
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
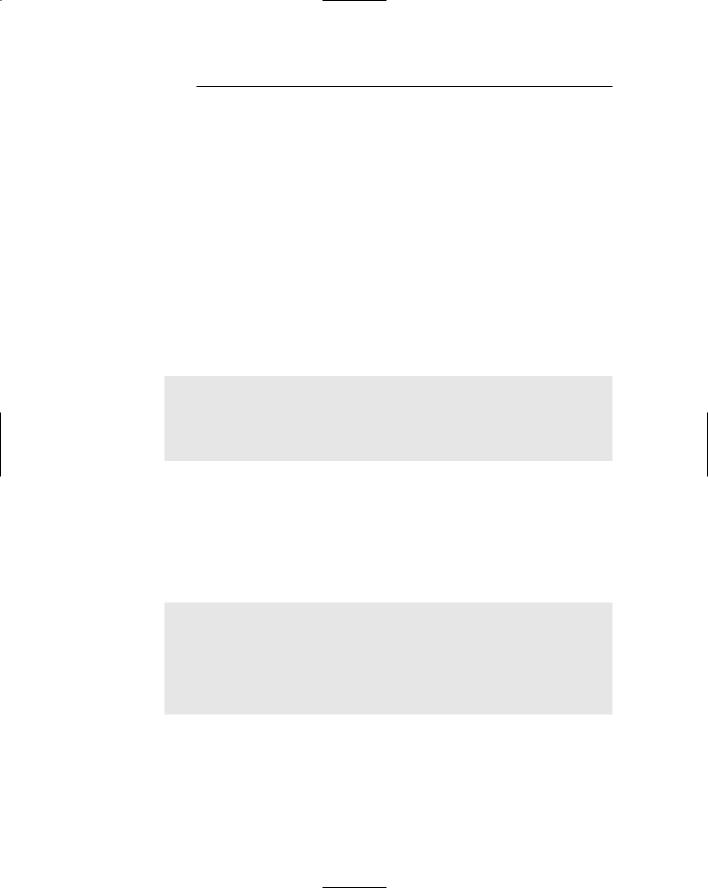
298 Part IV: C Level
Search through the file for some old friends, such as printf(), to see how it’s defined and prototyped inside the header file.
Writing your own dot-H file
You have absolutely no need to write your own header file. Not now, not ever. Of course, if you dive deeply into C, you may one day write some multimodule monster and need your own custom header, one that you can proudly show your fellow C Masters and one that they will ponder and say, jealously and guardedly, “I wish I could do that.”
To get a feel for it, and because this chapter would be unduly short other wise, create the following header file, HEAD.H, in your editor. Type the lines exactly as written, matching lowercase for lowercase and uppercase for uppercase:
/* This is my wee li’l header file */
#define HAPPY 0x01
#define BELCH printf #define SPIT { #define SPOT }
Save the file to disk as HEAD.H. You have to type the dot-H, lest your editor save it to disk with a C, TXT, or DOC extension.
You don’t compile or “run” a header file. Instead, you use it by #include-ing it in your source code. This type of program is listed next. Granted, it may not look at all like a C program. In fact, you may just shake your head in dis gust and toss this book down right now. Please, I beg, give me a few moments before you run off all huffy to Mr. Software and buy a Visual Basic book:
#include <stdio.h> #include “head.h”
int main()
SPIT
BELCH(“This guy is happy: %c\n”,HAPPY); return(0);
SPOT
Start over on a new slate in your editor. Type the preceding source code exactly. The second #include brings in your header file; note how it has head.h in double quotes. That’s how the compiler finds your header file instead of looking for it with the other, traditional headers. Also, don’t let the SPIT, BELCH, SPOT stuff toss you. It’s explained later.
