
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
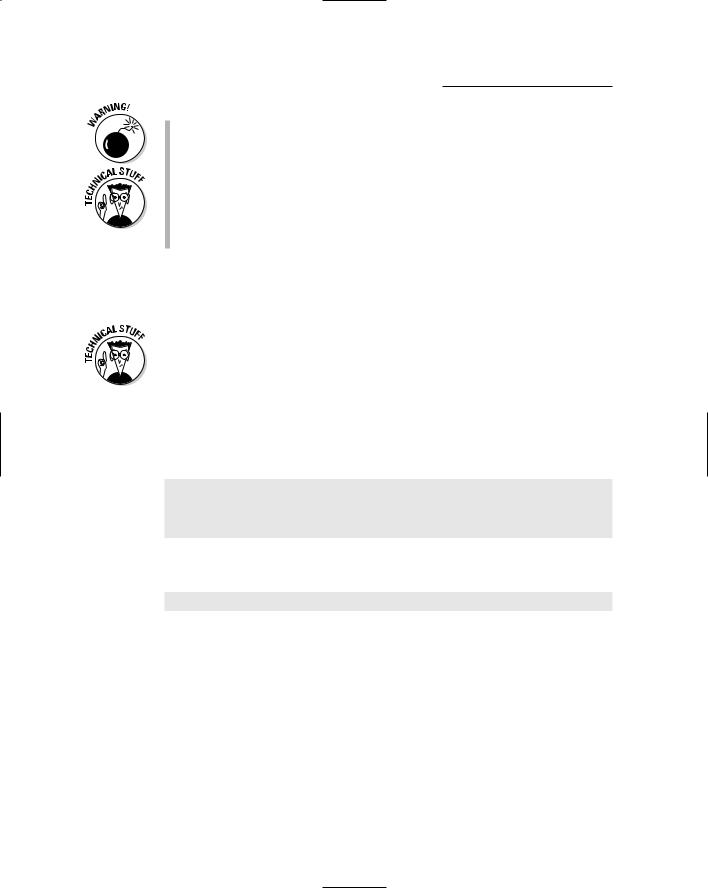
154 Part III: Giving Your Programs the Ability to Run Amok
If you use one equal sign rather than two, you don’t get an error; how ever, the program is wrong. The nearby Technical Stuff sidebar attempts to explain why.
If you have programmed in other computer languages, keep in mind that the C language has no 2ewd or fi word. The final curly brace signals to the compiler that the if statement has ended.
Also, no then word is used with if, as in the if-then thing they have in the BASIC or Pascal programming language.
A question of formatting the if statement
The if statement is your first “complex” C language statement. The C lan guage has many more, but if is the first and possibly the most popular, though I doubt that a popularity contest for programming language words has ever been held (and, then again, if would be great as Miss Congeniality but definitely come up a little thin in the swimsuit competition).
Though you probably have seen the if statement used only with curly braces, it can also be displayed as a traditional C language statement. For example, consider the following — one of the modifications from the GENIE1 program:
if(number==5)
{
printf(“That number is 5!\n”);
}
In C, it’s perfectly legitimate to write this as a more traditional type of state ment. To wit:
if(number==5) printf(“That number is 5!\n”);
This line looks more like a C language statement. It ends in a semicolon. Everything still works the same; if the value of the number variable is equal to 5, the printf() statement is executed. If number doesn’t equal 5, the rest of the statement is skipped.
Although all this is legal and you aren’t shunned in the C programming com munity for using it, I recommend using curly braces with your if statements until you feel comfortable reading the C language.
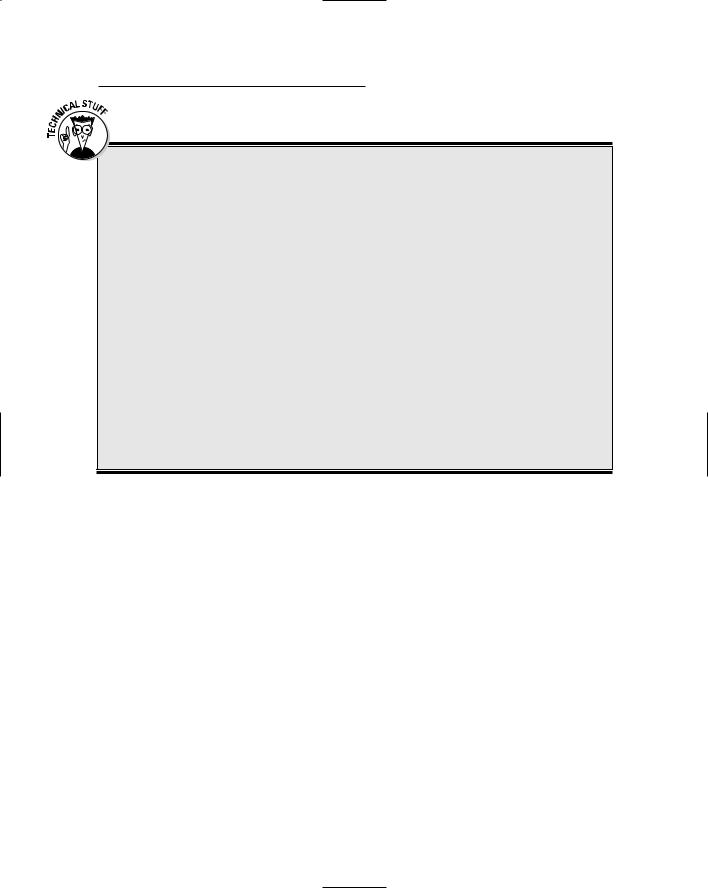
Chapter 12: C the Mighty if Command 155
Clutter not thy head with this comparison nonsense
The comparison in the if statement doesn’t have to use any symbols at all! Strange but true. What the C compiler does is to figure out what you have put between the parentheses. Then it weighs whether it’s true or false.
For a comparison using <, >, ==, or any of the horde in Table 12-1, the compiler figures out whether the comparison is true or false. However, you can stick just about anything — any valid C statement — between the paren theses and the compiler determines whether it works out to true or false. For example:
if(input=1)
This if statement doesn’t figure out whether the value of the input variable is equal to 1.
No, you need two equal signs for that. Instead, what happens between these parentheses is that the numeric variable input is given the value 1. It’s the same as
input=1;
The C compiler obeys this instruction, stuffing 1 into the input variable. Then, it sits back and strokes its beard and thinks, “Does that work out to be true or false?” Not knowing any better, it figures that the statement must be true. It tells the if keyword, and the cluster of statements that belong to the if statement are then executed.
The final solution to the income-tax problem
I have devised what I think is the fairest and most obviously well-intentioned way to decide who must pay the most in income taxes. You should pay more taxes if you’re taller and more taxes if it’s warmer outside. Yessir, it would be hard to dodge this one.
This problem is ideal for the if keyword to solve. You pay taxes based on either your height or the temperature outside, multiplied by your favorite number and then 10. Whichever number is higher is the amount of tax you pay. To figure out which number is higher, the program TAXES.C uses the if keyword with the greater-than symbol. It’s done twice — once for the height value and again for the temperature outside:
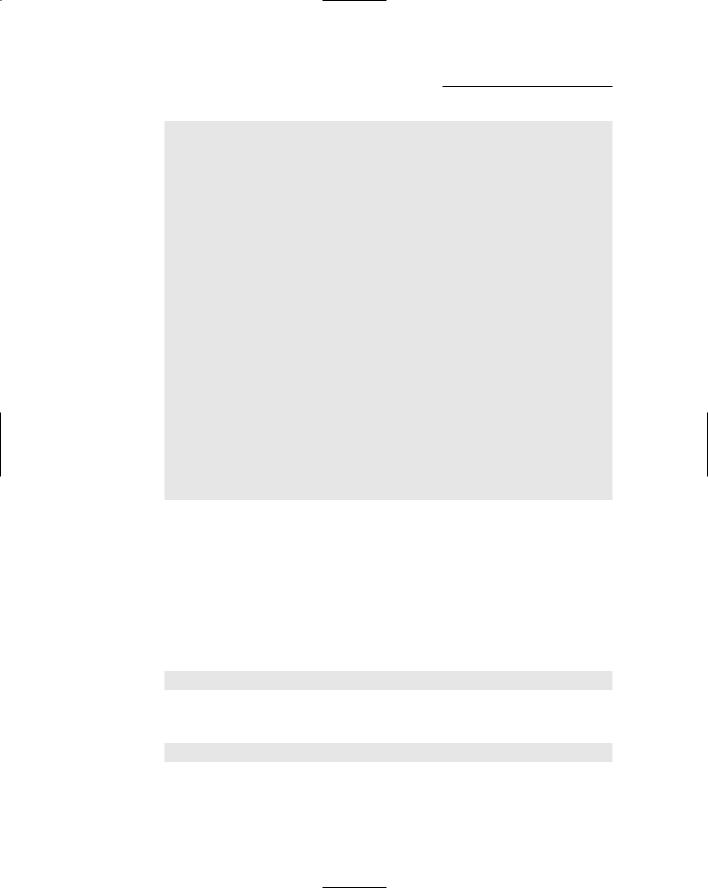
156 Part III: Giving Your Programs the Ability to Run Amok
#include <stdio.h> #include <stdlib.h>
int main()
{
int tax1,tax2;
char height[4],temp[4],favnum[5];
printf(“Enter your height in inches:”); gets(height);
printf(“What temperature is it outside?”); gets(temp);
printf(“Enter your favorite number:”); gets(favnum);
tax1 = atoi(height) * atoi(favnum); tax2 = atoi(temp) * atoi(favnum);
if(tax1>tax2)
{
printf(“You owe $%d in taxes.\n”,tax1*10);
}
if(tax2>=tax1)
{
printf(“You owe $%d in taxes.\n”,tax2*10);
}
return(0);
}
This program is one of the longer ones in this book. Be extra careful when you’re typing it. It has nothing new in it, but it covers almost all the informa tion I present in the first several chapters. Double-check each line as you type it into your editor.
Save the file to disk as TAXES.C.
Compile TAXES.C. Fix any errors you see.
Run the program:
Enter your height in inches:
Type your height in inches. Five feet is 60 inches; six feet is 72 inches. The average person is 5'7" tall or so — 67 inches. Press Enter.
What temperature is it outside?
Right now, in the bosom of winter in the Pacific Northwest, it’s 18 degrees. That’s Fahrenheit, by the way. Don’t you dare enter the smaller Celsius number. If you do, the IRS will hunt you down like a delinquent country music star and make you pay, pay, pay.
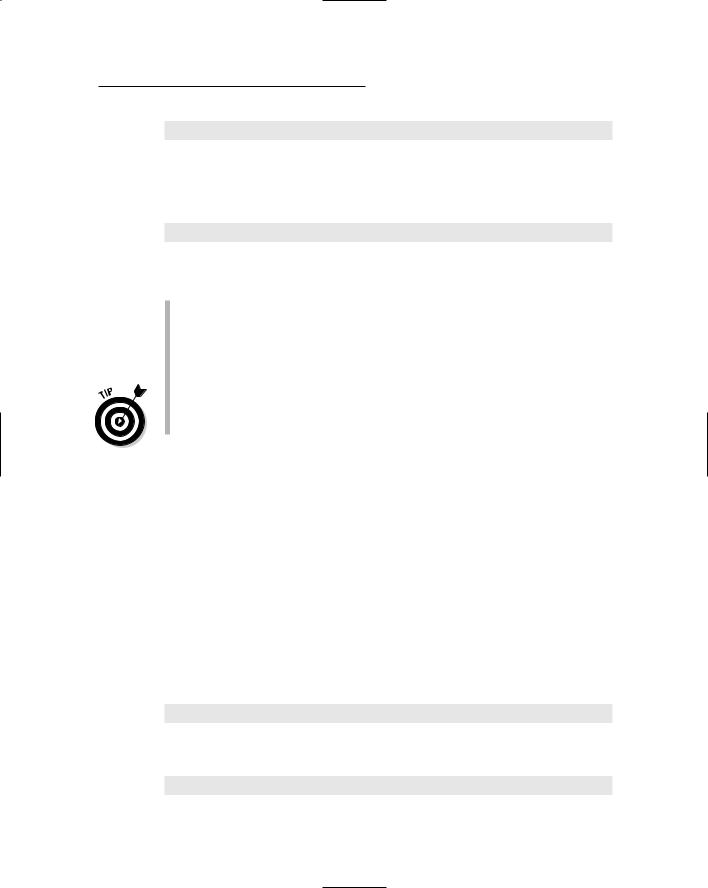
Chapter 12: C the Mighty if Command 157
Enter your favorite number:
Type your favorite number. Mine is 11. Press Enter.
If I type 72 (my height), 18, and 11, for example, I see the following result, due April 15:
You owe $7920 in taxes.
Sheesh! And I thought the old system was bad. I guess I need a smaller favorite number.
The second if comparison is “greater than or equal to.” This catches the case when your height is equal to the temperature. If both values are equal, the values of both the tax1 and tax2 variables are equal. The first if comparison, “tax1 is greater than tax2,” fails because both are equal. The second comparison, “tax1 is greater than or equal to tax2,” passes when tax1 is greater than tax2 or when both values are equal.
If you enter zero as your favorite number, the program doesn’t say that you owe any tax. Unfortunately, the IRS does not allow you to have zero — or any negative numbers — as your favorite number. Sad, but true.
If It Isn’t True, What Else?
Hold on to that tax problem!
No, not the one the government created. Instead, hold on to the TAXES.C source code introduced in the preceding section. If it’s already in your text editor, great. Otherwise, open it in your editor for editing.
The last part of the TAXES.C program consists of two if statements. The second if statement, which should be near Line 23 in your editor, really isn’t necessary. Rather than use if in that manner, you can take advantage of another word in the C language, else.
Change Line 23 in the TAXES.C program. It looks like this now:
if(tax2>=tax1)
Edit that line: Delete the if keyword and the comparison in parentheses and replace it with this:
else
That’s it — just else by itself. No comparison and no semicolon, and make sure that you type it in lowercase.