
- •preface
- •acknowledgments
- •about this book
- •Who should read this book?
- •Roadmap
- •Code conventions
- •Code downloads
- •Author Online
- •About the title
- •About the cover illustration
- •Rethinking the web application
- •A new design for the Web
- •1.1 Why Ajax rich clients?
- •1.1.1 Comparing the user experiences
- •1.1.2 Network latency
- •1.1.3 Asynchronous interactions
- •1.1.4 Sovereign and transient usage patterns
- •1.1.5 Unlearning the Web
- •1.2 The four defining principles of Ajax
- •1.2.1 The browser hosts an application, not content
- •1.2.2 The server delivers data, not content
- •1.2.3 User interaction with the application can be fluid and continuous
- •1.2.4 This is real coding and requires discipline
- •1.3 Ajax rich clients in the real world
- •1.3.1 Surveying the field
- •1.3.2 Google Maps
- •1.4 Alternatives to Ajax
- •1.4.2 Java Web Start and related technologies
- •1.5 Summary
- •1.6 Resources
- •First steps with Ajax
- •2.1 The key elements of Ajax
- •2.2 Orchestrating the user experience with JavaScript
- •2.3 Defining look and feel using CSS
- •2.3.1 CSS selectors
- •2.3.2 CSS style properties
- •2.3.3 A simple CSS example
- •2.4 Organizing the view using the DOM
- •2.4.1 Working with the DOM using JavaScript
- •2.4.2 Finding a DOM node
- •2.4.3 Creating a DOM node
- •2.4.4 Adding styles to your document
- •2.4.5 A shortcut: Using the innerHTML property
- •2.5 Loading data asynchronously using XML technologies
- •2.5.1 IFrames
- •2.5.2 XmlDocument and XMLHttpRequest objects
- •2.5.3 Sending a request to the server
- •2.5.4 Using callback functions to monitor the request
- •2.5.5 The full lifecycle
- •2.6 What sets Ajax apart
- •2.7 Summary
- •2.8 Resources
- •Introducing order to Ajax
- •3.1 Order out of chaos
- •3.1.1 Patterns: creating a common vocabulary
- •3.1.2 Refactoring and Ajax
- •3.1.3 Keeping a sense of proportion
- •3.1.4 Refactoring in action
- •3.2 Some small refactoring case studies
- •3.2.2 Managing event handlers: Observer pattern
- •3.2.3 Reusing user action handlers: Command pattern
- •3.2.4 Keeping only one reference to a resource: Singleton pattern
- •3.3 Model-View-Controller
- •3.4 Web server MVC
- •3.4.1 The Ajax web server tier without patterns
- •3.4.2 Refactoring the domain model
- •3.4.3 Separating content from presentation
- •3.5 Third-party libraries and frameworks
- •3.5.2 Widgets and widget suites
- •3.5.3 Application frameworks
- •3.6 Summary
- •3.7 Resources
- •Core techniques
- •The page as an application
- •4.1 A different kind of MVC
- •4.1.1 Repeating the pattern at different scales
- •4.1.2 Applying MVC in the browser
- •4.2 The View in an Ajax application
- •4.2.1 Keeping the logic out of the View
- •4.2.2 Keeping the View out of the logic
- •4.3 The Controller in an Ajax application
- •4.3.1 Classic JavaScript event handlers
- •4.3.2 The W3C event model
- •4.3.3 Implementing a flexible event model in JavaScript
- •4.4 Models in an Ajax application
- •4.4.1 Using JavaScript to model the business domain
- •4.4.2 Interacting with the server
- •4.5 Generating the View from the Model
- •4.5.1 Reflecting on a JavaScript object
- •4.5.2 Dealing with arrays and objects
- •4.5.3 Adding a Controller
- •4.6 Summary
- •4.7 Resources
- •The role of the server
- •5.1 Working with the server side
- •5.2 Coding the server side
- •5.2.1 Popular implementation languages
- •5.3 The big picture: common server-side designs
- •5.3.1 Naive web server coding without a framework
- •5.3.2 Working with Model2 workflow frameworks
- •5.4 The details: exchanging data
- •5.4.2 Introducing the planet browser example
- •5.5 Writing to the server
