
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
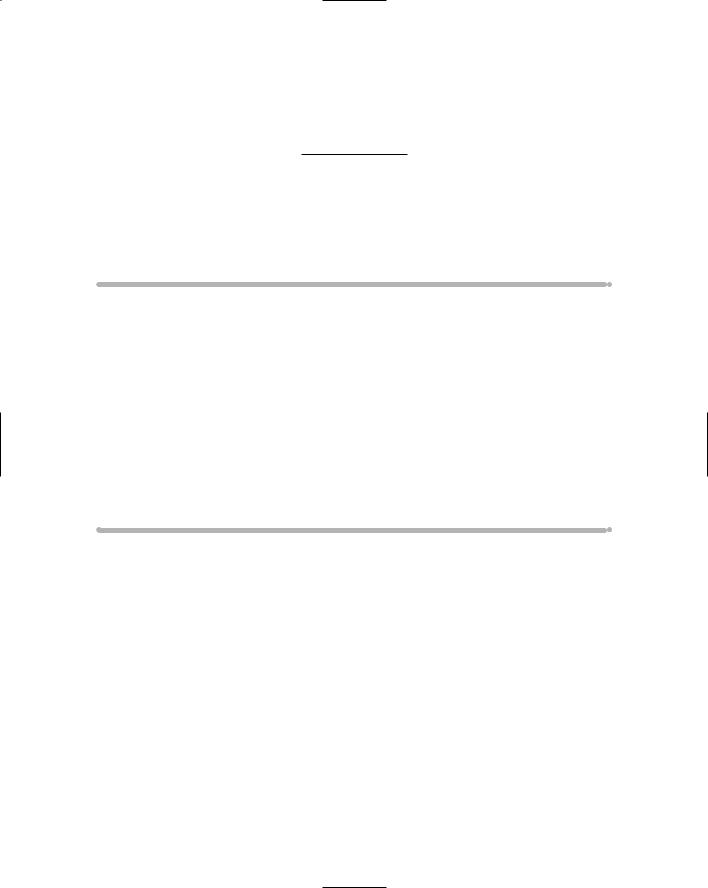
Chapter 29
Ten Ways to Avoid Adding Bugs to Your Program
In This Chapter
Enabling all warnings and error messages
Insisting on clean compiles
Using a clear and consistent coding style
Limiting the visibility
Adding comments to your code while you write it
Single-stepping every path at least once
Avoiding overloaded operators
Heap handling
Using exceptions to handle errors
Avoiding multiple inheritance
In this chapter, I look at several ways to minimize errors, as well as ways to make debugging the errors that are introduced easier.
Enabling All Warnings
and Error Messages
The syntax of C++ allows for a lot of error checking. When the compiler encounters a construct that it cannot decipher, it has no choice but to gener ate an error message. Although the compiler attempts to sync back up with the next statement, it does not attempt to generate an executable program.

378 Part VI: The Part of Tens
Disabling warning and error messages is a bit like unplugging the Check Engine light on your car dashboard because it bothers you: Ignoring the problem doesn’t make it go away. If your compiler has a Syntax Check from Hell mode, enable it. Both Visual Studio.NET and Dev-C++ have an Enable All Messages option — set it. You save time in the end.
During all its digging around in your source code, a good C++ compiler also looks for suspicious-looking syntactical constructs, such as the following code snippet:
#include “student.h” #include “MyClass.h”
Student* addNewStudent(MyClass myObject, char *pName, SSNumber ss)
{
Student* pS;
if (pName != 0)
{
pS = new Student(pName, ss); myObject.addStudent(pS);
}
return pS;
}
Here you see that the function first creates a new Student object that it then adds to the MyClass object provided. (Presumably addStudent() is a member function of MyClass.)
If a name is provided (that is, pName is not 0), a new Student object is created and added to the class. With that done, the function returns the Student cre ated to the caller. The problem is that if pName is 0, pS is never initialized to anything. A good C++ compiler can detect this path and generate a warning that “pS might not be initialized when it’s returned to the caller and maybe you should look into the problem,” or words to that effect.
Insisting on Clean Compiles
Don’t start debugging your code until you remove or at least understand all the warnings generated during compilation. Enabling all the warning mes sages if you then ignore them does you no good. If you don’t understand the warning, look it up. What you don’t know will hurt you.
