
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
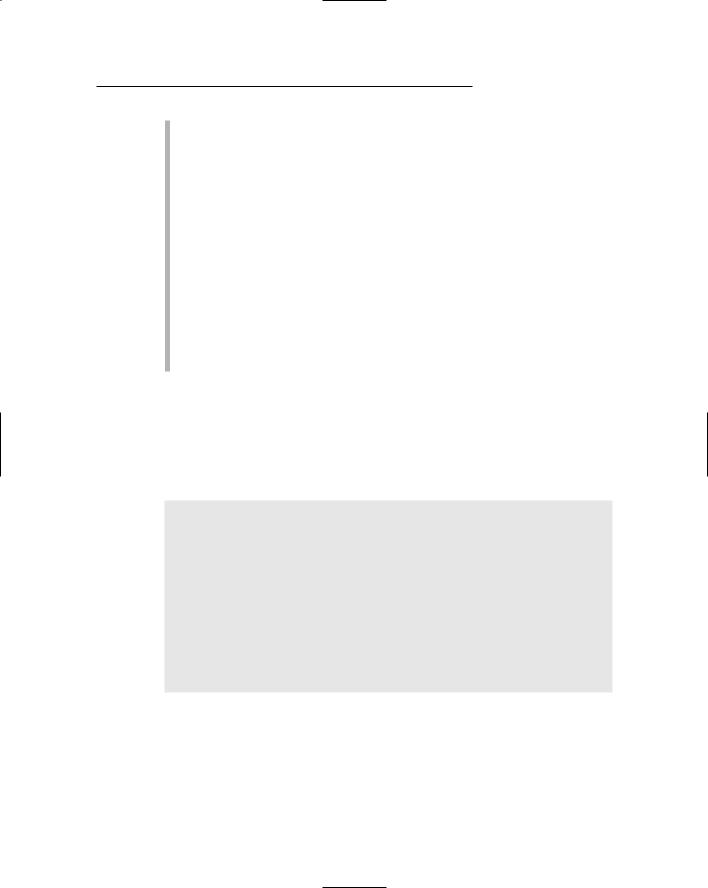
Chapter 4: C What I/O 51
If you’re writing a C program that requires input, you must create a place to store it. For text input, that place is a string variable, which you create by using the char keyword.
Variables are officially introduced in Chapter 8 in this book. For now, con sider the string variable that scanf() uses as merely a storage chamber for text you type.
The formatting codes used by scanf() are identical to those used by printf(). In real life, you use them mostly with printf() because there are better ways to read the keyboard than to use scanf(). Refer to Table 24-2 in Chapter 24 for a list of the formatting percent-sign place holder codes.
Forgetting to stick the & in front of scanf()’s variable is a common mis take. Not doing so leads to some wonderful null pointer assignment errors that you may relish in the years to come. As a weird quirk, however, the ampersand is optional when you’re dealing with string variables. Go figure.
The miracle of scanf()
Consider the following pointless program, COLOR.C, which uses two string variables, name and color. It asks for your name and then your favorite color. The final printf() statement then displays what you enter.
#include <stdio.h>
int main()
{
char name[20]; char color[20];
printf(“What is your name?”); scanf(“%s”,name);
printf(“What is your favorite color?”); scanf(“%s”,color);
printf(“%s’s favorite color is %s\n”,name,color); return(0);
}
Enter this source code into your editor. Save this file to disk as COLOR.C. Compile.
If you get any errors, double-check your source code and reedit the file. A common mistake: forgetting that there are two commas in the final printf() statement.