- •5.5.1 Using HTML forms
- •5.5.2 Using the XMLHttpRequest object
- •5.5.3 Managing user updates effectively
- •5.6 Summary
- •5.7 Resources
- •Professional Ajax
- •The user experience
- •6.1 Getting it right: building a quality application
- •6.1.1 Responsiveness
- •6.1.2 Robustness
- •6.1.3 Consistency
- •6.1.4 Simplicity
- •6.1.5 Making it work
- •6.2 Keeping the user informed
- •6.2.1 Handling responses to our own requests
- •6.2.2 Handling updates from other users
- •6.3 Designing a notification system for Ajax
- •6.3.1 Modeling notifications
- •6.3.2 Defining user interface requirements
- •6.4 Implementing a notification framework
- •6.4.1 Rendering status bar icons
- •6.4.2 Rendering detailed notifications
- •6.4.3 Putting the pieces together
- •6.5 Using the framework with network requests
- •6.6 Indicating freshness of data
- •6.6.1 Defining a simple highlighting style
- •6.6.2 Highlighting with the Scriptaculous Effects library
- •6.7 Summary
- •6.8 Resources
- •Security and Ajax
- •7.1 JavaScript and browser security
- •7.1.1 Introducing the “server of origin” policy
- •7.1.2 Considerations for Ajax
- •7.1.3 Problems with subdomains
- •7.2 Communicating with remote services
- •7.2.1 Proxying remote services
- •7.2.2 Working with web services
- •7.3 Protecting confidential data
- •7.3.1 The man in the middle
- •7.3.2 Using secure HTTP
- •7.3.3 Encrypting data over plain HTTP using JavaScript
- •7.4 Policing access to Ajax data streams
- •7.4.1 Designing a secure web tier
- •7.4.2 Restricting access to web data
- •7.5 Summary
- •7.6 Resources
- •Performance
- •8.1 What is performance?
- •8.2 JavaScript execution speed
- •8.2.1 Timing your application the hard way
- •8.2.2 Using the Venkman profiler
- •8.2.3 Optimizing execution speed for Ajax
- •8.3 JavaScript memory footprint
- •8.3.1 Avoiding memory leaks
- •8.3.2 Special considerations for Ajax
- •8.4 Designing for performance
- •8.4.1 Measuring memory footprint
- •8.4.2 A simple example
- •8.5 Summary
- •8.6 Resources
- •Ajax by example
- •Dynamic double combo
- •9.1 A double-combo script
- •9.2 The client-side architecture
- •9.2.1 Designing the form
- •9.2.2 Designing the client/server interactions
- •9.3 Implementing the server: VB .NET
- •9.3.1 Defining the XML response format
- •9.4 Presenting the results
- •9.4.1 Navigating the XML document
- •9.4.2 Applying Cascading Style Sheets
- •9.5 Advanced issues
- •9.5.2 Moving from a double combo to a triple combo
- •9.6 Refactoring
- •9.6.1 New and improved net.ContentLoader
- •9.7 Summary
- •Type-ahead suggest
- •10.1 Examining type-ahead applications
- •10.1.2 Google Suggest
- •10.2.1 The server and the database
- •10.3 The client-side framework
- •10.3.1 The HTML
- •10.3.2 The JavaScript
- •10.3.3 Accessing the server
- •10.5 Refactoring
- •10.5.1 Day 1: developing the TextSuggest component game plan
- •10.5.3 Day 3: Ajax enabled
- •10.5.4 Day 4: handling events
- •10.5.6 Refactor debriefing
- •10.6 Summary
- •11.1 The evolving portal
- •11.1.1 The classic portal
- •11.1.2 The rich user interface portal
- •11.2 The Ajax portal architecture using Java
- •11.3 The Ajax login
- •11.3.1 The user table
- •11.4 Implementing DHTML windows
- •11.4.1 The portal windows database
- •11.4.3 Adding the JS external library
- •11.5 Adding Ajax autosave functionality
- •11.5.1 Adapting the library
- •11.5.2 Autosaving the information to the database
- •11.6 Refactoring
- •11.6.1 Defining the constructor
- •11.6.2 Adapting the AjaxWindows.js library
- •11.6.3 Specifying the portal commands
- •11.6.4 Performing the Ajax processing
- •11.6.5 Refactoring debrief
- •11.7 Summary
