
- •preface
- •acknowledgments
- •about this book
- •Who should read this book?
- •Roadmap
- •Code conventions
- •Code downloads
- •Author Online
- •About the title
- •About the cover illustration
- •Rethinking the web application
- •A new design for the Web
- •1.1 Why Ajax rich clients?
- •1.1.1 Comparing the user experiences
- •1.1.2 Network latency
- •1.1.3 Asynchronous interactions
- •1.1.4 Sovereign and transient usage patterns
- •1.1.5 Unlearning the Web
- •1.2 The four defining principles of Ajax
- •1.2.1 The browser hosts an application, not content
- •1.2.2 The server delivers data, not content
- •1.2.3 User interaction with the application can be fluid and continuous
- •1.2.4 This is real coding and requires discipline
- •1.3 Ajax rich clients in the real world
- •1.3.1 Surveying the field
- •1.3.2 Google Maps
- •1.4 Alternatives to Ajax
- •1.4.2 Java Web Start and related technologies
- •1.5 Summary
- •1.6 Resources
- •First steps with Ajax
- •2.1 The key elements of Ajax
- •2.2 Orchestrating the user experience with JavaScript
- •2.3 Defining look and feel using CSS
- •2.3.1 CSS selectors
- •2.3.2 CSS style properties
- •2.3.3 A simple CSS example
- •2.4 Organizing the view using the DOM
- •2.4.1 Working with the DOM using JavaScript
- •2.4.2 Finding a DOM node
- •2.4.3 Creating a DOM node
- •2.4.4 Adding styles to your document
- •2.4.5 A shortcut: Using the innerHTML property
- •2.5 Loading data asynchronously using XML technologies
- •2.5.1 IFrames
- •2.5.2 XmlDocument and XMLHttpRequest objects
- •2.5.3 Sending a request to the server
- •2.5.4 Using callback functions to monitor the request
- •2.5.5 The full lifecycle
- •2.6 What sets Ajax apart
- •2.7 Summary
- •2.8 Resources
- •Introducing order to Ajax
- •3.1 Order out of chaos
- •3.1.1 Patterns: creating a common vocabulary
- •3.1.2 Refactoring and Ajax
- •3.1.3 Keeping a sense of proportion
- •3.1.4 Refactoring in action
- •3.2 Some small refactoring case studies
- •3.2.2 Managing event handlers: Observer pattern
- •3.2.3 Reusing user action handlers: Command pattern
- •3.2.4 Keeping only one reference to a resource: Singleton pattern
- •3.3 Model-View-Controller
- •3.4 Web server MVC
- •3.4.1 The Ajax web server tier without patterns
- •3.4.2 Refactoring the domain model
- •3.4.3 Separating content from presentation
- •3.5 Third-party libraries and frameworks
- •3.5.2 Widgets and widget suites
- •3.5.3 Application frameworks
- •3.6 Summary
- •3.7 Resources
- •Core techniques
- •The page as an application
- •4.1 A different kind of MVC
- •4.1.1 Repeating the pattern at different scales
- •4.1.2 Applying MVC in the browser
- •4.2 The View in an Ajax application
- •4.2.1 Keeping the logic out of the View
- •4.2.2 Keeping the View out of the logic
- •4.3 The Controller in an Ajax application
- •4.3.1 Classic JavaScript event handlers
- •4.3.2 The W3C event model
- •4.3.3 Implementing a flexible event model in JavaScript
- •4.4 Models in an Ajax application
- •4.4.1 Using JavaScript to model the business domain
- •4.4.2 Interacting with the server
- •4.5 Generating the View from the Model
- •4.5.1 Reflecting on a JavaScript object
- •4.5.2 Dealing with arrays and objects
- •4.5.3 Adding a Controller
- •4.6 Summary
- •4.7 Resources
- •The role of the server
- •5.1 Working with the server side
- •5.2 Coding the server side
- •5.2.1 Popular implementation languages
- •5.3 The big picture: common server-side designs
- •5.3.1 Naive web server coding without a framework
- •5.3.2 Working with Model2 workflow frameworks
- •5.4 The details: exchanging data
- •5.4.2 Introducing the planet browser example
- •5.5 Writing to the server
- •5.5.1 Using HTML forms
- •5.5.2 Using the XMLHttpRequest object
- •5.5.3 Managing user updates effectively
- •5.6 Summary
- •5.7 Resources
- •Professional Ajax
- •The user experience
- •6.1 Getting it right: building a quality application
