
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index

Introduction
Welcome to C++ For Dummies, 5th Edition. Think of this book as C++: Reader’s Digest Edition, bringing you everything you need to know
without the boring stuff.
What’s in This Book
C++ For Dummies is an introduction to the C++ language. C++ For Dummies starts from the beginning (where else?) and works its way from early concepts and through more sophisticated techniques. It doesn’t assume that you have any prior knowledge, at least, not of programming.
C++ For Dummies is rife with examples. Every concept is documented in numer ous snippets and several complete programs.
Unlike other C++ programming books, C++ For Dummies considers the “why” just as important as the “how.” The features of C++ are like pieces of a jigsaw puzzle. Rather than just present the features, I think it’s important that you understand how they fit together.
If you don’t understand why a particular feature is in the language, you won’t truly understand how it works. After you finish this book, you’ll be able to write a reasonable C++ program, and, just as important, you’ll understand why and how it works.
C++ For Dummies can also be used as a reference: If you want to understand what’s going on with all the template stuff, just flip to Chapter 27, and you’re there. Each chapter contains necessary references to other earlier chapters in case you don’t read the chapters in sequence.
C++ For Dummies is not operatingor system-specific. It is just as useful to Unix or Linux programmers as it is to Windows-based developers. C++ For Dummies doesn’t cover Windows or .NET programming. You have to master C++ before you can move on to Windows and .NET programming.
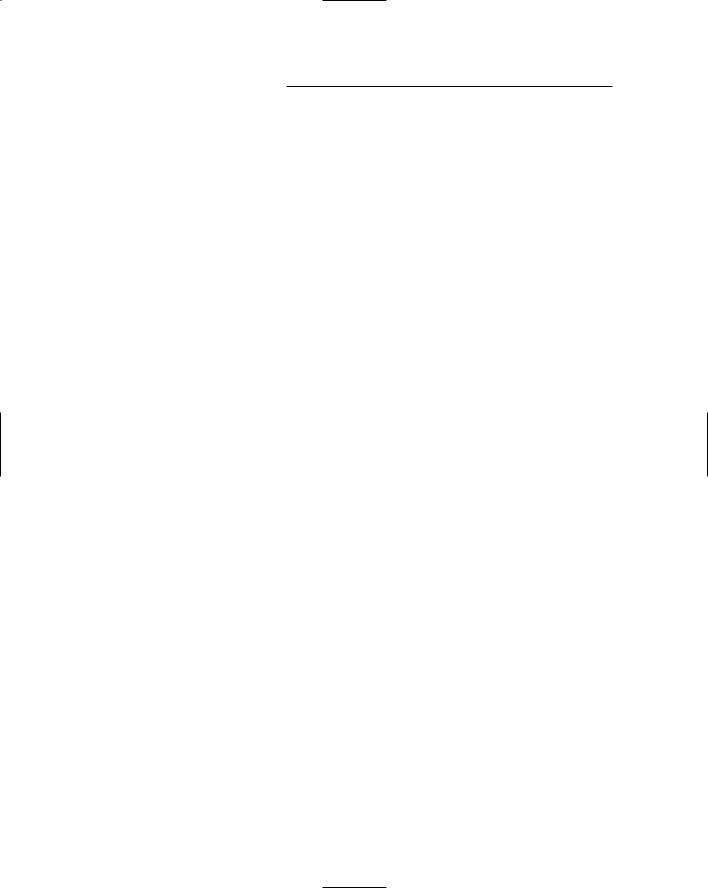
2C++ For Dummies, 5th Edition
What’s on the CD
The CD-ROM included with C++ For Dummies contains the source code for the examples in this book. This can spare you considerable typing.
Your computer can’t execute these or any other C++ program directly. You have to run your C++ programs through a C++ development environment, which spits out an executable program. (Don’t worry, this procedure is explained in Chapter 1.)
The programs in C++ For Dummies are compatible with any standard C++ envi ronment, but don’t worry if you don’t already own one. A full-featured C++ envi ronment known as Dev-C++ is contained on the enclosed CD-ROM. You can use this tool to write your own C++ programs as well as explore the programs from the book.
