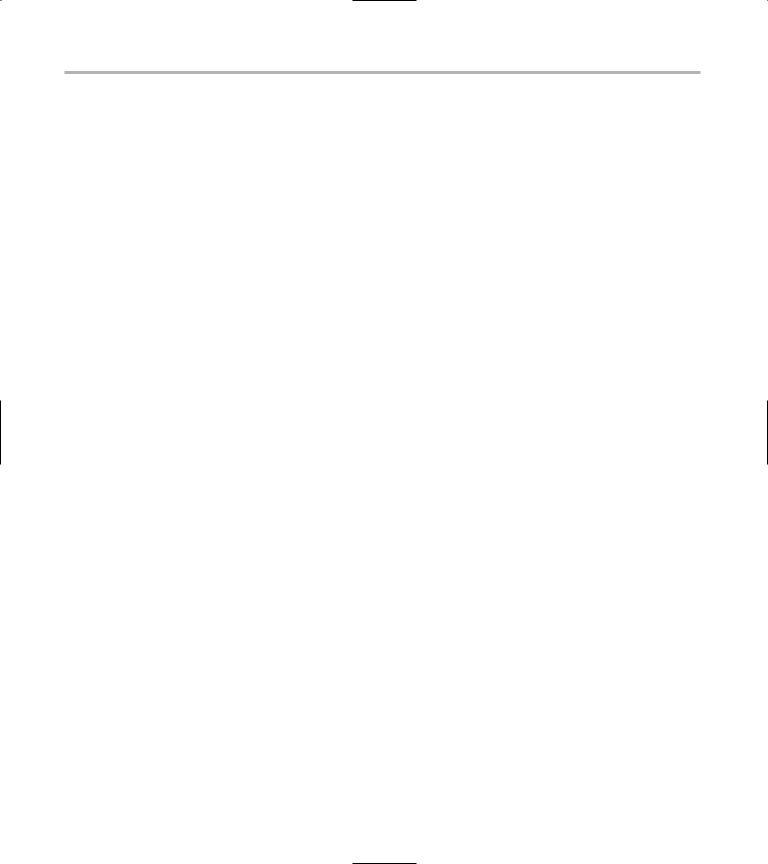
418 Technique 54: Timesaving PHP Tricks
This script is very simple:
Line 5: The script calls loadWeather() to retrieve the current conditions and store them in $xmlData.
Line 6: parseWeather() parses the XML stream and stores the result in $weather.
Line 7: displayWeather() picks apart the $weather array and creates an HTML table that’s sent to the Web browser.
Line 9: The loadWeather() function (starting at line 9) downloads an XML stream from the URL shown on line 11. (Change the zip code at the end of the URL to match the location that you’re interested in.)
Line 12: Before you can start a transfer using libcurl, you initialize the library and save a copy of the handle returned by curl_init().
Lines 14 and 15: Next, you see two calls to curl_setopt(). The first call (line 14) gives the URL to libcurl, and the second call (line 15) tells libcurl that you want to download data and store it in a variable (as opposed to storing the data in a file).
Line 17: curl_exec() starts the download, waits for it to complete, and stores the result in $xmlData. When curl_exec() finishes, $xmlData contains the current weather conditions, in XML form, as shown in Listing 54-1.
Line 19–21: Finally, loadWeather() closes its connection to libcurl (see line 19) and returns the XML data to the caller (line 21).
Line 24: parseWeather() (lines 24–34) expects two parameters: the destination array ($weather) and the raw XML data ($data). parseWeather() doesn’t do any of the hard work itself; instead, it calls on PHP’s XML library to pick apart the XML data.
Line 26: You see a call to the xml_parser_create() function. xml_parser_create() creates a new XML parser and returns a handle. An XML parser doesn’t do much by itself; it just identifies the various pieces of an XML stream. To make use of an XML parser, you have to provide callback functions. When an XML parser recognizes a chunk of data that you’re interested in, it calls the callback function that you provide.
Line 28: The call to xml_set_element_handler() tells the parser that you’re interested in elements (or, as we call them, tags). When the parser finds the beginning of an element, it calls the startTag function. When the parser finds the end of an element, it calls endTag (you see startTag and endTag in a moment).
Here’s a typical XML element:
<Location>Redmond, WA</Location>
When the parser sees <Location>, it calls startTag(); when it sees </Location>, it calls endTag(). The stuff in between the tags is called character data — we can trap that, too (see the call to xml_set_character_ data_handler() at line 29).
Line 31: parseWeather() starts the XML parser with a call to xml_parse(), passing the raw XML data that you want to parse.
Line 32: When xml_parse() completes, the call to xml_parser_free() shuts down the parser and frees up any lingering resources.
Lines 36–42: startTag() and endTag() are simple. When the parser calls startTag(), it sends along the tag name, which you store in a global variable named $tag. The tagAttributes parameter contains XML element attributes (if there are any); the weather information that you’re looking at won’t contain attributes, so you can safely ignore this argument.
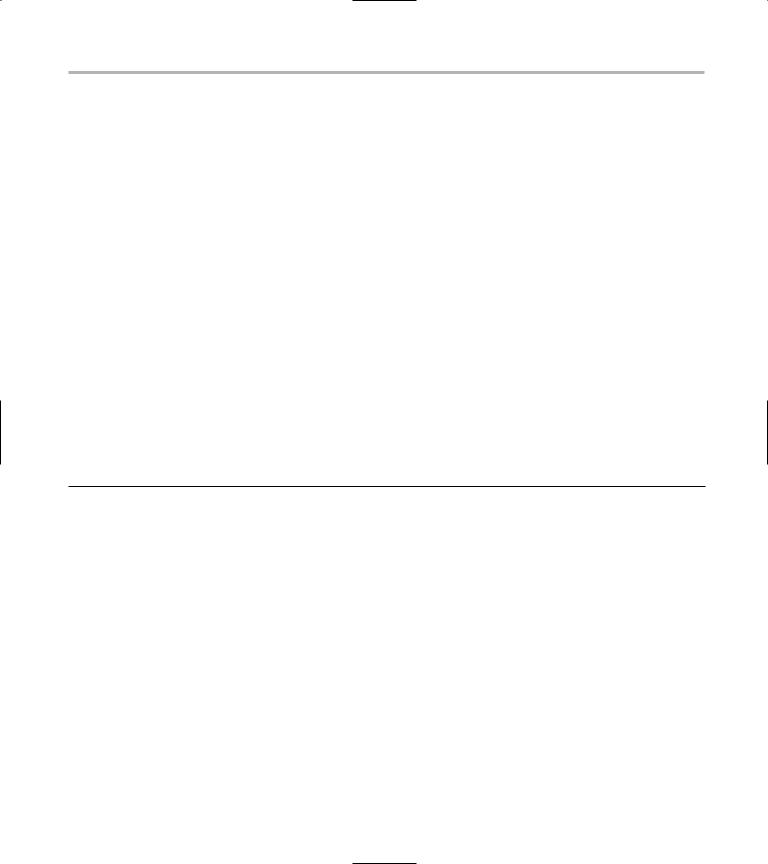
Doing the curl E-shuffle with PHP 419
Lines 43–48: The endTag() function (lines 43–48) is called whenever the parser sees an end tag. In this function, you simply set the global variable $tag to an empty string.
Lines 50–56: In between calls to startTag() and endTag(), the XML parser calls dataHandler() to handle the data within the tags. dataHandler() simply stores the data that it’s given (a string contained between a pair of tags) in the $weather associative array.
The process is clear when you follow a typical element through the parser. Say that the parser runs into the following text:
<Location>Redmond, WA</Location>
When the parser sees the start tag, it calls startTag(). startTag() stores the tag name (Location) in the $tag global variable. (Actually, the parser converts all tag names to uppercase, so $tag is set to LOCATION.) Next, the parser calls
dataHandler() with the chunk of text (Redmond, WA) between the start tag and the end tag. dataHandler() stores the data in an element of the $weather array using the tag name as in index into the array. In other words: $weather[“LOCATION”] = “Redmond, WA”. Finally, endTag() wipes out the global $tag name (that’s not strictly necessary, but it makes your code a bit more obvious).
When the parser finishes its work, $weather looks like this:
$weather[“LOCATION”] |
= “Redmond, WA” |
$weather[“ICONINDEX”] |
= “26” |
$weather[“TEMPRATURE”] |
= “47°F” |
$weather[“FEELSLIKE”] |
= “44°F” |
$weather[“FORECAST”] |
= “Cloudy” |
$weather[“VISIBILITY”] |
= “Unlimited” |
... |
... |
Lines 57–81: The displayWeather() function builds an HTML page that it sends to the Web browser. The result looks similar to Listing 54-3.
LISTING 54-3: WEATHER CONDITIONS IN HTML
<html>
<head>
<title>Current Conditions At Redmond, WA</title> </head>
<body>
<h4>Current Weather for: Redmond, WA<br></h4>
<TABLE CELLPADDING=”2” CELLSPACING=”0” BORDER=1>
<TR><TD>Forecast</TD> |
<TD>Cloudy</TD></TR> |
<TR><TD>Temperature</TD> |
<TD>48°F</TD></TR> |
<TR><TD>Wind</TD> |
<TD>From the South at 6 mph</TD></TR> |
<TR><TD>Wind Chill</TD> |
<TD>45°F</TD></TR> |
<TR><TD>Dewpoint</TD> |
<TD>42°F</TD></TR> |
<TR><TD>Pressure</TD> |
<TD>30.22 inches and rising</TD></TR> |
<TR><TD>UV Index</TD> |
<TD>0 Minimal</TD></TR> |
</TABLE> |
|
</body> |
|
</html> |
|
|
|