
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
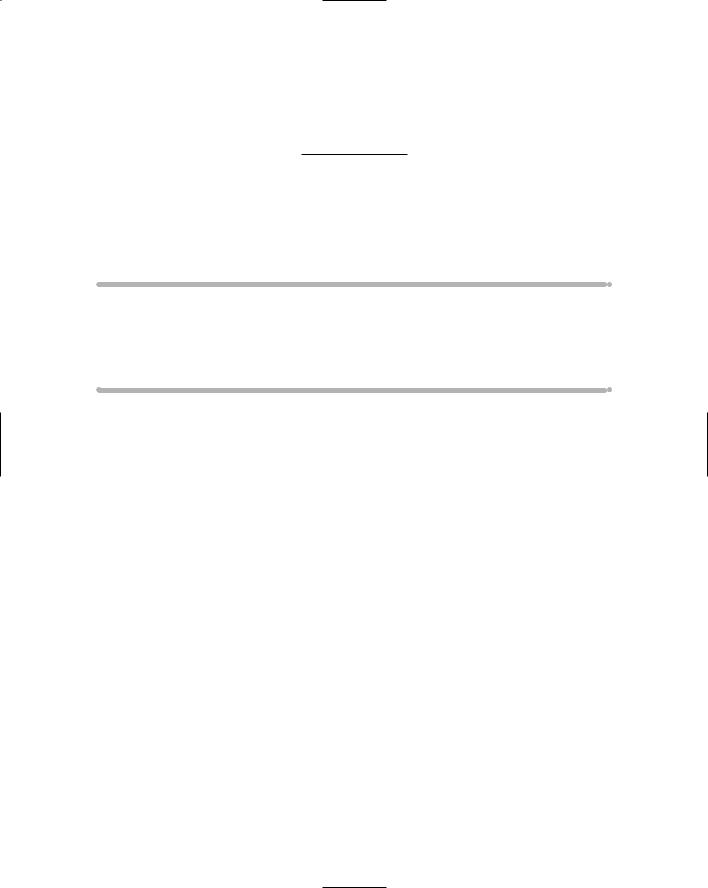
Chapter 19
Switch Case, or, From ‘C’ to Shining ‘c’
In This Chapter
Solving the endless else-if puzzle
Using switch-case
Creating a switch-case structure
Honestly, I don’t believe that switch-case is really a loop. But the word loop works so much better than my alternative, structure thing. That’s
because the statements held inside the switch-case structure thing aren’t really repeated, yet in a way they are. Well, anyway.
This chapter uncovers the final kind-of-loop thing in the C language, which is called switch-case. It’s not so much a loop as it’s a wonderful method of cleaning up a potential problem with multiple if statements. As is true with most things in a programming language, it’s just better for me to show you an example than to try to explain it. That’s what this chapter does.
The Sneaky switch-case Loops
Let’s all go to the lobby,
Let’s all go to the lobby,
Let’s all go to the lobby,
And get ourselves a treat!
— Author unknown
And, when you get to the lobby, you probably order yourself some goodies from the menu. In fact, management at your local theater has just devised an
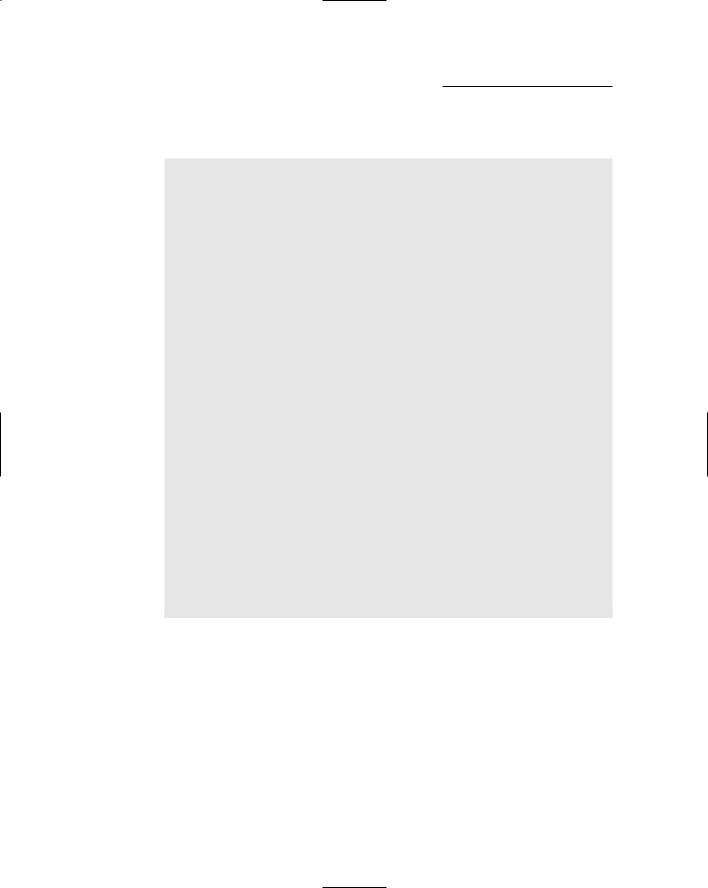
240 Part III: Giving Your Programs the Ability to Run Amok
