
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
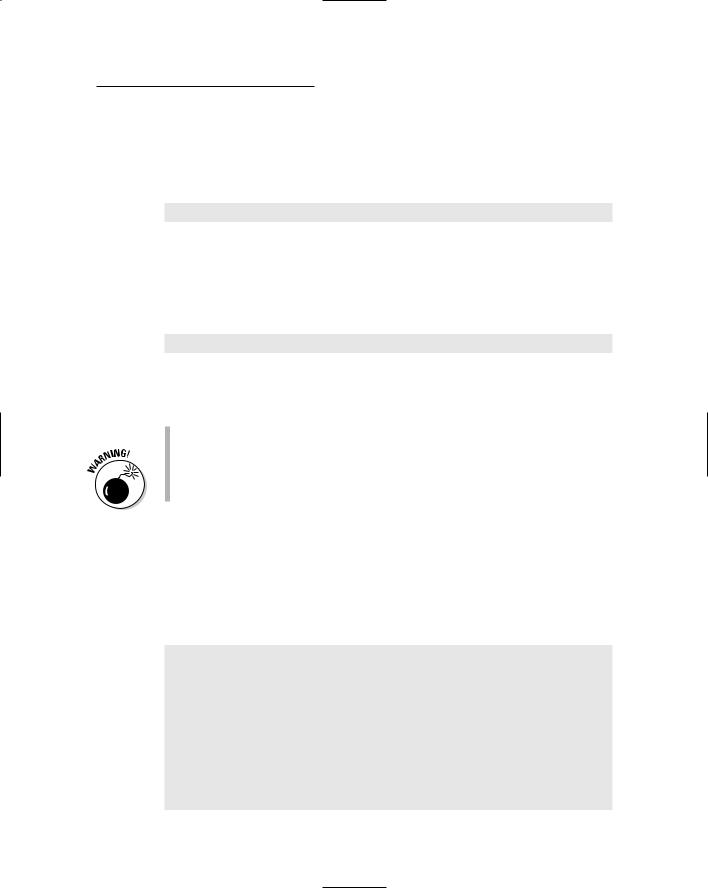
Chapter 26: The Old Random-Number Function 329
the random numbers more random. To plant the seed, you use the srand() function.
The srand function is used to help kick off the computer’s random-number machine in a more random manner. Here’s the format:
void srand((unsigned)seed)
The seed value is an unsigned integer value or variable, ranging from 0 up to 65,000-something. It’s that value the compiler uses to help seed the random- number-generation equipment located in the bowels of your PC.
You must include the following line at the beginning of your source code to make the srand() function behave:
#include <stdlib.h>
Because the rand() function already requires this line, you have no need to specify it twice (unless you’re just seeding the random-number generator out of some perverse horticultural lust).
The (unsigned) deal is used to ensure that the number srand() uses is of the unsigned type (not negative). It’s known as type casting.
Using the value 1 (one) to seed the random-number generator causes the compiler to start over, by using the same, uninspirational numbers you witness when srand() isn’t used. Avoid doing that, if possible.
