
- •preface
- •acknowledgments
- •about this book
- •Who should read this book?
- •Roadmap
- •Code conventions
- •Code downloads
- •Author Online
- •About the title
- •About the cover illustration
- •Rethinking the web application
- •A new design for the Web
- •1.1 Why Ajax rich clients?
- •1.1.1 Comparing the user experiences
- •1.1.2 Network latency
- •1.1.3 Asynchronous interactions
- •1.1.4 Sovereign and transient usage patterns
- •1.1.5 Unlearning the Web
- •1.2 The four defining principles of Ajax
- •1.2.1 The browser hosts an application, not content
- •1.2.2 The server delivers data, not content
- •1.2.3 User interaction with the application can be fluid and continuous
- •1.2.4 This is real coding and requires discipline
- •1.3 Ajax rich clients in the real world
- •1.3.1 Surveying the field
- •1.3.2 Google Maps
- •1.4 Alternatives to Ajax
- •1.4.2 Java Web Start and related technologies
- •1.5 Summary
- •1.6 Resources
- •First steps with Ajax
- •2.1 The key elements of Ajax
- •2.2 Orchestrating the user experience with JavaScript
- •2.3 Defining look and feel using CSS
- •2.3.1 CSS selectors
- •2.3.2 CSS style properties
- •2.3.3 A simple CSS example
- •2.4 Organizing the view using the DOM
- •2.4.1 Working with the DOM using JavaScript
- •2.4.2 Finding a DOM node
- •2.4.3 Creating a DOM node
- •2.4.4 Adding styles to your document
- •2.4.5 A shortcut: Using the innerHTML property
- •2.5 Loading data asynchronously using XML technologies
- •2.5.1 IFrames
- •2.5.2 XmlDocument and XMLHttpRequest objects
- •2.5.3 Sending a request to the server
- •2.5.4 Using callback functions to monitor the request
- •2.5.5 The full lifecycle
- •2.6 What sets Ajax apart
- •2.7 Summary
- •2.8 Resources
- •Introducing order to Ajax
- •3.1 Order out of chaos
- •3.1.1 Patterns: creating a common vocabulary
- •3.1.2 Refactoring and Ajax
- •3.1.3 Keeping a sense of proportion
- •3.1.4 Refactoring in action
- •3.2 Some small refactoring case studies
- •3.2.2 Managing event handlers: Observer pattern
- •3.2.3 Reusing user action handlers: Command pattern
- •3.2.4 Keeping only one reference to a resource: Singleton pattern
- •3.3 Model-View-Controller
- •3.4 Web server MVC
- •3.4.1 The Ajax web server tier without patterns
- •3.4.2 Refactoring the domain model
- •3.4.3 Separating content from presentation
- •3.5 Third-party libraries and frameworks
- •3.5.2 Widgets and widget suites
- •3.5.3 Application frameworks
- •3.6 Summary
- •3.7 Resources
- •Core techniques
- •The page as an application
- •4.1 A different kind of MVC
- •4.1.1 Repeating the pattern at different scales
- •4.1.2 Applying MVC in the browser
- •4.2 The View in an Ajax application
- •4.2.1 Keeping the logic out of the View
- •4.2.2 Keeping the View out of the logic
- •4.3 The Controller in an Ajax application
- •4.3.1 Classic JavaScript event handlers
- •4.3.2 The W3C event model
- •4.3.3 Implementing a flexible event model in JavaScript
- •4.4 Models in an Ajax application
- •4.4.1 Using JavaScript to model the business domain
- •4.4.2 Interacting with the server
- •4.5 Generating the View from the Model
- •4.5.1 Reflecting on a JavaScript object
- •4.5.2 Dealing with arrays and objects
- •4.5.3 Adding a Controller
- •4.6 Summary
- •4.7 Resources
- •The role of the server
- •5.1 Working with the server side
- •5.2 Coding the server side
- •5.2.1 Popular implementation languages
- •5.3 The big picture: common server-side designs
- •5.3.1 Naive web server coding without a framework
- •5.3.2 Working with Model2 workflow frameworks
- •5.4 The details: exchanging data
