
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
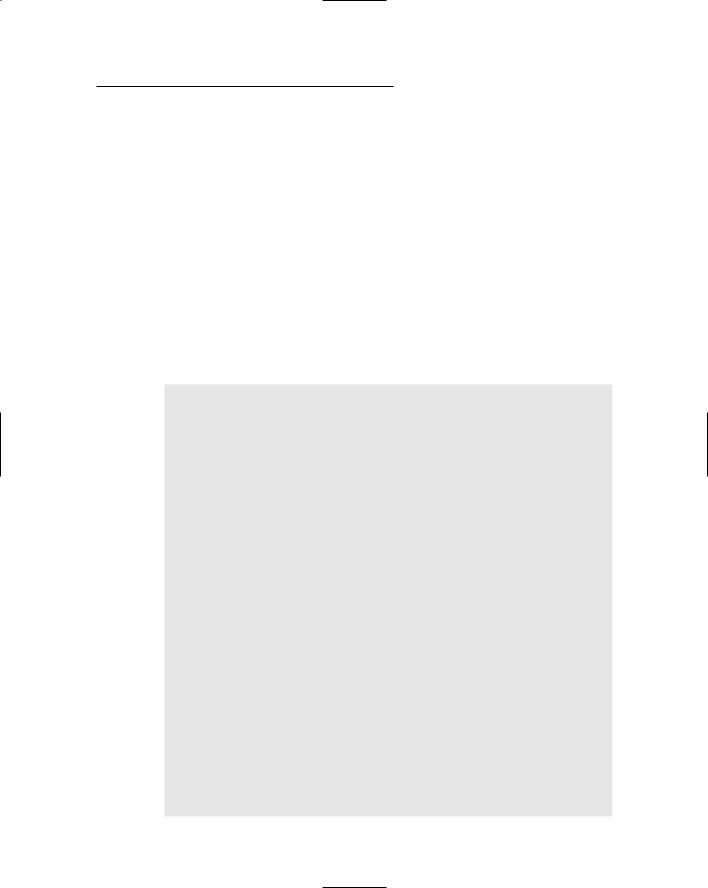
Chapter 13: Making Classes Work 181
This class definition contains nothing more than a prototype declaration for the function deposit(). The function definition appears separately. The member function prototype declaration in the structure is analogous to any other prototype declaration and, like all prototype declarations, is required.
Notice how the function nickname deposit() was good enough when the function was defined within the class. When defined outside the class, how ever, the function requires its extended name.
Overloading Member Functions
Member functions can be overloaded in the same way that conventional func tions are overloaded (see Chapter 6 if you don’t remember what that means). Remember, however, that the class name is part of the extended name. Thus, the following functions are all legal:
class Student
{
public:
//grade -- return the current grade point average float grade();
//grade -- set the grade and return previous value float grade(float newGPA);
//...data members and other stuff...
};
class Slope
{
public:
//grade -- return the percentage grade of the slope float grade();
//...stuff goes here too...
};
// grade -- return the letter equivalent of a numerical grade char grade(float value);
int main(int argcs, char* pArgs[])
{
Student s;
s.grade(3.5); // Student::grade(float) float v = s.grade(); // Student::grade()
char c = grade(v); // ::grade(float)
Slope o;
float m = o.grade(); // Slope::grade() return 0;
}
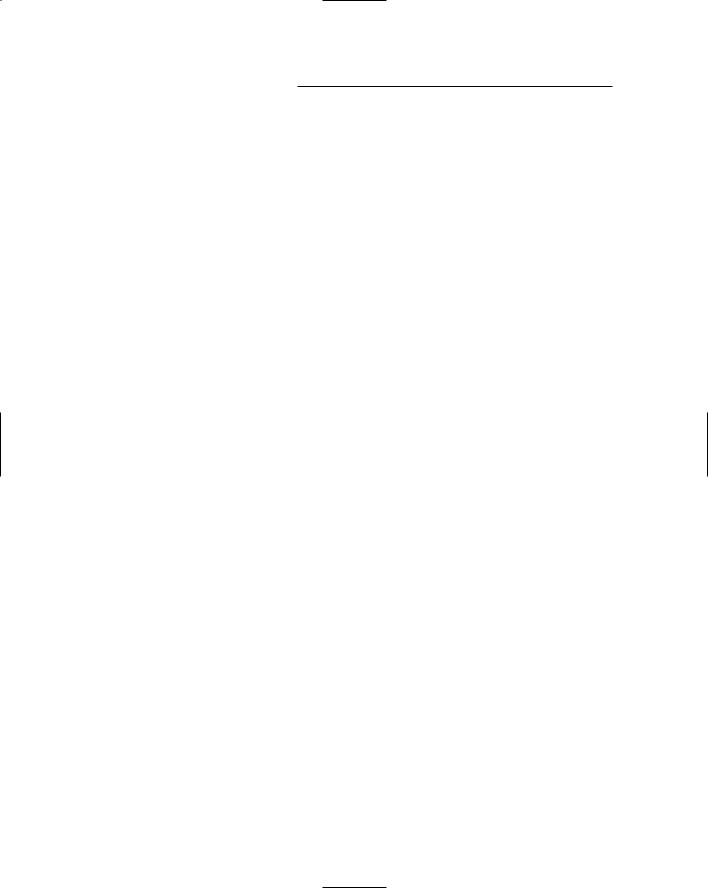
182 Part III: Introduction to Classes
Each call made from main() is noted in the comments with the extended name of the function called.
