
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index

364 Part V: Optional Features
The string Container
The most common form of array is the zero-terminated character string used to display text, which clearly shows both the advantages and disadvantages of the array. Consider how easy the following appears:
cout << “This is a string”;
But things go sour quickly when you try to perform an operation even as simple as concatenating two strings:
char* concatCharString(char* s1, char* s2)
{
int length = strlen(s1) + strlen(s2) + 1; char* s = new char[length];
strcpy(s, s1); strcat(s, s2); return s;
}
The STL provides a string container to handle display strings. The string class provides a number of operations (including overloaded operators) to simplify the manipulation of character strings. The same concat() operation is performed as follows using string objects:
string concat(string s1, string s2)
{
return s1 + s2;
}
I tried to avoid using string class in other chapters in this book because I don’t explain it until here. However, most programmers use the string class more often than they use null terminated character arrays.
The following STLString program demonstrates just a few of the capabilities of the string class:
//STLString - demonstrates just a few of the features
//of the string class which is part of the
//Standard Template Library
#include <string> #include <cstdlib> #include <iostream> using namespace std;
// concat - return the concatenation of two strings string concat(string s1, string s2)
{
return s1 + s2;
}
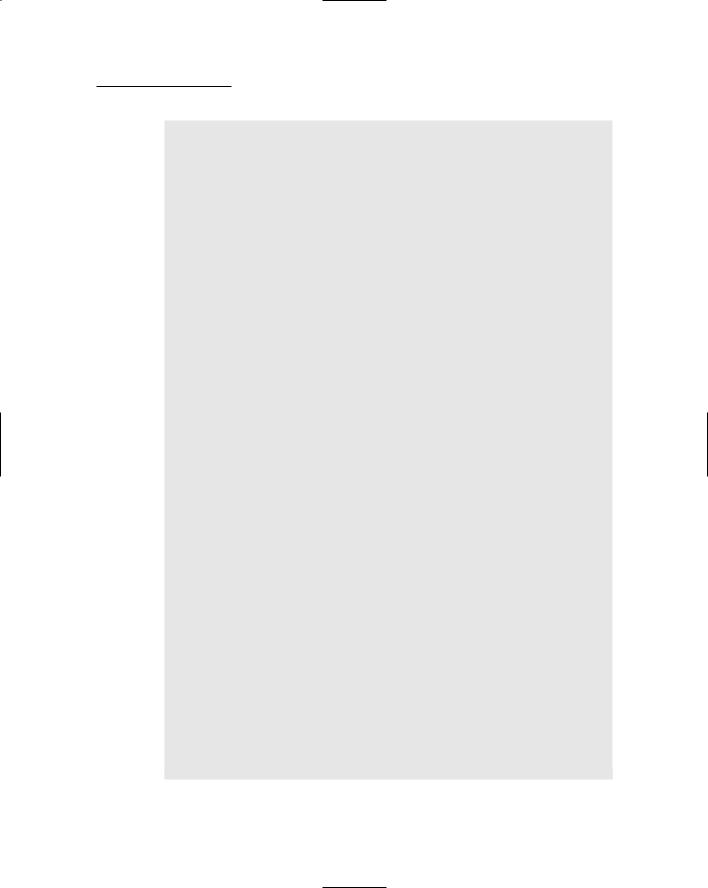
Chapter 28: Standardizing on the Standard Template Library 365
// removeSpaces - remove any spaces within a string string removeSpaces(string s)
{
//find the offset of the first space;
//keep searching the string until no more spaces found size_t offset;
while((offset = s.find(“ “)) != -1)
{
//remove the space just discovered
s.erase(offset, 1);
}
return s;
}
// insertPhrase - insert a phrase in the position of // <ip> for insertion point
string insertPhrase(string source)
{
size_t offset = source.find(“<ip>”); if (offset != -1)
{
source.erase(offset, 4); source.insert(offset, “Randall”);
}
return source;
}
int main(int argc, char* pArgs[])
{
//create a string that is the sum of two smaller strings cout << “string1 + string2 = “
<<concat(“string1 “, “string2”)
<<endl;
//create a test string and then remove all spaces from
//it using simple string methods
string s2(“The phrase”);
cout << “<” << s2 << “> minus spaces = <”
<<removeSpaces(s2) << “>” << endl;
//insert a phrase within the middle of an existing
//sentence (at the location of “<ip>”)
string s3 = “Stephen <ip> Davis”;
cout << s3 + “ -> “ + insertPhrase(s3) << endl;
system(“PAUSE”); return 0;
}
The operator+() operation performs the concatenation function that earlier sessions implemented using the concatCharacterString() method.