Randoming up the RANDOM program
Now comes the time for some really random numbers. The following source code is for RANDOM2.C, a mild modification to the original program. This time, a new function is added, seedrnd(), which lets you reset the randomnumber generator and produce more random numbers:
#include <stdio.h> #include <stdlib.h>
int rnd(void); void seedrnd(void);
int main()
{
int x;
seedrnd();
puts(“Behold! 100 Random Numbers!”);
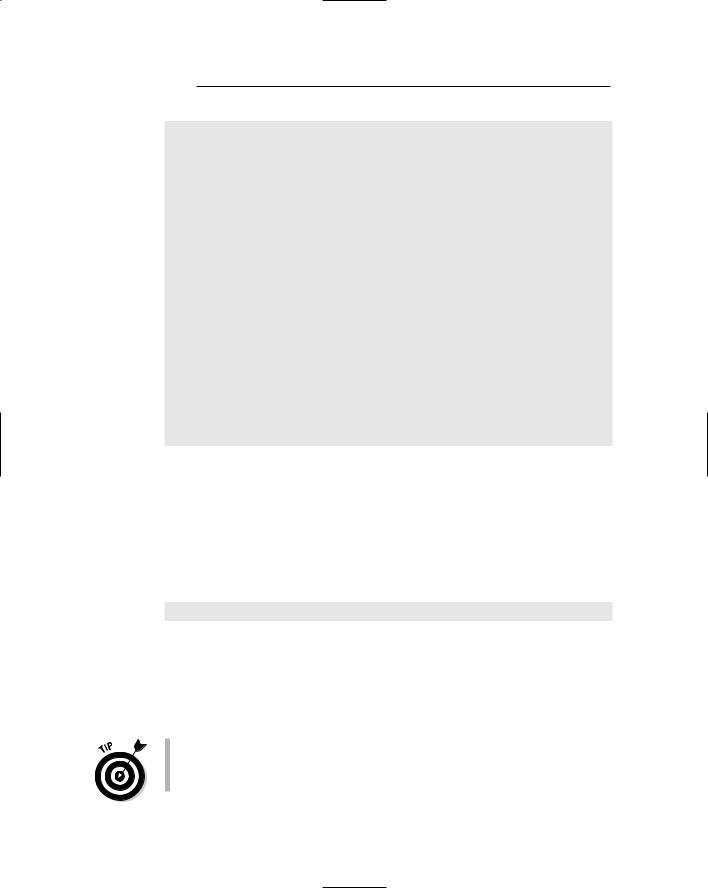
330 Part IV: C Level
for(x=0;x<100;x++)
printf(“%d\t”,rnd());
return(0);
}
int rnd(void)
{
int r;
r=rand();
return(r);
}
/* seed the random number */
void seedrnd(void)
{
int seed; char s[6];
printf(“Enter a random number seed (2 - 65000):”); seed=(unsigned)atoi(gets(s));
srand(seed);
}
Type this program into your editor. You can start by editing the RANDOM1.C source code. Add the prototype for seedrnd() up front, and then insert the call to seedrnd() in the main() function. Finally, tack the seedrnd() func tion itself to the end of the source code. Double-check the whole thing before you save it to make sure that you don’t leave anything out.
Use your editor’s Save As command to save the file to disk as RANDOM2.C.
Compile and run. You see this line:
Enter a random number seed (2-65000):
Type a number, from 0 up to 65,000-something. Press Enter and you see a new and more random bunch of numbers displayed.
The true test that it worked is to run the program again. This time, type a dif ferent number as the seed. The next batch of random numbers is completely different from the first.
You have to seed the randomizer only once, as this program does up in the main() function. Some purists insist on calling the seedrnd() func tion (or its equivalent) lots of times. Hey, random is random as random can be with a computer. No sense in wasting time.
