
- •preface
- •acknowledgments
- •about this book
- •Who should read this book?
- •Roadmap
- •Code conventions
- •Code downloads
- •Author Online
- •About the title
- •About the cover illustration
- •Rethinking the web application
- •A new design for the Web
- •1.1 Why Ajax rich clients?
- •1.1.1 Comparing the user experiences
- •1.1.2 Network latency
- •1.1.3 Asynchronous interactions
- •1.1.4 Sovereign and transient usage patterns
- •1.1.5 Unlearning the Web
- •1.2 The four defining principles of Ajax
- •1.2.1 The browser hosts an application, not content
- •1.2.2 The server delivers data, not content
- •1.2.3 User interaction with the application can be fluid and continuous
- •1.2.4 This is real coding and requires discipline
- •1.3 Ajax rich clients in the real world
- •1.3.1 Surveying the field
- •1.3.2 Google Maps
- •1.4 Alternatives to Ajax
- •1.4.2 Java Web Start and related technologies
- •1.5 Summary
- •1.6 Resources
- •First steps with Ajax
- •2.1 The key elements of Ajax
- •2.2 Orchestrating the user experience with JavaScript
- •2.3 Defining look and feel using CSS
- •2.3.1 CSS selectors
- •2.3.2 CSS style properties
- •2.3.3 A simple CSS example
- •2.4 Organizing the view using the DOM
- •2.4.1 Working with the DOM using JavaScript
- •2.4.2 Finding a DOM node
- •2.4.3 Creating a DOM node
- •2.4.4 Adding styles to your document
- •2.4.5 A shortcut: Using the innerHTML property
- •2.5 Loading data asynchronously using XML technologies
- •2.5.1 IFrames
- •2.5.2 XmlDocument and XMLHttpRequest objects
- •2.5.3 Sending a request to the server
- •2.5.4 Using callback functions to monitor the request
- •2.5.5 The full lifecycle
- •2.6 What sets Ajax apart
- •2.7 Summary
- •2.8 Resources
- •Introducing order to Ajax
- •3.1 Order out of chaos
- •3.1.1 Patterns: creating a common vocabulary
- •3.1.2 Refactoring and Ajax
- •3.1.3 Keeping a sense of proportion
- •3.1.4 Refactoring in action
- •3.2 Some small refactoring case studies
- •3.2.2 Managing event handlers: Observer pattern
- •3.2.3 Reusing user action handlers: Command pattern
- •3.2.4 Keeping only one reference to a resource: Singleton pattern
- •3.3 Model-View-Controller
- •3.4 Web server MVC
- •3.4.1 The Ajax web server tier without patterns
- •3.4.2 Refactoring the domain model
- •3.4.3 Separating content from presentation
- •3.5 Third-party libraries and frameworks
- •3.5.2 Widgets and widget suites
- •3.5.3 Application frameworks
- •3.6 Summary
- •3.7 Resources
- •Core techniques
- •The page as an application
- •4.1 A different kind of MVC
- •4.1.1 Repeating the pattern at different scales
- •4.1.2 Applying MVC in the browser
- •4.2 The View in an Ajax application
- •4.2.1 Keeping the logic out of the View
- •4.2.2 Keeping the View out of the logic
- •4.3 The Controller in an Ajax application
- •4.3.1 Classic JavaScript event handlers
- •4.3.2 The W3C event model
- •4.3.3 Implementing a flexible event model in JavaScript
- •4.4 Models in an Ajax application
- •4.4.1 Using JavaScript to model the business domain
- •4.4.2 Interacting with the server
