
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index

Chapter 13: Making Classes Work 171
Naming class members
A member function is a lot like a member of a family. The full name of the function addCourse(int, float) is Student::addCourse(int, float), just as my full name is Stephen Davis. The short name of the function is addCourse(int, float), just as my short name is Stephen. The class name at the beginning of the full name indicates that the function is a member of the class Student. (The :: between the class name and the function name is simply a separator.) The name Davis on the end of my name indicates that I am a member of the Davis family.
Another name for a full name is extended name.
You can define an addCourse(int, float) function that has nothing to do with Student — there are Stephens out there who have nothing to do with my family. (I mean this literally: I know several Stephens who want nothing to do with my family.)
You could have a function Teacher::addCourse(int, float) or even Golf::addCourse(). A function addCourse(int, float) without a class name is just a plain ol’ conventional non-member function.
The extended name for the non-member function is ::addCourse(int, float). (Note the colon without a family name in front.)
Calling a Member Function
Before you look at how to call a member function, remember how to access a data member:
class Student
{
public:
int semesterHours; float gpa;
};
Student s; void fn(void)
{
// access data members of s s.semesterHours = 10;
s.gpa |
= 3.0; |
}
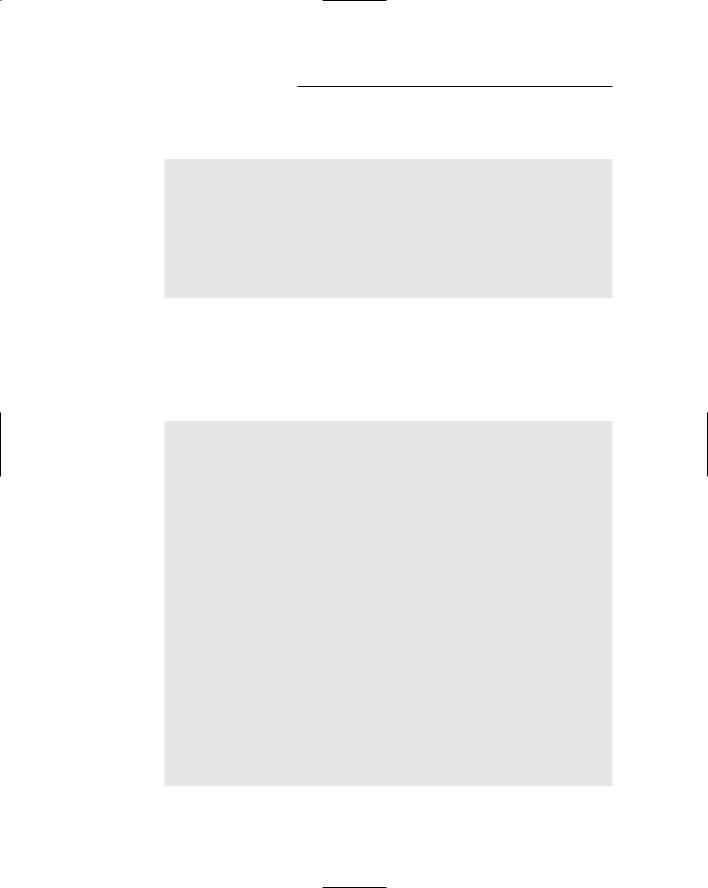
172 Part III: Introduction to Classes
Notice that you have to specify an object along with the member name. In other words, the following makes no sense:
Student s; void fn(void)
{
// neither of these is legal
semesterHours = 10; // member of what object of what
// class?
