
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
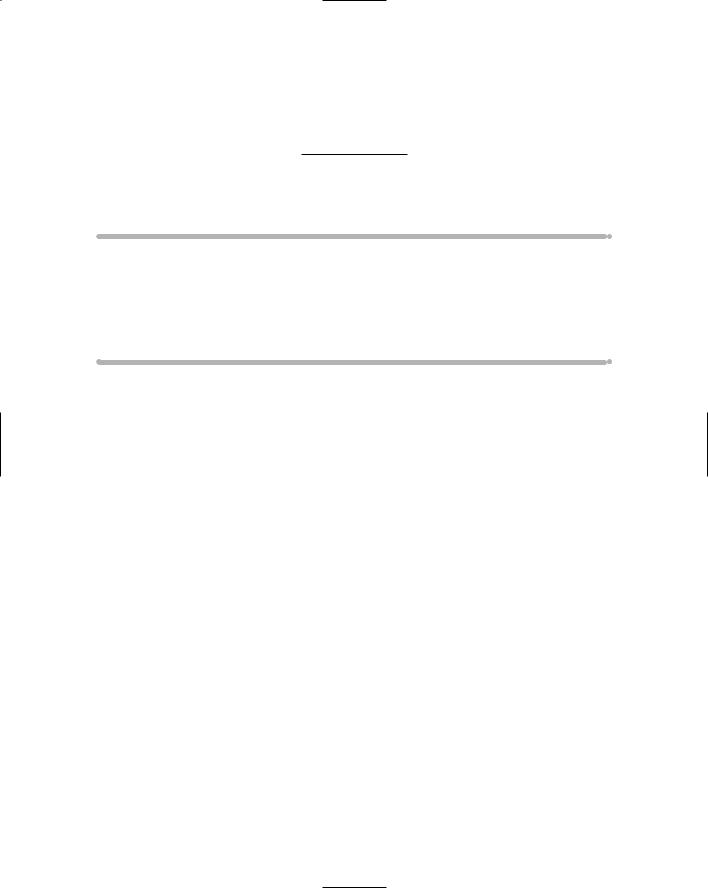
Chapter 26
The Old Random-Number Function
In This Chapter
Introducing the rand() function
Seeding the rand() function
Performing mod (%) calculations
Producing random numbers in a given range
The original plans for this book had it run about 1,600 pages. That’s because I never wanted to break the slow, easy pace with which the C language was taught. The problem is that you cannot fit 1,600 pages into a roughly 400-page
book without cutting something out. What to cut out?
You may think that it’s odd that I chose to cut what I did, yet kept this chapter on the random-number function. I did that because, believe it or not, random numbers are a big deal when it comes to programming a computer. They come in handy, for example, with computer games. No computer game would ever be possible without a random number here or there. Without them, the game would be predictable: “Oh, I’m passing by the planet Bothar again, and here is where the Omnivorous Space Slug tries to taunt me into a gavotte.”
On Being Random
What’s random? The next card drawn from a well-shuffled deck. The roll of a dice. The spin of a roulette wheel. Whether Cindy will overlook your huge beer gut as you attempt your first date after the divorce. These are all random, maybe-this-or-that events. If you want to include this random aspect in your programs, you need a special function that produces random numbers. After that’s done, no one can predict when the Enterprise will be attacked or when the tornado will whip through your village or whether Door Number 2 leads to the treasure this time or to certain peril.
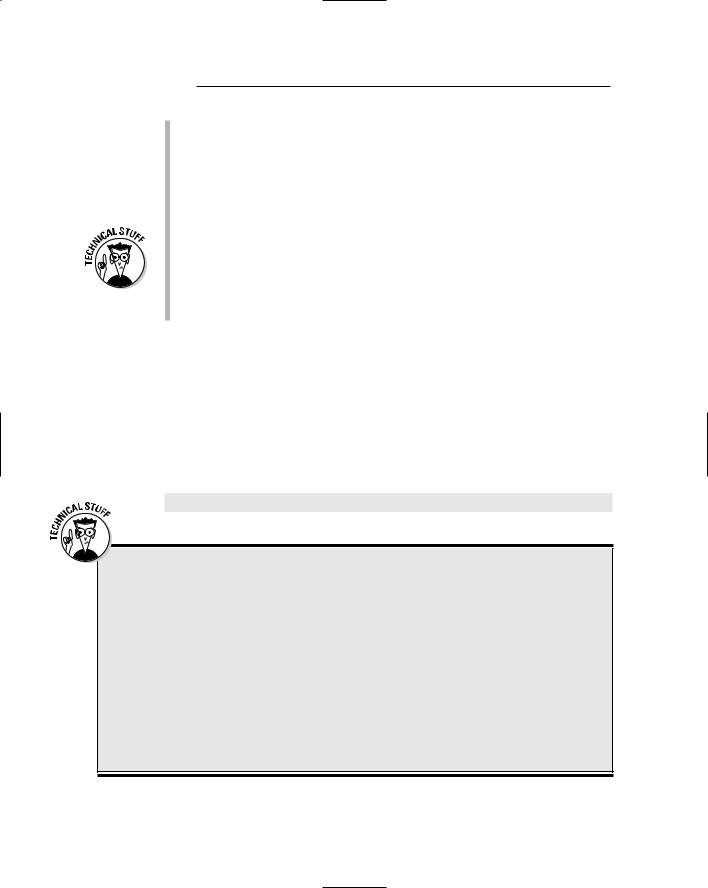
326 Part IV: C Level
The phone company is rumored to have a random-number program that it routinely uses to foul up your bill.