- •6.1.1 Responsiveness
- •6.1.2 Robustness
- •6.1.3 Consistency
- •6.1.4 Simplicity
- •6.1.5 Making it work
- •6.2 Keeping the user informed
- •6.2.1 Handling responses to our own requests
- •6.2.2 Handling updates from other users
- •6.3 Designing a notification system for Ajax
- •6.3.1 Modeling notifications
- •6.3.2 Defining user interface requirements
- •6.4 Implementing a notification framework
- •6.4.1 Rendering status bar icons
- •6.4.2 Rendering detailed notifications
- •6.4.3 Putting the pieces together
- •6.5 Using the framework with network requests
- •6.6 Indicating freshness of data
- •6.6.1 Defining a simple highlighting style
- •6.6.2 Highlighting with the Scriptaculous Effects library
- •6.7 Summary
- •6.8 Resources
- •Security and Ajax
- •7.1 JavaScript and browser security
- •7.1.1 Introducing the “server of origin” policy
- •7.1.2 Considerations for Ajax
- •7.1.3 Problems with subdomains
- •7.2 Communicating with remote services
- •7.2.1 Proxying remote services
- •7.2.2 Working with web services
- •7.3 Protecting confidential data
- •7.3.1 The man in the middle
- •7.3.2 Using secure HTTP
- •7.3.3 Encrypting data over plain HTTP using JavaScript
- •7.4 Policing access to Ajax data streams
- •7.4.1 Designing a secure web tier
- •7.4.2 Restricting access to web data
- •7.5 Summary
- •7.6 Resources
- •Performance
- •8.1 What is performance?
- •8.2 JavaScript execution speed
- •8.2.1 Timing your application the hard way
- •8.2.2 Using the Venkman profiler
- •8.2.3 Optimizing execution speed for Ajax
- •8.3 JavaScript memory footprint
- •8.3.1 Avoiding memory leaks
- •8.3.2 Special considerations for Ajax
- •8.4 Designing for performance
- •8.4.1 Measuring memory footprint
- •8.4.2 A simple example
- •8.5 Summary
- •8.6 Resources
- •Ajax by example
- •Dynamic double combo
- •9.1 A double-combo script
- •9.2 The client-side architecture
- •9.2.1 Designing the form
- •9.2.2 Designing the client/server interactions
- •9.3 Implementing the server: VB .NET
- •9.3.1 Defining the XML response format
- •9.4 Presenting the results
- •9.4.1 Navigating the XML document
- •9.4.2 Applying Cascading Style Sheets
- •9.5 Advanced issues
- •9.5.2 Moving from a double combo to a triple combo
- •9.6 Refactoring
- •9.6.1 New and improved net.ContentLoader
- •9.7 Summary
- •Type-ahead suggest
- •10.1 Examining type-ahead applications
- •10.1.2 Google Suggest
- •10.2.1 The server and the database
- •10.3 The client-side framework
- •10.3.1 The HTML
- •10.3.2 The JavaScript
- •10.3.3 Accessing the server
- •10.5 Refactoring
- •10.5.1 Day 1: developing the TextSuggest component game plan
- •10.5.3 Day 3: Ajax enabled
- •10.5.4 Day 4: handling events
- •10.5.6 Refactor debriefing
- •10.6 Summary
- •11.1 The evolving portal
- •11.1.1 The classic portal
- •11.1.2 The rich user interface portal
- •11.2 The Ajax portal architecture using Java
- •11.3 The Ajax login
- •11.3.1 The user table
- •11.4 Implementing DHTML windows
- •11.4.1 The portal windows database
- •11.4.3 Adding the JS external library
- •11.5 Adding Ajax autosave functionality
- •11.5.1 Adapting the library
- •11.5.2 Autosaving the information to the database
- •11.6 Refactoring
- •11.6.1 Defining the constructor
- •11.6.2 Adapting the AjaxWindows.js library
- •11.6.3 Specifying the portal commands
- •11.6.4 Performing the Ajax processing
- •11.6.5 Refactoring debrief
- •11.7 Summary
- •Live search using XSLT
- •12.1 Understanding the search techniques
- •12.1.1 Looking at the classic search
- •12.1.3 Examining a live search with Ajax and XSLT
- •12.1.4 Sending the results back to the client
- •12.2 The client-side code
- •12.2.1 Setting up the client
- •12.2.2 Initiating the process
- •12.3 The server-side code: PHP
- •12.3.1 Building the XML document
- •12.3.2 Building the XSLT document
- •12.4 Combining the XSLT and XML documents
