
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
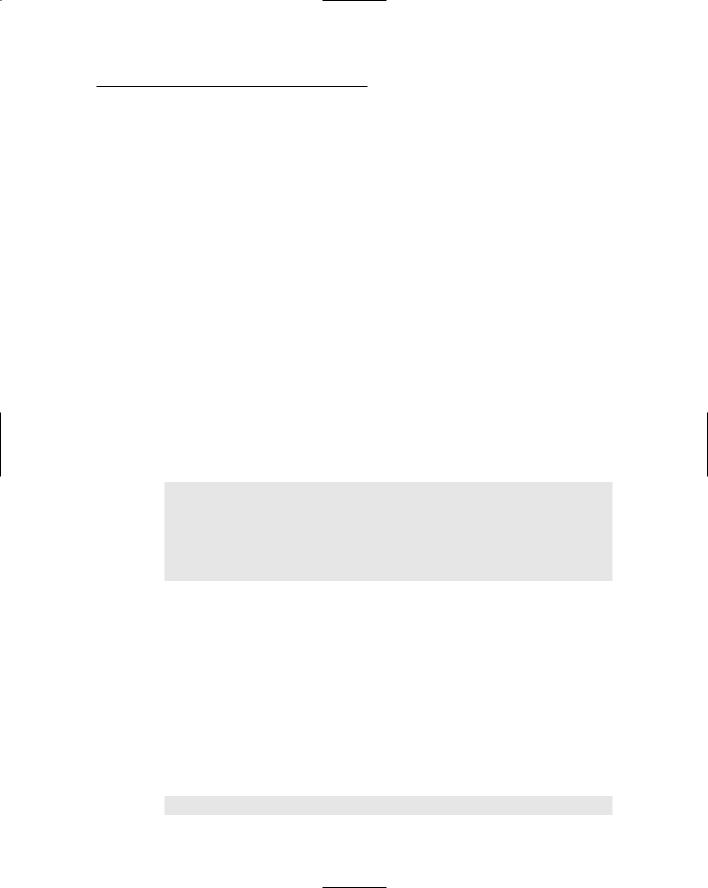
Chapter 14: Point and Stare at Objects 195
Linking Up with Linked Lists
The second most common structure after the array is called a list. Lists come in different sizes and types; however, the most common one is the linked list. In the linked list, each object points to the next member in a sort of chain that extends through memory. The program can simply point the last element in the list to an object to add it to the list. This means that the user doesn’t have to declare the size of the linked list at the beginning of the program — you can cause the linked list to grow (and shrink) as necessary by adding (and removing) objects.
The cost of such flexibility is speed of access. You can’t just reach in and grab the tenth element, for example, like you would in the case of an array. Now, you have to start at the beginning of the list and link ten times from one object to the next.
A linked list has one other feature besides its run-time expandability (that’s good) and its difficulty in accessing an object at random (that’s bad) — a linked list makes significant use of pointers. This makes linked lists a great tool for giving you experience in manipulating pointer variables.
Not every class can be used to create a linked list. You declare a linkable class as follows:
class LinkableClass
{
public:
LinkableClass* pNext;
// other members of the class
};
The key is using the pNext pointer to an object of class LinkableClass. At first blush, this seems odd indeed — a class contains a pointer to itself? Actually, this says that the class Linkable contains a pointer to another object also of class Linkable.
The pNext pointer is similar to the appendage used to form a chain of chil dren crossing the street. The list of children consists of a number of objects, all of type child. Each child holds onto another child.
The head pointer is simply a pointer of type LinkableClass*: To keep tor turing the child chain analogy, the teacher points to an object of class child. (It’s interesting to note that the teacher is not a child — the head pointer is not of type LinkableClass*.)
