
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index

Chapter 15
Protecting Members:
Do Not Disturb
In This Chapter
Declaring members protected
Accessing protected members from within the class
Accessing protected members from outside the class
Chapter 12 introduces the concept of the class. That chapter describes the public keyword as though it were part of the class declaration — just
something you do. In this chapter, you find out about an alternative to public.
Protecting Members
The members of a class can be marked protected, which makes them inac cessible outside the class. The alternative is to make the members public. Public members are accessible to all.
Please understand the term “inaccessible” in a weak sense. Any programmer can go into the source code, remove the protected keyword and do what ever she wants. Further, any hacker worth his salt can code into a protected section of code. The protected keyword is designed to protect a program mer from herself by preventing inadvertent access.
Why you need protected members
To understand the role of protected, think about the goals of object-oriented programming:
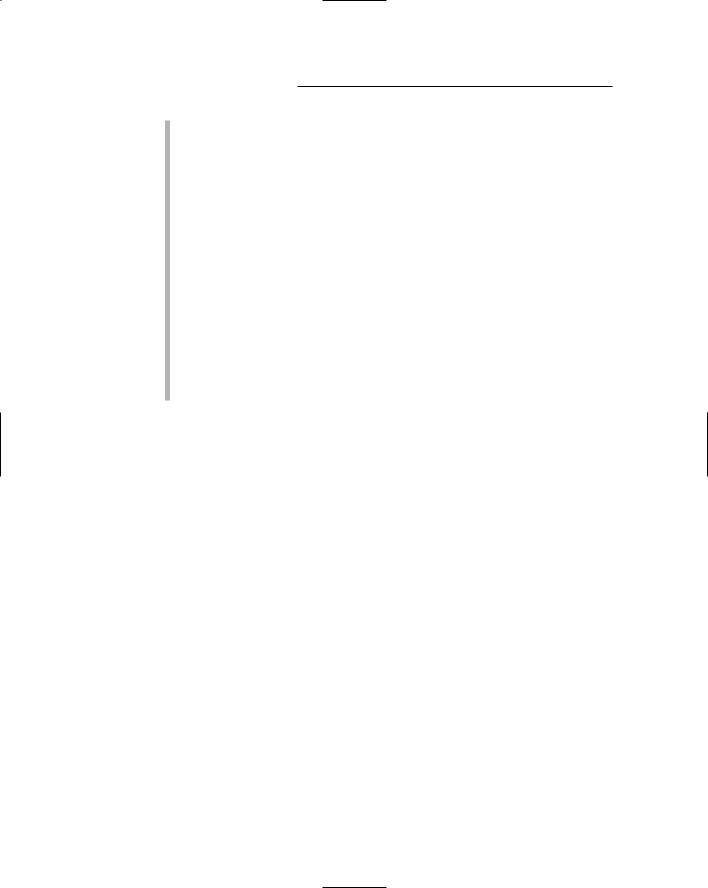
202 Part III: Introduction to Classes
To protect the internals of the class from outside functions. Suppose, for example, that you have a plan to build a software microwave (or what ever), provide it with a simple interface to the outside world, and then put a box around it to keep others from messing with the insides. The protected keyword is that box.
To make the class responsible for maintaining its internal state. It’s not fair to ask the class to be responsible if others can reach in and manipu late its internals (any more than it’s fair to ask a microwave designer to be responsible for the consequences of my mucking with a microwave’s internal wiring).
To limit the interface of the class to the outside world. It’s easier to figure out and use a class that has a limited interface (the public mem bers). Protected members are hidden from the user and need not be learned. The interface becomes the class; this is called abstraction (see Chapter 11 for more on abstraction).
To reduce the level of interconnection between the class and other code. By limiting interconnection, you can more easily replace one class with another or use the class in other programs.
Now, I know what you functional types out there are saying: “You don’t need some fancy feature to do all that. Just make a rule that says certain members are publicly accessible, and others are not.”
Although that is true in theory, it doesn’t work. People start out with all kinds of good intentions, but as long as the language doesn’t at least discourage direct access of protected members, these good intentions get crushed under the pressure to get the product out the door.
Discovering how protected members work
Adding the keyword public to a class makes subsequent members public, which means that they are accessible by non-member functions. Adding the keyword protected makes subsequent members of the class protected, which means they are not accessible by non-members of the class. You can switch between public and protected as often as you like.