- •12.4.1 Working with Microsoft Internet Explorer
- •12.4.2 Working with Mozilla
- •12.5 Completing the search
- •12.5.1 Applying a Cascading Style Sheet
- •12.5.2 Improving the search
- •12.5.3 Deciding to use XSLT
- •12.5.4 Overcoming the Ajax bookmark pitfall
- •12.6 Refactoring
- •12.6.1 An XSLTHelper
- •12.6.2 A live search component
- •12.6.3 Refactoring debriefing
- •12.7 Summary
- •Building stand-alone applications with Ajax
- •13.1 Reading information from the outside world
- •13.1.1 Discovering XML feeds
- •13.1.2 Examining the RSS structure
- •13.2 Creating the rich user interface
- •13.2.1 The process
- •13.2.3 Compliant CSS formatting
- •13.3 Loading the RSS feeds
- •13.3.1 Global scope
- •13.3.2 Ajax preloading functionality
- •13.4 Adding a rich transition effect
- •13.4.2 Implementing the fading transition
- •13.4.3 Integrating JavaScript timers
- •13.5 Additional functionality
- •13.5.1 Inserting additional feeds
- •13.5.2 Integrating the skipping and pausing functionality
- •13.6 Avoiding the project’s restrictions
- •13.6.1 Overcoming Mozilla’s security restriction
- •13.6.2 Changing the application scope
- •13.7 Refactoring
- •13.7.1 RSS reader Model
- •13.7.2 RSS reader view
- •13.7.3 RSS reader Controller
- •13.7.4 Refactoring debrief
- •13.8 Summary
- •The Ajax craftsperson’s toolkit
- •A.1 Working smarter with the right toolset
- •A.1.1 Acquiring tools that fit
- •A.1.2 Building your own tools
- •A.1.3 Maintaining your toolkit
- •A.2 Editors and IDEs
- •A.2.1 What to look for in a code editor
- •A.2.2 Current offerings
- •A.3 Debuggers
- •A.3.1 Why we use a debugger
- •A.3.2 JavaScript debuggers
- •A.3.3 HTTP debuggers
- •A.3.4 Building your own cross-browser output console
- •A.4 DOM inspectors
- •A.4.1 Using the Mozilla DOM Inspector
- •A.4.2 DOM inspectors for Internet Explorer
- •A.4.3 The Safari DOM Inspector for Mac OS X
- •A.5 Installing Firefox extensions
- •A.6 Resources
- •JavaScript for object-oriented programmers
- •B.1 JavaScript is not Java
- •B.2 Objects in JavaScript
- •B.2.1 Building ad hoc objects
- •B.2.2 Constructor functions, classes, and prototypes
- •B.2.3 Extending built-in classes
- •B.2.4 Inheritance of prototypes
- •B.2.5 Reflecting on JavaScript objects
- •B.2.6 Interfaces and duck typing
- •B.3 Methods and functions
- •B.3.1 Functions as first-class citizens
- •B.3.2 Attaching functions to objects
- •B.3.3 Borrowing functions from other objects
- •B.3.4 Ajax event handling and function contexts
- •B.3.5 Closures in JavaScript
- •B.4 Conclusions
- •B.5 Resources
- •Ajax frameworks and libraries
- •Accesskey Underlining Library
- •ActiveWidgets
- •Ajax JavaServer Faces Framework
- •Ajax JSP Tag Library
- •Ajax.NET
- •AjaxAC
- •AjaxAspects
- •AjaxCaller
- •AjaxFaces
- •BackBase
- •Behaviour
- •Bindows
- •BlueShoes
- •CakePHP
- •CL-Ajax
- •ComfortASP.NET
- •Coolest DHTML Calendar
- •Dojo
- •DWR (Direct Web Remoting)
- •Echo 2
- •FCKEditor
- •Flash JavaScript Integration Kit
- •Google AjaxSLT
- •Guise
- •HTMLHttpRequest
- •Interactive Website Framework
- •Jackbe
- •JPSpan
- •jsolait
- •JSON
- •JSRS (JavaScript Remote Scripting)
- •LibXMLHttpRequest
- •Mochikit
- •netWindows
- •Oddpost
- •OpenRico
- •Pragmatic Objects
- •Prototype
- •Qooxdoo
- •RSLite
- •Ruby on Rails
- •Sack
- •SAJAX
- •Sarissa
- •Scriptaculous
- •SWATO…
- •Tibet
- •TinyMCE
- •TrimPath Templates
- •Walter Zorn’s DHTML Libraries
- •WebORB for .NET
- •WebORB for Java
- •XAJAX
- •x-Desktop
- •XHConn
- •index
- •Symbols
- •Numerics
Writing to the server |
197 |
|
|
This covers the basic mechanics of submitting data to the server, whether based on textual input from a form or other activity such as drag and drop or mouse movements. In the following section, we’ll pick up our ObjectViewer example from chapter 4 and learn how to manage updates to the domain model in an orderly fashion.
