
- •Table of Contents
- •Introduction
- •What Is C++?
- •Conventions Used in This Book
- •How This Book Is Organized
- •Part I: Introduction to C++ Programming
- •Part III: Introduction to Classes
- •Part IV: Inheritance
- •Part V: Optional Features
- •Part VI: The Part of Tens
- •Icons Used in This Book
- •Where to Go from Here
- •Grasping C++ Concepts
- •How do I program?
- •Installing Dev-C++
- •Setting the options
- •Creating Your First C++ Program
- •Entering the C++ code
- •Building your program
- •Executing Your Program
- •Dev-C++ is not Windows
- •Dev-C++ help
- •Reviewing the Annotated Program
- •Examining the framework for all C++ programs
- •Clarifying source code with comments
- •Basing programs on C++ statements
- •Writing declarations
- •Generating output
- •Calculating Expressions
- •Storing the results of expression
- •Declaring Variables
- •Declaring Different Types of Variables
- •Reviewing the limitations of integers in C++
- •Solving the truncation problem
- •Looking at the limits of floating-point numbers
- •Declaring Variable Types
- •Types of constants
- •Special characters
- •Are These Calculations Really Logical?
- •Mixed Mode Expressions
- •Performing Simple Binary Arithmetic
- •Decomposing Expressions
- •Determining the Order of Operations
- •Performing Unary Operations
- •Using Assignment Operators
- •Why Mess with Logical Operations?
- •Using the Simple Logical Operators
- •Storing logical values
- •Using logical int variables
- •Be careful performing logical operations on floating-point variables
- •Expressing Binary Numbers
- •The decimal number system
- •Other number systems
- •The binary number system
- •Performing Bitwise Logical Operations
- •The single bit operators
- •Using the bitwise operators
- •A simple test
- •Do something logical with logical calculations
- •Controlling Program Flow with the Branch Commands
- •Executing Loops in a Program
- •Looping while a condition is true
- •Using the for loop
- •Avoiding the dreaded infinite loop
- •Applying special loop controls
- •Nesting Control Commands
- •Switching to a Different Subject?
- •Writing and Using a Function
- •Divide and conquer
- •Understanding the Details of Functions
- •Understanding simple functions
- •Understanding functions with arguments
- •Overloading Function Names
- •Defining Function Prototypes
- •Variable Storage Types
- •Including Include Files
- •Considering the Need for Arrays
- •Using an array
- •Initializing an array
- •Accessing too far into an array
- •Using arrays
- •Defining and using arrays of arrays
- •Using Arrays of Characters
- •Creating an array of characters
- •Creating a string of characters
- •Manipulating Strings with Character
- •String-ing Along Variables
- •Variable Size
- •Address Operators
- •Using Pointer Variables
- •Comparing pointers and houses
- •Using different types of pointers
- •Passing Pointers to Functions
- •Passing by value
- •Passing pointer values
- •Passing by reference
- •Limiting scope
- •Examining the scope problem
- •Providing a solution using the heap
- •Defining Operations on Pointer Variables
- •Re-examining arrays in light of pointer variables
- •Applying operators to the address of an array
- •Expanding pointer operations to a string
- •Justifying pointer-based string manipulation
- •Applying operators to pointer types other than char
- •Contrasting a pointer with an array
- •Declaring and Using Arrays of Pointers
- •Utilizing arrays of character strings
- •Identifying Types of Errors
- •Choosing the WRITE Technique for the Problem
- •Catching bug #1
- •Catching bug #2
- •Calling for the Debugger
- •Defining the debugger
- •Finding commonalities among us
- •Running a test program
- •Single-stepping through a program
- •Abstracting Microwave Ovens
- •Preparing functional nachos
- •Preparing object-oriented nachos
- •Classifying Microwave Ovens
- •Why Classify?
- •Introducing the Class
- •The Format of a Class
- •Accessing the Members of a Class
- •Activating Our Objects
- •Simulating real-world objects
- •Why bother with member functions?
- •Adding a Member Function
- •Creating a member function
- •Naming class members
- •Calling a Member Function
- •Accessing a member function
- •Accessing other members from a member function
- •Defining a Member Function in the Class
- •Keeping a Member Function After Class
- •Overloading Member Functions
- •Defining Arrays of and Pointers to Simple Things
- •Declaring Arrays of Objects
- •Declaring Pointers to Objects
- •Dereferencing an object pointer
- •Pointing toward arrow pointers
- •Passing Objects to Functions
- •Calling a function with an object value
- •Calling a function with an object pointer
- •Calling a function by using the reference operator
- •Returning to the Heap
- •Comparing Pointers to References
- •Linking Up with Linked Lists
- •Performing other operations on a linked list
- •Hooking up with a LinkedListData program
- •A Ray of Hope: A List of Containers Linked to the C++ Library
- •Protecting Members
- •Why you need protected members
- •Discovering how protected members work
- •Protecting the internal state of the class
- •Using a class with a limited interface
- •Creating Objects
- •Using Constructors
- •Why you need constructors
- •Making constructors work
- •Dissecting a Destructor
- •Why you need the destructor
- •Working with destructors
- •Outfitting Constructors with Arguments
- •Justifying constructors
- •Using a constructor
- •Defaulting Default Constructors
- •Constructing Class Members
- •Constructing a complex data member
- •Constructing a constant data member
- •Constructing the Order of Construction
- •Local objects construct in order
- •Static objects construct only once
- •Global objects construct in no particular order
- •Members construct in the order in which they are declared
- •Destructors destruct in the reverse order of the constructors
- •Copying an Object
- •Why you need the copy constructor
- •Using the copy constructor
- •The Automatic Copy Constructor
- •Creating Shallow Copies versus Deep Copies
- •Avoiding temporaries, permanently
- •Defining a Static Member
- •Why you need static members
- •Using static members
- •Referencing static data members
- •Uses for static data members
- •Declaring Static Member Functions
- •What Is This About, Anyway?
- •Do I Need My Inheritance?
- •How Does a Class Inherit?
- •Using a subclass
- •Constructing a subclass
- •Destructing a subclass
- •Having a HAS_A Relationship
- •Why You Need Polymorphism
- •How Polymorphism Works
- •When Is a Virtual Function Not?
- •Considering Virtual Considerations
- •Factoring
- •Implementing Abstract Classes
- •Describing the abstract class concept
- •Making an honest class out of an abstract class
- •Passing abstract classes
- •Factoring C++ Source Code
- •Defining a namespace
- •Implementing Student
- •Implementing an application
- •Project file
- •Creating a project file under Dev-C++
- •Comparing Operators with Functions
- •Inserting a New Operator
- •Overloading the Assignment Operator
- •Protecting the Escape Hatch
- •How Stream I/O Works
- •The fstream Subclasses
- •Reading Directly from a Stream
- •Using the strstream Subclasses
- •Manipulating Manipulators
- •Justifying a New Error Mechanism?
- •Examining the Exception Mechanism
- •What Kinds of Things Can I Throw?
- •Adding Virtual Inheritance
- •Voicing a Contrary Opinion
- •Generalizing a Function into a Template
- •Template Classes
- •Do I Really Need Template Classes?