When calling overloaded functions, not only the arguments of the function but also the type of the object (if any) with which the function is invoked are used to disambiguate the call. (The term disambiguate is object-oriented talk for “decide at compile time which overloaded function to call.” A mere mortal might say “differentiate.”)
In the example, the first two calls to the member functions,
Student::grade(float) and Student::grade(), are differentiated by their argument lists and the type of object used. The call to s.grade() calls
Student::grade() because s is of type Student.
The third call has no object, so it unambiguously denotes the non-member function ::grade(float).
The final call is made with an object of type Slope; it must refer to the member function Slope::grade().

Chapter 14
Point and Stare at Objects
In This Chapter
Examining the object of arrays of objects
Getting a few pointers on object pointers
Strong typing — getting picky about our pointers
Navigating through lists of objects
C++ programmers are forever generating arrays of things — arrays of ints, arrays of floats, so why not arrays of students? Students stand in
line all the time — a lot more than they care to. The concept of Student objects all lined up quietly awaiting their name to jump up to perform some mundane task is just too attractive to pass up.
Defining Arrays of and Pointers to Simple Things
An array is a sequence of identical objects much like the identical houses on a street that make up one of those starter neighborhoods. Each element in the array carries an index, which corresponds to the number of elements from the beginning of the array — the first element in the array carries an offset of 0.
C++ arrays are declared by using the bracket symbols containing the number of elements in the array:
int array[10]; |
// declare an array of 10 elements |
The individual elements of the array can be accessed by counting the offset from the beginning of the array:
array[0] = 10; // assign 10 to the first element
array[9] = 20; // assign 20 to the last element
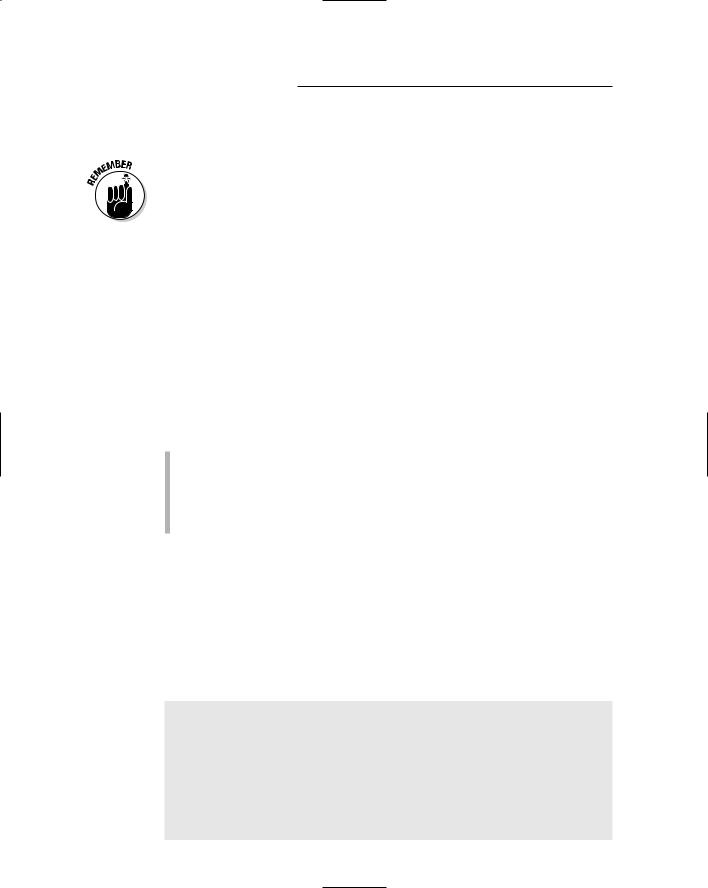
184 Part III: Introduction to Classes
The program first assigns the value 10 to the first element in the array — the element at the beginning of the array. The program then assigns 20 to the last element in the array — element at offset nine from the beginning.
Always remember that C++ indices start at 0 and go through the size of the array minus 1.
I like to use the analogy of a street with houses. The array name represents the name of the street, and the house number in that street represents the array index. Similarly, variables can be identified by their unique address in com puter memory. These addresses can be calculated and stored for later use.
int variable; |
// declare an int object |
int* pVariable = &variable; |
// store its address |
|
// in pVariable |
*pVariable = 10; |
// assign 10 into the int |
|
// pointed at by pVariable |
The pointer pVariable is declared to contain the address of variable. The assignment stores 10 into the int pointed at by pVariable.
If you apply the house analogy one last time (I promise):
variable is a house.
pVariable is like a piece of paper containing the address of the house.
The final assignment delivers the message 10 to the house whose address is written on pVariable just like a postman might (except unlike my postman, computers don’t deliver mail to the wrong address).
Chapter 7 goes into the care and feeding of arrays of simple (intrinsic) vari ables, and Chapter 8 and Chapter 9 describe simple pointers in detail.
Declaring Arrays of Objects
Arrays of objects work the same way arrays of simple variables work. Take, for example, the following snippet from ArrayOfStudents.cpp:
// ArrayOfStudents - define an array of Student objects
// |
and access an element in it. This |
// |
program doesn’t do anything |
class Student |
|
{ |
|
public:
int semesterHours; float gpa;
float addCourse(int hours, float grade);
};