
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
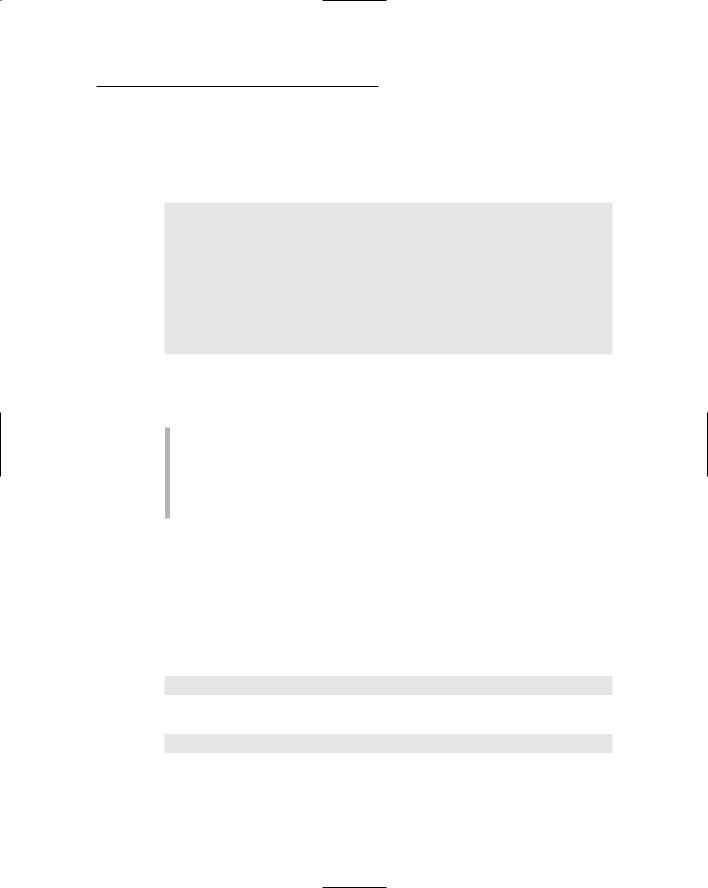
Chapter 16: C the Loop, C the Loop++ 205
Quit pondering and type.
Save the file to disk as OLLYOLLY.C. Compile it. Run it.
The output looks like this:
10
9
8
7
6
5
4
3
2
1
Ready or not, here I come!
Yes, indeed, the computer can count backward. And, it did it in a for loop with what looks like a minimum of fuss — but still a little techy. The following sec tion discusses the details.
To prove that you’re not going crazy, refer to Chapter 15’s 100.C program. It counted from 1 to 100 using a for loop. The only difference now, aside from the printf() statements, is that the loop worked itself backward.
Backward-counting loops in C are rare. Most of the time, whenever you have to do something 10 times, you do it forward, from 0 through 9 or from 1 through 10 or however you set up your for loop.
O, to count backward
Counting backward or forward makes no difference to the computer. You just have to tell the C language in which direction you want to go.
To count forward, you increment a variable’s value. So, if you have the vari able f, you do this:
f=f+1;
or even this:
f++;
Either way, the value of variable f is 1 greater than it was before; it has been incremented.
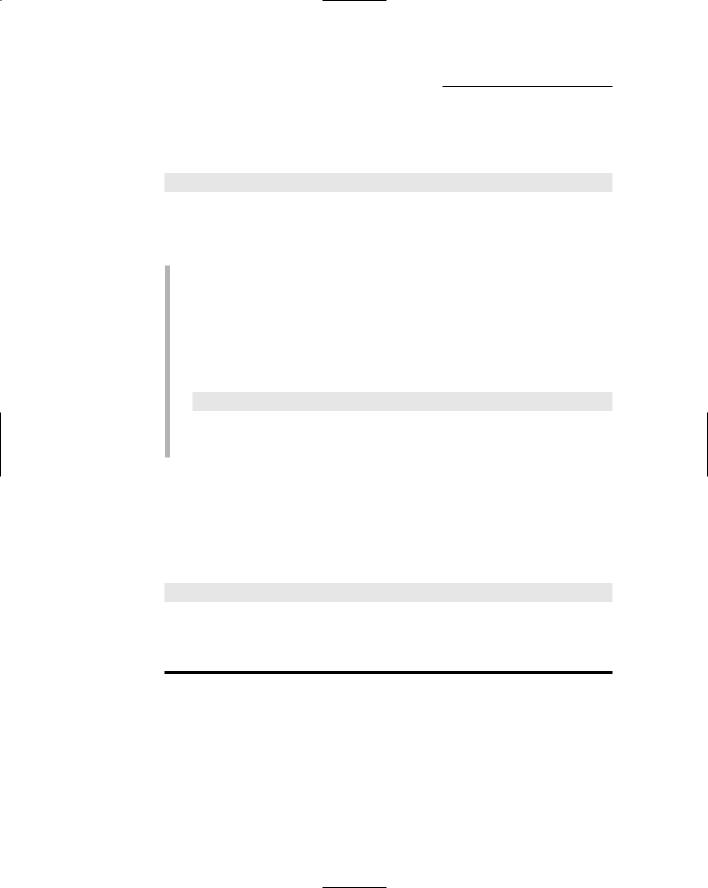
206 Part III: Giving Your Programs the Ability to Run Amok
To count backward, you subtract 1 from a variable’s value, which is exactly the way you do it in your head: 10, 9, 8, 7, and so on. It looks identical to the incrementing statement, except for the minus sign:
b=b-1;
The value of variable b is 1 less than it was before. If b came in with a value of 5, this statement sets b’s value to 4. This process is known as decrementing a variable’s value.
Decrementing, or subtracting 1 (or any number) from a variable’s value is just common subtraction. The only big deal here is that decrementing is done in a loop, which makes the loop count backward.
Incrementing means adding (1) to a variable’s value.
Decrementing means subtracting (1) from a variable’s value.
Decrementing works because C first figures out what’s on the right side of the equal sign:
b=b-1;
First comes b-1, so the computer subtracts 1 from the value of variable b.
Then, that value is slid through the equal signs, back into the variable b.
The variable is decremented.
How counting backward fits into the for loop
Take another look at Line 7 from the OLLYOLLY.C program:
for(count=10;count>0;count=count-1)
It’s basic for loop stuff. The loop has a starting place, a while-true condition, and a do-this thing. The parts are listed in Table 16-1.
Table 16-1 |
How the for Loop Counts Backward |
Loop Part |
Condition |
Starting |
count=10 |
|
|
While-true |
count>0 |
|
|
Do-this |
count=count-1 |
|
|
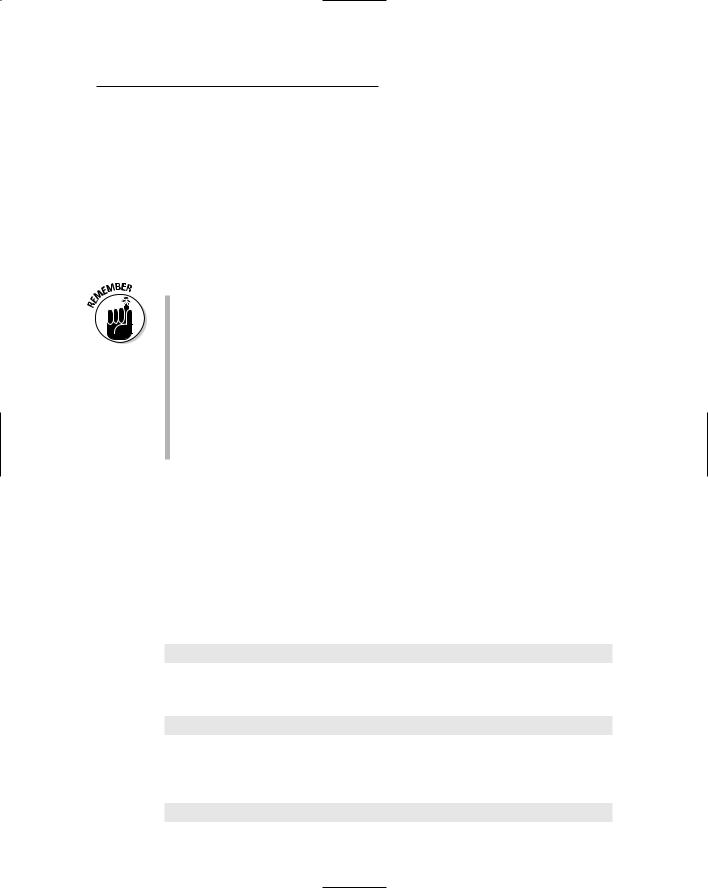
Chapter 16: C the Loop, C the Loop++ 207
Everything in the backward-counting for loop works per specifications. The loop begins by setting the value of the count variable equal to 10. And, it loops as long as the value of the count variable is greater than 0 (count>0). But, each time the loop repeats, the value of the count variable is decremented. It works backward.
Again, you have no reason to loop backward — except that the printf() state ment belonging to the loop displays the numbers 10 through 1 in a countdown manner. Normally (which means about 99 percent of the time), you only loop forward. It’s not only easier to do in your head, but it’s also less likely to be a source for programming boo-boos than when you try to loop backward.
Most loops count forward. The backward-counting loop in C is possible but rarely used, mostly because counting is counting and it’s just easier (for humans) to do it forward.