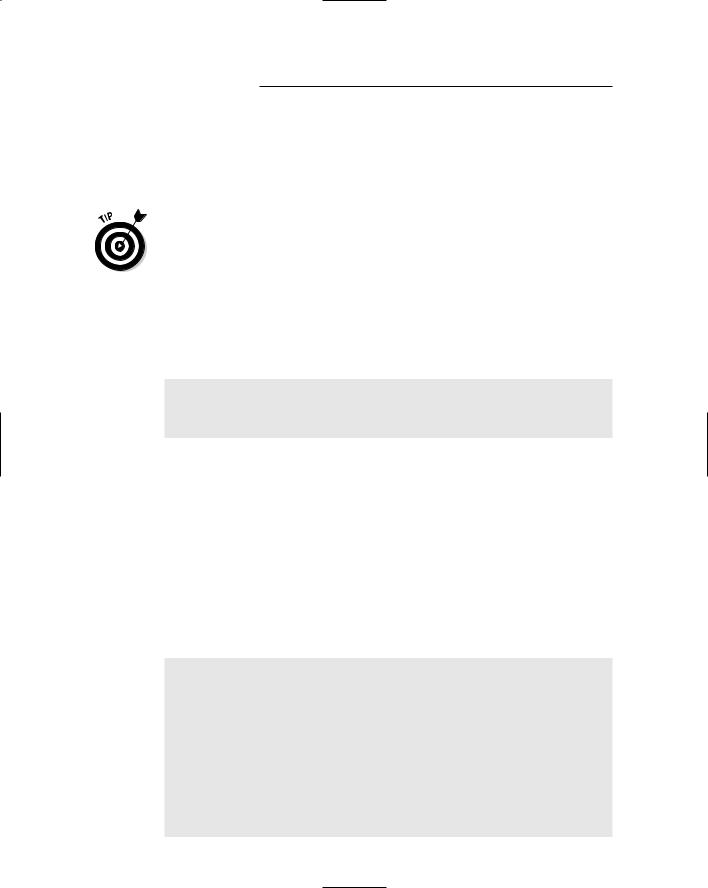
366 Part V: Optional Features
The removeSpaces() method removes any spaces found within the string provided. It does this by using the string.find() operation to return the offset of the first “ ” that it finds. Once found, removeSpaces() uses the erase() method to remove the space. The find() method returns an offset of –1 when no more spaces are left.
The type size_t is defined within the STL include files as an integer that can handle the largest array index possible on your machine. This is typically a long of some type; however, the size_t is used to further source code porta bility between computers. Visual Studio C++.NET will generate a warning if you use int instead.
The insertPhrase() method uses the find() method to find the insertion point. It then calls erase to remove the “<ip>” flag and the string.insert() to insert a new string within the middle of an existing string.
The resulting output is as follows:
string1 + string2 = string1 string2
<this is a test string> minus spaces = <thisisateststring>
Stephen <ip> Davis -> Stephen Randall Davis
Press any key to continue . . .
The list Containers
The Standard Template Library provides a large number of containers — many more than I can describe in a single session. However, I provide here a description of two of the more useful families of containers.
The STL list container retains objects by linking them together like Lego blocks. Objects can be snapped apart and snapped back together in any order. This makes the list ideal for inserting objects, sorting, merging, and otherwise rearranging objects. The following example STLList program uses the list container to sort a set of names:
//STLList - use the list container of the
//Standard Template Library to input
//and sort a string of names #include <list>
#include <string> #include <cstdio> #include <cstdlib> #include <iostream>
//declare a list of string objects
using namespace std; list<string> names;

Chapter 28: Standardizing on the Standard Template Library 367
int main(int argc, char* pArgs[])
{
// input a string of names
cout << “Input a name (input a null to terminate list)” << endl;
while(true)
{
string name; cin >> name;
if ((name.compare(“x”) == 0) || (name.compare(“X”) == 0))
{
break;
}
names.push_back(name);
}
//sort the list
//(this works since String implements a comparison
operator)
names.sort();
//display the sorted list
//keep displaying names until the collection is empty cout << “\nSorted output:” << endl; while(!names.empty())
{
//get the first name in the list
string name = names.front(); cout << name << endl;
// remove that name from the list names.pop_front();
}
system(“PAUSE”); return 0;
}
This example defines the variable names to be a list of string objects. The program starts by reading names from the keyboard. Each name is added to the end of the list names using the push_back() method. The program exits the loop when the user enters the name “x”. The list of names is sorted by invoking the single list method sort().
The program displays the sorted list of names by removing objects from the front of the list until the list is empty.
The following is an example output from the program: