
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
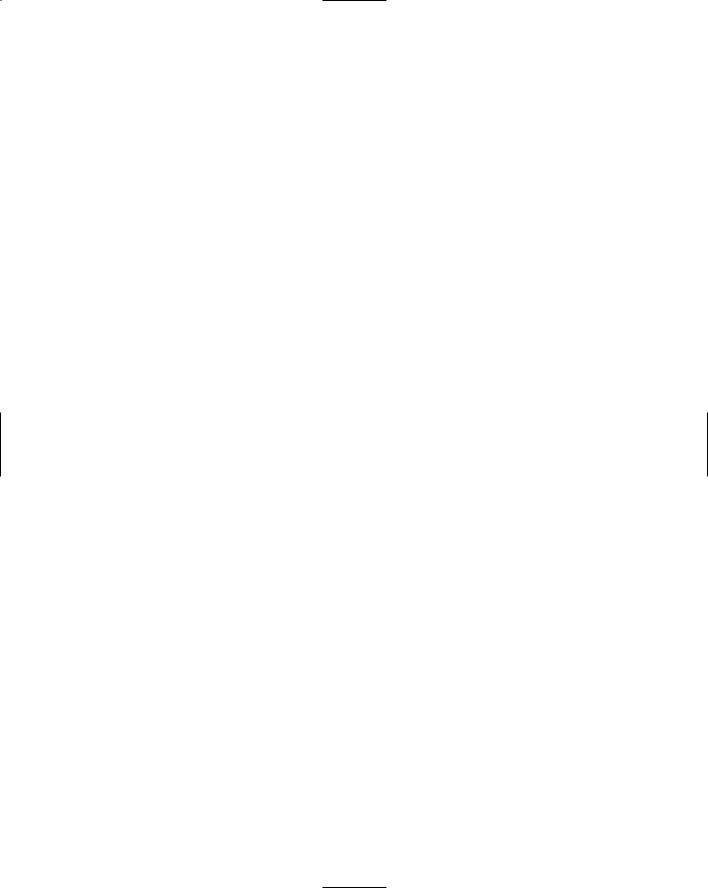
|
|
|
|
Chapter 28: Ten Tips for the Budding Programmer 351 |
|
|
|
|
|
||
|
|
|
|
|
|
|
Windows/DOS |
Unix |
What It Does |
|
|
|
cd |
pwd |
Displays the name of the current directory or folder. |
||
|
|
|
|
Use this command to ensure that you’re in the prog/ |
|
|
|
|
|
c/learn directory. |
|
|
type |
cat |
Displays a text file’s contents on the screen; follow |
||
|
|
|
|
type or cat with the name of the file you want |
|
|
|
|
|
displayed: |
|
|
|
|
|
type source.c |
|
|
|
|
|
cat source.c |
|
|
|
|
|
|
|
|
del |
rm |
Deletes a file; follow del or rm with the name of the |
||
|
|
|
|
file to delete, as in del bye.c or rm bye.c. |
|
|
exit |
exit |
Closes the command-prompt window and closes the |
||
|
|
|
|
terminal. |
|
|
|
|
|
|
|
Refer to a good book or reference about the command prompt for more details on these and other handy commands you can use while you program.
Carefully Name Your Variables
Though I use a lot of single-letter variable names in this book, be a better, wiser person when it comes to naming variables in your own programs. For example, x is an okay variable name, but counter is much better.
This may seem like a silly thing for a tiny program, but when your programs get larger, you may find that that a quick x or a variable you declared is being used by some other part of the program. Bad news!
Know Your Postand Pre-Incrementing
and Decrementing Riddles
The ++ and -- operators can certainly come in handy for incrementing or decrementing a variable’s value. But keep all your C language statements on a single line, and remember that ++ or -- before the variable name does its job before the variable’s value is used. If you put the ++ or -- after the variable name, the operation takes place afterward.
Refer to Chapter 25 to find out about this concept.

352 Part V: The Part of Tens
Breaking Out of a Loop
All your loops need an exit point. Whether that point is defined in the loop’s controlling statement or set inside the loop by using a break command, be sure that it’s there!
I recall many a time sitting at the computer and waiting for the program to “wake up,” only to realize that it was stuck in a loop I had programmed with no escape clause. This is especially easy to do when you work on larger pro grams with “tall” loops; after the source code for the loop extends past the height of your text editor, it’s easy to lose track of things.
