
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index

|
|
|
Chapter 8: Taking a First Look at C++ Pointers 117 |
|
n |
= |
0 |
||
|
||||
lower = |
0 |
|
||
Storing |
13.0 into the location &n |
The final results are:
upper |
= 1076494336 |
|
n |
= |
0 |
lower |
= |
0 |
Press |
any key to continue . . . |
The house equivalent goes something like this:
House* houseAddress = &”123 Main Street”;
Hotel* hotelAddress;
hotelAddress = (Hotel*)houseAddress; *hotelAddress = TheRitz;
houseAddress is initialized to point to my house. The variable hotelAddress is a pointer to a hotel. Now, the house address is cast into the address of a hotel and saved off into the variable hotelAddress. Finally, TheRitz is plopped down on top of my house. Because TheRitz is slightly bigger than my house (okay, a lot bigger than my house), it isn’t surprising that TheRitz wipes out my neighbors’ houses as well.
The type of pointer saves the programmer from stuffing an object into a space that is too big or too small. The assignment *pintVar = 100.0; actu ally causes no problem — because C++ knows that pintVar points to an int, C++ knows to demote the 100.0 to an int before making the assignment.
Passing Pointers to Functions
One of the uses of pointer variables is in passing arguments to functions. To understand why this is important, you need to understand how arguments are passed to a function.
Passing by value
You may have noticed that it is not normally possible to change the value of a variable passed to a function from within the function. Consider the following example code segment:
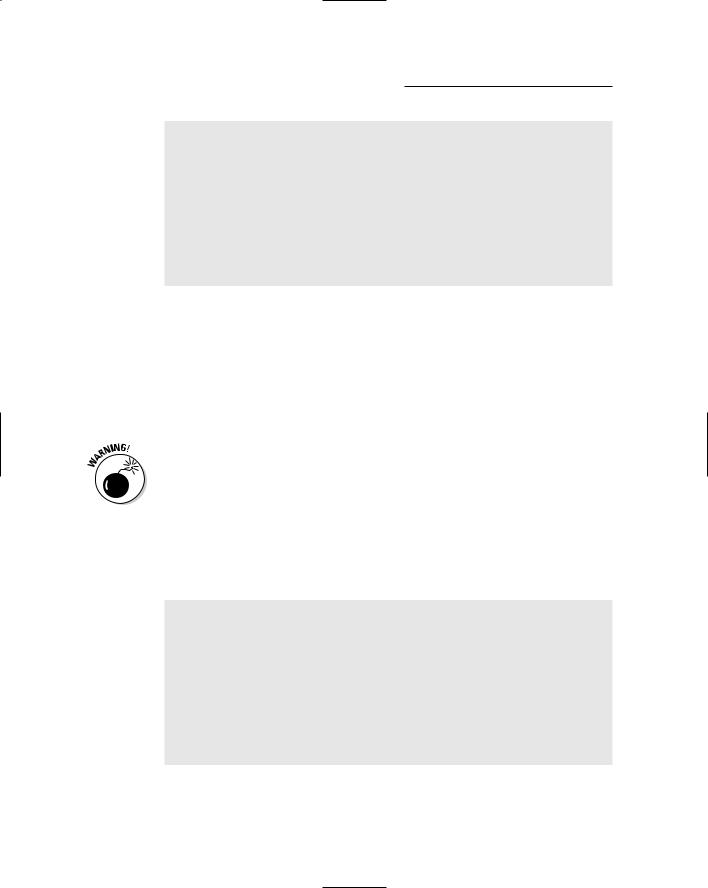
118 Part II: Becoming a Functional C++ Programmer
void fn(int intArg)
{
intArg = 10;
// value of intArg at this point is 10
}
void parent(void)
{
int n1 = 0; fn(n1);
// value of n1 at this point is 0
}
Here the parent() function initializes the integer variable n1 to zero. The value of n1 is then passed to fn(). Upon entering the function, intArg is equal to 10, the value passed. fn() changes the value of intArg before returning to parent(). Perhaps surprisingly, upon returning to parent(), the value of n1 is still 0.
The reason is that C++ doesn’t pass a variable to a function. Instead, C++ passes the value contained in the variable at the time of the call. That is, the expression is evaluated, even if it is just a variable name, and the result is passed.
It is easy for a speaker to get lazy and say something like, “Pass the variable x to the function fn().” This really means to pass the value of the expression x.
Passing pointer values
Like any other intrinsic type, a pointer may be passed as an argument to a function:
void fn(int* pintArg)
{
*pintArg = 10;
}
void parent(void)
{
int n = 0; |
|
fn(&n); |
// this passes the address of i |
} |
// now the value of n is 10 |
|
In this case, the address of n is passed to the function fn() rather than the value of n. The significance of this difference is apparent when you consider the assignment within fn().
