
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
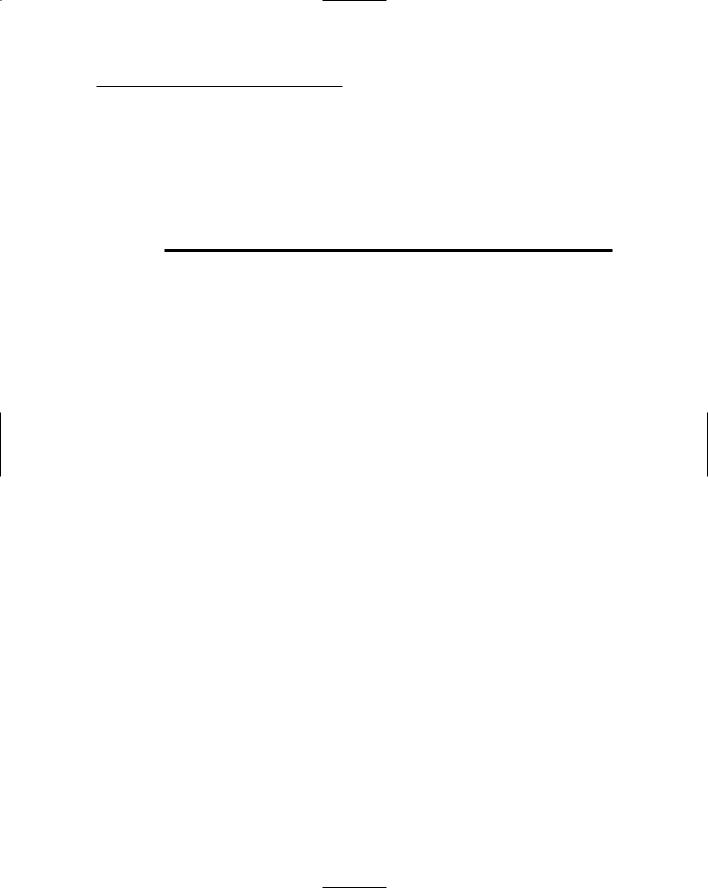
Chapter 2: Declaring Variables Constantly |
33 |
Declaring Variable Types
So far this chapter has been trumpeting that variables must be declared and that they must be assigned a type. Fortunately (ta-dah!), C++ provides a num ber of different variable types. See Table 2-1 for a list of variables, their advan tages, and limitations.
Table 2-1 |
|
C++ Variables |
Variable |
Example |
Purpose |
int |
1 |
A simple counting number, either positive or |
|
|
negative. |
|
|
|
unsigned int |
1U |
A counting number that’s only non-negative. |
|
|
|
long |
10L |
A potentially larger version of int. There is |
|
|
no difference between long and int with |
|
|
Dev-C++ and Microsoft Visual C++.NET. |
|
|
|
unsigned long |
10UL |
A nonnegative long integer. |
|
|
|
float |
1.0F |
A single precision real number. This smaller |
|
|
version takes less memory than a double |
|
|
but has less accuracy and a smaller range. |
double |
1.0 |
A standard floating-point variable. |
|
|
|
char |
‘c’ |
A single char variable stores a single alpha |
|
|
betic or digital character. Not suitable for |
|
|
arithmetic. |
|
|
|
string |
“this is |
A string of characters forms a sentence or |
|
a string” |
phrase. |
bool |
true |
The only other value is false. No I mean, it’s |
|
|
really false. Logically false. Not “false” as |
|
|
in fake or ersatz or . . . never mind. |
|
|
|
It may seem odd that the standard floating length variable is called double while the “off size” is float. In days gone by, memory was an expensive asset — you could reap significant space savings by using a float variable. This is no longer the case. That, combined with the fact that modern processors perform double precision calculations at the same speed as float, makes the double the default. Bigger is better, after all.
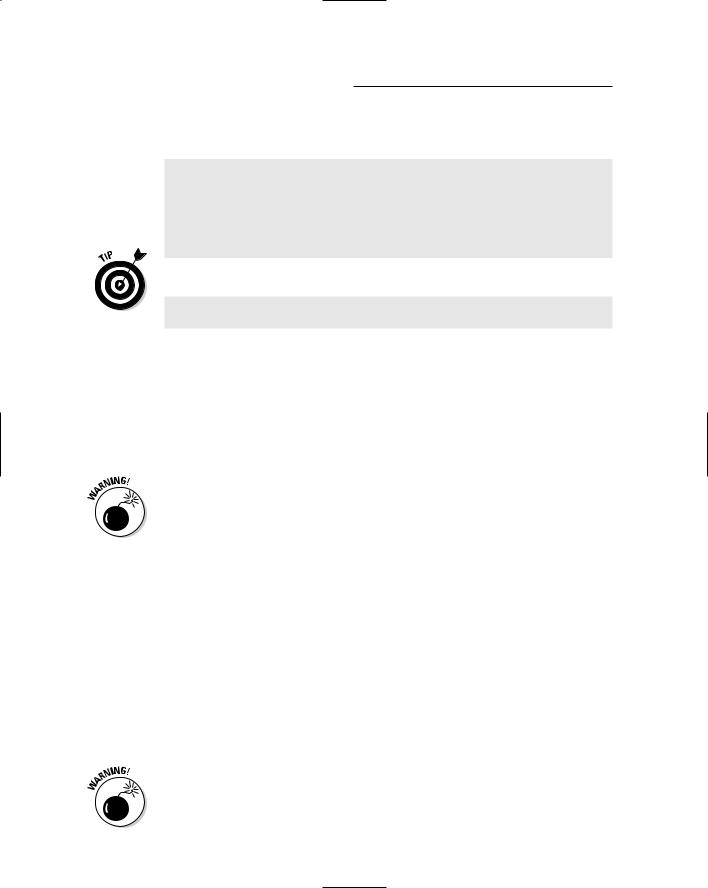
34 |
Part I: Introduction to C++ Programming |
The following statement declares a variable lVariable as type long and sets it equal to the value 1, while dVariable is a double set to the value 1.0:
//declare a variable and set it to 1 long lVariable;
lVariable = 1;
//declare a variable of type double and set it to 1.0 double dVariable;
dVariable = 1.0;
You can declare a variable and initialize it in the same statement:
int nVariable = 1; // declare a variable and
// initialize it to 1
Although such declarations are common, the only benefit to initializing a vari able in the declaration is that it saves typing.