5.5.3Managing user updates effectively
In chapter 4, we introduced the ObjectViewer, a generic piece of code for browsing complex domain models, and provided a simple example for viewing planetary data. The objects representing the planets in the solar system each contained several parameters, and we marked a couple of simple textual properties—the diameter and distance from the sun—as editable. Changes made to any properties in the system were captured by a central event listener function, which we used to write some debug information to the browser status bar. (The ability to write to the status bar is being restricted in recent builds of Mozilla Firefox. In appendix A, we present a pure JavaScript logging console that could be used to provide status messages to the user in the absence of a native status bar.) This event listener mechanism also provides an ideal way of capturing updates in order to send them to the server.
Let’s suppose that we have a script updateDomainModel.jsp running on our server that captures the following information:
■The unique ID of the planet being updated
■The name of the property being updated
■The value being assigned to the property
We can write an event handler to fire all changes to the server like so:
function updateServer(propviewer){
var planetObj=propviewer.viewer.object; var planetId=planetObj.id;
var propName=propviewer.name; var val=propviewer.value; net.ContentLoader(
'updateDomainModel.jsp',
someResponseHandler,
null,
'POST',
'planetId='+encodeURI(planetId)
+'&propertyName='+encodeURI(propName)
+'&value='+encodeURI(val)
);
}

198CHAPTER 5
The role of the server
And we can attach it to our ObjectViewer:
myObjectViewer.addChangeListener(updateServer);
This is easy to code but can result in a lot of very small bits of traffic to the server, which is inefficient and potentially confusing. If we want to control our traffic, we can capture these updates and queue them locally and then send them to the server in batches at our leisure. A simple update queue implemented in JavaScript is shown in listing 5.13.
Listing 5.13 CommandQueue object
net.CommandQueue=function(id,url,freq){ b Create a queue object this.id=id;
net.cmdQueues[id]=this;
this.url=url; this.queued=new Array(); this.sent=new Array(); if (freq){
this.repeat(freq);
}
}
net.CommandQueue.prototype.addCommand=function(command){ if (this.isCommand(command)){
this.queue.append(command,true);
}
}
net.CommandQueue.prototype.fireRequest=function(){ c Send request to server if (this.queued.length==0){
return;
}
var data="data=";
for(var i=0;i<this.queued.length;i++){ var cmd=this.queued[i];
if (this.isCommand(cmd)){ data+=cmd.toRequestString(); this.sent[cmd.id]=cmd;
}
}
this.queued=new Array(); this.loader=new net.ContentLoader(
this.url,
net.CommandQueue.onload,net.CommandQueue.onerror,
"POST",data
);
}

Writing to the server |
199 |
|
|
net.CommandQueue.prototype.isCommand=function(obj){ d Test object type return (
obj.implementsProp("id")
&&obj.implementsFunc("toRequestString")
&&obj.implementsFunc("parseResponse")
);
}
net.CommandQueue.onload=function(loader){ e Parse server response var xmlDoc=net.req.responseXML;
var elDocRoot=xmlDoc.getElementsByTagName("commands")[0]; if (elDocRoot){
for(i=0;i<elDocRoot.childNodes.length;i++){
elChild=elDocRoot.childNodes[i]; if (elChild.nodeName=="command"){ var attrs=elChild.attributes;
var id=attrs.getNamedItem("id").value; var command=net.commandQueue.sent[id]; if (command){
command.parseResponse(elChild);
}
}
}
} |
|
} |
|
net.CommandQueue.onerror=function(loader){ |
|
alert("problem sending the data to the server"); |
|
} |
|
net.CommandQueue.prototype.repeat=function(freq){ |
f Poll the server |
this.unrepeat(); |
|
if (freq>0){ |
|
this.freq=freq;
var cmd="net.cmdQueues["+this.id+"].fireRequest()";