- •Tips for Using Templates
- •The string Container
- •The list Containers
- •Iterators
- •Using Maps
- •Enabling All Warnings and Error Messages
- •Insisting on Clean Compiles
- •Limiting the Visibility
- •Avoid Overloading Operators
- •Heap Handling
- •Using Exceptions to Handle Errors
- •Avoiding Multiple Inheritance
- •Customize Editor Settings to Your Taste
- •Highlight Matching Braces/Parentheses
- •Enable Exception Handling
- •Include Debugging Information (Sometimes)
- •Create a Project File
- •Customize the Help Menu
- •Reset Breakpoints after Editing the File
- •Avoid Illegal Filenames
- •Include #include Files in Your Project
- •Executing the Profiler
- •System Requirements
- •Using the CD with Microsoft Windows
- •Using the CD with Linux
- •Development tools
- •Program source code
- •Index
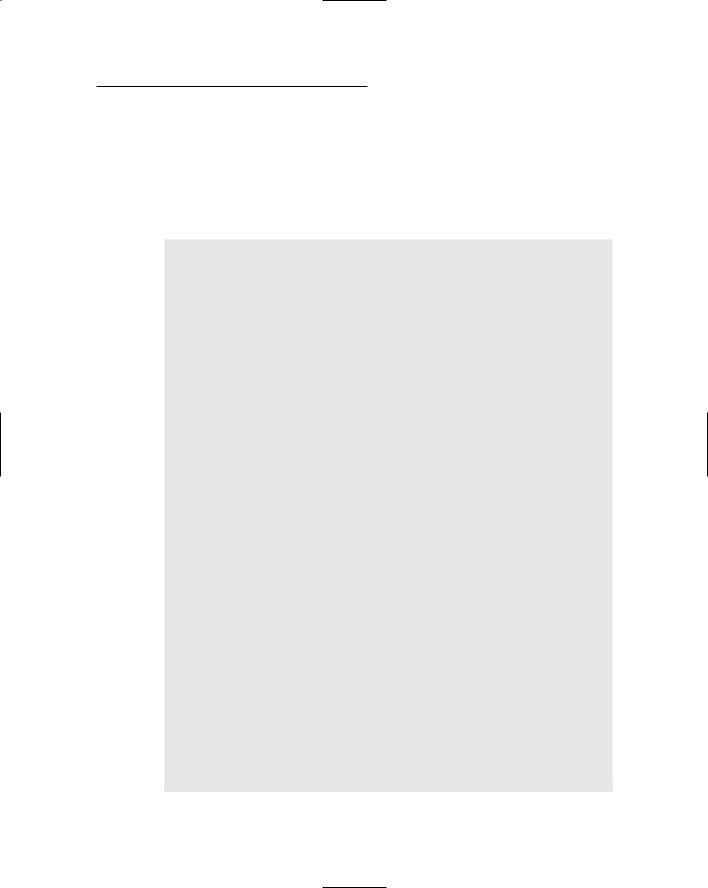
Chapter 14: Point and Stare at Objects 191
Calling a function by using the reference operator
The reference operator described in Chapter 9 works for user-defined objects. The following PassObjRef demonstrates references to user-defined objects:
//PassObjRef - change the contents of an object in
//a function by using a reference #include <cstdio>
#include <cstdlib> #include <iostream> using namespace std;
class Student
{
public:
int semesterHours; float gpa;
};
// same as before, but this time using references void someFn(Student& refS)
{
refS.semesterHours = 10; refS.gpa = 3.0;
cout << “The value of refS.gpa = “ << refS.gpa << “\n”;
}
int main(int nNumberofArgs, char* pszArgs[])
{
Student s; s.gpa = 0.0;
//display the value of s.gpa before calling someFn() cout << “The value of s.gpa = “ << s.gpa << “\n”;
//pass the address of the existing object
cout << “Calling someFn(Student*)\n”; someFn(s);
cout << “Returned from someFn(Student&)\n”;
// the value of s.gpa is now 3.0
cout << “The value of s.gpa = “ << s.gpa << “\n”;
//wait until user is ready before terminating program
//to allow the user to see the program results
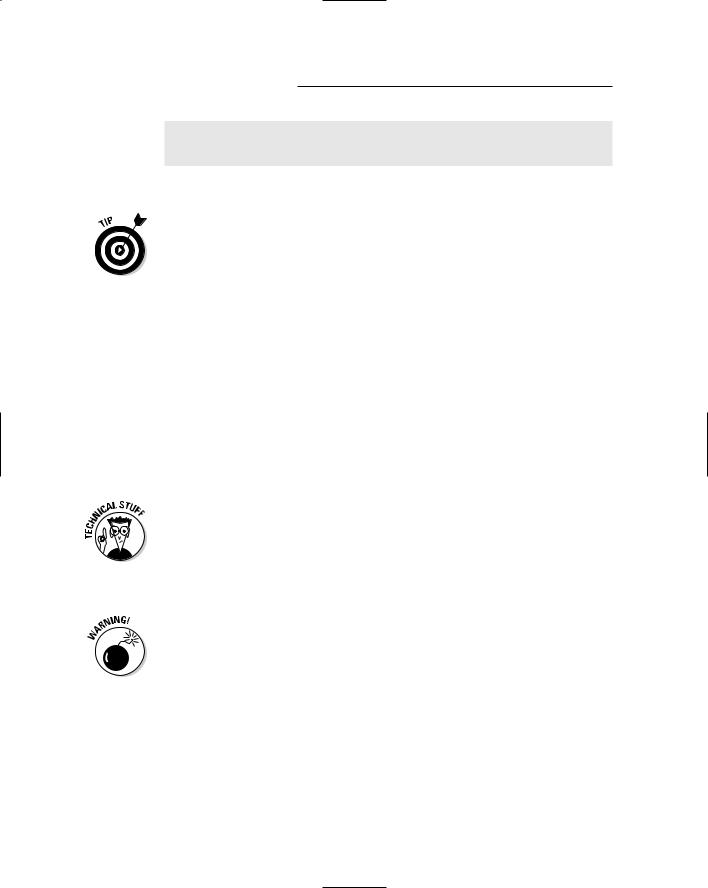
192 Part III: Introduction to Classes
system(“PAUSE”); return 0;
}
In this example, C++ passes a reference to s rather than a copy. Changes made in someFn() are retained in main().
Passing by reference is just another way of passing the address of the object. C++ keeps track of the address of a reference, whereas you manipulate the address in a pointer.
Why Bother with Either
Pointers or References?
Okay, so both pointers and references provide relative advantages, but why bother with either one? Why not just always pass the object?
I discussed one obvious answer earlier in this chapter: You can’t modify the object from a function that gets nothing but a copy of the structure object.
Here’s a second reason: Some objects are large — I mean really large. Passing such an object by value means copying the entire thing into the function’s memory.
The area used to pass arguments to a function is called the call stack.
The object will need to be copied again should that function call another, and so on. After a while, you can end up with dozens of copies of this object. That consumes memory, and copying all the objects can make execution of your program slower than booting up Windows.
The problem of copying objects actually gets worse. You see in Chapter 18 that making a copy of an object can be even more painful than simply copy ing some memory around.
Returning to the Heap
The problems that exist for simple types of pointers plague class object pointers as well. In particular, you must make sure that the pointer you’re using actually points to a valid object. For example, don’t return a reference to an object defined local to the function:

Chapter 14: Point and Stare at Objects 193
MyClass* myFunc()
{
// the following does not work MyClass mc;
MyClass* pMC = &mc; return pMC;
}
Upon return from myFunc(), the mc object goes out of scope. The pointer returned by myFunc() is not valid in the calling function.
The problem of returning memory that’s about to go out of scope is dis cussed in Chapter 9.
Allocating the object off the heap solves the problem:
MyClass* myFunc()
{
MyClass* pMC = new MyClass; return pMC;
}
The heap is used to allocate objects in a number of different situations.
Comparing Pointers to References
I hate to keep referencing pointers and pointing to references, but new pro grammers often wonder why both are needed.
Actually, you could argue that you don’t need both. C# and most other lan guages don’t use pointers. However, pointer variables are an ingrained part of good ol’ standard non-Visual Studio.NET–specific C++.
Why Not Use References
Rather Than Pointers?
The syntax for manipulating a reference is similar to that used with normal objects. So why not just stick with references and never look back at pointers?
Objects and their addresses aren’t the same thing. Many times, the syntax for a reference actually becomes more complicated than that for pointers. Consider the following examples:

194 Part III: Introduction to Classes
class Student
{
public:
int semesterHours; float gpa;
Student valFriend; Student& refFriend; Student* ptrFriend;
};
int main(int nNumberofArgs, char* pszArgs[])
{
//the following declares a reference off of the heap
//(simple enough)
Student& student = *new Student; student.gpa = 10;
// ditto
Student& studentFriend = *new Student; studentFriend.gpa = 20;
//the following copies the value of one Student
//object into the second
student.valFriend = studentFriend;
//this doesn’t work at all Student& refFriend; refFriend = studentFriend;
//this does work student.pFriend = &studentFriend;
return 0;
}
As you can see, I modified that Student class so that one Student can refer ence her best buddy. I tried to use the reference variable type to do so. I cre ated two students in main() in an attempt to link the one student object to its studentFriend.
The first assignment in the body of the program copies the contents of the friend into the data member — Student object contains a body double. The second assignment doesn’t work at all — C++ can’t differentiate assigning an object to a reference variable from assignment to an object itself. Only the third assignment works. The student object points to the address of the studentFriend, which is exactly what you want.