- •5.4.2 Introducing the planet browser example
- •5.5 Writing to the server
- •5.5.1 Using HTML forms
- •5.5.2 Using the XMLHttpRequest object
- •5.5.3 Managing user updates effectively
- •5.6 Summary
- •5.7 Resources
- •Professional Ajax
- •The user experience
- •6.1 Getting it right: building a quality application
- •6.1.1 Responsiveness
- •6.1.2 Robustness
- •6.1.3 Consistency
- •6.1.4 Simplicity
- •6.1.5 Making it work
- •6.2 Keeping the user informed
- •6.2.1 Handling responses to our own requests
- •6.2.2 Handling updates from other users
- •6.3 Designing a notification system for Ajax
- •6.3.1 Modeling notifications
- •6.3.2 Defining user interface requirements
- •6.4 Implementing a notification framework
- •6.4.1 Rendering status bar icons
- •6.4.2 Rendering detailed notifications
- •6.4.3 Putting the pieces together
- •6.5 Using the framework with network requests
- •6.6 Indicating freshness of data
- •6.6.1 Defining a simple highlighting style
- •6.6.2 Highlighting with the Scriptaculous Effects library
- •6.7 Summary
- •6.8 Resources
- •Security and Ajax
- •7.1 JavaScript and browser security
- •7.1.1 Introducing the “server of origin” policy
- •7.1.2 Considerations for Ajax
- •7.1.3 Problems with subdomains
- •7.2 Communicating with remote services
- •7.2.1 Proxying remote services
- •7.2.2 Working with web services
- •7.3 Protecting confidential data
- •7.3.1 The man in the middle
- •7.3.2 Using secure HTTP
- •7.3.3 Encrypting data over plain HTTP using JavaScript
- •7.4 Policing access to Ajax data streams
- •7.4.1 Designing a secure web tier
- •7.4.2 Restricting access to web data
- •7.5 Summary
- •7.6 Resources
- •Performance
- •8.1 What is performance?
- •8.2 JavaScript execution speed
- •8.2.1 Timing your application the hard way
- •8.2.2 Using the Venkman profiler
- •8.2.3 Optimizing execution speed for Ajax
- •8.3 JavaScript memory footprint
- •8.3.1 Avoiding memory leaks
- •8.3.2 Special considerations for Ajax
- •8.4 Designing for performance
- •8.4.1 Measuring memory footprint
- •8.4.2 A simple example
- •8.5 Summary
- •8.6 Resources
- •Ajax by example
- •Dynamic double combo
- •9.1 A double-combo script
- •9.2 The client-side architecture
- •9.2.1 Designing the form
- •9.2.2 Designing the client/server interactions
- •9.3 Implementing the server: VB .NET
- •9.3.1 Defining the XML response format
- •9.4 Presenting the results
- •9.4.1 Navigating the XML document
- •9.4.2 Applying Cascading Style Sheets
- •9.5 Advanced issues
- •9.5.2 Moving from a double combo to a triple combo
- •9.6 Refactoring
- •9.6.1 New and improved net.ContentLoader
- •9.7 Summary
- •Type-ahead suggest
- •10.1 Examining type-ahead applications
- •10.1.2 Google Suggest
- •10.2.1 The server and the database
- •10.3 The client-side framework
- •10.3.1 The HTML
- •10.3.2 The JavaScript
- •10.3.3 Accessing the server
- •10.5 Refactoring
- •10.5.1 Day 1: developing the TextSuggest component game plan
- •10.5.3 Day 3: Ajax enabled
- •10.5.4 Day 4: handling events
- •10.5.6 Refactor debriefing
- •10.6 Summary
- •11.1 The evolving portal
- •11.1.1 The classic portal
- •11.1.2 The rich user interface portal
- •11.2 The Ajax portal architecture using Java
- •11.3 The Ajax login
- •11.3.1 The user table
- •11.4 Implementing DHTML windows
- •11.4.1 The portal windows database
- •11.4.3 Adding the JS external library
- •11.5 Adding Ajax autosave functionality
- •11.5.1 Adapting the library
- •11.5.2 Autosaving the information to the database
- •11.6 Refactoring
- •11.6.1 Defining the constructor
- •11.6.2 Adapting the AjaxWindows.js library
