
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
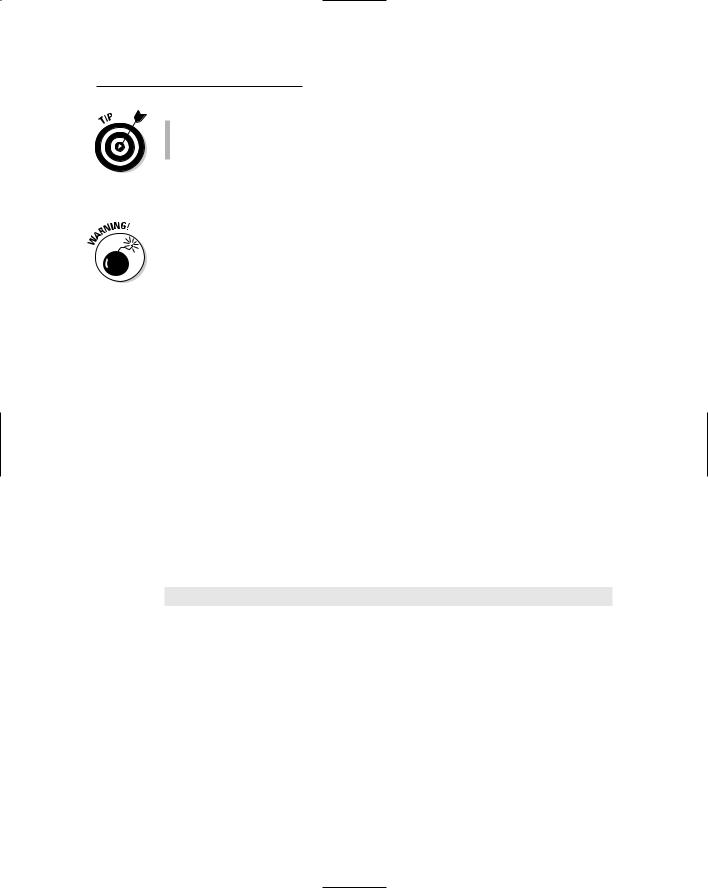
Chapter 6: C More I/O with gets() and puts() |
67 |
You can pronounce gets() as “get-string” in your head. “Get a string of text from the keyboard.” However, it probably stands for “Get stdin,” which means “Get from standard input.” “Get string” works for me, though.
And now, the bad news about gets()
The latest news from the C language grapevine is not to use the gets() func tion, at least not in any serious, secure programs you plan on writing. That’s because gets() is not considered a safe, secure function to use.
The reason for this warning — which may even appear when you compile a program using gets() — is that you can type more characters at the keyboard than were designed to fit inside the char variable associated with gets(). This flaw, known as a keyboard overflow, is used by many of the bad guys out there to write worms and viruses and otherwise exploit well-meaning programs.
For the duration of this book, don’t worry about using gets(). It’s okay here as a quick way to get input while finding out how to use C. But for “real” pro grams that you write, I recommend concocting your own keyboard-reading functions.
The Virtues of puts()
In a way, the puts() function is a simplified version of the printf() function. puts() displays a string of text, but without all printf()’s formatting magic. puts() is just a boneheaded “Yup, I display this on the screen” command. Here’s the format:
puts(text);
puts() is followed by a left paren, and then comes the text you want to dis play. That can either be a string variable name or a string of text in double quotes. That’s followed by a right paren. The puts() function is a complete C language statement, so it always ends with a semicolon.
The puts() function’s output always ends with a newline character, \n. It’s like puts() “presses Enter” after displaying the text. You cannot avoid this side effect, though sometimes it does come in handy.
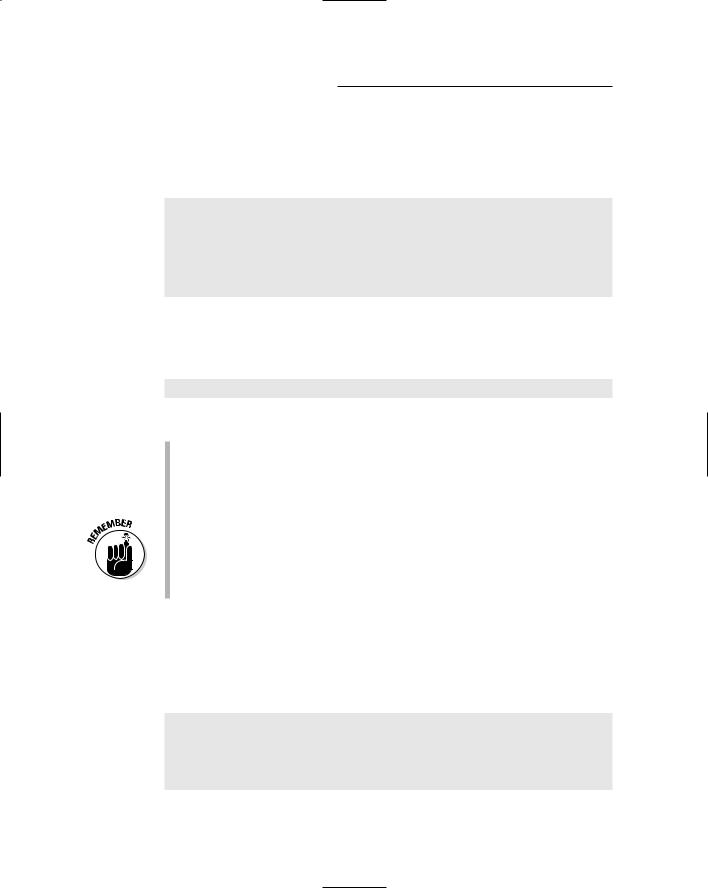
68 |
Part I: Introduction to C Programming |
Another silly command-prompt program
To see how puts() works, create the following program, STOP.C. Yeah, this program is really silly, but you’re just starting out, so bear with me:
#include <stdio.h>
int main()
{
puts(“Unable to stop: Bad mood error.”); return(0);
}
Save this source code to disk as STOP.C. Compile it, link it, run it.
This program produces the following output when you type stop or ./stop at the command prompt:
Unable to stop: Bad mood error.
Ha, ha.
puts() is not pronounced “putz.”
Like printf(), puts() slaps a string of text up on the screen. The text is hugged by double quotes and is nestled between two parentheses.
Like printf(), puts() understands escape sequences. For example, you can use \” if you want to display a string with a double quote in it.
You don’t have to put a \n at the end of a puts() text string. puts() always displays the newline character at the end of its output.
If you want puts() not to display the newline character, you must use printf() instead.
puts() and gets() in action
The following program is a subtle modification to INSULT1.C. This time, the first printf() is replaced with a puts() statement:
#include <stdio.h>
int main()
{
char jerk[20];
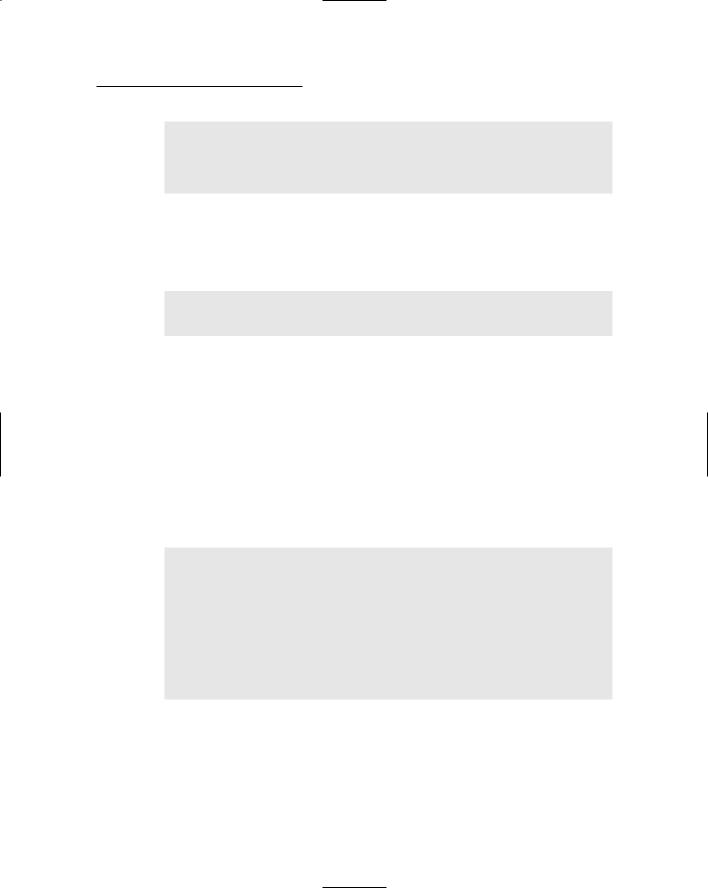
Chapter 6: C More I/O with gets() and puts() |
69 |
puts(“Name some jerk you know:”); gets(jerk);
printf(“Yeah, I think %s is a jerk, too.”,jerk); return(0);
}
Load the source code for INSULT1.C into your editor. Change Line 7 so that it reads as just shown; the printf is changed to puts.
Use your editor’s Save As command to give this modified source code a new name on disk: INSULT2.C. Save. Compile. Run.
Name some jerk you know:
Rebecca
Yeah, I think Rebecca is a jerk, too.
Note that the first string displayed (by puts()) has that newline appear after ward. That’s why input takes place on the next line. But considering how many command-line or text-based programs do that, it’s really no big deal. Other wise, the program runs the same as INSULT1. But you’re not done yet; con tinue reading with the next section.
More insults
The following source code is another modification to the INSULT series of pro grams. This time, you replace the final printf() with a puts() statement. Here’s how it looks:
#include <stdio.h>
int main()
{
char jerk[20];
puts(“Name some jerk you know:”); gets(jerk);
puts(“Yeah, I think %s is a jerk, too.”,jerk); return(0);
}
Load the source code for INSULT2.C into your editor. Make the changes just noted, basically replacing the printf in Line 9 with puts. Otherwise, the rest of the code is the same.
Save the new source code to disk as INSULT3.C. Compile and run.
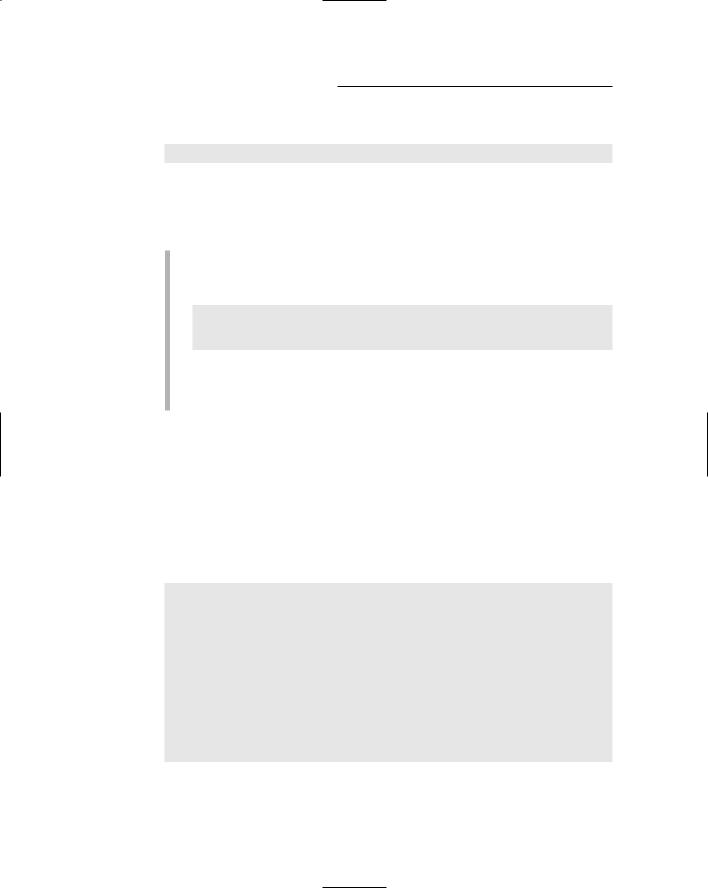
70 |
Part I: Introduction to C Programming |
Whoops! Error! Error!
Insult3.c:9: too many arguments to function ‘puts’
The compiler is smart enough to notice that more than one item appears to be specified for the puts() function; it sees a string, and then a variable is specified. According to what the compiler knows, you need only one or the other, not both. Oops.
puts() is just not a simpler printf().
If you got the program to run — and some compilers may — the output looks like this:
Name some jerk you know:
Bruce
Yeah, I think that %s is a jerk, too.
Ack! Who is this %s person who is such a jerk? Who knows! Remember that puts() isn’t printf(), and it does not process variables the same way. To puts(), the %s in a string is just %s — characters — nothing special.
puts() can print variables
puts() can display a string variable, but only on a line by itself. Why a line by itself? Because no matter what, puts() always tacks on that pesky newline character. You cannot blend a variable into another string of text by using the puts() function.
Consider the following source code, the last in the INSULT line of programs:
#include <stdio.h>
int main()
{
char jerk[20];
puts(“Name some jerk you know:”); gets(jerk);
puts(“Yeah, I think”); puts(jerk);
puts(“is a jerk, too.”); return(0);
}
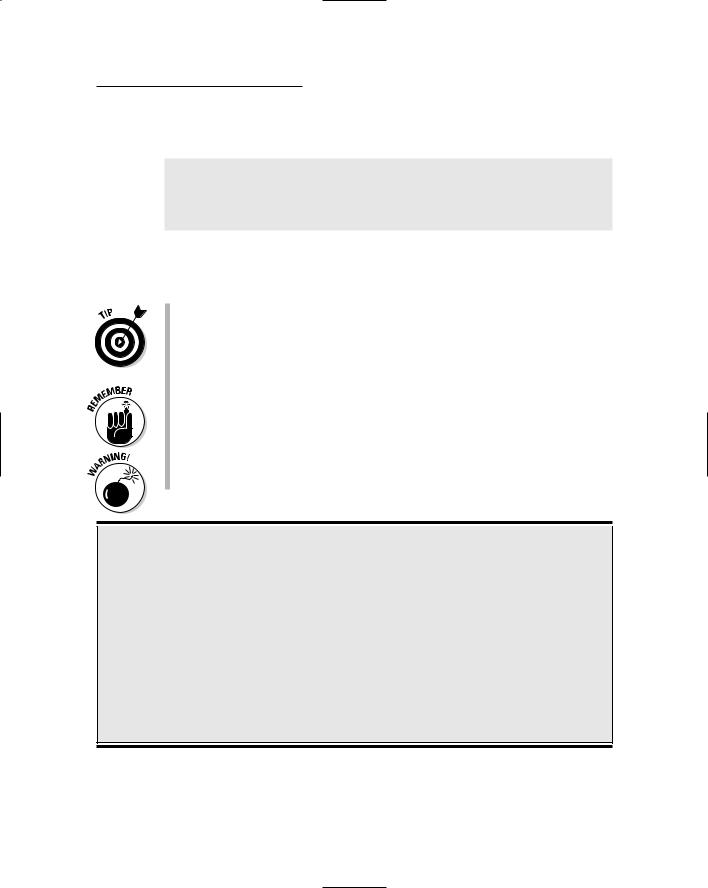
Chapter 6: C More I/O with gets() and puts() |
71 |
Feel free to make the preceding modifications to your INSULT3.C program in your editor. Save the changes to disk as INSULT4.C. Compile. Run.
Name some jerk you know: David
Yeah, I think David
is a jerk, too.
The output looks funky, like one of those “you may be the first person on your block” sweepstakes junk mailers. But the program works the way it was intended.
Rather than replace printf() with puts(), you have to rethink your program’s strategy. For one, puts() automatically sticks a newline on the end of a string it displays. No more strings ending in \n! Second, puts() can display only one string variable at a time, all by itself, on its own line. And, last, the next bit of code shows the program the way it should be written by using only puts() and gets().
You must first “declare” a string variable in your program by using the char keyword. Then you must stick something in the variable, which you can do by using the scanf() or gets function. Only then does dis playing the variable’s contents by using puts() make any sense.
Do not use puts() with a nonstring variable. The output is weird. (See Chapter 8 for the lowdown on variables.)
When to use puts()
When to use printf()
Use puts() to display a single line of text — nothing fancy.
Use puts() to display the contents of a string variable on a line by itself.
Use printf() to display the contents of a variable nestled in the middle of another string.
Use printf() to display the contents of more than one variable at a time.
Use printf() when you don’t want the newline (Enter) character to be displayed after every line, such as when you’re prompting for input.
Use printf() when fancy formatted output is required.
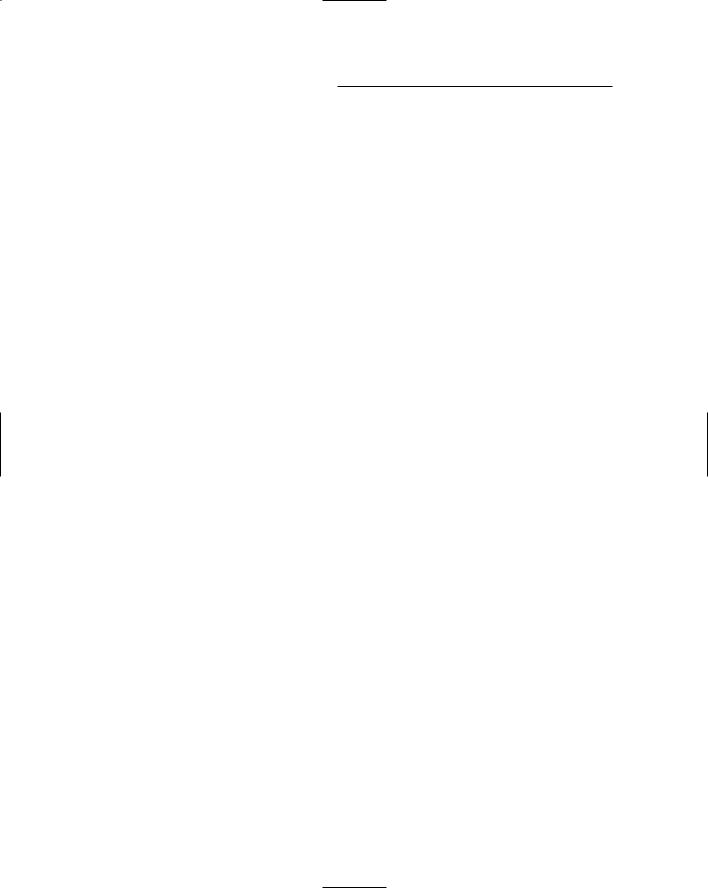
72 |
Part I: Introduction to C Programming |

Part II
Run and Scream
from Variables
and Math
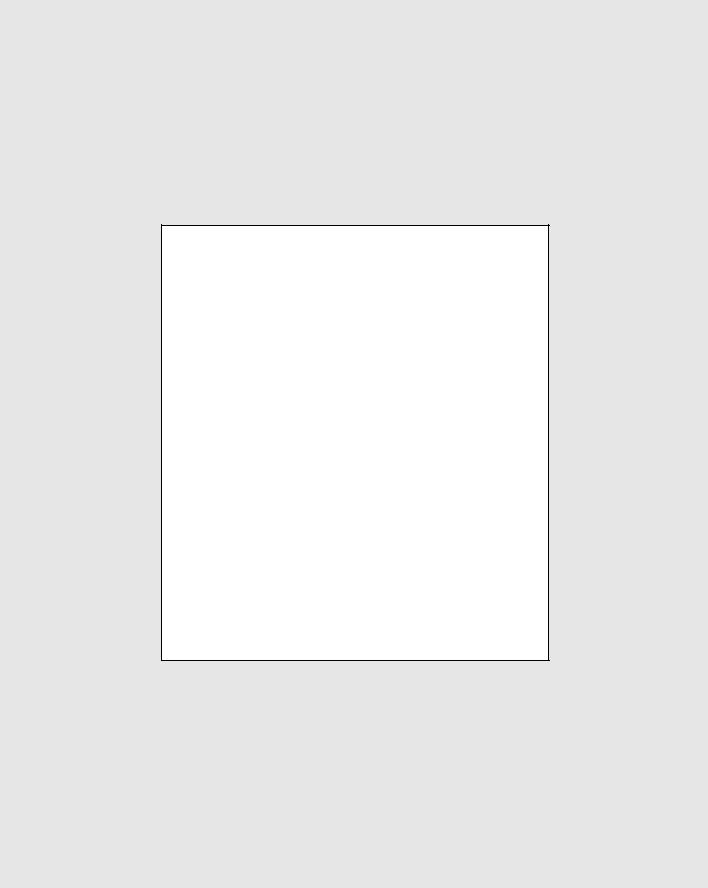
In this part . . .
Programming a computer involves more than just splattering text on a screen. In fact, when you ask
most folks, they assume that programming is some branch of mathematics or engineering. After all, computers have their roots in the calculators and adding machines of years gone by. And, early computers were heavily involved with math, from computing missile trajectories to landing men on the moon.
I have to admit: Programming a computer does involve math. That’s the subject of the next several chapters, along with an official introduction to the concept of a variable (also a math thing). Before you go running and screaming from the room, however, consider that it’s the computer that does the math. Unlike those sweaty days in the back of eighth-grade math class, with beady-eyed Mr. Perdomo glowering at you like a fat hawk eyeing a mouse, you merely have to jot down the problem. The computer solves it for you.
Relax! Sit back and enjoy reading about how you can slav ishly make the computer do your math puzzles. And, if it gets the answer wrong, feel free to berate it until the com puter feels like it’s only about yay high.