
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
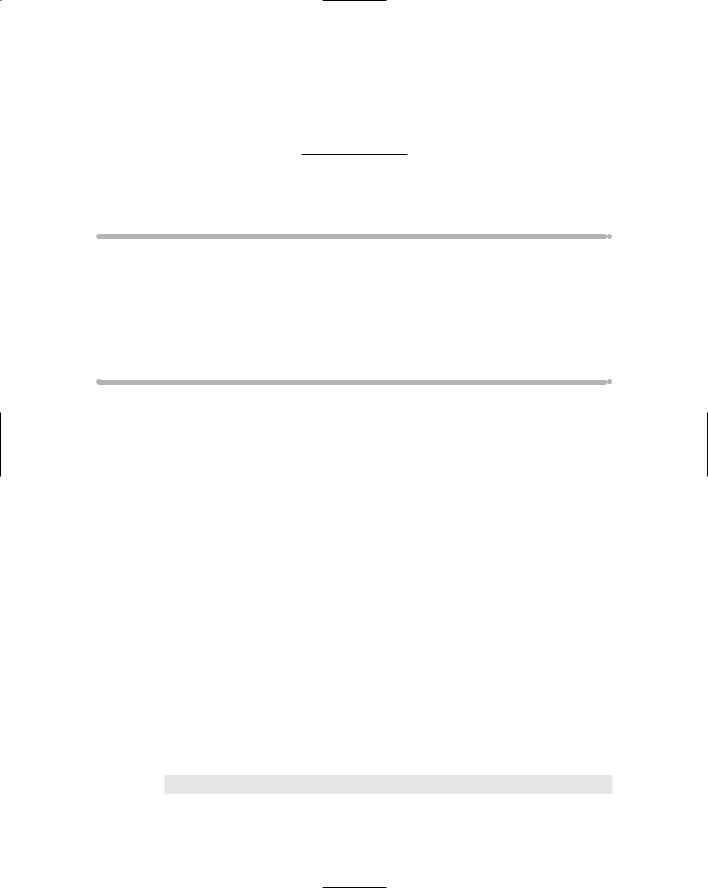
Chapter 18
Do C While You Sleep
In This Chapter
Introducing the do-while loop
Using a delay loop
Nesting loops
Creating a grid
Understanding the continue keyword
You’re not done looping!
You’re not done looping!
You’re not done looping!
You’re not done looping!
The for and while commands may be an odd couple, and they may be the offi cial looping keywords of the C language, but that’s not the end of the story. No, there’s more than one way to weave a loop, particularly a while loop. This chapter covers this oddity of nature, even showing you a few situations where the mythical “upside-down” while loop becomes a necessity.
The Down-Low on Upside-Down do-while Loops
A while loop may not repeat — no, not ever. If the condition it examines is false before the loop starts, the block of statements designed to repeat is skipped over like so many between-meal snacks would be if you really knew what’s in them.
Witness the cold, cruel while statement:
while(v==0)
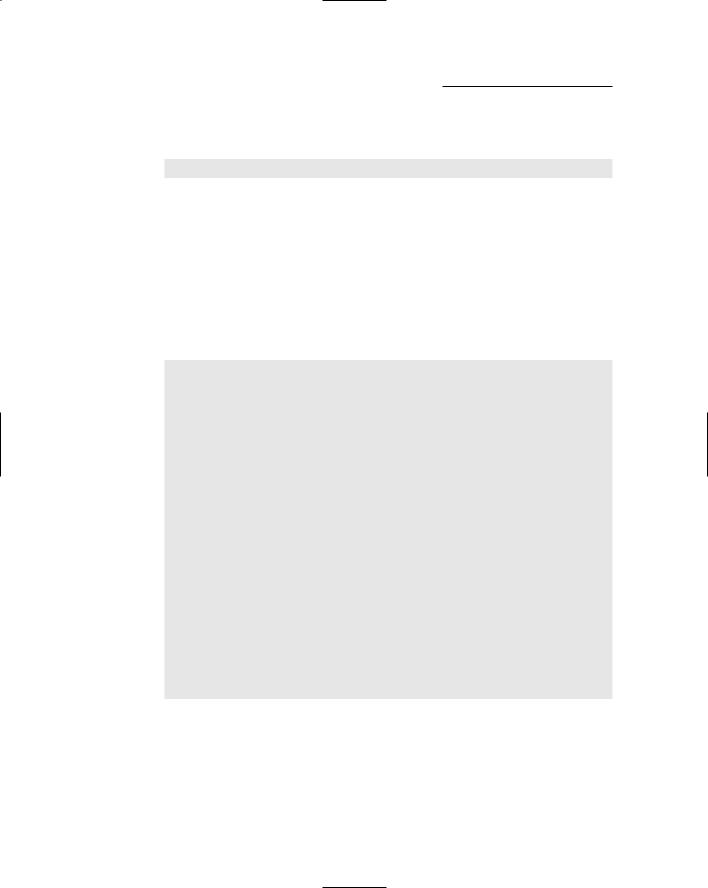
226 Part III: Giving Your Programs the Ability to Run Amok
If v doesn’t equal 0, the statements clinging to the underside of the while loop are skipped, just as though you had written this:
if(v==0)
There’s an exception, of course — a kind of loop you can fashion that always executes at least once. It’s the upside-down while loop, called a do-while loop. This type of loop is rare, but it has the charming aspect of always want ing to go through with it once.