- •4.5 Generating the View from the Model
- •4.5.1 Reflecting on a JavaScript object
- •4.5.2 Dealing with arrays and objects
- •4.5.3 Adding a Controller
- •4.6 Summary
- •4.7 Resources
- •The role of the server
- •5.1 Working with the server side
- •5.2 Coding the server side
- •5.2.1 Popular implementation languages
- •5.3 The big picture: common server-side designs
- •5.3.1 Naive web server coding without a framework
- •5.3.2 Working with Model2 workflow frameworks
- •5.4 The details: exchanging data
- •5.4.2 Introducing the planet browser example
- •5.5 Writing to the server
- •5.5.1 Using HTML forms
- •5.5.2 Using the XMLHttpRequest object
- •5.5.3 Managing user updates effectively
- •5.6 Summary
- •5.7 Resources
- •Professional Ajax
- •The user experience
- •6.1 Getting it right: building a quality application
- •6.1.1 Responsiveness
- •6.1.2 Robustness
- •6.1.3 Consistency
- •6.1.4 Simplicity
- •6.1.5 Making it work
- •6.2 Keeping the user informed
- •6.2.1 Handling responses to our own requests
- •6.2.2 Handling updates from other users
- •6.3 Designing a notification system for Ajax
- •6.3.1 Modeling notifications
- •6.3.2 Defining user interface requirements
- •6.4 Implementing a notification framework
- •6.4.1 Rendering status bar icons
- •6.4.2 Rendering detailed notifications
- •6.4.3 Putting the pieces together
- •6.5 Using the framework with network requests
- •6.6 Indicating freshness of data
- •6.6.1 Defining a simple highlighting style
- •6.6.2 Highlighting with the Scriptaculous Effects library
- •6.7 Summary
- •6.8 Resources
- •Security and Ajax
- •7.1 JavaScript and browser security
- •7.1.1 Introducing the “server of origin” policy
- •7.1.2 Considerations for Ajax
- •7.1.3 Problems with subdomains
- •7.2 Communicating with remote services
- •7.2.1 Proxying remote services
- •7.2.2 Working with web services
- •7.3 Protecting confidential data
- •7.3.1 The man in the middle
- •7.3.2 Using secure HTTP
- •7.3.3 Encrypting data over plain HTTP using JavaScript
- •7.4 Policing access to Ajax data streams
- •7.4.1 Designing a secure web tier
- •7.4.2 Restricting access to web data
- •7.5 Summary
- •7.6 Resources
- •Performance
- •8.1 What is performance?
- •8.2 JavaScript execution speed
- •8.2.1 Timing your application the hard way
- •8.2.2 Using the Venkman profiler
- •8.2.3 Optimizing execution speed for Ajax
- •8.3 JavaScript memory footprint
- •8.3.1 Avoiding memory leaks
- •8.3.2 Special considerations for Ajax
- •8.4 Designing for performance
- •8.4.1 Measuring memory footprint
- •8.4.2 A simple example
- •8.5 Summary
- •8.6 Resources
- •Ajax by example
- •Dynamic double combo
- •9.1 A double-combo script
- •9.2 The client-side architecture
- •9.2.1 Designing the form
- •9.2.2 Designing the client/server interactions
- •9.3 Implementing the server: VB .NET
- •9.3.1 Defining the XML response format
- •9.4 Presenting the results
- •9.4.1 Navigating the XML document
- •9.4.2 Applying Cascading Style Sheets
- •9.5 Advanced issues
- •9.5.2 Moving from a double combo to a triple combo
- •9.6 Refactoring
- •9.6.1 New and improved net.ContentLoader