- •Live search using XSLT
- •12.1 Understanding the search techniques
- •12.1.1 Looking at the classic search
- •12.1.3 Examining a live search with Ajax and XSLT
- •12.1.4 Sending the results back to the client
- •12.2 The client-side code
- •12.2.1 Setting up the client
- •12.2.2 Initiating the process
- •12.3 The server-side code: PHP
- •12.3.1 Building the XML document
- •12.3.2 Building the XSLT document
- •12.4 Combining the XSLT and XML documents
- •12.4.1 Working with Microsoft Internet Explorer
- •12.4.2 Working with Mozilla
- •12.5 Completing the search
- •12.5.1 Applying a Cascading Style Sheet
- •12.5.2 Improving the search
- •12.5.3 Deciding to use XSLT
- •12.5.4 Overcoming the Ajax bookmark pitfall
- •12.6 Refactoring
- •12.6.1 An XSLTHelper
- •12.6.2 A live search component
- •12.6.3 Refactoring debriefing
- •12.7 Summary
- •Building stand-alone applications with Ajax
- •13.1 Reading information from the outside world
- •13.1.1 Discovering XML feeds
- •13.1.2 Examining the RSS structure
- •13.2 Creating the rich user interface
- •13.2.1 The process
- •13.2.3 Compliant CSS formatting
- •13.3 Loading the RSS feeds
- •13.3.1 Global scope
- •13.3.2 Ajax preloading functionality
- •13.4 Adding a rich transition effect
- •13.4.2 Implementing the fading transition
- •13.4.3 Integrating JavaScript timers
- •13.5 Additional functionality
- •13.5.1 Inserting additional feeds
- •13.5.2 Integrating the skipping and pausing functionality
- •13.6 Avoiding the project’s restrictions
- •13.6.1 Overcoming Mozilla’s security restriction
- •13.6.2 Changing the application scope
- •13.7 Refactoring
- •13.7.1 RSS reader Model
- •13.7.2 RSS reader view
- •13.7.3 RSS reader Controller
- •13.7.4 Refactoring debrief
- •13.8 Summary
- •The Ajax craftsperson’s toolkit
- •A.1 Working smarter with the right toolset
- •A.1.1 Acquiring tools that fit
- •A.1.2 Building your own tools
- •A.1.3 Maintaining your toolkit
- •A.2 Editors and IDEs
- •A.2.1 What to look for in a code editor
- •A.2.2 Current offerings
- •A.3 Debuggers
- •A.3.1 Why we use a debugger
- •A.3.2 JavaScript debuggers
- •A.3.3 HTTP debuggers
- •A.3.4 Building your own cross-browser output console
- •A.4 DOM inspectors
- •A.4.1 Using the Mozilla DOM Inspector
- •A.4.2 DOM inspectors for Internet Explorer
- •A.4.3 The Safari DOM Inspector for Mac OS X
- •A.5 Installing Firefox extensions
- •A.6 Resources
- •JavaScript for object-oriented programmers
- •B.1 JavaScript is not Java
- •B.2 Objects in JavaScript
- •B.2.1 Building ad hoc objects
- •B.2.2 Constructor functions, classes, and prototypes
- •B.2.3 Extending built-in classes
- •B.2.4 Inheritance of prototypes
- •B.2.5 Reflecting on JavaScript objects
- •B.2.6 Interfaces and duck typing
- •B.3 Methods and functions
- •B.3.1 Functions as first-class citizens
- •B.3.2 Attaching functions to objects
- •B.3.3 Borrowing functions from other objects
- •B.3.4 Ajax event handling and function contexts
- •B.3.5 Closures in JavaScript
- •B.4 Conclusions
- •B.5 Resources
- •Ajax frameworks and libraries
- •Accesskey Underlining Library
- •ActiveWidgets
- •Ajax JavaServer Faces Framework
- •Ajax JSP Tag Library
- •Ajax.NET
- •AjaxAC
- •AjaxAspects
- •AjaxCaller
- •AjaxFaces
- •BackBase
- •Behaviour
- •Bindows
- •BlueShoes
- •CakePHP
- •CL-Ajax
- •ComfortASP.NET
- •Coolest DHTML Calendar
- •Dojo
- •DWR (Direct Web Remoting)
- •Echo 2
- •FCKEditor
- •Flash JavaScript Integration Kit
- •Google AjaxSLT
- •Guise
- •HTMLHttpRequest
- •Interactive Website Framework
- •Jackbe
- •JPSpan
- •jsolait
- •JSON
- •JSRS (JavaScript Remote Scripting)
- •LibXMLHttpRequest
- •Mochikit
- •netWindows
- •Oddpost
- •OpenRico
- •Pragmatic Objects
- •Prototype
- •Qooxdoo
- •RSLite
- •Ruby on Rails
- •Sack
- •SAJAX
- •Sarissa
- •Scriptaculous
- •SWATO…
- •Tibet
- •TinyMCE
- •TrimPath Templates
- •Walter Zorn’s DHTML Libraries
- •WebORB for .NET
- •WebORB for Java
- •XAJAX
- •x-Desktop
- •XHConn
- •index
- •Symbols
- •Numerics

Some small refactoring case studies |
77 |
|
|
3.2 Some small refactoring case studies
The following sections address some issues in Ajax development and look at some common solutions to them. In each case, we’ll show you how to refactor to ease the pain associated with that issue, and then we’ll identify the elements of the solution that can be reused elsewhere.
In keeping with an honorable tradition in design patterns literature, we will present each issue in terms of a problem, the technical solution, and then a discussion of the larger issues involved.
3.2.1Cross-browser inconsistencies: Façade and Adapter patterns
If you ask any web developers—be they coders, designers, graphics artists, or all- rounders—for their pet peeves in relation to their work, there’s a good chance that getting their work to display correctly on different browsers will be on their list. The Web is full of standards for technology, and most browser vendors implement most of the standards more or less completely most of the time. Sometimes the standards are vague and open to different interpretations, sometimes the browser vendors extended the standards in useful but inconsistent ways, and sometimes the browsers just have good old-fashioned bugs in them.
JavaScript coders have resorted since the early days to checking in their code which browser they’re using or to testing whether or not an object exists. Let’s take a very simple example.
Working with DOM elements
As we discussed in chapter 2, a web page is exposed to JavaScript through the Document Object Model (DOM), a tree-like structure whose elements correspond to the tags of an HTML document. When manipulating a DOM tree programmatically, it is quite common to want to find out an element’s position on the page. Unfortunately, browser vendors have provided various nonstandard methods for doing so over the years, making it difficult to write fail-safe crossbrowser code to accomplish the task. Listing 3.2—a simplified version of a function from Mike Foster’s x library (see section 3.5)—shows a comprehensive way of discovering the pixel position of the left edge of the DOM element e passed in as an argument.
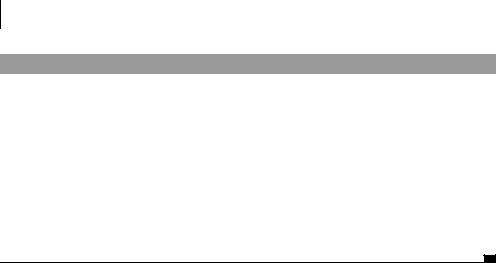
78CHAPTER 3
Introducing order to Ajax
Listing 3.2 getLeft() function
function getLeft(e){ if(!(e=xGetElementById(e))){
return 0;
}
var css=xDef(e.style);
if (css && xStr(e.style.left)) { iX=parseInt(e.style.left); if(isNaN(iX)) iX=0;
}else if(css && xDef(e.style.pixelLeft)) { iX=e.style.pixelLeft;
}
return iX;
}
Different browsers offer many ways of determining the position of the node via the style array that we encountered in chapter 2. The W3C CSS2 standard supports a property called style.left, defined as a string describing value and units, such as 100px. Units other than pixels may be supported. style.pixelLeft, in contrast, is numeric and assumes all values to be measured in pixels. pixelLeft is supported only in Microsoft Internet Explorer. The getLeft() method discussed here first checks that CSS is supported and then tests both values, trying the W3C standard first. If no values are found, then a value of zero is returned by default. Note that we don’t explicitly check for browser names or versions but use the more robust object-detection technique that we discussed in chapter 2.