LinkableClass* pHead = (LinkableClass*)0;
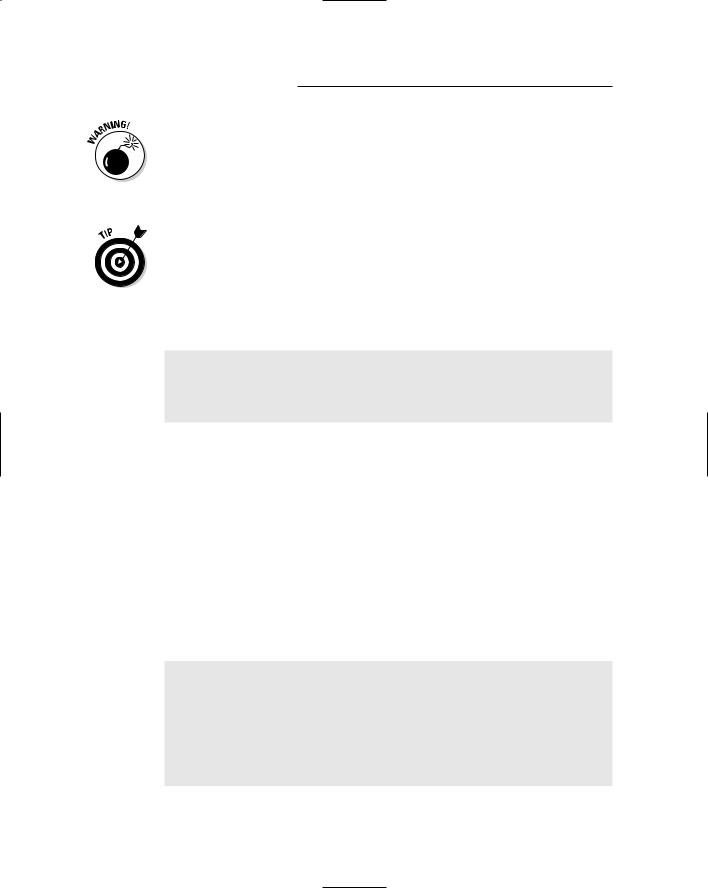
196 Part III: Introduction to Classes
Always initialize any pointer to 0. Zero, generally known as null when used in the context of pointers, is universally known as the non-pointer. In any case, referring to address 0 always causes the program to halt immediately. The cast from the int 0 to LinkableClass* is not necessary. C++ understands 0 to be of all types, sort of the “universal pointer.” However, I find the use of explicit casts a good practice.
The pointer to the first member in a linked list is called the head pointer. The pointer to the last member, if there is one, is called the tail pointer — hence, the name pHead in this example. (I also like the name because it sounds like you’re insulting someone by calling them a “pea head.” Don’t even get me started on a pointer to a pHead.)
To see how linked lists work in practice, consider the following function, which adds the argument passed it to the beginning of a list:
void addHead(LinkableClass* pLC)
{
pLC->pNext = pHead; pHead = pLC;
}
Here, the pNext pointer of the object is set to point to the first member of the list. This is akin to grabbing the hand of the first kid in the chain. The second line points the head pointer to the object, sort of like having the teacher let go of the kid we’re holding onto and grabbing us. That makes us the first kid in the chain.
Performing other operations on a linked list
Adding an object to the head of a list is the simplest operation on a linked list. Moving through the elements in a list gives you a better idea about how a linked list works:
// navigate through a linked list LinkableClass* pL = pHead; while(pL)
{
//perform some operation here
//get the next entry
pL = pL->pNext;
}
The program initializes the pL pointer to the first object of a list of LinkableClass objects through the pointer pHead. (Grab the first kid’s hand.)
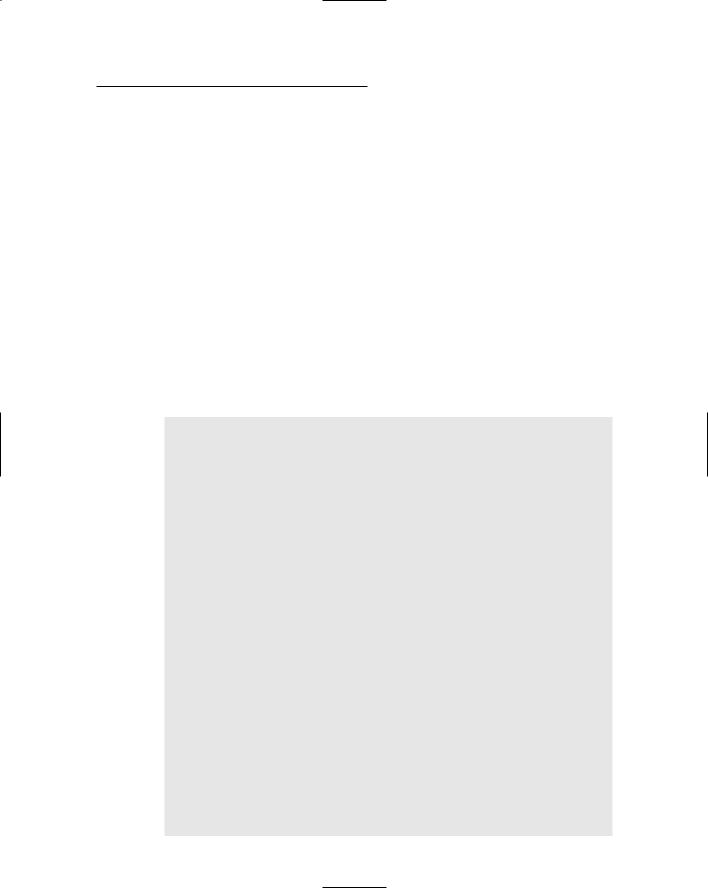
Chapter 14: Point and Stare at Objects 197
The program then enters the while loop. If the pL pointer is not null, it points to some LinkableClass object. Control enters the loop, where the program can then perform whatever operations it wants on the object pointed at by pL.
The assignment pL = pL->pNext “moves” the pL pointer over to the next kid in the list of objects. The program checks to see if pL is null, meaning that we’ve exhausted the list . . . I mean run out of kids, not exhausted all the kids in the list.
Hooking up with a LinkedListData program
The LinkedListData program shown here implements a linked list of objects containing a person’s name. The program could easily contain whatever other data you might like, such as social security number, grade point aver age, height, weight, and bank account balance. I’ve limited the information to just a name to keep the program as simple as possible.
//LinkedListData - store data in a linked list of objects #include <cstdio>
#include <cstdlib> #include <iostream> #include <string.h> using namespace std;
//NameDataSet - stores a person’s name (these objects
// |
could easily store any other information |
// |
desired). |
class NameDataSet |
|
{
public:
char szName[128];
// the link to the next entry in the list NameDataSet* pNext;
};
// the pointer to the first entry in the list NameDataSet* pHead = 0;
// add - add a new member to the linked list void add(NameDataSet* pNDS)
{
// point the current entry to the beginning of // the list...
pNDS->pNext = pHead;
// point the head pointer to the current entry pHead = pNDS;
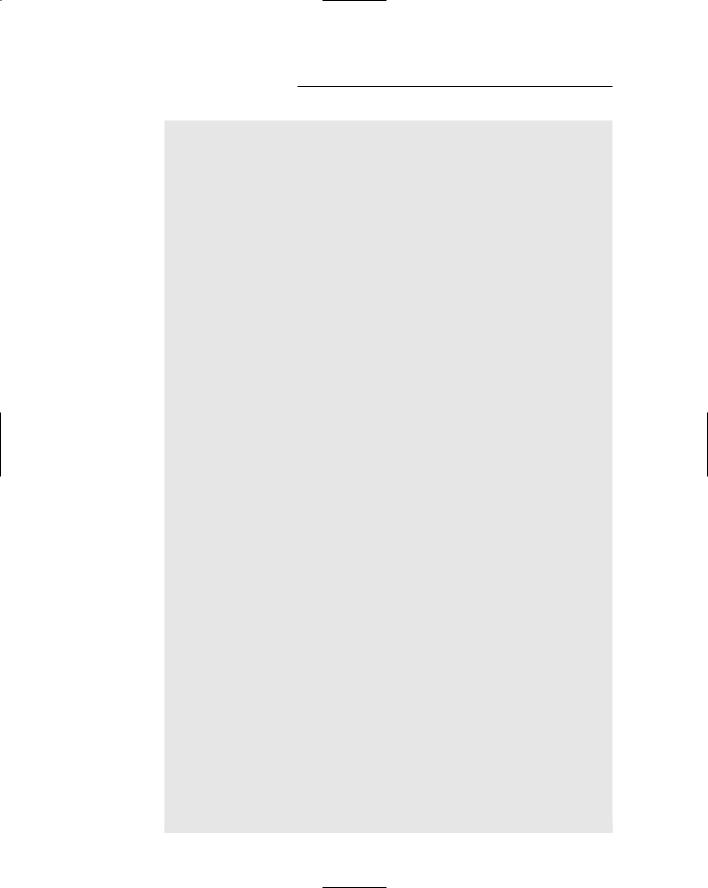
198 Part III: Introduction to Classes
}
//getData - read a name and social security
//number; return null if no more to
//read
NameDataSet* getData()
{
//read the first name char nameBuffer[128]; cout << “\nEnter name:”; cin >> nameBuffer;
//if the name entered is ‘exit’...