- •9.7 Summary
- •Type-ahead suggest
- •10.1 Examining type-ahead applications
- •10.1.2 Google Suggest
- •10.2.1 The server and the database
- •10.3 The client-side framework
- •10.3.1 The HTML
- •10.3.2 The JavaScript
- •10.3.3 Accessing the server
- •10.5 Refactoring
- •10.5.1 Day 1: developing the TextSuggest component game plan
- •10.5.3 Day 3: Ajax enabled
- •10.5.4 Day 4: handling events
- •10.5.6 Refactor debriefing
- •10.6 Summary
- •11.1 The evolving portal
- •11.1.1 The classic portal
- •11.1.2 The rich user interface portal
- •11.2 The Ajax portal architecture using Java
- •11.3 The Ajax login
- •11.3.1 The user table
- •11.4 Implementing DHTML windows
- •11.4.1 The portal windows database
- •11.4.3 Adding the JS external library
- •11.5 Adding Ajax autosave functionality
- •11.5.1 Adapting the library
- •11.5.2 Autosaving the information to the database
- •11.6 Refactoring
- •11.6.1 Defining the constructor
- •11.6.2 Adapting the AjaxWindows.js library
- •11.6.3 Specifying the portal commands
- •11.6.4 Performing the Ajax processing
- •11.6.5 Refactoring debrief
- •11.7 Summary
- •Live search using XSLT
- •12.1 Understanding the search techniques
- •12.1.1 Looking at the classic search
- •12.1.3 Examining a live search with Ajax and XSLT
- •12.1.4 Sending the results back to the client
- •12.2 The client-side code
- •12.2.1 Setting up the client
- •12.2.2 Initiating the process
- •12.3 The server-side code: PHP
- •12.3.1 Building the XML document
- •12.3.2 Building the XSLT document
- •12.4 Combining the XSLT and XML documents
- •12.4.1 Working with Microsoft Internet Explorer
- •12.4.2 Working with Mozilla
- •12.5 Completing the search
- •12.5.1 Applying a Cascading Style Sheet
- •12.5.2 Improving the search
- •12.5.3 Deciding to use XSLT
- •12.5.4 Overcoming the Ajax bookmark pitfall
- •12.6 Refactoring
- •12.6.1 An XSLTHelper
- •12.6.2 A live search component
- •12.6.3 Refactoring debriefing
- •12.7 Summary
- •Building stand-alone applications with Ajax
- •13.1 Reading information from the outside world
- •13.1.1 Discovering XML feeds
- •13.1.2 Examining the RSS structure
- •13.2 Creating the rich user interface
- •13.2.1 The process
- •13.2.3 Compliant CSS formatting
- •13.3 Loading the RSS feeds
- •13.3.1 Global scope
- •13.3.2 Ajax preloading functionality
- •13.4 Adding a rich transition effect
- •13.4.2 Implementing the fading transition
- •13.4.3 Integrating JavaScript timers
- •13.5 Additional functionality
- •13.5.1 Inserting additional feeds
- •13.5.2 Integrating the skipping and pausing functionality
- •13.6 Avoiding the project’s restrictions
- •13.6.1 Overcoming Mozilla’s security restriction
- •13.6.2 Changing the application scope
- •13.7 Refactoring
- •13.7.1 RSS reader Model
- •13.7.2 RSS reader view
- •13.7.3 RSS reader Controller
- •13.7.4 Refactoring debrief
- •13.8 Summary
- •The Ajax craftsperson’s toolkit
- •A.1 Working smarter with the right toolset
- •A.1.1 Acquiring tools that fit
- •A.1.2 Building your own tools
- •A.1.3 Maintaining your toolkit
- •A.2 Editors and IDEs