The devil made me do-while it!
The following program contains a simple do-while loop that counts backward. To add some drama, you supply the number it starts at:
/* An important program for NASA to properly launch America’s spacecraft. */
#include <stdio.h>
int main()
{
int start;
printf(“Please enter the number to start\n”); printf(“the countdown (1 to 100):”); scanf(“%d”,&start);
/* The countdown loop */
do
{
printf(“T-minus %d\n”,start); start--;
}
while(start>0);
printf(“Zero!\nBlast off!\n”); return(0);
}
Type this source code into your editor. Save the file to disk as COUNTDWN.C.
Compile. Fix any errors. Notice that a semicolon is required after the end of the do-while loop. If you forget it, you get an error.
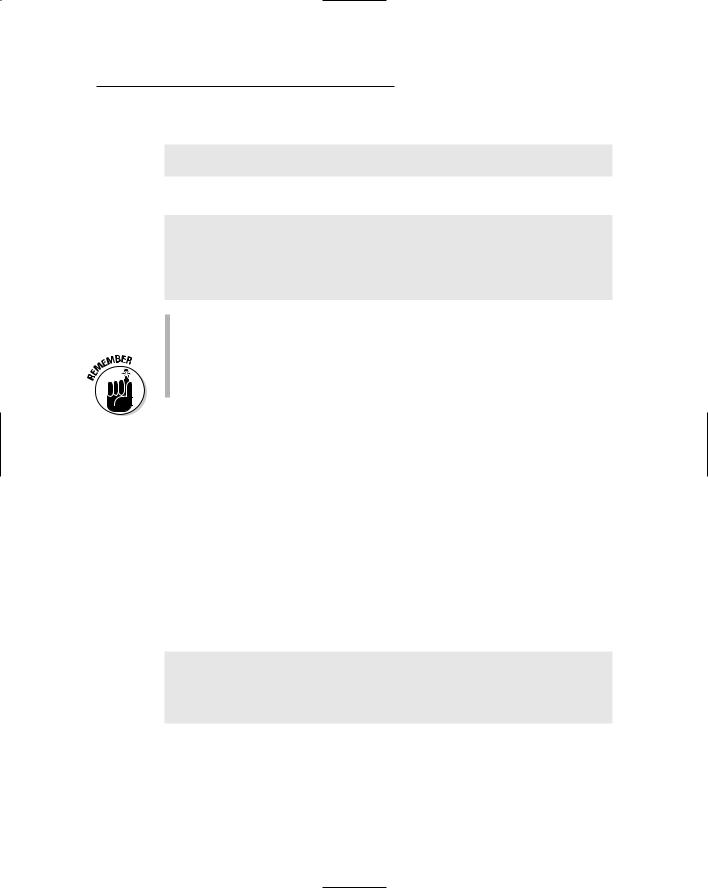
Chapter 18: Do C While You Sleep 227
Run the program.
Please enter the number to start
the countdown (1 to 100):
Be a traditionalist and type 10. Press Enter:
T-minus 10
T-minus 9
et cetera . . .
T-minus 1
Zero!
Blast off!
The do while loop executes once, no matter what.
In a do-while loop, a semicolon is required at the end of the monster, after while’s condition (see Line 21 in the program).
