
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index

56 |
Part I: Introduction to C++ Programming |
Roman numeral expressions
On a historical note, I should mention that some numbering systems actually hinder computa tions. The Roman numeral system is a (so to speak) classic example that greatly hindered the development of math.
Adding two Roman numerals isn’t too difficult:
XIX + XXVI = XLV
Think this one out:
a) IX + VI: The I after the V cancels out the I before the X so the result is V carry the X.
b) X + XX: Plus the carry X is XXXX, which is expressed as XL.
Subtraction is only slightly more difficult.
Ah, but multiplying two Roman numerals all but requires a bachelor’s degree in mathematics. (You end up with rules like X promotes the digits on the right by 1 letter so that X –* IV becomes XL.) Division practically required a Ph.D., and higher operations such as integration would have been completely impossible.
Love those Arabic numerals . . .
Performing Bitwise Logical Operations
All C++ numbers can be expressed in binary form. Binary numbers use only the digits 1 and 0 to represent a value. The following Table 4-2 defines the set of operations that work on numbers one bit at a time, hence the term bitwise operators.
Table 4-2 |
Bitwise Operators |
Operator |
Function |
~ |
NOT: Toggle each bit from 1 to 0 and from 0 to 1 |
|
|
& |
AND each bit of the left-hand argument with that on the right |
|
|
| |
OR each bit of the left-hand argument with that on the right |
|
|
^ |
XOR (exclusive OR) each bit of the left-hand argument with that on |
|
the right |
Bitwise operations can potentially store a lot of information in a small amount of memory. There are a lot of traits in the world that have only two (or, at most, four) possibilities — that are either this way or that way. You are either married or you’re not (you might be divorced but you are still not currently
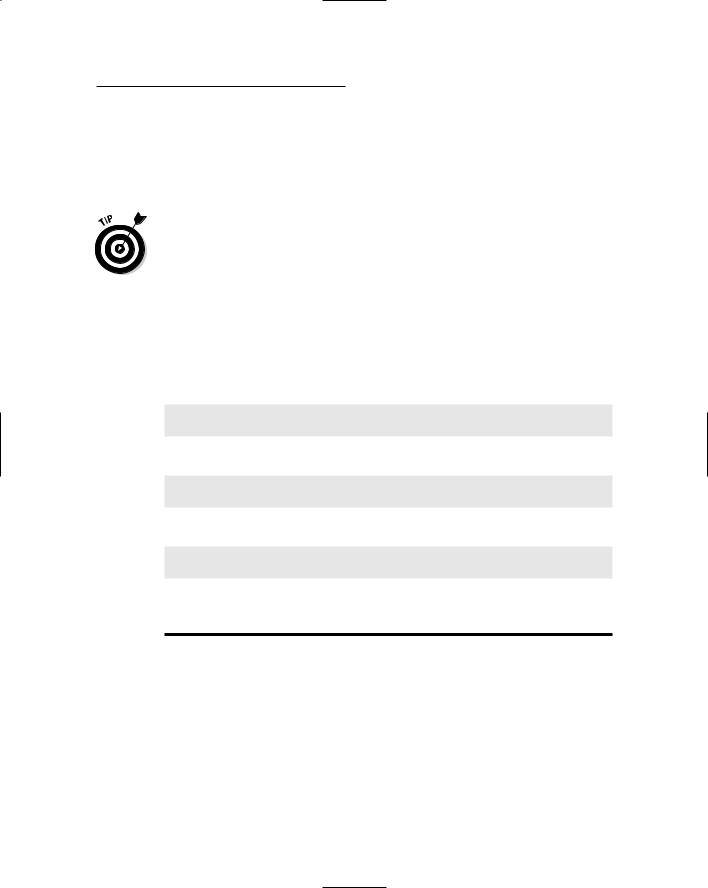
Chapter 4: Performing Logical Operations |
57 |
married). You are either male or female (at least that’s what my driver’s license says). In C++, you can store each of these traits in a single bit — in this way, you can pack 32 separate properties into a single 32-bit int.