- •A.2.1 What to look for in a code editor
- •A.2.2 Current offerings
- •A.3 Debuggers
- •A.3.1 Why we use a debugger
- •A.3.2 JavaScript debuggers
- •A.3.3 HTTP debuggers
- •A.3.4 Building your own cross-browser output console
- •A.4 DOM inspectors
- •A.4.1 Using the Mozilla DOM Inspector
- •A.4.2 DOM inspectors for Internet Explorer
- •A.4.3 The Safari DOM Inspector for Mac OS X
- •A.5 Installing Firefox extensions
- •A.6 Resources
- •JavaScript for object-oriented programmers
- •B.1 JavaScript is not Java
- •B.2 Objects in JavaScript
- •B.2.1 Building ad hoc objects
- •B.2.2 Constructor functions, classes, and prototypes
- •B.2.3 Extending built-in classes
- •B.2.4 Inheritance of prototypes
- •B.2.5 Reflecting on JavaScript objects
- •B.2.6 Interfaces and duck typing
- •B.3 Methods and functions
- •B.3.1 Functions as first-class citizens
- •B.3.2 Attaching functions to objects
- •B.3.3 Borrowing functions from other objects
- •B.3.4 Ajax event handling and function contexts
- •B.3.5 Closures in JavaScript
- •B.4 Conclusions
- •B.5 Resources
- •Ajax frameworks and libraries
- •Accesskey Underlining Library
- •ActiveWidgets
- •Ajax JavaServer Faces Framework
- •Ajax JSP Tag Library
- •Ajax.NET
- •AjaxAC
- •AjaxAspects
- •AjaxCaller
- •AjaxFaces
- •BackBase
- •Behaviour
- •Bindows
- •BlueShoes
- •CakePHP
- •CL-Ajax
- •ComfortASP.NET
- •Coolest DHTML Calendar
- •Dojo
- •DWR (Direct Web Remoting)
- •Echo 2
- •FCKEditor
- •Flash JavaScript Integration Kit
- •Google AjaxSLT
- •Guise
- •HTMLHttpRequest
- •Interactive Website Framework
- •Jackbe
- •JPSpan
- •jsolait
- •JSON
- •JSRS (JavaScript Remote Scripting)
- •LibXMLHttpRequest
- •Mochikit
- •netWindows
- •Oddpost
- •OpenRico
- •Pragmatic Objects
- •Prototype
- •Qooxdoo
- •RSLite
- •Ruby on Rails
- •Sack
- •SAJAX
- •Sarissa
- •Scriptaculous
- •SWATO…
- •Tibet
- •TinyMCE
- •TrimPath Templates
- •Walter Zorn’s DHTML Libraries
- •WebORB for .NET
- •WebORB for Java
- •XAJAX
- •x-Desktop
- •XHConn
- •index
- •Symbols
- •Numerics
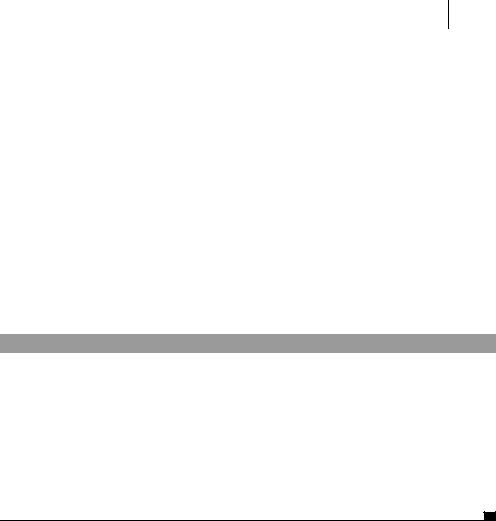
Refactoring 541
provide extended metadata for future features. We could also define accessor methods for the attributes to provide a more formal contract for accessing them. For example, we could write a getTitle()/setTitle() method pair for accessing the title attribute. Since JavaScript doesn’t support visibility semantics like other object-oriented languages (for example, the private/protected keywords in Java), we didn’t bother. Now let’s take a gander at our View.
13.7.2RSS reader view
With our Model classes securely in place, we can now consider a View class. We could develop a View class for the RSSFeed, and another for the RSSItem, but because our RSSReader doesn’t really view a feed independently of an item, we’ll define a single View class called RSSItemView, which encapsulates the View for an RSSItem in the context of its parent RSSFeed. Since the View in this case is obviously HTML, our View class is really just responsible for the generation of HTML. Let’s start by looking at the constructor in listing 13.25.