this.repeater=setInterval(cmd,freq*1000); |
|
|
} |
|
|
} |
g Switch polling off |
|
net.CommandQueue.prototype.unrepeat=function(){ |
||
if (this.repeater){ |
|
|
clearInterval(this.repeater); |
|
|
} |
|
|
this.repeater=null; |
|
|
} |
|
|
|
|
|
|
|
|
The CommandQueue object (so called because it queues Command objects— we’ll get to that in a minute) is initialized bwith a unique ID, the URL of a serverside script, and, optionally, a flag indicating whether to poll repeatedly. If it
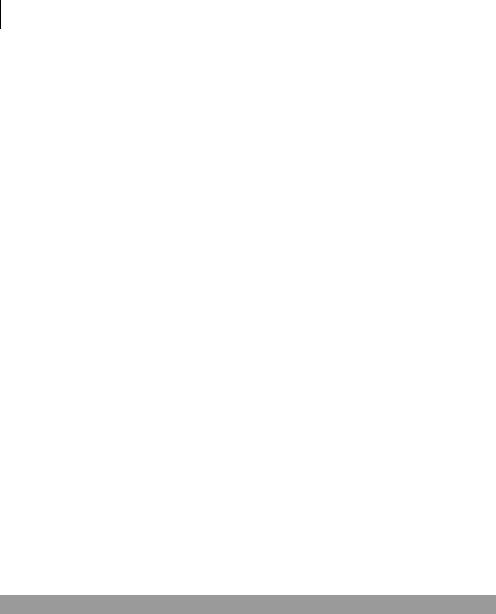
200CHAPTER 5
The role of the server
doesn’t, then we’ll need to fire it manually every so often. Both modes of operation may be useful, so both are included here. When the queue fires a request to the server, it converts all commands in the queue to strings and sends them with the request c.
The queue maintains two arrays. queued is a numerically indexed array, to which new updates are appended. sent is an associative array, containing those updates that have been sent to the server but that are awaiting a reply. The objects in both queues are Command objects, obeying an interface enforced by the isCommand() function d. That is:
■It can provide a unique ID for itself.
■It can serialize itself for inclusion in the POST data sent to the server (see c).
■It can parse a response from the server (see e) in order to determine whether it was successful or not, and what further action, if any, it should take.
We use a function implementsFunc() to check that this contract is being obeyed. Being a method on the base class Object, you might think it is standard JavaScript, but we actually defined it ourselves in a helper library like this:
Object.prototype.implementsFunc=function(funcName){
return this[funcName] && this[funcName] instanceof Function;
}
Appendix B explains the JavaScript prototype in greater detail. Now let’s get back to our queue object. The onload method of the queue eexpects the server to return with an XML document consisting of <command> tags inside a central
<commands> tag.
Finally, the repeat() f and unrepeat() g methods are used to manage the repeating timer object that will poll the server periodically with updates.
The Command object for updating the planet properties is presented in listing 5.14.
Listing 5.14 UpdatePropertyCommand object
planets.commands.UpdatePropertyCommand=function(owner,field,value){ this.id=this.owner.id+"_"+field;
this.obj=owner;
this.field=field;
this.value=value;
}
planets.commands.UpdatePropertyCommand.toRequestString=function(){

Writing to the server |
201 |
|
|
return { type:"updateProperty", id:this.id, planetId:this.owner.id, field:this.field, value:this.value
}.simpleXmlify("command");
}
planets.commands.UpdatePropertyCommand.parseResponse=function(docEl){ var attrs=docEl.attributes;
var status=attrs.getNamedItem("status").value; if (status!="ok"){
var reason=attrs.getNamedItem("message").value; alert("failed to update "
+this.field+" to "+this.value +"\n\n"+reason);
}
}
The command simply provides a unique ID for the command and encapsulates the parameters needed on the server. The toRequestString() function writes itself as a piece of XML, using a custom function that we have attached to the Object prototype:
Object.prototype.simpleXmlify=function(tagname){ var xml="<"+tagname;
for (i in this){
if (!this[i] instanceof Function){ xml+=" "+i+"=\""+this[i]+"\"";
}
}
xml+="/>"; return xml;
}
This will create a simple XML tag like this (formatted by hand for clarity):
<command type='updateProperty' id='001_diameter' planetId='mercury' field='diameter'
value='3'/>
Note that the unique ID consists only of the planet ID and the property name. We can’t send multiple edits of the same value to the server. If we do edit a property several times before the queue fires, each later value will overwrite earlier ones.