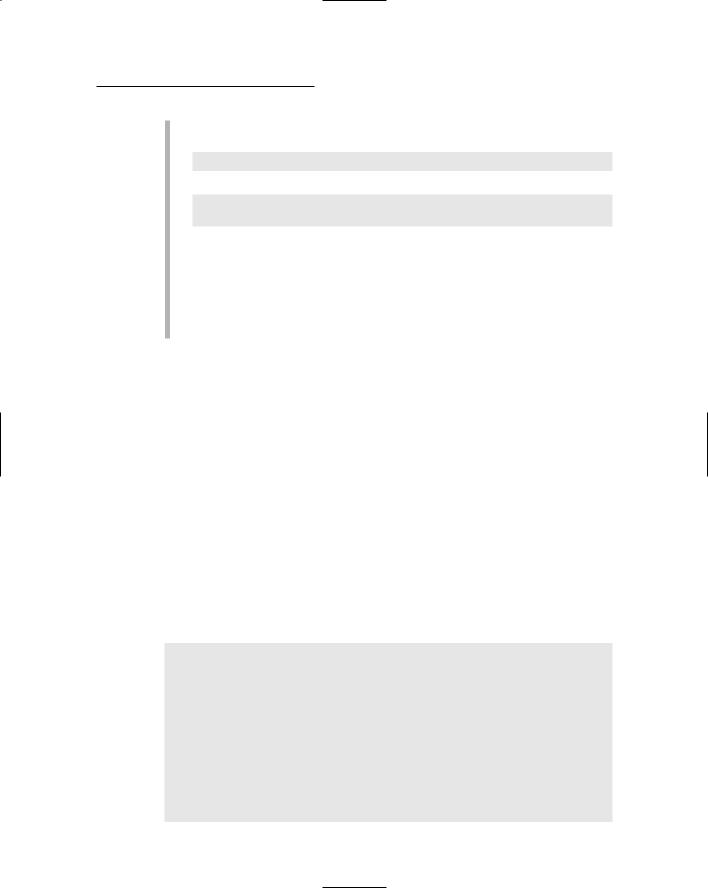
Chapter 26: The Old Random-Number Function 331
This program combines three C statements into one. The statement appears in Line 34:
seed=(unsigned)atoi(gets(s));
This line is a compilation of the following two statements:
gets(s);
seed=(unsigned)atoi(s);
First, gets() reads in a string variable. Second, that value is converted into an integer variable by the atoi function, which is passed over to the seed variable in the proper unsigned format.
There’s no need for the seedrnd() function to return any values. As I explain in Chapter 22, some functions neither require nor return values. This function is one of them. Yet, calling the function is important because it seeds the randomizer used elsewhere in the program.
Streamlining the randomizer
Nothing annoys me like a klutzy program. I’m talking not about how it’s writ ten, but, rather, about how it looks — its presentation. When a program must ask you to seed the randomizer, something is wrong. A computer should be smart enough to do that itself. And it can, as long as it finds a source of everchanging numbers that it can use to seed the randomizer.
One source is the computer’s clock. Most PCs keep time down to the hun dredths of seconds: Every 1⁄100th of a second, a new number is available to seed the randomizing gears. Heck, even the current second would be good enough to seed the randomizer. It just needs to be a number that’s different from one second to the next, that’s all.
The following source code is for the RANDOM3.C program. This program is nearly identical to RANDOM2.C except that the seedrnd() function now uses the current time to seed the random-number generator:
#include <stdio.h> #include <stdlib.h> #include <time.h>
int rnd(void); void seedrnd(void);
int main()
{
int x;
seedrnd();
puts(“Behold! 100 Random Numbers!”);
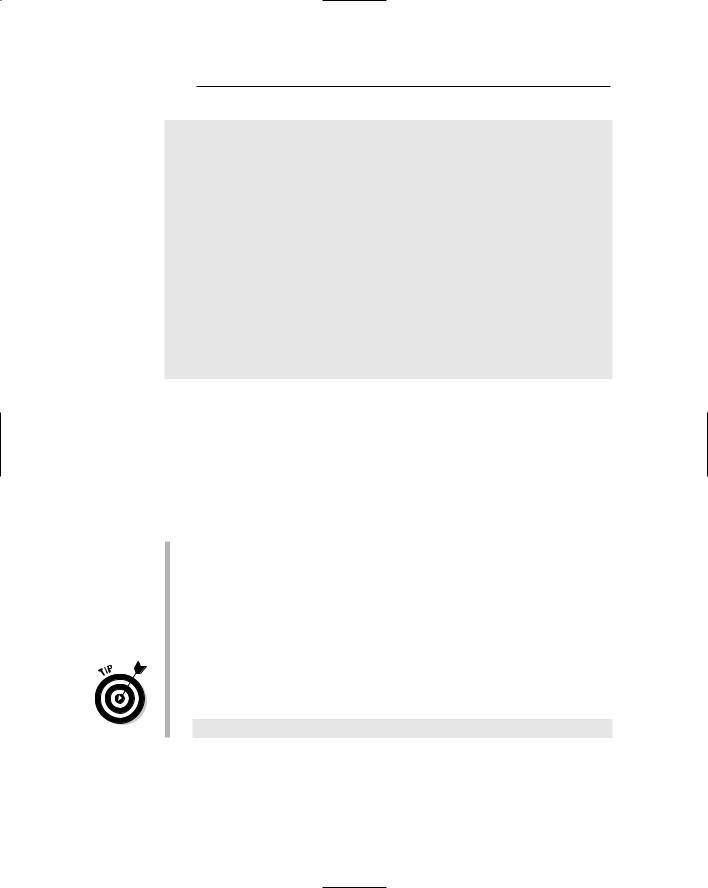