Suppose you have a class named Student. In this example, the following capabilities are all that a fully functional, upstanding Student needs (notice the absence of spendMoney() and drinkBeer() — this is a highly stylized student):
addCourse(inthours, float grade) — adds a course
grade() — returns the current grade point average
hours() — returns the number of hours earned toward graduation
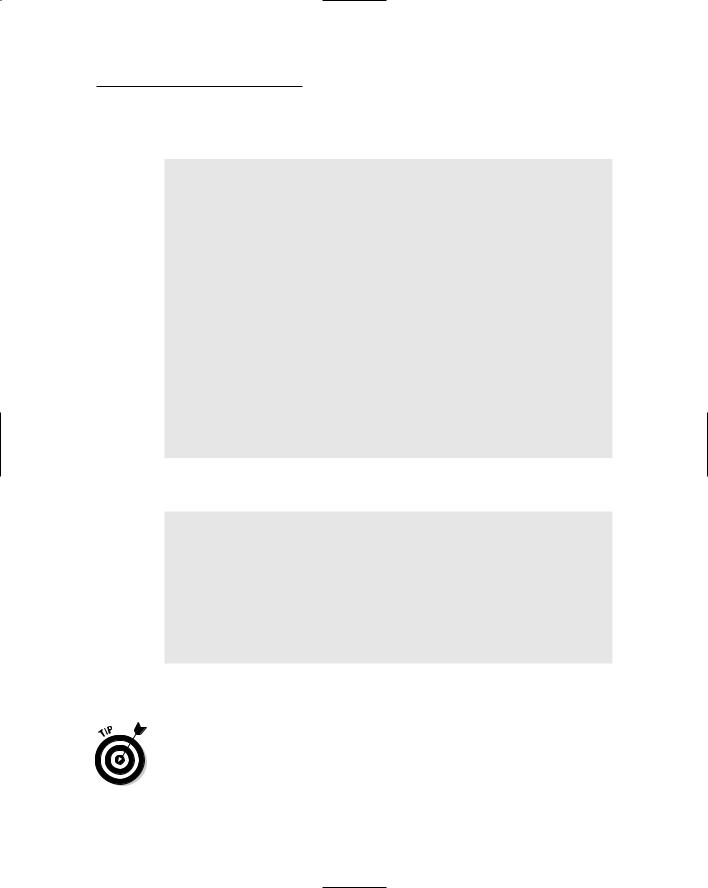
Chapter 15: Protecting Members: Do Not Disturb 203
The remaining members of Student can be declared protected to keep other functions’ prying expressions out of Student’s business.
class Student
{
public:
//grade - return the current grade point average float grade()
{
return gpa;
}
//hours - return the number of semester hours int hours()
{
return semesterHours;
}
//addCourse - add another course to the student’s record float addCourse(int hours, float grade);
//the following members are off-limits to others protected:
int semesterHours; // hours earned toward graduation
float gpa; |
// grade point average |
};
Now the members semester hours and gpa are accessible only to other members of Student. Thus, the following doesn’t work:
Student s;
int main(int argcs, char* pArgs[])
{
//raise my grade (don’t make it too high; otherwise, no
//one would believe it)
s.gpa = 3.5; |
// |
<- generates compiler error |
float gpa = s.grade(); // |
<- this public function reads |
|
|
// |
a copy of the value, but you |
return 0; |
// can’t change it from here |
|
|
|
}
The application’s attempt to change the value of gpa is flagged with a com piler error.
It’s considered good form not to rely on the default and specify either public or private at the beginning of the class. Most of the time, people start with the public members because they make up the interface of the class. Protected members are saved until later.
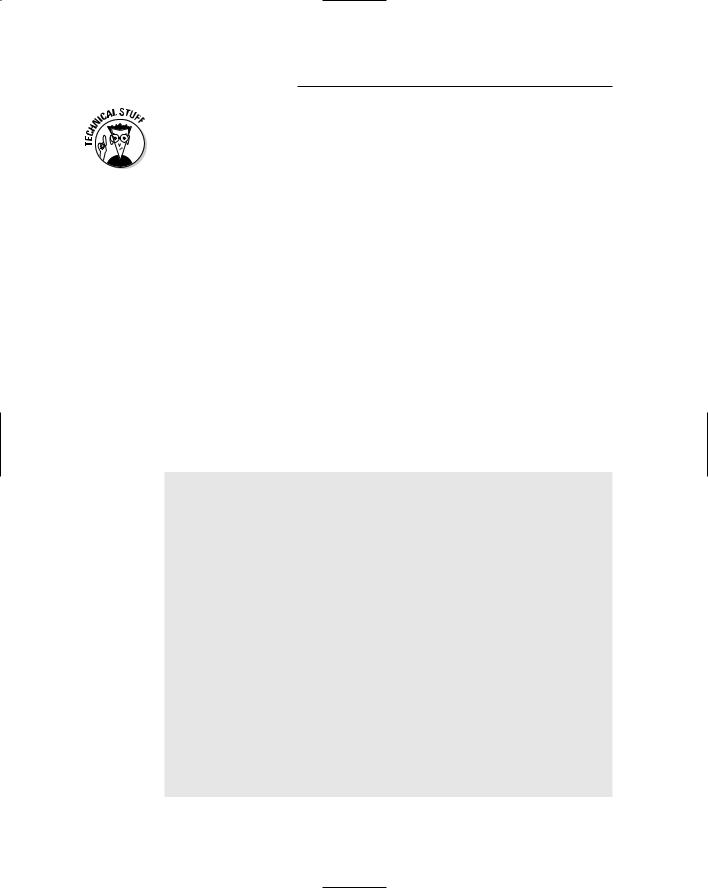
204 Part III: Introduction to Classes
A class member can also be protected by declaring it private. In this book, I use the protected keyword exclusively since it expresses the more generic concept.
Making an Argument for Using
Protected Members
Now that you know a little more about how to use protected members in an actual class, I can replay the arguments for using protected members.
Protecting the internal state of the class
Making the gpa member protected precludes the application from setting the grade point average to some arbitrary value. The application can add courses, but it can’t change the grade point average.
If the application has a legitimate need to set the grade point average directly, the class can provide a member function for that purpose, as follows:
class Student
{
public:
//same as before float grade()
{
return gpa;
}
//here we allow the grade to be changed float grade(float newGPA)
{
float oldGPA = gpa;
//only if the new value is valid if (newGPA > 0 && newGPA <= 4.0)
{
gpa = newGPA;
}
return oldGPA;
}
// ...other stuff is the same including the data members: protected:
int semesterHours; // hours earned toward graduation float gpa;
};