No worries if you already own Visual Studio.NET. Some people need an intro duction to C++ before going into the many features offered by .NET. C++ For Dummies is just as happy with Visual Studio as it is with its own Dev-C++. C++ For Dummies does not contain Visual Studio.NET. However, the programs in the book have been tested for compatibility with the industry standard “unman aged C++” portion of Visual Studio.NET.
What Is C++?
C++ is an object-oriented, low-level ANSI and ISO standard programming lan guage. As a low-level language similar to and compatible with its predecessor C, C++ can generate very efficient, very fast programs.
As an object-oriented language, C++ has the power and extensibility to write large-scale programs. C++ is one of the most popular programming languages for all types of programs. Most of the programs you use on your PC every day are written in C++.
C++ has been certified as a 99.9 percent pure standard. This makes it a portable language. There is a C++ compiler for every major operating system, and they all support the same C++ language. (Some operating systems support exten sions to the basic language, but all support the C++ core.)
Conventions Used in This Book
When I describe a message or information that you see onscreen, it appears like this:
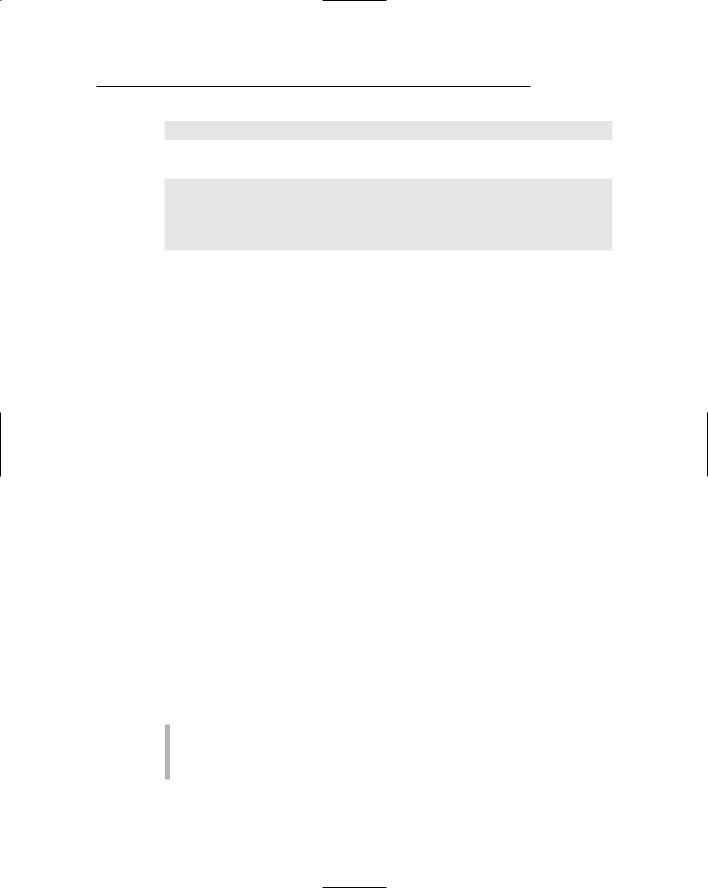
Introduction 3
Hi mom!
In addition, code listings appear as follows:
// some program void main()
{
...
}
If you are entering these programs by hand, you must enter the text exactly as shown with one exception: The number of spaces is not critical, so don’t worry if you enter one too many or one too few spaces.
C++ words are usually based on English words with similar meanings. This can make reading a sentence containing both English and C++ difficult to make out without a little help. To help out, C++ commands and function names appear in a different font like this. In addition, function names are always followed by an open and closed parenthesis like myFavoriteFunction(). The argu ments to the function are left off except when there’s a specific need to make them easier to read. It’s a lot easier to say: “this is myFavoriteFunction()” than “this is myFavoriteFunction(int, float).”
Sometimes, the book directs you to use specific keyboard commands. For exam ple, when the text instructs you to press Ctrl+C, it means that you should hold down the Ctrl key while pressing the C key and then release both together.
Don’t type the plus sign.