Listing 13.25 The RSSItemView View class
RSSItemView = Class.create();
RSSItemView.prototype = {
initialize: function(rssItem, feedIndex, itemIndex, numFeeds) { this.rssItem = rssItem;
this.feedIndex = feedIndex + 1; this.itemIndex = itemIndex + 1; this.numFeeds = numFeeds;
},
}
Let’s take a moment to consider the parameters. The first parameter is an instance of an RSSItem. This tells the View what Model instance it’s providing a view for. Note that it’s not generally copasetic for the Model classes to have any knowledge of the View, but the View by necessity typically has intimate knowledge of the Model. The other parameters provide some supplemental context for the View. The feedIndex tells the View which feed number it’s in. The itemIndex tells the View where this item resides within its parent RSSFeed’s array of items. The numFeeds tells the View how many feeds there are. All of these index-based parameters are for the View to indicate its place in the world, so to speak. The View might want to display a context area that indicates, for example, “this is feed
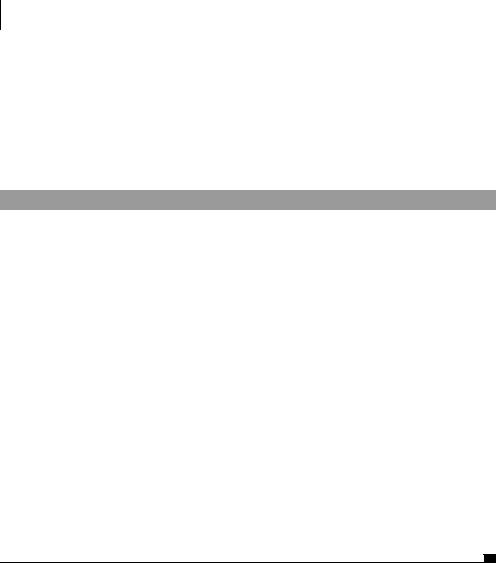
542CHAPTER 13
Building stand-alone applications with Ajax
number 1 of 7 and article number 3 of 5.” These attributes could be embedded within the Model, but they’re not really attributes that the Model should typically care about, so this context that the View needs is passed into the View constructor by the client.
As mentioned previously, the responsibility of the View is to generate HTML. So our View class will need a single method that does precisely that. Let’s see what that might look like in listing 13.26.
Listing 13.26 The HTML generation method
toHTML: function() { var out = ""
out += '<span class="rssFeedTitlePrompt">RSS Feed '
out += '(' + this.feedIndex + ' of ' + this.numFeeds + ') : '; out += '</span>';
out += '<span class="rssFeedTitle">';
out += '<a href="' + this.rssItem.rssFeed.link + '">' + this.rssItem.rssFeed.title + '</a>';
out += '</span>'; out += '<br/>';
out += '<span class="rssFeedItemTitlePrompt">Article '; out += '(' + this.itemIndex + ' of ' +
this.rssItem.rssFeed.items.length + ') : ';
out += '</span>';
out += '<span class="rssFeedItemTitle">';
out += '<a href="' + this.rssItem.link + '">' + this.rssItem.title + '</a>';
out += '</span>';
out += '<div class="rssItemContent">'; out += this.rssItem.description;
out += '</div>';
return out;
},
The toHTML method produces the contextual elements of the display followed by the text of the article. The first portion of the code displays the RSS Feed (x of y) : RSS Feed Title. The link attribute of the rssFeed parent is used to generate the HREF of the anchor produced, and the title is used to generate the text of the anchor. A CSS class name is generated for each span, one for the prompt, and another for the anchor, allowing each to be styled independently. This is illustrated in figure 13.15.
