
- •Table of Contents
- •Introduction
- •Saving Time with This Book
- •Foolish Assumptions
- •Part I: Making the Desktop Work for You
- •Part II: Getting the Most from Your File System
- •Part III: Good Housekeeping with Linux
- •Part IV: Tweaking the Kernel on Your Linux System
- •Part V: Securing Your Workspace
- •Part VI: Networking Like a Professional
- •Part VII: Monitoring Your System
- •Part VIII: Serving Up the Internet and More
- •Part X: Programming Tricks
- •Part XI: The Scary (Or Fun!) Stuff
- •Icons Used in This Book
- •Discovering Your Protocols
- •Managing Snapshots with the camera: Protocol
- •Remote File Management with fish:
- •Getting Help with help:, info:, and man:
- •Other KDE Protocols
- •Using GNOME VFS Modules
- •Stacking VFS Modules
- •Working with Packages: rpm and rpms
- •Putting VFS to Work at the Command Line
- •Burning CDs with a VFS
- •Skinning Your Desktop with VFS
- •Classifying Data with MIME
- •Creating KDE File Associations
- •Creating New MIME Types with GNOME
- •Making Basic Prompt Transformations
- •Adding Dynamically Updated Data to Your Prompt
- •Colorizing Your Prompt
- •Seeing a Red Alert When You Have Superuser Privileges
- •Saving Your Work
- •Completing Names Automatically
- •Using the Escape Key to Your Advantage
- •Customizing Completion for Maximum Speed
- •Using cd and ls to Navigate through bash
- •Setting Your CDPATH Variables to Find Directories Fast
- •Streamlining Archive Searches
- •Turning the Output of a Command into a Variable with $( )
- •Using $UID and $EUID in Shell Scripts
- •Customizing Variables for Rapid Transit
- •Finding the Right Shell Script
- •Choosing your victims
- •Timing is everything
- •Cleaning up made easy
- •Changing prototype scripts
- •Customizing Your Autostart File
- •Navigating the History List
- •Scrolling
- •Summoning a command by number
- •Searching through history
- •Customizing the History List
- •Adjusting key default settings
- •Filtering the history list
- •Executing Commands Quickly with History Variables
- •Viewing Your Aliases
- •Using Aliases for Complex Commands
- •Automating Tedious Tasks with Functions
- •Filtering file searches by file type
- •Automatic downloading
- •Monitoring Your System in a Snap
- •Un-tarring the Easy Way
- •What Is Samba?
- •Getting Up and Running with Samba
- •Checking whether Samba is installed
- •Enabling Samba
- •Adjusting the workgroup name and creating user accounts
- •Giving a Windows machine access to your home directory
- •Sharing Linux files and directories with other computers
- •Hooking Everyone Up to the Printer
- •Sharing Linux printers with SWAT
- •Using a Windows printer from Linux
- •Plugging In to Remote Data with Linux Programs Quickly
- •Finding Files with locate
- •Finding Files with find
- •Qualifying Your Search with the find Command
- •Doing updated filename searches
- •Adding time-based qualifications
- •Filtering by file size
- •Perusing commonly used qualifications
- •Acting on What You Find
- •Displaying specific info with -printf
- •Checking disk usage by user
- •Executing commands with find
- •Building Complex Commands with xargs
- •Creating Archives with File Roller
- •Inspecting and Extracting Archives with File Roller
- •Adding Functionality to tar with Complex Commands
- •Building archives from the command line
- •Archiving complex search results
- •Backing up an installed package
- •Uprooting Entire Directory Trees with scp
- •Splitting Big Files into Manageable Chunks
- •Building Software from Downloaded tarballs
- •Compiling a tarball: The basic steps
- •Downloading and compiling SuperKaramba
- •Versatile Downloading with wget
- •Mirroring sites with wget
- •Verifying your bookmarks with wget
- •Downloading files with wget
- •Downloading and unpacking in one quick step
- •Downloading and Uploading with curl
- •Setting Up ADIOS
- •Downloading ADIOS
- •Burning ADIOS to CD
- •Installing ADIOS
- •Finding Your Way around UML
- •Connecting to the Internet from an ADIOS VM
- •Using a GUI with UML
- •Installing Software into UML
- •Merging Changes to Your Prototype
- •Querying RPM Packages for Content
- •Digesting Information
- •Creating a Package Index
- •Querying for Prerequisites