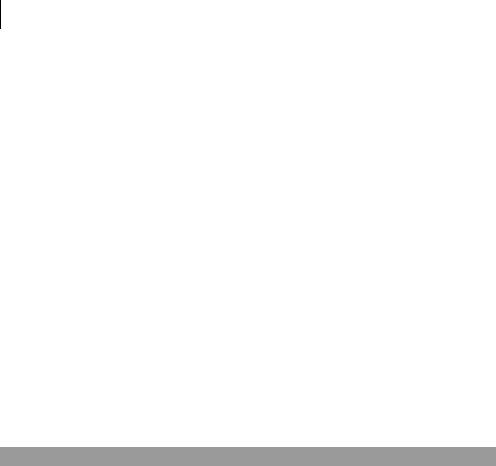
202CHAPTER 5
The role of the server
The POST data sent to the server will contain one or more of these tags, depending on the polling frequency and how busy the user is. The server process needs to process each command and store the results in a similar response. Our CommandQueue’s onload will match each tag in the response to the Command object in the sent queue and then invoke that Command’s parseResponse method. In this case, we are simply looking for a status attribute, so the response might look like this:
<commands>
<command id='001_diameter' status='ok'/>
<command id='003_albedo' status='failed' message='value out of range'/> <command id='004_hairColor' status='failed' message='invalid property name'/>
</commands>
Mercury’s diameter has been updated, but two other updates have failed, and a reason has been given in each case. Our user has been informed of the problems (in a rather basic fashion using the alert() function) and can take remedial action.
The server-side component that handles these requests needs to be able to break the request data into commands and assign each command to an appropriate handler object for processing. As each command is processed, the result will be written back to the HTTP response. A simple implementation of a Java servlet for handling this task is given in listing 5.15.
Listing 5.15 CommandServlet.java
public class CommandServlet extends HttpServlet {
private Map commandTypes=null;
public void init() throws ServletException { ServletConfig config=getServletConfig();
commandTypes=new HashMap(); b Configure handlers on startup boolean more=true;
for(int counter=1;more;counter++){
String typeName=config.getInitParameter("type"+counter); String typeImpl=config.getInitParameter("impl"+counter); if (typeName==null || typeImpl==null){
more=false;
}else{
try{
Class cls=Class.forName(typeImpl); commandTypes.put(typeName,cls);
}catch (ClassNotFoundException clanfex){ this.log(
|
|
Writing to the server |
203 |
"couldn't resolve handler class name " |
|
||
|
|||
+typeImpl); |
|
|
|
} |
|
|
|
} |
|
|
|
} |
|
|
|
} |
|
|
|
protected void doPost( |
|
|
|
HttpServletRequest req, |
|
|
|
HttpServletResponse resp |
|
|
|
) throws IOException{ |
c Process a request |
|
|
resp.setContentType("text/xml"); |
|
||
Reader reader=req.getReader(); |
|
|
|
Writer writer=resp.getWriter(); |
|
|
|
try{ |
|
|
|
SAXBuilder builder=new SAXBuilder(false); |
|
||
Document doc=builder.build(reader); |
d Process XML data |
|
Element root=doc.getRootElement();
if ("commands".equals(root.getName())){
for(Iterator iter=root.getChildren("command").iterator(); iter.hasNext();){
Element el=(Element)(iter.next());
String type=el.getAttributeValue("type"); XMLCommandProcessor command=getCommand(type,writer); if (command!=null){
Element result=command.processXML(el); e Delegate to handler writer.write(result.toString());
}
}
}else{
sendError(writer,
"incorrect document format - " +"expected top-level command tag");
}
}catch (JDOMException jdomex){
sendError(writer,"unable to parse request document");
}
}
private XMLCommandProcessor getCommand
(String type,Writer |
writer) |
throws IOException{ |
f Match handler to command |
XMLCommandProcessor cmd=null;
Class cls=(Class)(commandTypes.get(type)); if (cls!=null){
try{
cmd=(XMLCommandProcessor)(cls.newInstance()); }catch (ClassCastException castex){