You can’t do a backward loop without decrementing the loop’s variable.
Other than decrementing the loop’s variable, the only difference is in the loop’s while-true condition (the one in the middle). That requires a little more mental overhead to figure out than with normal for loops. It’s another reason that this type of loop is rare.
Okay. The question arises: “Why bother?” Because you have to know about decrementing and the cryptic -- operator, covered in the next section.
Cryptic C operator symbols, Volume II:
The dec operator (--)
Just as C has a shortcut for incrementing a variable’s value, there is also a shortcut for decrementing a variable’s value. (If you read the first half of this chapter, you saw this coming from a mile back, most likely.) As a quick review, you can add 1 to a variable’s value — increment it — by using the following C language statement:
i=i+1;
Or, if you’re cool and remember the shortcut, you can use the cryptic ++ opera tor to do the same thing:
i++;
Both examples add 1 to the value of variable i.
Consider this example:
d=d-1
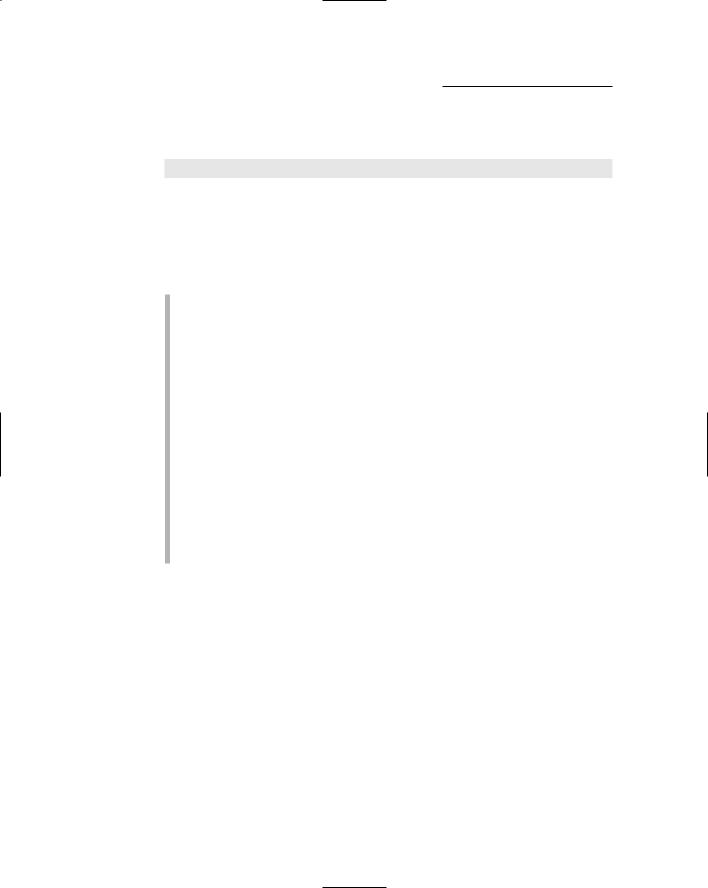
208 Part III: Giving Your Programs the Ability to Run Amok
This statement subtracts 1 from the value of variable d, decrementing it. Here’s the whiz-bang, cryptomic shortcut:
d––;
The -- is the decrement operator, which subtracts 1 from a variable’s value.
Just like the incrementation operator, ++, the decrementing operator, --, works like other mathematical operators in the C language. In keeping with the theme of being cryptic, however, there is no equal sign. The -- tells the compiler to subtract 1 from the associated variable’s value and — poof! — it’s done.
I pronounce -- as “deck deck,” though I may be the only one in the world who does so. I haven’t heard anyone say “minus-minus” — at least not aloud.
The equation d-- is the same as d=d-1.
You suffer no penalty for using d=d-1 if you forget about the -- thing.
Don’t bother with an equal sign when you use the decrementing opera tor, --. Glue it to the end of the variable you want to decrement and you’re done.
Here we go (again):
|
var=3; |
/* the value of variable var is three */ |
|
var--; |
/* Whoa! var is decremented here */ |
|
|
/* Now the value of var equals two */ |
The -- operator is used this way in a for looping statement: |
||
|
|
|
|
for(d=7;d>0;d--) |
|
|
The d-- replaces the d=d-1, as I demonstrate in the past few sections. |
A final improvement to OLLYOLLY.C
Now that you know about --, you can improve the awkward and potentially embarrassing OLLYOLLY.C program, by spiffing it up with the decrementation operator.
Load OLLYOLLY.C into your editor again (if it’s not there right now) and clickety-clack the down-arrow key to Line 7, where the for loop starts. Edit the for statement there, replacing the count=count-1 part with the cryptic, though proper, count-- thing. Here’s how that line should look when you’re done editing: