
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
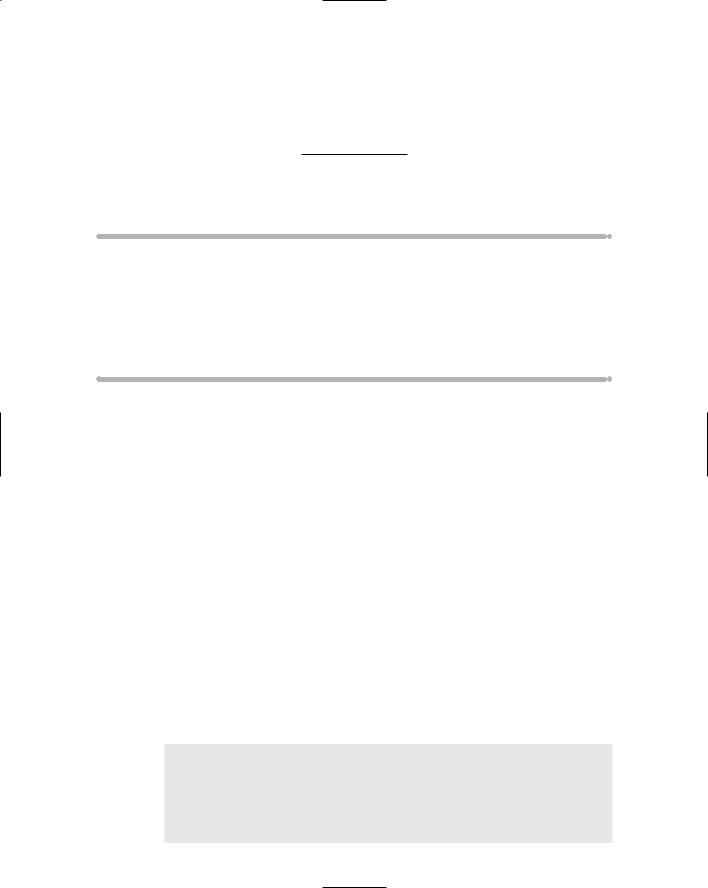
Chapter 17
Making Constructive Arguments
In This Chapter
Making argumentative constructors
Overloading the constructor
Creating objects by using constructors
Invoking member constructors
Constructing the order of construction and destruction
Aclass represents a type of object in the real world. For example, in ear lier chapters, I use the class Student to represent the properties of a
student. Just like students, classes are autonomous. Unlike a student, a class is responsible for its own care and feeding — a class must keep itself in a valid state at all times.
The default constructor presented in Chapter 16 isn’t always enough. For example, a default constructor can initialize the student ID to zero so that it doesn’t contain a random value; however, a Student ID of 0 is probably not valid. It’s up to the class to make sure that the ID is initialized to a legal value when the object is created.
C++ programmers require a constructor that accepts some type of argument in order to initialize an object to other than its default value. This chapter examines constructors with arguments.
Outfitting Constructors with Arguments
C++ enables programmers to define a constructor with arguments, as shown here:
class Student
{
public:
Student(char *pName);
// ...class continues...
};

222 Part III: Introduction to Classes
Justifying constructors
Something as straightforward as adding arguments to the constructor shouldn’t require much justification, but let me take a shot at it anyway. First, allowing arguments to constructors is convenient. It’s a bit silly to make pro grammers construct a default object and then immediately call an initializa tion function to store data in it. A constructor with arguments is like one-stop shopping — sort of a full-service constructor.
Another more important reason to provide arguments to constructors is that it may not be possible to construct a reasonable default object. Remember that a constructor’s job is to construct a legal object (legal as defined by the class). If some default object is not legal, the constructor isn’t doing its job.
For example, a bank account without an account number is probably not legal. (C++ doesn’t care one way or the other, but the bank might get snippy.) You could construct a numberless BankAccount object and then require that the application use some other member function to initialize the account number before it’s used. This “create now/initialize later” approach breaks the rules, however, because it forces the class to rely on the application for initialization.
