
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
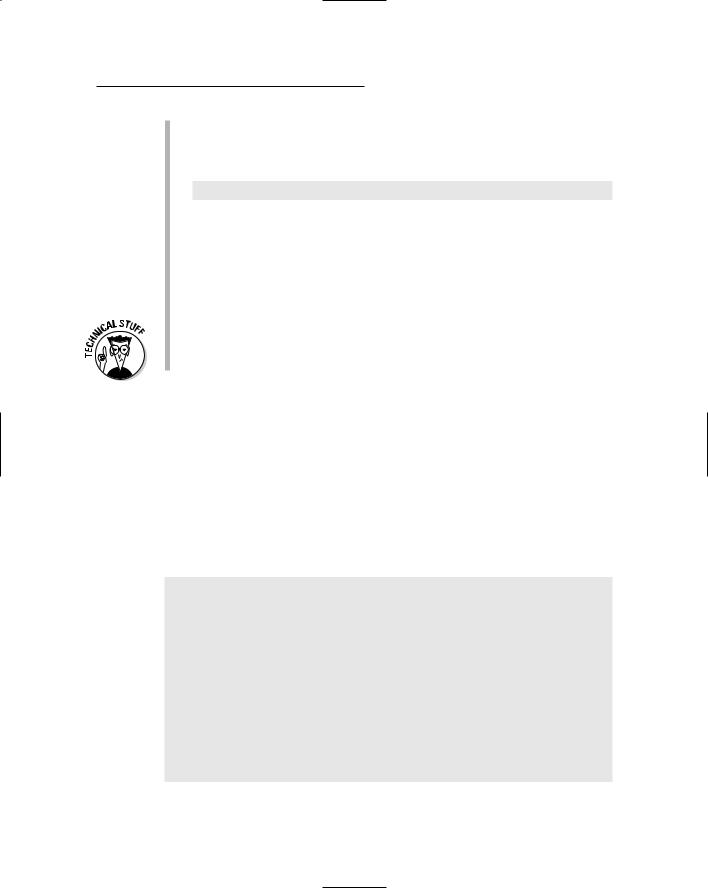
Chapter 20: Writing That First Function 255
Some functions return a value. That is, they produce something that your program can use, examine, compare, or whatever. The getchar() func tion returns a character typed at the keyboard, which is typically stored in a character variable; thus:
thus=getchar();
Some functions require parentheses stuff and return a value.
Other functions (such as playAlex()) neither require parentheses stuff nor return a value.
Functions are nothing new. Most C programs are full of them, such as printf(), getchar(), atoi(), and others. Unlike your own functions, these functions are part of the compiler’s library of functions. Even so, the functions you write work just like those others do.
Creating a function doesn’t really add a new word to the C language. How ever, you can use the function just like any other function in your pro grams; printf() and scanf(), for example.
A potentially redundant program in need of a function
I like to think of functions as removing the redundancy from programs. If any thing must be done more than once, shuffle it off into a function. That makes writing the program so much easier. It also breaks up the main() function in your source code (which can get tediously long).
The following sample program is BIGJERK1.C, a litany of sorts devoted to some one named Bill, who is a jerk:
#include <stdio.h>
int main()
{
printf(“He calls me on the phone with nothing say\n”); printf(“Not once, or twice, but three times a day!\n”); printf(“Bill is a jerk!\n”);
printf(“He insulted my wife, my cat, my mother\n”); printf(“He irritates and grates, like no other!\n”); printf(“Bill is a jerk!\n”);
printf(“He chuckles it off, his big belly a-heavin’\n”); printf(“But he won’t be laughing when I get even!\n”); printf(“Bill is a jerk!\n”);
return(0);
}
Type this program into your editor. Double-check all the parentheses and double quotes. They’re maddening. Maddening!
