
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index

Chapter 8: Charting Unknown Cs with Variables 101
The integer variables are declared on one line, and the floating-point (non integer) variables on another.
Keep variables that are defined with a value on a line by themselves. To wit:
int first=1;
int the_rest;
Constants and Variables
In addition to the variable, the C language has something called a constant.
It’s used like a variable, though its value never changes.
Suppose that one day someone dupes you into writing a trigonometry pro gram. In this type of program, you have to use the dreaded value π (pi). That’s equal to 3.1415926 (and on and on). Because it never changes, you can create a constant named pi that is equal to that value.
Another example of a constant is a quoted string of text:
printf(“%s”,”This is a string of text”);
The text “This is a string of text” is a constant used with this printf() function. A variable can go there, though a string constant — a literal, quoted string — is used instead.
A constant is used just like a variable, though its value never changes.
A numeric constant is a number value, like π, that remains the same throughout your program.
π is pronounced “pie.” It’s the Greek letter p. We pronounce the English letter p as “pee.”
A string constant is a bit of text that never changes, though that’s really true of most text in a C language program. This chapter, therefore, con centrates primarily on numeric constants.
Dreaming up and defining constants
All this constant nonsense can seem silly. Then one day, you’re faced with a program like SPEED.C — except that the program is much longer — and you truly come to realize the value of a C language constant and the nifty #define directive you read about later in this chapter:

102 Part II: Run and Scream from Variables and Math
#include <stdio.h>
int main()
{
printf(“Now, the speed limit here is %i.\n”,55); printf(“But I clocked you doin’ %i.\n”,55+15); printf(“Didn’t you see that %i MPH sign?\n”,55); return(0);
}
Start over with a new slate in your editor. Carefully type the preceding source code. There’s nothing new or repulsive in it. Save the file to disk as SPEED.C.
Compile it! Fix it (if you have to)! Run it!
The output is pretty plain; something like this example is displayed:
Now, the speed limit here is 55.
But I clocked you doin’ 70.
Didn’t you see that 55 MPH sign?
Eh? No big deal.
But what if the speed limit were really 45? That would mean that you would have to edit the program and replace 55 with 45 all over. Better still, what if the program were 800 lines long and you had to do that? Not only that, but what if you had to change several other instances in which constants were used, and using your editor to hunt down each one and replace it properly would take months or years? Fortunately, the C language has a handy way around this dilemma.
You can easily argue that this isn’t a problem. After all, most editors have a search-and-replace command. Unfortunately, searching and replacing numbers in a computer program is a dangerous thing to do! Suppose that the number is 1. Searching and replacing it would change other values as well: 100, 512, 3.141 — all those would be goofed up by a search-and- replace.