Random-number routines are the root of the most evil program ever devised for a budding programmer: The Guess the Number program. I spare you that torture in this book. (Well, maybe not.)
In the C language, random numbers are produced by using the rand() function. I formally introduce it next.
Random numbers must be seeded in order for them to be more unpre dictable. I cover this subject also, later in this chapter.
Random numbers generated by a computer aren’t truly random. See the nearby sidebar, “You too can waste a few seconds reading this informa tion about random numbers,” for more information.
Using the rand() function
Random numbers are generated in C by using the rand() function. It spits back a random number depending on the whims of your PC’s microprocessor carefully combined with the birthdate of the guy who wrote your C compiler plus his girlfriend’s weight in drams. Or something like that.
Here’s the format for the rand() function:
int rand();
You too can waste a few seconds reading this information about random numbers
Are they random numbers? Only if they can’t be predicted. Unfortunately, with computers, the numbers can be predicted. They’re still more or less jumbled, like street numbers in Seattle. But, overall, the random numbers a computer gen erates aren’t truly random. Instead, they’re pseudorandom.
A pseudo- (“SOO-doh”) random number is random enough for most purposes. But because
the number is based on a computer algorithm, or set routine, its outcome isn’t truly random. Even if you base the random number on the time of day — or seed the random number by using another, potentially random value — the results still aren’t random enough to appease the math ematical purists. So you live with it.
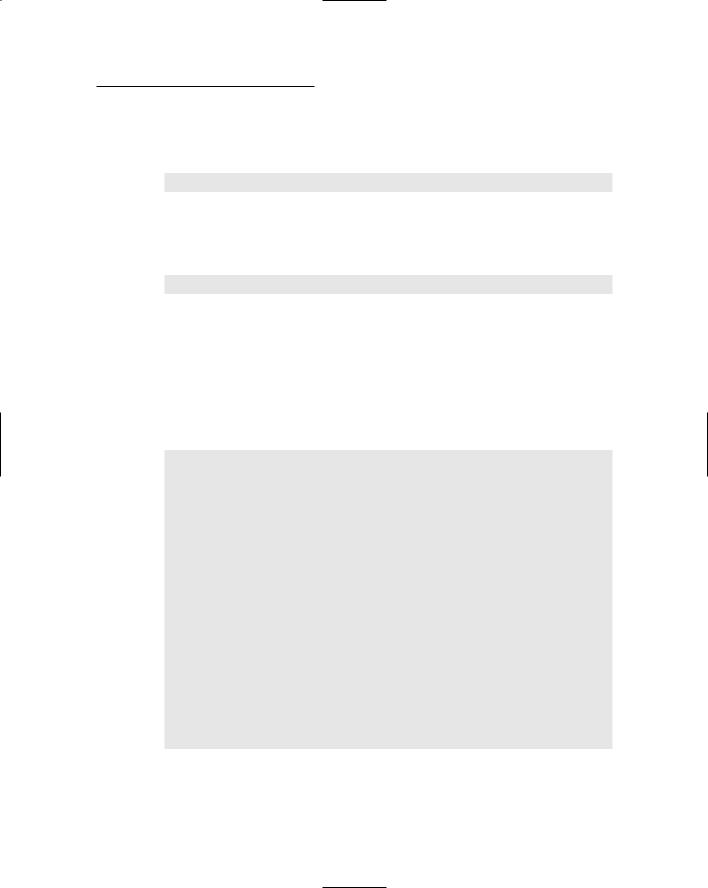
Chapter 26: The Old Random-Number Function 327
The rand() function returns an integer value, somewhere in the range of 0 through 32,767. If you want to save the random number into the r variable, you use the following statement:
r=rand();
Cinchy stuff.
To make the compiler understand the rand() function, you must add this line at the beginning of your source code:
#include <stdlib.h>
This line tells the compiler all about the rand() function and makes every one happy.