Student::semesterHours = 10; // okay, I know the class // but I still don’t know // the object
}
Accessing a member function
Remember that member functions function like data members functionally. The following CallMemberFunction shows how to invoke the member func tion addCourse():
//
//CallMemberFunction - define and invoke a function that’s
// |
a member of the class Student |
// |
|
#include <cstdio> |
|
#include <cstdlib> |
|
#include <iostream> |
|
using namespace std; |
|
class Student
{
public:
// add a completed course to the record float addCourse(int hours, float grade)
{
//calculate the sum of all courses times
//the average grade
float weightedGPA;
weightedGPA = semesterHours * gpa;
//now add in the new course semesterHours += hours; weightedGPA += grade * hours;
gpa = weightedGPA / semesterHours;
//return the new gpa
return gpa;
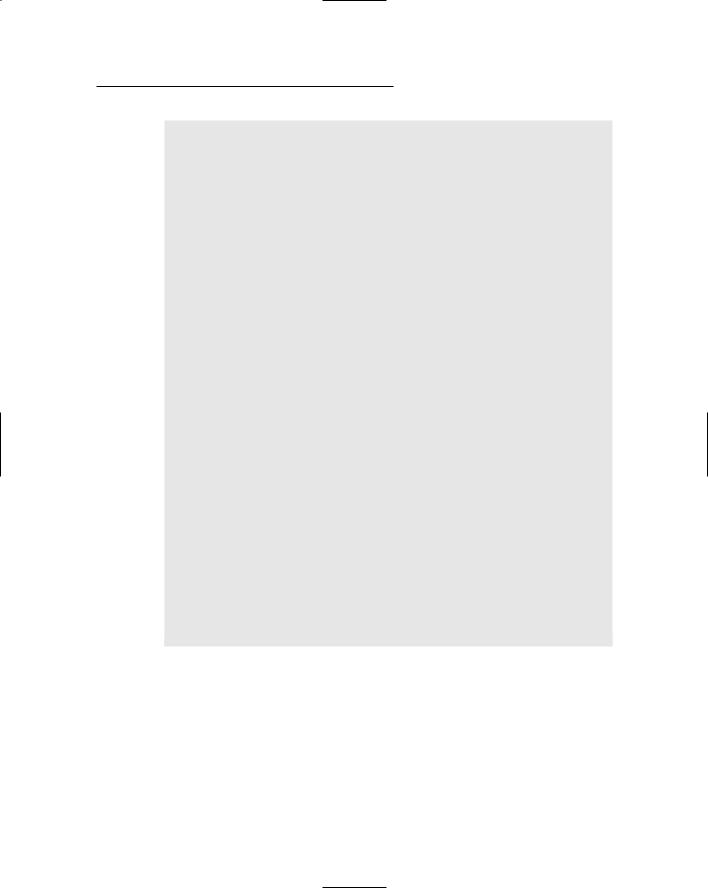
Chapter 13: Making Classes Work 173
}
int semesterHours; float gpa;
};
int main(int nNumberofArgs, char* pszArgs[])
{
Student s; s.semesterHours = 10;
s.gpa |
= 3.0; |
// the values before the call
cout << “Before: s = (“ << s.semesterHours
<<“, “ << s. gpa
<<endl;
//the following subjects the data members of the s
//object to the member function addCourse() s.addCourse(3, 4.0); // call the member function
//the values are now changed
cout << “After: s = (“ << s.semesterHours
<<“, “ << s. gpa
<<“)” << endl;
//access another object just for the heck of it Student t;
t.semesterHours = 6;
t.gpa |
= 1.0; |
// |
not doing so good |
t.addCourse(3, 1.5); |
// |
things aren’t getting any better |
//wait until user is ready before terminating program
//to allow the user to see the program results system(“PAUSE”);
return 0;
}
The syntax for calling a member function looks like a cross between the syntax for accessing a data member and that used for calling a function. The right side of the dot looks like a conventional function call, but an object is on the left of the dot.
We say that “addCourse() operates on the object s” or, said another way, s is the student to which the course is to be added. You can’t fetch the number of semester hours without knowing from which student — you can’t add a stu dent to a course without knowing which student to add. Calling a member func tion without an object makes no more sense than referencing a data member without an object.
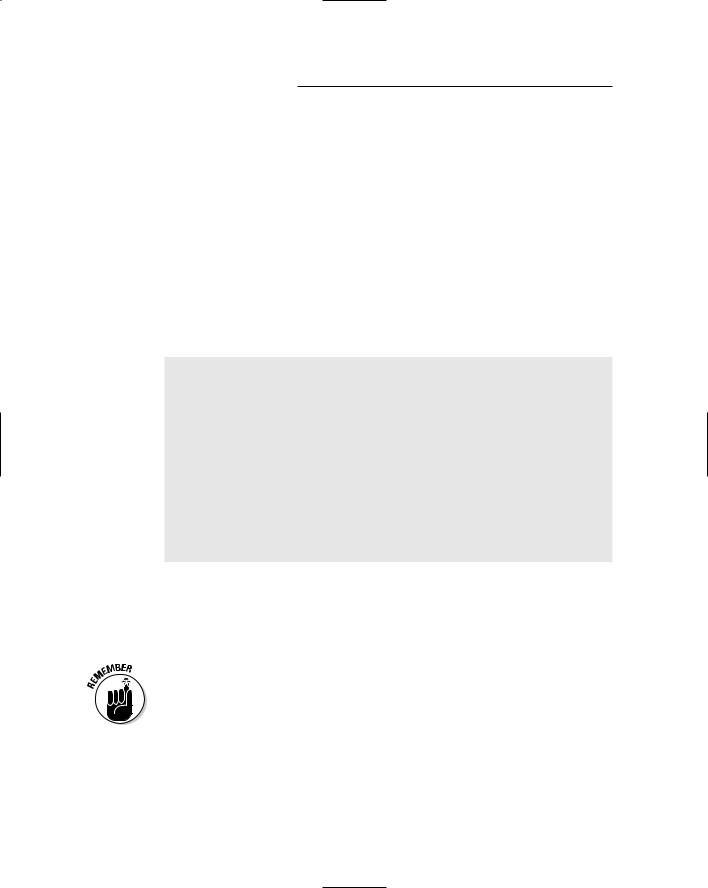
174 Part III: Introduction to Classes
Accessing other members from a member function
I can see it clearly: You repeat to yourself, “Accessing a member without an object makes no sense. Accessing a member without an object. Accessing . . .” Just about the time you’ve accepted this, you look at the member function
Student::addCourse() and Wham! It hits you: addCourse() accesses other class members without reference to an object. Just like the TV show: “How Do They Do That?”
Okay, which is it, can you or can’t you? Believe me, you can’t. When you ref erence a member of Student from addCourse(), that reference is against the Student object with which the call to addCourse() was made. Huh? Go back to the CallMemberFunction example. The critical subsections appear here:
int main(int nNumberofArgs, char* pszArgs[])
{
Student s; s.semesterHours = 10;
s.gpa |
= 3.0; |
s.addCourse(3, 4.0); // call the member function
Student t; t.semesterHours = 6;
t.gpa = 1.0; // not doing so good t.addCourse(3, 1.5); // things aren’t getting any better
system(“PAUSE”); return 0;
}
When addCourse() is invoked with the object s, all of the otherwise unquali fied member references in addCourse() refer to s as well. Thus, the refer ence to semesterHours in addCourse() refers to s.semesterHours, and gpa refers to s.gpa. But when addCourse() is invoked with the Student t object, these same references are to t.semesterHours and t.gpa instead.
The object with which the member function was invoked is the “current” object, and all unqualified references to class members refer to this object. Put another way, unqualified references to class members made from a member function are always against the current object.
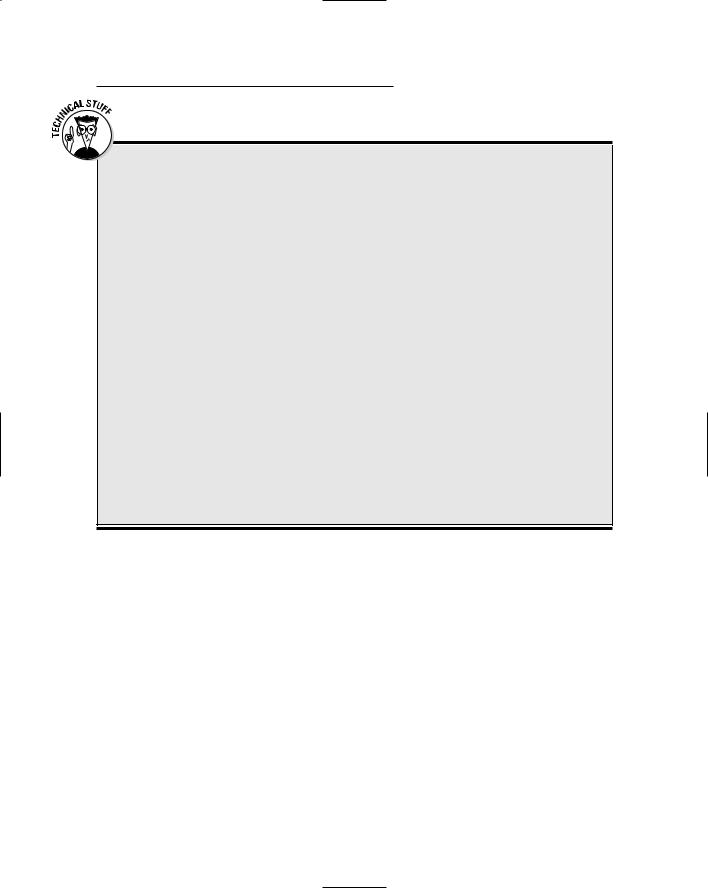
Chapter 13: Making Classes Work 175
Naming the current object
How does the member function know what the current object is? It’s not magic — the address of the object is passed to the member function as an implicit and hidden first argument. In other words, the following conversion is taking place:
s.addCourse(3, 2.5) is like
Student::addCourse(&s, 3, 2.5)
(Note that you can’t actually use the syntax on the right; this is just the way C++ sees it.)
