
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index

228 Part III: Introduction to Classes
Avoiding the “object declaration trap”
Look again at the way the Student objects were declared in the ConstructorWDefaults example:
Student noName;
Student freshMan(“Smell E. Fish”); Student xfer(“Upp R. Classman”, 80, 2.5);
All Student objects except noName are declared with parentheses surrounding the arguments to the constructor. Why is noName declared without parentheses?
To be neat and consistent, you may think you could have declared noName as follows:
Student noName();
Unfortunately, C++ allows a declaration with only an open and close parentheses. However, it doesn’t mean what you think it does at all. Instead of declaring an object noName of class Student to be constructed with the default
constructor, it declares a function that returns an object of class Student by value. Surprise!
The following two declarations demonstrate how similar the new C++ format for declaring an object is to that of declaring a function. (I think this was a mistake, but what do I know?) The only difference is that the function declaration contains types in the parentheses, whereas the object declaration contains objects:
Student thisIsAFunc(int);
Student thisIsAnObject(10);
If the parentheses are empty, nothing can dif ferentiate between an object and a function. To retain compatibility with C, C++ chose to make a declaration with empty parentheses a function. (A safer alternative would have been to force the keyword void in the function case, but that would not have been compatible with existing C programs.)
Constructing Class Members
In the preceding examples, all data members are of simple types, such as int and float. With simple types, it’s sufficient to assign a value to the variable within the constructor. Problems arise when initializing certain types of data members, however.
Constructing a complex data member
Members of a class have the same problems as any other variable. It makes no sense for a Student object to have some default ID of zero. This is true even if the object is a member of a class. Consider the following example:
//
//ConstructingMembers - a class may pass along arguments
// |
to the members’ constructors |
// |
|
#include <cstdio> |
|
#include <cstdlib> |
|
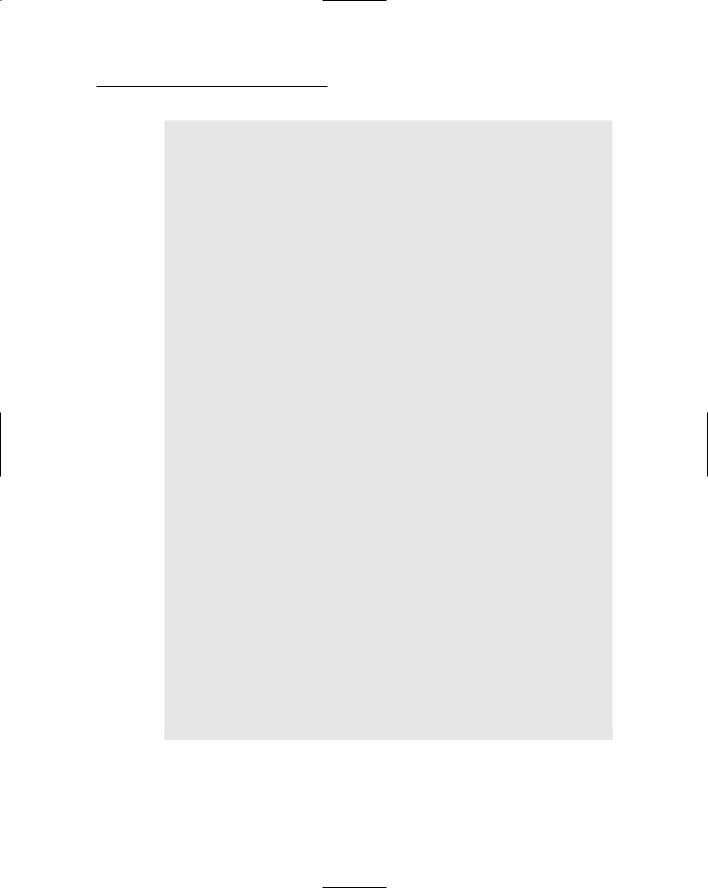
Chapter 17: Making Constructive Arguments 229
#include <iostream> #include <strings.h> using namespace std;
const int MAXNAMESIZE = 40;
int nextStudentId = 0; class StudentId
{
public:
StudentId()
{
value = ++nextStudentId;
cout << “Assigning student id “ << value << endl;
}
protected: int value;
};
class Student
{
public:
Student(char* pName)
{
strncpy(name, pName, MAXNAMESIZE); name[MAXNAMESIZE - 1] = ‘\0’; semesterHours = 0;
gpa = 0.0;
}
// ...other public members...