Writing functions like these to accommodate cross-browser peculiarities is a tedious business, but once it is done, the developer can get on with developing the application without having to worry about these issues. And with well-tested libraries such as x, most of the hard work has already been done for us. Having a reliable adapter function for discovering the on-page position of a DOM element can speed up the development of an Ajax user interface considerably.
Making requests to the server
We’ve already come across another similar cross-browser incompatibility in chapter 2. Browser vendors have provided nonstandard mechanisms for obtaining the XMLHttpRequest object used to make asynchronous requests to the server. When we wanted to load an XML document from the server, we needed to figure out which of the possibilities to use.
Internet Explorer will only deliver the goods if we ask for an ActiveX component, whereas Mozilla and Safari will play nice if we ask for a native built-in object. Only the XML loading code itself knew about those differences. Once the
Some small refactoring case studies |
79 |
|
|
XMLHttpRequest object was returned into the rest of the code, it behaved identically in both cases. Calling code doesn’t need to understand either the ActiveX or the native object subsystem; it only needs to understand the
Loader() constructor.
The Façade pattern
For both getLeft() and new net.ContentLoader(), the code that does the object detection is ugly and tedious. By defining a function to hide it from the rest of our code, we are making the rest of the code easier to read and isolating the objectdetection code in a single place. This is a basic principle in refactoring—don’t repeat yourself, often abbreviated to DRY. If we discover an edge case that our object-detection code doesn’t handle properly, then fixing it once rolls that change out to all calls to discover the left coordinate of a DOM element, create an XML Request object, or whatever else we are trying to do.
In the language of design patterns, we are using a pattern known as Façade. Façade is a pattern used to provide a common access point to different implementations of a service or piece of functionality. The XMLHttpRequest object, for example, offers a useful service, and our application doesn’t really care how it is delivered as long as it works (figure 3.1).
In many cases, we also want to simplify access to a subsystem. In the case of getting the left-edge coordinate of a DOM element, for example, the CSS spec provided us with a plethora of choices, allowing the value to be specified in pixels, points, ems, and other units. This freedom of expression may be more than we need. The getLeft() function in listing 3.2 will work as long as we are using pixels as the unit throughout our layout system. Simplifying the subsystem in this way is another feature of the Façade pattern.
The Adapter pattern
A closely related pattern is Adapter. In Adapter, we also work with two subsystems that perform the same function, such as the Microsoft and Mozilla approaches to getting an XMLHttpRequest object. Rather than constructing a new Façade for each to use, as we did earlier, we provide an extra layer over one of the subsystems that presents the same API as the other subsystem. This layer is known as the Adapter. The Sarissa XML library for Ajax, which we will discuss in section 3.5.1, uses the Adapter pattern to make Internet Explorer’s ActiveX control look like the Mozilla built-in XMLHttpRequest. Both approaches are valid and can help to integrate legacy or third-party code (including the browsers themselves) into your Ajax project.
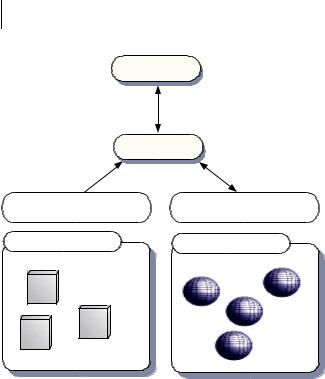
80CHAPTER 3
Introducing order to Ajax
Calling code
loadXML() function
Implicit XMLHttpRequest interface |
Implicit XMLHttpRequest interface |
Native XMLHttpRequest |
ActiveX XMLHttpRequest |
Figure 3.1 Schematic of the Façade pattern, as it relates to the XMLHttpRequest object across browsers. The loadXML() function requires an XMLHttpRequest object, but doesn't care about its actual implementation. Underlying implementations may offer considerably more complex HTTP Request semantics, but both are simplified here to provide the basic functionality required by the calling function.
Let’s move on to the next case study, in which we consider issues with JavaScript’s event-handling model.
3.2.2Managing event handlers: Observer pattern
We can’t write very much Ajax code without coming across event-based programming techniques. JavaScript user interfaces are heavily event-driven, and the introduction of asynchronous requests with Ajax adds a further set of callbacks and events for our application to deal with. In a relatively simple application, an event such as a mouse click or the arrival of data from the server can be handled by a single function. As an application grows in size and complexity, though, we may want to notify several distinct subsystems and even to expose a mechanism whereby interested parties can sign themselves up for such notification. Let’s explore an example to see what the issues are.
Some small refactoring case studies |
81 |
|
|
Using multiple event handlers
It’s common practice when scripting DOM nodes using JavaScript to define the script in the window.onload function, which is executed after the page (and therefore the DOM tree) is fully loaded. Let’s say that we have a DOM element on our page that will display dynamically generated data fetched from the server at regular intervals once the page is loaded. The JavaScript that coordinates the data fetching and the display needs a reference to the DOM node, so it gets it by defining a window.onload event:
window.onload=function(){
displayDiv=document.getElementById('display');
}
All well and good. Let’s say that we now want to add a second visual display that provides alerts from a news feed, for example (see chapter 13 if you’re interested in implementing this functionality). The code that controls the news feed display also needs to grab references to some DOM elements on startup. So it defines a window.onload event handler, too:
window.onload=function(){
feedDiv=document.getElementById('feeds');
}
We test both sets of code on separate pages and find them both to work fine. When we put them together, the second window.onload function overwrites the first, and the data feed fails to display and starts to generate JavaScript errors. The problem lies in the fact that the window object allows only a single onload function to be attached to it.
Limitations of a composite event handler
Our second event handler overrides the first one. We can get around this by writing a single composite function:
window.onload=function(){
displayDiv=document.getElementById('display');
feedDiv=document.getElementById('feeds');
}
This works for our current example, but it tangles together code from the data display and the news feed viewer, which are otherwise unrelated to each other. If we were dealing with 10 or 20 systems rather than 2, and each needed to get references to several DOM elements, then a composite event handler like this would become hard to maintain. Swapping individual components in and out would become difficult and error prone, leading to exactly the sort of situation that we

82CHAPTER 3
Introducing order to Ajax
described in the introduction, where nobody wants to touch the code in case it should break. Let’s try to refactor a little further, by defining a loader function for each subsystem:
window.onload=function(){
getDisplayElements();
getFeedElements();
}
function getDisplayElements(){ displayDiv=document.getElementById('display');
}
function getFeedElements(){ feedDiv=document.getElementById('feeds');
}
This introduces some clarity, reducing our composite window.onload() to a single line for each subsystem, but the composite function is still a weak point in the design and is likely to cause us trouble. In the following section, we’ll examine a slightly more complex but more scalable solution to the problem.
The Observer pattern
It can be helpful sometimes to ask where the responsibility for an action lies. The composite function approach places responsibility for getting the references to DOM elements on the window object, which then has to know which subsystems are present in the current page. Ideally, each subsystem should be responsible for acquiring its own references. That way, if it is present on a page, it will get them, and if it isn’t present, it won’t.
To set the division of responsibility straight, we can allow systems to register for notification of the onload event happening by passing a function to call when the window.onload event is fired. Here’s a simple implementation:
window.onloadListeners=new Array(); window.addOnLoadListener(listener){
window.onloadListeners[window.onloadListeners.length]=listener;
}
When the window is fully loaded, then the window object need only iterate through its array of listeners and call each one in turn:
window.onload=function(){
for(var i=0;i<window.onloadListeners.length;i++){ var func=window.onlloadListeners[i]; func.call();
}
}