- •Dissecting an RPM Package
- •Using RPM at the Command Line
- •Removing RPMs
- •Flagging Down RPM
- •Getting Graphic with RPM
- •Using Rpmdrake to install from media
- •Installing from your Konqueror browser
- •Verifying Your System
- •Reading the Tamper-Proof Seal
- •Setting Up Synaptic and apt in a Snap
- •Keeping Up-to-Date with apt and Synaptic: The Basics
- •Handy Hints about Synaptic
- •Changing repositories
- •Viewing package details
- •Installing new packages with Synaptic
- •Importing the Keys to the Repository
- •Letting Task Scheduler Work for You
- •Scheduling a new task
- •Editing a task
- •Adding environment variables
- •Reining In Resources with Disk Quotas
- •Installing the quota RPM package
- •Enabling file system quotas
- •Getting your files together
- •Setting quotas
- •Reviewing your quotas
- •Using System Accounting to Keep Track of Users
- •Setting up system accounting
- •Looking up user login hours
- •Checking out command and program usage
- •Running Down the Runlevels
- •Runlevel basics
- •Customizing runlevels in Fedora
- •Customizing runlevels in SuSE
- •Customizing runlevels in Mandrake
- •Customizing runlevels at the command line
- •Switching to a new runlevel
- •Disabling Unused Services
- •Removing Unneeded Services
- •Learning about modules
- •Installing a module with insmod
- •Taking care of dependencies automatically with modprobe and depmod
- •Loading a module for a slightly different kernel with insmod and modprobe
- •Removing modules with rmmod
- •Step 1: Making an Emergency Plan, or Boot Disk
- •Step 2: Finding the Source Code
- •Step 4: Customizing the Kernel
- •Step 5: Building the Kernel
- •Understanding the Principles of SELinux
- •Everything is an object
- •Identifying subjects in SELinux
- •Understanding the security context
- •Disabling or Disarming SELinux
- •Playing the Right Role
- •Exploring the Process-Related Entries in /proc
- •Surveying Your System from /proc
- •Popping the Cork: Speeding Up WINE with /proc
- •Reading and Understanding File Permissions
- •Controlling Permissions at the Command Line
- •Changing File Permissions from a Desktop
- •Encryption Made Easy with kgpg and the KDE Desktop
- •Creating keys with kgpg
- •Sharing your key with the world
- •Importing a public key from a public-key server
- •Encrypting and decrypting documents with drag-and-drop ease
- •Encrypting Documents with gpg at the Command Line
- •Sharing a secret file
- •Creating a key pair and receiving encrypted documents
- •Encrypting documents on your home system
- •Encrypting E-Mail for Added Security
- •Encrypting with Ximian Evolution
- •Setting up Mozilla e-mail for encryption
- •Sending and receiving encrypted messages with Mozilla mail
- •Using Cross-Platform Authentication with Linux and Windows
- •Prepping for cross-platform authentication
- •Setting up cross-platform authentication
- •Using PAM and Kerberos to Serve Up Authentication
- •Establishing synchronized system times
- •Testing your domain name server (DNS)
- •Setting up a Key Distribution Center
- •Setting up automatic ticket management with Kerberos and PAM
- •Adding users to the Key Distribution Center
- •Building Good Rules with PAM
- •Phase
- •Control level
- •Module pathname
- •Arguments
- •Dissecting a Configuration File
- •Skipping a Password with PAM
- •Feeling the Power
- •Gaining Superuser Privileges
- •Pretending to Be Other Users
- •Limiting Privileges with sudo
- •Installing sudo
- •Adding Up the Aliases
- •Adding Aliases to the sudo Configuration File
- •Defining the Alias
- •Creating a User_Alias
- •Creating a Runas_Alias
- •Simplifying group managment with a Host_Alias
- •Mounting and unmounting CDs without the superuser password
- •Managing access to dangerous commands with command aliases
- •Using SSH for Top-Speed Connections
- •Setting Up Public-Key Authentication to Secure SSH
- •Generating the key pair
- •Distributing your public key
- •Passing on your passphrase
- •Logging In with SSH and Key Authentication
- •Starting from the command line
- •Getting graphic
- •Creating Shortcuts to Your Favorite SSH Locations
- •Copying Files with scp
- •Secure (And Fast) Port Forwarding with SSH