332 Part IV: C Level
for(x=0;x<100;x++)
printf(“%d\t”,rnd());
return(0);
}
int rnd(void)
{
int r;
r=rand();
return(r);
}
/* seed the random number */
void seedrnd(void)
{
srand((unsigned)time(NULL));
}
Create the source code for RANDOM3.C in your editor. You can start with RANDOM2.C. Add the new third line, #include <time.h>, which lets you use the computer’s clock to seed the random-number generator. Then, modify the seedrnd() function as shown in this block of code. Save the file to disk as RANDOM3.C by using your editor’s Save As command.
Compile and run the program. Notice that the output is similar and randomly different. What you don’t notice is the program begging you to seed the ran domizer. That’s all handled automatically now.
The time() function in Line 31 returns the time of day from the computer’s internal clock. The function is called by using the constant value NULL, which is essentially the value 0. (This constant is defined in the stdio.h header file — the #include <stdio.h> line sets it all up; see Chapter 23 for more information.)
The value returned by the time() function is some number, which isn’t important. What’s important is that it’s an unsigned integer value, which is what the (unsigned) type cast does.
No matter what, know that the following statement in any of your C pro grams properly and somewhat randomly seeds the random-number- generating apparatus:
srand((unsigned)time(NULL));
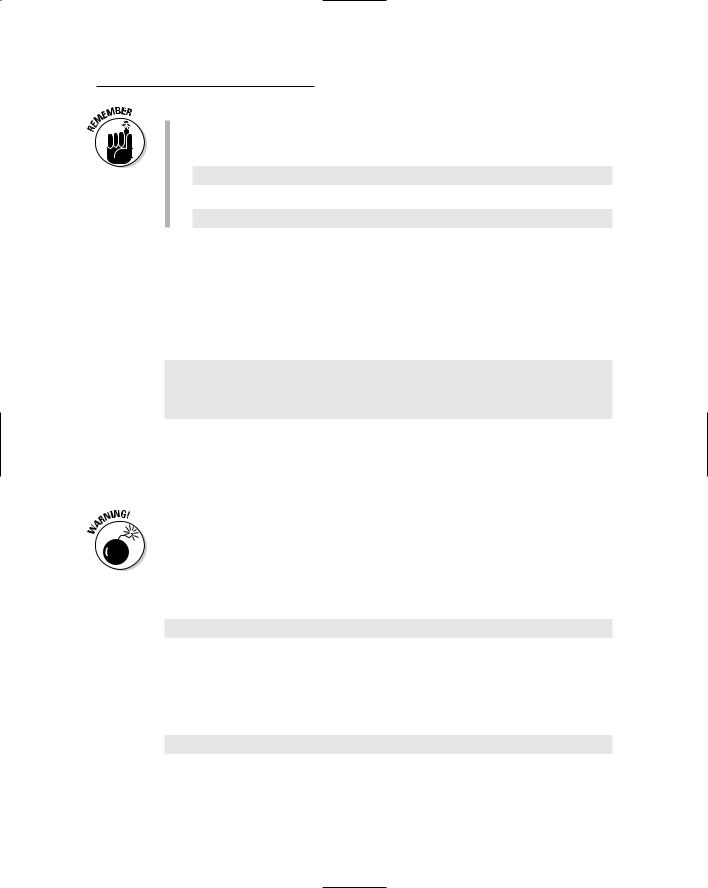
Chapter 26: The Old Random-Number Function 333
The time() function (and therefore the preceding randomizing seeding statement) requires the following statement at the beginning of your source code:
#include <time.h>
And, this one:
#include <stdlib.h>
The Diabolical Dr. Modulus
You may have noticed that the random numbers produced by the RANDOM series of programs have been wildly random and often unsuitable. For example:
Your turn, BOB.