sendError(writer, "class "+cls.getName()
+" is not a command");
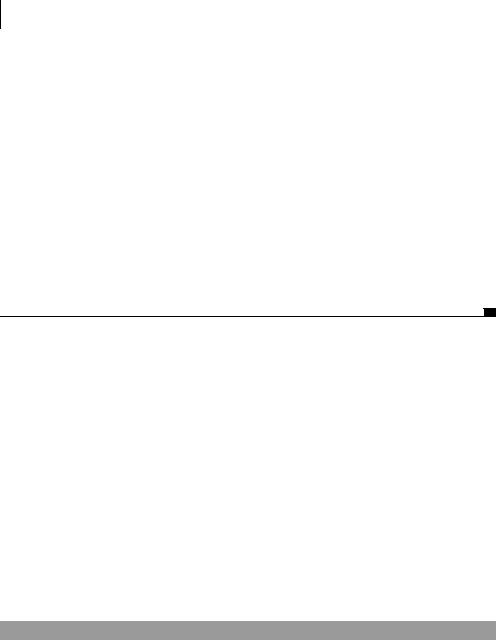
204CHAPTER 5
The role of the server
}catch (InstantiationException instex) { sendError(writer,
"not able to create class "+cls.getName());
}catch (IllegalAccessException illex) { sendError(writer,
"not allowed to create class "+cls.getName());
}
}else{
sendError(writer,"no command type registered for "+type);
}
return cmd;
}`
private void sendError
(Writer writer,String message) throws IOException{ writer.write("<error msg='"+message+"'/>"); writer.flush();
}
}
The servlet maintains a map of XMLCommandProcessor objects that are configured here through the ServletConfig interface b. A more mature framework might provide its own XML config file. When processing an incoming POST request c, we use JDOM to parse the XML data d and then iterate through the <command> tags matching type attributes to XMLCommandProcessors e. The map holds class definitions, from which we create live instances using reflection in the getCommand() method f.
The XMLCommandProcessor interface consists of a single method:
public interface XMLCommandProcessor { Element processXML(Element el);
}
The interface depends upon the JDOM libraries for a convenient object-based representation of XML, using Element objects as both argument and return type. A simple implementation of this interface for updating planetary data is given in listing 5.16.
Listing 5.16 PlanetUpdateCommandProcessor.java
public class PlanetUpdateCommandProcessor implements XMLCommandProcessor {
public Element processXML(Element el) {
Element result=new Element("command"); b Create XML result node String id=el.getAttributeValue("id");

|
Writing to the server |
205 |
result.setAttribute("id",id); |
|
|
|
||
String status=null; |
|
|
String reason=null; |
|
|
String planetId=el.getAttributeValue("planetId"); |
|
|
String field=el.getAttributeValue("field"); |
|
|
String value=el.getAttributeValue("value"); |
|
|
Planet planet=findPlanet(planetId); c Access domain model |
|
|
if (planet==null){ |
|
|
status="failed"; |
|
|
reason="no planet found for id "+planetId; |
|
|
}else{ |
|
|
Double numValue=new Double(value); |
|
|
Object[] args=new Object[]{ numValue }; |
|
|
String method = "set"+field.substring(0,1).toUpperCase() |
|
|
+field.substring(1); |
|
|
Statement statement=new Statement(planet,method,args); |
|
|
try { |
d Update domain model |
|
statement.execute(); |
|
|
status="ok"; |
|
|
}catch (Exception e) { status="failed";
reason="unable to set value "+value+" for field "+field;
}
}
result.setAttribute("status",status); if (reason!=null){
result.setAttribute("reason",reason);
}
return result;
}
private Planet findPlanet(String planetId) {
// TODO use hibernate |
e Use ORM for domain model |
return null; |
|
} |
|
}
As well as using JDOM to parse the incoming XML, we use it here to generate XML, building up a root node b and its children programmatically in the processXML() method. We access the server-side domain model using the Planet() method c, once we have a unique ID to work with. findPlanet() isn’t implemented here, for the sake of brevity—typically an ORM such as Hibernate would be used to talk to the database behind the scenes e. We use reflection to update the domain model dand then return the JDOM object that we have constructed, where it will be serialized by the servlet.