In addition, bit operations can be extremely fast. There is no performance penalty paid for that 32-to-1 saving.
Even though memory is cheap these days, it’s not unlimited. Sometimes, when you’re storing large amounts of data, this ability to pack a whole lot of properties into a single word is a big advantage.
The single bit operators
The bitwise operators — AND (&), OR (|) and NOT (~) — perform logic oper ations on single bits. If you consider 0 to be false and 1 to be true (it doesn’t have to be this way, but it’s a common convention), you can say things like the following for the NOT operator:
NOT 1 (true) is 0 (false)
NOT 0 (false) is 1 (true)
The AND operator is defined as following:
1 (true) AND 1 (true) is 1 (true)
1 (true) AND 0 (false) is 0 (false)
It’s a similar situation for the OR operator:
1 (true) OR 0 (false) is 1 (true)
0 (false) OR 0 (false) is 0 (false)
The definition of the AND operator appears in Table 4-3.
Table 4-3 |
|
Truth Table for the AND Operator |
AND |
1 |
0 |
1 |
1 |
0 |
|
|
|
0 |
0 |
0 |
|
|
|
You read this table as the column corresponding to the value of one of the arguments while the row corresponds to the other. Thus, 1 & 0 is 0. (Column 1
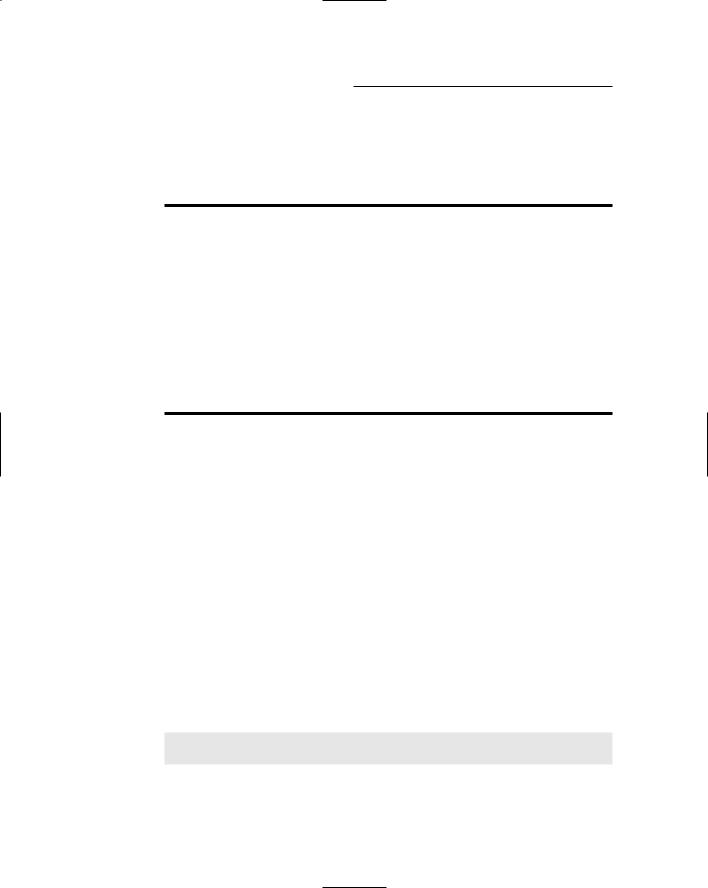
58 |
Part I: Introduction to C++ Programming |
and row 0.) The only combination that returns anything other than 0 is 1 & 1. (This is known as a truth table.)
Similarly, the truth table for the OR operator is shown in Table 4-4.
Table 4-4 |
|
Truth Table for the OR Operator |
XOR |
1 |
0 |
1 |
1 |
1 |
|
|
|
0 |
1 |
0 |
|
|
|
One other logical operation that is not so commonly used in day-to-day living is the OR ELSE operator commonly contracted to XOR. XOR is true if either argument is true but not if both are true. The truth table for XOR is shown in Table 4-5.