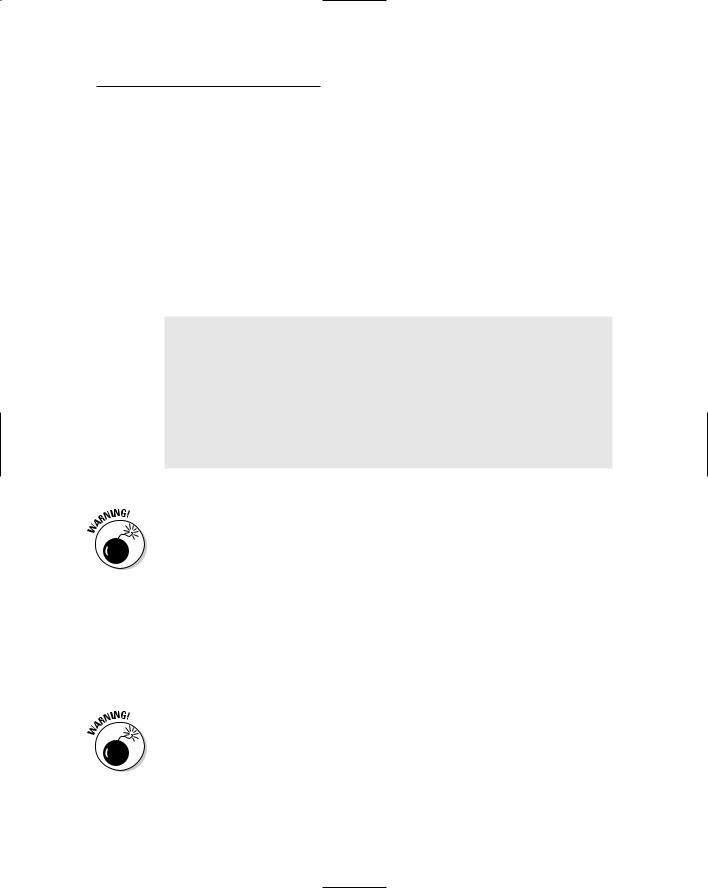
Chapter 8: Taking a First Look at C++ Pointers 119
Suppose n is located at address 0x102. Rather than the value 10, the call fn(&n) passes the value 0x102. Within fn(), the assignment *pintArg = 10 stores the value 10 into the int variable located at location 0x102, thereby overwriting the value 0. Upon returning to parent(), the value of n is 10 because n is just another name for 0x102.
Passing by reference
C++ provides a shorthand for passing arguments by address — a shorthand that enables you to avoid having to hassle with pointers. In the following example, the variable n is passed by reference.
void fn(int& intArg)
{
intArg = 10;
}
void parent(void)
{
int n = 0; fn(n);
// here the value of n is 10
}
In this case, a reference to n rather than its value is passed to fn(). The fn() function stores the value 10 into int location referenced by intArg.
Notice that reference is not an actual type. Thus, the function’s full name is fn(int) and not fn(int&).
Making Use of a Block of
Memory Called the Heap
The heap is an amorphous block of memory that your program can access as necessary. This section describes why it exists and how to use it.
Visual C++.NET allows the programmer to write code in what is known as managed mode in addition to the conventional, “unmanaged mode.” In man aged mode, the compiler handles the allocation and deallocation of memory. Managed programs rely upon the .NET framework. Only Visual C++.NET cur rently supports managed mode. This book only covers unmanaged mode programming.
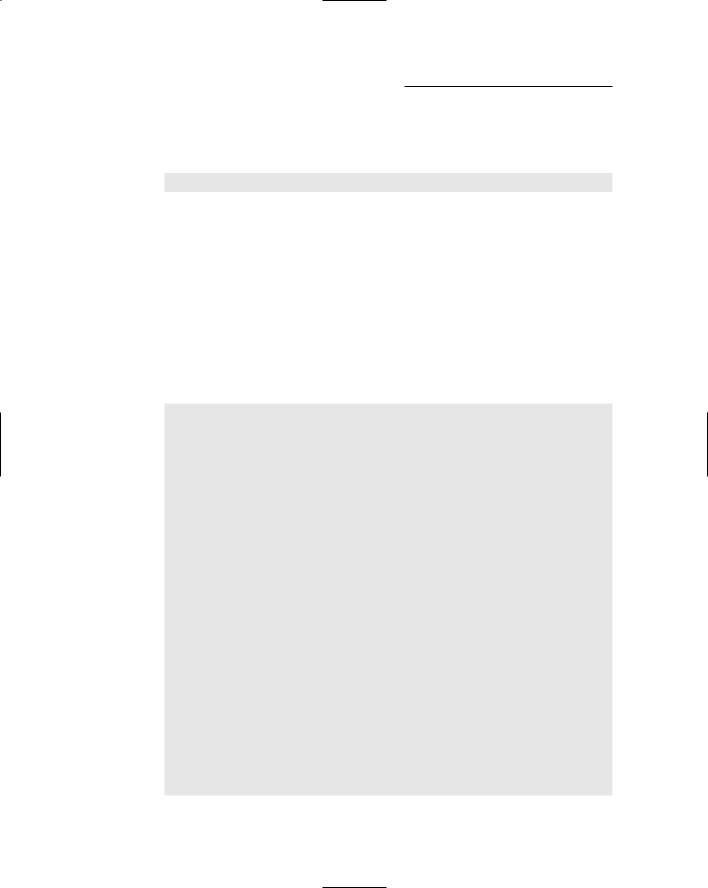
120 Part II: Becoming a Functional C++ Programmer
Just as it is possible to pass a pointer to a function, it is possible for a func tion to return a pointer. A function that returns the address of a double is declared as follows:
double* fn(void);
However, you must be very careful when returning a pointer. In order to understand the dangers, you must know something about variable scope. (No, I don’t mean a variable zoom rifle scope.)
Limiting scope
C++ variables have a property in addition to their value and type known as scope. Besides being a mouthwash, scope is the range over which a variable is defined.
Consider the following code snippet:
//the following variable is accessible to
//all functions and defined as long as the
//program is running(global scope)
int intGlobal;
//the following variable intChild is accessible
//only to the function and is defined only
//as long as C++ is executing child() or a
//function which child() calls (function scope) void child(void)
{
int intChild;
}
//the following variable intParent has function
//scope
void parent(void)
{
int intParent = 0; child();
int intLater = 0; intParent = intLater;
}
int main(int nArgs, char* pArgs[])
{
parent();
}