interesting computer program to help cut down on pesky, hourly-wage employ ees. The program they have devised is shown right here:
/* Theater lobby snack bar program */
#include <stdio.h>
int main()
{
char c;
printf(“Please make your treat selection:\n”); printf(“1 - Beverage.\n”);
printf(“2 - Candy.\n”); printf(“3 - Hot dog.\n”); printf(“4 - Popcorn.\n”); printf(“Your choice:”);
/* Figure out what they typed in. */
c=getchar();
if(c==’1’)
printf(“Beverage\nThat will be $8.00\n”); else if(c==’2’)
printf(“Candy\nThat will be $5.50\n”); else if(c==’3’)
printf(“Hot dog\nThat will be $10.00\n”); else if(c==’4’)
printf(“Popcorn\nThat will be $7.50\n”); else
{
printf(“That is not a proper selection.\n”); printf(“I’ll assume you’re just not hungry.\n”); printf(“Can I help whoever’s next?\n”);
}
return(0);
}
Type this source code into your editor. Save it to disk as LOBBY1.C. This should brighten your heart because you know that more LOBBY programs are on the way. . . .
Compile. Fix any errors. You may get a few because it’s such a long program. Watch your spelling and remember your semicolons. Recompile after fixing any errors.
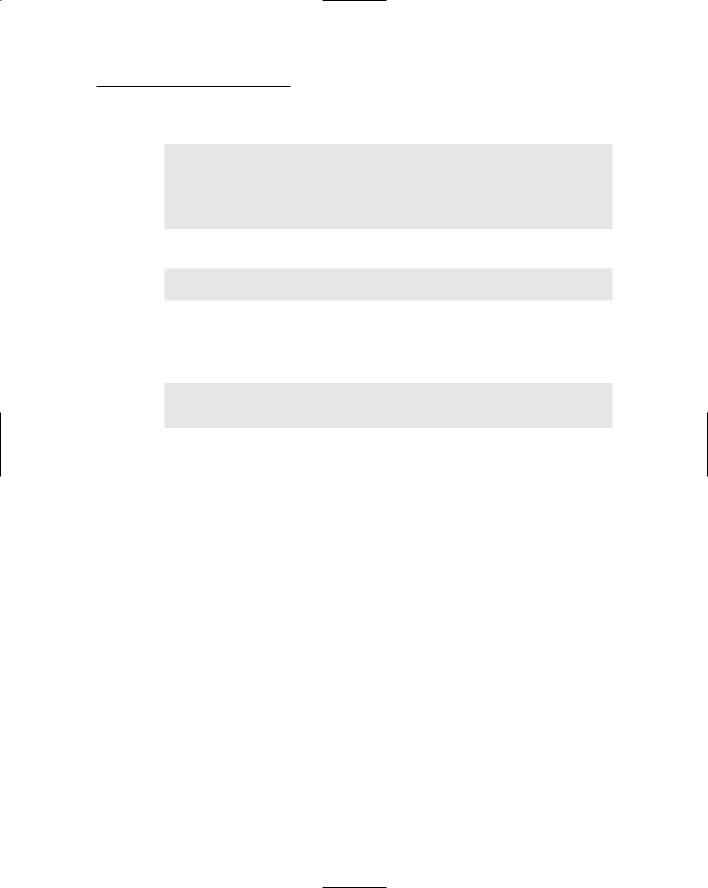
Chapter 19: Switch Case, or, From ‘C’ to Shining ‘c’ 241
Run:
Please make your treat selection: 1 - Beverage.