- •Finding Your Firewall
- •Setting up a simple firewall in Mandrake Linux
- •Setting up a simple firewall in Fedora Linux
- •Setting up a simple firewall in SuSE Linux
- •Editing the Rules with Webmin
- •Starting a Webmin session
- •Reading the rules with Webmin
- •Changing the rules
- •Editing existing rules
- •Adding a new rule with Webmin
- •Sharing Desktops with VNC
- •Inviting Your Friends to Use Your Desktop
- •Serving Up a New Desktop with VNC Server
- •Using tsclient to View Remote Desktops from Linux
- •Using tsclient with a VNC server
- •Using tsclient with an RDP server
- •Creating New VNC Desktops on Demand
- •Switching display managers in SuSE Linux
- •Switching display managers in Mandrake Linux
- •Connecting gdm and VNC
- •Exploring Your Network with lsof
- •Running lsof
- •Interpreting the lsof output
- •Reading file types
- •Discovering Network Connections
- •Other Timesaving lsof Tricks
- •Packet Sniffing with the Ethereal Network Analyzer
- •Starting Ethereal
- •Capturing packets
- •Applying filters to screen packets
- •Peeking in packets
- •Color-coding packets coming from your network
- •Getting Up and Running with Nessus
- •Installing programs Nessus needs to run
- •Installing Nessus
- •Adding a user to Nessus
- •Generating a certificate
- •Starting the daemon and the interface
- •Reading the grim results
- •Keeping Your Plug-ins Up-to-Date
- •Chatting in the Fedora Chat Room
- •Looking for Answers in the SuSE Chat Room
- •Processing Processes with procps
- •Using ps to filter process status information
- •Viewing ps output the way you want to see it
- •Making parent-child relationships stand out in a ps listing
- •Climbing the family tree with pstree
- •Finding processes with pgrep
- •Killing Processes with pkill
- •Killing Processes with killall
- •Closing Windows with xkill
- •Managing Users and Groups with the Fedora/Mandrake User Manager
- •Adding new users
- •Modifying user accounts
- •Adding groups
- •Filtering users and groups
- •Managing Users and Groups with the SuSE User Administrator
- •Adding new users
- •Modifying user accounts
- •Adding groups
- •Filtering users and groups
- •Adding and deleting log files from the viewer
- •Setting up alerts and warnings
- •Viewing your log files from SuSE
- •Monitoring your log files from SuSE
- •Customizing Your Log Files
- •Keeping an Eye on Resources with KDE System Guard
- •Finding and killing runaway processes
- •Prioritizing processes to smooth a network bottleneck
- •Watching your system load
- •Creating a new worksheet
- •Creating system resource logs
- •Displaying network resources
- •Using Synaptic to download and install Apache
- •Installing Apache from disc
- •Starting the Apache Service
- •Building a Quick Web Page with OpenOffice.org
- •Taking Your Site Public with Dynamic DNS
- •Understanding how dynamic DNS works
- •Setting up dynamic DNS
- •Updating your IP address
- •Installing the Fedora HTTP Configuration tool
- •Putting the HTTP Configuration tool to work
- •Watching Your Web Server Traffic with apachetop
- •Installing apachetop
- •Running and exiting apachetop
- •Navigating apachetop
- •Switching among the log files (or watching several at once)
- •Changing the display time of apachetop statistics
- •Accessing MySQL Control Center features
- •Viewing, managing, and repairing a database with the Databases controls
- •Putting the Server Administration controls to work
- •Adding a new user
- •Watching Your MySQL Traffic with mtop
- •Gathering all the packages that mtop needs
- •Installing mtop
- •Monitoring traffic
- •Building a MySQL Server
- •Installing the necessary packages
- •Starting the MySQL server
- •Replicating MySQL Data
- •Configuring replication: The three topologies
- •Setting up replication for a single slave and master
- •Choosing a Method to Back Up MySQL Data
- •Backing Up and Restoring with mysqldump
- •mysqldump backup options
- •Backing up multiple databases
- •Compressing the archive
- •Restoring a mysqldump archive
- •Making a mysqlhotcopy of Your Database
- •Archiving a Replication Slave
- •Taking Care of Business with MySQL Administrator
- •Installing MySQL Administrator
- •Starting MySQL Administrator