Sometimes, I’ll tell you to use menu commands, such as File Open. This nota tion means to use the keyboard or mouse to open the File menu and then choose the Open option. Finally, both Dev-C++ and Visual Studio.NET define function keys for certain common operations — unfortunately, they don’t use the same function keys. To avoid confusion, I rarely use function keys in the book — I couldn’t have kept the two straight anyway.
How This Book Is Organized
Each new feature is introduced by answering the following three questions:
What is this new feature?
Why was it introduced into the language?
How does it work?
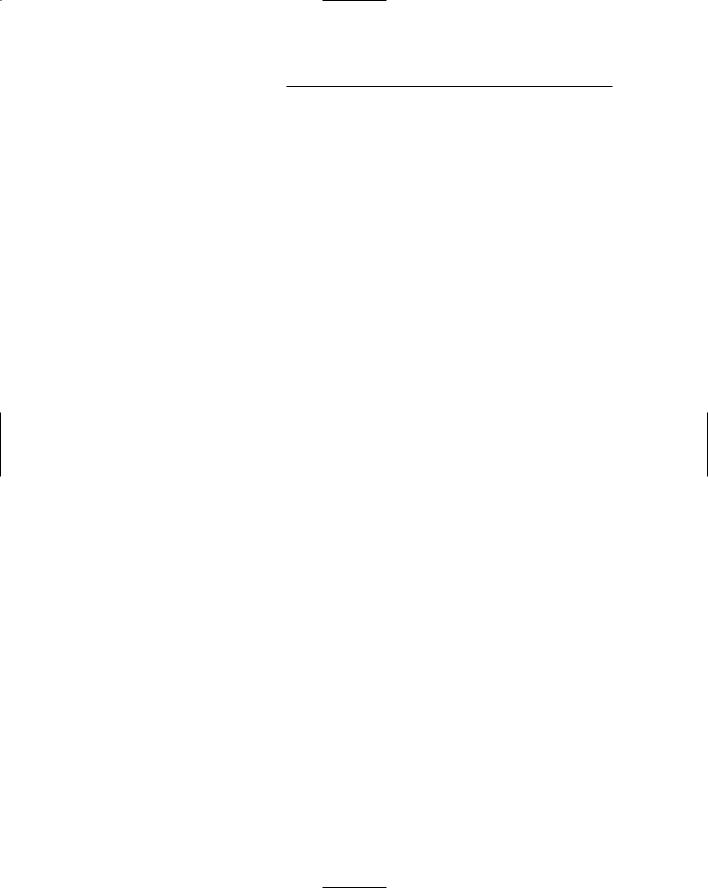
4C++ For Dummies, 5th Edition
Small pieces of code are sprinkled liberally throughout the chapters. Each demonstrates some newly introduced feature or highlights some brilliant point I’m making. These snippets may not be complete and certainly don’t do any thing meaningful. However, every concept is demonstrated in at least one functional program.
Note: A good programmer doesn’t let lines of code extend too far because it makes them hard to read. I have inserted newlines appropriately to limit my programs to the width of the book page.
And There’s More
A real-world program can take up lots of pages. However, seeing such a pro gram is an important didactic tool for any reader. I have included a series of programs along with an explanation of how these programs work on the enclosed CD-ROM.
I use one simple example program that I call BUDGET. This program starts life as a simple, functionally-oriented BUDGET1. This program maintains a set of simple checking and savings accounts. The reader is encouraged to review this program at the end of Part II. The subsequent version, BUDGET2, adds the object-oriented concepts presented in Part III. The examples work their way using ever more features of the language, culminating with BUDGET5, which you should review after you master all the chapters in the book. The BUDGET programs can be found on the book’s CD-ROM. For a complete overview of the CD-ROM’s contents, see this book’s Appendix.
Part I: Introduction to C++ Programming
Part I starts you on your journey. You begin by examining what it means to write a computer program. From there, you step through the syntax of the language (the meaning of the C++ commands).
Part II: Becoming a Functional
C++ Programmer
In this part, you expand upon your newly gained knowledge of the basic com mands of C++ by adding the capability to bundle sections of C++ code into mod ules and reusing these modules in programs.