You rolled a 23415 on the dice. This lands you on Boardwalk,
but you’ve passed Go 585 times, which nets you a gross of $117,000!
That gets old after a while.
You need to have a way to round down the numbers — a hacksaw to chop off excess values and just give you numbers in a certain range, for example.
Lo, you have a way. It’s the mathematical concept modulus.
Ah-ooga! Ah-ooga! Mathematical concept alert! Ah-ooga!
Modulus — bah! This term is better known to you as “the remainder,” as in “When you divide 6 into 10, you get a remainder of 4.” Well, 6 gazinta 10 is modulo 4. It’s written in C as
4 = 10 % 6;
I don’t expect you to remember what a modulus is or does, nor do I expect you to know when to use modulus, or the ablative, modulo. The format is the same for any of C’s mathematical operators. What’s important to know is that you can use the modulus doohickey to pare down larger numbers into smaller, more convenient chunks.
modulus = big % little;
Read it this way: If you take the huge number big and divide it by the smaller number little, you get a remainder, which is the modulo value.
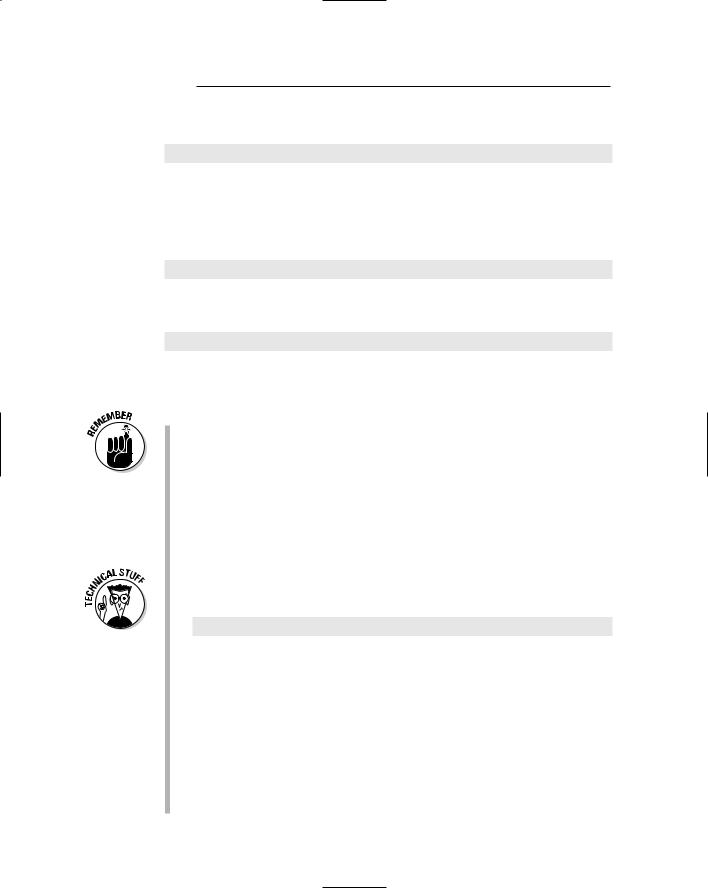
334 Part IV: C Level
Suppose that big is a big number in this statement:
m = blah % 5;
The values of variable m are in the range of 0 through 4, depending on the remainder of big divided by 5.