if ((stricmp(nameBuffer, “exit”) == 0))
{
// ...return a null to terminate input return 0;
}
//get a new entry to fill NameDataSet* pNDS = new NameDataSet;
//fill in the name and zero the link pointer strncpy(pNDS->szName, nameBuffer, 128); pNDS->szName[127] = ‘\0’; // ensure string is terminated pNDS->pNext = 0;
//return the address of the object created
return pNDS;
}
int main(int nNumberofArgs, char* pszArgs[])
{
cout << “Read names of people\n”
<<“Enter ‘exit’ for first name to exit\n”;
//create (another) NameDataSet object NameDataSet* pNDS;
while (pNDS = getData())
{
//add it onto the end of the list of
//NameDataSet objects
add(pNDS);
}
//to display the objects, iterate through the
//list (stop when the next address is NULL) cout << “Entries:\n”;
pNDS = pHead; while(pNDS)
{
//display current entry
cout << pNDS->szName << “\n”;
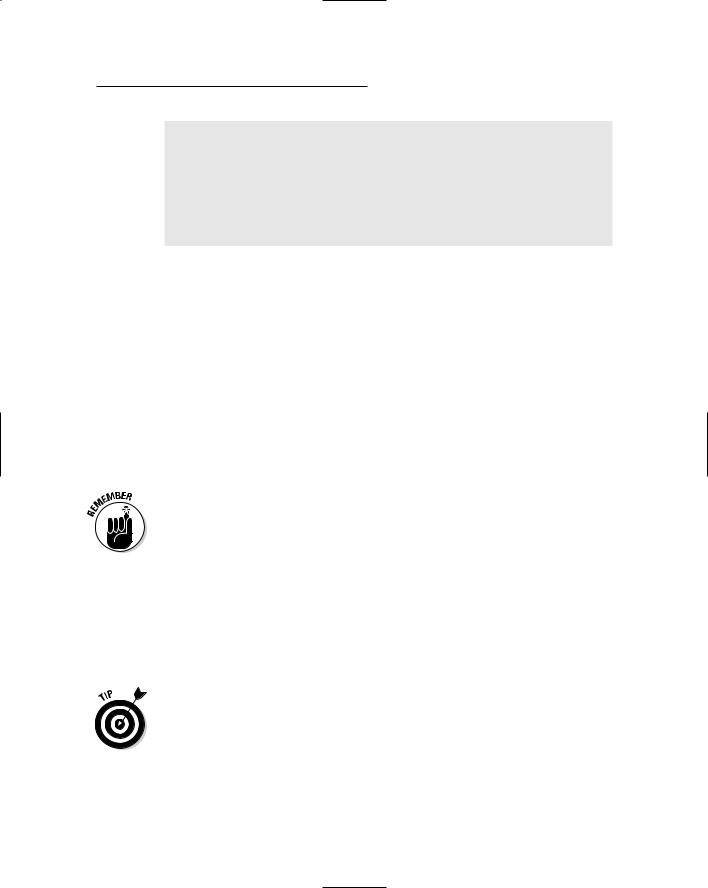
Chapter 14: Point and Stare at Objects 199
// get the next entry pNDS = pNDS->pNext;
}
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
Although somewhat lengthy, the LinkedListData program is simple if you take it in parts. The NameDataSet structure has room for a person’s name and a link to the next NameDataSet object in a linked list. I mentioned earlier that this class would have other members in a real-world application.
The main() function starts looping, calling getData() on each iteration to fetch another NameDataSet entry from the user. The program exits the loop if getData() returns a null, the “non-address,” for an address.
The getData() function prompts the user for a name and reads in whatever the user enters. The program just hopes that the number of characters is less than 128, since it makes no checks. If the string entered is equal to exit, the function returns a null to the caller, thereby exiting the while loop. The stricmp() compares two strings without regard to case. If the string entered is not exit, the program creates a new NameDataSet object, populates the name, and zeroes out the pNext pointer.
Never leave link pointers uninitialized. Use the old programmer’s wives’ tale: “When in doubt, zero it out.” (I mean “old tale,” not “tale of an old wife.”)
Finally, getData() returns the object’s address to main().
main() adds each object returned from getData() to the beginning of the linked list pointed at by the global variable pHead. Control exits the initial while loop when the getData() returns a null. main() then enters a second section that iterates through the completed list, displaying each object. The second while loop terminates when it reaches the last object, the object with a pNext pointer whose value is null.
The program outputs the names entered in the opposite order. This is because each new object is added to the beginning of the list. Alternatively, the program could have added each object to the end of the list — doing so just takes a little more code.