- •Choosing an SSL Certificate
- •Creating a Certificate Signing Request
- •Creating a Signing Authority with openssl
- •Creating a certificate authority
- •Signing a CSR
- •Exploring Your Certificate Collection with Mozilla
- •Introducing hotway
- •Getting Started with hotway
- •Setting Up Evolution to Read HTTPMail Accounts with hotway
- •Ringing the Bells and Blowing the Whistles: Your Evolution Summary Page
- •Installing SpamAssassin
- •Installing from the distribution media
- •Installing from RPM downloads
- •Starting the service
- •Fine-Tuning SpamAssassin to Separate the Ham from the Spam
- •Customizing settings
- •Saving your settings
- •Adding a New Filter to Evolution
- •Serving Up a Big Bowl of the RulesDuJour
- •Registering Your Address
- •Taming a Sendmail Server
- •Tweaking Your Configuration Files with Webmin
- •Serving up mail for multiple domains
- •Relaying e-mail
- •Using aliases to simplify mail handling
- •Deciding What to Archive
- •Choosing Archive Media
- •Tape drives
- •Removable and external disk drives
- •Removable media
- •Optical media (CDs and DVDs)
- •Online storage
- •Choosing an Archive Scheme
- •Full backups
- •Differential backups
- •Incremental backups
- •Incremental versus differential backups
- •Choosing an Archive Program
- •Estimating Your Media Needs
- •Creating Data Archives with tar
- •Backing up files and directories
- •Backing up account information and passwords
- •Targeting bite-sized backups for speedier restores
- •Rolling whole file systems into a tarball
- •Starting an Incremental Backup Cycle
- •Restoring from Backup with tar
- •Backing Up to CD (Or DVD) with cdbackup
- •Creating the backup
- •Restoring from a CD or DVD backup
- •Restoring from a disc containing multiple archives
- •Combining the Power of tar with ssh for Quick Remote Backups
- •Testing the ssh connection to the remote host
- •Creating a tar archive over the ssh connection
- •Backing up to tape drives on remote machines
- •Backing Up to a Remote Computer with rdist and ssh
- •Testing the ssh connection to the remote host
- •Creating the distfile
- •Backing up
- •Getting Started with CVS
- •Checking whether CVS is installed
- •Discovering what to use CVS for
- •Creating a CVS Repository
- •Populating Your Repository with Files
- •Simplifying CVS with cervisia
- •Installing cervisia
- •Putting files in your sandbox
- •Adding more files to your repository
- •Committing your changes
- •Browsing your log files
- •Marking milestones with tags
- •Branching off with cervisia
- •Using the libcurl Library (C Programming)
- •Uploading a File with a Simple Program Using libcurl
- •Line 7: Defining functions and data types
- •Line 14: Calling the initialization function
- •Lines 18– 21: Defining the transfer
- •Line 23: Starting the transfer
- •Line 26: Finishing the upload
- •Installing the Ming Library
- •Building a Simple Flash Movie with Ming
- •Examining the program
- •Compiling the program
- •Running the program
- •Building Interactive Movies with Ming
- •Examining the program
- •Compiling the program
- •Running the program
- •Doing the curl E-shuffle with PHP
- •Combining PHP with curl and XML: An overview
- •Checking out the XML file
- •Downloading and displaying the XML file with a PHP script (and curl)
- •Sending E-Mail from PHP When Problems Occur
- •Debugging Perl Code with DDD
- •Installing and starting DDD
- •Examining the main window
- •Reviewing and stepping through source code
- •Making Stop Signs: Using Breakpoints to Watch Code
- •Setting a breakpoint
- •Modifying a breakpoint
- •Opening the data window
- •Adding a variable to the data window
- •Changing the display to a table
- •Using the Backtrace feature
- •Using the Help menu
- •Making Fedora Distribution CDs
- •Downloading the ISO images
- •Verifying the checksums
- •Burning an ISO File to Disc at the Command Line
- •Finding the identity of your drive
- •Running a test burn
- •Burning the distribution discs
- •Burning CDs without Making an ISO First
- •Finding setuid quickly and easily with kfind
- •Finding setuid and setgid programs at the command line
- •Deciding to Turn Off setuid or setgid
- •Changing the setuid or setgid Bit
- •Who Belongs in Jail?