Table 4-5 |
|
Truth Table for the XOR Operator |
XOR |
1 |
0 |
1 |
0 |
1 |
|
|
|
0 |
1 |
0 |
|
|
|
Armed with these single bit operators, we can take on the C++ bitwise logical operations.
Using the bitwise operators
The bitwise operators operate on each bit separately.
The bitwise operators are used much like any other binary arithmetic operator. The NOT operator is the easiest to understand. To NOT a number is to NOT each bit that makes up that number (and to a programmer, that sentence makes perfect sense — honest). Consider this example:
~01102 (0x6)
10012 (0x9)
Thus we say that ~0x6 equals 0x9.
The following calculation demonstrates the & operator:
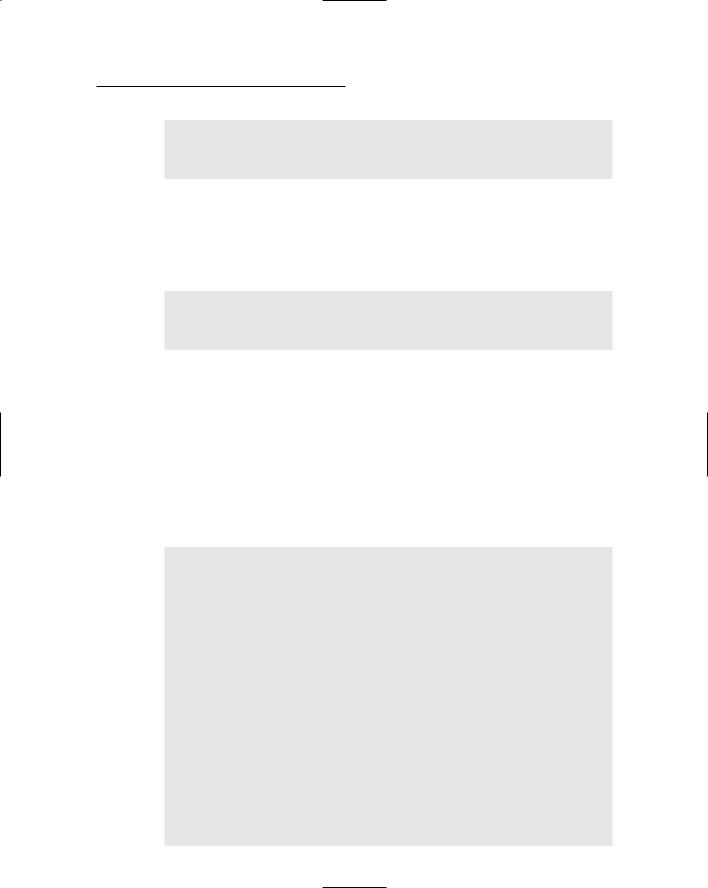
Chapter 4: Performing Logical Operations |
59 |
01102
&
00112
00102
Beginning with the most significant bit, 0 AND 0 is 0. In the next bit, 1 AND 0 is 0. In bit 3, 1 AND 1 is 1. In the least significant bit, 0 AND 1 is 0.
The same calculation can be performed in hexadecimal by first converting the number in binary, performing the operation and then converting the result back.
0x6 |
01102 |
& |
& |
0x3 |
00112 |
0x2 |
00102 |
In shorthand, we say that 0x6 & 0x3 equals 0x2.
(Try this test: What is the value of 0x6 | 0x3? Get this in 7 seconds, and you can give yourself 7 pats on the back.)
A simple test
The following program illustrates the bitwise operators in action. The pro gram initializes two variables and outputs the result of ANDing, ORing, and XORing them.
//BitTest - initialize two variables and output the
//results of applying the ~,& , | and ^
//operations
#include <cstdio> #include <cstdlib> #include <iostream> using namespace std;
int main(int nNumberofArgs, char* pszArgs[])
{
//set output format to hexadecimal cout.setf(cout.hex);
//initialize two arguments
int nArg1; nArg1 = 0x1234;
int nArg2; nArg2 = 0x00ff;
//now perform each operation in turn
//first the unary NOT operator