- •11.6.3 Specifying the portal commands
- •11.6.4 Performing the Ajax processing
- •11.6.5 Refactoring debrief
- •11.7 Summary
- •Live search using XSLT
- •12.1 Understanding the search techniques
- •12.1.1 Looking at the classic search
- •12.1.3 Examining a live search with Ajax and XSLT
- •12.1.4 Sending the results back to the client
- •12.2 The client-side code
- •12.2.1 Setting up the client
- •12.2.2 Initiating the process
- •12.3 The server-side code: PHP
- •12.3.1 Building the XML document
- •12.3.2 Building the XSLT document
- •12.4 Combining the XSLT and XML documents
- •12.4.1 Working with Microsoft Internet Explorer
- •12.4.2 Working with Mozilla
- •12.5 Completing the search
- •12.5.1 Applying a Cascading Style Sheet
- •12.5.2 Improving the search
- •12.5.3 Deciding to use XSLT
- •12.5.4 Overcoming the Ajax bookmark pitfall
- •12.6 Refactoring
- •12.6.1 An XSLTHelper
- •12.6.2 A live search component
- •12.6.3 Refactoring debriefing
- •12.7 Summary
- •Building stand-alone applications with Ajax
- •13.1 Reading information from the outside world
- •13.1.1 Discovering XML feeds
- •13.1.2 Examining the RSS structure
- •13.2 Creating the rich user interface
- •13.2.1 The process
- •13.2.3 Compliant CSS formatting
- •13.3 Loading the RSS feeds
- •13.3.1 Global scope
- •13.3.2 Ajax preloading functionality
- •13.4 Adding a rich transition effect
- •13.4.2 Implementing the fading transition
- •13.4.3 Integrating JavaScript timers
- •13.5 Additional functionality
- •13.5.1 Inserting additional feeds
- •13.5.2 Integrating the skipping and pausing functionality
- •13.6 Avoiding the project’s restrictions
- •13.6.1 Overcoming Mozilla’s security restriction
- •13.6.2 Changing the application scope
- •13.7 Refactoring
- •13.7.1 RSS reader Model
- •13.7.2 RSS reader view
- •13.7.3 RSS reader Controller
- •13.7.4 Refactoring debrief
- •13.8 Summary
- •The Ajax craftsperson’s toolkit
- •A.1 Working smarter with the right toolset
- •A.1.1 Acquiring tools that fit
- •A.1.2 Building your own tools
- •A.1.3 Maintaining your toolkit
- •A.2 Editors and IDEs
- •A.2.1 What to look for in a code editor
- •A.2.2 Current offerings
- •A.3 Debuggers
- •A.3.1 Why we use a debugger
- •A.3.2 JavaScript debuggers
- •A.3.3 HTTP debuggers
- •A.3.4 Building your own cross-browser output console
- •A.4 DOM inspectors
- •A.4.1 Using the Mozilla DOM Inspector
- •A.4.2 DOM inspectors for Internet Explorer
- •A.4.3 The Safari DOM Inspector for Mac OS X
- •A.5 Installing Firefox extensions
- •A.6 Resources
- •JavaScript for object-oriented programmers
- •B.1 JavaScript is not Java
- •B.2 Objects in JavaScript
- •B.2.1 Building ad hoc objects
- •B.2.2 Constructor functions, classes, and prototypes
- •B.2.3 Extending built-in classes
- •B.2.4 Inheritance of prototypes
- •B.2.5 Reflecting on JavaScript objects
- •B.2.6 Interfaces and duck typing
- •B.3 Methods and functions
- •B.3.1 Functions as first-class citizens
- •B.3.2 Attaching functions to objects
- •B.3.3 Borrowing functions from other objects
- •B.3.4 Ajax event handling and function contexts
- •B.3.5 Closures in JavaScript
- •B.4 Conclusions
- •B.5 Resources
- •Ajax frameworks and libraries
- •Accesskey Underlining Library
- •ActiveWidgets
- •Ajax JavaServer Faces Framework
- •Ajax JSP Tag Library
- •Ajax.NET
- •AjaxAC
- •AjaxAspects
- •AjaxCaller
- •AjaxFaces
- •BackBase
- •Behaviour
- •Bindows
- •BlueShoes
- •CakePHP
- •CL-Ajax
- •ComfortASP.NET
- •Coolest DHTML Calendar
- •Dojo
- •DWR (Direct Web Remoting)
- •Echo 2
- •FCKEditor
- •Flash JavaScript Integration Kit