Using a constructor
Conceptually, the idea of adding an argument is simple. A constructor is a member function, and member functions can have arguments. Therefore, constructors can have arguments.
Remember, though, that you don’t call the constructor like a normal function. Therefore, the only time to pass arguments to the constructor is when the object is created. For example, the following program creates an object s of the class Student by calling the Student(char*) constructor. The object s is destructed when the function main() returns.
//
// ConstructorWArg - provide a constructor with arguments
//
#include <cstdio> #include <cstdlib> #include <iostream> using namespace std;
const int MAXNAMESIZE = 40; class Student
{
public:
Student(char* pName)
{
strncpy(name, pName, MAXNAMESIZE); name[MAXNAMESIZE - 1] = ‘\0’;
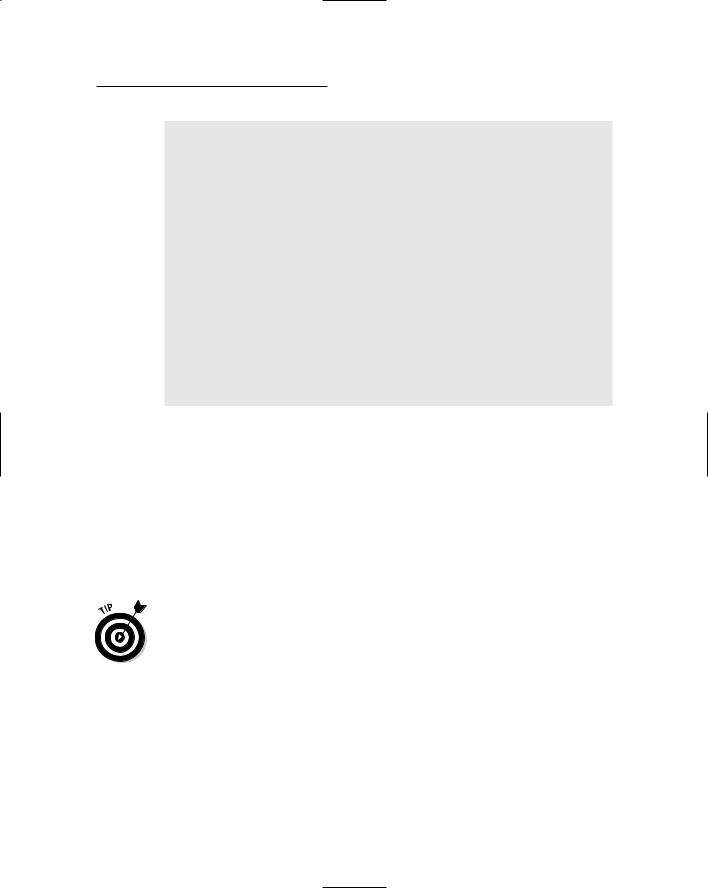
Chapter 17: Making Constructive Arguments 223
semesterHours = 0; gpa = 0.0;
}
// ...other public members...
protected:
char name[MAXNAMESIZE]; int semesterHours; float gpa;
};
int main(int argcs, char* pArgs[])
{
Student s(“O. Danny Boy”);
Student* pS = new Student(“E. Z. Rider”);
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
The constructor looks like the constructors shown in Chapter 16 except for the addition of the char* argument pName. The constructor initializes the data members to their empty start-up values, except for the data member name, which gets its initial value from pName.
The object s is created in main(). The argument to be passed to the con structor appears in the declaration of s, right next to the name of the object. Thus, the student s is given the name Danny in this declaration. The closed brace invokes the destructor on poor little Danny.
The arguments to the constructor appear next to the name of the class when the object is allocated off the heap.
Many of the constructors in this chapter violate the “functions with more than three lines shouldn’t be inlined” rule. I decided to make them inline anyway because I think they’re easier for you to read that way. Aren’t I a nice guy?
Placing Too Many Demands on the Carpenter: Overloading the Constructor
I can draw one more parallel between constructors and other, more normal member functions in this chapter: Constructors can be overloaded.