- •Using UML to Jail Programs
- •Using lsof to Find Out Which Files Are Open
- •Debugging Your Environment with strace
- •Investigating Programs with ltrace
- •Handy strace and ltrace Options
- •Recording Program Errors with valgrind
- •Hardening Your Hat with Bastille
- •Downloading and installing Bastille and its dependencies
- •Welcome to the Bastille
- •Addressing file permission issues
- •Clamping down on SUID privileges
- •Moving on to account security
- •Making the boot process more secure
- •Securing connection broker
- •Limiting compiler access
- •Limiting access to hackers
- •Logging extra information
- •Keeping the daemons in check
- •Securing sendmail
- •Closing the gaps in Apache
- •Keeping temporary files safe
- •Building a better firewall
- •Port scanning with Bastille
- •Turning LIDS On and Off
- •Testing LIDS before Applying It to Your System
- •Controlling File Access with LIDS
- •Hiding Processes with LIDS
- •Running Down the Privilege List
- •Getting Graphical at the Command Line
- •Getting graphical in GNOME
- •Getting graphical with KDE
- •Staying desktop neutral
- •Index
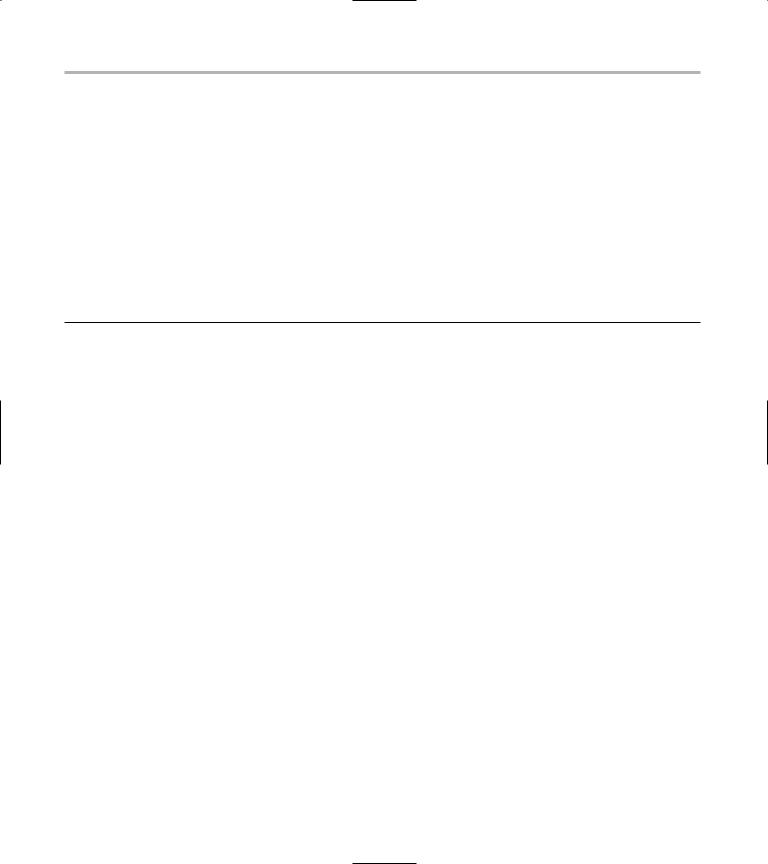
408 Technique 53: Using Open-Source APIs to Save Time
Building a Simple Flash Movie with Ming
Listing 53-2 shows a simple Ming program that slowly rotates the colors in a given photo.
Examining the program
The following sections take a closer look at the colors program, shown in Listing 53-2.
LISTING 53-2: COLORS.C
Line 6: Defining the functions and data types
Every Ming program must include the ming.h header file (line 6). ming.h defines the data types and function prototype for the Ming library.