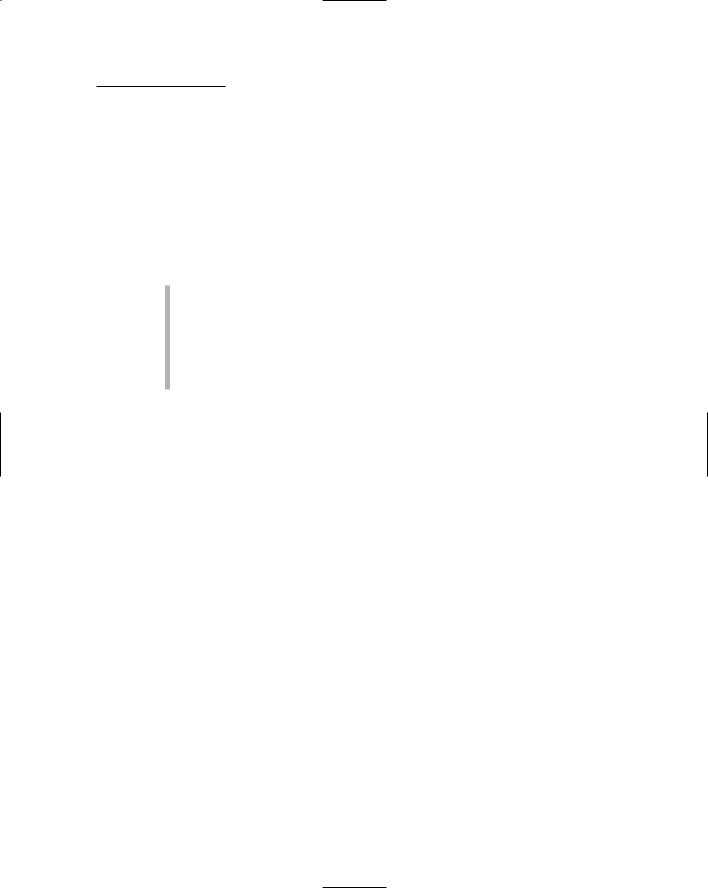
Chapter 29: Ten Ways to Avoid Adding Bugs to Your Program 379
Adopting a Clear and Consistent
Coding Style
Coding in a clear and consistent style not only enhances the readability of the program but also results in fewer coding mistakes. Remember, the less brain power you have to spend deciphering C++ syntax, the more you have left over for thinking about the logic of the program at hand. A good coding style enables you to do the following with ease:
Differentiate class names, object names, and function names
Know something about the object based on its name
Differentiate preprocessor symbols from C++ symbols (that is, #defined objects should stand out)
Identify blocks of C++ code at the same level (this is the result of consis tent indentation)
In addition, you need to establish a standard module header that provides information about the functions or classes in the module, the author (pre sumably, that’s you), the date, the version of the compiler you’re using, and a modification history.
Finally, all programmers involved in a single project should use the same style. Trying to decipher a program with a patchwork of different coding styles is confusing.
Limiting the Visibility
Limiting the visibility of class internals to the outside world is a cornerstone of object-oriented programming. The class is responsible for its own internals; the application is responsible for using the class to solve the problem at hand.
Specifically, limited visibility means that data members should not be acces sible outside the class — that is, they should be marked as protected. (There is another storage class, private, that is not discussed in this book.) In addi tion, member functions that the application software does not need to know about should also be marked protected. Don’t expose any more of the class internals than necessary.
A related rule is that public member functions should trust application code as little as possible. Any argument passed to a public member function should be treated as though it might cause bugs until it has been proven safe. A function such as the following is an accident waiting to happen:
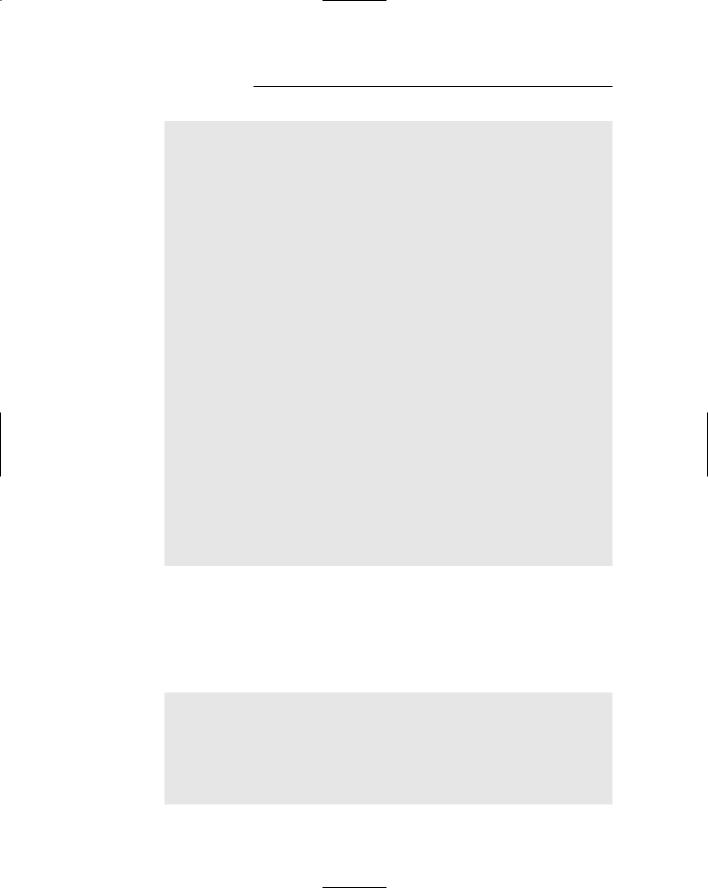
380 Part VI: The Part of Tens
class Array
{
public: Array(int s)
{
size = 0;
pData = new int[s]; if (pData)
{
size = s;
}
}
~Array()
{
delete pData; size = 0; pData = 0;
}
//either return or set the array data int data(int index)
{
return pData[index];
}
int data(int index, int newValue)
{
int oldValue = pData[index]; pData[index] = newValue; return oldValue;
}
protected: int size; int *pData;
};
The function data(int) allows the application software to read data out of Array. This function is too trusting; it assumes that the index provided is within the data range. What if the index is not? The function data(int, int) is even worse because it overwrites an unknown location.