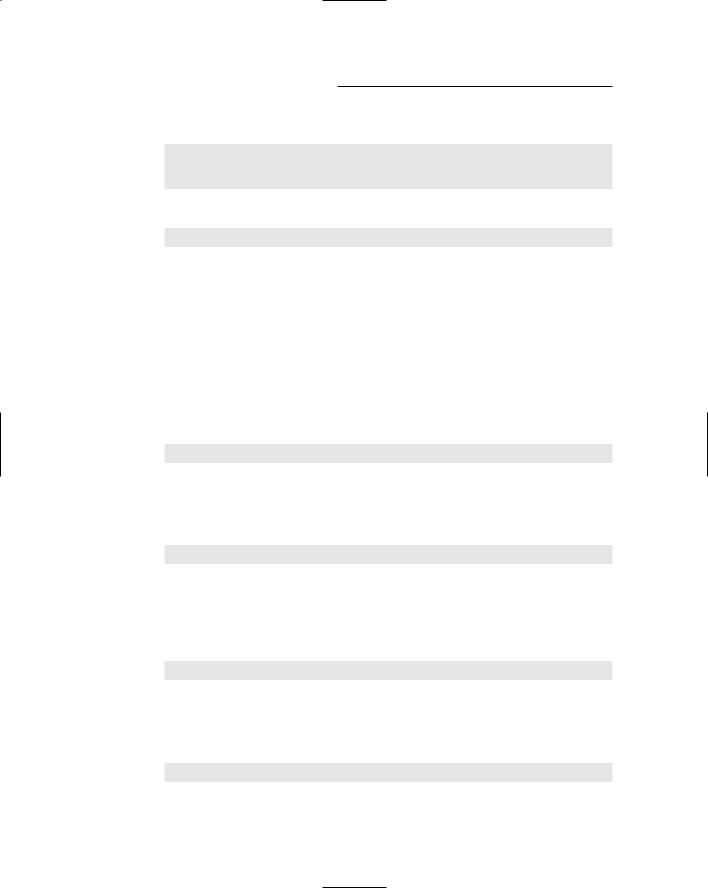
52 |
Part I: Introduction to C Programming |
Run the program! The output looks something like this:
What is your name?dan
What is your favorite color?brown dan’s favorite color is brown
In Windows XP, you have to run the command by using the following line:
.\color
The reason is that COLOR is a valid console command in Windows XP, used to change the foreground and background color of the console window.
Experimentation time!
Which is more important: the order of the %s doodads or the order of the variables — the arguments — in a printf statement? Give up? I’m not going to tell you the answer. You have to figure it out for yourself.
Make the following modification to Line 12 in the COLOR.C program:
printf(“%s’s favorite color is %s\n”,color,name);
The order of the variables here is reversed: color comes first and then name. Save this change to disk and recompile. The program still runs, but the output is different because you changed the variable order. You may see something like this:
brown’s favorite color is Dan.
See? Computers are stupid! The point here is that you must remember the order of the variables when you have more than one listed in a printf() function. The %s thingies? They’re just fill-in-the-blanks.
How about making this change:
printf(“%s’s favorite color is %s\n”,name,name);
This modification uses the name variable twice — perfectly allowable. All printf() needs are two string variables to match the two %s signs in its for matting string. Save this change and recompile. Run the program and exam ine the output:
Dan’s favorite color is Dan
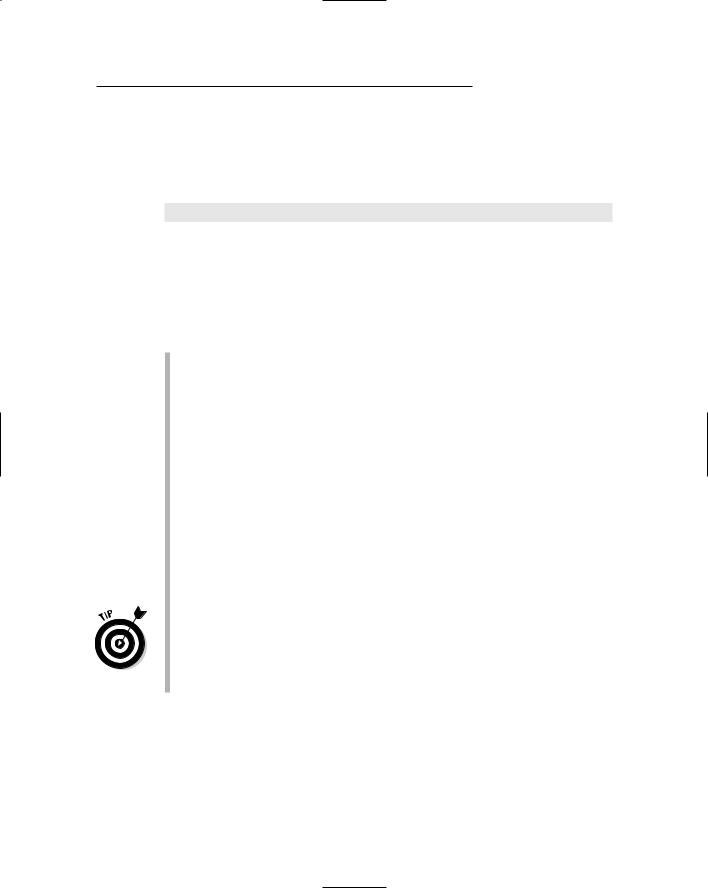
Chapter 4: C What I/O 53
Okay, Lois — have you been drinking again? Make that mistake on an IRS form and you may spend years playing golf with former stockbrokers and congressmen. (Better learn to order your variables now.)