For all you Mensa people out there, it’s true that the nature of the pro gram changes if the speed limit is lowered to 45. After all, was the scofflaw doing 70 or 60? To me, it doesn’t matter. If you’re wasting your excess IQ points on the problem, you can remedy it on your own.
The handy shortcut
The idea in this section is to come up with a handy shortcut for using number constants in C. I give you two solutions.
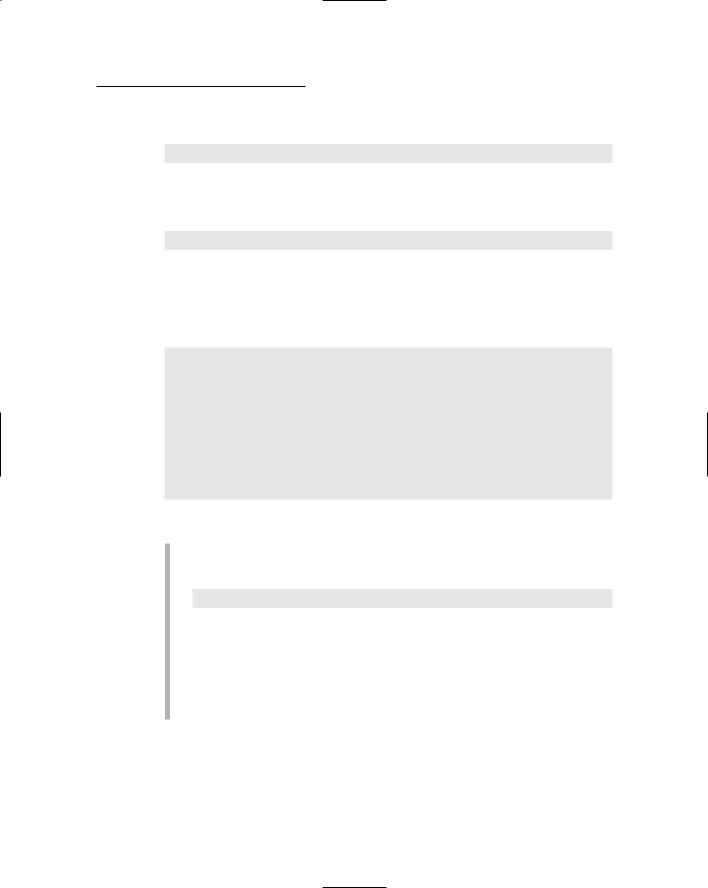
Chapter 8: Charting Unknown Cs with Variables 103
The first solution is to use a variable to hold the constant value:
int speed=55;
This line works because the compiler sticks the value 55 into the speed inte ger variable, and you can then use speed rather than 55 all over your program. To change the speed, you have to make only one edit:
int speed=45;
Although this line works, it’s silly because a variable is designed to hold a value that changes. The compiler goes to all that work, fluffing up the pillows and making things comfy for the variable, and then you misuse it as a con stant. No, the true solution is to define the constant value as a symbolic con stant. It’s really cinchy, as the updated SPEED.C program shows:
#include <stdio.h>
#define SPEED 55
int main()
{
printf(“Now, the speed limit here is %i.\n”,SPEED); printf(“But I clocked you doin’ %i.\n”,SPEED+15); printf(“Didn’t you see that %i MPH sign?\n”,SPEED); return(0);
}
Several changes are made here:
The program’s new, third line is another one of those doohickeys that begins with a pound sign (#). This one, #define, sets up a numeric con stant that can be used throughout the program:
#define SPEED 55
As with the #include thing, a semicolon doesn’t end the line. In fact, two big boo-boos with #define are using an equal sign and ending the line with a semicolon. The compiler will surely hurl error-message chunks your way if you do that.
The shortcut word SPEED is then used in the program’s three printf() statements to represent the value 55. There, it appears just like a number or variable in the printf statement.
Secretly, what happens is that the compiler sees the #define thing and all by itself does a search-and-replace. When the program is glued together, the value 55 is stuck into the printf() statements. The advantage is that you can easily update the constant values by simply editing the #define directive.
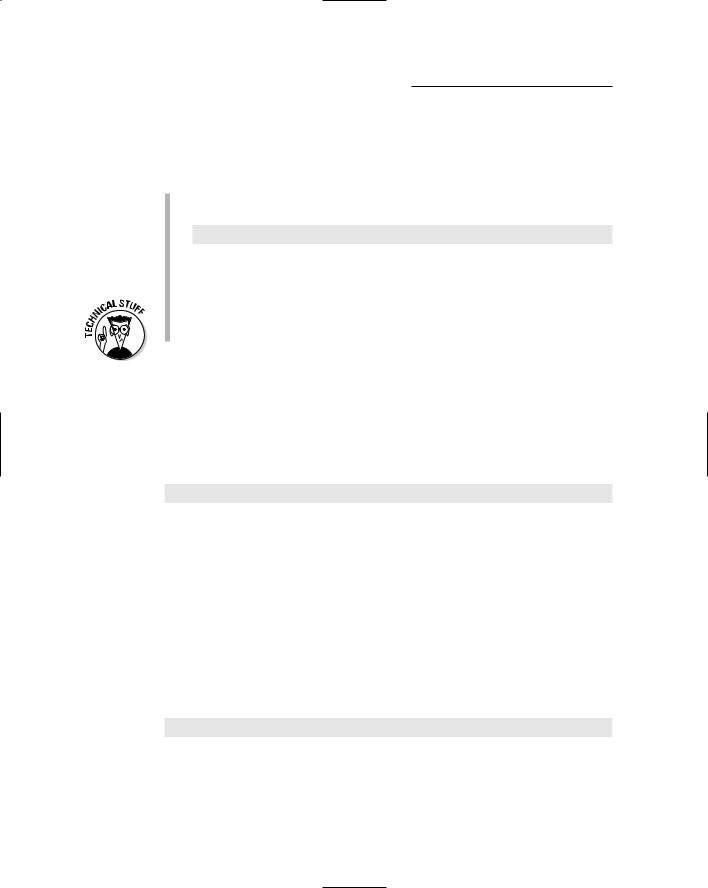
104 Part II: Run and Scream from Variables and Math
Carefully edit your SPEED.C source code so that it matches what you see listed here. Save the file to disk again and then recompile. It has the same output because your only change was to make the value 55 a real, live con stant rather than a value inside the program.
Again, the key is that it takes only one, quick edit to change the speed limit. If you edit Line 3 to read
#define SPEED 45
you have effectively changed the constant value 45 in three other places in the program. This change saves some time for the SPEED.C program — but it saves you even more time for longer, more complex programs that also use constant values.
Symbolic constant is C technospeak for a constant value created by the #define directive.
The #define directive
The #define construction (which is its official name, though I prefer to call it a directive) is used to set up what the C lords call a symbolic constant — a shortcut name for a value that appears over and over in your source code. Here’s the format:
#define SHORTCUT value
SHORTCUT is the name of the constant you’re defining. It’s traditional to name it like a variable and use ALL CAPS (no spaces).
value is the value the SHORTCUT takes on. It’s replaced by that value globally throughout the rest of your source code file. The value can be a number, equa tion, symbol, or string.
No semicolon is at the end of the line, but notice that the line absolutely must begin with a pound sign. This line appears at the beginning of your source code, before the main() function.
You should also tack a comment to the end of the #define line to remind you of what the value represents, as in this example:
#define SPEED 55 /* the speed limit */
Here, SPEED is defined to be the value 55. You can then use SPEED anywhere else in your program to represent 55.
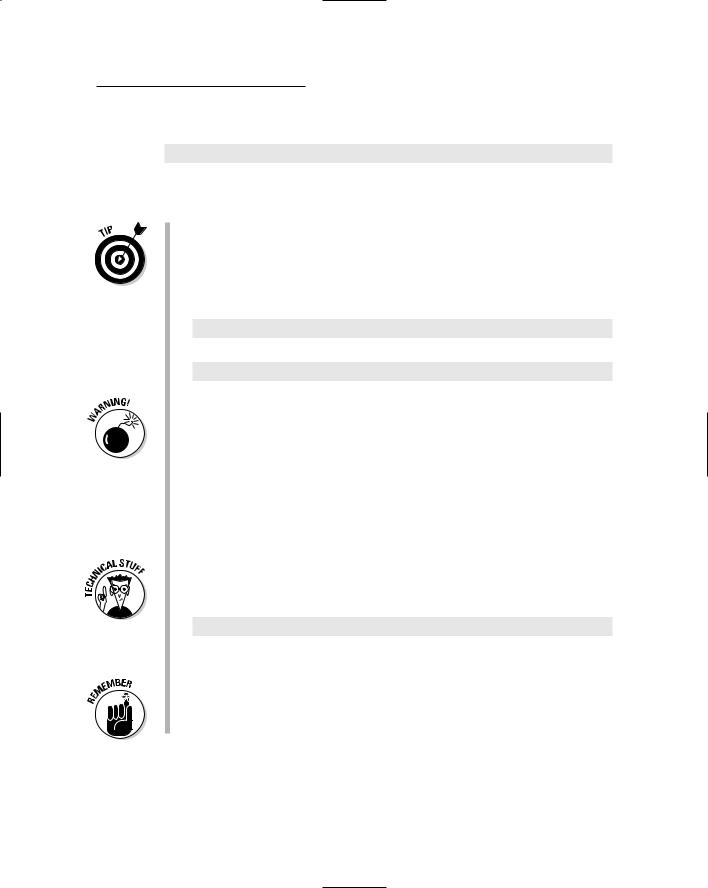
Chapter 8: Charting Unknown Cs with Variables 105
A string constant can be created in the same way, though it’s not as popular:
#define GIRLFRIEND “Brenda” /* This week’s babe */
The only difference is that the string is enclosed in double quotes, which is a traditional C-string thing.
The shortcut word is usually written in ALL CAPS so as not to confuse it with a variable name. Other than that, the rules that apply to variable names typically apply to the shortcut words. Keep ’em short and simple is my recommendation.
You have to keep track of which types of constants you have defined and then use them accordingly, as shown in this example:
printf(“The speed limit is %i.\n”,SPEED);
And in this one: puts(GIRLFRIEND);
These lines may look strange, but they’re legit because the compiler knows what SPEED and GIRLFRIEND are shortcuts for.
No equal sign appears after the shortcut word!
The line doesn’t end with a semicolon (it’s not a C language statement)!
You can also use escape-sequence, backslash-character things in a defined string constant.
String constants that are set up with #define are rare. Only if the string appears many times over and over in your program is it necessary to make it a constant. Otherwise, most programs use printf() or puts() to display text on the screen.
You can also use math and other strangeness in a defined numeric con stant. This book doesn’t go into that subject, but something as obnox ious as the following line is entirely possible:
#define SIZE 40*35
Here, the shortcut word SIZE is set up to be equal to 40*35, whatever that figures out to be.
Using the #define thing isn’t required, and you’re not penalized if you don’t use it. Sure, you can stick the numbers in there directly. And,
you can use variables to hold your constants. I won’t pout about it. You won’t go to C prison.