protected:
char name[MAXNAMESIZE]; int semesterHours; float gpa;
StudentId id;
};
int main(int argcs, char* pArgs[])
{
Student s(“Chester”);
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
A student ID is assigned to each student as the student object is constructed. In this example, IDs are handed out sequentially using the global variable nextStudentId to keep track.
This Student class contains a member id of class StudentId. The constructor for Student can’t assign a value to this id member because Student does not
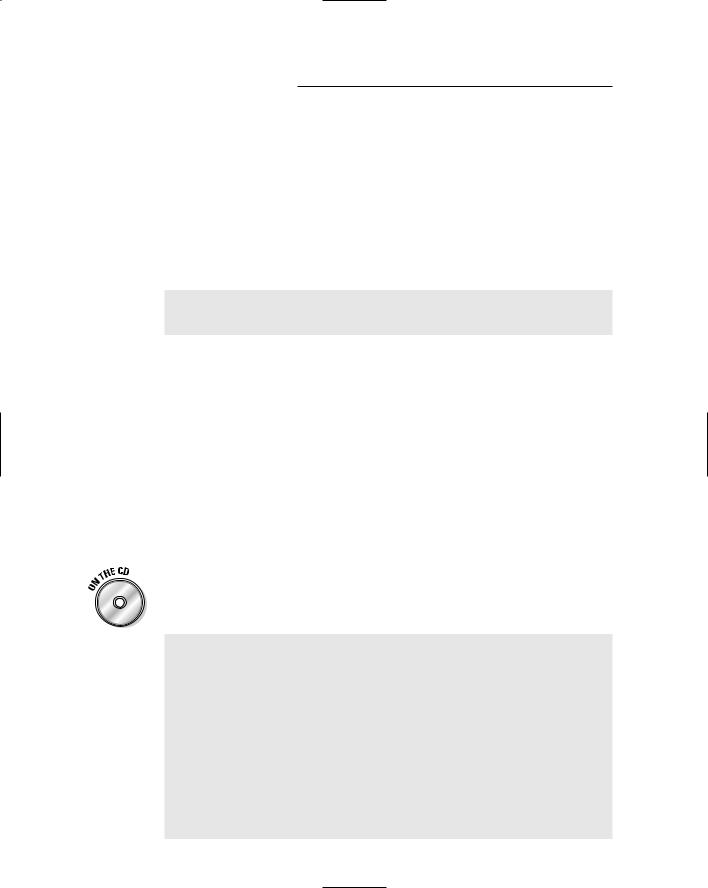
230 Part III: Introduction to Classes
have access to the protected members of StudentId. You could make Student a friend of StudentId, but that violates the “you take care of your business, I’ll take care of mine” philosophy. Somehow, you need to invoke the construc tor for StudentId when Student is constructed.
C++ does this for you automatically in this case, invoking the default con structor StudentId::StudentId() on id. This occurs after the Student constructor is called, but before control passes to the first statement in the constructor. (Single-step the preceding program in the debugger to see what I mean. As always, be sure that inline functions are forced outline.) Following is the output that results from executing this program:
assigning student id 1 constructing Student Chester Press any key to continue . . .
Notice that the message from the StudentId constructor appears before the output from the Student constructor. (By the way, with all these construc tors performing output, you may think that constructors must output some thing. Most constructors don’t output a bloody thing.)
If the programmer does not provide a constructor, the default constructor provided by C++ automatically invokes the default constructors for data members. The same is true come harvesting time. The destructor for the class automatically invokes the destructor for data members that have destructors. The C++-provided destructor does the same.
Okay, this is all great for the default constructor. But what if you want to invoke a constructor other than the default? Where do you put the object? For example, assume that a student ID is provided to the Student construc tor, which passes the ID to the constructor for class StudentId.
