
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
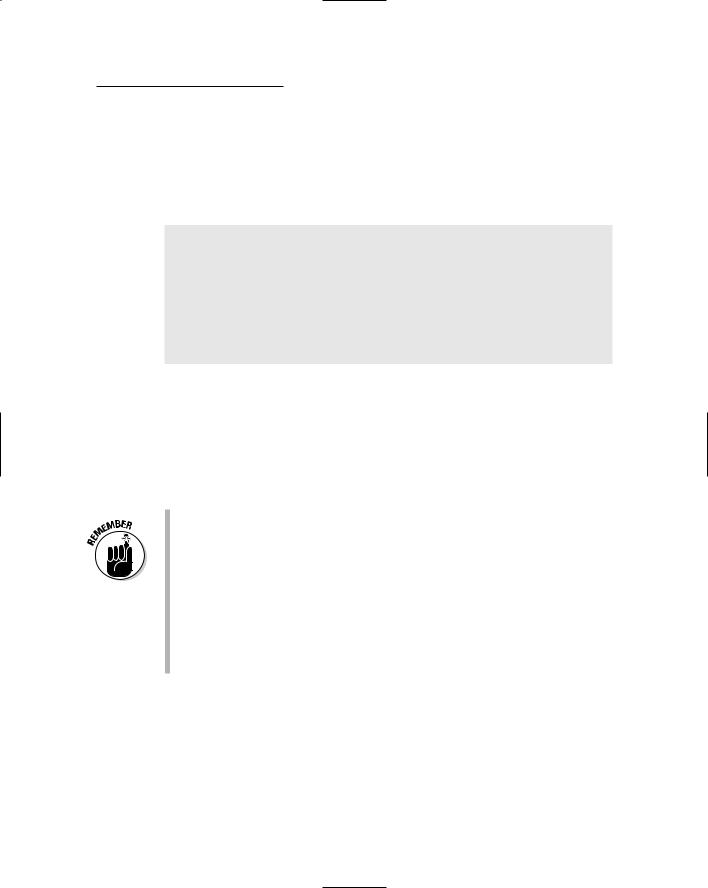
Chapter 21: Contending with Variables in Functions 267
Will the dual variable BOMBER.C program bomb?
Modify the source code for BOMBER.C in your editor. Change the main() func tion so that it reads:
int main()
{
char x;
printf(“Press Enter to drop the bomb:”);
x=getchar();
dropBomb();
printf(“Key code %d used to drop bomb.\n”,x); return(0);
}
What you’re doing is creating another x variable for use in the main() function. This variable operates independently of the x variable in the dropBomb() func tion. To prove it, save your changes to disk and then compile and run the program.
The output is roughly the same for this modified version of the program; the key code displayed for the Enter key “character” is either 10 or 13, depending on your computer.
See how the two x variables don’t confuse the computer? Each one works off by itself.
Variables in different functions can share the same name.
For example, you could have a dozen different functions in your program and have each one use the same variable names. No biggie. They’re all independent of each other because they’re nestled tightly in their own functions.
Variable x not only is used in two different functions — independently of each other — but also represents two different types of variable: a char acter and an integer. Weird.