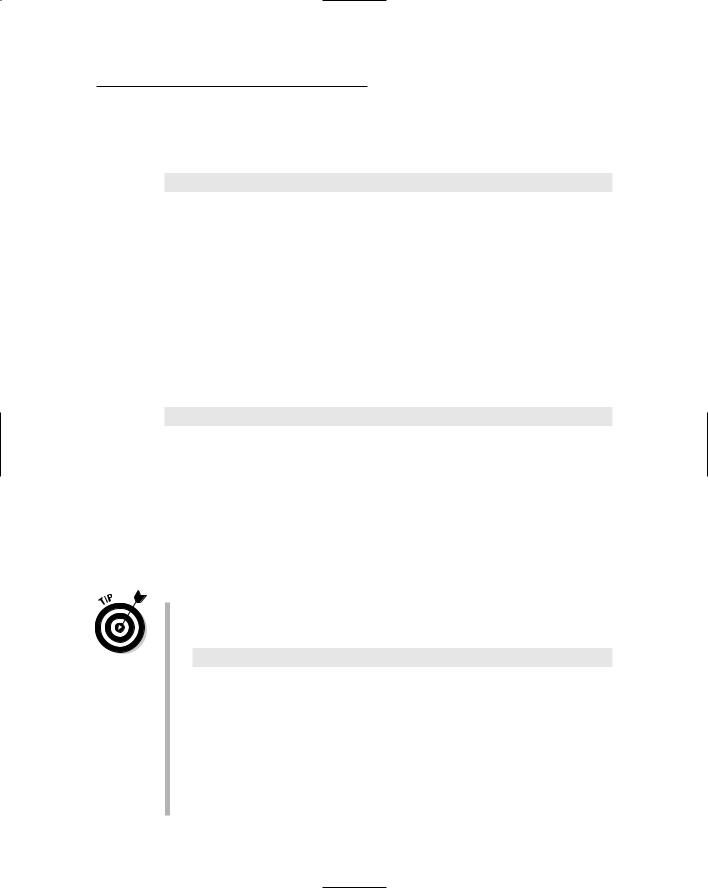
Chapter 23: The Stuff That Comes First 299
Save the file to disk and name it HTEST.C. Compile and run. Don’t be stunned if you find no errors. Believe it or not, everything should work out just fine. Here’s a peek at what the output should look like:
This guy is happy: J
Mr. HAPPY is happy. You may be happy too, after understanding what went on. Here’s the blow-by-blow:
The second #include (Line 2) brings into your source code the HEAD.H file you may have created earlier in this chapter. All the instructions in that file are magically included with your source code when HTEST1.C is compiled (as are the instructions from the standard I/O header file, STDIO.H).
If you recall, inside the HEAD.H header file are a few of those #define direc tives, which tell the compiler to substitute certain characters or C language words with happy euphemisms. (Refer to your HEAD.H file, if you need to.)
For example, the word SPIT was defined as equal to the left curly brace. So, SPIT is used in the program rather than the first curly brace in Line 5:
SPIT
The word BELCH was defined to be equal to the word printf, so it serves as a substitute for that function as well in Line 6, and in Line 8 you see the word SPOT used rather than the final curly brace.
Just about anything in a C program can wend its way into your own, personal header files. #define statements are the most popular. Also allowed are com ments, variable definitions, advanced things called structures, and even source code (though that’s rare). Remember that because it’s eventually copied into your source code, anything that normally would go there can also go into a header file.
Mostly, any header file you write yourself contains a lot of #defines. A doozy I wrote for one program, for example, listed all the #defines for strange key combinations on the keyboard. For example:
#define F1 0x3B00
This line allows me to use the characters F1 rather than have to remem ber (or constantly look up) the ugly value the computer understands as the F1 key.
Can’t remember the code for the happy face? Just #define it in your own header file and use it instead, which is what is done here.
There’s no reason to redefine C language words and functions (unless you want to goof someone up). However, the first #define in HEAD.H sets the word HAPPY equal to the value of the PC’s happy-face character,
0x01.
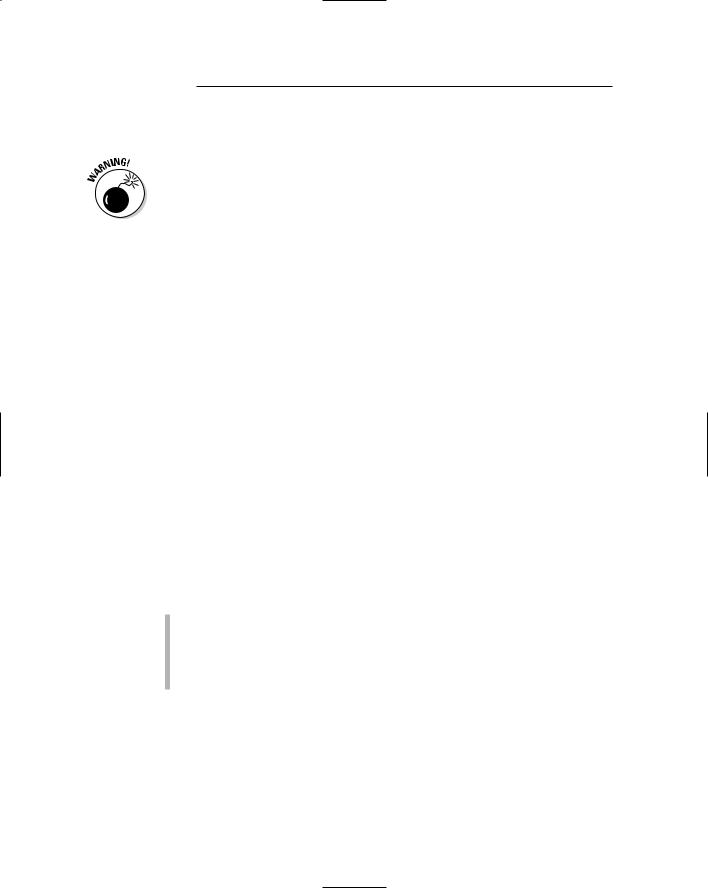
300 Part IV: C Level
A final warning about header files
The typical header file used in C contains lots of information. What it doesn’t contain, however, is programming code. By that, I mean that although the printf() function may be defined, prototyped, massaged, and oriented inside the STDIO.H file, the programming code that carries out printf()’s task isn’t in the header file.
I mention this because occasionally a reader writes to me and says something along the lines of “Dan! I need the DOS.H header file in order to compile an older DOS program. Can you find it for me?” My answer is that even if I could find it, the header file alone does not help. You also need the library file in order to do the job.
Library files are like object code: They contain instructions for the micro processor, telling it how to go about doing certain tasks. The instructions for printf() to do its thing are contained in a standard C language library file, not in the header file.
Figure 23-3 illustrates the process. First, you take your source code, named HOLLOW.C in the figure. STDIO.H is #included, so it’s combined with the source code by the compiler. The result is an object file named HOLLOW.O (or HOLLOW.OBJ).
To turn the object file into a program file, a library is added. The library con tains the instructions for the C language functions. These are carefully linked into your source code so that a final program, HOLLOW (or HOLLOW.EXE) is produced.
It’s the library that contains the code, not the header file! If you want to com pile an older DOS program, you need an older DOS library to link into your source code. Without it, the header file really means nothing.
Library files live in the LIB folder, which is usually found right next to the INCLUDE folder. For example, in Unix, the /usr/lib folder dwells right next-door to the /usr/include folder.
More information on using library files is in C All-in-One Desk Reference For Dummies (Wiley).
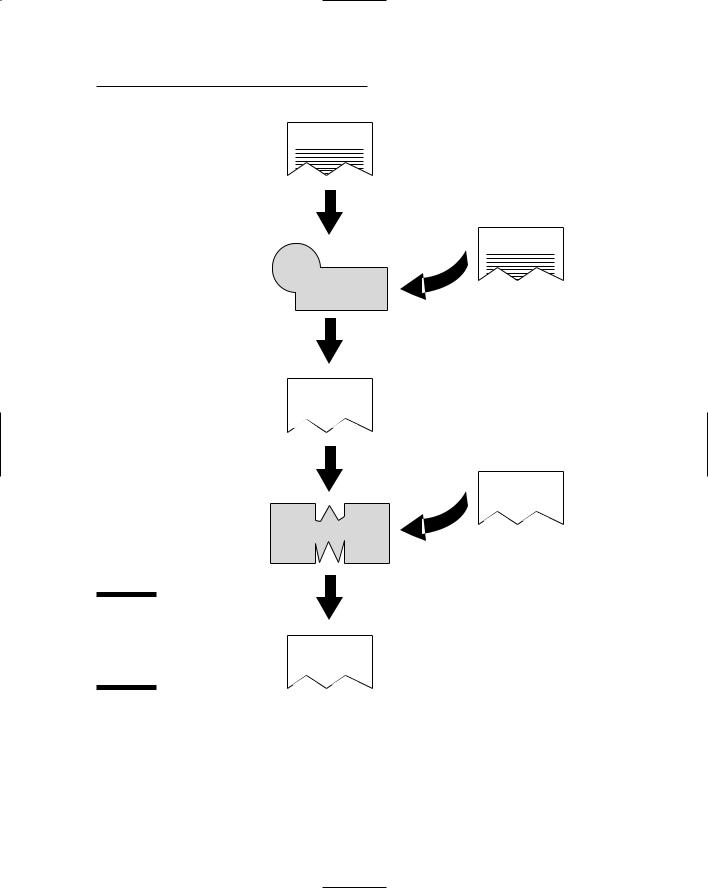
Source code
(gcc)
Object code
(gcc)
Figure 23-3:
How library files fit into
the big Executable file picture.
Chapter 23: The Stuff That Comes First 301
HOLLOW.C
STDIO.H
Compiler
HOLLOW.O
1010101010100
1101001111010
1001010010110
LIBC.A
1010101010100
1101001111010
1001010010110
Linker
HOLLOW
1010101010100
1101001111010
1001010010110