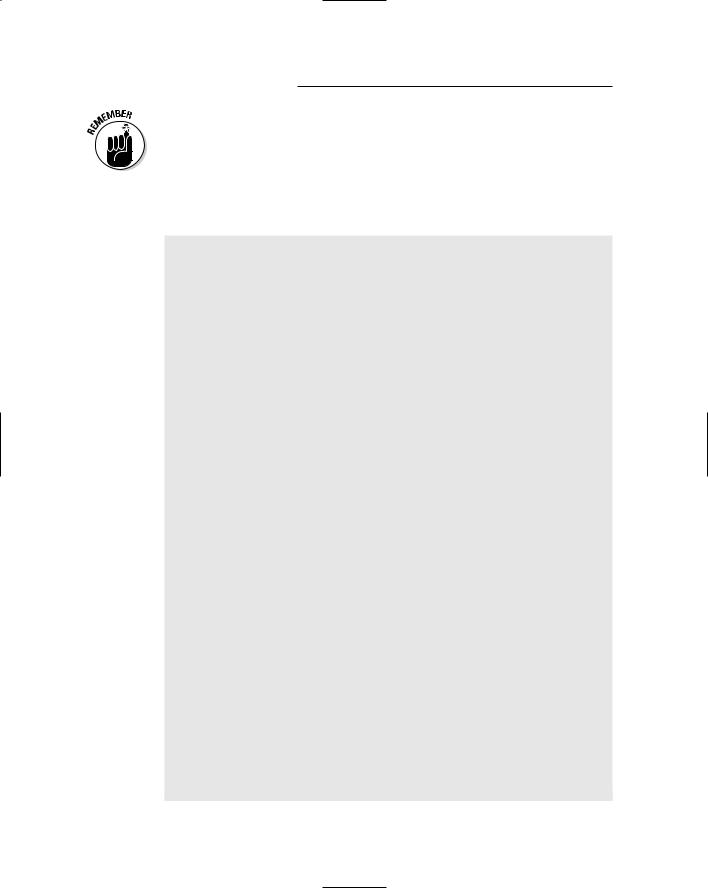
224 Part III: Introduction to Classes
Overloading a function means to define two functions with the same short name but with different types of arguments. See Chapter 6 for the latest news on function overloading.
C++ chooses the proper constructor based on the arguments in the declara tion of the object. For example, the class Student can have all three con structors shown in the following snippet at the same time:
//
//OverloadConstructor - provide the class multiple
// |
ways to create objects by |
// |
overloading the constructor |
// |
|
#include <cstdio> |
|
#include <cstdlib> |
|
#include <iostream> |
|
#include <strings.h> |
|
using namespace std;
const int MAXNAMESIZE = 40; class Student
{
public:
Student()
{
cout << “constructing student no name” << endl; semesterHours = 0;
gpa = 0.0; name[0] = ‘\0’;
}
Student(char *pName)
{
cout << “constructing student “ << pName << endl; strncpy(name, pName, MAXNAMESIZE); name[MAXNAMESIZE - 1] = ‘\0’;
semesterHours = 0; gpa = 0;
}
Student(char *pName, int xfrHours, float xfrGPA)
{
cout << “constructing student “ << pName << endl; strncpy(name, pName, MAXNAMESIZE); name[MAXNAMESIZE - 1] = ‘\0’;
semesterHours = xfrHours; gpa = xfrGPA;
}
~Student()
{
cout << “destructing student” << endl;
}
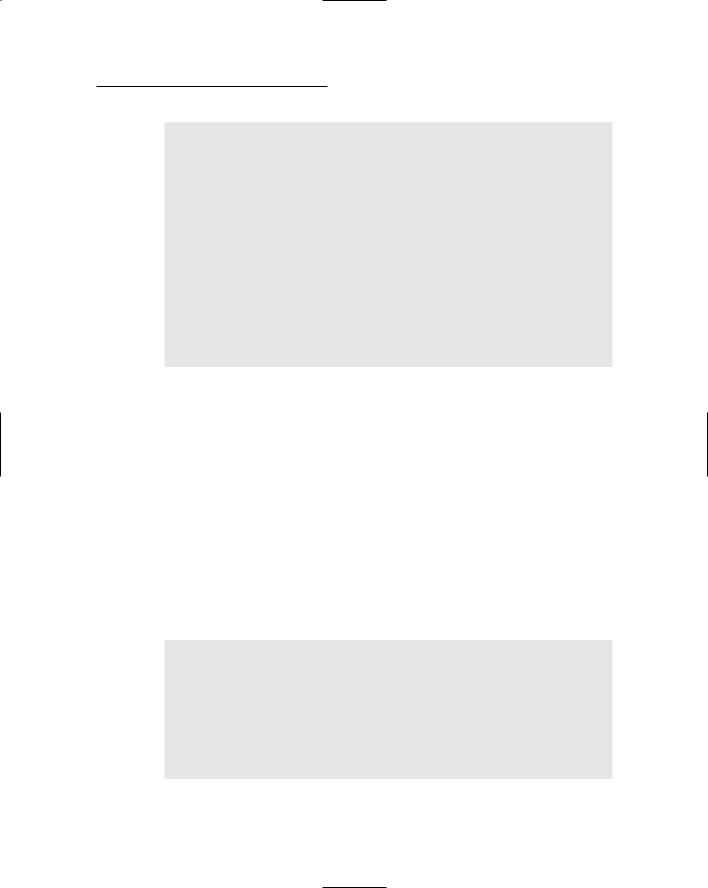
Chapter 17: Making Constructive Arguments 225
protected:
char name[40];
int semesterHours; float gpa;
};
int main(int argcs, char* pArgs[])
{
//the following invokes three different constructors Student noName;
Student freshman(“Marian Haste”);
Student xferStudent(“Pikumup Andropov”, 80, 2.5);
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
Because the object noName appears with no arguments, it’s constructed using the constructor Student::Student(). This constructor is called the default, or void, constructor. (I prefer the latter name, but the former is the more common one, so I use it in this book — I’m a slave to fashion.) The freshMan is constructed using the constructor that has only a char* argument, and the xferStudent uses the constructor with three arguments.