Adding some important tension
Face it: You’re going nowhere fast as a game programmer with BOMBER.C. What you need is some tension to heighten the excitement as the bomb
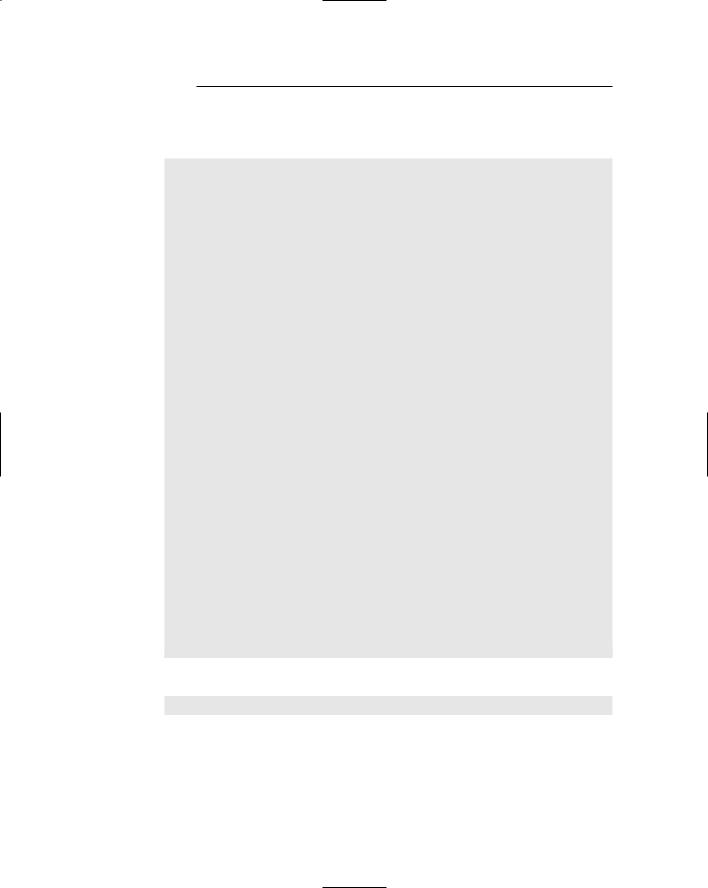
268 Part IV: C Level
drops. Why not concoct your own delay() function to make it happen? Here’s your updated source code:
#include <stdio.h> |
|
#define COUNT 20000000 |
/* 20,000,000 */ |
void dropBomb(void); |
/* prototype */ |
void delay(void); |
|
int main() |
|
{ |
|
char x; |
|
printf(“Press Enter to drop the bomb:”); x=getchar();
dropBomb();
printf(“Key code %d used to drop bomb.\n”,x); return(0);
}
void dropBomb()
{
int x;
for(x=20;x>1;x--)
{
puts(“ |
*”); |
delay(); |
|
} |
|
puts(“ |
BOOM!”); |
} |
|
void delay() |
|
{ |
|
long int x; |
|
for(x=0;x<COUNT;x++)
;
}
Mind the changes! Here they are:
#define COUNT 20000000 |
/* 20,000,000 */ |
A constant named COUNT is declared, equal to 20 million. It’s the delay value. (If the delay is too long, make the value smaller; if the delay is too short, make the value bigger.)
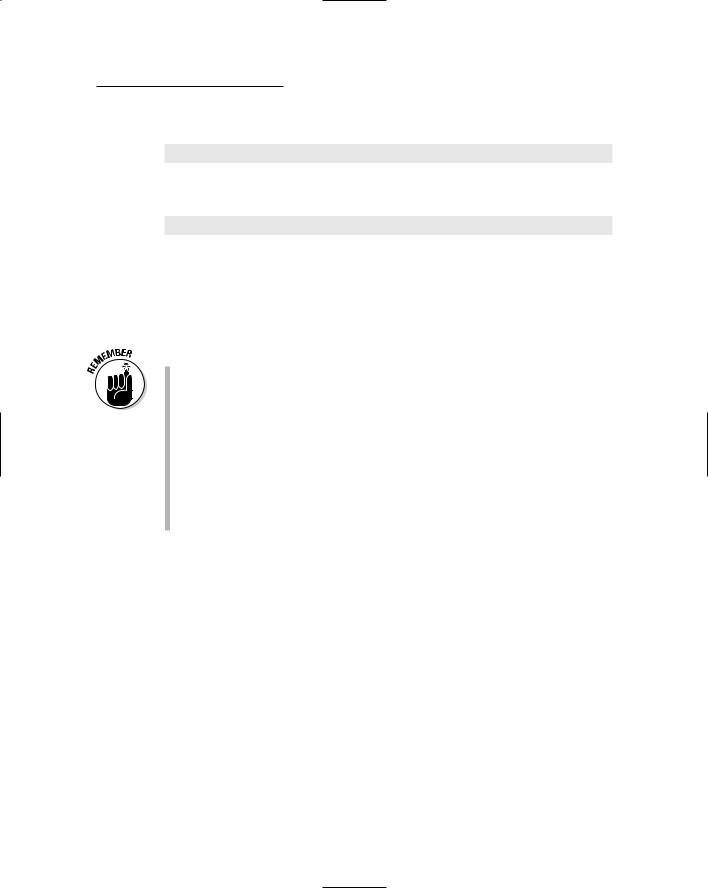
Chapter 21: Contending with Variables in Functions 269
Next, the delay() function must be prototyped:
void delay(void);
The delay() function is added into the dropBomb() function, right after puts() displays the “bomb:”
delay();
Finally, the delay() function is written. A long integer named x — a different x from any other used in any other function — is used in a for loop to create the delay.
Double-check your source code. Save to disk. Compile. Run. This time, the anticipation builds as the bomb slowly falls toward the ground and then —
BOOM!
The line used to create a constant (starting with #define) does not end with a semicolon! Go to Chapter 8 if you don’t know about this.
You can adjust the delay by changing the constant COUNT. Note how much easier it is than fishing through the program to find the exact delay loop and value — one of the chief advantages of using a constant variable.
Variables with the same names in different functions are different.
What’s the point again? I think it’s that you don’t have to keep on think ing of new variable names in each of your functions. If you’re fond of using the variable i in your for loops, for example, you can use i in all your functions. Just declare it and don’t worry about it.
How We Can All Share and
Love with Global Variables
Sometimes, you do have to share a variable between two or more functions. Most games, for example, store the score in a variable that’s accessible to a number of functions: the function that displays the score on the screen; the function that increases the score’s value; the function that decreases the value; and functions that store the score on disk, for example. All those functions have to access that one variable. That’s done by creating a global variable.
A global variable is one that any function in the program can use. The main() function can use it — any function. They can change, examine, modify, or do whatever to the variable. No problem.
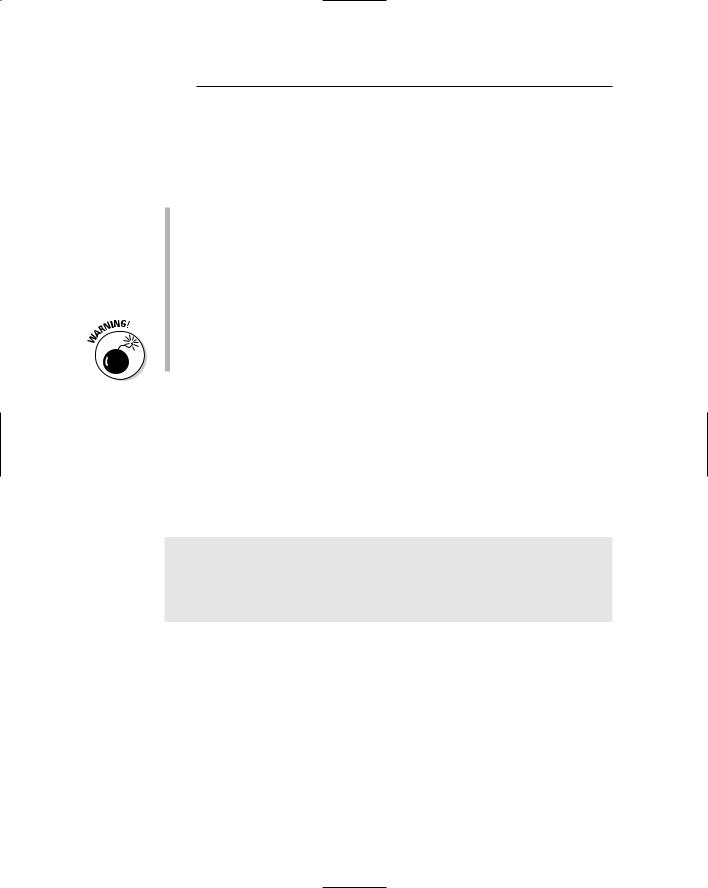
270 Part IV: C Level
The opposite of a global variable is a local variable. It’s what you have seen used elsewhere in this book. A local variable exists inside only one function — like the variable x in the BOMBER.C program. The x is a local variable, unique to the functions in which it’s created and ignored by other functions in the program.
A global variable is available to all functions in your program.
A local variable is available only to the function in which it’s created.
Global variables can be used in any function without having to redeclare them. If you have a global integer variable score, for example, you don’t have to stick an int score; declaration in each function which uses that variable. The variable has already been declared and is ready for use in any function.
Because global variables exist all over the place, naming them is impor tant. After you declare x as a global variable, for example, no other func tion can declare x as anything else without ticking off the compiler.
Making a global variable
Global variables differ from local variables in two ways. First, because they’re global variables, any function in the program can use them. Second, they’re declared outside of any function. Out there. In the emptiness. Midst the chaos. Strange, but true.
For example:
#include <stdio.h>
int score;
int main()
{
Etc. . . .
Think of this source code as the beginning of some massive program, the details of which aren’t important right now. Notice how the variable score is declared. It’s done outside of any function, traditionally just before the main() function and after the pound-sign dealies (and any prototyping non sense). That’s how global variables are made.
If more global variables are required, they’re created in the same spot, right there before the main() function. Declaring them works as it normally does; the only difference is that it’s done outside of any function.