
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
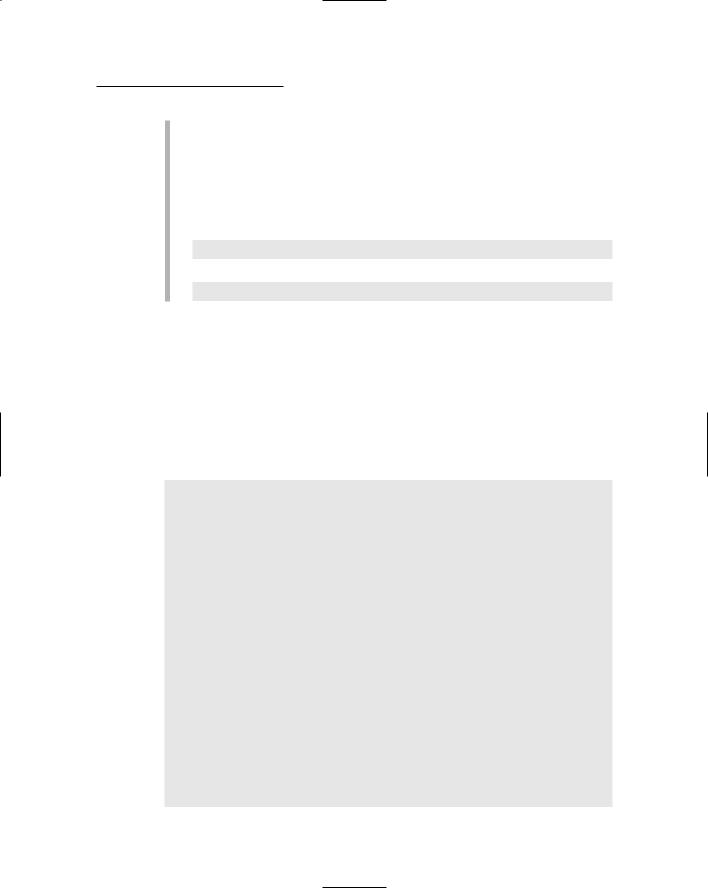
Chapter 21: Contending with Variables in Functions 271
Global variables are declared outside of any function. It’s typically done right before the main() function.
Everything you know about creating a variable, other than being declared outside a function, applies to creating global variables: You must specify the type of variable (int, char, and float, for example), the variable’s name, and the semicolon.
You can also declare a group of global variables at one time:
int score,tanks,ammo;
And, you can preassign values to global variables, if you want:
char prompt[]=”What?”;
An example of a global variable in a real, live program
For your pleasure, please refer again to the BOMBER.C source code. This final modification adds code that keeps a running total of the number of people you kill with the bombs. That total is kept in the global variable deaths, defined right up front. Here’s the final source code, with specific changes noted just afterward:
#include <stdio.h> |
|
#define COUNT 20000000 |
/* 20,000,000 */ |
void dropBomb(void); |
/* prototype */ |
void delay(void); |
|
int deaths; |
/* global variable */ |
int main() |
|
{ |
|
char x; |
|
deaths=0;
for(;;)
{
printf(“Press ~ then Enter to quit\n”); printf(“Press Enter to drop the bomb:”); x=getchar();
fflush(stdin); /* clear input buffer */ if(x==’~’)
{
break;
}
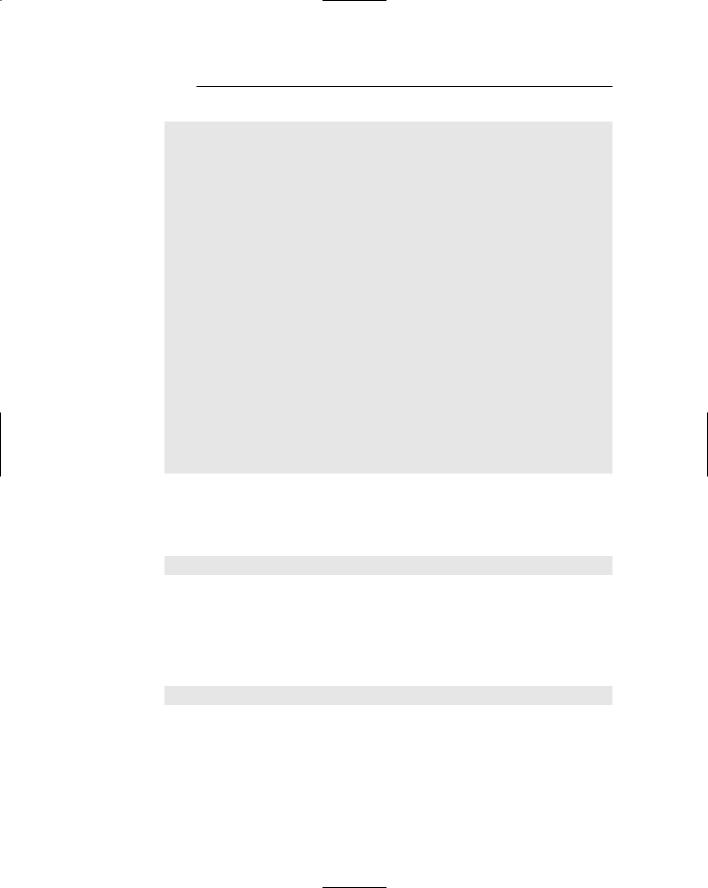
272 Part IV: C Level
dropBomb();
printf(“%d people killed!\n”,deaths);
}
return(0);
} |
|
void dropBomb() |
|
{ |
|
int x; |
|
for(x=20;x>1;x--) |
|
{ |
|
puts(“ |
*”); |
delay(); |
|
}
puts(“ BOOM!”); deaths+=1500;
}
void delay()
{
long int x;
for(x=0;x<COUNT;x++)
;
}
Bring up the source code for BOMBER.C in your editor. Make the necessary changes so that your code matches what’s shown here. Here are the details:
First, the global variable deaths is declared right before the main() function:
int deaths; |
/* global variable */ |
Second, the main() function is rewritten to contain an endless loop (refer to the preceding code block). The loop allows you to drop bombs over and over; pressing the ~ (tilde) key ends the loop.