The values of variable m for the following statement are either 0 or 1, depend ing on whether oddoreven is even or odd, respectively:
m = oddereven % 2;
For example, a die has six sides. Suppose that the computer coughs up the random value 23,415. To pare it to a multiple of 6, you use this line:
dice1=23415 % 6;
The computer calculates how many times 6 gazinta 23,415. It then places the remainder in the dice1 variable. (The result is the number 3, which is a more realistic roll of a die than 23,415.)
If the second value is larger than the first, as in 5 % 10, the result is always equal to the second value. Therefore, you want the larger value to come first in a modulus operation.
The modulus operator is %, the percent sign. Pronounce it “mod.”
No math! The modulus is used to help you pare your random numbers. That’s all! You can dwell on the mathematical aspects of the % in other C language books.
Gazinta means “goes into.” I point it out here because my managing editor loathes it when I use nondictionary words.
If you want to pare a large random number as a roll of the dice, you need this equation:
dice1=(random_value % 6)+1;
The random_value the computer produces must be pared via % 6 (mod 6). It produces a number in the range of 0 to 5 (0 to 5 as a remainder — you can’t have a remainder of 6 when you divide by 6.) After the % calculation, you add 1 to the number and get a value in the range of 1 to 6, which are the true numbers on any given side of a die.
In the My Dear Aunt Sally theme of things, a modulus operation comes just after division and before addition. See the nearby Technical Stuff sidebar, “Introducing My Dear Mother’s Aunt Sally (Ugh!).”
“Ah, yes, Dr. Modulus. I’m familiar with your work in astrogenetics. Is it true that you got kicked out of the academy for engineering a third gender in mice?” “You read too much, lad.”
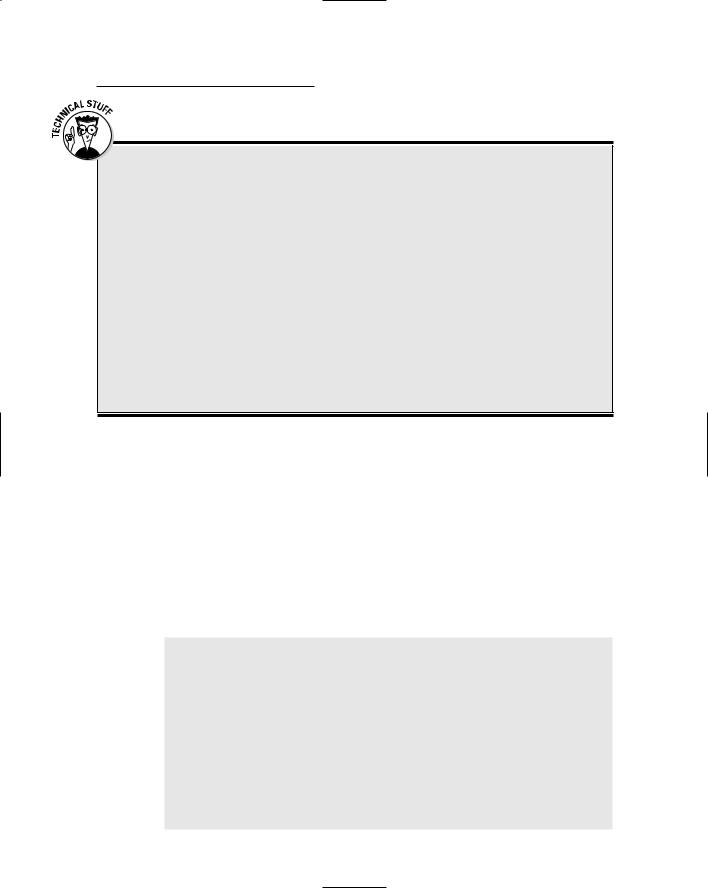
Chapter 26: The Old Random-Number Function 335
Introducing My Dear Mother’s Aunt Sally (Ugh!)