Inside the function, this implicit pointer to the current object has a name, in case you need to refer to it. It is called this, as in “Which object? This object.” Get it? The type of this is always a pointer to an object of the appropriate class.
Anytime a member function refers to another member of the same class without providing an object explicitly, C++ assumes “this.” You also can refer to this explicitly, if you like. You could
have written Student::addCourse() as follows:
float Student::addCourse(int hours, float grade)
{
float weightedGPA; weightedGPA = this- >semesterHours * this->gpa;
// now add in the new course this->semesterHours += hours;
weightedGPA += hours * grade;
this->gpa = weightedGPA / this->semesterHours; return this->gpa;
}
The effect is the same whether you explicitly include “this,” as in the preceding example, or leave it implicit, as you did before.
Scope Resolution (And I Don’t Mean How Well Your Microscope Works)
The :: between a member and its class name is called the scope resolution operator because it indicates the scope to which class a member belongs. The class name before the colon is like the family last name, while the func tion name after the colons is like the first name — the order is similar to an oriental name, family name first.
You use the :: operator to describe a non-member function by using a null class name. The non-member function addCourse, for example, can be referred to as ::addCourse(int, float), if you prefer. This is like a func tion without a home.
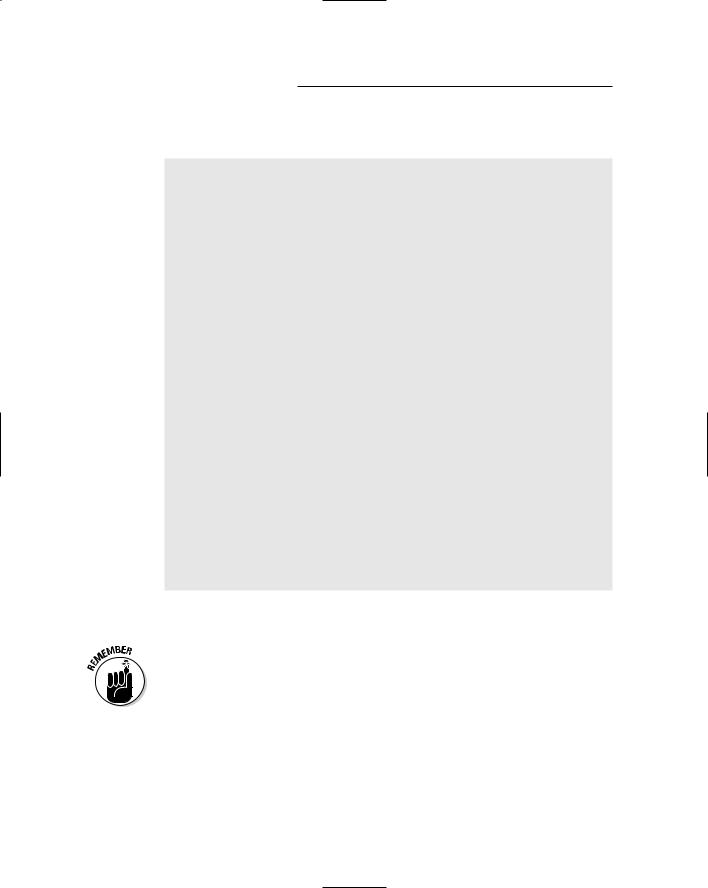
176 Part III: Introduction to Classes
Normally the :: operator is optional, but there are a few occasions when this is not so, as illustrated here:
//addCourse - combine the hours and grade into
//a weighted grade
float addCourse(int hours, float grade)
{
return hours * grade;
}
class Student
{
public:
int semesterHours; float gpa;
// add a completed course to the record float addCourse(int hours, float grade)
{
//call some external function to calculate the
//weighted grade
float weightedGPA = addCourse(semesterHours, gpa);
//now add in the new course semesterHours += hours;
//use the same function to calculate the weighted
//grade of this new course
weightedGPA += addCourse(hours, grade); gpa = weightedGPA / semesterHours;
// return the new gpa return gpa;
}
};
Here, I want the member function Student::addCourse() to call the non member function ::addCourse(). Without the :: operator, however, a call to addCourse() from Student refers to Student::addCourse().
One member of the family can use the short name when referring to another member of the same family. The family . . . I mean class name . . . is understood.
Not indicating the class name in this case results in the function calling itself, which is generally not a good thing. Adding the :: operator to the front directs the call to the global version, as desired: