
- •Table of Contents
- •Introduction
- •About This Here Dummies Approach
- •How to Work the Examples in This Book
- •Foolish Assumptions
- •Icons Used in This Book
- •Final Thots
- •The C Development Cycle
- •From Text File to Program
- •The source code (text file)
- •The compiler and the linker
- •Running the final result
- •Save It! Compile and Link It! Run It!
- •Reediting your source code file
- •Dealing with the Heartbreak of Errors
- •The autopsy
- •Repairing the malodorous program
- •Now try this error!
- •The Big Picture
- •Other C Language Components
- •Pop Quiz!
- •The Helpful RULES Program
- •The importance of being \n
- •Breaking up lines\ is easy to do
- •The reward
- •More on printf()
- •Printing funky text
- •Escape from printf()!
- •A bit of justification
- •Putting scanf together
- •The miracle of scanf()
- •Experimentation time!
- •Adding Comments
- •A big, hairy program with comments
- •Why are comments necessary?
- •Bizarr-o comments
- •C++ comments
- •Using Comments to Disable
- •The More I Want, the More I gets()
- •Another completely rude program example
- •And now, the bad news about gets()
- •The Virtues of puts()
- •Another silly command-prompt program
- •puts() and gets() in action
- •More insults
- •puts() can print variables
- •The Ever-Changing Variable
- •Strings change
- •Running the KITTY
- •Hello, integer
- •Using an integer variable in the Methuselah program
- •Assigning values to numeric variables
- •Entering numeric values from the keyboard
- •The atoi() function
- •So how old is this Methuselah guy, anyway?
- •Basic mathematical symbols
- •How much longer do you have to live to break the Methuselah record?
- •The direct result
- •Variable names verboten and not
- •Presetting variable values
- •The old random-sampler variable program
- •Maybe you want to chance two pints?
- •Multiple declarations
- •Constants and Variables
- •Dreaming up and defining constants
- •The handy shortcut
- •The #define directive
- •Real, live constant variables
- •Numbers in C
- •Why use integers? Why not just make every number floating-point?
- •Integer types (short, long, wide, fat, and so on)
- •How to Make a Number Float
- •The E notation stuff
- •Single-character variables
- •Char in action
- •Stuffing characters into character variables
- •Reading and Writing Single Characters
- •The getchar() function
- •The putchar() function
- •Character Variables As Values
- •Unhappily incrementing your weight
- •Bonus program! (One that may even have a purpose in life)
- •The Sacred Order of Precedence
- •A problem from the pages of the dentistry final exam
- •The confounding magic-pellets problem
- •Using parentheses to mess up the order of precedence
- •The computer-genie program example
- •The if keyword, up close and impersonal
- •A question of formatting the if statement
- •The final solution to the income-tax problem
- •Covering all the possibilities with else
- •The if format with else
- •The strange case of else-if and even more decisions
- •Bonus program! The really, really smart genie
- •The World of if without Values
- •The problem with getchar()
- •Meanwhile, back to the GREATER problem
- •Another, bolder example
- •Exposing Flaws in logic
- •A solution (but not the best one)
- •A better solution, using logic
- •A logical AND program for you
- •For Going Loopy
- •For doing things over and over, use the for keyword
- •Having fun whilst counting to 100
- •Beware of infinite loops!
- •Breaking out of a loop
- •The break keyword
- •The Art of Incrementation
- •O, to count backward
- •How counting backward fits into the for loop
- •More Incrementation Madness
- •Leaping loops!
- •Counting to 1,000 by fives
- •Cryptic C operator symbols, Volume III: The madness continues
- •The answers
- •The Lowdown on while Loops
- •Whiling away the hours
- •Deciding between a while loop and a for loop
- •Replacing those unsightly for(;;) loops with elegant while loops
- •C from the inside out
- •The Down-Low on Upside-Down do-while Loops
- •The devil made me do-while it!
- •do-while details
- •The always kosher number-checking do-while loop
- •Break the Brave and Continue the Fool
- •The continue keyword
- •The Sneaky switch-case Loops
- •The switch-case Solution to the LOBBY Program
- •The Old switch-case Trick
- •The Special Relationship between while and switch-case
- •A potentially redundant program in need of a function
- •The noble jerk() function
- •Prototyping Your Functions
- •Prototypical prototyping problems
- •A sneaky way to avoid prototyping problems
- •The Tao of Functions
- •The function format
- •How to name your functions
- •Adding some important tension
- •Making a global variable
- •An example of a global variable in a real, live program
- •Marching a Value Off to a Function
- •How to send a value to a function
- •Avoiding variable confusion (must reading)
- •Functions That Return Stuff
- •Something for your troubles
- •Finally, the computer tells you how smart it thinks you are
- •Return to sender with the return keyword
- •Now you can understand the main() function
- •Give that human a bonus!
- •Writing your own dot-H file
- •A final warning about header files
- •What the #defines Are Up To
- •Avoiding the Topic of Macros
- •A Quick Review of printf()
- •The printf() Escape Sequences
- •The printf() escape-sequence testing program deluxe
- •Putting PRINTFUN to the test
- •The Complex printf() Format
- •The printf() Conversion Characters
- •More on Math
- •Taking your math problems to a higher power
- •Putting pow() into use
- •Rooting out the root
- •Strange Math? You Got It!
- •Something Really Odd to End Your Day
- •The perils of using a++
- •Oh, and the same thing applies to a --
- •Reflections on the strange ++a phenomenon
- •On Being Random
- •Using the rand() function
- •Planting a random-number seed
- •Randoming up the RANDOM program
- •Streamlining the randomizer
- •Arrays
- •Strings
- •Structures
- •Pointers
- •Linked Lists
- •Binary Operators
- •Interacting with the Command Line
- •Disk Access
- •Interacting with the Operating System
- •Building Big Programs
- •Use the Command-Line History
- •Use a Context-Colored Text Editor
- •Carefully Name Your Variables
- •Breaking Out of a Loop
- •Work on One Thing at a Time
- •Break Up Your Code
- •Simplify
- •Talk through the Program
- •Set Breakpoints
- •Monitor Your Variables
- •Document Your Work
- •Use Debugging Tools
- •Use a C Optimizer
- •Read More Books!
- •Setting Things Up
- •The C language compiler
- •The place to put your stuff
- •Making Programs
- •Finding your learn directory or folder
- •Running an editor
- •Compiling and linking
- •Index
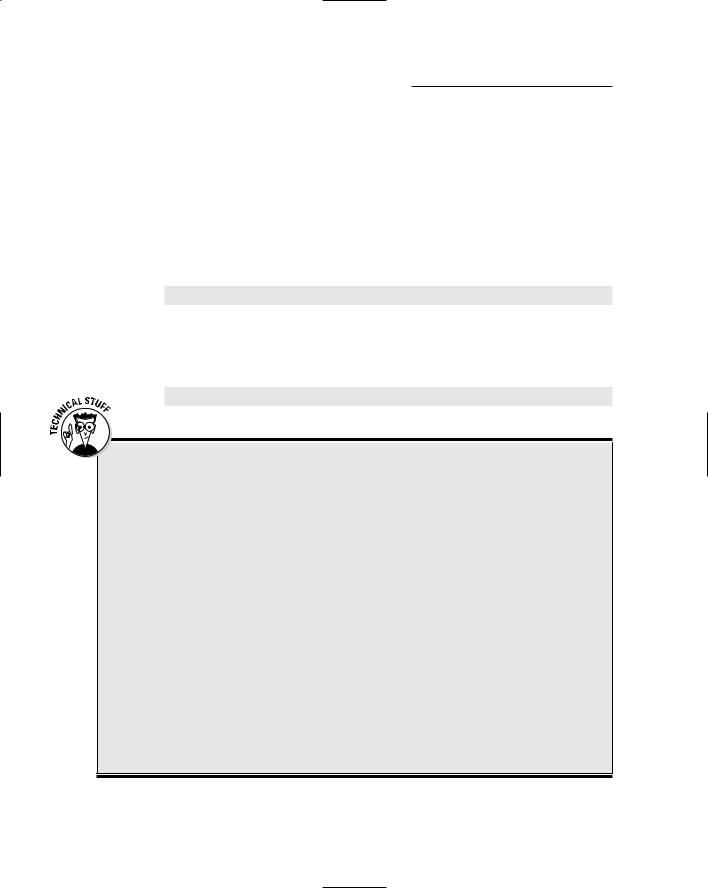
122 Part II: Run and Scream from Variables and Math
Single-character variables
Like a string of text, the single-character variable is declared by using the char keyword. Unlike a string, the single-character variable holds only one character — no more. In a way, the character variable is like a padded cell. The string variable is merely several padded cells one after the other — like an asylum.
The char keyword is used to set aside storage space for a single-character variable. Here’s the format:
char var;
char is written in lowercase, followed by a space and then var, the name of the variable to be created.
In the following format, you can predefine a single-character value:
char var=’c’;
Typing those hard-to-reach characters
Some characters can’t be typed at the keyboard or entered by using escape sequences. For example, the extended ASCII characters used on most PCs — which include the line-drawing characters, math symbols, and some foreign characters — require some extra effort to stuff into character variables. It’s possible — just a little technical. Follow these steps:
1.Look up the character’s secret code value — its ASCII or extended ASCII code number.
2.Convert that code number into base 16, the hexadecimal, or “hex,” system. (That’s why hexadecimal values are usually shown in the ASCII tables and charts.)
3.Specify that hex value, which is two digits long, after the \x escape sequence.
4.Remember to enclose the entire escape sequence — four characters long — in single quotes.
Suppose that you want to use the British pound symbol, £, in your program. That character’s secret code number is 156. Look it up in Appendix B. You can see that the hexadecimal value is 9C. (Hex numbers contain letters.) So you specify the following escape sequence in your program:
‘\x9C’
Notice that it’s enclosed in single quotes. The C, or any other hexadecimal letter, can be upperor lowercase. When the escape sequence is assigned to a character variable, the C compiler takes the preceding number and converts it into a character — the £ — which sits snugly until needed.
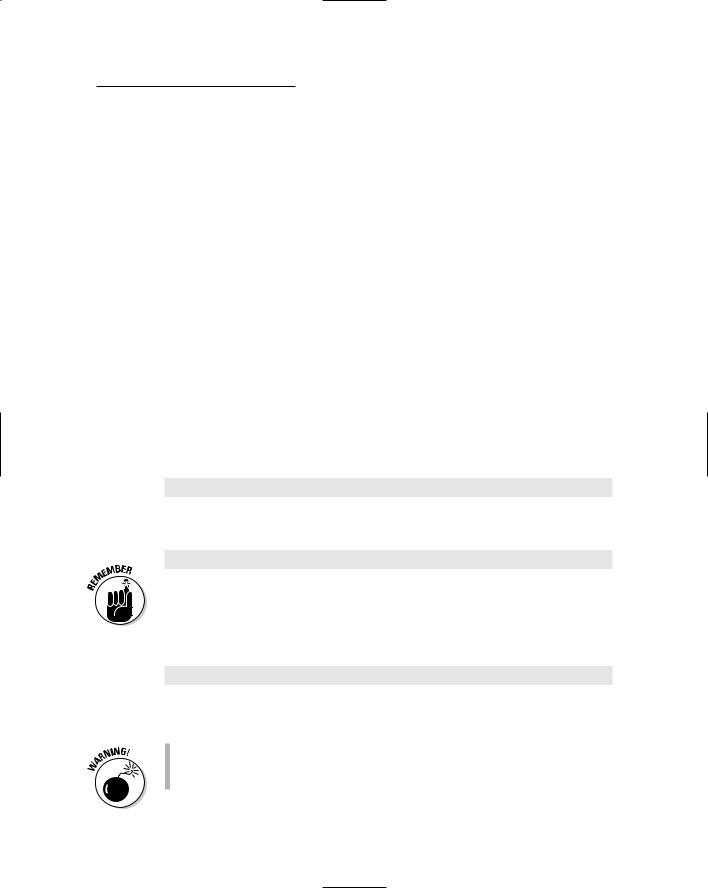
Chapter 10: Cook That C Variable Charred, Please 123
char is followed by a space. The name of the variable you’re creating, var, is followed by an equal sign and then a character in single quotes. The statement ends in a semicolon.
Inside the single quotes is a single character, which is assigned to the vari able var. You can specify any single character or use one of the escape sequences to specify a nontypable character, such as a double-quote, a single quote, or the Enter key press. (See Table 24-1 in Chapter 24 for the full list of escape sequences; also see the nearby sidebar, “Typing those hard-to- reach characters.”)
The single-character variable is ideal for reading one character (obviously) from the keyboard. By using miracles of the C language not yet known, you can compare that character with other characters and make your programs do wondrous things. This is how a menu system works, how you can type single-key commands without having to press Enter, and how you can write your own keyboard-reading programs. Oh, it can be fun.
Char in action
The following statement creates the character variable ch for use in the pro gram (you can also predefine the variable if need be):
char ch;
This next statement creates the character variable x and assigns to it the char acter value ‘X’ (big X):
char x=’X’;
When you assign a character to a single-character variable, you use single quotes. It’s a must!
Some characters, you can’t really type at the keyboard. For example, to pre define a variable and stick the Tab key into it, you use an escape sequence:
char tab=’\t’;
This statement creates the character variable tab and places in that variable the tab character, represented by the \t escape sequence.
Single-character variables are created by using the char keyword.
Don’t use the square brackets when you’re declaring single-character variables.
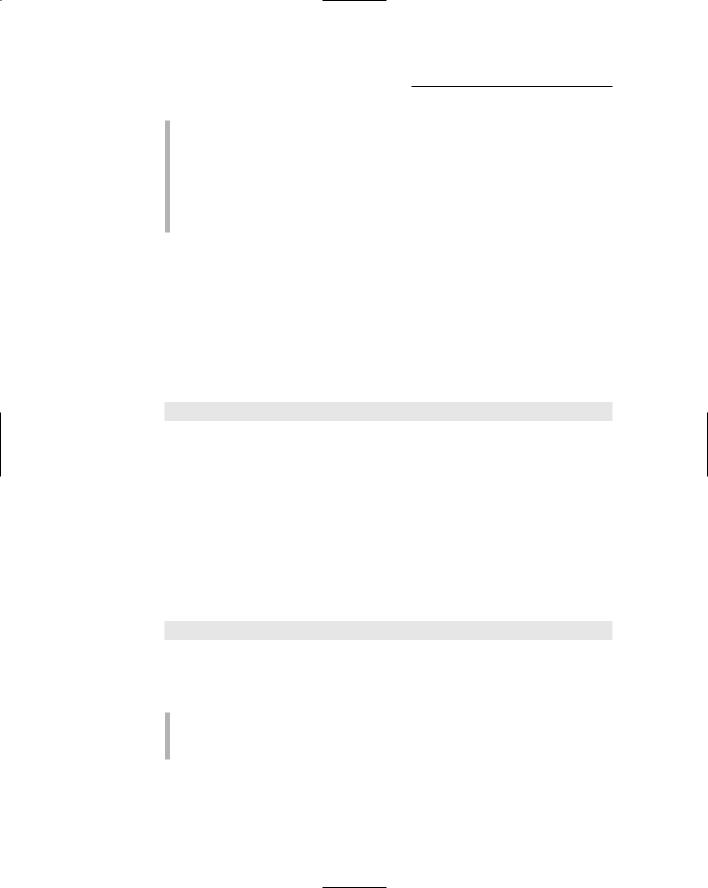
124 Part II: Run and Scream from Variables and Math
If you predefine the variable’s value with a character, enclose that char acter in single quotes. Don’t use double quotes.
You can assign almost any character value to the character variable. Special, weird, and other characters can be assigned by using the escape sequences.
Information about creating string variables is presented in Chapter 4. This chapter deals primarily with single-character variables.
Stuffing characters into character variables
You can assign a character variable a value in one of several ways. The first way is to just stuff a character in there, similar to the way you stuff a value into a numeric variable or your foot into a sock. If key is a character variable, for example, you can place the character ‘T’ in it with this statement:
key=’T’;
The T, which must be in single quotes, ladies and gentlemen, slides through the equal sign and into the key variable’s single-character holding bin. The statement ends in a semicolon. (I assume that key was defined as a character variable by using the char key; statement, earlier in the program.)
In addition to single characters, you can specify various escape sequences (the \-character things), values, and whatnot. As long as it’s only one charac ter long, you’re hunky-dory.
Another way to stick a character into a single-character variable is to slide one from another character variable. Suppose that both old and new are charac ter variables. The following is acceptable:
old=new;
The character in new squirts through the equal sign and lands in the charac ter variable old. After the preceding statement, both variables hold the same character. (It’s a copy operation, not a move.)
You can assign single characters to single-character variables. But. . . .
You still cannot use the equal sign to put a string of text into a string variable. Sorry. It just can’t be done.