Did you read about My Dear Aunt Sally in Chapter 11? It’s a mnemonic device (a thing that makes you remember something) for multipli cation, division, addition, and subtraction, which is the order in which things get done in a long C math statement. Well, add to that the modulus operation, which takes precedence over addi tion and subtraction. It goes like this:
My Dear Mother’s Aunt Sally:
*Multiplication
/ Division
%Modulus
+Addition
–Subtraction
Therefore, the following statement to get a roll of the dice:
dice1=(23415 % 6)+1;
does the same thing without the parentheses:
dice1=23415 % 6+1;
The modulus operation (23415 % 6) comes first regardless, and then 1 is added to the result. Of course, putting the parentheses in there for readability’s sake is always forgivable.
Rolling the Dice with the Final
RANDOM Program
If you have been working through this chapter, you should have a whole pile of random numbers spewing forth from your computer, like New Yorkers flee ing their buildings during an August power outage. One more keen improve ment to the rnd() function remains. It’s time to add an automatic range-finder. The following program, RANDOM4.C (the last in the suite), has a rnd() func tion that coughs up random values only in the range of 0 to whatever number you specify. This program should bring some order to your random numbers:
#include <stdio.h> #include <stdlib.h> #include <time.h>
int rnd(int range); void seedrnd(void);
int main()
{
int x;
seedrnd();
for(x=0;x<100;x++)
printf(“%i\t”,rnd(10));

336 Part IV: C Level
return(0);
}
int rnd(int range)
{
int r;
r=rand()%range;
return(r);
}
void seedrnd(void)
{
srand((unsigned)time(NULL));
}
Create the source code for RANDOM4.C. Start with your RANDOM3.C pro gram, and make modifications per the source code just shown. Save the file to disk by using the name RANDOM4.C.
Compile and run the program. Here’s a sample of the output you may see:
4 |
1 |
3 |
0 |
6 |
6 |
1 |
0 |
8 |
9 |
2 |
9 |
5 |
9 |
8 |
7 |
6 |
8 |
0 |
9 |
5 |
6 |
2 |
0 |
5 |
8 |
5 |
5 |
9 |
0 |
9 |
9 |
2 |
6 |
1 |
2 |
0 |
2 |
0 |
7 |
8 |
4 |
4 |
7 |
1 |
6 |
0 |
0 |
5 |
1 |
3 |
7 |
1 |
2 |
1 |
2 |
5 |
0 |
8 |
5 |
9 |
2 |
0 |
7 |
9 |
8 |
4 |
5 |
6 |
0 |
8 |
8 |
7 |
6 |
0 |
8 |
3 |
9 |
3 |
4 |
0 |
4 |
0 |
5 |
5 |
6 |
3 |
0 |
4 |
3 |
7 |
6 |
1 |
2 |
2 |
7 |
6 |
7 |
4 |
8 |
Everything is in the range of 0 through 9, which is what the rnd(10) call does in Line 14.
The rnd() and seedrnd() functions become handy as you write your own C programs — specifically, games. Feel free to copy and paste these functions to other programs you may write. Remember that both require the #include <stdlib> directive, with seedrnd() also requiring
#include <time.h>.
To generate a roll of the dice, you stick the rnd() function in your pro gram and use this statement:
dice=rnd(6)+1; |
/* Roll dem bones! */ |
Using the ever-collapsing C language function ability, you can rewrite the rnd() function to only one statement:
return(rand()%range);
You’re now only moments from writing your own Monopoly game. . . .