Don’t forget the ampersand (&), required in front of the variable used in a scanf statement. Without that & there, the program really screws up.
do-while details
A do-while loop has only one advantage over the traditional while loop: It always works through once. It’s as though do is an order: “Do this loop once, no matter what.” It’s guaranteed to repeat itself. If it doesn’t, you’re entitled to a full refund; write to your congressman for the details.
The do keyword belongs to while when the traditional while loop stands on its head. The only bonus is that the while loop always works through once, even when the condition it examines is false. It’s as though the while doesn’t even notice the condition until after the loop has wended its way through one time.
Here’s the standard format thing:
do
{
statement(s);
}
while(condition);
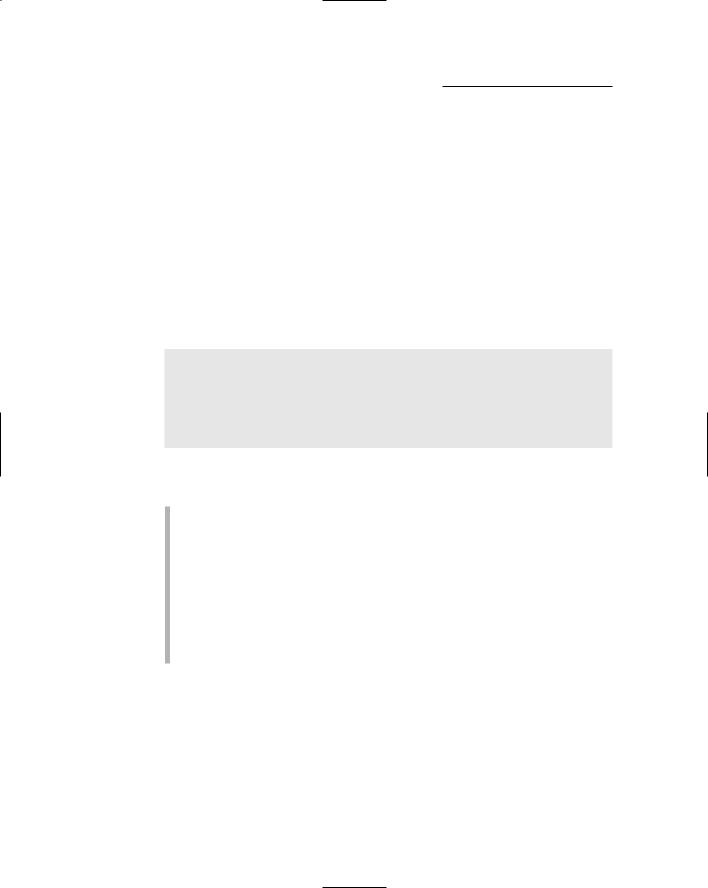
228 Part III: Giving Your Programs the Ability to Run Amok
The condition is a true-or-false comparison that while examines — the same type of deal you find in an if comparison. If the condition is true, the statements in the loop are repeated. They keep doing so until the condition is false, and then the program continues. But, no matter what, the statements are always gone through once.
An important thing to remember here is that the while at the end of the loop requires a semicolon. Mess it up and it’s sheer torture later to figure out what went wrong.
One strange aspect of the do-while loop is that it seriously lacks the starting, while-true, and do-this aspects of the traditional while and for loops. It has no starting condition because the loop just dives right into it. Of course, this sentence doesn’t mean that the loop would lack those three items. In fact, it may look like this:
starting; do
{
statement(s); do_this;
}
while(while_true);
Yikes! Better stick with the basic while loop and bother with this jobbie only when something needs to be done once (or upside down).
The condition that while examines is either TRUE or FALSE, according to the laws of C, the same as a comparison made by an if statement. You can use the same symbols used in an if comparison, and even use the logical doodads (&& or ||) as you see fit.
This type of loop is really rare. It has been said that only a mere 5 percent of all loops in C are of the do-while variety.
You can still use break to halt a do-while loop. Only by using break, in fact, can you halt the statements in the midst of the loop. Otherwise, as with a while or for loop, all the statements within the curly braces repeat as a single block.
A flaw in the COUNTDWN.C program
Run the COUNTDWN program again. When it asks you to type a number, enter 200.
There isn’t a problem with this task; the program counts down from 200 to 0 and blasts off as normal. But, 200 is out of the range the program asks you to type.