
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
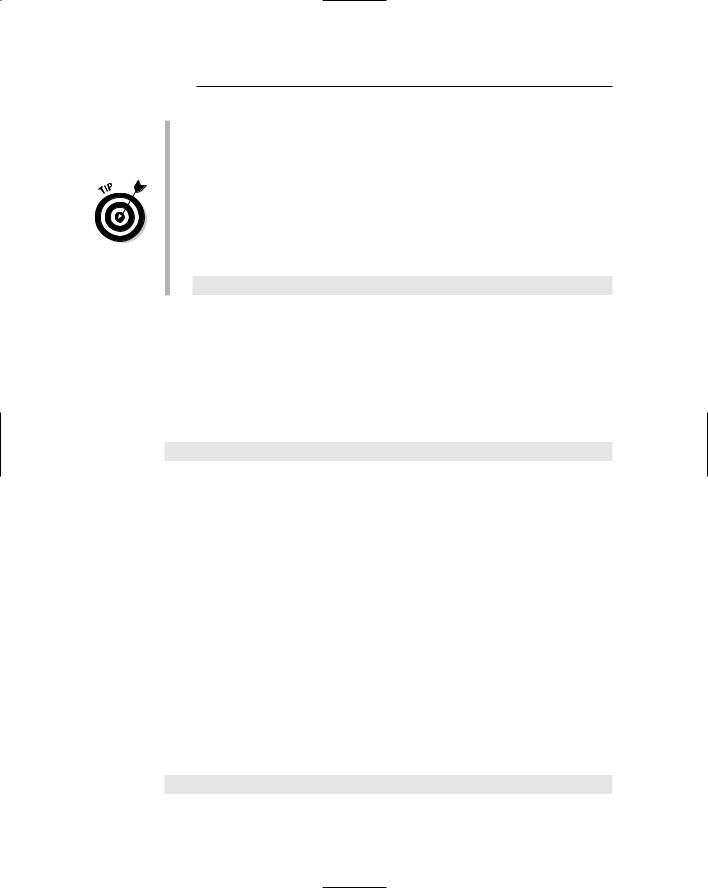
320 Part IV: C Level
The absolute value of a number is its value without the minus sign.
Even more complex functions than this one exist. Wise men truly run from them.
The best source for discovering what these commands do and how they
work is to look in the C language library reference that came with your compiler. If you’re lucky, it’s in a book; otherwise, it’s in your compiler’s online help feature.
Most of the time, you can replace the variables in a math function’s paren theses with constant values. For example:
x=sqrt(1024);
Something Really Odd to End Your Day
It’s your old pal ++ again, and his sister, -- — the increment and decrement operators from days gone by. They’re favorites, to be sure, but they’re kind of puzzling in a way. For example, consider the following snippet of code that you may find lurking in someone else’s program:
b=a++;
The variable b equals the contents of variable a plus 1. Or does it?
Remember that a++ can be a statement by itself. As such, doesn’t it work out first? If so, does b equal a+1, or does b equal a and then a is incremented after it all? And will Cody realize that Miranda is cheating on him so that he’ll be free to marry Jessica? Hmmm. . . .
It’s a puzzle that has an answer — an important answer that you have to know if you don’t want your programs getting all goofy on you.
The perils of using a++
Here’s the rule about using ++ to increment a variable so that you know what happens before running the sample program:
The variable is incremented last when ++ appears after it.
So:
b=a++;
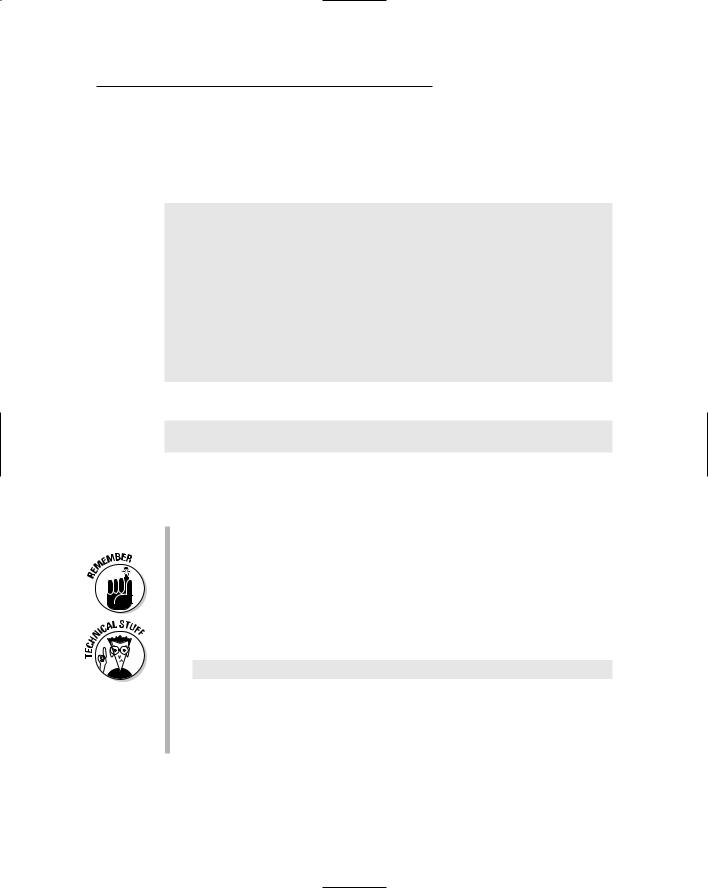
Chapter 25: Math Madness! 321
The compiler instantly slides the value of variable a into variable b when it sees this statement. Then, the value of a is incremented. To drive this point home, test out the INCODD.C program.
Type this program into your editor. Save it to disk as INCODD.C:
#include <stdio.h>
int main()
{
int a,b;
a=10;
b=0;
printf(“A=%d and B=%d before incrementing.\n”,a,b); b=a++;
printf(“A=%d and B=%d after incrementing.\n”,a,b); return(0);
}
Compile and run! Here’s what the output looks like:
A=10 and B=0 before incrementing.
A=11 and B=10 after incrementing.
The first line makes sense: The a and b variables were given those values right up front. The second line proves the ++ conundrum. First, b is assigned the value of a, which is 10; then a is incremented to 11.
Whenever you see a variable followed by ++ in a C statement, know that the variable’s value is incremented last, after any other math or assign ments (equal-sign things) elsewhere on that line.
A good way to remember this rule is that the ++ comes after the variable. It’s as though C sees the variable first, raids its contents, and then — oh, by the way — it increments its value.
This rule screws you up if you ever combine the ++ with something else inside an if comparison:
if(a=b++)
This technique is common — sticking the b++ inside the comparison rather than on a line before or afterward. You have to keep in mind, however, that b is incremented after the if command compares them. If the comparison is true, b is still incremented. Remember that when you notice that your program is acting funny.
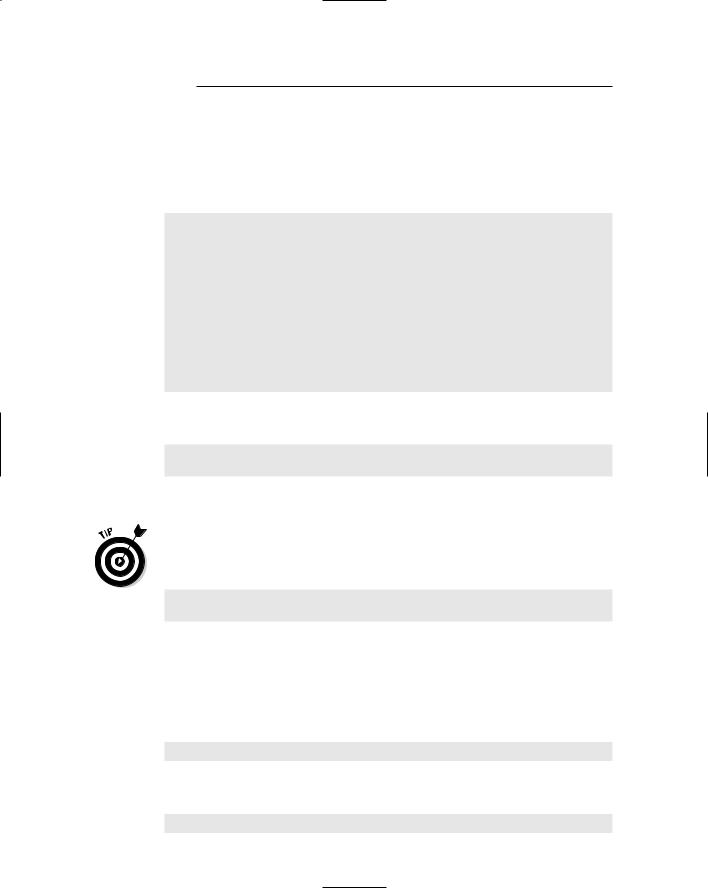
322 Part IV: C Level
Oh, and the same thing applies to a --
The decrementing operator, --, also affects a variable’s value after any other math or equal-sign stuff is done in the program. To witness this effect, here’s the DECODD.C program:
#include <stdio.h>
int main()
{
int a,b;
a=10;
b=0;
printf(“A=%d and B=%d before decrementing.\n”,a,b); b=a--;
printf(“A=%d and B=%d after decrementing.\n”,a,b); return(0);
}
Save the file to disk as DECODD.C. Compile and run. Here’s the output, prov ing that decrementing happens after any math or assignments:
A=10 and B=0 before decrementing.
A=9 and B=10 after decrementing.
The value of b is equal to 10, which means that the a variable was decre mented last.
All this makes sense, and you should understand it by now — or at least be aware of what’s happening. If you can’t remember the rule, just keep your incrementing or decrementing on a line by itself, as shown in this example:
a++;
b=a;
Reflections on the strange ++a phenomenon
Load the program INCODD.C back into your editor. Change Line 10 to read
b=++a;
This line looks stranger than it is. You can type it like this to avoid thinking that =++ is some weird C command:
b = ++a;
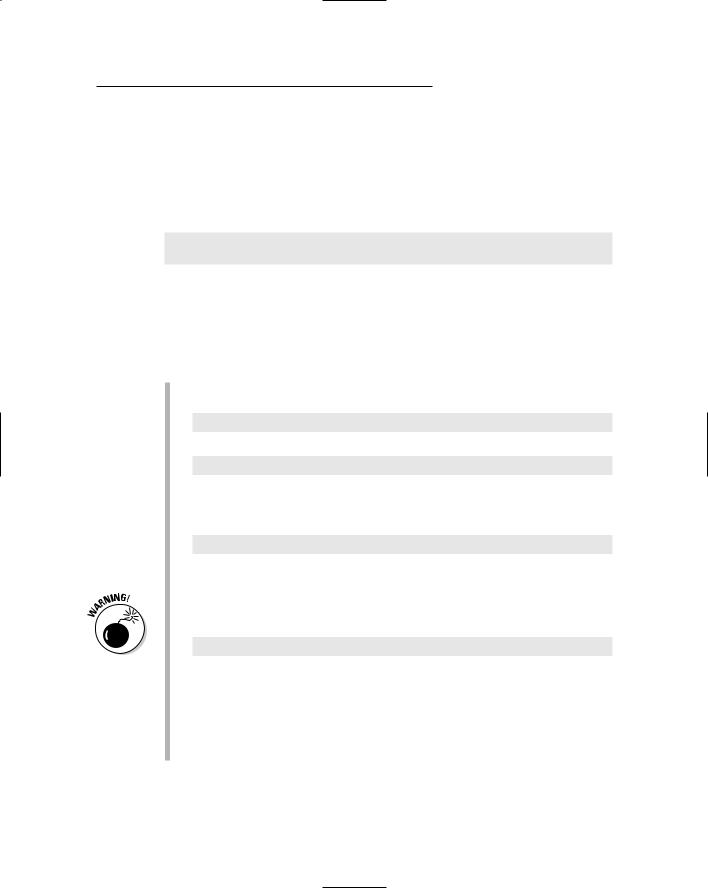
Chapter 25: Math Madness! 323
The ++ is still the incrementing operator. It still increases the value of the a variable. Because it comes before the variable, however, the incrementing happens first. It reads “Increase me first, and then do whatever else comes next.”
Save the source code for INCODD.C to disk. Compile and run it. Here’s what you see:
A=10 and B=0 before incrementing.
A=11 and B=11 after incrementing.
It worked! The ++a operation came first.
Again, this is just something strange you should know about. Rarely have I seen the ++ operator appear before a variable, but it can be done. It may help iron out some screwy things if you notice trouble when you’re putting ++ after a variable name.
You can use ++ before or after a variable when it appears on a line by itself. There’s no difference between this:
a++;
and this:
++a;
a++ is known as post-incrementing. ++a is known as pre-incrementing.
Yes, this process also works with decrementing. You can change Line 10 in DECODD.C to this:
b=--a;
Save, compile, and run the program. Variable a is decremented first, and then its new value is given to variable b.
Most people use the word anxious when they really mean eager.
Don’t even bother with this:
++a++;
Your logical mind may ponder that this statement first increments the a variable and then increments it again. Wrong! The compiler thinks that you forgot something when you try to compile this type of statement; you get one of the dreadful Lvalue required errors. That message means that the compiler was expecting another variable or number in there somewhere. Sorry, but the “fortress effect” of the incrementing or decre menting operators just doesn’t work.
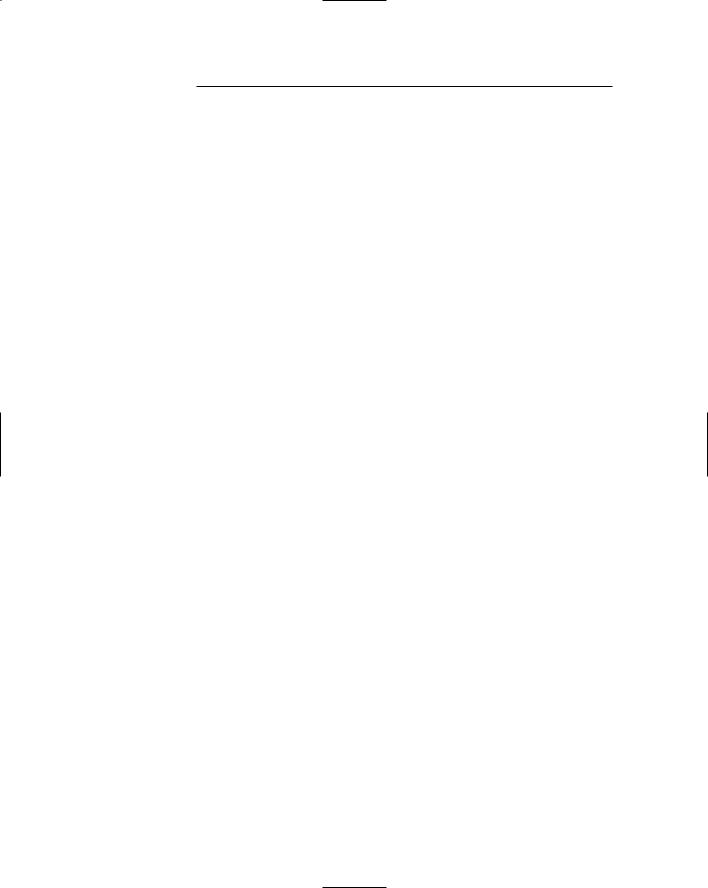
324 Part IV: C Level