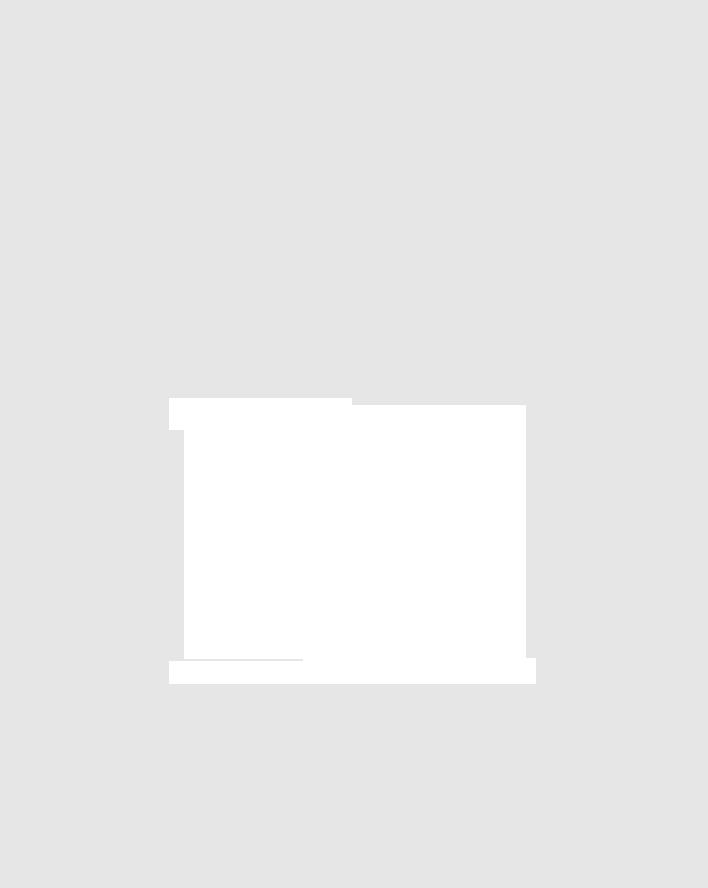
Part V
The Part of Tens
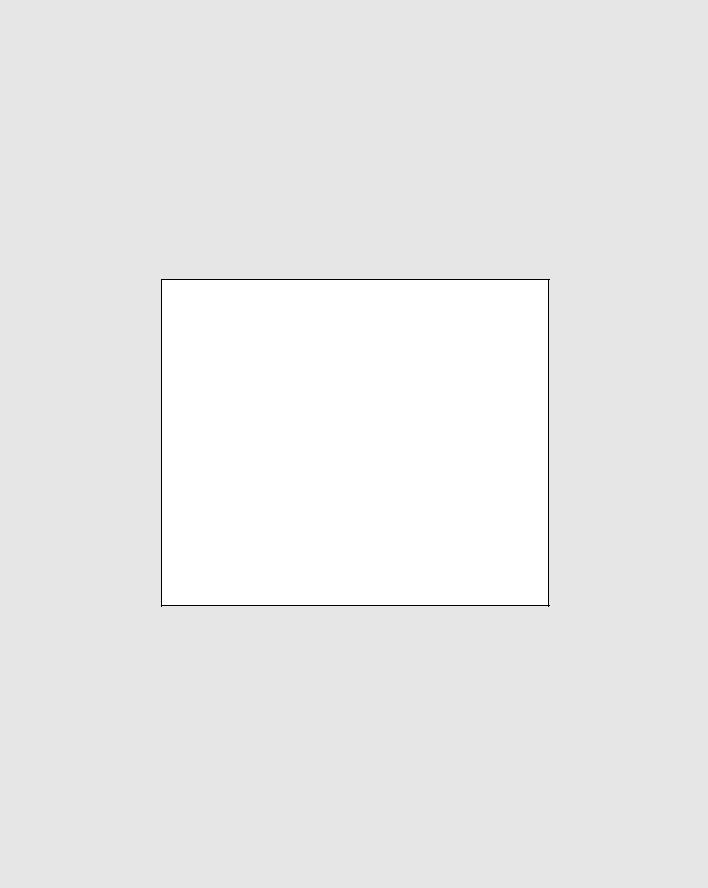
In this part . . .
for(item=1;item<11;item++)
{
printf(“This part of the book contains\n”); printf(“various lists; ten items to a\n”); printf(“chapter. It’s the traditional\n”); printf(“last part of a For Dummies
book.\n”);
printf(“In the chapters that follow, you’ll\n”);
printf(“find numerous lists, each with ten\n”);
printf(“items that offer tips, tricks, and\n”);
printf(“bonus information to help conclude\n”);
printf(“your first voyage into the\n”); printf(“C programming language.\n”);
}