A char variable can hold a single character; a string (which isn’t really a vari able but works like one for most purposes) holds a string of characters. Thus, ‘C’ is a char that contains the character C, whereas “C” is a string with one character in it. A rough analogy is that a ‘C’ corresponds to a nail in your hand, whereas “C” corresponds to a nail gun with one nail left in the magazine. (Chap ter 9 describes strings in detail.)
If an application requires a string, you’ve gotta provide one, even if the string contains only a single character. Providing nothing but the character just won’t do the job.
Types of constants
A constant is an explicit number or character (such as 1, 0.5, or ‘c’) that doesn’t change. As with variables, every constant has a type. In an expression such as n = 1; (for example), the constant 1 is an int. To make 1 a long integer, write the statement as n = 1L;. The analogy is as follows: 1 represents a single ball in the bed of a pickup truck, whereas 1L is a single ball in the bed of a dump truck. The ball is the same, but the capacity of its container is much larger.
Following the int to long comparison, 1.0 represents the value 1, but in a floating-point container. Notice, however, that the default for floating-point constants is double. Thus, 1.0 is a double number and not a float.
true is a constant of type bool. However, “true” (note the quotation marks) is a string of characters that spell out the word true. In addition, in keeping with C++’s attention to case, true is a constant, but TRUE has no meaning.
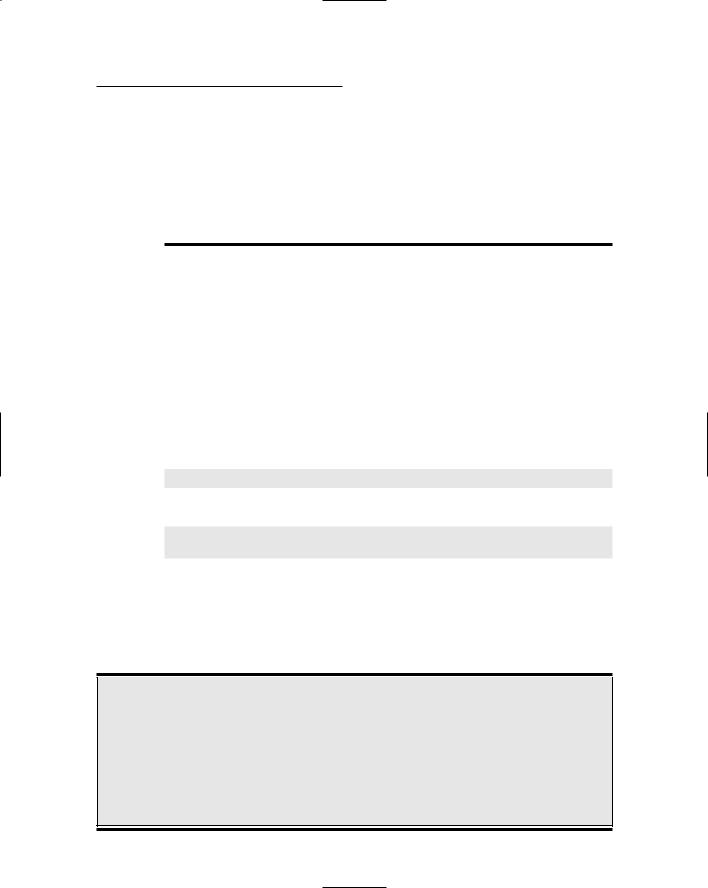
Chapter 2: Declaring Variables Constantly |
35 |
Special characters
You can store any printable character you want in a char or string vari able. You can also store a set of non-printable characters that is used as character constants. See Table 2-2 for a description of these important nonprintable characters.
Table 2-2 |
Special Characters |
Character Constant |
Action |
‘\n’ |
newline |
|
|
‘\t’ |
tab |
|
|
‘\0’ |
null |
|
|
‘\\’ |
backslash |
|
|
You have already seen the newline character at the end of strings. This char acter breaks a string and puts the parts on separate lines. A newline charac ter may appear anywhere within a string. For example,
“This is line 1\nThis is line 2”
appears on the output as
This is line 1
This is line 2
Similarly, the \t tab character moves output to the next tab position. (This position can vary, depending on the type of computer you’re using to run the program.) Because the backslash character is used to signify special charac ters, a character pair for the backslash itself is required. The character pair \\ represents the backslash.
C++ collision with file names
Windows uses the backslash character to sep arate folder names in the path to a file. (This is a remnant of MS-DOS that Windows has not been able to shake.) Thus, Root\FolderA\ File represents File within FolderA, which is a subdirectory of Root.
Unfortunately, MS-DOS’s use of backslash con flicts with the use of backslash to indicate an escape character in C++. The character \\ is a backslash in C++. The MS-DOS path Root\ FolderA\File is represented in C++ string as
Root\\FolderA\\File.