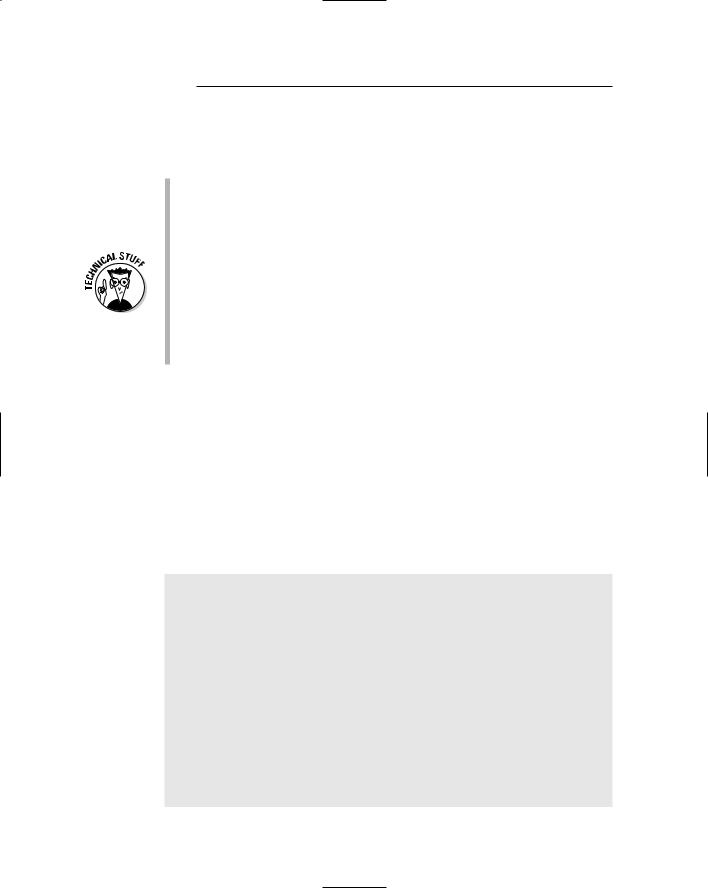
256 Part IV: C Level
Compile and run BIGJERK1.C. It displays the litany on the screen. Ho-hum.
Nothing big. Notice that one chunk of the program is repeated three times.
Smells like a good opportunity for a function.
Most of the redundancy a function removes is much more complex than a simple printf() statement. Ah, but this is a demo.
None of the Bills I personally know is a jerk. Feel free to change the name Bill into someone else’s name, if you feel the urge.
In the olden days (and I’m showing my age here), every byte in a program
was vital. A message such as Bill is a jerk repeated over and over meant that precious bytes of data were being wasted on a silly text string. Ancient programmers, such as myself, honed their skills by removing excess bytes from programs like this one. Shaving a program’s size from 4,096 bytes to 3,788 bytes was considered a worthy accomplishment. Of course, with today’s mega-/gigacomputers, saving space like that is con sidered trivial.
The noble jerk() function
It’s time to add your first new word to the C language — the jerk() function. Okay, jerk isn’t a C language word. It’s a function. But you use it in a program just as you would use any other C language word or function. The compiler doesn’t know the difference — as long as you set everything up properly.
Next, the new, improved “Bill is a jerk” program contains the noble jerk() function, right in there living next to the primary main() function. This pro gram is a major step in your programming skills — a moment to be savored. Pause to enjoy a beverage after typing it:
#include <stdio.h>
int main()
{
printf(“He calls me on the phone with nothing say\n”); printf(“Not once, or twice, but three times a day!\n”); jerk();
printf(“He insulted my wife, my cat, my mother\n”); printf(“He irritates and grates, like no other!\n”); jerk();
printf(“He chuckles it off, his big belly a-heavin’\n”); printf(“But he won’t be laughing when I get even!\n”); jerk();
return(0);
}
/* This is the jerk() function */

Chapter 20: Writing That First Function 257
jerk()
{
printf(“Bill is a jerk\n”);
}
Type the source code for BIGJERK2.C in your editor. Pay special attention to the formatting and such. Save the file to disk as BIGJERK2.C. Compile and run. The output from this program is the same as the first BIGJERK program.
Depending on your compiler, and how sensitive it has been set up for error reporting, you may see a slew of warning errors when you’re compil ing BIGJERK2.C: You may see a no prototype error; a Function should return a value error; a ‘jerk’ undefined error; or even a no return value error.
Despite the errors, the program should run. Those are mere “warning” errors — violations of C language protocol — not the more deadly, fatal errors, which means that the program is unable to work properly.
I show you how to cover — and cure — the errors in a few sections. Hold fast.
How the jerk() function works in BIGJERK2.C
A function works like a magic box. It produces something. In the case of the jerk() function in BIGJERK2.C, it produces a string of text displayed on the screen. Bill is a jerk — like that.
In the BIGJERK2.C program, the computer ambles along, executing C language instructions as normal, from top to bottom. Then, it encounters this line:
jerk();
That’s not a C language keyword, and it’s not a function known to the com piler. The computer looks around and finds a jerk() function defined in your source code. Contented, it jumps over to that spot and executes those state ments in that function. When it reaches the last curly brace in the function, the computer figures that it must be done, so it returns to where it was in the main program. This happens three times in BIGJERK2.C.
Figure 20-1 illustrates what’s happening in a graphic sense. Each time the com puter sees the jerk() function, it executes the commands in that function. This works just as though those statements were right there in the code (as shown in the figure) — which, incidentally, is exactly how the BIGJERK1.C program works.