- •Google AjaxSLT
- •Guise
- •HTMLHttpRequest
- •Interactive Website Framework
- •Jackbe
- •JPSpan
- •jsolait
- •JSON
- •JSRS (JavaScript Remote Scripting)
- •LibXMLHttpRequest
- •Mochikit
- •netWindows
- •Oddpost
- •OpenRico
- •Pragmatic Objects
- •Prototype
- •Qooxdoo
- •RSLite
- •Ruby on Rails
- •Sack
- •SAJAX
- •Sarissa
- •Scriptaculous
- •SWATO…
- •Tibet
- •TinyMCE
- •TrimPath Templates
- •Walter Zorn’s DHTML Libraries
- •WebORB for .NET
- •WebORB for Java
- •XAJAX
- •x-Desktop
- •XHConn
- •index
- •Symbols
- •Numerics

Generating the View from the Model |
151 |
|
|
iterating over the object in the autoSpec() function. The objViewSpec property is a numerical array, with each element being a lookup table of properties. For now, we’re only concerned with generating the name property. The PropertyViewer is passed the spec for this property in its constructor and can take hints from the spec as to how it should render itself.
If we provide a specification property to an object that we want to inspect in the ObjectViewer, then we can limit the properties being displayed to those that we think are relevant.
A second problem with our ObjectViewer is that it doesn’t handle complex properties very well. When objects, arrays, and functions are appended to a string, the toString() method is called. In the case of an object, this generally returns something nondescriptive such as [Object object]. In the case of a Function object, the entire source code for the function is returned. We need to discriminate between the different types of properties, which we can do using the instanceof operator. With that in place, let’s see how we can improve on our viewer.
4.5.2Dealing with arrays and objects
One way of handling arrays and objects is to allow the user to drill down into them using separate ObjectViewer objects for each property. There are several ways of representing this. We have chosen here to represent child objects as popout windows, somewhat like a hierarchical menu.
To achieve this, we need to do two things. First, we need to add a type property to the object specification and define the types that we support:
objviewer.TYPE_SIMPLE="simple"; objviewer.TYPE_ARRAY="array"; objviewer.TYPE_FUNCTION="function"; objviewer.TYPE_IMAGE_URL="image url"; objviewer.TYPE_OBJECT="object";
We modify the function that generates specs for objects that don’t come with their own to take account of the type, as shown in listing 4.13.
Listing 4.13 Modified autoSpec() function
objviewer.autoSpec=function(obj){ var members=new Array();
for (var propName in obj){ var propValue=obj[name];
var propType=objviewer.autoType(value); var spec={name:propName,type:propType};

152CHAPTER 4
The page as an application
members.append(spec);
}
if (obj && obj.length>0){ for(var i=0;i<obj.length;i++){
var propName="array ["+i+"]"; var propValue=obj[i];
var propType=objviewer.ObjectViewer.autoType(value); var spec={name:propName,type:propType}; members.append(spec);
}
}
return members;
}
objviewer.autoType=function(value){ var type=objviewer.TYPE_SIMPLE; if ((value instanceof Array)){
type=objviewer.TYPE_ARRAY;
}else if (value instanceof Function){ type=objviewer.TYPE_FUNCTION;
}else if (value instanceof Object){ type=objviewer.TYPE_OBJECT;
}
return type;
}
Note that we also add support for numerically indexed arrays, whose elements wouldn’t be discovered by the for...in style of loop.
The second thing that we need to do is to modify the PropertyViewer to take account of the different types and render them accordingly, as shown in listing 4.14.