2 - Candy.
3 - Hot dog.
4 - Popcorn. Your choice:
Press 2, for Candy. Love them Hot Tamales! You see
Candy
That will be $5.50
Gadzooks! For Hot Tamales? I’m sneaking food in next time. . . .
Run the program again and try a few more options. Then, try an option not on the list. Type M for a margarita:
That is not a proper selection.
I’ll assume you’re just not hungry.
Can I help whoever’s next?
Oh, well.
The switch-case Solution to the LOBBY Program
Don’t all those else-if things in the LOBBY1.C program look funny? Doesn’t it appear awkward? Maybe not. But it is rather clumsy. That’s because you have a better way to pick one of several choices in C. What you need is a switch-case loop.
Right away, I need to tell you that switch-case isn’t really a loop. Instead, it’s a selection statement, which is the official designation of what an if state ment is. switch-case allows you to select from one of several items, like a long, complex string of if statements — the kind that’s now pestering the LOBBY1.C program.
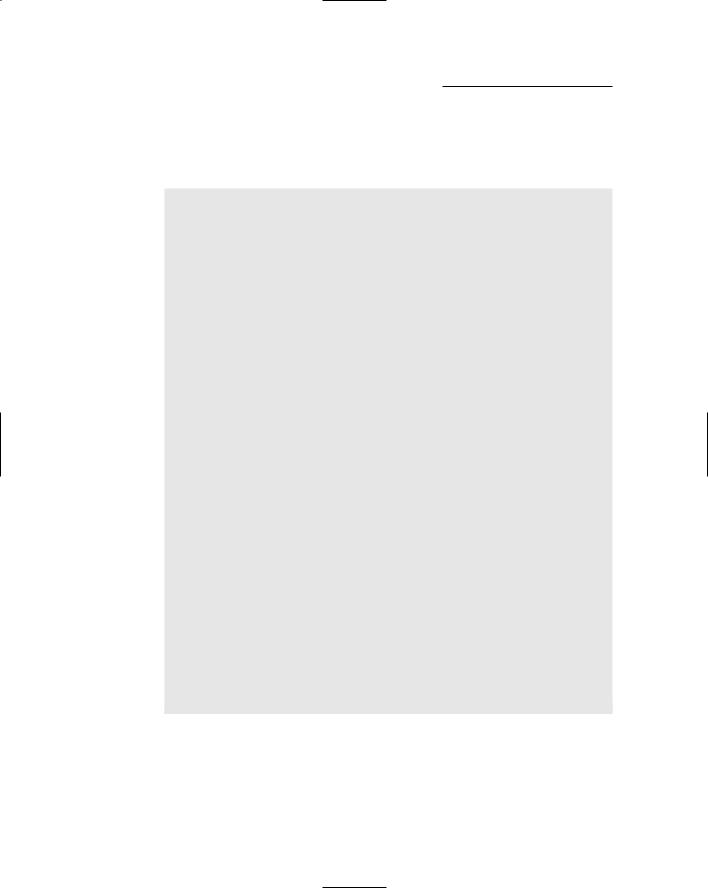
242 Part III: Giving Your Programs the Ability to Run Amok
Next is the source code for LOBBY2.C, an internal improvement to the LOBBY1.C program. It’s internal because you’re just messing with the program’s guts here — making them more elegant. Externally, the program still works the same:
/* Theater lobby snack bar program */
#include <stdio.h>
int main()
{
char c;
printf(“Please make your treat selection:\n”); printf(“1 - Beverage.\n”);
printf(“2 - Candy.\n”); printf(“3 - Hot dog.\n”); printf(“4 - Popcorn.\n”); printf(“Your choice:”);
/* Figure out what they typed in. */
c=getchar();
switch(c)
{
case ‘1’:
printf(“Beverage\nThat will be $8.00\n”); break;
case ‘2’:
printf(“Candy\nThat will be $5.50\n”); break;
case ‘3’:
printf(“Hot dog\nThat will be $10.00\n”); break;
case ‘4’:
printf(“Popcorn\nThat will be $7.50\n”); break;
default:
printf(“That is not a proper selection.\n”); printf(“I’ll assume you’re just not hungry.\n”); printf(“Can I help whoever’s next?\n”);
}
return(0);
}
Keep the LOBBY1.C program in your editor. Use this source code for LOBBY2.C as a guide and edit what you see on your screen.