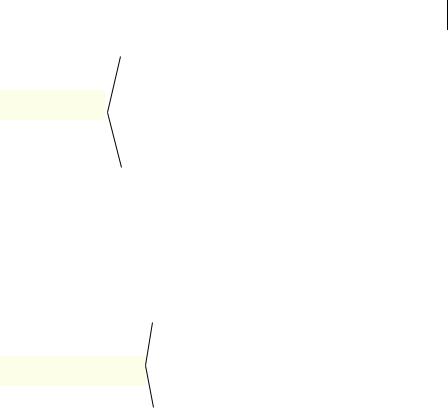
Refactoring 543
RSS Feed (2 of 4) : Eric's weblog
var out = ""
out += '<span class="rssFeedTitlePrompt">Rss Feed '
out += '(' + this.feedIndex + ' of ' + this.nubFeeds + ') : '; out += '</span>';
out += '<span class="rssFeedTitle">';
out += '<a href="' + this.rssItem.rssFeed.link + '">'
+ this.rssItem.rssFeed.title + '</a>';
out += '</span>'; out += '<br/>';
Figure 13.15 RSS Feed (x of y) : RSS Feed Title
The next portion of code generates the Article (x of y) : RSS Item Title. The link attribute of the RSS item is used to generate the HREF of the anchor produced, and the title of the item is used to generate the text of the anchor. This code also provides CSS class names for both the prompt and the title, as illustrated in figure 13.16.
Article (1 of 3) :
Sending XML to the Server
out += '<span class="rssFeedItemTitlePrompt">Article '; out += '(' + this.itemIndex + ' of '
+ this.rssItem.rssFeed.items.length + ') : '; out += '</span>';
out += '<span class="rssFeedItemTitle">';
+ this.rssItem.title + '</a>';
out += '</span>';
Figure 13.16 Article (x of y) : RSS Item Title
The last few lines of the toHTML method generate a div element to hold the content of the RSSItem’s article (the description attribute). The code for this is as follows:
out += '<div class="rssItemContent">'; out += this.rssItem.description;
out += '</div>';
The CSS class name rssItemContent is generated for the article content. It should have a little bit of margin and padding in order for the content to visually reside within the display without touching any borders. It should also have a fixed height and overflow set to auto so that the content scrolls when needed—inde- pendently of the contextual information shown previously. A representative CSS definition for this class is shown here:
.rssItemContent {
border-top : 1px solid black;
width |
: |
590px; |
height |
: |
350px; |
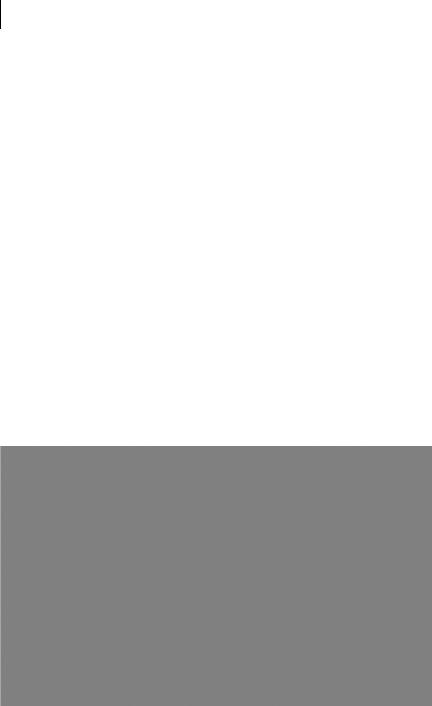
544CHAPTER 13
Building stand-alone applications with Ajax
overflow |
: |
auto; |
padding |
: |
5px; |
margin-top : 2px;
}
Given the code and style shown in this code, the content area produced should look something like the sample shown in figure 13.17.
Putting it all together, the view generated by our RSSItemView class is shown in figure 13.18.
Before we leave the topic of our View, let’s add one more little method to the View to make its usage more convenient:
toString: function() { return this.toHTML();
}
The reason we give the View a toString method is that it allows us to use the View instance and the HTML string that it generates interchangeably. For example, we can assign the View to the innerHTML attribute of an element, and the string representation, which is the HTML that it generates, will be used. For instance, the following code would assign the generated HTML of a view to the innerHTML of a div with the ID contentDiv:
var rssItemView = new RSSItemView( anRSSFeed, 0, 0, 5 ); $('contentDiv'). innerHTML = rssItemView;
Figure 13.17 Article (x of y) : RSS Item Title