Let me first show you what doesn’t work. Consider the following program segment (only the relevant parts are included here — the entire program, ConstructSeparateID, is on the CD-ROM that accompanies this book):
class Student
{
public:
Student(char *pName = “no name”, int ssId = 0)
{
cout << “constructing student “ << pName << endl; strncpy(name, pName, MAXNAMESIZE);
name[MAXNAMESIZE - 1] |
= ‘\0’; |
// don’t try this at home kids. It doesn’t work |
|
StudentId id(ssId); |
// construct a student id |
}
protected:
char name[MAXNAMESIZE]; StudentId id;
};
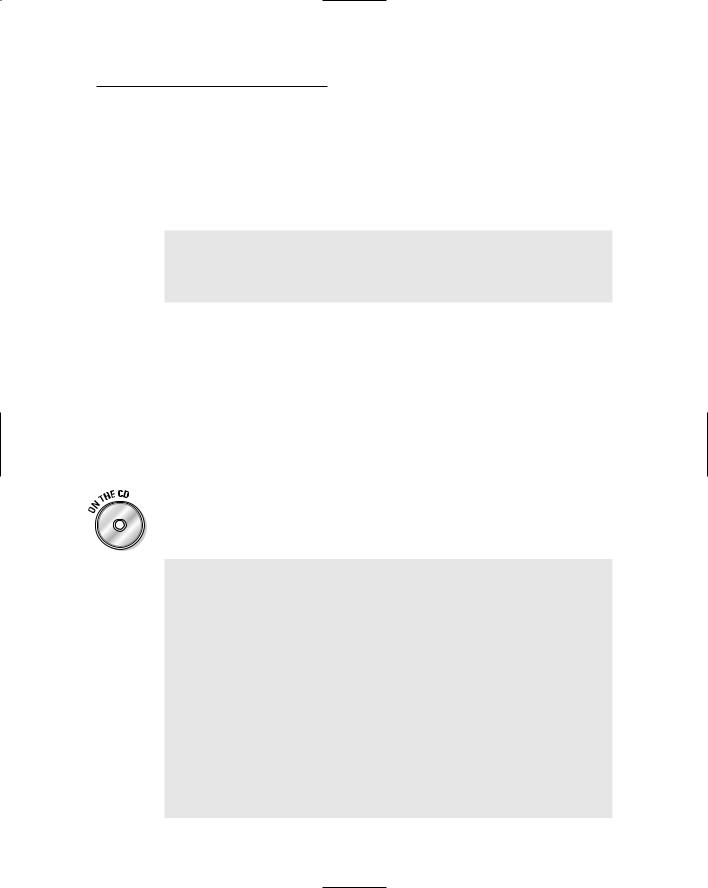
Chapter 17: Making Constructive Arguments 231
The constructor for StudentId has been changed to accept a value exter nally (the default value is necessary to get the example to compile, for rea sons that will become clear shortly). Within the constructor for Student, the programmer (that’s me) has (cleverly) attempted to construct a StudentId object named id.
If you look at the output from this program, you notice a problem:
assigning student id 0 constructing student Chester assigning student id 1234 This message from main
Press any key to continue . . .
The first problem is that the constructor for StudentId appears to be invoked twice, once with zero and a second time with the expected 1234. Then you can see that the 1234 object is destructed before the output string in main(). Apparently the StudentId object is destructed within the Student constructor.
The explanation for this rather bizarre behavior is clear. The data member id already exists by the time the body of the constructor is entered. Instead of constructing the existing data member id, the declaration provided in the constructor creates a local object of the same name. This local object is destructed upon returning from the constructor.
Somehow, you need a different mechanism to indicate “construct the existing member; don’t create a new one.” This mechanism needs to appear before the open brace, before the data members are declared. C++ provides a con struct for this, as shown in the following ConstructDataMembers program:
//
//ConstructDataMember - construct a data member
// |
to a value other than the default |
// |
|
#include <cstdio> |
|
#include <cstdlib> |
|
#include <iostream> |
|
#include <strings.h> |
|
using namespace std;
const int MAXNAMESIZE = 40; class StudentId
{
public:
StudentId(int id = 0)
{
value = id;
cout << “assigning student id “ << value << endl;
}