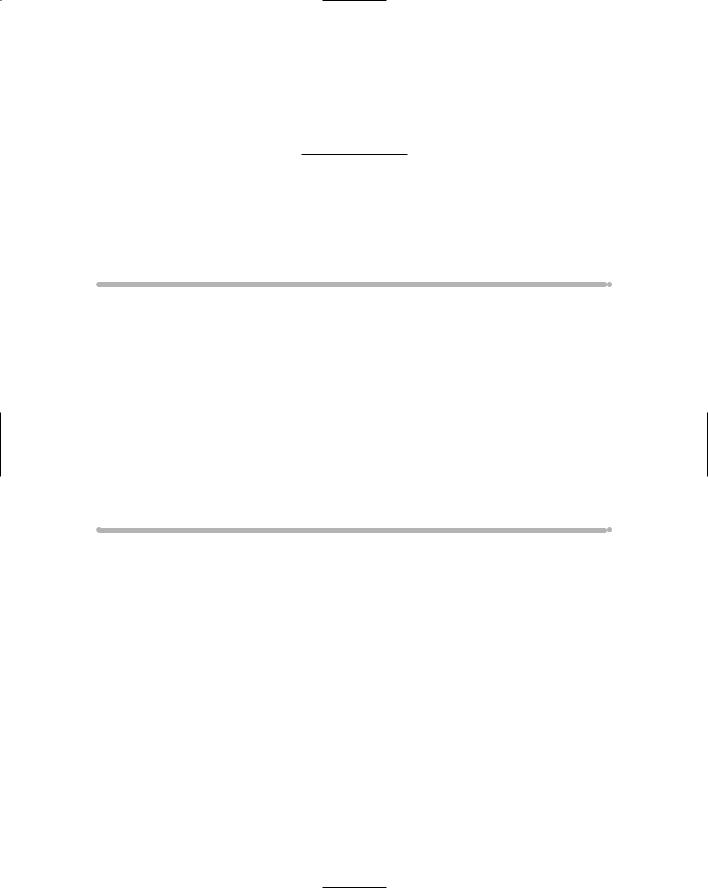
Chapter 29
Ten Ways to Solve Your Own Programming Problems
In This Chapter
Work on one thing at a time
Break up your code
Simplify your job
Talk through problems
Set breakpoints
Monitor variables
Document
Use debugging tools
Use an optimizer
Read more books!
Welcome to the world of debugging. In my travels, I’ve met only one pro grammer who could sit and code a whole project from scratch and
have it compile and run the first time. As it turns out, he was able to do it only once — and it was a project he was well familiar with. Although he’s one of the world’s best programmers, the dream of writing, compiling, and running, all in that order, remains a dream for most programmers.
Yes, your programs error. You have typos that the compiler shouts out at you. But your programs also have bugs. That is, they compile and link just fine, but when they run, they do unexpected things. Well, all programs obey their orders. It just happens that the programmer may forget something now and then. It’s those times when you need to turn to this chapter and review my ten ways of solving your own programming problems. Do this before you phone, e-mail, or post your problem to the public. The public will thank you!
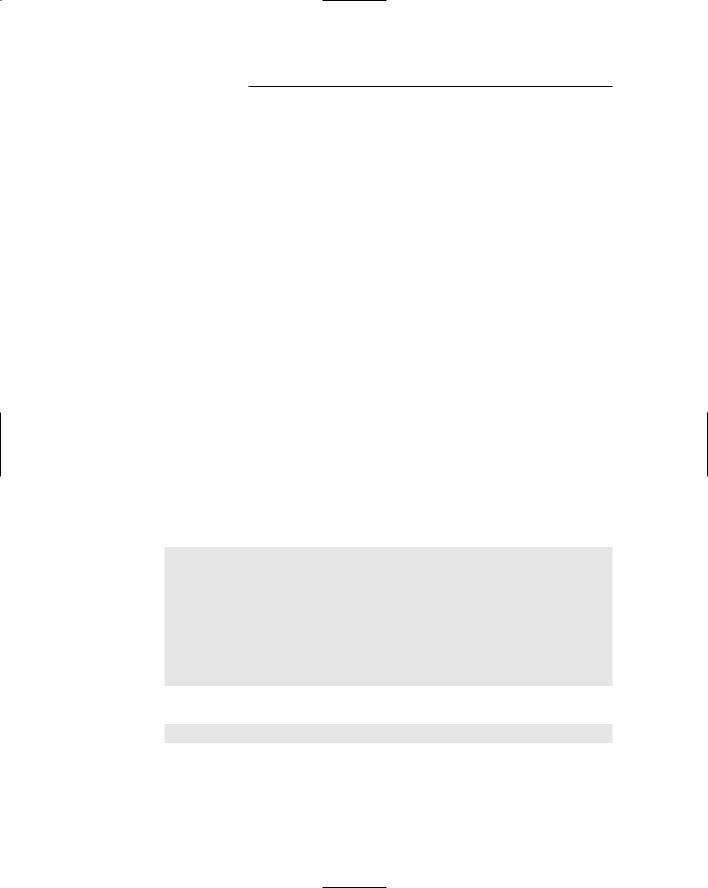
354 Part V: The Part of Tens
Work on One Thing at a Time
Address your bugs one at a time. Even if you’re aware that the program has several things wrong with it, fix them methodically.
For example: You notice that the title is too far to the right, random characters are at the bottom of the screen, and the scrolling technique doesn’t move the top row. Avoid the temptation to address all three issues in the same editing job. Instead, fix one problem. Compile and run to see how that works. Then fix the next problem.
The problem you run into when you try to fix too much at once is that you may introduce new errors. Catching those is easier if you remember that you were working on, for example, only Lines 173 and 174 of your source code.
Break Up Your Code
As your source code gets larger, consider breaking off portions into separate modules. I know that this topic isn’t covered in this book — and it probably isn’t a problem you will encounter soon — but separate modules can really make tracking bugs easy.
Even if you don’t use modules, consider using comments to help visually break up your code into separate sections. Even consider announcing the purpose of each section, such as
/********************************************* Verification function
---------------------
This function takes the filename passed to it and confirms that it’s a valid filename and
that a file with that name doesn’t already exist.
Returns TRUE/FALSE as defined in the header.
*********************************************/
I also put a break between functions, just to keep them visually separated:
/********************************************/