What’s needed is a check to make sure that the index is in range. In the fol lowing, only the data(int) function is shown for brevity:
int data(unsigned int index)
{
if (index >= size)
{
throw Exception(“Array index out of range”);
}
return pData[index];
}
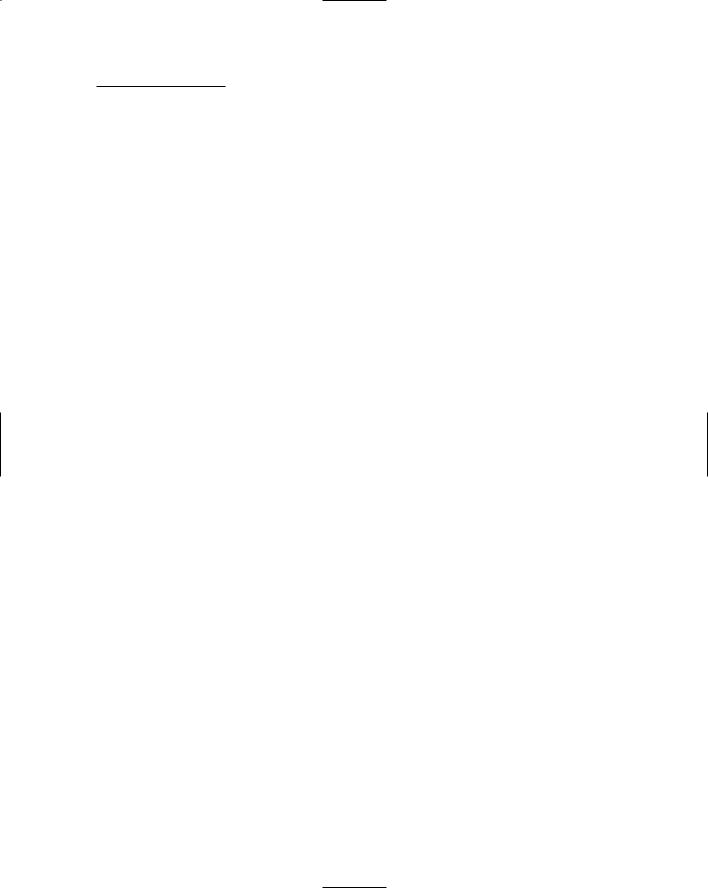
Chapter 29: Ten Ways to Avoid Adding Bugs to Your Program 381
Now an out-of-range index will be caught by the check. (Making index unsigned precludes the necessity of adding a check for negative index values.)
Commenting Your Code
While You Write It
You can avoid errors if you comment your code as you write it rather than waiting until everything works and then go back and add comments. I can understand not taking the time to write voluminous headers and function descriptions until later, but you always have time to add short comments while writing the code.
Short comments should be enlightening. If they’re not, they aren’t worth much. You need all the enlightenment you can get while you’re trying to make your program work. When you look at a piece of code you wrote a few days ago, comments that are short, descriptive, and to the point can make a dra matic contribution to helping you figure out exactly what it was you were trying to do.
In addition, consistent code indentation and naming conventions make the code easier to understand. It’s all very nice when the code is easy to read after you’re finished with it, but it’s just as important that the code be easy to read while you’re writing it. That’s when you need the help.
Single-Stepping Every
Path at Least Once
It may seem like an obvious statement, but I’ll say it anyway: As a program mer, it’s important for you to understand what your program is doing. Nothing gives you a better feel for what’s going on under the hood than single-stepping the program with a good debugger. (The debugger in both Dev-C++ and Visual Studio.NET work just fine.)
Beyond that, as you write a program, you sometimes need raw material in order to figure out some bizarre behavior. Nothing gives you that material better than single-stepping new functions as they come into service.
Finally, when a function is finished and ready to be added to the program, every logical path needs to be traveled at least once. Bugs are much easier to