Listing 4.14 Modified PropertyViewer constructor
objviewer.PropertyViewer=function
(objectViewer,memberSpec,appendAtTop){
this.objectViewer=objectViewer;
this.spec=memberSpec;
this.name=this.spec.name;
this.type=this.spec.type;
this.value=objectViewer.object[this.name];
this.rowTr=document.createElement("tr");
this.rowTr.className='objViewRow';
var isComplexType=(this.type==objviewer.TYPE_ARRAY ||this.type==objviewer.TYPE_OBJECT);
if ( !(isComplexType && this.objectViewer.isInline
)
){
this.nameTd=this.renderSideHeader();

Generating the View from the Model |
153 |
|
|
this.rowTr.appendChild(this.nameTd);
}
this.valTd=document.createElement("td");
this.valTd.className='objViewValue';
this.valTd.viewer=this;
this.rowTr.appendChild(this.valTd); if (isComplexType){
if (this.viewer.isInline){ this.valTd.colSpan=2;
var nameDiv=this.renderTopHeader(); this.valTd.appendChild(nameDiv);
var valDiv=this.renderInlineObject(); this.valTd.appendChild(valDiv);
}else{
var valDiv=this.renderPopoutObject(); this.valTd.appendChild(valDiv);
}
}else if (this.type==objviewer.TYPE_IMAGE_URL){ var valImg=this.renderImage(); this.valTd.appendChild(valImg);
}else if (this.type==objviewer.TYPE_SIMPLE){ var valTxt=this.renderSimple(); this.valTd.appendChild(valTxt);
}
if (appendAtTop){ styling.insertAtTop(viewer.tbod,this.rowTr);
}else{
viewer.tbod.appendChild(this.rowTr);
}
}
To accommodate the various types of properties, we have defined a number of rendering methods, the implementation of which is too detailed to reproduce in full here. Source code for the entire ObjectViewer can be downloaded from the website that accompanies this book.
We now have a fairly complete way of viewing our domain model automatically. To make the domain model objects visible, all that we need to do is to assign objViewSpec properties to their prototypes. The Planet object backing the view shown in figure 4.7, for example, has the following statement in the constructor:
this.objViewSpec=[ |
|
|
{name:"name", |
type:"simple"}, |
|
{name:"distance", |
type:"simple", |
editable:true}, |
{name:"diameter", |
type:"simple", |
editable:true}, |
{name:"image", |
type:"image url"}, |
|
{name:"facts", |
type:"array", |
addNew:this.newFact, inline:true } |
]; |
|
|
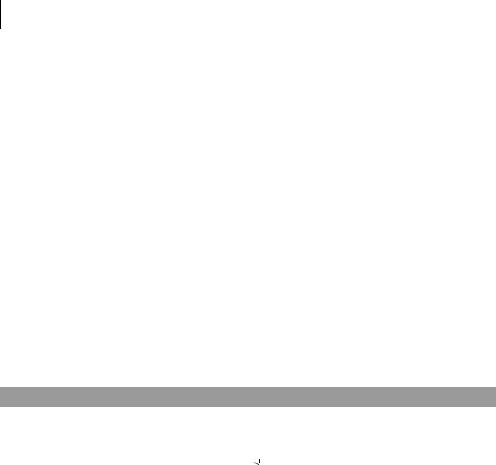
154CHAPTER 4
The page as an application
The notation for this specification is the JavaScript object notation, known as JSON. Square braces indicate a numerical array, and curly braces an associative array or object (the two are really the same). We discuss JSON more fully in appendix B.
There are a few unexplained entries here. What do addNew, inline, and editable mean? Their purpose is to notify the View that these parts of the domain model can not only be inspected but also modified by the user, bringing in the Controller aspects of our system, too. We’ll look at this in the next section.
4.5.3Adding a Controller
It’s nice to be able to look at a domain model, but many everyday applications require us to modify them too—download the tune, edit the document, add items to the shopping basket, and so on. Mediating between user interactions and the domain model is the responsibility of the Controller, and we’ll now add that functionality to our ObjectViewer.
The first thing that we’d like to do is to be able to edit simple text values when we click on them, if our specification object flags them as being editable. Listing 4.15 shows the code used to render a simple text property.
Listing 4.15 renderSimple() function
objviewer.PropertyViewer.prototype.renderSimple=function(){ var valDiv=document.createElement("div");
var valTxt=document
.createTextNode(this.value); |
|
Show read-only value |
|
|
|||
valDiv.appendChild(valTxt); |
b Add interactivity if editable |
||
if (this.spec.editable){ |
valDiv.className+=" editable";
|
valDiv.viewer=this; |
|
|
valDiv.onclick=objviewer.PropertyViewer.editSimpleProperty; |
|
} |
|
|
return valDiv; |
|
|
} |
|
c Begin editing |
objviewer.PropertyViewer.editSimpleProperty=function(e){ |
||
var |
viewer=this.viewer; |
|
if (viewer){ viewer.edit();
}
}
objviewer.PropertyViewer.prototype.edit=function(){ if (this.type=objviewer.TYPE_SIMPLE){
var editor=document.createElement("input"); editor.value=this.value; document.body.appendChild(editor);

Generating the View from the Model |
155 |
|
|
var td=this.valTd; xLeft(editor,xLeft(td)); xTop(editor,xTop(td)); xWidth(editor,xWidth(td)); xHeight(editor,xHeight(td));
td.replaceChild(editor,td.firstChild); d Replace with read/write view editor.onblur=objviewer.
PropertyViewer.editBlur; |
e Add commit callback |
|
editor.viewer=this; |
|
|
editor.focus(); |
|
|
} |
|
|
} |
|
|
objviewer.PropertyViewer |
f Finish editing |
|
.editBlur=function(e){ |
||
var viewer=this.viewer; |
|
|
if (viewer){ viewer.commitEdit(this.value);
}
}
objviewer.PropertyViewer.prototype.commitEdit=function(value){ if (this.type==objviewer.TYPE_SIMPLE){
this.value=value;
var valDiv=this.renderSimple(); var td=this.valTd;
td.replaceChild(valDiv,td.firstChild);
this.objectViewer |
g Notify observers |
.notifyChange(this); |
}
}
Editing a property involves several steps. First, we want to assign an onclick handler to the DOM element displaying the value, if the field is editable b. We also assign a specific CSS classname to editable fields, which will make them change color when the mouse hovers over them. We need the user to be able to realize that she can edit the field, after all.
editSimpleProperty() cis a simple event handler that retrieves the reference to the PropertyViewer from the clicked DOM node and calls the edit() method. This way of connecting the View and Controller should be familiar from section 4.3.1. We check that the property type is correct and then replace the read-only label with an equivalent-sized HTML form text input, containing the value d. We also attach an onblur handler to this text area e, which replaces the editable area with a read-only label fand updates the domain model.
We can manipulate the domain model in this way, but in general, we would often like to take some other action when the model is updated. The notifyChange()

156CHAPTER 4
The page as an application
method of the ObjectViewer g, invoked in the commitEdit() function, comes into play here. Listing 4.16 shows this function in full.
Listing 4.16 ObjectViewer.notifyChange()
objviewer.ObjectViewer.prototype
.notifyChange=function(propViewer){ if (this.onchangeRouter){
this.onchangeRouter.notify(propViewer);
}
if (this.parentObjViewer){ this.parentObjViewer.notifyChange(propViewer);
}
}
objviewer.ObjectViewer.prototype
.addChangeListener=function(lsnr){ if (!this.onchangeRouter){
this.onchangeRouter=new jsEvent.EventRouter(this,"onchange");
}
this.onchangeRouter.addListener(lsnr);
}
objviewer.ObjectViewer.prototype
.removeChangeListener=function(lsnr){ if (this.onchangeRouter){
this.onchangeRouter.removeListener(lsnr);
}
}
The problem we are facing—notifying arbitrary processes of a change in our domain model—is ideally solved by the Observer pattern and the EventRouter object that we defined in section 4.3.3. We could attach an EventRouter to the onblur event of the editable fields, but a complex model may contain many of these, and our code shouldn’t have visibility of such fine details in the ObjectViewer implementation.
Instead, we define our own event type on the ObjectViewer itself, an onchange event, and attach an EventRouter to that. Because our ObjectViewers are arranged in a tree structure when drilling down on object and array properties, we pass onchange events to the parent, recursively. Thus, in general, we can attach listeners to the root ObjectViewer, the one that we create in our application code, and changes to model properties several layers down the object graph will propagate back up to us.
A simple example of an event handler would be to write a message to the browser status bar. The top-level object in a model of planets is the solar system, so we can write