The following program shows the rand() function in action. RANDOM1.C produces 100 random numbers, which it displays neatly on the screen. The random numbers are produced by the rand function inside this program’s rnd() function. The reason that this was done, as you may be fearing, is that you modify the rnd() function to new levels of spiffiness as this chap ter progresses.
#include <stdio.h> #include <stdlib.h>
int rnd(void);
int main()
{
int x;
puts(“Behold! 100 Random Numbers!”); for(x=0;x<100;x++)
printf(“%d\t”,rnd());
return(0);
}
int rnd(void)
{
int r;
r=rand();
return(r);
}
Type the source code for RANDOM1.C into your editor. Double-check every thing. Save the file to disk as RANDOM1.C.
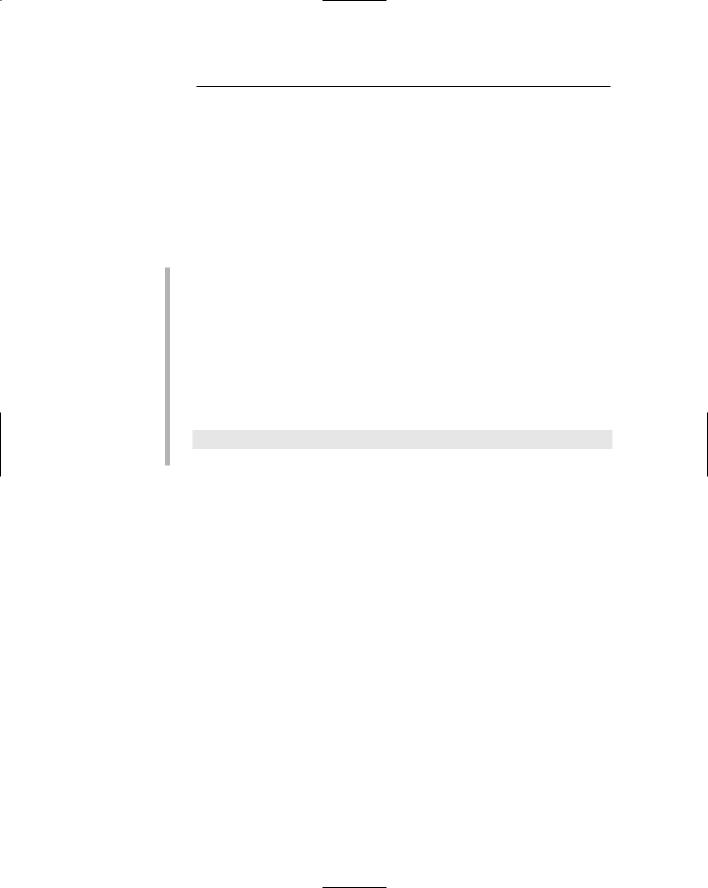
328 Part IV: C Level
Compile the program. Fix any errors, which are probably limited to missing semicolons or forgotten parentheses.
Run it!
The output displays ten columns by ten rows of random numbers on the typi cal console display — no point in repeating that here! But, on your screen, note how the numbers are different — nay, random. I mean, would you have thought of that many that quickly?
The random numbers are probably in the range of 0 to 32,000something — as promised. The numbers you see on your screen are probably different from those from the code just shown.
Some versions of the GCC compiler produce larger random number values than 0 to 32,000-something. That maximum value is set by the value of RAND_MAX, as defined in the SDTLIB.H header. On my FreeBSD machine, for example, the value of RAND_MAX is 0x7FFFFFF (hexadeci mal), which translates to 2,147,483,647.
To see the value of RAND_MAX on your computer, add this line to the pro gram before the return(0); in the main() function:
printf(“\nRAND_MAX is equal to %u\n”,RAND_MAX);
%u is the placeholder for an unsigned long integer.
Planting a random-number seed
Ho! Before you get all happy about the new CASINO program you’re about to write and sell for millions to Las Vegas, try rerunning the RANDOM1.C program again. Just choose Run from your compiler’s integrated environ ment or type the RANDOM1 command again at the DOS prompt:
(Output suppressed!)
Yup, on your screen, you see 100 more random numbers. Wait a second. Aren’t those the same numbers? Identically the same numbers? Did the compiler goof? Did you foul up?
Fortunately, that was no error. The computer generated the set of random numbers because that’s all it knows. Sure, they’re random, all right, but they’re the same numbers because, between then and now, the compiler’s rand() function remained unchanged.
Don’t feel cheated! This situation is a common one. The rand() function is only quasirandom. To make it more random (more pseudorandom), you have to plant a wee, tiny seed. This seed is a value the compiler uses to help make