Line 19: Creating an empty movie
To create a new Flash movie, start with a call to the newSWFMovie() function (see line 19). newSWFMovie() creates a new, empty movie and returns a handle that you can use later when you call other Ming functions.
1 |
/* File: colors.c |
|
|
2 |
** |
|
|
3 |
** |
Rotate colors within a photo |
|
4 |
*/ |
|
|
5 |
|
|
|
6 |
#include <ming.h> |
|
|
7 |
#include <stdio.h> |
|
|
8 |
#include <stdlib.h> |
|
|
9 |
|
|
|
10 |
static SWFDisplayItem load_photo( SWFMovie movie, const char * name ); |
||
11 |
|
|
|
12 |
int main( int argc, char * argv[] ) |
||
13 |
{ |
|
|
14 |
|
SWFMovie |
movie; |
15 |
|
SWFDisplayItem item; |
|
16 |
|
int |
frames = 40; |
17 |
|
float |
count; |
18 |
|
|
|
19movie = newSWFMovie();
20item = load_photo( movie, argv[1] );
22for( count = 0; count < frames; ++count )
23{
24SWFDisplayItem_multColor( item, 1.0, 1.0, 1.0 - count/frames, 1.0 );
25SWFDisplayItem_addColor( item, 255 * count/frames, 0, 0, 1.0 );
26
27SWFMovie_nextFrame( movie );
28}
29
30for( count = frames; count > 0; --count )
31{
32SWFDisplayItem_multColor( item, 1.0, 1.0, 1.0 - count/frames, 1.0 );
33SWFDisplayItem_addColor( item, 255 * count/frames, 0, 0, 1.0 );
34
35 SWFMovie_nextFrame( movie );
36 }

Building a Simple Flash Movie with Ming |
409 |
37
38 SWFMovie_save( movie, “colors.swf”, -1 );
39
40exit( EXIT_SUCCESS );
41}
42 |
|
|
43 |
static SWFDisplayItem load_photo( SWFMovie movie, const char * name ) |
|
44 |
{ |
|
45 |
SWFBitmap |
bitmap; |
46 |
SWFInput |
photo_file; |
47 |
FILE |
* photo; |
48 |
int |
height; |
49 |
int |
width; |
50 |
|
|
51if(( photo = fopen( name, “rb” )) == NULL )
52{
53fprintf( stderr, “can’t open photo\n” );
54exit( EXIT_FAILURE );
55}
56else
57{
58photo_file = newSWFInput_file( photo );
59}
60
61 bitmap = newSWFBitmap_fromInput( photo_file ); 62
63width = SWFBitmap_getWidth( bitmap );
64height = SWFBitmap_getHeight( bitmap );
66SWFMovie_setDimension( movie, width, height );
68return( SWFMovie_add( movie, (SWFBlock) bitmap ));
70}
Line 20: Adding a photo to the movie
At line 20, you see a call to the load_photo() function. load_photo() is not a Ming function (we show it to you in a moment in the section, “Line 43: Telling Ming how to read the photo file”). load_photo() returns a handle to a bitmap that’s been added to the new movie.
Lines 22–28 and 30–36: Making the photo move through frames
Line 24 adjusts each pixel in the photo. In a Flash movie, every pixel has four values: a red value, a green value, a blue value, and an opaqueness value. The color values range from zero (no color) to 255 (full color). The opaqueness value (also known as the alpha value) determines how much of the background shows through. An opaqueness value of zero means the pixel is invisible, and a value of 255 means the pixel is completely opaque (none of the background shows through).
Lines 22–28 create a number of frames (a movie is made up of one or more frames), each with a slightly modified version of the photo.
Each time through the loop (lines 22–28),
SWDisplayItem_multColor() multiplies each color value in each pixel. The first argument identifies the
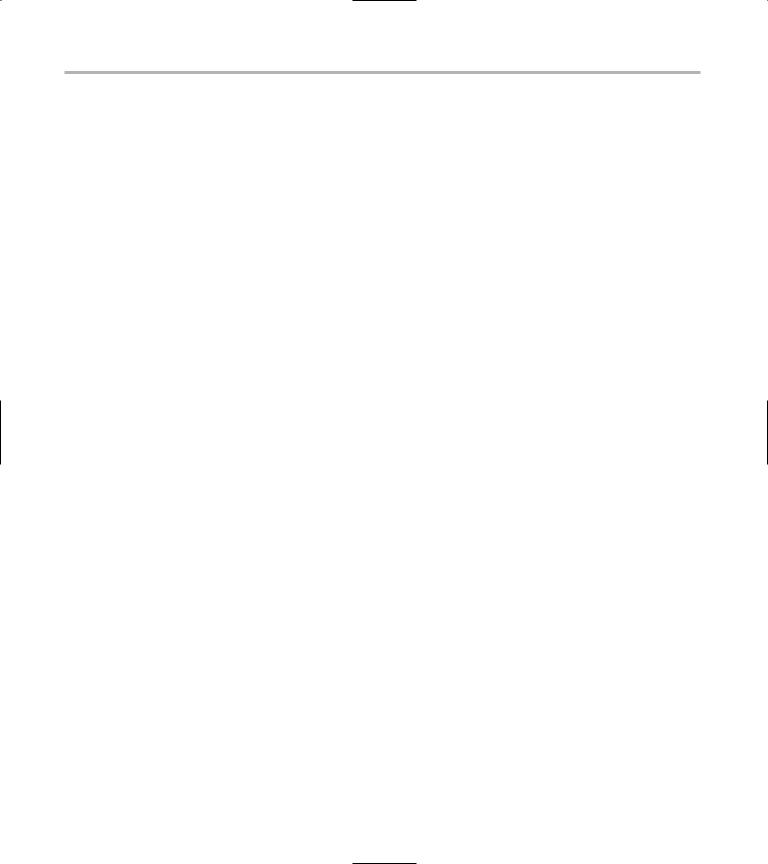
410 Technique 53: Using Open-Source APIs to Save Time
display item you want to adjust. The second item specifies a multiplier for the red value of each pixel (multiplying by 1.0 means the red value is unchanged). The third, fourth, and fifth arguments specify the multipliers applied to the green, blue, and opaqueness values for each pixel.
Line 24 decreases the blue value by a fraction each time through the loop but leaves the other values unchanged. The net effect is that the blueness of your photo slowly fades away with each frame. Line 25 adds a dash of red to each pixel in the frame (again, leaving the other color components unchanged).
Lines 30–36 do essentially the same thing as lines 22–28, but rotate through colors in the opposite direction — subtracting some red and adding back the blue in each frame.
Line 38: Saving the completed movie
After the movie is complete, call SWFMovie_save() to write it to disk (in this case, to a file named colors.swf).
Line 43: Telling Ming how to read the photo file
Now take a look at the load_photo() function (starting at line 43). load_photo() starts by opening the file whose name was passed as the second argument. The Ming library can’t really do much with a FILE handle.
To read the content of the photo, you have to convert the FILE handle into an SWFInput. Whenever the Ming library needs to read information from some source, it uses an SWFInput handle. Ming can create SWFInput handles from a number of different source types. For example, the newSWFInput_buffer() function will create an SWFInput that can read data from an area of memory. Because you already have a FILE handle, you can use newSWFInput_file() to convert it into an SWFInput (see line 58).
Line 61 and 63–66: Showing Ming how to work with the photo
Line 61 reads the photo bits and creates a new structure called an SWFBitmap by calling newSWFBitmap_ fromInput().
Lines 63 and 64 interrogate the bitmap to find the width and height (in pixels), and line 66 changes the size of the movie to match the bitmap. Finally, load_ photo() adds the bitmap to the current frame of the movie and returns a handle to the caller.
Compiling the program
Before you can run colors, you have to compile it, and the following steps show you how to do that using make:
1. Open your favorite editor and enter the following text:
LDFLAGS += -lming -lz
LDFLAGS += -lungif -lpng12 -lm colors: colors.c
2. Save your changes in a file named Makefile and close the editor.
3. Type make and press Enter.
The compiler starts running.
If everything goes well, you have a new program in your current directory named colors. If you see any error messages, go back and correct the errors before proceeding.
Running the program
To run colors, find a photo (JPEGs work well) that you like and then run the following command:
$ ./colors /path-to-photo
Substitute the name of your photo for path-to-photo. When colors is finished, you’ll have a new Flash movie in your current directory named colors.swf.