Notice how similar all three constructors are. The number of semester hours and the GPA default to zero if only the name is provided. Otherwise, there is no difference between the two constructors. You wouldn’t need both con structors if you could just specify a default value for the two arguments.
C++ enables you to specify a default value for a function argument in the dec laration to be used in the event that the argument is not present. By adding defaults to the last constructor, all three constructors can be combined into one. For example, the following class combines all three constructors into a single, clever constructor:
//
//ConstructorWDefaults - multiple constructors can often
// |
be combined with the definition |
// |
of default arguments |
// |
|
#include <cstdio> |
|
#include <cstdlib> |
|
#include <iostream> |
|
#include <strings.h> |
|
using namespace std; |
|

226 Part III: Introduction to Classes
const int MAXNAMESIZE = 40; class Student
{
public:
Student(char *pName = “no name”, int xfrHours = 0,
float xfrGPA = 0.0)
{
cout << “constructing student “ << pName << endl; strncpy(name, pName, MAXNAMESIZE); name[MAXNAMESIZE - 1] = ‘\0’;
semesterHours = xfrHours; gpa = xfrGPA;
}
~Student()
{
cout << “destructing student “ << endl;
}
// ...other public members...
protected:
char name[MAXNAMESIZE]; int semesterHours; float gpa;
};
int main(int argcs, char* pArgs[])
{
//the following invokes three different constructors Student noName;
Student freshman(“Marian Haste”);
Student xferStudent(“Pikumup Andropov”, 80, 2.5);
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
Now all three objects are constructed using the same constructor; defaults are provided for nonexistent arguments in noName and freshMan.
In earlier versions of C++, you couldn’t create a default constructor by pro viding defaults for all the arguments. The default constructor had to be a separate explicit constructor. Although this restriction was lifted in the stan dard (it seems to have had no good basis), some older compilers may still impose it.