Finally, make the following modification:
printf(“%s’s favorite color is %s\n”,name,”blue”);
Rather than the color variable, a string constant is used. A string constant is simply a string enclosed in quotes. It doesn’t change, unlike a variable, which can hold anything. (It isn’t variable!)
Save the change to disk and recompile your efforts. The program still works, though no matter which color you enter, the computer always insists that it’s “blue.”
The string constant “blue” works because printf()’s %s placeholder looks for a string of text. It doesn’t matter whether the string is a variable or a “real” text string sitting there in double quotes. (Of course, the advan tage to writing a program is that you can use variables to store input; using the constant is a little silly because the computer already knows what it’s going to print. I mean, ladies and gentlemen, where is the I/O?)
The %s placeholder in a printf() function looks for a corresponding string variable and plugs it in to the text that is displayed.
You need one string variable in the printf() function for each %s that appears in printf()’s formatting string. If the variable is missing, a syntax boo-boo is generated by the compiler.
In addition to string variables, you can use string constants, often called literal strings. That’s kind of dumb, though, because there’s no point in wasting time with %s if you already know what you’re going to display. (I have to demonstrate it here, however, or else I have to go to C Teacher’s Prison in Connecticut.)
Make sure that you get the order of your variables correct. This advice is especially important when you use both numeric and string variables in printf.
The percent sign (%) is a holy character. Om! If you want a percent sign
(%) to appear in printf’s output, use two of them: %%.
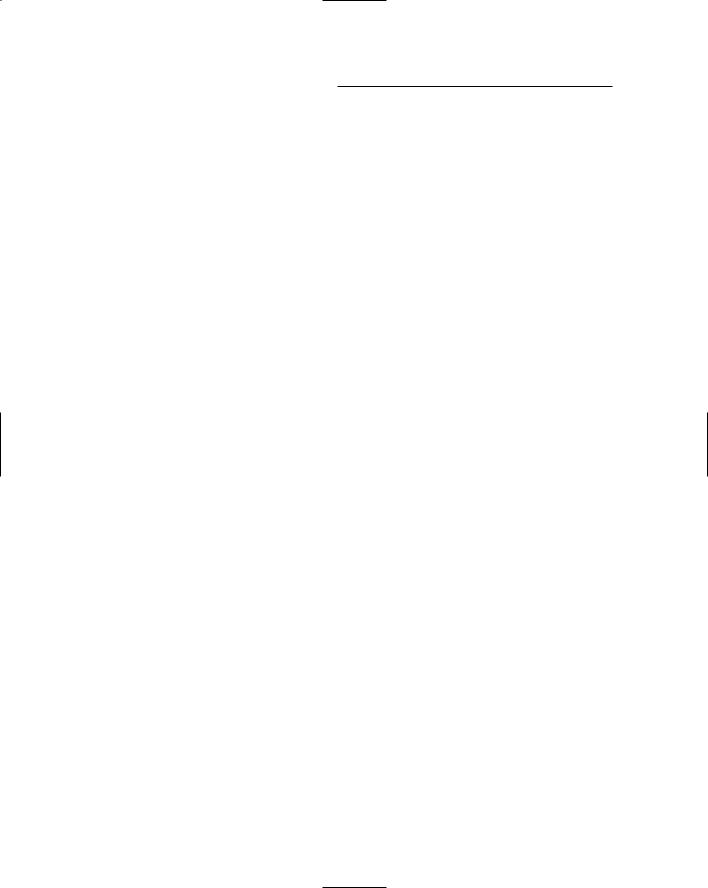
54 |
Part I: Introduction to C Programming |