Third, the global variable deaths is incremented inside the dropBomb() func tion by a simple addition to that function:
deaths+=1500;
Double-check your source code! Then, save BOMBER.C to disk one more time (though you don’t quite finish it until you read Chapter 22).
Compile and run the program.
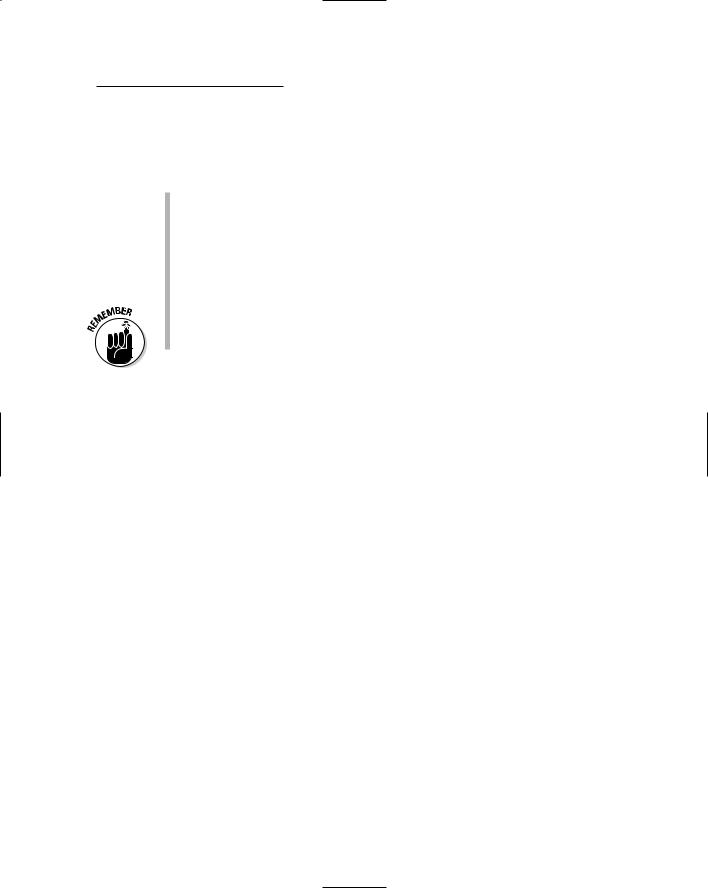
Chapter 21: Contending with Variables in Functions 273
The program effectively keeps a running tally of the dead by using the global deaths variable. The variable is manipulated (incremented) in the dropBomb() function and is displayed in the main() function. Both functions share that global variable.
See how the global variable is declared right up front? The #includes usually come first, and then the prototyping stuff, and then the global variables.
No, this game doesn’t challenge you.
For more information about the endless for loop, see Chapter 25.
The fflush() function is used to clear extra characters that getchar() might read. Refer to Chapter 13 for the details.
In Unix-like operating systems, be sure to use fpurge(stdin) in Line 20 rather than fflush(stdin). Refer to Chapter 13.
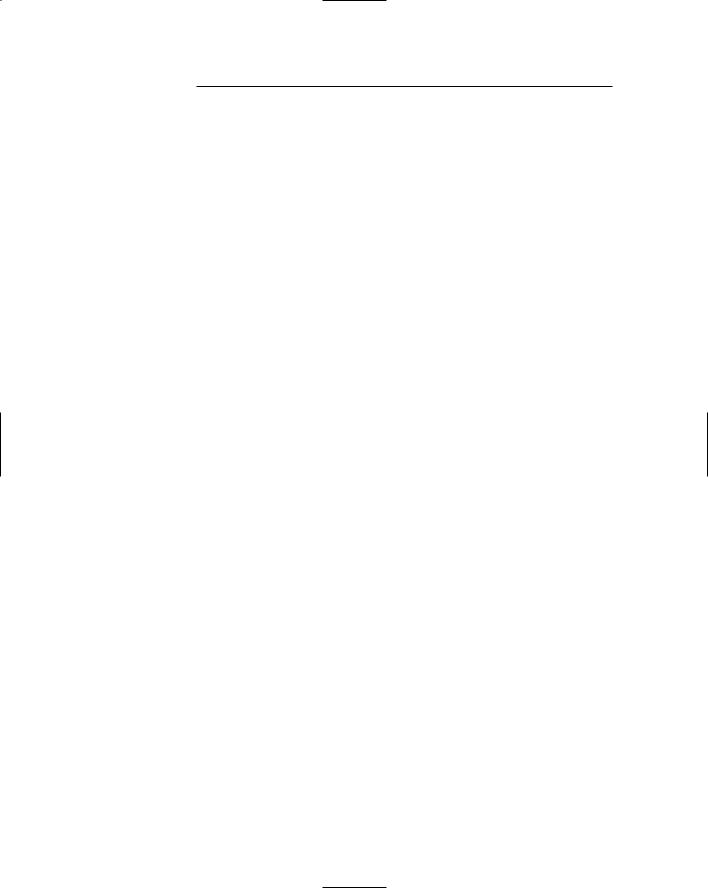
274 Part IV: C Level